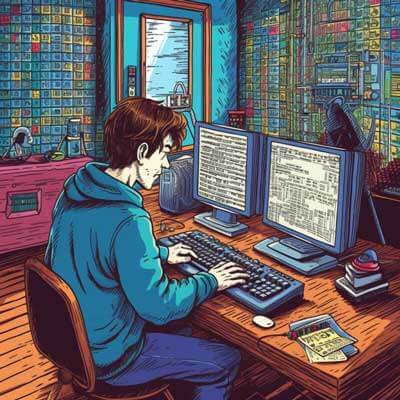
Table of Contents
Copying files is a common task in software development and data processing. In Python, there are several ways to copy files, depending on the requirements and the level of control needed. This answer will cover different methods to copy files in Python, along with their advantages and use cases.
Using shutil Module
The shutil
module in Python provides a high-level interface for copying files and directories. It offers functions that handle various file operations, including file copying. The shutil.copy()
function is used to copy a single file, while the shutil.copytree()
function is used to copy directories recursively.
Here is an example of using shutil.copy()
to copy a file:
import shutil # Source file path src_file = '/path/to/source/file.txt' # Destination file path dst_file = '/path/to/destination/file.txt' # Copy the file shutil.copy(src_file, dst_file)
In the above example, the shutil.copy()
function is used to copy the file from the source path to the destination path. The function takes two arguments: the source file path and the destination file path. If the destination file already exists, it will be overwritten.
To copy directories recursively, you can use the shutil.copytree()
function:
import shutil # Source directory path src_dir = '/path/to/source/directory' # Destination directory path dst_dir = '/path/to/destination/directory' # Copy the directory recursively shutil.copytree(src_dir, dst_dir)
The shutil.copytree()
function copies the entire directory tree from the source directory to the destination directory. If the destination directory already exists, an error will be raised.
Using the shutil
module provides a simple and convenient way to copy files and directories in Python. It handles all the necessary file operations, such as creating directories and handling file permissions.
Related Article: How To Convert a Python Dict To a Dataframe
Using os Module
Another way to copy files in Python is by using the os
module. The os
module provides low-level operating system interfaces and can be used to perform various file operations, including file copying.
To copy a file using the os
module, you can use the os.path
functions to manipulate file paths and the os
functions to perform file operations. Here is an example:
import os # Source file path src_file = '/path/to/source/file.txt' # Destination file path dst_file = '/path/to/destination/file.txt' # Copy the file os.makedirs(os.path.dirname(dst_file), exist_ok=True) shutil.copy2(src_file, dst_file)
In the above example, the os.makedirs()
function is used to create the destination directory if it doesn't exist. The os.path.dirname()
function is used to extract the directory path from the destination file path.
The shutil.copy2()
function is used to copy the file while preserving the original file attributes, such as timestamps and permissions.
Using the os
module gives you more control over the file copying process, but it also requires more manual handling of file operations.
Why is the question asked?
The question "How to copy files in Python" is commonly asked by developers who need to perform file operations as part of their Python programs. Copying files is a fundamental task in many applications, such as backup systems, data processing pipelines, and file synchronization tools.
Potential reasons for asking this question include:
1. Needing to create a backup or duplicate of a file for archival purposes.
2. Moving files to a different location or directory.
3. Implementing a file synchronization mechanism.
4. Performing data processing on files without modifying the original files.
Suggestions and Alternative Ideas
When copying files in Python, consider the following suggestions and alternative ideas:
1. Check if the source and destination files exist before copying. You can use the os.path.exists()
function to check if a file or directory exists.
2. Handle errors and exceptions. When copying files, there might be situations where the operation fails due to file permissions, disk space limitations, or other issues. Wrap the file copying code in a try-except block to handle any potential errors gracefully.
3. Use file compression libraries. If you need to copy large files or directories, consider using file compression libraries, such as zipfile
or tarfile
, to compress the files before copying. This can reduce the overall file size and speed up the copying process.
Related Article: How to Change Column Type in Pandas
Best Practices
When copying files in Python, it is recommended to follow these best practices:
1. Use the appropriate file copying method based on your requirements. If you need a high-level interface with simplified file operations, use the shutil
module. If you require more control over file operations, use the os
module.
2. Handle file permissions. When copying files, ensure that the destination file retains the correct permissions. The shutil.copy()
function preserves the permissions by default, but if you are using other methods, make sure to set the correct permissions on the destination file.
3. Check for disk space. Before copying large files or directories, check if there is enough disk space available on the destination drive. This can help prevent errors and ensure a successful file copying process.
4. Test with sample files and directories. Before implementing file copying in a production environment, test the code with sample files and directories to ensure it performs as expected. This can help identify any potential issues or performance bottlenecks.
5. Consider using libraries for advanced file operations. If you need to perform more complex file operations, such as file syncing or differential copying, consider using third-party libraries that provide advanced functionality. Examples of such libraries include rsync
for efficient syncing and diff-match-patch
for generating file diffs.