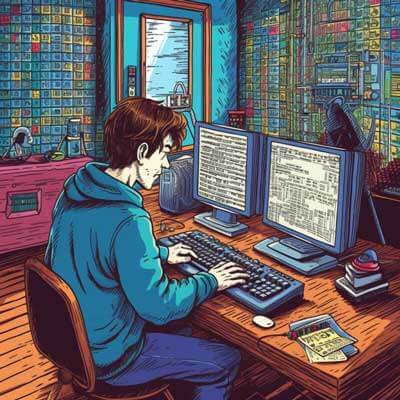
Table of Contents
To get the current directory and the directory of a specific file in Python, you can use the os
module. The os
module provides a set of functions that allow you to interact with the operating system. Specifically, you can use the os.getcwd()
function to get the current working directory and the os.path.dirname()
function to get the directory of a file.
Here are two possible approaches to get the current directory and file directory in Python:
Approach 1: Using the os.getcwd()
and os.path.dirname()
functions
You can use the os.getcwd()
function to get the current working directory and the os.path.dirname()
function to get the directory of a file.
Here's an example that demonstrates how to use these functions:
import os # Get the current working directory current_directory = os.getcwd() print("Current Directory:", current_directory) # Get the directory of a file file_path = "/path/to/file.txt" file_directory = os.path.dirname(file_path) print("File Directory:", file_directory)
In this example, the os.getcwd()
function returns the current working directory, which is then printed to the console. The os.path.dirname()
function is used to get the directory of a file, specified by its path. The file directory is also printed to the console.
Related Article: How To Round To 2 Decimals In Python
Approach 2: Using the os.path
module
Alternatively, you can use the os.path
module, which is a submodule of the os
module, to get the current directory and the directory of a file.
Here's an example that demonstrates how to use the os.path
module:
import os.path # Get the current working directory current_directory = os.path.abspath(os.path.dirname(__file__)) print("Current Directory:", current_directory) # Get the directory of a file file_path = "/path/to/file.txt" file_directory = os.path.abspath(os.path.dirname(file_path)) print("File Directory:", file_directory)
In this example, the os.path.abspath()
function is used to get the absolute path of the current directory and the directory of a file. The os.path.dirname()
function is used to get the directory name from the file path. The __file__
variable represents the current file, and os.path.dirname(__file__)
returns the directory containing the current file.
Why would you need to get the current directory and file directory?
There are several reasons why you might need to get the current directory and the directory of a file in Python:
1. File operations: When working with files, you may need to access files in the current directory or in a specific directory. By getting the current directory and file directory, you can easily construct file paths and perform file operations such as reading, writing, or deleting files.
2. Configuration files: Many applications use configuration files to store settings and preferences. By getting the current directory, you can locate the configuration file relative to the current directory. This allows you to load and parse the configuration file easily.
3. File organization: If you are working on a project that involves multiple files and directories, knowing the current directory and file directory can help you organize and manage your files more effectively. You can use the information to create relative paths and navigate between directories.
Alternative ideas and suggestions
While the approaches mentioned above are common and widely used, there are alternative ideas and suggestions that you can consider when working with directories and files in Python:
1. Using the pathlib
module: Instead of using the os
module, you can use the pathlib
module, which provides an object-oriented approach to working with paths. The pathlib.Path.cwd()
method can be used to get the current working directory, and the pathlib.Path(file_path).parent
attribute can be used to get the directory of a file. Here's an example:
from pathlib import Path # Get the current working directory current_directory = Path.cwd() print("Current Directory:", current_directory) # Get the directory of a file file_path = "/path/to/file.txt" file_directory = Path(file_path).parent print("File Directory:", file_directory)
2. Using the inspect
module: If you want to get the directory of the currently executing script, you can use the inspect
module. The inspect
module provides functions for introspecting live objects, including the inspect.getfile()
function, which returns the file name of a module, class, method, function, traceback, frame, or code object. Here's an example:
import inspect # Get the directory of the currently executing script script_directory = Path(inspect.getfile(inspect.currentframe())).parent print("Script Directory:", script_directory)
Note that the inspect
module should be used with caution, as it is primarily intended for debugging and development purposes.
Related Article: How To Iterate Over Dictionaries Using For Loops In Python
Best practices
Here are some best practices to keep in mind when getting the current directory and file directory in Python:
1. Use absolute paths when working with files and directories: Absolute paths provide an unambiguous and consistent way to refer to files and directories, regardless of the current working directory. It is generally recommended to use absolute paths, especially when dealing with file operations or configuration files.
2. Handle exceptions: When working with files and directories, it is important to handle exceptions that may occur. For example, the file or directory you are trying to access may not exist, or you may not have the necessary permissions. Use try-except blocks to catch and handle any exceptions that may occur during file operations.
3. Use platform-independent path manipulation: Python provides various functions and modules for manipulating paths, such as os.path
and pathlib
. When working with paths, it is recommended to use platform-independent path manipulation functions to ensure your code works correctly on different operating systems.
4. Document your code: When working with directories and files, it is important to document your code and provide clear explanations of what each function or method does. This will make it easier for other developers to understand and maintain your code.
Overall, getting the current directory and file directory in Python is a common task when working with files and directories. By using the os
module or the pathlib
module, you can easily retrieve this information and perform various file operations or configuration tasks. Remember to handle exceptions, use absolute paths, and document your code for clarity and maintainability.