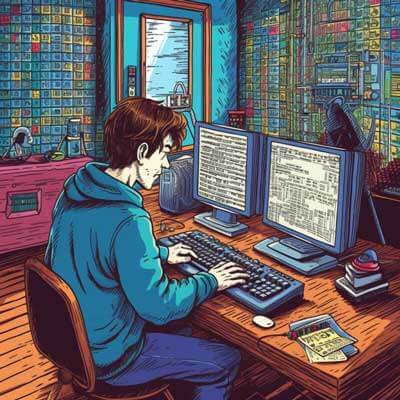
Table of Contents
Installing Docker CLI
To get started with Docker CLI, you first need to install Docker on your system. Docker provides installation packages for various operating systems, such as Windows, macOS, and Linux. In this section, we will walk through the installation process for each of these operating systems.
Windows:
1. Visit the official Docker website at https://www.docker.com/products/docker-desktop.
2. Click on the "Download Docker Desktop" button.
3. Once the installer is downloaded, double-click on it to start the installation process.
4. Follow the on-screen instructions to complete the installation.
5. After the installation is complete, Docker Desktop will be running, and you will be able to access Docker CLI from the command prompt or PowerShell.
macOS:
1. Visit the official Docker website at https://www.docker.com/products/docker-desktop.
2. Click on the "Download Docker Desktop for Mac" button.
3. Once the installer is downloaded, double-click on it to start the installation process.
4. Drag the Docker.app file to the Applications folder to complete the installation.
5. Launch Docker Desktop from the Applications folder.
6. After Docker Desktop is running, you will be able to access Docker CLI from the Terminal.
Linux:
The installation process for Docker CLI on Linux may vary depending on the distribution you are using. Docker provides installation instructions for various Linux distributions on their website. You can find the installation instructions for your specific distribution at https://docs.docker.com/engine/install/.
Once Docker is installed on your system, you can verify the installation by opening a command prompt or terminal and running the following command:
docker version
If Docker is installed correctly, you should see information about the Docker version and the API version.
Congratulations! You have successfully installed Docker CLI on your system. In the next section, we will explore some basic Docker CLI commands to get you started with Docker containers.
Related Article: How to Use the Host Network in Docker Compose
Basic Docker Commands
The Docker CLI (Command Line Interface) provides a set of commands that allow you to interact with Docker and manage containers, images, networks, and volumes. In this section, we will cover some of the most commonly used basic Docker commands to help you get started.
1. docker version
The docker version
command displays the version of Docker installed on your system, along with the version of the Docker client and server.
$ docker version
2. docker info
The docker info
command provides detailed information about the Docker installation, including the number of containers, images, networks, and volumes on your system.
$ docker info
3. docker run
The docker run
command is used to create and run a container based on a Docker image. It pulls the image from the Docker registry if it is not already available on your system.
$ docker run hello-world
This command will run the hello-world
image, which is a simple test image provided by Docker to verify that your installation is working correctly.
4. docker ps
The docker ps
command lists all the running containers on your system.
$ docker ps
If you want to see all containers, including the ones that are not currently running, you can use the -a
flag.
$ docker ps -a
5. docker images
The docker images
command lists all the Docker images available on your system.
$ docker images
6. docker pull
The docker pull
command is used to download Docker images from a Docker registry.
$ docker pull nginx
This command will pull the latest version of the nginx
image from the Docker Hub registry.
7. docker stop
The docker stop
command is used to stop a running container.
$ docker stop container_name_or_id
Replace container_name_or_id
with the name or ID of the container you want to stop.
8. docker rm
The docker rm
command is used to remove one or more containers from your system.
$ docker rm container_name_or_id
Replace container_name_or_id
with the name or ID of the container you want to remove.
9. docker rmi
The docker rmi
command is used to remove one or more Docker images from your system.
$ docker rmi image_name_or_id
Replace image_name_or_id
with the name or ID of the image you want to remove.
These are just a few of the basic Docker commands that you will frequently use. The Docker CLI provides many more commands and options for managing your containers, images, networks, and volumes. To learn more about Docker commands, you can refer to the official Docker documentation(source).
Managing Containers
Once you have created containers using Docker, you will need to manage and interact with them. This section will cover various Docker CLI commands that will help you manage your containers efficiently.
List Containers
To list all the containers on your system, you can use the docker ps
command. By default, this command only displays running containers. If you also want to see stopped containers, you can use the docker ps -a
command.
$ docker ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 12a34b56c78d nginx:latest "nginx -g…" 3 hours ago Up 2 hours 80/tcp mynginx $ docker ps -a CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 12a34b56c78d nginx:latest "nginx -g…" 3 hours ago Up 2 hours 80/tcp mynginx 987zyx654wvu mysql:latest "docker-e…" 6 days ago Exited (0) 5 days 3306/tcp mymysql
Related Article: How to Use Environment Variables in Docker Compose
Start and Stop Containers
To start a stopped container, you can use the docker start
command followed by the container name or ID.
$ docker start mymysql mymysql $ docker ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 12a34b56c78d nginx:latest "nginx -g…" 3 hours ago Up 2 hours 80/tcp mynginx 987zyx654wvu mysql:latest "docker-e…" 6 days ago Up 10 seconds 3306/tcp mymysql
To stop a running container, you can use the docker stop
command followed by the container name or ID.
$ docker stop mynginx mynginx $ docker ps CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 987zyx654wvu mysql:latest "docker-e…" 6 days ago Up 3 minutes 3306/tcp mymysql
Delete Containers
To delete a container, you can use the docker rm
command followed by the container name or ID. Note that you cannot delete a running container, so make sure to stop it first if necessary.
$ docker rm mynginx mynginx $ docker ps -a CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 987zyx654wvu mysql:latest "docker-e…" 6 days ago Up 5 minutes 3306/tcp mymysql
Inspect Containers
To get detailed information about a container, you can use the docker inspect
command followed by the container name or ID.
$ docker inspect mymysql [ { "Id": "987zyx654wvuts9876", "Created": "2021-01-01T12:34:56.000Z", "State": { "Status": "running", "Running": true, "Paused": false, "Restarting": false, ... }, "Config": { "Image": "mysql:latest", "Cmd": [ "docker-entrypoint.sh", "mysqld" ], ... }, ... } ]
Rename Containers
To rename a container, you can use the docker rename
command followed by the current container name and the new name.
$ docker rename mymysql newmysql $ docker ps -a CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 987zyx654wvu mysql:latest "docker-e…" 6 days ago Up 10 minutes 3306/tcp newmysql
Managing containers is an essential part of working with Docker. By using the Docker CLI commands mentioned in this chapter, you can easily list, start, stop, delete, inspect, and rename containers as needed.
Related Article: How to Use Docker Exec for Container Commands
Working with Images
In Docker, an image is a lightweight, standalone, and executable package that includes everything needed to run a piece of software, including the code, a runtime, libraries, environment variables, and config files. Images are the building blocks of Docker containers.
To work with images in Docker, you can use the Docker CLI (Command Line Interface). The Docker CLI provides a set of commands that you can use to manage images, such as pulling, building, listing, and removing images.
Pulling Images
To pull an image from a remote registry, you can use the docker pull
command followed by the image name and optionally the tag. For example, to pull the latest version of the official Ubuntu image, you can run:
docker pull ubuntu
If you want to pull a specific version of an image, you can specify the tag as follows:
docker pull ubuntu:18.04
Listing Images
To list the images available on your local machine, you can use the docker images
command. This command will display a list of images along with their repository, tag, image ID, and size. For example:
docker images
Building Images
To build your own Docker image, you need to create a Dockerfile that contains instructions for building the image. The Dockerfile is a text file that specifies the base image, any additional dependencies or packages, environment variables, and commands to run when the container starts.
Once you have created the Dockerfile, you can use the docker build
command to build the image. The command takes the path to the directory containing the Dockerfile as an argument. For example:
docker build -t myimage:1.0 .
This command will build an image with the tag myimage:1.0
using the Dockerfile in the current directory (.
).
Related Article: How To Delete All Docker Images
Removing Images
To remove an image from your local machine, you can use the docker rmi
command followed by the image ID or tag. For example, to remove an image with the ID abc123
, you can run:
docker rmi abc123
If the image has multiple tags, you can specify the tag to remove a specific version of the image:
docker rmi myimage:1.0
If you want to remove all unused images, you can use the -f
flag:
docker image prune -a
This command will remove all unused images, including those that are not referenced by any containers.
Managing Networks
One of the key features of Docker is its ability to create and manage networks. Docker provides a powerful networking model that allows containers to communicate with each other and with the outside world. In this chapter, we will explore how to manage networks using Docker CLI.
List Networks
To view the list of networks available on your Docker host, you can use the following command:
docker network ls
This command will display a table with information about the networks, including their names, IDs, and driver types.
Create a Network
To create a new network, you can use the docker network create
command followed by the desired network name. By default, Docker creates networks with the bridge
driver.
docker network create mynetwork
This command will create a new network called mynetwork
using the bridge
driver. You can specify a different driver by using the --driver
option.
Related Article: How to Copy Files From Host to Docker Container
Connect Containers to a Network
To connect a container to a network, you can use the --network
option when running a container. For example, to start a container and connect it to the mynetwork
network, you can use the following command:
docker run --network mynetwork myimage
This will start a container using the myimage
image and connect it to the mynetwork
network. The container will be able to communicate with other containers on the same network.
Inspect a Network
To inspect the details of a network, such as its IP range and connected containers, you can use the docker network inspect
command followed by the network name or ID. For example:
docker network inspect mynetwork
This command will display detailed information about the mynetwork
network, including its subnet, gateway, and connected containers.
Remove a Network
To remove a network, you can use the docker network rm
command followed by the network name or ID. For example:
docker network rm mynetwork
This command will remove the mynetwork
network, including all its configurations and connected containers.
Volumes and Data Management
Docker provides a feature called volumes that allows you to manage the data within your containers. A volume is a directory that is stored outside the container's file system and is used to persist data even if the container is stopped or deleted.
Using volumes has several advantages. It allows you to separate the data from the container, making it easier to manage and backup. Volumes also enable you to share data between multiple containers.
There are two types of volumes in Docker: named volumes and bind mounts.
Named volumes are managed by Docker and are created and managed using the Docker CLI or API. They have a unique name and can be used across multiple containers. To create a named volume, you can use the docker volume create
command:
$ docker volume create myvolume
Once created, you can use the volume in your containers by specifying it in the docker <a href="/docker-how-to-workdir-run-cmd-env-variables/">run
command:
$ docker run -v myvolume:/path/in/container myimage
On the other hand, bind mounts are directories on the host machine that are mounted into a container. This allows you to directly access files on the host machine from within the container. To use a bind mount, you need to specify the source directory on the host and the target directory in the container in the docker run
command:
$ docker run -v /host/path:/container/path myimage
Volumes can also be used with Docker Compose, which is a tool for defining and running multi-container Docker applications. In a Docker Compose file, you can define volumes using the volumes
keyword. Here's an example:
version: '3' services: web: image: myimage volumes: - myvolume:/path/in/container - /host/path:/container/path volumes: myvolume:
In this example, we define a named volume called myvolume
and a bind mount from /host/path
to /container/path
. These volumes are then used by the web
service.
To manage volumes, Docker provides a set of commands, such as docker volume ls
to list the volumes, docker volume rm
to remove a volume, and docker volume prune
to remove all unused volumes.
Volumes are an essential part of managing data in Docker containers. They provide a way to persist data, share data between containers, and separate data from the container itself. By understanding how to use and manage volumes, you can effectively manage the data within your Docker environment.
Related Article: How to Stop and Remove All Docker Containers
Docker Compose: Simplifying Container Orchestration
Docker Compose is a powerful tool that simplifies the management and orchestration of multiple containers. It allows you to define and manage multi-container applications using a simple YAML file. With Docker Compose, you can easily spin up and tear down complex environments with just a single command.
Installing Docker Compose
Before we dive into using Docker Compose, let's make sure it is installed on your system. Docker Compose can be installed as a separate package, independent of the Docker engine. Here are the steps to install Docker Compose:
1. Visit the Docker Compose installation page: https://docs.docker.com/compose/install/
2. Choose the installation method suitable for your operating system.
3. Follow the instructions provided to complete the installation.
Once installed, you can verify the installation by running the following command:
docker-compose --version
If Docker Compose is successfully installed, you will see the version number displayed in the output.
Creating a Docker Compose File
To define and manage your multi-container applications, you need to create a Docker Compose file. This file is written in YAML format and specifies the services, networks, and volumes required for your application.
Let's create a simple Docker Compose file to run a web application along with a database. Create a new file called docker-compose.yml
and add the following content:
version: '3' services: web: image: nginx:latest ports: - 80:80 db: image: mysql:latest environment: - MYSQL_ROOT_PASSWORD=secret - MYSQL_DATABASE=mydb ports: - 3306:3306
In this example, we have defined two services: web
and db
. The web
service uses the nginx
image and exposes port 80. The db
service uses the mysql
image, sets environment variables for the root password and database name, and exposes port 3306.
Running Docker Compose
To start the containers defined in the Docker Compose file, navigate to the directory containing the docker-compose.yml
file and run the following command:
docker-compose up
This command will download the necessary images, create and start the containers. You will see the logs for each container displayed in the terminal. To run the containers in detached mode, use the -d
flag:
docker-compose up -d
To stop and remove the containers, networks, and volumes defined in the Docker Compose file, run the following command:
docker-compose down
Related Article: How to Mount a Host Directory as a Volume in Docker Compose
Working with Docker Compose
Docker Compose provides many other commands and options to manage your multi-container applications. Here are a few commonly used ones:
- docker-compose ps
: Display the status of the containers defined in the Docker Compose file.
- docker-compose logs
: View the logs for the containers.
- docker-compose exec
: Run a command inside a running container.
- docker-compose build
: Build or rebuild the images defined in the Docker Compose file.
- docker-compose restart
: Restart the containers.
- docker-compose scale
: Scale the number of containers for a service.
Refer to the Docker Compose documentation for more detailed information on these commands and other advanced options.
Docker Compose is a valuable tool for simplifying the management and orchestration of containerized applications. It allows you to define and manage complex environments with ease, making it an essential part of any Docker workflow.
Dockerfile: Building Custom Images
A Dockerfile is a text file that contains a set of instructions to build a Docker image. It provides a way to automate the creation of custom Docker images, allowing you to configure and package your application along with its dependencies.
To create a Docker image using a Dockerfile, you need to follow these steps:
1. Create a new file named Dockerfile
in your project directory.
2. Open the Dockerfile
in a text editor and start writing the instructions. Each instruction represents a step in the build process.
3. The first line of the Dockerfile should specify the base image to use. A base image is a pre-built image that forms the starting point for your custom image. For example, to use the official Node.js 14 image as the base, you can include the following line:
FROM node:14
4. Next, you can specify any additional dependencies or packages required by your application. For example, if your application needs to install some packages using the package manager apt-get
, you can include the following line:
RUN apt-get update && apt-get install -y
5. After installing any dependencies, you can copy your application code into the image using the COPY
instruction. For example, if your application code is in a directory named src
, you can include the following line:
COPY src /app
6. You can also set environment variables using the ENV
instruction. This allows you to configure your application with specific values. For example, to set the NODE_ENV
environment variable to production
, you can include the following line:
ENV NODE_ENV=production
7. Finally, you can specify the command to run when a container is started from the image using the CMD
instruction. This command is typically the entry point for your application. For example, to run a Node.js application, you can include the following line:
CMD ["node", "app.js"]
Once you have written the Dockerfile, you can build the Docker image using the following command:
docker build -t .
The -t
flag is used to specify the name and optionally a tag for the image. The .
at the end specifies the build context, which is the current directory.
After the build is complete, you can run a container from your custom image using the following command:
docker run
This will start a container based on your custom image and execute the command specified in the CMD
instruction.
Creating custom Docker images using Dockerfiles allows you to define the exact configuration and dependencies needed for your application. It also makes it easy to share and reproduce your environment with others.
For more information on Dockerfiles and their syntax, you can refer to the official Docker documentation on building Docker images.
Advanced Docker Networking
Docker provides powerful networking capabilities that allow you to manage and configure network settings for your containers. In this chapter, we will explore some advanced networking features that Docker offers.
Bridge Networking
By default, Docker uses bridge networking, which creates a virtual network interface on the host machine called docker0
. This virtual interface is used to connect containers to each other and to the external world. When you start a container, Docker assigns it an IP address within the bridge network.
To see the details of the bridge network, you can use the docker network inspect
command:
$ docker network inspect bridge
You can also create your own custom bridge networks using the docker network create
command:
$ docker network create my-network
This creates a new bridge network called my-network
. Containers connected to this network can communicate with each other using their container names as hostnames.
Related Article: How to Run a Docker Instance from a Dockerfile
Host Networking
In addition to bridge networking, Docker also provides the option to use host networking. With host networking, containers use the network stack of the host machine, bypassing Docker's network isolation. This can be useful when you need to access services running on the host machine or when you want to use a specific network interface.
To run a container with host networking, use the --network host
option:
$ docker run --network host my-image
This command runs the container with host networking enabled.
Overlay Networking
Overlay networking is a feature in Docker that allows you to create multi-host networks spanning across multiple Docker hosts. This is particularly useful in a distributed environment where containers need to communicate with each other across different hosts.
To create an overlay network, you can use the docker network create
command with the --driver overlay
option:
$ docker network create --driver overlay my-overlay-network
This creates a new overlay network called my-overlay-network
. You can then connect containers to this network using the --network
option when running the containers.
External Networking
Docker also supports external networking, which allows containers to directly connect to an external network interface on the host machine. This can be useful when you want to expose a container to the outside world or when you need to connect to a specific network interface.
To run a container with external networking, use the --network=host
option followed by the interface name:
$ docker run --network=host --name=my-container my-image
This command runs the container with external networking enabled, using the specified interface.
Container Security and Isolation
Container security is a critical aspect of using Docker and other containerization technologies. Containers provide isolation and control over the resources they use, but it is still important to take precautions to ensure the security of your containers and the data they contain.
Here are some best practices to consider for container security and isolation:
1. Use Official Images: When creating containers, it is recommended to use official images from trusted sources. Official images are maintained by the Docker community and are regularly updated to address security vulnerabilities. You can find official images on the Docker Hub (https://hub.docker.com/).
2. Limit Privileges: By default, containers run with root privileges inside the container. It is good practice to run containers with non-root users whenever possible. This reduces the potential impact of any security vulnerabilities that may be present in the container.
To run a container as a non-root user, you can specify the user ID and group ID using the --user
flag when running the docker run
command:
docker run --user :
3. Isolate Containers: Containers should be isolated from each other to prevent unauthorized access to sensitive data. Docker provides multiple isolation mechanisms, such as namespaces and cgroups, to limit the resources and system calls available to a container.
To isolate containers, Docker uses namespaces to create separate instances of various operating system resources (such as the process ID space, network stack, and file system) for each container. Docker also uses cgroups to control the allocation of system resources like CPU, memory, and disk I/O.
4. Secure Container Images: It is important to ensure that the container images you use are free from vulnerabilities and malicious code. You can use vulnerability scanning tools like Anchore (https://anchore.com/) or Clair (https://github.com/quay/clair) to scan container images for known vulnerabilities.
Additionally, you should regularly update your container images to include the latest security patches and updates. This helps to mitigate potential security risks and ensures that your containers are running the most secure versions of software.
5. Network Security: By default, Docker containers can communicate with each other and with the host system. It is important to configure network security to restrict container communication and prevent unauthorized access.
You can use Docker's network features to create separate networks for different containers and control the traffic flow between them. You can also use firewall rules to restrict incoming and outgoing network connections for containers.
6. Container Runtime Security: The container runtime, such as Docker Engine, should also be secured to prevent unauthorized access and ensure the integrity of the containers. Regularly update the container runtime to include the latest security patches and use secure configurations.
7. Regularly Monitor and Audit Containers: Monitoring and auditing the containers in your environment is crucial for detecting and mitigating potential security threats. Use tools like Prometheus (https://prometheus.io/) or ELK stack (Elasticsearch, Logstash, and Kibana) to monitor and analyze container logs, metrics, and events.
By following these best practices, you can enhance the security and isolation of your Docker containers, reducing the risk of security breaches and data leaks.
Remember that security is an ongoing process, and it is important to stay informed about the latest security best practices, vulnerabilities, and updates in the Docker ecosystem.
Related Article: Copying a Directory to Another Using the Docker Add Command
Scaling and Load Balancing with Docker Swarm
Docker Swarm is a native clustering and orchestration solution for Docker. It allows you to create and manage a swarm of Docker nodes, which can be either physical or virtual machines, and distribute containers across them. With Docker Swarm, you can easily scale your applications horizontally and load balance the traffic between them.
To use Docker Swarm, you need to have Docker installed on all the nodes that you want to include in the swarm. Once you have the nodes ready, you can initialize a swarm by running the following command:
$ docker swarm init
This will initialize a new swarm and give you a command to join other nodes to the swarm. You can use this command on other nodes to join them to the swarm as worker nodes. For example:
$ docker swarm join --token :
Once you have a swarm up and running, you can start deploying services to it. A service is a definition of the tasks to run on the swarm, where a task is an instance of a container running on a node. To deploy a service, you can use the following command:
$ docker service create --name --replicas
This will create a new service with the specified name and number of replicas. The Docker Swarm manager will automatically distribute the replicas across the available nodes in the swarm.
To scale a service, you can use the docker service scale
command. For example, to scale a service named "web" to 5 replicas, you can run:
$ docker service scale web=5
Docker Swarm will automatically adjust the number of replicas to match the desired scale.
Load balancing is an important aspect of scaling applications. Docker Swarm provides built-in load balancing for services. When you deploy a service, Docker Swarm automatically assigns a virtual IP address and port to it. Requests to this virtual IP address and port will be load balanced across the replicas of the service.
To access a service, you can use the virtual IP address and port assigned to it. For example, if a service has the virtual IP address 10.0.0.10 and port 8080, you can access it using http://10.0.0.10:8080
.
Docker Swarm also supports routing mesh, which allows you to access services on any node in the swarm, regardless of the node where the service is running. This provides a seamless experience for accessing services in a swarm.
In conclusion, Docker Swarm is a powerful tool for scaling and load balancing applications in a Docker environment. With its native clustering and orchestration capabilities, you can easily scale your applications horizontally and distribute the traffic between them.
Debugging and Troubleshooting
When working with Docker, it is common to encounter issues or bugs that require debugging and troubleshooting. In this section, we will explore some techniques and tools that can help you diagnose and resolve problems in your Docker environment.
Logging
One of the first steps in troubleshooting is to examine the logs generated by Docker containers and the Docker daemon. Docker provides a command-line interface (CLI) option to view container logs:
docker logs
This command will display the logs generated by the specified container, allowing you to see any error messages, warnings, or other relevant information.
To view the logs of the Docker daemon itself, you can use the journalctl
command on Linux systems:
journalctl -u docker.service
On Windows, you can use the Event Viewer to access the Docker daemon logs.
Debugging Containers
Sometimes you may need to debug a running container to troubleshoot issues. Docker provides a command-line option to start a container in interactive mode, allowing you to access its shell and investigate the problem:
docker run -it /bin/bash
This command starts a new container instance and opens a shell prompt inside it. You can then execute commands and inspect the container's filesystem and processes.
If you need to debug a container that is already running, you can use the docker exec
command to start a new process inside the container:
docker exec -it /bin/bash
This command attaches to the running container and opens a new shell prompt, similar to the previous example.
Related Article: Tutorial: Building a Laravel 9 Real Estate Listing App
Inspecting Docker Objects
Docker provides the docker inspect
command to retrieve detailed information about various Docker objects, including containers, images, networks, and volumes. This command can be useful when troubleshooting issues related to these objects.
For example, to inspect a container and retrieve its detailed information, you can use the following command:
docker inspect
This command will display a JSON-formatted output containing information such as the container's configuration, networking details, and mount points.
Monitoring and Performance Analysis
Monitoring and analyzing the performance of your Docker environment can help identify and troubleshoot issues related to resource usage, networking, or application performance.
Docker provides a built-in monitoring tool called Docker Stats, which provides real-time statistics about CPU, memory, disk I/O, and network usage for running containers. You can use the following command to view the stats for a specific container:
docker stats
Additionally, there are numerous third-party monitoring tools available, such as Prometheus and Grafana, which can provide more advanced monitoring and visualization capabilities for your Docker environment.
Using Docker Debugging Tools
In addition to the built-in Docker commands and tools, there are several debugging tools specifically designed for troubleshooting Docker-related issues.
One such tool is "Docker Debug", which provides a set of features to help diagnose and resolve problems in Docker containers. It allows you to attach to running containers, collect diagnostic information, and even perform remote debugging of containerized applications.
To use Docker Debug, you need to install it on your Docker host and then follow the instructions provided by the tool's documentation.
Monitoring and Logging
Monitoring and logging are crucial aspects of managing Docker containers in production environments. By monitoring the performance and health of containers, you can identify and address any issues before they impact your applications. Logging helps you collect and analyze important information about container activities, enabling you to troubleshoot problems and gain insights into your system's behavior.
Related Article: Build a Chat Web App with Flask, MongoDB, Reactjs & Docker
Monitoring Docker Containers
Docker provides various tools and techniques to monitor containers:
1. Docker Stats: The docker stats
command provides real-time CPU, memory, and network usage statistics for running containers. For example, to monitor a specific container named mycontainer
, you can run the following command:
docker stats mycontainer
2. Prometheus and Grafana: Prometheus is an open-source monitoring system that collects and stores time series data. Grafana is a visualization tool that can be used to create dashboards based on Prometheus data. Together, they provide powerful monitoring capabilities for Docker containers. You can use the node_exporter
Docker image to collect host-level metrics and configure Prometheus to scrape these metrics. Then, use Grafana to create visualizations and alerts based on the collected data.
3. Docker Healthcheck: Docker allows you to define health checks for your containers, which are commands used to periodically check the container's health status. You can define a health check using the HEALTHCHECK
instruction in your Dockerfile or by using the --healthcheck
flag when running a container. Docker will automatically monitor the health of the container and take action based on the defined rules.
Logging Docker Containers
Docker provides built-in logging capabilities that allow you to collect and manage container logs:
1. Docker Logs: The docker logs
command allows you to retrieve the logs generated by a container. By default, it shows the entire log output, but you can use options like --tail
or --since
to filter the log entries. For example, to display the last 10 lines of logs for a container named mycontainer
, you can run:
docker logs --tail=10 mycontainer
2. Logging Drivers: Docker supports multiple logging drivers that allow you to send container logs to different destinations, such as local files, syslog, or remote logging services. You can configure the logging driver by setting the --log-driver
flag when running a container or by using the logging
section in the Docker Compose file.
3. Third-Party Tools: Many third-party tools and services are available for centralized log management. Tools like ELK Stack (Elasticsearch, Logstash, and Kibana), Splunk, and Fluentd can be integrated with Docker to collect, analyze, and visualize container logs.
Monitoring and logging should be an integral part of your Docker container management strategy. By effectively monitoring and logging your containers, you can ensure the smooth operation of your applications and quickly troubleshoot any issues that arise.
To learn more about monitoring and logging with Docker, you can refer to the official Docker documentation: https://docs.docker.com/config/containers/logging/.
Deploying Docker in Production
Deploying Docker containers in a production environment requires careful planning and consideration. In this chapter, we will explore some best practices and advanced commands to help you deploy Docker containers effectively and securely.
1. Building Production-Ready Images
Before deploying Docker containers in production, it's important to ensure that your images are optimized and secure. Here are some best practices for building production-ready images:
- Use minimal base images: Start with a lightweight base image, such as Alpine Linux, to reduce the attack surface and improve performance.
- Minimize image layers: Reduce the number of layers in your Docker images to improve build speed and reduce complexity.
- Secure your images: Regularly update your base images and application dependencies to patch security vulnerabilities.
- Use a multi-stage build: Use multi-stage builds to separate the build environment from the runtime environment, reducing the size of your final image.
Here's an example of a Dockerfile using multi-stage build:
# Build stage FROM golang:1.16 AS builder WORKDIR /app COPY . . RUN go build -o myapp # Final stage FROM alpine:latest WORKDIR /app COPY --from=builder /app/myapp . CMD ["./myapp"]
Related Article: How to Install and Use Docker
2. Managing Configuration with Environment Variables
When deploying Docker containers in production, it's common to have different configurations for different environments. Docker allows you to manage these configurations using environment variables.
Here's an example of using environment variables in a Dockerfile:
FROM nginx:latest ENV MY_APP_ENV=production COPY nginx.conf /etc/nginx/nginx.conf
You can set environment variables when running containers using the -e
or --env
flag:
docker run -e MY_APP_ENV=staging mynginx
3. Scaling Docker Services
Scaling Docker services horizontally is a key aspect of deploying in production. Docker Swarm and Kubernetes are popular options for orchestrating and scaling containerized applications.
Here's an example of scaling a Docker service using Docker Swarm:
docker swarm init docker service create --replicas 3 myapp
This command creates a Docker service with 3 replicas, distributing the workload across multiple containers.
4. Monitoring and Logging
Monitoring and logging are essential for maintaining the health and performance of your Docker containers in production. Docker provides several tools and integrations for monitoring and logging, including:
- Docker Stats: Use the docker stats
command to monitor resource usage of running containers.
- Docker Logging Drivers: Configure Docker to send logs to external logging systems like Elasticsearch or Splunk.
- Container Monitoring Solutions: Use third-party tools like Prometheus or Datadog for advanced container monitoring and alerting.
5. Securing Docker Containers
Securing Docker containers in production is crucial to protect your applications and data. Here are some best practices for securing Docker containers:
- Regularly update Docker: Keep Docker and its dependencies up to date to benefit from security patches.
- Limit container privileges: Run containers with minimal privileges by using the --cap-drop
and --cap-add
options.
- Use Docker Content Trust: Enable Docker Content Trust to verify the authenticity and integrity of images.
- Monitor container behavior: Use tools like Docker Security Scanning or Falco to detect and respond to security threats.
Related Article: Comparing Kubernetes vs Docker
6. Continuous Deployment with Docker
Docker can be integrated into a continuous deployment pipeline to automate the deployment process. Tools like Jenkins, GitLab CI/CD, or Travis CI can be used to build, test, and deploy Docker containers.
Here's an example of a Jenkins pipeline stage to deploy Docker containers:
stage('Deploy') { steps { script { docker.withRegistry('https://registry.example.com', 'registry-credentials') { docker.image('myapp:latest').push('latest') } } } }
By integrating Docker into your continuous deployment workflow, you can achieve faster and more reliable deployments.
Deploying Docker containers in production requires careful planning and consideration. By following best practices and using advanced commands, you can ensure the security, scalability, and reliability of your containerized applications.
Real World Use Cases
Docker is a versatile tool that can be used in a wide range of real-world scenarios. In this section, we will explore some common use cases where Docker can greatly benefit developers and system administrators.
1. Application Development and Testing
One of the most popular use cases for Docker is in application development and testing. Docker allows developers to package their application and all its dependencies into a single container, ensuring that it runs consistently across different environments. This makes it easier to reproduce and debug issues, as well as to share the development environment with other team members.
For example, let's say you are developing a web application using Node.js. With Docker, you can create a Docker image that includes Node.js, your application code, and any other dependencies. You can then use this image to easily spin up multiple instances of your application for testing, without worrying about compatibility issues between different development machines.
Here is an example Dockerfile for a Node.js application:
# Use the official Node.js image as the base image FROM node:14 # Set the working directory in the container WORKDIR /app # Copy the package.json and package-lock.json files to the container COPY package*.json ./ # Install the dependencies RUN npm install # Copy the application code to the container COPY . . # Expose the port that the application listens on EXPOSE 3000 # Define the command to run the application CMD [ "node", "app.js" ]
2. Continuous Integration and Deployment
Docker can also be used to streamline the continuous integration and deployment process. By packaging your application and its dependencies into a Docker image, you can ensure that the same image is used throughout the entire development lifecycle, from development to production.
Many popular continuous integration and deployment tools, such as Jenkins and GitLab CI/CD, have built-in support for Docker. This allows you to easily build and test your application in a containerized environment, and then deploy it to production using the same Docker image.
For example, here is a simple GitLab CI/CD configuration file that builds and deploys a Docker image to a Kubernetes cluster:
# .gitlab-ci.yml stages: - build - deploy build: stage: build script: - docker build -t myapp . - docker push myapp deploy: stage: deploy script: - kubectl apply -f deployment.yaml
Related Article: How to Secure Docker Containers
3. Microservices Architecture
Docker is a perfect fit for building and deploying applications based on a microservices architecture. In a microservices architecture, an application is split into multiple smaller, loosely coupled services that can be developed, deployed, and scaled independently.
Each microservice can be packaged into its own Docker container, allowing it to be managed and scaled independently. This makes it easier to maintain and update individual services without affecting the entire application.
For example, let's say you are building an e-commerce application with separate services for user authentication, product catalog, and order processing. By using Docker, you can package each service into a separate container, making it easier to develop and deploy each service independently.
4. Hybrid Cloud and Multi-Cloud Environments
Docker also provides a consistent environment across different cloud platforms, making it easier to deploy applications in hybrid cloud and multi-cloud environments. With Docker, you can package your application and its dependencies into a container, and then deploy it to any cloud platform that supports Docker.
This allows you to take advantage of the benefits of different cloud providers, such as scalability and fault tolerance, without having to re-architect your application for each platform.
For example, you can use Docker to package your application and deploy it to both AWS and Azure, without making any changes to your application code. This gives you the flexibility to choose the cloud provider that best suits your needs, without being locked into a specific platform.
In this chapter, we explored some real-world use cases where Docker can be a valuable tool for developers and system administrators. From application development and testing to continuous integration and deployment, Docker offers a wide range of benefits that can greatly simplify the development and deployment process.
Best Practices
When working with Docker CLI and advanced commands, it is important to follow best practices to ensure the smooth functioning of your Docker containers and the overall efficiency of your development process. Here are some best practices to consider:
1. Use descriptive and meaningful names for your containers, images, and volumes. This will make it easier for you and your team to understand and manage the Docker resources.
2. Keep your containers lightweight by using the appropriate base image. Choose the smallest base image that meets your application's requirements. This helps reduce the size of the final image and improves the performance of your containers.
3. Use version tags for your images to ensure reproducibility and avoid unexpected changes. When pulling or running an image, specify the version tag to ensure you are using the desired version. For example:
docker pull nginx:1.19.2 docker run -d nginx:1.19.2
4. Regularly update your base images and dependencies to take advantage of security patches and bug fixes. Docker Hub provides automated builds and notifications for updated images, allowing you to stay up-to-date with the latest releases.
5. Avoid running containers as root whenever possible. Running containers as non-root users improves security by reducing the potential impact of any security vulnerabilities.
6. Limit container resource usage to prevent one container from monopolizing system resources. Use resource constraints such as CPU and memory limits to ensure fair resource allocation among containers.
docker run -d --name mycontainer --cpus=2 --memory=2g nginx
7. Utilize Docker volumes for persistent data storage. Mounting volumes to containers allows you to separate data from the container itself, making it easier to manage and backup data.
docker run -d -v /path/to/host/directory:/path/to/container/directory nginx
8. Use environment variables for configuration instead of hardcoding values in your Dockerfiles. This makes it easier to manage and modify configuration settings without having to rebuild the image.
docker run -d -e MYSQL_ROOT_PASSWORD=secretpassword mysql
9. Take advantage of Docker's networking capabilities to isolate containers and control their communication. Use Docker networks to create logical networks for your containers and assign them to specific networks as needed.
docker network create mynetwork docker run -d --network=mynetwork nginx
10. Properly clean up unused resources to avoid clutter and free up disk space. Remove stopped containers, unused images, and orphaned volumes periodically using the appropriate Docker CLI commands.
docker container prune docker image prune docker volume prune
By following these best practices, you can optimize your Docker workflow, improve security, and ensure the smooth operation of your containers.