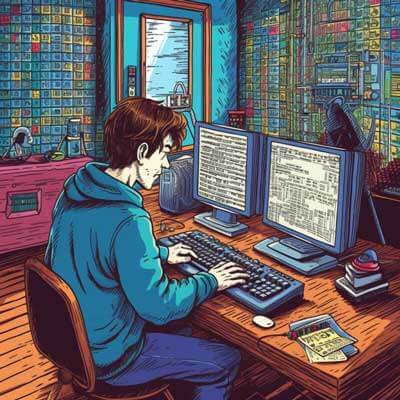
Table of Contents
Introduction to Secure File Transfer Protocol (SFTP)
SFTP stands for Secure File Transfer Protocol, and it is a secure alternative to the more traditional FTP protocol. SFTP allows for encrypted file transfers between a client and a remote server, ensuring the confidentiality and integrity of the transferred data.
To establish an SFTP connection, you will need an SFTP client and an SFTP server. The client is responsible for initiating the connection and requesting file transfers, while the server handles authentication and file access.
Related Article: Displaying Memory Usage in Bash Scripts on Linux
Code Snippet: Establishing SFTP Connection
sftp username@hostname
The above command establishes an SFTP connection to the specified hostname using the provided username. Upon successful connection, you will be prompted for the password associated with the username.
Code Snippet: Transferring Files Using SFTP
To transfer files using SFTP, you can use the put
command to upload files from your local machine to the remote server, or the get
command to download files from the remote server to your local machine.
put local_file [remote_path]
The put
command transfers the specified local file to the remote server. If a remote path is provided, the file will be placed in the specified directory. Otherwise, it will be placed in the current working directory on the remote server.
get remote_file [local_path]
The get
command downloads the specified remote file to your local machine. If a local path is provided, the file will be saved in the specified directory. Otherwise, it will be saved in the current working directory on your local machine.
Installing and Configuring SFTP
Before you can start using SFTP, you need to install and configure an SFTP server on your remote Linux machine. This section will guide you through the installation and configuration process.
Related Article: How to Continue a Bash Script Post cURL Command
Setting Up User Authentication for SFTP
To enable SFTP access for a specific user, you need to configure their authentication method. One common approach is to use password-based authentication, which requires users to enter their password when connecting via SFTP.
Code Snippet: Creating Directories with SFTP
To create a directory using SFTP, you can use the mkdir
command followed by the directory name.
mkdir directory_name
The above command creates a directory with the specified name in the current working directory on the remote server.
Code Snippet: Renaming Files with SFTP
To rename a file using SFTP, you can use the rename
command followed by the current file name and the desired new file name.
rename current_file_name new_file_name
The above command renames the file with the current name to the new specified name in the current working directory on the remote server.
Code Snippet: Deleting Files with SFTP
To delete a file using SFTP, you can use the rm
command followed by the file name.
rm file_name
The above command deletes the file with the specified name from the current working directory on the remote server.
Related Article: Exploring Local Scope of Bash Variables in Linux Scripts
Managing Files and Directories with SFTP
SFTP provides various commands to manage files and directories on the remote server. In this section, we will explore some of the most commonly used commands.
Code Snippet: Listing Files and Directories with SFTP
To list the contents of a directory using SFTP, you can use the ls
command.
ls
The above command lists the files and directories in the current working directory on the remote server.
Code Snippet: Changing to a Different Directory with SFTP
To change to a different directory using SFTP, you can use the cd
command followed by the directory path.
cd directory_path
The above command changes the current working directory to the specified directory path on the remote server.
Use Case: Secure Backup with SFTP
SFTP can be an excellent solution for securely backing up your important files to a remote server. By leveraging the encryption and authentication features of SFTP, you can ensure the confidentiality and integrity of your backup data.
Related Article: Indentation Importance in Bash Scripts on Linux
Code Snippet: Automated Backup with SFTP
To automate the backup process using SFTP, you can create a script that connects to the remote server and transfers the desired files.
#!/bin/bashsftp username@hostname <<EOFput local_file remote_pathquitEOF
The above script establishes an SFTP connection to the specified hostname using the provided username, transfers the specified file from the local machine to the remote server, and then terminates the connection.
Code Snippet: Scheduled Backup with Cron
To schedule the backup script to run at regular intervals, you can use the cron utility in Linux.
# Edit the crontab filecrontab -e# Add the following line to run the backup script daily at 2 AM0 2 * * * /path/to/backup_script.sh
The above cron configuration executes the backup script daily at 2 AM. You can adjust the timing according to your requirements.
Best Practice: Using Key-Based Authentication
One of the best practices for securing SFTP connections is to use key-based authentication instead of password-based authentication. Key-based authentication provides a more secure and convenient way to authenticate to the remote server.
Code Snippet: Generating SSH Key Pair
To generate an SSH key pair for key-based authentication, you can use the ssh-keygen
command.
ssh-keygen -t rsa -b 4096 -C "your_email@example.com"
The above command generates an RSA key pair with a key size of 4096 bits and associates it with the provided email address.
Related Article: Integrating a Bash Script into a Makefile in Linux
Code Snippet: Configuring SFTP Server for Key-Based Authentication
To configure the SFTP server to accept key-based authentication, you need to add the public key to the authorized keys file.
# Append the public key to the authorized keys filecat public_key.pub >> ~/.ssh/authorized_keys
The above command appends the contents of the public key file to the authorized keys file in the user's home directory.
Best Practice: Limiting SFTP Access to Specific Directories
To enhance the security of your SFTP server, it is recommended to restrict user access to specific directories. This prevents users from accessing files and directories outside their designated areas.
Code Snippet: Configuring Chroot Jail for SFTP Users
To configure a chroot jail for SFTP users, you need to modify the SSH server configuration file.
# Edit the SSH server configuration filesudo nano /etc/ssh/sshd_config# Add the following line at the end of the fileMatch User username ChrootDirectory /path/to/chroot_directory ForceCommand internal-sftp
The above configuration restricts the specified user to the specified chroot directory and forces the use of the internal SFTP server.
Best Practice: Monitoring and Logging SFTP Activity
Monitoring and logging SFTP activity can help identify potential security issues and track file transfer activities. By analyzing the logs, you can gain insights into the usage patterns and take appropriate actions if any suspicious activities are detected.
Related Article: How to Choose the Preferred Bash Shebang in Linux
Code Snippet: Configuring SFTP Logging
To enable SFTP logging, you need to modify the SSH server configuration file.
# Edit the SSH server configuration filesudo nano /etc/ssh/sshd_config# Uncomment the following line (if commented)# Subsystem sftp /usr/lib/openssh/sftp-server# Add the following line at the end of the fileSubsystem sftp /usr/lib/openssh/sftp-server -l INFO
The above configuration enables SFTP logging with the INFO log level, which provides detailed information about the SFTP session.
Real World Example: SFTP for Large Scale Data Transfer
SFTP can be an efficient solution for transferring large volumes of data between systems. Its secure and reliable nature makes it suitable for scenarios where data integrity and confidentiality are of utmost importance.
Code Snippet: Parallel File Transfers with SFTP
To improve the performance of large-scale data transfers, you can leverage parallel file transfers with SFTP. The following script demonstrates how to transfer multiple files in parallel using SFTP.
#!/bin/bash# List of files to transferfile_list=( file1.txt file2.txt file3.txt)# Number of parallel transfersparallel_count=3# Start parallel transfersfor file in "${file_list[@]}"; do ( sftp username@hostname <<EOFput $filequitEOF ) & ((parallel_count--)) if ((parallel_count == 0)); then wait parallel_count=3 fidonewait
The above script transfers multiple files in parallel using SFTP. The number of parallel transfers is controlled by the parallel_count
variable.
Real World Example: SFTP for Secure Remote Backup
SFTP can be an excellent choice for securely backing up your important data to a remote server. By leveraging the encryption and authentication features of SFTP, you can ensure the confidentiality and integrity of your backup data.
Related Article: How To Recursively Grep Directories And Subdirectories
Code Snippet: Encrypted Remote Backup with SFTP
To perform an encrypted remote backup using SFTP, you can use the tar
command to create a compressed archive of the files you want to back up and then transfer the archive to the remote server using SFTP.
# Create a compressed archive of the filestar -czvf backup.tar.gz /path/to/files# Transfer the archive to the remote server using SFTPsftp username@hostname <<EOFput backup.tar.gzquitEOF
The above script creates a compressed archive of the specified files using the tar
command and then transfers the archive to the remote server using SFTP.
Performance Consideration: Transfer Speeds
When using SFTP for file transfers, the transfer speeds can have a significant impact on the overall performance. Several factors can affect the transfer speeds, including network conditions, server resources, and encryption overhead.
Code Snippet: Monitoring Transfer Speeds with SFTP
To monitor the transfer speeds during an SFTP file transfer, you can use the progress
option with the get
or put
command.
put -P local_file [remote_path]
The above command transfers the specified local file to the remote server while displaying the progress and transfer speeds.
Performance Consideration: CPU Usage
During SFTP file transfers, the CPU usage on both the client and server machines can increase, especially when encryption is enabled. Higher CPU usage can impact the performance of other processes running on the machines.
Related Article: How To Find Files Based On Wildcard In Linux
Code Snippet: Limiting Encryption Strength with SFTP
To reduce the CPU usage during SFTP file transfers, you can limit the encryption strength by modifying the SSH server configuration file.
# Edit the SSH server configuration filesudo nano /etc/ssh/sshd_config# Add the following line at the end of the fileCiphers aes128-ctr,aes192-ctr,aes256-ctr
The above configuration limits the encryption strength to AES with 128-bit, 192-bit, and 256-bit key lengths, which can help reduce CPU usage.
Performance Consideration: Network Bandwidth
The available network bandwidth can significantly impact the transfer speeds during SFTP file transfers. Insufficient bandwidth can lead to slower transfers and increased transfer times.
Code Snippet: Monitoring Network Bandwidth with SFTP
To monitor the network bandwidth utilization during an SFTP file transfer, you can use network monitoring tools such as iftop
or nload
on Linux.
# Install the network monitoring tool (example using iftop)sudo apt-get install iftop# Start monitoring network bandwidthiftop
The above commands install the iftop
network monitoring tool and start monitoring the network bandwidth utilization in real-time.
Advanced Technique: Scripting Automated File Transfers
To automate file transfers with SFTP, you can create scripts that perform specific file transfer tasks. These scripts can be scheduled to run at regular intervals using cron or triggered by specific events.
Related Article: How to Use Getopts in Bash: A Practical Example
Code Snippet: Scripting Automated File Transfer with SFTP
#!/bin/bash# List of files to transferfile_list=( file1.txt file2.txt file3.txt)# Start file transfersfor file in "${file_list[@]}"; do sftp username@hostname <<EOFput $filequitEOFdone
The above script transfers multiple files to the remote server using SFTP. You can modify the file_list
array to specify the files you want to transfer.
Advanced Technique: Integrating SFTP with Other Software
SFTP can be integrated with other software or scripting languages to automate complex workflows or incorporate SFTP functionality into existing systems.
Code Snippet: Uploading Files to SFTP Server Using Python
import pysftp# Connect to the SFTP serverwith pysftp.Connection('hostname', username='username', password='password') as sftp: # Upload a file to the remote server sftp.put('local_file', 'remote_path') # List files on the remote server sftp.listdir()
The above Python code demonstrates how to use the pysftp
library to connect to an SFTP server, upload a file, and list files on the remote server.
Error Handling: Troubleshooting Connection Issues
When working with SFTP, you may encounter connection issues that prevent you from establishing a successful connection to the remote server. Troubleshooting these issues requires identifying the root cause and applying the appropriate solutions.
Related Article: Executing Bash Scripts Without Permissions in Linux
Code Snippet: Troubleshooting Connection Issues
sftp -v username@hostname
The above command initiates an SFTP connection in verbose mode, providing detailed information about the connection process. This can help identify potential issues and guide you in troubleshooting them.
Error Handling: Resolving Authentication Problems
Authentication problems can occur when attempting to establish an SFTP connection. These problems can be caused by incorrect credentials, misconfigured authentication methods, or other factors.
Code Snippet: Resolving Authentication Problems
# Check the SSH server logssudo tail -f /var/log/auth.log# Verify the authentication configurationsudo nano /etc/ssh/sshd_config
The above commands allow you to check the SSH server logs for authentication-related messages and verify the authentication configuration in the SSH server configuration file.
Error Handling: Dealing with File Transfer Errors
During file transfers with SFTP, errors can occur due to various factors, such as network interruptions, insufficient disk space, or file permission issues. Handling these errors effectively can help ensure successful file transfers.
Related Article: How to Format Numbers to Two Decimal Places in Bash
Code Snippet: Retry File Transfers on Error
To automatically retry file transfers in case of errors, you can use a loop structure in your script to retry the transfer until it succeeds or reaches a maximum number of attempts.
#!/bin/bashmax_attempts=3attempts=0success=falsewhile [ "$attempts" -lt "$max_attempts" ] && [ "$success" != true ]; do sftp username@hostname <<EOFput local_file remote_pathquitEOF if [ $? -eq 0 ]; then success=true else attempts=$((attempts + 1)) sleep 5 fidone
The above script attempts to transfer the specified file using SFTP, retrying the transfer up to a maximum number of attempts if an error occurs.
This concludes the comprehensive tutorial on using SFTP for secure file transfers on Linux. By following the provided instructions and utilizing the code snippets, you can confidently set up, configure, and troubleshoot SFTP connections, as well as automate file transfers and implement best practices for enhanced security and performance.