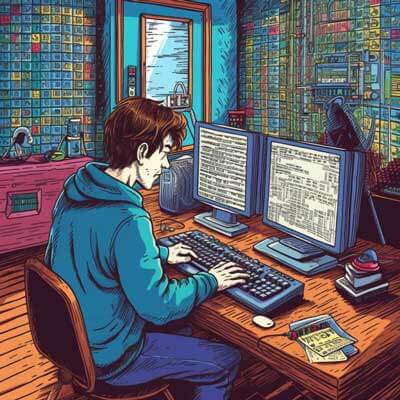
- Getting Started with Docker
- Understanding Containers and Containerization
- Installing Docker on Your Machine
- Windows
- macOS
- Linux
- Creating Your First Docker Container
- Working with Docker Images
- Pulling Docker Images
- Building Docker Images
- Managing Docker Images
- Running and Managing Docker Containers
- Running a Docker Container
- Managing Docker Containers
- Working with Container Logs
- Copying Files to and from Containers
- Using Docker Compose for Multi-Container Applications
- Containerizing Your Existing Applications
- Step 1: Understand Your Application
- Step 2: Create a Dockerfile
- Step 3: Build the Docker Image
- Step 4: Run the Containerized Application
- Step 5: Test and Iterate
- Docker Networking and Linking Containers
- Types of Networks
- Linking Containers
- User-Defined Networks
- Managing Data in Docker Containers
- Volumes
- Bind Mounts
- tmpfs Mounts
- Persistent Storage with Plugins and Drivers
- Scaling and Load Balancing with Docker Swarm
- Securing Docker Containers
- Monitoring and Logging Docker Containers
- 1. Docker Stats
- 2. cAdvisor
- 3. ELK Stack
- 4. Prometheus and Grafana
- Optimizing Docker Performance
- 1. Use Lightweight Base Images
- 2. Minimize Layers
- 3. Utilize Caching
- 4. Optimize Volumes
- 5. Resource Allocation
- 6. Monitor and Tune
- Docker in Production: Best Practices and Tips
- Real World Examples of Docker in Action
Getting Started with Docker
Docker is an open-source platform that allows you to automate the deployment, scaling, and management of applications using containerization. Containers are lightweight and isolated environments that package all the necessary dependencies and libraries required to run an application. This makes it easier to develop, deploy, and run applications consistently across different environments.
To get started with Docker, follow these steps:
Step 1: Install Docker
The first step is to install Docker on your machine. Docker provides installation packages for various operating systems, including Windows, macOS, and Linux. You can find the installation instructions for your specific operating system on the official Docker website: https://docs.docker.com/get-docker/
Step 2: Verify Installation
Once Docker is installed, you can verify the installation by opening a terminal or command prompt and running the following command:
docker version
This command will display the version of Docker installed on your machine, along with information about the client and server components.
Step 3: Run Your First Container
Now that Docker is installed and verified, let’s run our first container. Docker images are the building blocks of containers. You can think of an image as a blueprint that contains all the instructions and dependencies to create a container.
To run a container, you need to pull an image from a Docker registry. Docker Hub is the default public registry that contains a wide range of pre-built images for various applications and technologies. For example, to run a basic web server, you can use the following command:
docker run -d -p 80:80 nginx
This command pulls the latest version of the Nginx image from Docker Hub and starts a container based on that image. The -d
flag runs the container in the background, and the -p
flag maps port 80 of the host machine to port 80 of the container.
Step 4: Explore Docker Commands
Docker provides a comprehensive set of commands to manage containers, images, networks, and volumes. Here are a few commonly used commands:
– docker ps
: Lists all running containers.
– docker images
: Lists all available images.
– docker pull
: Pulls an image from a Docker registry.
– docker stop
: Stops a running container.
– docker rm
: Removes a container.
– docker rmi
: Removes an image.
You can explore the full list of Docker commands in the official Docker documentation: https://docs.docker.com/engine/reference/commandline/docker/
Step 5: Build Your Own Images
While Docker provides a vast collection of pre-built images, you may need to create your own custom images for specific applications or configurations. Docker uses a file called Dockerfile
to define the instructions for building an image.
Here’s a simple example of a Dockerfile for a Node.js application:
# Use an official Node.js runtime as the base image FROM node:14 # Set the working directory in the container WORKDIR /usr/src/app # Copy package.json and package-lock.json to the working directory COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application source code COPY . . # Expose a port for the application to listen on EXPOSE 3000 # Define the command to run the application CMD [ "npm", "start" ]
Once you have a Dockerfile, you can build an image using the docker build
command. For example:
docker build -t my-node-app .
This command builds an image with the tag my-node-app
based on the Dockerfile in the current directory (.
).
These are the basic steps to get started with Docker. As you dive deeper into Docker, you’ll discover more advanced features and techniques for managing containers and orchestrating applications.
Related Article: How to Run a Docker Instance from a Dockerfile
Understanding Containers and Containerization
Containers have become an essential part of modern software development and deployment. They provide a lightweight and portable way to package applications and their dependencies, allowing them to run consistently across different environments. Docker, one of the most popular containerization platforms, has revolutionized the way developers build, ship, and run applications.
At its core, a container is an isolated environment that encapsulates an application and all its dependencies, including the operating system, libraries, and runtime. Unlike traditional virtual machines, containers share the host operating system kernel, which makes them more lightweight and faster to start.
Containerization is the process of creating, running, and managing containers. Docker, the de facto standard for containerization, simplifies this process by providing a platform that automates the creation and management of containers. With Docker, you can package your application, along with its dependencies, into a single container image that can be easily distributed and run on any Docker-enabled host.
To understand how containerization works, let’s take a look at a simple example. Suppose you have a Node.js application that you want to run in a container. First, you need to create a Dockerfile, which is a text file that contains instructions for building a Docker image. Here’s an example of a Dockerfile for a Node.js application:
# Use the official Node.js image as the base FROM node:14 # Set the working directory inside the container WORKDIR /app # Copy the package.json and package-lock.json files COPY package*.json ./ # Install the dependencies RUN npm install # Copy the rest of the application files COPY . . # Expose the port that the application listens on EXPOSE 3000 # Define the command to run the application CMD [ "node", "app.js" ]
In this example, we start with the official Node.js image from Docker Hub. We set the working directory inside the container and copy the package.json and package-lock.json files. Then, we install the dependencies using the npm install
command. Next, we copy the rest of the application files to the container. We expose port 3000, which is the port that the application listens on, and finally, we define the command to run the application.
Once you have created the Dockerfile, you can build the Docker image by running the following command in the same directory as the Dockerfile:
docker build -t myapp .
This command instructs Docker to build an image based on the instructions in the Dockerfile and tag it with the name myapp
. The dot (.
) at the end of the command indicates that the Dockerfile is in the current directory.
After the image is built, you can run it in a container using the following command:
docker run -p 3000:3000 myapp
This command tells Docker to run a container based on the myapp
image and map port 3000 on the host to port 3000 in the container.
Containerization provides several benefits for software development and deployment. It allows developers to package applications with their dependencies, ensuring consistent and reproducible deployments. Containers also provide isolation, which improves security and makes it easier to manage multiple applications running on the same host. Additionally, containers are portable and can be easily moved between different environments, such as development, testing, and production.
In summary, containers and containerization have revolutionized the way we build, ship, and run applications. Docker simplifies the process of creating and managing containers, making it easier for developers to embrace containerization and benefit from its advantages. With Docker, you can package your applications into lightweight and portable containers, ensuring consistent deployments across different environments.
Installing Docker on Your Machine
To get started with Docker, the first step is to install it on your machine. Docker provides installation packages for various operating systems, including Windows, macOS, and Linux.
Windows
If you are using Windows, you can install Docker Desktop, which includes both Docker Engine and Docker CLI. Follow these steps to install Docker on your Windows machine:
1. Go to the official Docker website at https://www.docker.com/get-started.
2. Click on the “Download Docker Desktop” button.
3. Run the installer and follow the on-screen instructions.
4. Once the installation is complete, Docker will start automatically.
Related Article: Build a Movie Search App with GraphQL, Node & TypeScript
macOS
For macOS users, Docker Desktop is also available. Follow these steps to install Docker on your macOS machine:
1. Visit the official Docker website at https://www.docker.com/get-started.
2. Click on the “Download Docker Desktop” button.
3. Run the installer and drag the Docker.app to the Applications folder.
4. Open Docker.app from the Applications folder.
5. Docker will start and prompt you to authorize the Docker Desktop application with your system password.
Linux
Linux users have different ways to install Docker depending on their distribution. Docker provides installation instructions for popular Linux distributions such as Ubuntu, Debian, Fedora, and CentOS. Here’s a general guide to installing Docker on Linux:
1. Visit the official Docker website at https://www.docker.com/get-started.
2. Click on the “Download Docker” button.
3. Select your Linux distribution from the dropdown menu.
4. Follow the step-by-step instructions provided for your specific distribution.
After successfully installing Docker on your machine, you can verify the installation by opening a terminal and running the following command:
docker version
This command will display the installed Docker version and provide information about the Docker Engine and Docker CLI.
Congratulations! You have successfully installed Docker on your machine. In the next chapter, we will learn how to work with Docker containers.
Creating Your First Docker Container
To get started with Docker containerization, you need to create your first Docker container. In this section, we will walk you through the process step by step.
1. Install Docker: Before you can create Docker containers, you need to have Docker installed on your machine. If you haven’t done so already, head over to the official Docker website and follow the instructions for your specific operating system: https://docs.docker.com/get-docker/
2. Verify Docker installation: Once Docker is installed, open a terminal or command prompt and run the following command to verify that Docker is correctly installed and running:
docker --version
This command should display the version of Docker installed on your machine.
3. Choose a base image: Docker containers are built from base images, which are essentially pre-configured operating system images. You can choose from a variety of base images available on the Docker Hub (a public registry of Docker images). For example, if you want to create a container running Ubuntu, you can use the official Ubuntu base image. To pull the Ubuntu base image, run the following command:
docker pull ubuntu
This will download the latest Ubuntu image to your local machine.
4. Create a Dockerfile: A Dockerfile is a text file that contains a set of instructions for Docker to build an image. Create a new file named Dockerfile
(without any file extension) in a directory of your choice. Open the file in a text editor and add the following content:
FROM ubuntu RUN apt-get update && apt-get install -y
Replace with the name of the package you want to install in your container. This package will be installed during the image build process.
5. Build the Docker image: In the same directory where you created the Dockerfile, open a terminal or command prompt and run the following command to build the Docker image:
docker build -t my-container .
This command will build a Docker image named my-container
using the instructions specified in the Dockerfile.
6. Run the Docker container: Once the Docker image is built, you can run a container based on that image. Run the following command to start a container from the my-container
image:
docker run -it my-container
This command starts an interactive shell session inside the container, allowing you to interact with it.
Congratulations! You have created and run your first Docker container. You can now explore further and customize your containers by adding more instructions to the Dockerfile or using different base images.
In the next chapter, we will explore more advanced Docker concepts and features.
Related Article: Tutorial: Managing Docker Secrets
Working with Docker Images
Docker images are the building blocks of containers. They contain everything needed to run a piece of software, including the code, runtime, libraries, and system tools. In this chapter, we will explore the basics of working with Docker images.
To get started, you first need to have Docker installed on your system. If you haven’t done so already, you can download and install Docker from the official website: https://www.docker.com/get-started.
Pulling Docker Images
Docker images are typically hosted in registries, which act as centralized repositories for sharing and distributing images. The most popular Docker registry is Docker Hub, where you can find a vast collection of pre-built images for various applications.
To pull an image from Docker Hub, you can use the docker pull
command followed by the image name and optional tag. For example, to pull the official Ubuntu image, you would run:
docker pull ubuntu
By default, Docker pulls the latest version of the image. If you want to specify a particular version, you can append a tag to the image name. For instance, to pull Ubuntu 18.04, you would run:
docker pull ubuntu:18.04
Building Docker Images
While you can pull pre-built images from registries, you also have the option to build your own custom images. Docker provides a simple and declarative way to define images using Dockerfiles.
A Dockerfile is a text document that contains a set of instructions for building an image. It specifies the base image, copies files, installs dependencies, runs commands, and sets environment variables, among other things.
Here’s an example of a simple Dockerfile that builds an image for a Python web application:
# Use the official Python base image FROM python:3.9 # Set the working directory in the container WORKDIR /app # Copy the requirements file COPY requirements.txt . # Install the dependencies RUN pip install -r requirements.txt # Copy the application code COPY . . # Expose the port EXPOSE 8000 # Define the command to run the application CMD ["python", "app.py"]
To build an image from a Dockerfile, navigate to the directory where the Dockerfile is located and run the following command:
docker build -t myapp .
The -t
flag allows you to specify a tag for the image, in this case, myapp
. The .
at the end indicates the build context, which includes the files and directories in the current directory.
Related Article: Build a Chat Web App with Flask, MongoDB, Reactjs & Docker
Managing Docker Images
Once you have pulled or built Docker images, you can manage them using various Docker commands.
To list all the images on your system, you can use the docker images
command:
docker images
To remove an image, you can use the docker rmi
command followed by the image ID or tag:
docker rmi myapp
If the image is currently being used by a running container, you will need to stop and remove the container before removing the image.
Running and Managing Docker Containers
Running and managing Docker containers is at the core of containerization. Docker provides a simple and efficient way to create, run, and manage containers. In this chapter, we will learn the basic commands and techniques to run and manage Docker containers effectively.
Running a Docker Container
To run a Docker container, you need to use the docker run
command followed by the name of the image you want to run. For example, to run a container based on the official Ubuntu image, you can use the following command:
docker run ubuntu
This command will pull the Ubuntu image from the Docker Hub if it doesn’t exist locally and start a new container based on that image. By default, Docker will run the container in the foreground and attach the console to its standard input, output, and error streams.
If you want to run a container in the background and detach the console, you can use the -d
or --detach
option:
docker run -d ubuntu
This will run the container in the background, and you can later attach to it using the docker attach
command.
Related Article: Tutorial: Building a Laravel 9 Real Estate Listing App
Managing Docker Containers
Docker provides several commands to manage containers. Here are some of the most commonly used ones:
– docker ps
: Lists all the running containers.
– docker ps -a
: Lists all the containers, including the stopped ones.
– docker start
: Starts a stopped container.
– docker stop
: Stops a running container.
– docker restart
: Restarts a running container.
– docker attach
: Attaches the console to a running container.
– docker rm
: Removes a stopped container.
– docker rm -f
: Forces the removal of a running container.
You can also use the container ID instead of the container name in the above commands.
Working with Container Logs
Container logs are essential for troubleshooting and debugging. Docker provides the docker logs
command to retrieve the logs of a container. Here’s an example:
docker logs
This command will display the logs of the specified container. You can also use the -f
or --follow
option to continuously stream the logs.
Copying Files to and from Containers
Sometimes, you may need to copy files to or from a running container. Docker provides the docker cp
command for this purpose. Here’s how you can copy a file from a container to your local machine:
docker cp :
And to copy a file from your local machine to a container:
docker cp :
Make sure to specify the correct container name, file paths, and paths on your local machine.
Related Article: How to Force Docker for a Clean Build of an Image
Using Docker Compose for Multi-Container Applications
Docker Compose is a powerful tool that allows you to define and manage multi-container applications. With Compose, you can easily define the services, networks, and volumes required for your application in a single YAML file.
To get started with Docker Compose, you’ll need to have it installed on your machine. If you haven’t installed Docker Compose yet, you can follow the official installation guide for your platform on the Docker website.
Once you have Docker Compose installed, you can start using it to manage your multi-container applications. Let’s take a look at how to define a simple multi-container application using Compose.
Create a new file called docker-compose.yml
in the root directory of your project. This file will contain the configuration for your multi-container application. In this example, we’ll create a basic web application that consists of a web server and a database.
version: '3' services: web: build: . ports: - 8080:80 db: image: mysql:5.7 environment: - MYSQL_ROOT_PASSWORD=password - MYSQL_DATABASE=myapp
In the above example, we define two services: web
and db
. The web
service builds an image using the Dockerfile located in the current directory and exposes port 80 of the container to port 8080 on the host machine. The db
service uses the official MySQL 5.7 image and sets the root password and database name as environment variables.
To start the multi-container application, navigate to the directory containing the docker-compose.yml
file and run the following command:
docker-compose up
This command will start the containers defined in the docker-compose.yml
file and display their logs in the console. You can also use the -d
flag to run the containers in detached mode, which will keep them running in the background.
To stop and remove the containers, you can use the following command:
docker-compose down
Docker Compose also provides additional features that allow you to scale your services, configure networks, and manage volumes. You can refer to the official Docker Compose documentation for more details on these features.
Using Docker Compose for multi-container applications simplifies the deployment and management process. It provides a declarative way to define and run complex applications with multiple services. With just a single command, you can easily spin up your entire application stack.
In the next chapter, we will explore how to use Docker Compose to manage environments and configurations effectively.
Containerizing Your Existing Applications
Containerization offers a great way to modernize and optimize your existing applications by encapsulating them into lightweight, portable containers. This approach allows you to easily deploy and manage your applications across different environments, without worrying about the underlying infrastructure.
To containerize your existing applications, you’ll need to follow a few steps:
Step 1: Understand Your Application
Before containerizing your application, it’s important to understand its requirements and dependencies. Identify the components and libraries your application relies on, as well as any specific configurations it requires to run properly.
Related Article: How to Stop and Remove All Docker Containers
Step 2: Create a Dockerfile
A Dockerfile is a text file that defines the instructions to build a Docker image for your application. It specifies the base image, copies your application code into the container, and sets up any necessary dependencies and configurations. Here’s a basic example for a Node.js application:
# Use the official Node.js image as the base FROM node:14 # Set the working directory inside the container WORKDIR /usr/src/app # Copy package.json and package-lock.json COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code COPY . . # Specify the command to run the application CMD [ "npm", "start" ]
Step 3: Build the Docker Image
Once you have your Dockerfile, you can build the Docker image using the docker build
command. Make sure you are in the same directory as your Dockerfile and run the following command:
docker build -t your-image-name .
This command builds the Docker image and tags it with the given name (your-image-name
in this example). The .
at the end of the command specifies the build context, which is the directory containing the Dockerfile.
Step 4: Run the Containerized Application
With the Docker image built, you can now run your containerized application using the docker run
command. Here’s an example for running a containerized Node.js application:
docker run -p 8080:8080 your-image-name
This command starts a container based on the specified image (your-image-name
), maps port 8080 from the container to port 8080 on the host machine, and runs the application.
Related Article: How to Use Environment Variables in Docker Compose
Step 5: Test and Iterate
Once your application is running inside a container, it’s important to test it thoroughly to ensure everything is working as expected. If any issues arise, you can iterate on the Dockerfile and rebuild the image to make necessary changes.
Containerizing your existing applications offers numerous benefits, such as improved scalability, faster deployments, and easier maintenance. By following the steps outlined above, you can simplify the process and unlock the full potential of containerization.
For more detailed information about Docker and containerization, refer to the official Docker documentation.
Docker Networking and Linking Containers
Docker allows you to create and manage networks for your containers, enabling them to communicate with each other and with the outside world. In this chapter, we will explore Docker networking and how to link containers together.
Types of Networks
Docker provides three types of networks: bridge, host, and overlay.
The bridge network is the default network created when you run Docker. It enables containers to communicate with each other using IP addresses. By default, containers on the bridge network can communicate with each other, but not with the host machine or other networks.
The host network allows containers to use the network stack of the host machine. This means that containers on the host network share the same network interfaces as the host machine, enabling direct communication with the host and other containers on the host network.
The overlay network is used for connecting multiple Docker daemons together in a swarm. It enables containers to communicate across multiple hosts, even if they are running on different physical or virtual machines.
Related Article: Copying a Directory to Another Using the Docker Add Command
Linking Containers
Linking containers allows you to establish a secure connection between them, enabling them to communicate with each other. When you link containers, Docker sets up an encrypted tunnel between them and updates the environment variables of the linked containers with the necessary connection information.
To link two containers, you can use the –link option when running a container. Here’s an example:
docker run --name container1 --link container2:mysql -d image1
In this example, we are linking container1
with container2
and exposing the MySQL port of container2
to container1
.
After linking, Docker sets environment variables in container1
that contain the connection information for container2
. You can access these variables in your application code to establish a connection with the linked container.
It’s important to note that linking containers is considered a legacy feature in Docker. It is recommended to use user-defined networks instead, as they provide better isolation and flexibility.
User-Defined Networks
User-defined networks allow you to create custom networks for your containers, providing better control over the network configuration. You can create a user-defined network using the docker network create
command.
Here’s an example of creating a user-defined network:
docker network create mynetwork
Once you have created a user-defined network, you can start containers on that network using the --network
option.
docker run --name container1 --network mynetwork -d image1
By default, containers on the same user-defined network can communicate with each other using their container names. You can also specify a custom network IP address for a container using the --ip
option.
User-defined networks provide better isolation and control over your containerized applications. They allow you to define network policies and secure communication between containers.
Docker networking is a powerful feature that enables seamless communication between containers, making it easier to build and manage complex applications. By understanding Docker networking, you can simplify the process of containerization and ensure smooth operation of your containerized applications.
Managing Data in Docker Containers
One of the key considerations when working with Docker containers is managing data. Containers are ephemeral by nature, meaning that any data stored inside them is typically lost when the container is stopped or destroyed. However, Docker provides several mechanisms to manage data and ensure its persistence. In this section, we will explore some of these data management techniques.
Related Article: How to Use the Host Network in Docker Compose
Volumes
Volumes are the preferred way to manage persistent data in Docker containers. A volume is a directory that exists outside the container’s filesystem, which can be used to store and share data between containers or between a container and the host system.
To create a volume, you can use the docker volume create
command:
$ docker volume create myvolume
Once the volume is created, you can mount it to a container using the -v
or --mount
flag:
$ docker run -d -v myvolume:/data myimage
This command mounts the myvolume
volume to the /data
directory inside the container.
Volumes can also be specified in a Docker Compose file:
version: "3" services: myservice: image: myimage volumes: - myvolume:/data volumes: myvolume:
Bind Mounts
Bind mounts provide a way to mount a directory on the host system into a container. Unlike volumes, bind mounts can be used to access data that already exists on the host, making them a convenient option for development or debugging purposes.
To create a bind mount, you need to specify the source directory on the host and the target directory inside the container when running the container:
$ docker run -d -v /host/data:/container/data myimage
This command mounts the /host/data
directory on the host to the /container/data
directory inside the container.
tmpfs Mounts
tmpfs mounts are mounts that exist only in memory and are not persisted to the host system or any other storage medium. They can be useful for storing temporary or sensitive data that you don’t want to persist.
To create a tmpfs mount, you can use the --tmpfs
flag when running the container:
$ docker run -d --tmpfs /data myimage
This command creates a tmpfs mount at the /data
directory inside the container.
Related Article: How to Mount a Host Directory as a Volume in Docker Compose
Persistent Storage with Plugins and Drivers
Docker also provides plugins and drivers that allow you to use external storage systems for persistent data. These plugins and drivers extend Docker’s capabilities and provide additional options for managing data in containers.
Some popular storage plugins and drivers include:
– Docker Volume Plugins: These plugins enable the use of external storage systems like Amazon Web Services (AWS) Elastic Block Store (EBS) or Google Cloud Persistent Disk as volumes in Docker containers.
– Docker Storage Drivers: These drivers enable the use of different storage backends, such as overlay2, aufs, or btrfs, to store container data.
To use a storage plugin or driver, you need to install and configure it according to the plugin’s or driver’s documentation.
Scaling and Load Balancing with Docker Swarm
Docker Swarm is a native clustering and orchestration solution for Docker containers. It allows you to create and manage a swarm of Docker nodes, which can be scaled horizontally to distribute the workload across multiple containers. Swarm also provides built-in load balancing capabilities, ensuring that traffic is evenly distributed among the containers in the swarm.
To get started with scaling and load balancing in Docker Swarm, you first need to initialize a swarm. This can be done by running the following command:
docker swarm init
This command will initialize a new swarm and make the current node the swarm manager. Once the swarm is initialized, you can join other nodes to the swarm by running the command displayed in the output of the docker swarm init
command on the other nodes.
To scale a service in Docker Swarm, you can use the docker service scale
command followed by the name of the service and the desired number of replicas. For example, to scale a service named web
to have 5 replicas, you can run the following command:
docker service scale web=5
This command will scale the web
service to have 5 replicas, distributing the workload across the swarm nodes.
Docker Swarm also provides built-in load balancing capabilities. When you create a service in Docker Swarm, it automatically assigns a Virtual IP (VIP) to the service. This VIP acts as a single entry point for accessing the service, and all requests to the VIP are automatically load balanced across the containers running the service.
To access a service in Docker Swarm, you can use the VIP assigned to the service. For example, if you have a service named web
running on port 80, you can access it using the VIP assigned to the service:
http://:80
Docker Swarm uses a routing mesh to distribute incoming requests to the containers running the service. The routing mesh automatically routes traffic to the appropriate container based on the service’s VIP and the published ports of the containers.
In addition to the built-in load balancing capabilities, Docker Swarm also supports external load balancers. You can use an external load balancer, such as NGINX or HAProxy, to distribute traffic to the containers in the swarm. To do this, you would configure the load balancer to forward incoming requests to the swarm nodes based on the published ports of the containers.
Scaling and load balancing with Docker Swarm allows you to easily distribute the workload across multiple containers, ensuring high availability and efficient resource utilization. With the built-in load balancing capabilities and support for external load balancers, you have flexibility in how you distribute traffic to your containers.
To learn more about scaling and load balancing with Docker Swarm, you can refer to the official Docker documentation on Docker Swarm.
Securing Docker Containers
Docker provides a secure environment for running and isolating applications, but it’s important to take additional measures to ensure the security of your Docker containers. In this section, we will explore some best practices for securing Docker containers.
1. Use Official Images: When building your Docker images, it is recommended to use official images from trusted sources, such as the Docker Hub. Official images are maintained and regularly updated by the Docker community, ensuring the latest security patches and bug fixes.
2. Update Regularly: It is crucial to keep your Docker installation and containers up to date with the latest security patches. Docker regularly releases updates that address vulnerabilities and improve security. To update Docker, run the following command:
$ docker update
3. Limit Container Capabilities: By default, Docker containers have access to all the capabilities of the host system. To enhance security, it is advisable to restrict container capabilities to only what is necessary for the application to function. You can do this by using the --cap-drop
and --cap-add
flags when running containers.
For example, to drop the SYS_ADMIN
capability from a container, use the following command:
$ docker run --cap-drop=SYS_ADMIN mycontainer
4. Use Appropriate User Permissions: To minimize potential security risks, it is recommended to run containers using non-root users whenever possible. Running containers as non-root users can limit the impact of any potential container breakout.
To specify a non-root user within a Dockerfile, use the USER
instruction:
FROM ubuntu USER myuser
5. Implement Network Segmentation: Docker containers communicate with each other using networks. To enhance security, it is advisable to implement network segmentation by creating separate networks for different applications or services. This helps isolate container communication and prevents unauthorized access.
You can create a new network using the following command:
$ docker network create mynetwork
6. Enable Content Trust: Docker Content Trust (DCT) ensures the integrity and authenticity of Docker images. By enabling content trust, you can prevent the execution of potentially malicious or tampered images.
To enable content trust globally, set the DOCKER_CONTENT_TRUST
environment variable:
$ export DOCKER_CONTENT_TRUST=1
7. Monitor Container Activity: Regularly monitoring container activity can help identify any suspicious or unauthorized behavior. Docker provides several tools, such as Docker logs and Docker events, to monitor container activity and investigate any security incidents.
For example, to view the logs of a running container, use the following command:
$ docker logs mycontainer
By following these best practices, you can significantly enhance the security of your Docker containers and minimize the risk of potential security breaches.
Related Article: How To List Containers In Docker
Monitoring and Logging Docker Containers
Monitoring and logging are crucial aspects of managing Docker containers. They help you gain insights into the performance, availability, and behavior of your containers. In this chapter, we will explore some tools and techniques to effectively monitor and log Docker containers.
1. Docker Stats
Docker provides a built-in command called docker stats
that allows you to monitor the resource usage of your running containers. It provides real-time information about CPU, memory, network I/O, and disk I/O usage. This can be particularly useful when debugging performance issues or optimizing resource allocation.
To use docker stats
, simply run the command followed by the name or ID of the container you want to monitor:
docker stats
2. cAdvisor
cAdvisor (Container Advisor) is an open-source tool developed by Google that provides detailed information about the resource usage and performance characteristics of running containers. It automatically collects and analyzes metrics such as CPU usage, memory consumption, and network statistics.
To use cAdvisor, you can deploy it as a separate container using the following command:
docker run -d --name=cadvisor --volume=/:/rootfs:ro --volume=/var/run:/var/run:rw --volume=/sys:/sys:ro --volume=/var/lib/docker/:/var/lib/docker:ro --publish=8080:8080 --detach=true google/cadvisor:latest
Once cAdvisor is running, you can access its web interface by navigating to http://localhost:8080
in your web browser. From there, you can monitor the resource usage of your containers and view historical data.
Related Article: Installing Docker on Ubuntu in No Time: a Step-by-Step Guide
3. ELK Stack
The ELK Stack (Elasticsearch, Logstash, and Kibana) is a popular combination of open-source tools for centralized logging and log analysis. It provides a scalable and flexible solution to collect, index, and visualize log data from Docker containers.
To set up the ELK Stack, you can follow the official documentation provided by Elastic. Once configured, you can configure Docker to send logs to Logstash using the --log-driver
flag. For example:
docker run --log-driver=syslog --log-opt syslog-address=udp://:
You can then use Kibana to search, filter, and visualize the log data stored in Elasticsearch.
4. Prometheus and Grafana
Prometheus is an open-source monitoring system that collects and stores time-series data. Grafana is a visualization tool that works well with Prometheus and allows you to create custom dashboards for monitoring Docker containers.
To set up Prometheus and Grafana, you can follow their respective documentation. Once set up, you can configure Prometheus to scrape metrics from Docker containers using the cadvisor
exporter. Grafana can then be used to create visualizations and set up alerts based on the collected metrics.
These are just a few examples of the many monitoring and logging tools available for Docker containers. Depending on your specific requirements, you may explore other tools such as Datadog, Sysdig, or the Docker Enterprise Edition.
Monitoring and logging your Docker containers allows you to gain valuable insights into their behavior, troubleshoot issues, and optimize resource usage. By leveraging the right tools and techniques, you can simplify the management of your containerized applications.
Optimizing Docker Performance
Docker is a powerful tool for containerization, but like any technology, it can benefit from optimization to improve performance. In this chapter, we will explore some techniques to optimize Docker performance and make your containerized applications run faster and more efficiently.
Related Article: How to Pass Environment Variables to Docker Containers
1. Use Lightweight Base Images
One way to optimize Docker performance is by using lightweight base images. The base image forms the foundation of your Docker containers, and using smaller base images can reduce the overall size and start-up time of your containers. For example, instead of using a general-purpose Linux distribution as your base image, you can use specialized images like Alpine Linux, which is known for its small size and fast boot time.
To specify the base image in your Dockerfile, use the FROM
directive followed by the image name and version tag. Here’s an example using the Alpine Linux base image:
FROM alpine:3.14
2. Minimize Layers
Docker images are built using layers, and each layer adds to the overall size of the image. To optimize Docker performance, it’s important to minimize the number of layers in your images. This can be achieved by combining multiple commands into a single RUN
instruction in your Dockerfile. For example:
RUN apt-get update && apt-get install -y \ package1 \ package2 \ package3 \ && rm -rf /var/lib/apt/lists/*
By chaining the commands together with &&
, you can reduce the number of layers created during the build process.
3. Utilize Caching
Docker provides a caching mechanism that can significantly speed up the build process. When you build an image, Docker caches the intermediate layers and only rebuilds the layers that have changed. This can greatly reduce the time required for subsequent builds.
To take advantage of caching, order your Dockerfile instructions from least likely to change to most likely to change. For example, install dependencies first, then copy application code. This way, if you make changes to your code, Docker can reuse the previously built layers up until the point where the code is copied.
Related Article: How to Copy Files From Host to Docker Container
4. Optimize Volumes
Volumes in Docker allow you to persist data outside of the container’s writable layer. While volumes are convenient for data persistence, they can impact performance if not used properly.
To optimize volume performance, consider using bind mounts instead of named volumes for development environments. Bind mounts directly map a host directory into the container, eliminating the overhead of the Docker volume system. However, named volumes are still recommended for production environments where data persistence and portability are crucial.
5. Resource Allocation
Docker containers run within a host environment, and optimizing resource allocation can improve performance. You can specify the amount of CPU and memory resources allocated to a container using Docker’s resource constraints.
For example, to limit a container to use only one CPU core and 512MB of memory, you can use the --cpus
and --memory
flags when running the container:
docker run --cpus=1 --memory=512m my-container
By appropriately allocating resources, you can prevent containers from consuming excessive resources and improve the overall performance of your Docker environment.
6. Monitor and Tune
Lastly, it’s important to monitor your Docker environment and fine-tune its performance as needed. Docker provides various tools and commands to monitor container and host resource usage. These include docker stats
, docker top
, and docker system df
, among others.
Additionally, you can use container orchestration tools like Kubernetes or Docker Swarm to automatically manage and optimize the performance of your Dockerized applications.
By regularly monitoring and fine-tuning your Docker environment, you can identify and resolve performance bottlenecks, ensuring that your containerized applications are running at their best.
In the next chapter, we will explore advanced Docker networking concepts. Stay tuned!
Related Article: How to Use Docker Exec for Container Commands
Docker in Production: Best Practices and Tips
Docker has become a popular choice for containerization in production environments due to its ease of use and scalability. However, there are certain best practices and tips that can help ensure a smooth deployment and operation of Docker containers in a production setting. In this chapter, we will explore some of these practices and tips.
1. Use a Lightweight Base Image: When creating Docker images, it is important to start with a lightweight base image. This helps reduce the overall size of the image and improves the container’s performance. Alpine Linux is a popular choice for a lightweight base image as it is minimalistic and has a small footprint.
2. Optimize Docker Images: Docker images should be optimized to minimize their size and improve container startup times. This can be achieved by using multi-stage builds, where the build environment is separate from the runtime environment. Additionally, removing unnecessary files and dependencies from the image can further reduce its size.
3. Limit Container Capabilities: Containers should be run with the least privileges necessary to perform their intended tasks. By limiting container capabilities, you reduce the attack surface and minimize the impact of any potential security vulnerabilities. The Docker --cap-drop
flag can be used to drop specific capabilities when starting a container.
4. Implement Resource Constraints: To prevent containers from consuming excessive resources and impacting the performance of other containers, it is important to implement resource constraints. Docker provides various options for limiting CPU, memory, and I/O usage of containers. For example, the --cpu-shares
flag can be used to allocate CPU shares to containers.
5. Monitor Docker Containers: Monitoring is crucial in a production environment to identify any performance issues or abnormalities. Docker provides built-in monitoring tools such as the Docker Stats API and the docker stats
command. Additionally, there are third-party monitoring tools like Prometheus and Grafana that can be integrated with Docker for more advanced monitoring and visualization.
6. Implement Container Orchestration: When running Docker containers in a production environment, it is often necessary to manage multiple containers across multiple hosts. Container orchestration platforms like Kubernetes and Docker Swarm can help automate the deployment, scaling, and management of containers. These platforms provide features such as service discovery, load balancing, and automatic container recovery.
7. Implement High Availability: To ensure high availability of Docker containers, it is important to deploy containers across multiple hosts and implement mechanisms for automatic container recovery. Container orchestration platforms like Kubernetes and Docker Swarm provide built-in features for high availability, such as replication and automatic failover.
8. Implement Security Best Practices: Docker containers should be secured following best practices to protect against potential vulnerabilities or attacks. Some recommended security practices include using secure base images, keeping containers up to date with security patches, and implementing network segmentation to isolate containers.
By following these best practices and tips, you can ensure a smooth and secure operation of Docker containers in a production environment. Remember to regularly update and monitor your containers to stay on top of any potential issues or security vulnerabilities.
Next, we will explore some advanced Docker networking concepts and techniques.
Real World Examples of Docker in Action
Docker has become an essential tool for many developers and system administrators due to its ability to simplify the process of containerization. It allows for easy packaging and deployment of applications, ensuring consistency across different environments. In this chapter, we will explore some real-world examples of Docker in action and how it can be used to solve common problems.
Example 1: Web Application Development
One of the most common use cases for Docker is in web application development. Docker allows developers to package their applications and all their dependencies into containers, ensuring that the application will run consistently across different development machines. This eliminates the dreaded “it works on my machine” problem.
Let’s take a look at a basic example of a Dockerfile for a Node.js web application:
# Specify the base image FROM node:14 # Set the working directory WORKDIR /app # Copy package.json and package-lock.json COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code COPY . . # Expose the port EXPOSE 3000 # Define the command to run the application CMD [ "npm", "start" ]
By building an image from this Dockerfile, developers can easily spin up containers running their web application, regardless of the underlying host system. This makes it much easier to collaborate with other developers and ensures that the application will work the same way in different environments.
Example 2: Continuous Integration and Deployment
Docker is also widely used in the world of continuous integration and deployment (CI/CD). By packaging applications into containers, developers can easily create reproducible build and deployment environments. This allows for seamless integration with popular CI/CD tools like Jenkins, Travis CI, and GitLab CI/CD.
Here’s an example of a Jenkins pipeline script that uses Docker to build and deploy a web application:
pipeline { agent any stages { stage('Build') { steps { sh 'docker build -t myapp .' } } stage('Test') { steps { sh 'docker run myapp npm test' } } stage('Deploy') { steps { sh 'docker push myapp:latest' sh 'kubectl apply -f deployment.yaml' } } } }
In this example, the pipeline runs various stages, including building the Docker image, running tests inside a container, and deploying the application using Kubernetes. Docker provides a consistent and isolated environment for each stage, ensuring that the build and deployment process remains reliable and reproducible.
Example 3: Microservices Architecture
Docker is particularly well-suited for implementing a microservices architecture. By packaging each microservice into a separate container, developers can easily scale, update, and manage individual components of their application independently.
Let’s consider a scenario where we have a microservices-based e-commerce application. Each microservice, such as the product catalog, user authentication, and payment processing, can be packaged into its own container. This allows for easy scaling of individual services based on demand and seamless deployment of new versions without impacting the entire application.
version: '3' services: catalog: build: context: ./catalog ports: - 8000:8000 depends_on: - database auth: build: context: ./auth ports: - 8001:8001 depends_on: - database payment: build: context: ./payment ports: - 8002:8002 depends_on: - database database: image: postgres:latest environment: - POSTGRES_USER=myuser - POSTGRES_PASSWORD=mypassword
In this example, each microservice is defined as a separate service in a docker-compose.yml
file. The dependencies between services are specified using the depends_on
directive. This allows for easy management and deployment of the entire microservices-based application.
These are just a few examples of how Docker can be used in real-world scenarios. Its flexibility and ease of use make it a powerful tool for simplifying containerization and improving the overall development and deployment process. With Docker, developers can focus more on writing code and less on managing complex infrastructure setups.