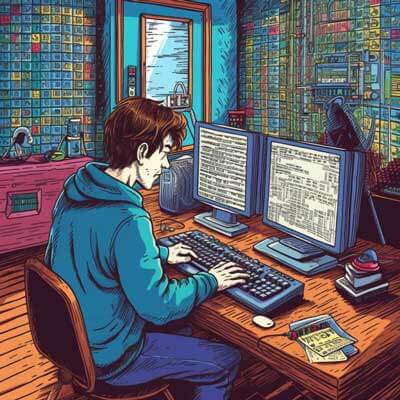
- Continuing a Bash Script After Executing a Curl Command
- Common Techniques for Handling Curl Output in a Bash Script
- Checking the Response Code of a Curl Command in a Bash Script
- Special Considerations when Using Curl in a Shell Script
- Best Practices for Writing a Bash Script that Uses Curl
- Passing Variables to a Curl Command in a Bash Script
- Running Multiple Curl Commands in Parallel in a Bash Script
- Handling Errors or Failures in a Curl Command within a Bash Script
- Syntax for a Curl Command in a Bash Script
- Additional Resources
Continuing a Bash Script After Executing a Curl Command
#!/bin/bash curl -s https://example.com echo "Curl command completed"
In this example, the -s
option tells curl to operate in silent mode, which means it will not output any progress or error messages. After executing the curl command, the script will print “Curl command completed” to the terminal.
Related Article: Tutorial on Traceroute in Linux
Common Techniques for Handling Curl Output in a Bash Script
When working with curl in a Bash script, there are several techniques you can use to handle the output. Here are a few common ones:
1. Saving the output to a file:
#!/bin/bash curl -s https://example.com > output.txt
In this example, the output of the curl command is redirected to a file named “output.txt” using the >
symbol. You can then read the contents of the file or process it further in your script.
2. Assigning the output to a variable:
#!/bin/bash response=$(curl -s https://example.com) echo "Response: $response"
In this example, the output of the curl command is assigned to the variable response
using the $()
syntax. You can then use the variable in your script as needed.
3. Piping the output to another command:
#!/bin/bash curl -s https://example.com | grep "pattern"
In this example, the output of the curl command is piped to the grep
command, which searches for a specific pattern in the output. You can replace “pattern” with the desired pattern to filter the output.
These are just a few examples of how you can handle the output of a curl command in a Bash script. Depending on your specific requirements, you may need to use different techniques or combine multiple techniques.
Checking the Response Code of a Curl Command in a Bash Script
When making HTTP requests with curl in a Bash script, it is often useful to check the response code to determine if the request was successful or if there was an error. The response code indicates the status of the request, with 2xx codes indicating success and 4xx or 5xx codes indicating an error.
You can use the -w
option in curl to specify a format string that includes the response code. Here’s an example:
#!/bin/bash response_code=$(curl -s -o /dev/null -w "%{http_code}" https://example.com) echo "Response code: $response_code"
In this example, the -s
option tells curl to operate in silent mode, so it does not output any progress or error messages. The -o /dev/null
option tells curl to discard the downloaded content and not write it to a file. The -w "%{http_code}"
option tells curl to output only the response code. The response code is then assigned to the variable response_code
, which can be used in the script as needed.
You can also use the response code in an if statement to perform different actions based on the result of the curl command. Here’s an example:
#!/bin/bash response_code=$(curl -s -o /dev/null -w "%{http_code}" https://example.com) if [[ $response_code -eq 200 ]]; then echo "Request successful" else echo "Request failed with response code $response_code" fi
In this example, if the response code is 200 (indicating success), the script will print “Request successful”. Otherwise, it will print “Request failed with response code $response_code”, where $response_code
is the actual response code returned by the curl command.
Special Considerations when Using Curl in a Shell Script
When using curl in a shell script, there are a few special considerations to keep in mind:
1. Error handling: By default, curl does not exit with a non-zero status code if an error occurs during the request. To enable proper error handling in your script, you can use the -f
option, which stands for “fail on error”. For example:
#!/bin/bash curl -f https://example.com if [[ $? -ne 0 ]]; then echo "Error: Request failed" fi
In this example, the -f
option tells curl to exit with a non-zero status code if an error occurs. The $?
variable contains the exit status of the last command, so we can check if it is not equal to 0 to detect errors.
2. Timeout: By default, curl does not have a timeout set, which means it will wait indefinitely for a response. This can cause your script to hang if the server is unresponsive. To set a timeout for the request, you can use the --max-time
option. For example:
#!/bin/bash curl --max-time 10 https://example.com
In this example, the --max-time 10
option tells curl to timeout after 10 seconds if no response is received.
3. SSL certificate verification: By default, curl verifies the SSL certificate of the server it is connecting to. If the certificate is invalid or self-signed, curl will display an error and the request will fail. To disable SSL certificate verification, you can use the -k
option. However, this is not recommended in production environments, as it can leave your script vulnerable to man-in-the-middle attacks. Here’s an example:
#!/bin/bash curl -k https://example.com
In this example, the -k
option tells curl to skip SSL certificate verification.
These are some of the special considerations you should keep in mind when using curl in a shell script. Depending on your specific requirements, you may need to use additional options or techniques.
Related Article: Displaying Images using Wget Bash Script in Linux
Best Practices for Writing a Bash Script that Uses Curl
When writing a Bash script that uses curl, it’s important to follow best practices to ensure your script is reliable, efficient, and maintainable. Here are some best practices to consider:
1. Use command-line options: Use command-line options to make your script more flexible. For example, you can pass the URL as an argument to the script, allowing it to be easily reused for different URLs.
2. Error handling: Implement proper error handling in your script. Check the exit status of the curl command and take appropriate action if an error occurs. You can also use the set -e
option at the beginning of your script to make it exit immediately if any command fails.
3. Logging: Add logging to your script to make it easier to troubleshoot issues. You can redirect the output of the curl command to a log file or use the logger
command to write log messages to the system log.
4. Use variables: Use variables to store commonly used values, such as the URL or API key. This makes your script more readable and allows for easy modification of these values.
5. Comment your code: Add comments to explain the purpose and logic of your script. This makes it easier for others (or your future self) to understand and maintain the script.
6. Test your script: Test your script with different scenarios and edge cases to ensure it behaves as expected. This can help uncover any potential issues or bugs.
7. Keep it modular: Break your script into smaller, reusable functions to improve readability and maintainability. This makes it easier to understand the different parts of your script and makes it more reusable in other scripts.
8. Use proper indentation: Indent your code consistently to improve readability. This makes it easier to understand the flow and logic of your script.
These are just a few best practices to consider when writing a Bash script that uses curl. By following these practices, you can create scripts that are easier to understand, maintain, and debug.
Passing Variables to a Curl Command in a Bash Script
In a Bash script, you can pass variables to a curl command by using string interpolation or concatenation. Here are two examples:
1. String interpolation:
#!/bin/bash url="https://example.com/api" api_key="YOUR_API_KEY" curl -s "$url?api_key=$api_key"
In this example, the $url
and $api_key
variables are interpolated within the string using double quotes. The resulting URL will be something like https://example.com/api?api_key=YOUR_API_KEY
.
2. String concatenation:
#!/bin/bash url="https://example.com/api" api_key="YOUR_API_KEY" curl -s $url"?api_key="$api_key
In this example, the $url
and $api_key
variables are concatenated with the string using the =
operator. The resulting URL will be the same as in the previous example.
Both approaches are valid and will produce the same result. Choose the one that you find more readable and maintainable.
Running Multiple Curl Commands in Parallel in a Bash Script
To run multiple curl commands in parallel in a Bash script, you can use background processes and the wait
command. Here’s an example:
#!/bin/bash curl -s https://example.com/api/1 & curl -s https://example.com/api/2 & curl -s https://example.com/api/3 & wait
In this example, we have three curl commands that are executed concurrently using the &
symbol at the end of each command. This starts each command in the background as a separate process.
The wait
command is then used to wait for all background processes to complete before continuing with the script. This ensures that the script does not exit before all curl commands have finished.
You can add as many curl commands as needed, and they will all run in parallel. However, keep in mind that running too many concurrent requests can put a strain on the server and may result in slower response times or errors.
Related Article: How to Set LD_LIBRARY_PATH in Linux
Handling Errors or Failures in a Curl Command within a Bash Script
When a curl command fails or returns an error, it’s important to handle it properly in your Bash script. There are several techniques you can use to handle errors or failures in a curl command:
1. Check the exit status: After executing a curl command, you can check its exit status using the $?
variable. A value of 0 indicates success, while a non-zero value indicates an error. Here’s an example:
#!/bin/bash curl -s https://example.com if [[ $? -ne 0 ]]; then echo "Error: Curl command failed" fi
In this example, if the exit status of the curl command is not equal to 0, the script will print an error message.
2. Use the -f
option: The -f
option, also known as “fail on error”, tells curl to exit with a non-zero status code if an error occurs during the request. You can use this option to enable proper error handling in your script. Here’s an example:
#!/bin/bash curl -f https://example.com if [[ $? -ne 0 ]]; then echo "Error: Curl command failed" fi
In this example, if the curl command encounters an error, it will exit with a non-zero status code and the script will print an error message.
3. Redirect error output: You can redirect the error output of a curl command to a file using the 2>
operator. This allows you to capture and analyze any error messages that are generated. Here’s an example:
#!/bin/bash curl -s https://example.com 2> error.log if [[ -s error.log ]]; then echo "Error: Curl command failed. Check error.log for details." fi
In this example, any error messages generated by the curl command will be redirected to a file named “error.log”. The script then checks if the file is not empty (-s
checks if the file size is greater than zero) and prints an error message if it is.
These are just a few techniques you can use to handle errors or failures in a curl command within a Bash script. Depending on your specific requirements, you may need to use different techniques or combine multiple techniques.
Syntax for a Curl Command in a Bash Script
Curl is a useful command-line tool for making HTTP requests. It is commonly used in Bash scripts to interact with REST APIs or download files from the internet. The syntax for a curl command in a Bash script is as follows:
curl [options] [URL]
Here, [options]
represents any additional flags or parameters that you want to pass to the curl command, and [URL]
is the URL of the resource you want to interact with or download.
Let’s see an example of a curl command in a Bash script that downloads a file from a remote server:
#!/bin/bash curl -O https://example.com/file.txt
In this example, the -O
option tells curl to write the output to a file instead of printing it to the terminal. The URL https://example.com/file.txt
specifies the location of the file we want to download. When you run this script, curl will download the file and save it as “file.txt” in the current directory.
Additional Resources
– LinuxQuestions.org – Example of continuing a bash script after a curl command