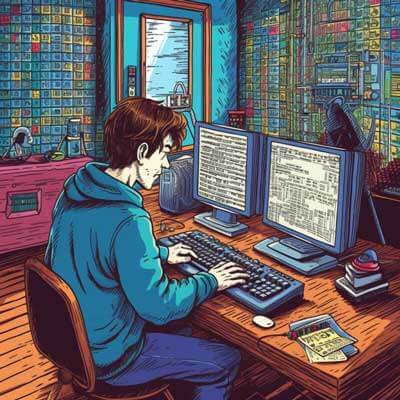
Table of Contents
What are local variables in bash scripting?
A local variable is a variable that is only accessible within a specific scope, such as a function or a block of code. Local variables are not visible or accessible outside of their defined scope, making them useful for encapsulating data and avoiding naming conflicts with other variables.
To declare a variable as local in bash, we use the local
keyword followed by the variable name. Here's an example:
#!/bin/bash my_function() { local message="Hello, world!" echo $message } my_function echo $message # This will result in an empty value
In the above example, the message
variable is declared as local within the my_function
function. It is only accessible within the function, and any attempt to access it outside of the function will result in an empty value.
Related Article: Displaying Images using Wget Bash Script in Linux
How to declare a variable in a bash script?
To declare a variable in a bash script, you simply assign a value to it. Bash variables are untyped, meaning you don't need to specify a data type when declaring them. Here's an example:
#!/bin/bash name="John Doe" age=30 is_student=true
In the above example, we declare three variables: name
, age
, and is_student
. The name
variable stores a string, the age
variable stores an integer, and the is_student
variable stores a boolean value.
How to assign a value to a variable in a bash script?
Assigning a value to a variable in a bash script is done by using the =
operator. Here's an example:
#!/bin/bash name="John Doe" age=30 is_student=true
In the above example, we assign the value "John Doe" to the name
variable, the value 30 to the age
variable, and the value true to the is_student
variable.
Are bash variables local to a script by default?
However, it is possible to declare variables as local within a specific scope, such as a function or a block of code. This allows for better encapsulation and prevents conflicts with variables of the same name in other parts of the script.
Related Article: How to Loop Through an Array of Strings in Bash
What is the scope of bash variables?
The scope of a bash variable determines where it can be accessed and modified. Bash variables can have either a local or global scope.
- Global scope: Variables with global scope are accessible from anywhere within the script. They can be accessed and modified by any function or block of code. Global variables are declared outside of any function or block of code and are typically declared at the top of the script.
- Local scope: Variables with local scope are only accessible within a specific scope, such as a function or a block of code. They cannot be accessed or modified by code outside of their defined scope. Local variables are declared using the local
keyword within a function or block of code.
Here's an example that demonstrates the scope of bash variables:
#!/bin/bash global_variable="I am global" my_function() { local local_variable="I am local" echo $local_variable echo $global_variable } my_function echo $local_variable # This will result in an empty value echo $global_variable # This will print "I am global"
In the above example, we have a global variable named global_variable
and a local variable named local_variable
within the my_function
function. The local variable is only accessible within the function, while the global variable is accessible from anywhere within the script.
How to make a bash variable global?
To make a bash variable global, you simply declare it outside of any function or block of code. Global variables are accessible from anywhere within the script. Here's an example:
#!/bin/bash global_variable="I am global" my_function() { echo $global_variable } my_function echo $global_variable # This will print "I am global"
In the above example, the global_variable
is declared outside of any function or block of code, making it a global variable. It can be accessed and modified by any part of the script.
How to access environment variables in a bash script?
Environment variables are variables that are set in the shell environment and are accessible to all processes running on the system. In bash scripts, we can access environment variables using the $
symbol followed by the variable name. Here's an example:
#!/bin/bash echo $HOME # This will print the value of the HOME environment variable echo $PATH # This will print the value of the PATH environment variable
In the above example, we access the values of the HOME
and PATH
environment variables using the $
symbol.
How to pass variables between bash scripts?
There are several ways to pass variables between bash scripts. Here are two common methods:
1. Command-line arguments: You can pass variables to a bash script as command-line arguments. The variables can be accessed within the script using special variables like $1
, $2
, etc., which represent the first, second, and so on, command-line arguments. Here's an example:
#!/bin/bash echo "Hello, $1!"
In the above example, the script takes a name as a command-line argument and prints a greeting message.
2. Environment variables: You can also pass variables between bash scripts using environment variables. The variables can be set in one script and accessed in another script using the $
symbol followed by the variable name. Here's an example:
Script 1:
#!/bin/bash export my_variable="Hello, world!"
Script 2:
#!/bin/bash echo $my_variable # This will print "Hello, world!"
In the above example, the my_variable
environment variable is set in Script 1 using the export
command, and it is accessed in Script 2 using the $
symbol.
Related Article: How To Split A String On A Delimiter In Bash
Can a variable declared in one script be accessed in another script?
If you want to share variables between scripts without using environment variables, you can write the variable value to a file in one script and read it from the file in another script. Alternatively, you can use inter-process communication techniques such as pipes or sockets to transfer data between scripts.
What is the difference between shell variables and environment variables?
Shell variables and environment variables are both used to store and retrieve data within a shell or a shell script, but they have some key differences:
- Scope: Shell variables have a local scope and are only accessible within the shell or script in which they are declared. Environment variables, on the other hand, have a global scope and are accessible to all processes running on the system.
- Visibility: Shell variables are not visible to child processes spawned by the shell or script. Environment variables, however, are inherited by child processes and can be accessed by them.
- Persistence: Shell variables are not persistent and are lost when the shell or script terminates. Environment variables, on the other hand, are persistent and can be accessed by other processes even after the shell or script that set them has terminated.
- Setting: Shell variables are typically set and modified within a shell or script using the =
operator. Environment variables are set using the export
command or by specifying them in shell configuration files.
It's important to note that environment variables can be accessed as shell variables within a script. When accessing an environment variable as a shell variable, its scope will be limited to the script.