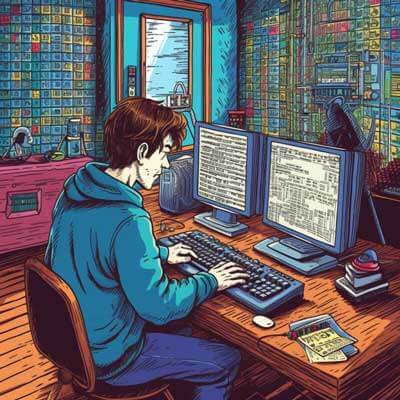
Table of Contents
Integrating MongoDB with Django
When it comes to integrating MongoDB with Django, there are several options available. One popular choice is to use the djongo
library, which allows you to seamlessly integrate MongoDB as the backend database for your Django project.
To get started, you'll first need to install the djongo
library. You can do this by running the following command:
pip install djongo
Once you have djongo
installed, you can update your Django settings to use MongoDB as the database engine. Here's an example of how you can configure your settings:
DATABASES = { 'default': { 'ENGINE': 'djongo', 'NAME': 'mydatabase', 'HOST': 'localhost', 'PORT': 27017, 'USER': 'myuser', 'PASSWORD': 'mypassword', } }
In this example, we're specifying the MongoDB database name, host, port, and authentication credentials. You can customize these values based on your MongoDB setup.
Once you've configured your settings, you can start using MongoDB with Django. You can define Django models as you would with any other database backend. Here's an example of a Django model using MongoDB:
from djongo import models class Product(models.Model): name = models.CharField(max_length=100) price = models.DecimalField(decimal_places=2, max_digits=10) description = models.TextField()
In this example, we're defining a Product
model with three fields: name
, price
, and description
. These fields will be stored in MongoDB as part of the Product
collection.
You can now use this model to perform CRUD operations on MongoDB. For example, to create a new product, you can do the following:
product = Product(name='Example Product', price=9.99, description='This is an example product') product.save()
This will create a new document in the Product
collection in MongoDB.
Overall, integrating MongoDB with Django using the djongo
library is a useful way to leverage the flexibility and scalability of a NoSQL database in your Django projects.
Related Article: Advanced Django Views & URL Routing: Mixins and Decorators
Advantages of Using NoSQL Databases with Django
NoSQL databases, such as MongoDB, offer several advantages when used with Django:
1. Schema Flexibility: Unlike traditional SQL databases, NoSQL databases do not require a predefined schema. This means that you can store data with varying structures in the same collection, providing more flexibility for your applications.
2. Scalability: NoSQL databases are designed to scale horizontally, making them ideal for handling large amounts of data and high traffic loads. This scalability is crucial for modern web applications that need to handle millions of users and massive amounts of data.
3. Performance: NoSQL databases are optimized for read and write operations, making them faster than traditional SQL databases in certain use cases. This can lead to improved performance and responsiveness in your Django applications.
4. Aggregation and Analytics: NoSQL databases often provide useful aggregation and analytics capabilities, allowing you to perform complex queries and analysis on large datasets. This can be beneficial for generating insights and making data-driven decisions.
5. Flexibility in Data Modeling: NoSQL databases allow you to model your data in a way that best suits your application's needs. You can store nested data structures, arrays, and other complex data types without the need for complex join operations.
While NoSQL databases offer these advantages, it's essential to carefully consider the requirements and characteristics of your application before deciding to use them. NoSQL databases may not be suitable for all use cases, and it's crucial to evaluate the trade-offs in terms of consistency, transaction support, and query capabilities.
Optimizing Search Functionality with ElasticSearch in Django
ElasticSearch is a useful search engine that can be integrated with Django to enhance search functionality in your applications. It provides features like full-text search, filtering, faceted navigation, and relevance scoring, making it an excellent choice for building robust search capabilities.
To integrate ElasticSearch with Django, you'll need to install the elasticsearch-dsl
library, which provides a high-level Python interface to interact with ElasticSearch. You can install it using the following command:
pip install elasticsearch-dsl
Once you have elasticsearch-dsl
installed, you can define an ElasticSearch index for your Django models. Here's an example:
from elasticsearch_dsl import Document, Text class ProductIndex(Document): name = Text() description = Text()
In this example, we're defining an ElasticSearch index called ProductIndex
with two fields: name
and description
. These fields will be indexed and searchable in ElasticSearch.
Next, you can define a mapping between your Django models and ElasticSearch indexes. Here's an example for the Product
model:
from django_elasticsearch_dsl import Document, fields from .models import Product class ProductIndex(Document): name = fields.TextField() description = fields.TextField() class Index: name = 'products'
In this example, we're using the django_elasticsearch_dsl
library, which provides integration between Django and ElasticSearch. We're defining the mapping between the Product
model and the ProductIndex
index. The Index
class specifies the name of the index.
Once you've defined the mapping, you can use the ElasticSearch index to perform search operations. Here's an example of searching for products:
from elasticsearch_dsl import Search from .documents import ProductIndex def search_products(query): s = Search().query('multi_match', query=query, fields=['name', 'description']) response = s.execute() products = [] for hit in response.hits: product = hit.to_dict() products.append(product) return products
In this example, we're using the elasticsearch_dsl
library to construct a search query that matches the query
parameter against the name
and description
fields. We execute the search query and retrieve the matching products.
Benefits of Using Message Brokers in Django
Message brokers play a vital role in building scalable and reliable distributed systems. They act as intermediaries between different components of a system, enabling asynchronous communication and decoupling the sender and receiver.
When it comes to Django, integrating message brokers can bring several benefits:
1. Asynchronous Processing: Message brokers allow you to offload time-consuming or resource-intensive tasks to background workers, freeing up your Django application to handle other requests. This can improve the responsiveness and scalability of your application.
2. Decoupling Components: Message brokers enable decoupling between different components of your system. Instead of direct communication between components, they communicate via messages, providing loose coupling and enabling independent evolution and scaling of components.
3. Reliability and Fault Tolerance: Message brokers provide mechanisms for ensuring reliable message delivery, even in the presence of failures. They can handle message retries, acknowledgments, and persistent storage, ensuring that messages are not lost or duplicated.
4. Scalability: By using message brokers, you can scale individual components of your system independently. You can add more consumers to handle high message loads or distribute messages across multiple queues or topics.
5. Flexibility: Message brokers support various messaging patterns, including publish-subscribe, point-to-point, and request-reply. This flexibility allows you to choose the most suitable pattern for your application's requirements.
There are several message brokers available that can be integrated with Django, including RabbitMQ and Kafka. Each has its strengths and use cases.
Related Article: How to Use Python's isnumeric() Method
Integrating RabbitMQ with Django
RabbitMQ is a widely-used open-source message broker that provides robust messaging capabilities. To integrate RabbitMQ with Django, you'll need to install the pika
library, which is a Python client for RabbitMQ. You can install it using the following command:
pip install pika
Once you have pika
installed, you can start using RabbitMQ in your Django application. Here's an example of how you can publish a message to a RabbitMQ queue:
import pika def publish_message(message): connection = pika.BlockingConnection(pika.ConnectionParameters('localhost')) channel = connection.channel() channel.queue_declare(queue='myqueue') channel.basic_publish(exchange='', routing_key='myqueue', body=message) connection.close()
In this example, we establish a connection to the RabbitMQ server, create a channel, and declare a queue called 'myqueue'. We then publish a message to the queue using the basic_publish
method.
To consume messages from a RabbitMQ queue in your Django application, you can use the following code:
import pika def consume_message(): connection = pika.BlockingConnection(pika.ConnectionParameters('localhost')) channel = connection.channel() channel.queue_declare(queue='myqueue') channel.basic_consume(queue='myqueue', on_message_callback=handle_message, auto_ack=True) channel.start_consuming() def handle_message(ch, method, properties, body): print(body)
In this example, we establish a connection to the RabbitMQ server, create a channel, and declare a queue called 'myqueue'. We then use the basic_consume
method to register a callback function handle_message
that will be called whenever a message is received from the queue.
Integrating Kafka with Django
Kafka is a distributed streaming platform that provides high-throughput, fault-tolerant messaging. It is widely used for building real-time data pipelines and streaming applications. To integrate Kafka with Django, you'll need to install the confluent-kafka-python
library, which is a Python client for Kafka. You can install it using the following command:
pip install confluent-kafka
Once you have confluent-kafka-python
installed, you can start using Kafka in your Django application. Here's an example of how you can produce messages to a Kafka topic:
from confluent_kafka import Producer def produce_message(message): producer = Producer({'bootstrap.servers': 'localhost:9092'}) producer.produce('mytopic', value=message) producer.flush()
In this example, we create a Kafka producer and use the produce
method to send a message to the 'mytopic' topic. The flush
method ensures that all messages are sent before the producer is closed.
To consume messages from a Kafka topic in your Django application, you can use the following code:
from confluent_kafka import Consumer, KafkaException def consume_message(): consumer = Consumer({ 'bootstrap.servers': 'localhost:9092', 'group.id': 'mygroup', 'auto.offset.reset': 'earliest' }) consumer.subscribe(['mytopic']) try: while True: msg = consumer.poll(1.0) if msg is None: continue if msg.error(): if msg.error().code() == KafkaError._PARTITION_EOF: continue else: raise KafkaException(msg.error()) print('Received message: {}'.format(msg.value().decode('utf-8'))) except KeyboardInterrupt: pass finally: consumer.close()
In this example, we create a Kafka consumer and use the poll
method to receive messages from the 'mytopic' topic. The consumer is subscribed to the topic using the subscribe
method. We continuously poll for new messages and print their values.
Libraries and Packages for MongoDB Integration with Django
There are several libraries and packages available for integrating MongoDB with Django. Here are some popular choices:
1. djongo: Djongo is a SQL to MongoDB query compiler that allows you to use MongoDB as the backend database for your Django application. It provides seamless integration with Django's ORM, allowing you to define models and perform CRUD operations on MongoDB. You can install it using the following command:
pip install djongo
2. mongoengine: Mongoengine is a Document-Object Mapper (DOM) that provides a high-level API for interacting with MongoDB in Python. It allows you to define models as Python classes and provides a rich set of query and aggregation methods. You can install it using the following command:
pip install mongoengine
3. django-mongodb-engine: Django MongoDB Engine is a Django database backend that allows you to use MongoDB as the backend database for your Django application. It provides support for most of Django's features, including models, transactions, and migrations. You can install it using the following command:
pip install django-mongodb-engine
These libraries and packages provide different levels of integration with MongoDB and offer various features and capabilities. It's essential to evaluate your project's requirements and choose the one that best suits your needs.
Best Practices for Integrating NoSQL Databases with Django
When integrating NoSQL databases like MongoDB with Django, there are several best practices to keep in mind:
1. Model Design: Design your models in a way that leverages the strengths of NoSQL databases. Take advantage of the flexibility in schema design and consider denormalizing your data to avoid complex join operations.
2. Indexing: Define appropriate indexes on your collections to optimize query performance. Identify the most frequently used query patterns and create indexes to support them.
3. Data Access Patterns: Understand the data access patterns of your application and design your queries accordingly. NoSQL databases excel at certain types of queries, such as document retrieval and aggregation, so leverage these capabilities to improve performance.
4. Concurrency and Locking: NoSQL databases often provide optimistic concurrency control mechanisms to handle concurrent updates. Understand how your chosen NoSQL database handles concurrency and implement appropriate locking mechanisms in your Django application.
5. Monitoring and Performance Tuning: Monitor the performance of your NoSQL database and make necessary adjustments to optimize performance. Use tools like MongoDB's built-in profiler and query optimizer to identify and address performance bottlenecks.
6. Backup and Disaster Recovery: Implement regular backups and disaster recovery strategies to ensure the integrity and availability of your NoSQL database. Consider using features like replica sets and sharding for high availability and fault tolerance.
7. Security: Implement proper security measures to protect your NoSQL database from unauthorized access. Use authentication and authorization mechanisms provided by the database and follow security best practices.
Related Article: How to Calculate the Square Root in Python
Setting Up ElasticSearch for Search Optimization in Django
To set up ElasticSearch for search optimization in Django, you'll need to perform the following steps:
1. Install ElasticSearch: First, you'll need to install ElasticSearch on your server. You can download the latest version of ElasticSearch from the official website (https://www.elastic.co/downloads/elasticsearch) and follow the installation instructions for your operating system.
2. Configure ElasticSearch: Once ElasticSearch is installed, you'll need to configure it to work with your Django application. Open the elasticsearch.yml
configuration file and update the following settings:
- cluster.name
: Set the name of your ElasticSearch cluster.
- network.host
: Set the network host to bind ElasticSearch to. By default, it binds to localhost, but you can change it to the IP address or hostname of your server.
- http.port
: Set the HTTP port on which ElasticSearch should listen. The default port is 9200.
Save the changes and restart the ElasticSearch service.
3. Install Python Libraries: Next, you'll need to install the necessary Python libraries to interact with ElasticSearch in your Django application. You can install the elasticsearch
and django-elasticsearch-dsl
libraries using the following command:
pip install elasticsearch django-elasticsearch-dsl
4. Define ElasticSearch Documents: In your Django application, you'll need to define ElasticSearch documents that map to your Django models. These documents specify the fields to be indexed and their respective data types. Here's an example of a document for a Product
model:
from django_elasticsearch_dsl import Document, fields from .models import Product class ProductDocument(Document): name = fields.TextField() description = fields.TextField() class Index: name = 'products'
In this example, we're defining a ProductDocument
that maps to the Product
model. We specify the fields to be indexed (name
and description
) and the index name (products
).
5. Index Data: Once you've defined your ElasticSearch documents, you'll need to index your existing data. You can do this by running the following command:
python manage.py search_index --rebuild
This command will rebuild the search index for all your indexed models.
6. Perform Search Operations: With ElasticSearch set up, you can now perform search operations in your Django application. Here's an example of how you can search for products:
from elasticsearch_dsl import Search from .documents import ProductDocument def search_products(query): s = Search().query('multi_match', query=query, fields=['name', 'description']) response = s.execute() products = [] for hit in response.hits: product = hit.to_dict() products.append(product) return products
In this example, we're using the elasticsearch_dsl
library to construct a search query that matches the query
parameter against the name
and description
fields. We execute the search query and retrieve the matching products.
Ensuring Reliable Message Delivery with Message Brokers in Django
When working with message brokers in Django, ensuring reliable message delivery is crucial to building robust and scalable distributed systems. Here are some best practices to follow:
1. Message Acknowledgment: When consuming messages from a message broker, make sure to acknowledge the successful processing of each message. This ensures that the message broker doesn't re-deliver the message in case of failures. Most message brokers provide mechanisms for acknowledging messages, such as acknowledgments in RabbitMQ or offsets in Kafka.
2. Retry Mechanisms: Implement retry mechanisms for failed message processing. If a message fails to be processed, you can retry the processing after a certain delay or back off exponentially with each retry. This helps handle transient failures and increases the chances of successful message processing.
3. Dead Letter Queues: Use dead letter queues to handle messages that repeatedly fail to be processed. Dead letter queues are special queues where failed messages are sent for manual inspection and resolution. This allows you to identify and fix issues that prevent successful message processing.
4. Message Durability: Configure your message broker to store messages persistently to ensure durability. In case of broker failures, persistent messages can be recovered and processed once the broker is back online. This is especially important for critical messages that should not be lost.
5. Monitoring and Alerting: Set up monitoring and alerting for your message broker to detect issues and failures. Monitor message throughput, queue sizes, and consumer lag to ensure that your system is running smoothly. Use tools like Prometheus, Grafana, or built-in monitoring features provided by the message broker.
6. Load Balancing and Scaling: If your message broker supports it, distribute message processing across multiple consumers to achieve load balancing and scalability. This allows you to handle higher message volumes and ensures that processing is not bottlenecked by a single consumer.
Additional Resources
- Integrating RabbitMQ with Django