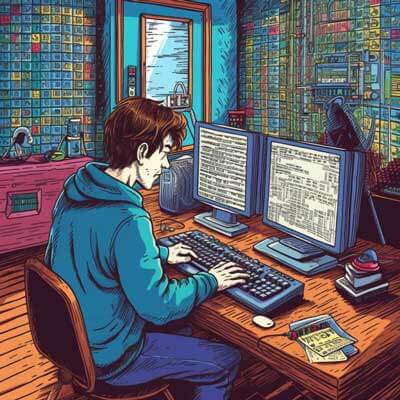
- Introduction to Typing Module
- Typing Module: Installation and Setup
- Example: Installing Python 3.9
- Anatomy of Typing Module
- Example: Using List and Tuple
- Various Annotations in Typing Module
- Example: Using Dict and Optional
- How to Use Annotations
- Example: Annotating Function Parameters and Return Types
- Application: Annotations in Function Definitions
- Example: Function with Annotations
- Application: Annotations in Variable Assignments
- Example: Variable Assignment with Annotation
- Best Practices: Typing Module Use Cases
- Code Snippet: Function Annotations
- Code Snippet: Variable Annotations
- Code Snippet: Class Annotations
- Advanced Techniques: Using Optional
- Example: Using Optional
- Advanced Techniques: Using Union
- Example: Using Union
- Advanced Techniques: Using Any
- Example: Using Any
- Advanced Techniques: Using List, Tuple, Dict
- Example: Using List, Tuple, Dict
- Performance Considerations: Typing Module Overhead
- Performance Considerations: Runtime vs Static Typing
- Error Handling with Typing Module
- Real World Example: Typing in Large Codebase
- Real World Example: Typing in Data Science Project
- Real World Example: Typing in Web Application
Introduction to Typing Module
The Typing module in Python is a powerful tool that allows developers to add type hints to their code, enabling static type checking and improving code readability. With the introduction of type hints in Python 3.5, the Typing module provides a standardized way to express types and annotations within the language.
Related Article: How To Limit Floats To Two Decimal Points In Python
Typing Module: Installation and Setup
To start using the Typing module, you need to have Python 3.5 or later installed on your system. The Typing module is included in the standard library, so there is no need to install any additional packages.
Example: Installing Python 3.9
To install Python 3.9 on a Linux machine, you can use the following commands:
$ sudo apt update $ sudo apt install python3.9
Anatomy of Typing Module
The Typing module provides a variety of classes, functions, and types that can be used to annotate variables, function parameters, and return types. Some of the key components of the Typing module include:
– Any
: Represents a dynamically typed value.
– List
: Represents a list of elements of a specific type.
– Tuple
: Represents a tuple of elements of specific types.
– Dict
: Represents a dictionary with keys and values of specific types.
– Union
: Represents a type that can be one of several possible types.
– Optional
: Represents a type that can be either the specified type or None
.
– Callable
: Represents a function or method signature.
– TypeVar
: Represents a generic type variable.
Related Article: How To Rename A File With Python
Example: Using List and Tuple
from typing import List, Tuple def process_data(data: List[str]) -> Tuple[str, int]: # Process the data return data[0], len(data) result = process_data(["apple", "banana", "cherry"]) print(result) # Output: ("apple", 3)
Various Annotations in Typing Module
The Typing module provides a wide range of annotations to express different types in Python. Some of the commonly used annotations include:
– List[int]
: Represents a list of integers.
– Tuple[str, int]
: Represents a tuple with a string and an integer.
– Dict[str, int]
: Represents a dictionary with string keys and integer values.
– Optional[int]
: Represents an optional integer value (can be None
).
– Union[str, int]
: Represents a type that can be either a string or an integer.
Example: Using Dict and Optional
from typing import Dict, Optional def format_person_details(name: str, age: Optional[int] = None) -> Dict[str, str]: details = {"name": name} if age is not None: details["age"] = str(age) return details person1 = format_person_details("Alice", 25) person2 = format_person_details("Bob") print(person1) # Output: {"name": "Alice", "age": "25"} print(person2) # Output: {"name": "Bob"}
Related Article: How To Check If List Is Empty In Python
How to Use Annotations
To use annotations in your code, you simply need to add them as type hints to variables, function parameters, and return types. Python’s dynamic nature allows you to use type hints without enforcing them, but you can use static type checkers like Mypy to analyze your code and catch potential type-related errors.
Example: Annotating Function Parameters and Return Types
def calculate_total(items: List[float]) -> float: total = sum(items) return total prices = [9.99, 4.99, 2.99] total_price = calculate_total(prices) print(total_price) # Output: 17.97
Application: Annotations in Function Definitions
Annotations in function definitions can be used to specify the types of parameters and the return type of the function. This helps in documenting the expected input and output of the function and can be useful for understanding the purpose of the function.
Related Article: How To Check If a File Exists In Python
Example: Function with Annotations
def greet(name: str) -> str: return f"Hello, {name}!" message = greet("Alice") print(message) # Output: "Hello, Alice!"
Application: Annotations in Variable Assignments
Annotations can also be used to specify the type of a variable. This can provide additional information about the expected type of the variable and help in catching potential type-related errors.
Example: Variable Assignment with Annotation
count: int = 10 print(count) # Output: 10 total: float = 5.99 print(total) # Output: 5.99
Related Article: How to Use Inline If Statements for Print in Python
Best Practices: Typing Module Use Cases
The Typing module offers great flexibility in expressing types and annotations in Python. Here are some best practices to consider when using the Typing module:
1. Use type hints consistently throughout your codebase to improve code readability and maintainability.
2. Use specific types from the Typing module, such as List
, Tuple
, and Dict
, instead of generic types like list
and dict
.
3. Use Union types when a variable can have multiple possible types.
4. Use Optional types when a variable can be None
.
5. Avoid using Any
unless absolutely necessary, as it bypasses type checking.
6. Use type checkers like Mypy to catch potential type-related errors in your code.
Code Snippet: Function Annotations
def calculate_total(items: List[float]) -> float: total = sum(items) return total
Code Snippet: Variable Annotations
count: int = 10 total: float = 5.99
Related Article: How to Use Stripchar on a String in Python
Code Snippet: Class Annotations
class Person: name: str age: int def __init__(self, name: str, age: int): self.name = name self.age = age
Advanced Techniques: Using Optional
The Optional
type from the Typing module allows you to specify that a variable can have a specific type or can be None
. This can be useful when a value is optional or can be missing.
Example: Using Optional
from typing import Optional def find_user(username: str) -> Optional[str]: # Code to find user in database if user_found: return user_id else: return None result = find_user("alice") if result is not None: print(f"User found with ID: {result}") else: print("User not found")
Related Article: How To Delete A File Or Folder In Python
Advanced Techniques: Using Union
The Union
type from the Typing module allows you to specify that a variable can have one of several possible types. This can be useful when a variable can hold different types of values.
Example: Using Union
from typing import Union def process_data(data: Union[str, int]) -> str: if isinstance(data, str): return data.upper() elif isinstance(data, int): return str(data) else: raise ValueError("Unsupported data type") result1 = process_data("hello") result2 = process_data(42) print(result1) # Output: "HELLO" print(result2) # Output: "42"
Advanced Techniques: Using Any
The Any
type from the Typing module represents a dynamically typed value, similar to how variables are used in Python without type annotations. While the Any
type can be useful in certain scenarios, it should be used sparingly, as it bypasses static type checking.
Related Article: How To Move A File In Python
Example: Using Any
from typing import Any def process_data(data: Any) -> str: # Code to process data return str(data) result = process_data(42) print(result) # Output: "42"
Advanced Techniques: Using List, Tuple, Dict
The Typing module provides specific types for lists, tuples, and dictionaries. These types allow you to specify the expected types of the elements in a list or tuple, or the types of keys and values in a dictionary.
Example: Using List, Tuple, Dict
from typing import List, Tuple, Dict def process_data(data: List[Tuple[str, int]]) -> Dict[str, int]: result = {} for item in data: key, value = item result[key] = value return result input_data = [("apple", 10), ("banana", 5), ("cherry", 3)] output = process_data(input_data) print(output) # Output: {"apple": 10, "banana": 5, "cherry": 3}
Related Article: How to Implement a Python Foreach Equivalent
Performance Considerations: Typing Module Overhead
Adding type hints to your code using the Typing module has a minimal impact on performance. The type hints themselves are not executed at runtime and do not affect the speed of your code. However, if you use static type checkers like Mypy, they can introduce some overhead during the type checking phase.
Performance Considerations: Runtime vs Static Typing
Python is a dynamically typed language, which means that type checking is performed at runtime. However, with the introduction of type hints and static type checkers like Mypy, you can perform type checking statically, before the code is executed. Static type checking can catch potential type-related errors early on and improve code quality, but it comes with the trade-off of increased development time and potential overhead during the type checking phase.
Error Handling with Typing Module
When using the Typing module, you can specify the expected types of parameters and return values. This can help catch type-related errors early on and provide better error messages. However, the Typing module does not enforce type checking at runtime. To catch type-related errors, you can use static type checkers like Mypy.
Related Article: How to Use Slicing in Python And Extract a Portion of a List
Real World Example: Typing in Large Codebase
In a large codebase, using the Typing module can greatly improve code maintainability and readability. By adding type hints to function parameters, return types, and variables, you provide additional information to other developers and tools that can help in understanding the codebase and catching potential errors.
Real World Example: Typing in Data Science Project
In data science projects, where data manipulation and analysis are crucial, using the Typing module can help in documenting the expected types of input and output data. This can make the code more self-explanatory and help in catching potential type-related errors.
Real World Example: Typing in Web Application
In web applications, where multiple components interact with each other, using the Typing module can improve code maintainability and catch potential type-related errors early on. By annotating function parameters, return types, and variables, you provide a clear specification of the expected types, making it easier to understand and modify the code.