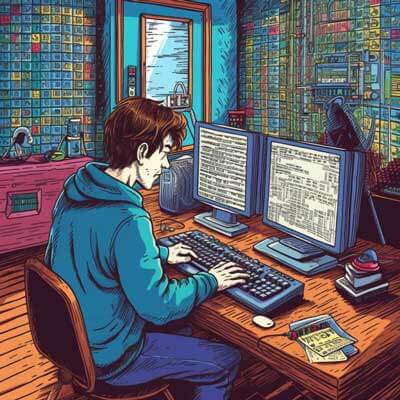
- Chapter 1: Introduction to Exec in Container Environment
- Example 1: Executing a Command in a Docker Container
- Example 2: Executing a Command with Interactive Shell
- Chapter 2: Setting Up the Environment
- Example 1: Creating and Running a Docker Container
- Example 2: Verifying Container Status
- Chapter 3: Basic Syntax and Parameters for Exec
- Example 1: Executing a Command with Different User
- Example 2: Executing a Command in a Detached Mode
Chapter 1: Introduction to Exec in Container Environment
In a containerized environment, executing commands within a running Docker container is a common requirement. Docker provides the docker exec
command to facilitate this task. With docker exec
, you can run commands inside a container without the need to start a new shell session. This allows for convenient interaction with and management of running containers.
Related Article: Build a Movie Search App with GraphQL, Node & TypeScript
Example 1: Executing a Command in a Docker Container
To execute a command within a Docker container, use the following syntax:
docker exec [OPTIONS] CONTAINER COMMAND [ARG...]
Where:
– OPTIONS
are additional options for the docker exec
command.
– CONTAINER
is the name or ID of the container in which the command will be executed.
– COMMAND
is the command to be executed.
– ARG...
are optional arguments to be passed to the command.
For example, to execute the command ls -l
inside a container named “my-container”, run the following command:
docker exec my-container ls -l
This will execute the ls -l
command within the specified container, displaying a detailed list of files and directories.
Example 2: Executing a Command with Interactive Shell
You can also open an interactive shell within a Docker container using docker exec
. This allows you to run multiple commands or perform interactive tasks within the container.
To open an interactive shell, add the -it
options to the docker exec
command:
docker exec -it CONTAINER /bin/bash
Replace CONTAINER
with the name or ID of the target container. This command will start an interactive shell session within the container, giving you full access to run commands and interact with the container’s environment.
Chapter 2: Setting Up the Environment
Before using docker exec
, it is crucial to set up the environment properly to ensure smooth execution of commands within Docker containers.
Related Article: Tutorial: Managing Docker Secrets
Example 1: Creating and Running a Docker Container
To demonstrate the usage of docker exec
, let’s first create and run a simple Docker container.
Create a file named “Dockerfile” with the following content:
FROM ubuntu:latest CMD tail -f /dev/null
This Dockerfile specifies an Ubuntu-based image and sets the command to tail -f /dev/null
, which keeps the container running indefinitely.
Build the Docker image using the following command:
docker build -t my-container .
Once the image is built, run the container:
docker run -d --name my-container my-container
The container will now be running in the background.
Example 2: Verifying Container Status
To verify the status of the container, use the docker ps
command:
docker ps
This command will display a list of running containers, including the newly created “my-container” container. Ensure that the container is running before proceeding to the next steps.
Now that the environment is set up, we can explore the different aspects of using docker exec
for various purposes.
Chapter 3: Basic Syntax and Parameters for Exec
Understanding the basic syntax and available parameters for docker exec
is essential for successfully executing commands within Docker containers.
Related Article: Build a Chat Web App with Flask, MongoDB, Reactjs & Docker
Example 1: Executing a Command with Different User
You can specify a user to execute the command as within the container using the --user
option. This can be useful when user permissions need to be considered.
To execute a command as a different user, use the following syntax:
docker exec --user USER CONTAINER COMMAND
Replace USER
with the desired username or UID, CONTAINER
with the container name or ID, and COMMAND
with the desired command.
For example, to execute the command whoami
as the root user within the “my-container” container, run the following command:
docker exec --user root my-container whoami
This will execute the whoami
command as the root user and display the output.
Example 2: Executing a Command in a Detached Mode
By default, docker exec
attaches the command’s standard input, output, and error streams to the current terminal session. However, you can run the command in a detached mode using the -d
option.
To execute a command in detached mode, use the following syntax:
docker exec -d CONTAINER COMMAND
Replace CONTAINER
with the name or ID of the container and COMMAND
with the desired command.
For example, to execute the command echo "Hello, world!"
within the “my-container” container in detached mode, run the following command:
docker exec -d my-container echo "Hello, world!"
This will execute the echo
command in detached mode, and the output will not be displayed in the current terminal session.
Continue to the next chapters to explore different use cases, best practices, performance considerations, advanced techniques, code snippet ideas, and error handling related to using docker exec
in a container environment.