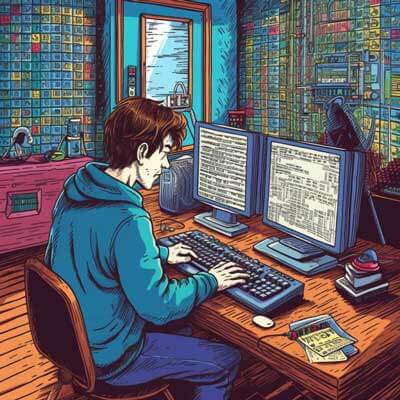
- Benefits of Using Bash Scripting
- Automation with Bash Scripting
- Commonly Used Bash Commands
- Executing Bash Scripts
- Working with Variables in Bash Scripts
- The Role of the Shell Environment in Bash Scripting
- Command Substitution in Bash Scripting
- Best Practices for Writing Bash Scripts
- Additional Resources
Bash scripting is a useful tool for automating tasks and managing Linux systems. It provides a simple and efficient way to write scripts that can execute commands, manipulate files, and perform various operations. In this article, we will explore the benefits of using bash scripting in Linux and how it can improve efficiency and productivity for system administrators and developers.
Benefits of Using Bash Scripting
Bash scripting offers several benefits for Linux users, including:
1. Automation: One of the key advantages of bash scripting is the ability to automate repetitive tasks. By writing a script, you can save time and effort by automating tasks such as system backups, log file analysis, and software installations. This can greatly improve efficiency and productivity, especially in environments where multiple tasks need to be performed regularly.
2. Flexibility: Bash scripting provides a high level of flexibility, allowing you to write scripts that can handle a wide range of tasks. Whether you need to process text files, manipulate data, or interact with system resources, bash scripting provides a versatile and useful toolset. This flexibility makes it suitable for a variety of use cases, from system administration to software development.
3. Customization: Bash scripting allows you to create customized solutions tailored to your specific needs. You can write scripts that automate complex workflows, integrate with other tools and services, and provide a personalized user experience. This level of customization can greatly enhance your productivity and make your Linux environment more efficient.
Related Article: How To Echo a Newline In Bash
Automation with Bash Scripting
Bash scripting excels at automating tasks in Linux. Let’s take a look at a couple of examples to illustrate its capabilities.
Example 1: Backing up files
#!/bin/bash # Define the source and destination directories source_dir="/path/to/source" destination_dir="/path/to/backup" # Create a timestamp for the backup timestamp=$(date +%Y%m%d%H%M%S) # Create a new directory for the backup backup_dir="${destination_dir}/backup_${timestamp}" mkdir "${backup_dir}" # Copy files from the source directory to the backup directory cp -r "${source_dir}" "${backup_dir}"
In this example, we create a simple bash script that backs up files from a source directory to a destination directory. The script uses variables to define the directories and creates a timestamp to create a unique backup directory. It then uses the cp
command to copy the files from the source directory to the backup directory.
Example 2: Installing software packages
#!/bin/bash # List of software packages to install packages=("package1" "package2" "package3") # Loop through the packages and install them for package in "${packages[@]}" do echo "Installing ${package}..." apt-get install -y "${package}" done
In this example, we create a bash script that installs multiple software packages using the apt-get
command. We define an array of package names and then loop through the array to install each package. The script provides feedback by echoing the name of each package being installed.
These examples demonstrate how bash scripting can automate tasks such as file backups and software installations, saving time and effort for Linux users.
Commonly Used Bash Commands
Bash scripting relies on a set of commonly used commands that allow you to interact with the Linux system. Here are a few examples:
– echo
: This command is used to print text or variables to the console. It is often used for debugging and providing feedback in scripts.
– if
: This command is used for conditional execution. It allows you to perform different actions based on the result of a condition.
– for
: This command is used for looping through a list of items. It allows you to perform a set of actions for each item in the list.
– while
: This command is used for creating loops that continue until a certain condition is met. It allows you to repeat a set of actions until a specific condition is satisfied.
These are just a few examples of the many commands available in bash scripting. Understanding and mastering these commands is essential for effective bash scripting.
Executing Bash Scripts
Executing bash scripts is straightforward. Once you have written your script, you need to make it executable using the chmod
command. For example, to make a script named backup.sh
executable, you would run the following command:
chmod +x backup.sh
Once the script is executable, you can run it by simply typing its name in the terminal:
./backup.sh
This will execute the script and perform the actions defined within it.
Related Article: How to Use If-Else Statements in Shell Scripts
Working with Variables in Bash Scripts
Variables are a fundamental component of bash scripting. They allow you to store and manipulate data within your scripts. Let’s take a look at a couple of examples.
Example 1: Using a variable to store a string
#!/bin/bash # Define a variable name="John Doe" # Print the value of the variable echo "Hello, ${name}"
In this example, we define a variable named name
and assign it the value “John Doe”. We then use the echo
command to print a greeting that includes the value of the variable.
Example 2: Using a variable in a calculation
#!/bin/bash # Define variables length=10 width=5 # Calculate the area area=$((length * width)) # Print the result echo "The area is ${area}"
In this example, we define variables for the length and width of a rectangle. We then calculate the area by multiplying the length and width variables together. Finally, we use the echo
command to print the result.
Variables in bash scripts can be used for a wide range of purposes, from storing user input to performing calculations and manipulating data.
The Role of the Shell Environment in Bash Scripting
The shell environment plays a crucial role in bash scripting. It provides a set of predefined variables and functions that can be accessed and manipulated within your scripts. Let’s take a look at a couple of examples.
Example 1: Accessing environment variables
#!/bin/bash # Access the value of the HOME variable echo "Home directory: ${HOME}" # Access the value of the PATH variable echo "Search path: ${PATH}"
In this example, we access the values of the HOME
and PATH
environment variables using the syntax ${VARIABLE_NAME}
. We then use the echo
command to print the values.
Example 2: Modifying the environment
#!/bin/bash # Add a directory to the PATH variable export PATH="${PATH}:/path/to/directory" # Print the modified PATH variable echo "Modified search path: ${PATH}"
In this example, we use the export
command to add a directory to the PATH
variable. This allows us to modify the environment and make the directory accessible for command execution.
Understanding and utilizing the shell environment in bash scripting gives you access to a wealth of information and functionality that can enhance the capabilities of your scripts.
Command Substitution in Bash Scripting
Command substitution is a useful feature of bash scripting that allows you to capture the output of a command and use it as input for another command. Let’s take a look at a couple of examples.
Example 1: Capturing command output
#!/bin/bash # Capture the output of the date command current_date=$(date) # Print the captured output echo "Current date: ${current_date}"
In this example, we use command substitution to capture the output of the date
command. We assign the captured output to the variable current_date
and then use the echo
command to print the value of the variable.
Example 2: Using command substitution in a command
#!/bin/bash # Count the number of files in a directory file_count=$(ls -l | wc -l) # Print the file count echo "Number of files: ${file_count}"
In this example, we use command substitution to capture the output of the ls -l
command, which lists the files in the current directory. We then pipe the output to the wc -l
command, which counts the number of lines in the output. Finally, we assign the result to the variable file_count
and use the echo
command to print the value.
Command substitution provides a useful way to capture and manipulate command output within your bash scripts, allowing for more complex and dynamic functionality.
Related Article: How to Manipulate Quotes & Strings in Bash Scripts
Best Practices for Writing Bash Scripts
When writing bash scripts, it is important to follow best practices to ensure readability, maintainability, and reliability. Here are a few best practices to consider:
1. Use meaningful variable and function names: Choose names that accurately describe the purpose of the variable or function. This improves readability and makes your script easier to understand.
2. Comment your code: Add comments to explain the purpose and functionality of your script. This helps other developers (including your future self) understand your code and troubleshoot issues.
3. Handle errors gracefully: Use error handling techniques such as conditional statements and error messages to handle potential errors or unexpected behavior. This improves the reliability of your script and helps users understand what went wrong.
4. Test your scripts: Before deploying your scripts, thoroughly test them to ensure they perform as expected. Check for edge cases, handle different scenarios, and validate the output.
5. Keep scripts modular: Break your scripts into reusable functions or separate files to improve maintainability and reusability. This allows you to easily update specific parts of your script without affecting the entire script.
Additional Resources
If you’re new to bash scripting or want to enhance your skills, here are some resources to help you get started:
– Bash Reference Manual – The official documentation for the bash shell. This manual provides in-depth information about bash scripting and its features.
– ShellCheck – A static analysis tool for shell scripts that helps identify common issues and improve script quality.
– r/bash – A community on Reddit dedicated to bash scripting. This is a great place to ask questions, share scripts, and learn from other bash enthusiasts.
These resources can provide you with the knowledge and guidance you need to become proficient in bash scripting.