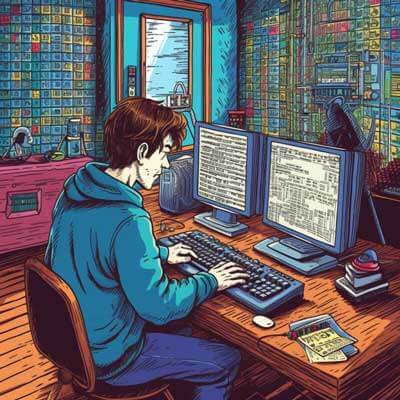
Table of Contents
The Importance of Indentation in Bash Scripts
Bash scripting is a useful tool for automating tasks and running shell commands on Linux systems. However, writing clean and well-structured bash scripts is crucial for maintaining code readability and ensuring that scripts are easy to understand and maintain. One key aspect of bash script readability is proper indentation.
Indentation refers to the use of whitespace at the beginning of lines to visually indicate the structure and hierarchy of code blocks. In bash scripts, indentation is used to group related commands, define loops and conditionals, and enhance overall script organization.
Proper indentation can significantly improve the readability and understandability of bash scripts. It helps developers quickly identify the scope and boundaries of code blocks, making it easier to follow the flow of the script. Additionally, well-indented code is less prone to errors and makes it easier to spot syntax mistakes or logical issues.
Let's take a look at an example that demonstrates the importance of indentation in bash scripts. Consider the following poorly indented script that aims to list all files in a directory and its subdirectories:
#!/bin/bash function list_files_recursive() { for file in $(ls "$1") do if [ -d "$1/$file" ]; then list_files_recursive "$1/$file" else echo "$1/$file" fi done } list_files_recursive "/path/to/directory"
In this example, the lack of indentation makes it difficult to understand the structure of the code. It is not immediately clear where the loop and conditional statements begin and end. This can lead to confusion and potential errors when modifying or debugging the script.
Now, let's improve the indentation of the script:
#!/bin/bash function list_files_recursive() { for file in $(ls "$1") do if [ -d "$1/$file" ]; then list_files_recursive "$1/$file" else echo "$1/$file" fi done } list_files_recursive "/path/to/directory"
With proper indentation, the script becomes much more readable and easier to understand. Each level of indentation represents a code block, and it is clear where each block begins and ends. This makes it easier to identify the structure of the loop and conditional statements.
Related Article: Interactions between Bash Scripts and Linux Command History
Best Practices for Indenting Bash Scripts
Proper indentation is essential for writing clean and maintainable bash scripts. Here are some best practices to follow when indenting bash scripts:
1. Use consistent indentation: Consistency is key when it comes to indentation. Choose a standard indent size, such as two or four spaces, and stick to it throughout the script. Mixing different indentation styles can make the code harder to read and understand.
2. Indent with spaces, not tabs: While both spaces and tabs can be used for indentation, it is generally recommended to use spaces for bash scripts. Spaces are more widely supported and ensure consistent indentation across different text editors and environments.
3. Indent nested code blocks: When writing loops, conditionals, or function definitions, indent the code inside these blocks to indicate their hierarchical structure. Each level of indentation should represent a new level of nesting.
4. Align related statements: If you have multiple statements that are related or belong to the same logical block, align them vertically to improve readability. For example, aligning assignment statements or function calls can make the code easier to scan and understand.
5. Use indentation for command grouping: In bash, parentheses or curly braces can be used to group commands. Indent the commands inside these groupings to visually indicate their relationship. This helps distinguish command groupings from other code blocks and improves code organization.
6. Avoid excessive indentation: While indentation is important, excessive indentation can make the code harder to read. If your code requires excessive indentation levels, consider refactoring it into smaller functions or scripts to improve readability.
Let's see an example that demonstrates these best practices:
#!/bin/bash function process_files() { for file in $(ls "$1") do if [ -d "$1/$file" ]; then process_files "$1/$file" else # Aligning related statements for readability file_size=$(du -h "$1/$file" | awk '{print $1}') file_name=$(basename "$1/$file") echo "File: $file_name, Size: $file_size" fi done } process_files "/path/to/directory"
In this example, we follow the best practices mentioned above. The code is consistently indented using spaces, related statements are aligned vertically, and nested code blocks are indented accordingly. This makes the script more readable and easier to understand.
The Impact of Proper Indentation on Script Readability
Proper indentation plays a crucial role in enhancing the readability of bash scripts. It allows developers to quickly and easily understand the structure and flow of the code, making it easier to modify, debug, and maintain scripts.
Here are some specific ways in which proper indentation improves script readability:
1. Visual hierarchy: Indentation visually represents the hierarchy of code blocks, such as loops and conditionals. Each level of indentation indicates a new level of nesting, allowing developers to quickly identify the scope and boundaries of code blocks. This makes it easier to follow the flow of the script and understand how different parts of the script interact with each other.
2. Code organization: Indentation helps organize code into logical sections and groups related commands together. By indenting code blocks, developers can easily identify the purpose and functionality of different parts of the script. This improves the overall organization of the script and allows developers to locate specific sections of code more efficiently.
3. Readability and understandability: Proper indentation improves the overall readability and understandability of bash scripts. Well-indented code is easier to read and comprehend, even for developers who are not familiar with the script. It reduces cognitive load and makes it easier to spot syntax errors, logical issues, or potential bugs.
Let's illustrate the impact of proper indentation on script readability with an example. Consider the following script that calculates the sum of even numbers in a given range:
#!/bin/bash function sum_even_numbers() { for ((i=1; i<=10; i++)) do if ((i % 2 == 0)); then sum=$((sum + i)) fi done echo "Sum of even numbers: $sum" } sum_even_numbers
In this example, the lack of indentation makes it challenging to understand the structure of the script. It is not immediately clear where the loop begins and ends, or where the conditional statement is located. This can lead to confusion and potential errors when modifying or debugging the script.
Now, let's improve the indentation of the script:
#!/bin/bash function sum_even_numbers() { for ((i=1; i<=10; i++)) do if ((i % 2 == 0)); then sum=$((sum + i)) fi done echo "Sum of even numbers: $sum" } sum_even_numbers
With proper indentation, the script becomes much more readable and easier to understand. Each level of indentation represents a code block, and it is clear where each block begins and ends. This makes it easier to identify the structure of the loop and conditional statements, improving the overall readability and understandability of the script.
The Significance of Formatting Bash Scripts Correctly
Formatting plays a crucial role in writing clean and professional-looking bash scripts. Properly formatted scripts are not only visually appealing but also easier to read, understand, and maintain.
Here are some reasons why formatting is significant in bash scripts:
1. Consistency: Formatting ensures consistent code style throughout the script. By following a consistent formatting pattern, developers can reduce confusion and make the script more predictable. Consistency is particularly important when working on large projects or collaborating with other developers.
2. Readability: Proper formatting improves the readability of bash scripts. Well-organized code with consistent indentation, spacing, and line breaks is easier to follow and understand. It reduces cognitive load and allows developers to focus on the logic and functionality of the script rather than struggling to decipher the code.
3. Maintainability: Well-formatted scripts are easier to maintain and modify. Clear and consistent formatting makes it easier to identify and fix issues, add new functionality, or refactor existing code. It also helps in code reviews and makes it easier for other developers to understand and contribute to the script.
4. Debugging: Properly formatted scripts can significantly speed up the debugging process. When encountering errors or unexpected behavior, well-formatted code allows developers to quickly locate and isolate the problematic sections. It also makes it easier to add debugging statements or comment out code for troubleshooting purposes.
Now, let's look at an example that demonstrates the significance of formatting in bash scripts. Consider the following poorly formatted script that calculates the factorial of a number:
#!/bin/bash function factorial() { if [ $1 -le 1 ]; then echo 1 else factorial=$(( $1 * $(factorial $(( $1 - 1 ))) )) echo $factorial fi } result=$(factorial 5) echo "Factorial of 5 is: $result"
In this example, the lack of consistent formatting makes the code difficult to read and understand. The inconsistent line spacing, inconsistent indentation, and lack of proper spacing around operators make it challenging to follow the logic of the script.
Now, let's improve the formatting of the script:
#!/bin/bash function factorial() { if [ $1 -le 1 ]; then echo 1 else factorial=$(( $1 * $(factorial $(( $1 - 1 ))) )) echo $factorial fi } result=$(factorial 5) echo "Factorial of 5 is: $result"
With proper formatting, the script becomes more readable and easier to understand. The consistent indentation, spacing, and line breaks make it easier to identify the structure of the script and follow the flow of the code.
Related Article: Executing Scripts in Linux Without Bash Command Line
Consequences of Improper Indentation in Bash Scripts
Improper indentation in bash scripts can have several negative consequences. It not only makes the code harder to read and understand but also increases the likelihood of introducing errors and bugs. Let's explore some of the consequences of improper indentation in more detail.
1. Reduced readability: Improper indentation makes the code harder to read and comprehend. Without clear indentation, it becomes challenging to identify the structure and hierarchy of code blocks, such as loops and conditionals. This can lead to confusion and make it difficult to understand the logic and flow of the script.
2. Increased cognitive load: When working with scripts that have poor indentation, developers need to spend more mental effort to understand and follow the code. This increased cognitive load can slow down the development process, increase the likelihood of mistakes, and make it harder to collaborate with other developers.
3. Difficulty in debugging: Improper indentation can make the debugging process more challenging. When encountering errors or unexpected behavior, it becomes harder to isolate the problematic sections of code and identify the root cause. This can lead to longer debugging cycles and delays in resolving issues.
4. Higher error-proneness: Scripts with improper indentation are more prone to errors and bugs. Developers may inadvertently introduce syntax errors or logical issues when modifying or extending poorly indented code. These errors can be difficult to detect and fix, leading to increased development time and potential production issues.
Let's consider an example that demonstrates the consequences of improper indentation in bash scripts. Consider the following poorly indented script that aims to calculate the sum of numbers in a given range:
#!/bin/bash function calculate_sum() { for (( i = 1; i <= 10; i++ )) do sum=$(( sum + i )) done echo "Sum of numbers: $sum" } calculate_sum
In this example, the lack of proper indentation makes it difficult to understand the structure of the script. It is not immediately clear where the loop begins and ends, and how the sum is calculated. This can lead to confusion and potential errors when modifying or debugging the script.
Now, let's improve the indentation of the script:
#!/bin/bash function calculate_sum() { for (( i = 1; i <= 10; i++ )) do sum=$(( sum + i )) done echo "Sum of numbers: $sum" } calculate_sum
With proper indentation, the script becomes much more readable and easier to understand. Each level of indentation represents a code block, and it is clear where each block begins and ends. This makes it easier to identify the structure of the loop and the calculation of the sum.
The Relationship Between Indentation and Script Execution
The relationship between indentation and script execution in bash is straightforward. Bash does not interpret or execute indentation as part of the script's logic or functionality. Indentation is purely for human readability and has no impact on how the script is executed by the interpreter.
However, proper indentation is essential for maintaining code readability and ensuring that scripts are easy to understand and maintain. By following indentation best practices, developers can improve the readability of their scripts, making them easier to follow and modify.
It's worth noting that inconsistent or incorrect indentation can introduce syntax errors or logical issues that may affect script execution. For example, forgetting to properly indent a command within a loop or conditional statement can lead to unexpected behavior or errors.
Let's consider an example that demonstrates the relationship between indentation and script execution. Consider the following script that calculates the sum of numbers in a given range:
#!/bin/bash function calculate_sum() { for ((i=1; i<=10; i++)) do sum=$((sum + i)) done echo "Sum of numbers: $sum" } calculate_sum
In this example, the indentation does not affect how the script is executed. The script will calculate the sum of numbers correctly regardless of the indentation style. However, improper indentation can make the script harder to read and understand, increasing the likelihood of introducing errors or bugs.
Now, let's consider an example of incorrect indentation that affects script execution:
#!/bin/bash function calculate_sum() { for ((i=1; i<=10; i++)) do sum=$((sum + i)) done echo "Sum of numbers: $sum" } calculate_sum
In this example, the improper indentation of the sum=$((sum + i))
line within the loop leads to a logical error. The variable sum
is not properly incremented because it is not part of the loop body. As a result, the calculated sum will be incorrect.
Tools for Automatic Indentation of Bash Scripts
Automatic indentation tools can significantly simplify the process of formatting bash scripts. These tools analyze the structure of the code and apply consistent indentation based on predefined rules or user-defined configurations. Let's explore some popular tools for automatic indentation of bash scripts.
1. GNU Indent: GNU Indent is a useful and widely-used code formatter that supports various programming languages, including bash. It provides numerous options for customizing the formatting style, such as indentation width, tab width, and brace style. GNU Indent can be run from the command line or integrated into text editors and IDEs.
2. ShellCheck: ShellCheck is a static analysis tool for bash scripts that not only detects common syntax errors but also provides suggestions for improving code quality and readability. While ShellCheck does not specifically focus on indentation, it can identify inconsistent or incorrect indentation as part of its analysis. ShellCheck can be run from the command line or integrated into text editors and CI/CD pipelines.
3. shfmt: shfmt is a command-line tool that automatically formats shell scripts, including bash scripts, according to a predefined set of rules. It supports various formatting options, such as indentation style, whitespace usage, and command grouping. shfmt can be easily integrated into development workflows, including text editors and IDEs.
4. Editor and IDE plugins: Many popular text editors and IDEs, such as Visual Studio Code, Sublime Text, and Vim, offer plugins or extensions that provide automatic indentation for bash scripts. These plugins typically leverage external tools or libraries to handle the indentation process. They offer customizable formatting options and can be configured to automatically format the code upon saving or manually triggered by the user.
It's important to note that automatic indentation tools may have different default formatting styles and options. It's recommended to review and customize the settings according to personal or project preferences. Additionally, it's a good practice to ensure that the selected indentation tool is compatible with the specific version of bash being used.
Let's see an example of using the shfmt
tool to automatically indent a bash script:
#!/bin/bash function calculate_sum() { for ((i=1; i<=10; i++)) do sum=$((sum + i)) done echo "Sum of numbers: $sum" } calculate_sum
Common Coding Conventions for Bash Scripts
Coding conventions provide a set of guidelines and rules for writing consistent and maintainable code. They define the preferred coding style, formatting, and naming conventions to be followed by developers. While coding conventions can vary among different organizations or projects, there are some common conventions for bash scripts that developers can consider.
1. Indentation: Use consistent indentation with two or four spaces. Avoid using tabs, as they may lead to inconsistent formatting across different text editors or environments.
2. Whitespace: Use whitespace to improve code readability. Add spaces around operators and after commas to enhance code clarity. For example, use sum=$((sum + 1))
instead of sum=$((sum+1))
. Additionally, consider adding an empty line between logical sections of code to improve visual separation.
3. Naming conventions: Follow meaningful and descriptive naming conventions for variables, functions, and files. Use lowercase letters and underscores for variable and function names (e.g., file_name
, sum_of_numbers
). Avoid using special characters or spaces in names to ensure compatibility and readability.
4. Quotes: Use single quotes (''
) for literal strings that do not require variable expansion or command substitution. Use double quotes (""
) for strings that require variable expansion or command substitution. For example, use echo 'Hello, World!'
or echo "The sum is: $sum"
.
5. Shebang: Always include a shebang line (#!/bin/bash
) at the beginning of the script to specify the interpreter to be used when executing the script.
6. Commenting: Add comments to explain the purpose and functionality of the script, functions, and complex code blocks. Use clear and concise comments that help other developers understand the code. Avoid excessive commenting or comments that state the obvious.
7. Error handling: Implement appropriate error handling mechanisms, such as checking the exit status of commands, handling unexpected inputs, and displaying informative error messages. Use set -o errexit
(or set -e
) to exit the script immediately if any command fails.
8. Function definitions: Define functions using the function
keyword followed by the function name and parentheses. Place the opening and closing braces on separate lines. For example:
function calculate_sum() { # Function code here }
9. Conditional statements: Use consistent formatting for conditional statements, such as if
, elif
, and else
. Indent the code within the conditional statements. Use spaces around operators for improved readability. For example:
if [ $variable -eq 1 ]; then # Code if condition is true elif [ $variable -eq 2 ]; then # Code if condition is true else # Code if all conditions are false fi
10. Loop statements: Use consistent formatting for loops, such as for
and while
. Indent the code within the loop. Use spaces around operators for improved readability. For example:
for item in "${array[@]}"; do # Loop code here done
These are some common coding conventions for bash scripts that can improve code consistency, readability, and maintainability. It's important to consider the specific conventions followed by the project or organization and adapt accordingly. Consistency within a codebase is key, and it's crucial to communicate and agree upon coding conventions among team members.
Related Article: Tutorial: Using Unzip Command in Linux
Improving Script Maintainability Through Indentation
Maintainability is a critical aspect of software development that focuses on making code easy to understand, modify, and extend. Proper indentation plays a crucial role in improving script maintainability in bash.
Here are some ways in which indentation improves script maintainability:
1. Code organization: Indentation helps organize code into logical sections and groups related commands together. It allows developers to quickly identify the purpose and functionality of different parts of the script. Well-organized code is easier to navigate, modify, and extend, reducing maintenance effort and minimizing the risk of introducing errors.
2. Readability: Proper indentation improves the overall readability of bash scripts. Well-indented code is easier to read and understand, even for developers who are not familiar with the script. This is particularly important when maintaining scripts over time or when collaborating with other developers. Readable code reduces cognitive load and speeds up the process of understanding and modifying the script.
3. Ease of modification: Indentation makes it easier to modify and extend existing code. By following consistent indentation practices, developers can quickly identify the scope and boundaries of code blocks, such as loops and conditionals. This allows for safer and more efficient modifications, as developers can focus on specific sections of the code without affecting unrelated parts.
4. Debugging and troubleshooting: Properly indented code simplifies the debugging process. When encountering errors or unexpected behavior, well-indented code allows developers to quickly locate and isolate problematic sections. It also makes it easier to add debugging statements or comment out code for troubleshooting purposes. This reduces the time required to identify and fix issues, improving script maintainability.
5. Collaboration and knowledge sharing: Well-indented code is easier to understand and collaborate on. When multiple developers are working on the same script, consistent indentation ensures that everyone can follow and modify the code more effectively. It facilitates knowledge sharing and allows new team members to quickly grasp the functionality and structure of the script.
Let's consider an example that demonstrates how indentation improves script maintainability. Consider the following script that performs a series of operations on a list of files:
#!/bin/bash function process_files() { for file in $(ls "$1") do if [ -d "$1/$file" ]; then process_files "$1/$file" else file_extension="${file##*.}" if [ "$file_extension" == "txt" ]; then process_txt_file "$1/$file" elif [ "$file_extension" == "csv" ]; then process_csv_file "$1/$file" elif [ "$file_extension" == "json" ]; then process_json_file "$1/$file" fi fi done } function process_txt_file() { # Code to process text file } function process_csv_file() { # Code to process CSV file } function process_json_file() { # Code to process JSON file } process_files "/path/to/directory"
In this example, the script is properly indented, making it easier to understand and maintain. Each level of indentation represents a code block, and it is clear where each block begins and ends. This improves the script's maintainability by allowing developers to quickly identify and modify specific parts of the code.
Style Guides and Standards for Bash Scripting
Style guides and coding standards provide a set of guidelines and rules for writing code in a consistent and maintainable manner. They define the preferred coding style, formatting, naming conventions, and best practices for a particular programming language or technology.
While there is no official style guide or coding standard specifically for bash scripting, there are several widely recognized conventions and recommendations that developers can follow. These conventions help improve code consistency, readability, and maintainability.
Here are some style guides and standards for bash scripting:
1. Google Shell Style Guide: The Google Shell Style Guide provides recommendations and best practices for writing shell scripts, including bash scripts. It covers various aspects of shell scripting, such as file naming, indentation, whitespace usage, variable naming, function definitions, error handling, and more. The guide emphasizes the importance of readability, maintainability, and portability.
2. Shell Style Guide by Google: While not specific to bash scripting, the Shell Style Guide by Google covers general shell scripting best practices. It provides guidelines for writing clear, concise, and efficient shell scripts, including recommendations for indentation, quoting, variable expansion, command substitution, and error handling.
3. Bash Guide for Beginners: The Bash Guide for Beginners is a comprehensive tutorial that covers various aspects of bash scripting, including style and best practices. It provides guidance on indentation, whitespace usage, quoting, variable naming, function definitions, loops, conditionals, error handling, and more. The guide focuses on writing clean, maintainable, and portable bash scripts.
4. Bash Pitfalls: The Bash Pitfalls website highlights common pitfalls and mistakes to avoid when writing bash scripts. While not specifically a style guide, it provides valuable insights into potential issues and anti-patterns that can affect script readability, maintainability, and correctness.
5. ShellCheck: ShellCheck is a static analysis tool for shell scripts, including bash scripts. While not a style guide per se, ShellCheck provides recommendations and suggestions for improving code quality and adherence to best practices. It analyzes shell scripts for common issues, such as syntax errors, quoting problems, variable misuse, and more. Integrating ShellCheck into the development workflow can help enforce best practices and improve script quality.
It's important to note that style guides and coding standards may vary depending on the project, organization, or personal preferences. Developers should consider the specific conventions followed by their team or project and adapt accordingly. Consistency within a codebase is key, and it's crucial to communicate and agree upon style guidelines among team members.
Tips for Enhancing Readability of Bash Scripts with Indentation
Proper indentation is crucial for enhancing the readability of bash scripts. It helps developers quickly understand the structure and flow of the code, making scripts easier to read, modify, and maintain. Here are some tips for enhancing the readability of bash scripts with indentation:
1. Consistent indentation: Use consistent indentation throughout the script. Choose a standard indent size, such as two or four spaces, and stick to it. Consistency makes the code more predictable and easier to read.
2. Indent nested code blocks: Indent the code inside nested code blocks, such as loops and conditionals. Each level of indentation should represent a new level of nesting. This visually indicates the hierarchical structure of the code.
3. Align related statements: Align related statements vertically to improve readability. For example, align assignment statements or function calls to make the code easier to scan and understand. Vertical alignment can help identify patterns and relationships between statements.
4. Use indentation for command grouping: Use indentation to visually indicate command grouping. Indent commands within parentheses or curly braces to make it clear that they belong to the same logical block. This enhances code organization and improves readability.
5. Avoid excessive indentation: Avoid excessive indentation levels, as they can make the code harder to read. If your code requires excessive indentation, consider refactoring it into smaller functions or scripts to improve readability. Keep indentation levels within a reasonable limit.
6. Use whitespace to improve readability: Use whitespace effectively to improve code readability. Add spaces around operators and after commas to improve code clarity. Additionally, consider adding an empty line between logical sections of code to improve visual separation.
7. Comment and document your code: Use comments to explain the purpose and functionality of the code. Comments provide additional context and make the code easier to understand. Use clear and concise comments that help other developers navigate and modify the code.
8. Follow a consistent coding style: Follow a consistent coding style throughout the script. Consistency improves readability and reduces cognitive load. Consider adopting a recognized coding style guide or establish a team-specific style guide for consistency.
9. Use tools for automatic indentation: Utilize automatic indentation tools, such as GNU Indent or shfmt, to automatically format your bash scripts. These tools analyze the code structure and apply consistent indentation based on predefined rules. They can save time and ensure consistent formatting.
10. Review and refactor your code: Regularly review and refactor your code for readability. Identify sections that are hard to read or understand and consider refactoring them for clarity. Continuously improve the codebase by applying lessons learned and incorporating feedback from code reviews.
Additional Resources
- Bash Style Guide - Indentation
- Linux Documentation Project - Bash Scripting Best Practices