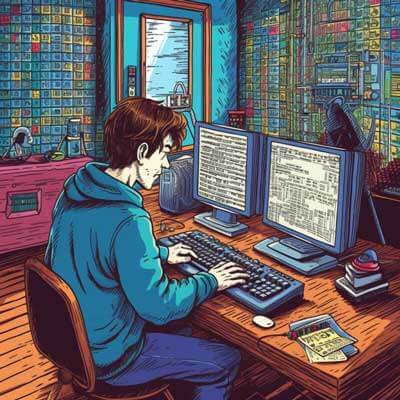
Table of Contents
Enumeration Types in Java: An Overview
Enumeration types, also known as enums, are a special type of data structure in Java that allow you to define a fixed set of constants. These constants are called enum constants and are typically used to represent a specific set of values that are related to each other.
In Java, enumeration types are declared using the enum keyword. Here's an example of how you can declare an enum type in Java:
enum Season { SPRING, SUMMER, AUTUMN, WINTER }
In this example, we have defined an enum type called "Season" that represents the four seasons of the year. The enum constants SPRING, SUMMER, AUTUMN, and WINTER are the possible values that a variable of type Season can take.
Enum types in Java are considered to be a special kind of class. They can have constructors, methods, and fields just like regular classes. However, enum constants are implicitly public, static, and final, which means they cannot be reassigned or extended.
Enums are often used when you have a fixed set of options to choose from. They provide a more structured and type-safe way of representing a set of related values compared to using plain constants or integers.
Let's take a look at an example that demonstrates how to use enum types in Java:
enum DayOfWeek { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY } public class Main { public static void main(String[] args) { DayOfWeek today = DayOfWeek.MONDAY; if (today == DayOfWeek.MONDAY || today == DayOfWeek.FRIDAY) { System.out.println("It's the start or end of the workweek!"); } else { System.out.println("It's a regular day."); } } }
In this example, we define an enum type called "DayOfWeek" that represents the days of the week. We then declare a variable called "today" of type DayOfWeek and assign it the value of DayOfWeek.MONDAY. We can then use this variable in an if statement to determine whether it's the start or end of the workweek.
Enums provide a more expressive and readable way of working with a fixed set of values compared to using plain constants or integers. They also help prevent errors by restricting the possible values that a variable can take.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Understanding Data Structures in Java
Data structures are a fundamental concept in computer science and programming. They are used to organize and store data in a way that allows for efficient operations and retrieval. In Java, there are several built-in data structures that you can use, such as arrays, lists, sets, maps, and queues.
Each data structure has its own strengths and weaknesses, and choosing the right one depends on the specific requirements of your program. Here's a brief overview of some commonly used data structures in Java:
1. Arrays: An array is a fixed-size, contiguous block of memory that stores a collection of elements of the same type. You can access elements in an array using their index, which is an integer value that represents their position in the array. Arrays in Java are zero-based, which means the first element is at index 0.
int[] numbers = new int[5]; numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5;
2. Lists: A list is an ordered collection of elements that allows for duplicates and maintains the insertion order. The two most commonly used list implementations in Java are ArrayList and LinkedList.
List names = new ArrayList(); names.add("Alice"); names.add("Bob"); names.add("Charlie");
3. Sets: A set is an unordered collection of unique elements. It does not allow duplicates and does not maintain any specific order. The two most commonly used set implementations in Java are HashSet and TreeSet.
Set numbers = new HashSet(); numbers.add(1); numbers.add(2); numbers.add(3);
4. Maps: A map is a collection of key-value pairs, where each key is unique. It provides fast lookup and retrieval of values based on their keys. The two most commonly used map implementations in Java are HashMap and TreeMap.
Map scores = new HashMap(); scores.put("Alice", 100); scores.put("Bob", 90); scores.put("Charlie", 80);
5. Queues: A queue is a collection that follows the FIFO (First-In-First-Out) principle, where the first element added is the first one to be removed. The two most commonly used queue implementations in Java are LinkedList and PriorityQueue.
Queue names = new LinkedList(); names.offer("Alice"); names.offer("Bob"); names.offer("Charlie");
These are just a few examples of the data structures available in Java. Each data structure has its own advantages and use cases, and understanding them is essential for writing efficient and maintainable code.
Declaring Variables in Java: Best Practices
In Java, variables are used to store data that can be modified during the execution of a program. When declaring variables in Java, it's important to follow certain best practices to ensure code readability, maintainability, and to avoid common pitfalls.
Here are some best practices for declaring variables in Java:
1. Use meaningful and descriptive names: Choose variable names that accurately describe the data they represent. Avoid using single-letter variable names or cryptic abbreviations, as they can make the code harder to understand.
// Bad example int x = 5; // Good example int age = 25;
2. Declare variables close to their usage: Declare variables as close as possible to where they are first used. This improves code readability and reduces the chances of accidentally using the wrong value or forgetting to initialize a variable.
// Bad example int x; // ... x = 5; // Good example int age = 25;
3. Initialize variables when declaring them: Always initialize variables when declaring them, even if it's with a default value. This ensures that the variable has a valid value before it is used.
// Bad example int count; // ... count = getCount(); // Good example int count = getCount();
4. Limit variable scope: Declare variables with the narrowest possible scope. This reduces the chances of accidental modification and improves code readability.
// Bad example for (int i = 0; i < 10; i++) { // ... } // Good example int count = 0; for (int i = 0; i < 10; i++) { // ... count++; }
5. Use final keyword for constants: If a variable's value should not be changed after initialization, declare it as final. This improves code readability and prevents accidental modifications.
// Bad example int PI = 3.14; // ... PI = 3.14159; // Good example final double PI = 3.14;
These best practices can help improve the readability, maintainability, and reliability of your Java code. By following these guidelines, you can write code that is easier to understand and less prone to bugs.
Difference between Primitive Types and Objects in Java
In Java, there are two main categories of types: primitive types and reference types (also known as objects). Understanding the differences between these two types is important for writing efficient and correct Java code.
Primitive types are basic data types that are built into the Java language. They represent simple values and are not objects. There are eight primitive types in Java:
1. boolean: Represents the boolean values true and false.
2. byte: Represents a signed 8-bit integer.
3. short: Represents a signed 16-bit integer.
4. int: Represents a signed 32-bit integer.
5. long: Represents a signed 64-bit integer.
6. float: Represents a single-precision floating-point number.
7. double: Represents a double-precision floating-point number.
8. char: Represents a single Unicode character.
Primitive types have a fixed size and default values. They are passed by value, which means that when you assign a primitive variable to another variable or pass it as an argument to a method, a copy of its value is made.
On the other hand, objects are instances of classes or arrays. They are created using the new keyword and can have fields and methods. Objects are passed by reference, which means that when you assign an object variable to another variable or pass it as an argument to a method, a reference to the object is passed.
One important difference between primitive types and objects is that primitive types are stored on the stack, while objects are stored on the heap. The stack is used for local variables and method calls, while the heap is used for dynamically allocated memory.
Another difference is that primitive types cannot be null, while objects can be null. This means that you can assign the value null to an object variable to indicate that it does not refer to any object.
Here's an example that demonstrates the difference between primitive types and objects:
int x = 5; int y = x; y = 10; System.out.println(x); // Output: 5 String s1 = "Hello"; String s2 = s1; s2 = "World"; System.out.println(s1); // Output: Hello
In this example, the value of x is copied to y, so changing the value of y does not affect x. On the other hand, s1 and s2 both refer to the same string object, so changing the value of s2 does not affect s1.
Understanding the differences between primitive types and objects is important for writing efficient and correct Java code. By choosing the appropriate type for your data and understanding how they are stored and passed, you can write code that is more readable, efficient, and reliable.
Related Article: Java Classloader: How to Load Classes in Java
Creating Arrays in Java: A Step-by-Step Guide
Arrays are a fundamental data structure in Java that allow you to store multiple values of the same type in a single variable. They provide a convenient way to work with collections of data and are widely used in Java programming.
Here's a step-by-step guide on how to create arrays in Java:
Step 1: Declare the array variable. The array variable is used to refer to the array object. It is declared by specifying the type of the elements followed by square brackets [].
int[] numbers;
Step 2: Create the array object. The array object is created using the new keyword, followed by the type of the elements and the number of elements in the array. The number of elements can be specified as a literal value or as a variable.
numbers = new int[5];
Step 3: Initialize the array elements. The elements of the array are initialized using the assignment operator =. You can assign values to individual elements using their index, which starts at 0.
numbers[0] = 1; numbers[1] = 2; numbers[2] = 3; numbers[3] = 4; numbers[4] = 5;
Alternatively, you can initialize the array elements at the time of creation using an array initializer. An array initializer is a comma-separated list of values enclosed in curly braces {}.
int[] numbers = {1, 2, 3, 4, 5};
Step 4: Access and modify array elements. You can access individual elements of an array using their index. Array elements are accessed using the array variable name followed by the index enclosed in square brackets [].
int firstNumber = numbers[0]; numbers[0] = 10;
Step 5: Use the array in your code. Once the array is created and initialized, you can use it in your code just like any other variable. You can iterate over the elements of the array using a loop or perform operations on the array elements.
for (int i = 0; i < numbers.length; i++) { System.out.println(numbers[i]); }
Arrays are a useful and flexible data structure in Java that allow you to store and manipulate collections of data. By following these steps, you can create and work with arrays in your Java programs.
Classes in Java: Explained with Examples
Classes are a fundamental concept in object-oriented programming and play a central role in Java. They are used to define objects, which are instances of a particular class. Understanding classes is essential for writing object-oriented Java programs.
Here's an explanation of classes in Java, along with examples:
A class is a blueprint or template for creating objects. It defines the properties and behaviors that objects of that class will have. The properties of a class are represented by variables, called fields, and the behaviors are represented by methods.
Here's an example of a simple class in Java:
public class Person { private String name; private int age; public Person(String name, int age) { this.name = name; this.age = age; } public String getName() { return name; } public int getAge() { return age; } public void sayHello() { System.out.println("Hello, my name is " + name + " and I'm " + age + " years old."); } }
In this example, we define a class called Person. It has two private fields, name and age, which represent the properties of a person. We also have a constructor that takes the name and age as parameters and initializes the fields.
The class also has three methods: getName, getAge, and sayHello. The getName and getAge methods are getter methods that return the values of the name and age fields, respectively. The sayHello method prints a greeting message to the console.
To create an object of the Person class, we use the new keyword followed by the class name and the constructor arguments:
Person person = new Person("Alice", 25);
We can then access the fields and methods of the object using the dot operator:
String name = person.getName(); int age = person.getAge(); person.sayHello();
Classes in Java provide a way to model real-world entities and define their properties and behaviors. By creating objects from classes, you can work with data and perform operations in a structured and organized manner.
Interfaces in Java: Key Concepts and Usage
Interfaces are a useful feature of the Java programming language that allow you to define a contract for classes to follow. They provide a way to achieve abstraction, polymorphism, and loose coupling in Java programs. Understanding interfaces is essential for writing flexible and reusable code.
Here's an explanation of interfaces in Java, along with examples:
An interface is a collection of abstract methods, which are methods without a body. It defines a contract that classes implementing the interface must follow. An interface can also contain constant fields, default methods, and static methods.
Here's an example of a simple interface in Java:
public interface Shape { double getArea(); double getPerimeter(); }
In this example, we define an interface called Shape. It contains two abstract methods, getArea and getPerimeter, which define the contract for classes implementing the Shape interface. Any class that implements the Shape interface must provide an implementation for these methods.
To implement an interface, a class must use the implements keyword followed by the interface name. The class must provide an implementation for all the abstract methods defined in the interface.
Here's an example of a class implementing the Shape interface:
public class Circle implements Shape { private double radius; public Circle(double radius) { this.radius = radius; } @Override public double getArea() { return Math.PI * radius * radius; } @Override public double getPerimeter() { return 2 * Math.PI * radius; } }
In this example, we define a class called Circle that implements the Shape interface. It provides an implementation for the getArea and getPerimeter methods defined in the Shape interface.
Interfaces in Java provide a way to define a contract for classes to follow. They allow for abstraction, polymorphism, and loose coupling, making code more flexible and reusable. By using interfaces effectively, you can write code that is more modular, extensible, and maintainable.
Introduction to Generic Types in Java
Generic types, also known as parameterized types, are a useful feature of the Java programming language that allow you to create classes and methods that can work with different types. They provide a way to write reusable and type-safe code.
Here's an introduction to generic types in Java, along with examples:
A generic type is a class or interface that can operate on different types. It is declared using angle brackets and a type parameter. The type parameter is a placeholder for a specific type that will be provided when creating an instance of the generic type.
Here's an example of a simple generic class in Java:
public class Box { private T value; public void setValue(T value) { this.value = value; } public T getValue() { return value; } }
In this example, we define a generic class called Box. It has a type parameter T, which represents the type of the value stored in the box. The setValue and getValue methods can work with any type specified for T.
To create an instance of the Box class with a specific type, we provide the type argument when creating the object:
Box stringBox = new Box(); Box intBox = new Box();
In this example, we create a Box object with the type argument String and another Box object with the type argument Integer. This allows us to create boxes that can store values of different types, while still providing type safety at compile time.
Generic types can also be used with interfaces and methods. Here's an example of a generic interface:
public interface List { void add(T element); T get(int index); }
In this example, we define a generic interface called List. It has a type parameter T, which represents the type of the elements in the list. The add and get methods can work with any type specified for T.
Generic types in Java provide a way to write reusable and type-safe code. They allow you to create classes and methods that can work with different types, while still providing compile-time type checking. By using generic types effectively, you can write code that is more flexible, reusable, and maintainable.
Related Article: How to Generate Random Integers in a Range in Java
Enum Types in Java: Handling Primitive Types
Enum types in Java can handle primitive types as their values. This provides a convenient way to represent a fixed set of related primitive values and perform operations on them. In this section, we will explore how to handle primitive types using enum types in Java.
Here's an example of an enum type that handles primitive types:
enum Size { SMALL(10), MEDIUM(20), LARGE(30); private int value; private Size(int value) { this.value = value; } public int getValue() { return value; } }
In this example, we define an enum type called Size that represents different sizes. The enum constants SMALL, MEDIUM, and LARGE are defined with the corresponding int values of 10, 20, and 30.
The enum type also has a private field value, which stores the int value of each enum constant. It has a private constructor that takes an int parameter and initializes the value field. Finally, it has a getter method getValue that returns the value field.
To use this enum type, you can access the enum constants and their values using the dot operator:
Size size = Size.MEDIUM; int value = size.getValue(); System.out.println(value); // Output: 20
In this example, we create a variable size of type Size and assign it the value Size.MEDIUM. We can then use the getValue method to get the int value of the enum constant.
Enum types in Java provide a convenient way to handle primitive types as their values. They allow you to represent a fixed set of related values and perform operations on them. By using enum types effectively, you can write code that is more expressive, readable, and maintainable.
Enum Types in Java: Working with Objects
Enum types in Java can also work with objects as their values. This provides a flexible and type-safe way to represent a fixed set of related objects and perform operations on them. In this section, we will explore how to work with objects using enum types in Java.
Here's an example of an enum type that works with objects:
enum Color { RED(ColorCode.RED), GREEN(ColorCode.GREEN), BLUE(ColorCode.BLUE); private ColorCode code; private Color(ColorCode code) { this.code = code; } public ColorCode getCode() { return code; } }
In this example, we define an enum type called Color that represents different colors. The enum constants RED, GREEN, and BLUE are defined with the corresponding ColorCode objects.
The enum type also has a private field code, which stores the ColorCode object of each enum constant. It has a private constructor that takes a ColorCode parameter and initializes the code field. Finally, it has a getter method getCode that returns the code field.
To use this enum type, you can access the enum constants and their objects using the dot operator:
Color color = Color.GREEN; ColorCode code = color.getCode(); System.out.println(code); // Output: GREEN
In this example, we create a variable color of type Color and assign it the value Color.GREEN. We can then use the getCode method to get the ColorCode object of the enum constant.
Additional Resources
- Enumerations in Java