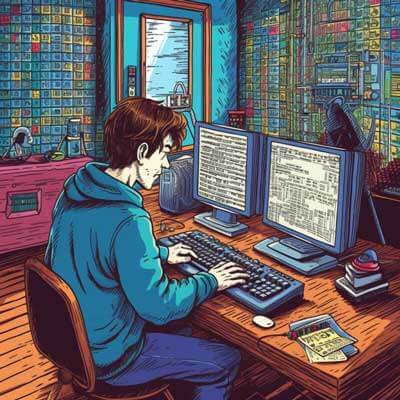
Table of Contents
A full outer join in MySQL allows you to combine the results of two tables, including the unmatched rows from both tables. In other words, it returns all the rows from both tables, matching them where possible and leaving null values where there is no match. This can be useful when you want to compare data from two tables or when you need to find records that exist in one table but not in the other.
Syntax of a Full Outer Join
The syntax of a full outer join in MySQL involves using the UNION
and LEFT JOIN
statements. Here is the general structure:
SELECT * FROM table1 LEFT JOIN table2 ON table1.column = table2.column UNION SELECT * FROM table1 RIGHT JOIN table2 ON table1.column = table2.column WHERE table1.column IS NULL
Let's break down this syntax:
- The LEFT JOIN
statement retrieves all the rows from the left table (table1
), along with the matching rows from the right table (table2
).
- The RIGHT JOIN
statement retrieves all the rows from the right table (table2
), along with the matching rows from the left table (table1
).
- The UNION
statement combines the results of the two SELECT
statements into a single result set.
- The WHERE
clause filters out the rows where the column from table1
is NULL
, which are the unmatched rows from the left table.
Related Article: How to Check and Change Postgresql's Default Port
Example: Performing a Full Outer Join
Let's consider the following two tables, customers
and orders
, which we want to join using a full outer join:
Table: customers
| customer_id | customer_name | |-------------|---------------| | 1 | John | | 2 | Jane | | 3 | Mike |
Table: orders
| order_id | customer_id | order_date | |----------|-------------|-------------| | 1 | 1 | 2022-01-01 | | 2 | 2 | 2022-02-01 | | 3 | 3 | 2022-03-01 | | 4 | 4 | 2022-04-01 |
To perform a full outer join on these tables and retrieve all the rows from both tables, you can use the following query:
SELECT customers.customer_id, customers.customer_name, orders.order_id, orders.order_date FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id UNION SELECT customers.customer_id, customers.customer_name, orders.order_id, orders.order_date FROM customers RIGHT JOIN orders ON customers.customer_id = orders.customer_id WHERE customers.customer_id IS NULL
This query will return the following result:
| customer_id | customer_name | order_id | order_date | |-------------|---------------|----------|------------| | 1 | John | 1 | 2022-01-01 | | 2 | Jane | 2 | 2022-02-01 | | 3 | Mike | 3 | 2022-03-01 | | NULL | NULL | 4 | 2022-04-01 |
In this result, you can see that all the rows from the customers
table and the orders
table are included, even though there is no matching customer for the order with order_id
4.
Alternative Approach: Using UNION ALL and NULL Columns
Another approach to perform a full outer join in MySQL is by using the UNION ALL
statement and adding NULL columns to match the structure of the two tables. Here is an example:
SELECT customers.customer_id, customers.customer_name, orders.order_id, orders.order_date FROM customers LEFT JOIN orders ON customers.customer_id = orders.customer_id UNION ALL SELECT customers.customer_id, customers.customer_name, orders.order_id, orders.order_date FROM customers RIGHT JOIN orders ON customers.customer_id = orders.customer_id WHERE customers.customer_id IS NULL AND orders.order_id IS NULL
This query will produce the same result as the previous example.
Best Practices
When using a full outer join in MySQL, consider the following best practices:
- Use proper indexing: Ensure that the columns used for joining the tables are indexed to optimize the join performance.
- Be cautious with large result sets: Full outer joins can potentially produce large result sets, especially when joining large tables. Make sure to limit the number of rows returned or consider filtering the data before joining.
- Understand the data: Before performing a full outer join, thoroughly analyze the data in both tables to ensure that you are joining the correct columns and that the result set aligns with your expectations.