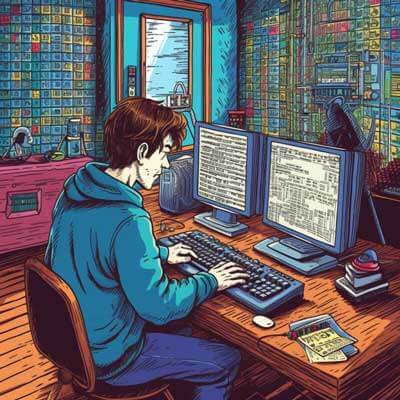
Table of Contents
Working with Scalar Values in SQL
Scalar values in SQL refer to single, atomic values that are not part of a table or a result set. These values can be integers, strings, dates, or any other data type supported by the database system. Scalar values are often used in SQL queries for filtering, sorting, or performing calculations.
Let's take a look at some examples of working with scalar values in SQL:
Example 1: Filtering Data with Scalar Values
Suppose we have a table called "employees" with the following columns: "id", "name", and "salary". We want to retrieve all employees with a salary greater than 50000.
SELECT * FROM employees WHERE salary > 50000;
In this example, the scalar value is 50000, and we use it to filter the rows in the "employees" table.
Example 2: Performing Calculations with Scalar Values
Suppose we have a table called "orders" with the following columns: "id", "product", "quantity", and "price". We want to calculate the total cost of each order by multiplying the quantity and price columns.
SELECT id, product, quantity, price, (quantity * price) AS total_cost FROM orders;
In this example, the scalar values are the quantity and price columns, and we use them to perform the calculation for the total_cost column.
Related Article: How to Implement Database Sharding in MongoDB
Structuring and Managing Database Tables
Structuring and managing database tables is a crucial aspect of database design and administration. Properly designed tables help improve data integrity, efficiency, and overall performance of the database system.
Here are some key considerations for structuring and managing database tables:
1. Identify Entities and Relationships:
Before creating tables, it's important to identify the entities (objects) and their relationships in the domain you are modeling. Entities represent real-world objects, and relationships define how these objects are related to each other.
2. Define Primary Keys:
Each table should have a primary key, which uniquely identifies each row in the table. Primary keys help maintain data integrity and enable efficient data retrieval.
Example:
CREATE TABLE employees ( id INT PRIMARY KEY, name VARCHAR(100), salary DECIMAL(10, 2) );
In this example, the "id" column serves as the primary key for the "employees" table.
3. Define Foreign Keys:
Foreign keys establish relationships between tables. They ensure referential integrity by enforcing constraints on the values stored in related tables.
Example:
CREATE TABLE orders ( id INT PRIMARY KEY, employee_id INT, product VARCHAR(100), quantity INT, price DECIMAL(10, 2), FOREIGN KEY (employee_id) REFERENCES employees(id) );
In this example, the "employee_id" column in the "orders" table is a foreign key that references the "id" column in the "employees" table.
4. Normalize the Database:
Normalization is the process of organizing data in a database to eliminate redundancy and improve data integrity. It involves breaking down tables into smaller, more manageable entities and establishing relationships between them.
5. Indexing:
Indexing is a technique used to improve the performance of database queries by creating data structures that enable faster data retrieval. Indexes are created on specific columns and allow the database system to locate data more efficiently.
Writing Basic SQL Queries
SQL (Structured Query Language) is a useful language used for managing and manipulating relational databases. Writing basic SQL queries is essential for retrieving and manipulating data stored in database tables.
Here are some examples of basic SQL queries:
Example 1: Retrieving Data from a Single Table
Suppose we have a table called "customers" with the following columns: "id", "name", "email", and "phone". We want to retrieve all customers from this table.
SELECT * FROM customers;
In this example, the asterisk (*) is a wildcard character that represents all columns in the "customers" table.
Example 2: Retrieving Specific Columns
Suppose we have a table called "products" with the following columns: "id", "name", "price", and "category". We want to retrieve only the name and price columns from this table.
SELECT name, price FROM products;
In this example, we specify the columns we want to retrieve in the SELECT statement.
Example 3: Filtering Data with WHERE Clause
Suppose we have a table called "orders" with the following columns: "id", "product", "quantity", and "status". We want to retrieve all orders with a quantity greater than 10.
SELECT * FROM orders WHERE quantity > 10;
In this example, the WHERE clause is used to filter the rows in the "orders" table based on the specified condition.
Common Syntax Errors to Avoid in SQL
When writing SQL queries, it's important to be aware of common syntax errors that can occur. These errors can prevent your queries from executing correctly or returning the expected results.
Here are some common syntax errors to avoid in SQL:
1. Missing or Mismatched Quotes:
When using strings in SQL queries, it's important to enclose them in single quotes ('') or double quotes (""). Forgetting to include or mismatching the quotes can result in syntax errors.
Example:
SELECT * FROM customers WHERE name = John;
In this example, the missing quotes around the name 'John' would cause a syntax error.
2. Missing or Extra Parentheses:
When using parentheses in SQL queries, it's important to ensure they are balanced and used correctly. Missing or extra parentheses can lead to syntax errors.
Example:
SELECT * FROM products WHERE (price > 100;
In this example, the missing closing parenthesis would cause a syntax error.
3. Misspelled Keywords:
SQL keywords are case-insensitive, but misspelling them can result in syntax errors. It's important to double-check the spelling of keywords to avoid errors.
Example:
SELET * FROM customers;
In this example, the misspelling of the SELECT keyword would cause a syntax error.
4. Improper Use of Clauses:
SQL queries consist of various clauses such as SELECT, FROM, WHERE, and ORDER BY. It's important to use these clauses in the correct order and to include all required clauses.
Example:
SELECT * customers WHERE name = 'John';
In this example, the missing FROM clause would cause a syntax error.
5. Incorrect Table or Column Names:
Using incorrect table or column names in SQL queries can result in syntax errors. It's important to double-check the names of tables and columns to ensure they are correct.
Example:
SELECT * FROM customerss;
In this example, the misspelled table name would cause a syntax error.
Related Article: Tutorial on SQL Like and SQL Not Like in Databases
Understanding Database Management Systems
A database management system (DBMS) is software that enables users to interact with databases. It provides an interface for creating, modifying, and querying databases, as well as managing data security, concurrency, and recovery.
Here are some key concepts to understand about database management systems:
1. Data Definition Language (DDL):
DDL is a subset of SQL used to define the structure and organization of databases. It includes statements such as CREATE, ALTER, and DROP for creating tables, modifying their structure, and deleting them.
Example:
CREATE TABLE customers ( id INT PRIMARY KEY, name VARCHAR(100), email VARCHAR(100) );
2. Data Manipulation Language (DML):
DML is a subset of SQL used to insert, update, and delete data in databases. It includes statements such as INSERT, UPDATE, and DELETE.
Example:
INSERT INTO customers (id, name, email) VALUES (1, 'John Doe', 'john@example.com');
3. Data Query Language (DQL):
DQL is a subset of SQL used to retrieve data from databases. It includes the SELECT statement, which allows users to specify the columns and conditions for data retrieval.
Example:
SELECT * FROM customers WHERE name = 'John Doe';
4. Transaction Management:
DBMSs provide transaction management capabilities to ensure data integrity and consistency. Transactions are units of work that consist of one or more database operations. They are typically used to group related operations and ensure that they are executed atomically.
Example:
BEGIN TRANSACTION; INSERT INTO orders (id, product, quantity) VALUES (1, 'Product A', 10); UPDATE inventory SET quantity = quantity - 10 WHERE product = 'Product A'; COMMIT;
5. Concurrency Control:
DBMSs employ concurrency control mechanisms to handle multiple users accessing the database simultaneously. These mechanisms ensure that transactions are executed in an isolated and consistent manner, preventing conflicts and data inconsistencies.
6. Data Security:
DBMSs provide features for securing data, such as user authentication, access control, and data encryption. These features help protect sensitive data from unauthorized access and ensure data privacy.
Retrieving Data from Databases with SQL Statements
Retrieving data from databases is a common task when working with SQL. SQL statements are used to specify the columns to retrieve, conditions for filtering data, and sort order.
Here are some examples of retrieving data from databases using SQL statements:
Example 1: Retrieving All Rows and Columns
To retrieve all rows and columns from a table, you can use the SELECT statement without any additional clauses.
SELECT * FROM employees;
In this example, the asterisk (*) is used as a wildcard character to represent all columns in the "employees" table.
Example 2: Retrieving Specific Columns
To retrieve specific columns from a table, you can specify the column names in the SELECT statement.
SELECT name, salary FROM employees;
In this example, the SELECT statement retrieves only the "name" and "salary" columns from the "employees" table.
Example 3: Filtering Data with WHERE Clause
To retrieve specific rows based on a condition, you can use the WHERE clause in the SELECT statement.
SELECT * FROM employees WHERE salary > 50000;
In this example, the WHERE clause filters the rows in the "employees" table to retrieve only those with a salary greater than 50000.
Example 4: Sorting Data with ORDER BY Clause
To retrieve data in a specific order, you can use the ORDER BY clause in the SELECT statement.
SELECT * FROM employees ORDER BY salary DESC;
In this example, the ORDER BY clause sorts the rows in the "employees" table in descending order based on the "salary" column.
The Role of SQL Databases in Application Development
SQL databases play a crucial role in application development by providing a reliable and efficient way to store, retrieve, and manipulate data. They are widely used in various domains, including web development, enterprise applications, and data analytics.
Here are some key roles of SQL databases in application development:
1. Data Storage:
SQL databases provide a structured and organized way to store data. They allow developers to define tables, columns, and relationships between tables, ensuring data integrity and consistency.
Example:
CREATE TABLE customers ( id INT PRIMARY KEY, name VARCHAR(100), email VARCHAR(100) );
In this example, the SQL database is used to create a "customers" table with columns for storing customer information.
2. Data Retrieval:
SQL databases enable efficient retrieval of data using SQL queries. Developers can specify the columns to retrieve, conditions for filtering data, and sort order to retrieve the desired data.
Example:
SELECT * FROM customers WHERE name = 'John Doe';
In this example, the SQL database is used to retrieve customers with the name 'John Doe' from the "customers" table.
3. Data Manipulation:
SQL databases allow developers to insert, update, and delete data using SQL statements. This enables applications to modify and maintain data in the database.
Example:
INSERT INTO customers (id, name, email) VALUES (1, 'John Doe', 'john@example.com');
In this example, the SQL database is used to insert a new customer into the "customers" table.
4. Data Integrity and Security:
SQL databases provide features for ensuring data integrity and security. They support constraints, such as primary keys and foreign keys, to enforce data integrity rules. They also provide mechanisms for securing data, such as user authentication and access control.
5. Scalability and Performance:
SQL databases are designed to handle large amounts of data and support high-performance data retrieval and manipulation. They provide optimization techniques, such as indexing and query optimization, to improve query performance. They also support replication and clustering for scalability and fault tolerance.
The Importance of Database Normalization
Database normalization is a process used to design databases in a way that reduces data redundancy and improves data integrity. It involves breaking down tables into smaller, more manageable entities and establishing relationships between them.
Here are some key reasons why database normalization is important:
1. Data Integrity:
Normalization helps maintain data integrity by reducing data redundancy. Redundant data can lead to inconsistencies and anomalies when inserting, updating, or deleting data. By eliminating redundancy, normalization ensures that each piece of data is stored in one place, reducing the risk of inconsistencies.
2. Efficient Storage:
Normalization optimizes storage space by eliminating duplicate data. This can result in significant storage savings, especially for large databases. It also improves query performance by reducing the amount of data that needs to be accessed and processed.
3. Easier Maintenance:
Normalized databases are easier to maintain and modify. Since data is organized into smaller, more manageable entities, it is easier to make changes to the database schema without affecting the entire database. This flexibility makes it easier to adapt to changing business requirements.
4. Improved Query Performance:
Normalized databases often have better query performance due to their optimized structure. By breaking down tables into smaller entities, queries can be more targeted and efficient. Indexing and other optimization techniques can be applied to individual tables, further improving query performance.
5. Data Consistency:
Normalization helps ensure data consistency by enforcing data integrity rules. By establishing relationships between tables and using foreign keys, referential integrity can be maintained. This ensures that data remains consistent and accurate across the database.
Related Article: Monitoring the PostgreSQL Service Health
Improving Performance with Database Indexing
Database indexing is a technique used to improve the performance of database queries by creating data structures that enable faster data retrieval. Indexes are created on specific columns and allow the database system to locate data more efficiently.
Here are some key points about improving performance with database indexing:
1. What is an Index?
An index is a data structure that stores a subset of the data in a table, organized in a way that allows for efficient data retrieval. It consists of one or more columns from the table and their corresponding values.
2. How Indexing Works
When an index is created on a column, the database system creates a separate data structure that maps the values of that column to the corresponding rows in the table. This data structure allows the database to quickly locate the rows that match a given value or range of values.
3. When to Use Indexing
Indexes are most effective for columns that are frequently used in WHERE clauses, JOIN conditions, or ORDER BY clauses. By creating indexes on these columns, the database system can quickly locate the relevant rows, improving query performance.
4. Types of Indexes
There are different types of indexes, including:
- B-Tree Index: This is the most common type of index, suitable for most situations. It organizes the data in a balanced tree structure, allowing for efficient data retrieval.
- Hash Index: This type of index uses a hash function to map the values to the corresponding rows. It is best suited for equality searches rather than range searches.
- Bitmap Index: This type of index uses a bitmap to represent the presence or absence of values in a column. It is most effective for columns with a limited number of distinct values.
5. Indexing Best Practices
To maximize the benefits of indexing, consider the following best practices:
- Identify the columns that are frequently used in queries and create indexes on these columns.
- Avoid creating too many indexes, as this can impact write performance and increase storage requirements.
- Regularly analyze and update the indexes to ensure they remain optimal for query performance.
- Consider using multi-column indexes for queries that involve multiple columns.
6. Indexing Caveats
While indexing can significantly improve query performance, it also has some caveats to consider:
- Indexes occupy storage space, so creating too many indexes can increase storage requirements.
- Indexes need to be maintained when data is inserted, updated, or deleted, which can impact write performance.
- Indexes are not suitable for columns with low selectivity, where the values are not distinct enough to provide significant performance improvements.
Additional Resources
- What is a SQL join?