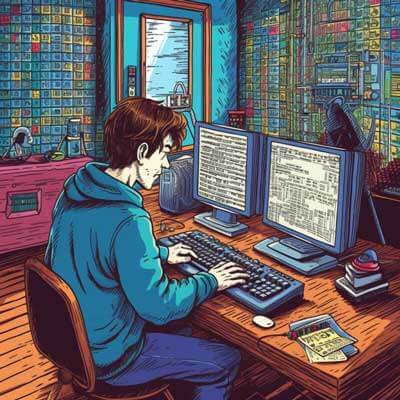
Table of Contents
Introduction to Hibernate
Hibernate is an open-source Java framework that simplifies the process of interacting with relational databases. It provides an object-relational mapping (ORM) solution, allowing developers to work with Java objects instead of SQL queries. This greatly reduces the amount of boilerplate code required and increases productivity.
To understand how Hibernate works, let's consider an example. Suppose we have a Java class called "Product" that represents a table in our database. With Hibernate, we can map this class to the corresponding database table and perform various operations on it, such as saving, updating, and deleting records.
Here's an example of how to define a simple entity class with Hibernate:
@Entity@Table(name = "products")public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @Column(name = "price") private BigDecimal price; // Getters and setters}
In this example, we use annotations to specify the mapping between the class fields and the corresponding database columns. The @Entity
annotation indicates that this class is an entity, and the @Table
annotation specifies the name of the database table.
Related Article: Proper Placement of MySQL Connector JAR File in Java
Setting Up a Hibernate Environment
To get started with Hibernate, we need to set up our development environment. Here are the steps to follow:
1. Add Hibernate as a dependency in your project's build file (e.g., Maven or Gradle). You can find the necessary dependencies on the Hibernate website.
2. Configure the database connection properties in a configuration file (e.g., hibernate.cfg.xml
or application.properties
). This file should contain details such as the database URL, username, password, and the dialect for the database you are using.
3. Create a Hibernate configuration object using the configuration file. Here's an example using the hibernate.cfg.xml
file:
Configuration configuration = new Configuration();configuration.configure("hibernate.cfg.xml");
4. Build a session factory from the configuration object. The session factory is a thread-safe object that provides sessions, which are used to interact with the database. Here's an example:
SessionFactory sessionFactory = configuration.buildSessionFactory();
5. Obtain a session from the session factory. The session represents a single unit of work with the database. Here's an example:
Session session = sessionFactory.openSession();
With these steps, you have set up the basic Hibernate environment and are ready to start working with the database using Hibernate.
Essential Hibernate Components
Hibernate consists of several essential components that work together to provide a powerful ORM solution. Understanding these components is crucial for effectively using Hibernate in your Java projects. Let's explore them briefly:
1. Entity: An entity is a Java class that represents a database table. It is annotated with the @Entity
annotation and typically contains fields that map to the table's columns.
2. Session Factory: The session factory is a heavyweight object that provides sessions. It is responsible for creating and managing database connections, as well as caching metadata. The session factory is created using the configuration information and can be shared across multiple sessions.
3. Session: A session represents a single unit of work with the database. It provides methods for performing CRUD (Create, Retrieve, Update, Delete) operations on entities, as well as querying the database using Hibernate Query Language (HQL) or Criteria API.
4. Transaction: A transaction encapsulates a set of operations that should be treated as a single atomic unit of work. It ensures data consistency and integrity by providing mechanisms for committing or rolling back changes made within the transaction.
5. Configuration: The configuration object represents the configuration settings for Hibernate. It includes information such as the database connection details, mapping files, and other properties.
6. Mapping: Mapping refers to the process of associating a Java class with a database table. Hibernate supports various mapping strategies, including annotations, XML files, and programmatic configuration.
These components work together to provide a seamless integration between your Java code and the underlying database, making it easier to develop and maintain database-driven applications.
Configuring Hibernate
Before we can start using Hibernate, we need to configure it properly. Configuration involves specifying various settings and properties that control the behavior of Hibernate. Let's look at some important configuration options:
1. Database Connection: Hibernate needs to know how to connect to the database. You can specify the database URL, username, password, and other connection properties in the Hibernate configuration file.
2. Dialect: The dialect determines the SQL dialect of the target database. Hibernate uses this information to generate appropriate SQL statements and query optimization techniques. You need to set the dialect that matches the database you are using.
3. Mapping Files or Annotations: Hibernate needs to know how to map your Java classes to database tables. You can use mapping files (XML) or annotations to define the mapping. Make sure to specify the location of the mapping files or enable the scanning of annotations in the Hibernate configuration.
4. Connection Pooling: Connection pooling improves performance by reusing database connections instead of creating a new connection for each session. Hibernate can be configured to use a connection pool such as Apache Commons DBCP or HikariCP.
5. Caching: Hibernate supports various levels of caching to improve performance. You can configure Hibernate to use first-level caching (session-level caching) and second-level caching (shared across sessions). Caching can significantly reduce the number of database queries and improve application performance.
Here's an example of a Hibernate configuration file (hibernate.cfg.xml
) that includes some of these configuration options:
<hibernate-configuration> <session-factory> <property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydatabase</property> <property name="hibernate.connection.username">root</property> <property name="hibernate.connection.password">password</property> <property name="hibernate.dialect">org.hibernate.dialect.MySQL8Dialect</property> <mapping class="com.example.Product"/> <property name="hibernate.connection.pool_size">10</property> <property name="hibernate.cache.provider_class">org.hibernate.cache.EhCacheProvider</property> </session-factory></hibernate-configuration>
In this example, we configure the database connection URL, username, and password. We also specify the dialect for MySQL 8, map the Product
class, set the connection pool size to 10, and enable second-level caching using the EhCache provider.
By configuring Hibernate correctly, you can tailor its behavior to suit your application's needs and take advantage of its powerful features.
Related Article: How to Sort a List of ArrayList in Java
Building Entity Classes in Hibernate
Entity classes are at the core of Hibernate's object-relational mapping (ORM) functionality. These classes represent database tables and provide a convenient way to interact with the underlying data. In this section, we'll explore the process of building entity classes in Hibernate.
To define an entity class, follow these steps:
1. Annotate the class with @Entity
to indicate that it is an entity.
2. Specify the table name using the @Table
annotation. By default, Hibernate assumes that the table name matches the entity class name.
3. Define the class fields and annotate them with appropriate annotations to specify the mapping to the corresponding database columns.
Let's consider an example where we have an entity class called Product
that maps to a products
table in the database. The table has columns for id
, name
, and price
. Here's how we can define the Product
entity class:
@Entity@Table(name = "products")public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @Column(name = "price") private BigDecimal price; // Getters and setters}
In this example, we use annotations to specify the mapping between the class fields and the corresponding database columns. The @Id
annotation indicates the primary key field, and the @GeneratedValue
annotation with the GenerationType.IDENTITY
strategy specifies that the primary key values are automatically generated by the database.
The @Column
annotation is used to specify the mapping for each field. By default, Hibernate assumes that the field name matches the column name, but you can override this behavior by specifying the name
attribute.
With this entity class defined, we can now use Hibernate to perform various operations on the products
table, such as saving, updating, and deleting records.
Implementing Hibernate Sessions
In Hibernate, a session represents a single unit of work with the database. It provides methods for performing CRUD (Create, Retrieve, Update, Delete) operations on entities, as well as querying the database using Hibernate Query Language (HQL) or the Criteria API.
To work with sessions in Hibernate, follow these steps:
1. Obtain a session from the session factory. The session factory is a heavyweight object that provides sessions. You can obtain a session using the openSession()
method of the session factory.
Session session = sessionFactory.openSession();
2. Begin a transaction. Transactions ensure that a set of operations is treated as a single atomic unit of work. You can start a transaction using the beginTransaction()
method of the session.
Transaction transaction = session.beginTransaction();
3. Perform operations on entities. Use the session's methods to save, update, or delete entities, or retrieve entities by their identifier or using queries.
// Save a new productProduct product = new Product();product.setName("Example Product");product.setPrice(BigDecimal.valueOf(9.99));session.save(product);// Retrieve a product by idProduct retrievedProduct = session.get(Product.class, 1L);// Update a productretrievedProduct.setName("New Product Name");session.update(retrievedProduct);// Delete a productsession.delete(retrievedProduct);
4. Commit or rollback the transaction. After performing the necessary operations, you can commit the transaction to persist the changes to the database, or rollback the transaction to discard the changes.
transaction.commit(); // Commit the transaction// ortransaction.rollback(); // Rollback the transaction
5. Close the session. Once you have completed your work with the session, make sure to close it to release any acquired resources.
session.close();
Management of Hibernate Transactions
Transactions play a crucial role in maintaining data consistency and integrity in a database. In Hibernate, transactions are used to group a set of operations that should be treated as a single atomic unit of work. To manage transactions effectively, follow these steps:
1. Begin a transaction. Use the beginTransaction()
method of the session to start a transaction.
Transaction transaction = session.beginTransaction();
2. Perform operations on entities. Within the transaction, you can perform various operations on entities, such as saving, updating, or deleting records.
Product product = new Product();product.setName("Example Product");product.setPrice(BigDecimal.valueOf(9.99));session.save(product);
3. Commit the transaction. Once you have completed the necessary operations, you can commit the transaction to persist the changes to the database.
transaction.commit();
4. Rollback the transaction (if necessary). In case an error or exception occurs during the transaction, you can rollback the transaction to discard the changes made so far.
transaction.rollback();
It is important to note that Hibernate provides automatic transaction management when used in a managed environment (e.g., Java EE container). In such cases, you don't need to explicitly begin or commit transactions as the container takes care of it.
However, in a standalone Java application, you need to manage transactions manually as shown above.
CRUD Operations with Hibernate
CRUD (Create, Retrieve, Update, Delete) operations are fundamental to working with databases. Hibernate provides convenient methods to perform these operations on entities. Let's explore how to perform CRUD operations with Hibernate:
1. Create (Save) Operation:
To save a new entity to the database, use the save()
method of the session:
Product product = new Product();product.setName("Example Product");product.setPrice(BigDecimal.valueOf(9.99));session.save(product);
2. Retrieve (Read) Operation:
To retrieve an entity by its identifier, use the get()
or load()
method of the session:
Product retrievedProduct = session.get(Product.class, 1L);
The get()
method returns null
if the entity does not exist, while the load()
method throws an exception.
3. Update Operation:
To update an existing entity, simply modify its properties and use the update()
method of the session:
retrievedProduct.setName("New Product Name");session.update(retrievedProduct);
Hibernate tracks changes to the entity and updates the corresponding database record when the transaction is committed.
4. Delete Operation:
To delete an entity, use the delete()
method of the session:
session.delete(retrievedProduct);
Hibernate removes the corresponding database record when the transaction is committed.
By leveraging these CRUD operations, you can effectively manage the persistence of entities in the database using Hibernate.
Related Article: How to Retrieve Current Date and Time in Java
Use Case: Implementing a One-to-Many Relationship
One-to-many relationships are common in database modeling, where one entity is associated with multiple instances of another entity. In Hibernate, we can represent this relationship using collections. Let's consider an example where we have a Department
entity that can have multiple Employee
entities associated with it.
Here's how we can define the entities with the one-to-many relationship:
1. Define the Department
entity:
@Entity@Table(name = "departments")public class Department { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @OneToMany(mappedBy = "department", cascade = CascadeType.ALL, orphanRemoval = true) private List<Employee> employees = new ArrayList<>(); // Getters and setters}
In this example, we use the @OneToMany
annotation to specify the one-to-many relationship. The mappedBy
attribute specifies that the Department
entity is the inverse side of the relationship, and the cascade
attribute ensures that any changes made to the department are cascaded to the associated employees. The orphanRemoval
attribute ensures that any employees not associated with a department are removed.
2. Define the Employee
entity:
@Entity@Table(name = "employees")public class Employee { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @ManyToOne(fetch = FetchType.LAZY) @JoinColumn(name = "department_id") private Department department; // Getters and setters}
In this example, we use the @ManyToOne
annotation to specify the many-to-one relationship. The fetch
attribute defines the fetching strategy, and the JoinColumn
annotation specifies the foreign key column.
With these entities defined, we can now perform operations that involve the one-to-many relationship. For example, to add an employee to a department:
Department department = new Department();department.setName("Engineering");Employee employee1 = new Employee();employee1.setName("John Doe");employee1.setDepartment(department);Employee employee2 = new Employee();employee2.setName("Jane Smith");employee2.setDepartment(department);department.getEmployees().add(employee1);department.getEmployees().add(employee2);session.save(department);
In this example, we create a department and two employees. We associate the employees with the department by setting the department
property. We also add the employees to the department's employees
collection. When we save the department, Hibernate automatically persists the associated employees.
By leveraging the one-to-many relationship in Hibernate, you can model and manage complex associations between entities effectively.
Use Case: Implementing a Many-to-Many Relationship
Many-to-many relationships occur when multiple instances of one entity are associated with multiple instances of another entity. In Hibernate, we can represent this relationship using collections. Let's consider an example where we have a Student
entity that can be enrolled in multiple Course
entities, and a Course
entity that can have multiple students enrolled.
Here's how we can define the entities with the many-to-many relationship:
1. Define the Student
entity:
@Entity@Table(name = "students")public class Student { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @ManyToMany(mappedBy = "students") private List<Course> courses = new ArrayList<>(); // Getters and setters}
In this example, we use the @ManyToMany
annotation to specify the many-to-many relationship. The mappedBy
attribute specifies that the Student
entity is the inverse side of the relationship.
2. Define the Course
entity:
@Entity@Table(name = "courses")public class Course { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @ManyToMany @JoinTable( name = "course_student", joinColumns = @JoinColumn(name = "course_id"), inverseJoinColumns = @JoinColumn(name = "student_id") ) private List<Student> students = new ArrayList<>(); // Getters and setters}
In this example, we use the @ManyToMany
annotation to specify the many-to-many relationship. We also use the @JoinTable
annotation to define the join table that holds the association between the Course
and Student
entities.
With these entities defined, we can now perform operations that involve the many-to-many relationship. For example, to enroll a student in a course:
Student student = new Student();student.setName("John Doe");Course course = new Course();course.setName("Java Programming");student.getCourses().add(course);course.getStudents().add(student);session.save(student);
In this example, we create a student and a course. We associate the student with the course by adding the course to the student's courses
collection, and vice versa. When we save the student, Hibernate automatically persists the associated course.
By leveraging the many-to-many relationship in Hibernate, you can model and manage complex associations between entities effectively.
Best Practice: Writing Efficient HQL Queries
Hibernate Query Language (HQL) is a powerful object-oriented query language that allows you to express database queries in terms of entities and their properties. Writing efficient HQL queries is important for optimizing performance and minimizing database round-trips. Here are some best practices to follow when writing HQL queries:
1. Use Lazy Fetching: By default, Hibernate fetches associated entities lazily, meaning that the associated entities are not loaded until accessed. Lazy fetching can significantly improve performance by reducing the amount of data fetched from the database. However, be aware of potential N+1 select problems when accessing lazy associations in a loop.
2. Use Joins Instead of Multiple Queries: If you need to fetch multiple entities and their associations, consider using joins instead of separate queries. Joins allow you to retrieve all the necessary data in a single database query, reducing the number of round-trips to the database.
3. Use Pagination for Large Result Sets: When fetching a large number of entities, consider using pagination to limit the number of results returned. This can prevent performance issues caused by loading a large amount of data into memory.
4. Use Query Caching: Hibernate provides query caching to improve performance by caching the results of queries. By enabling query caching, subsequent executions of the same query can be served from the cache instead of hitting the database. Use query caching for queries that are executed frequently but return relatively static data.
5. Avoid Cartesian Products: Be cautious when using joins and ensure that the join conditions are properly defined. Cartesian products occur when the join conditions are missing or incorrect, resulting in an incorrect number of rows being returned. Cartesian products can have a severe impact on performance and should be avoided.
6. Use Named Parameters: When writing parameterized queries, use named parameters instead of positional parameters. Named parameters make the query more readable and maintainable, especially when dealing with complex queries with multiple parameters.
7. Consider Indexing: If you frequently query based on a specific column, consider adding an index to improve query performance. Indexing can greatly reduce the time taken to retrieve data from the database.
Here's an example of an HQL query that demonstrates some of these best practices:
String hql = "SELECT p FROM Product p JOIN FETCH p.category WHERE p.price > :price";List<Product> products = session.createQuery(hql, Product.class) .setParameter("price", BigDecimal.valueOf(10.0)) .setMaxResults(100) .list();
In this example, we use a join to fetch the Product
entities along with their associated Category
entities. We use a named parameter price
to filter the products based on price. We also limit the result set to 100 products using setMaxResults()
.
Best Practice: Enhancing Performance with Second-Level Caching
Second-level caching is a powerful feature provided by Hibernate that can greatly enhance performance by caching entities and query results across sessions. Here are some best practices to follow when using second-level caching in Hibernate:
1. Enable Second-Level Caching: To use second-level caching, you need to enable it in the Hibernate configuration. Set the hibernate.cache.use_second_level_cache
property to true
.
2. Configure the Cache Provider: Hibernate supports various cache providers such as Ehcache, Infinispan, and Hazelcast. Choose a cache provider that suits your needs and configure it in the Hibernate configuration.
3. Enable Caching for Entities: To enable second-level caching for an entity, annotate it with the @Cacheable
annotation. This tells Hibernate to cache instances of the entity.
@Entity@Table(name = "products")@Cacheablepublic class Product { // ...}
4. Configure Caching Strategies: Hibernate provides different caching strategies, such as read-only, read-write, and transactional. Choose the appropriate caching strategy based on the requirements of your application.
5. Use Query Caching: Hibernate allows you to cache the results of queries using the @QueryHint
annotation. By enabling query caching, subsequent executions of the same query can be served from the cache instead of hitting the database.
@NamedQuery( name = "Product.findAll", query = "SELECT p FROM Product p", hints = @QueryHint(name = "org.hibernate.cacheable", value = "true"))
6. Monitor Cache Performance: Keep an eye on the cache performance by monitoring cache hit rates, memory usage, and eviction policies. Adjust the cache settings as necessary to optimize performance.
7. Use Cache Regions: Cache regions allow you to group related entities and queries together. By using cache regions, you can fine-tune the caching behavior for different parts of your application.
@Cache(usage = CacheConcurrencyStrategy.READ_WRITE, region = "products")@Entity@Table(name = "products")public class Product { // ...}
Related Article: Java Set Tutorial
Real World Example: Building a Shopping Cart with Hibernate
In this real-world example, we will demonstrate how to build a simple shopping cart using Hibernate. The shopping cart will allow users to add products, update quantities, and remove products. The cart data will be persisted in the database using Hibernate.
1. Define the Product
entity:
@Entity@Table(name = "products")public class Product { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "name") private String name; @Column(name = "price") private BigDecimal price; // Getters and setters}
2. Define the CartItem
entity:
@Entity@Table(name = "cart_items")public class CartItem { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @ManyToOne(fetch = FetchType.LAZY) @JoinColumn(name = "product_id") private Product product; @Column(name = "quantity") private int quantity; // Getters and setters}
3. Define the Cart
entity:
@Entity@Table(name = "carts")public class Cart { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @OneToMany(mappedBy = "cart", cascade = CascadeType.ALL, orphanRemoval = true) private List<CartItem> cartItems = new ArrayList<>(); // Getters and setters}
4. Implement the shopping cart functionality:
// Create a new cartCart cart = new Cart();// Add a product to the cartProduct product = session.get(Product.class, 1L);CartItem cartItem = new CartItem();cartItem.setProduct(product);cartItem.setQuantity(1);cartItem.setCart(cart);cart.getCartItems().add(cartItem);// Update the quantity of a cart itemCartItem cartItemToUpdate = cart.getCartItems().get(0);cartItemToUpdate.setQuantity(2);// Remove a cart itemCartItem cartItemToRemove = cart.getCartItems().get(0);cart.getCartItems().remove(cartItemToRemove);// Save the cartsession.save(cart);
In this example, we create a Cart
entity and associate it with one or more CartItem
entities. Each CartItem
represents a product in the cart with a specific quantity. We can add, update, and remove cart items by manipulating the cartItems
collection of the Cart
entity. Finally, we save the Cart
entity to persist the cart data in the database.
Real World Example: Managing User Authentication with Hibernate
In this real-world example, we will demonstrate how to manage user authentication using Hibernate. We will implement a basic user authentication system that allows users to register, log in, and log out. User credentials will be stored in the database using Hibernate.
1. Define the User
entity:
@Entity@Table(name = "users")public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; @Column(name = "username", unique = true) private String username; @Column(name = "password") private String password; // Getters and setters}
2. Implement the user authentication functionality:
// User registrationUser user = new User();user.setUsername("john.doe");user.setPassword("password123");session.save(user);// User loginString username = "john.doe";String password = "password123";User loggedInUser = session.createQuery("FROM User WHERE username = :username AND password = :password", User.class) .setParameter("username", username) .setParameter("password", password) .uniqueResult();if (loggedInUser != null) { // User authenticated // Perform further actions} else { // Invalid credentials}// User logout// Simply clear the current sessionsession.clear();
In this example, we create a User
entity with a unique username and a password. We save the user entity to the database during the registration process. During the login process, we retrieve the user from the database based on the provided username and password. If a matching user is found, the user is considered authenticated. If the user is not found or the password is incorrect, the login attempt fails. To log out, we simply clear the current session.
Performance Consideration: Optimizing Session Management
Managing Hibernate sessions efficiently is crucial for achieving optimal performance. Here are some performance considerations and best practices for optimizing session management:
1. Use Short-Lived Sessions: Hibernate sessions should be kept open for the shortest possible duration. Open a session, perform the necessary operations, and close the session promptly. Holding sessions open for a long time can lead to resource contention and degrade performance.
2. Use Session-per-Request Pattern: In web applications, it is common to use the session-per-request pattern, where a new session is opened at the beginning of a request and closed at the end of the request. This ensures that each request is isolated and has its own session.
3. Use Connection Pooling: Hibernate supports connection pooling, which allows you to reuse database connections instead of creating a new connection for each session. Connection pooling reduces the overhead of creating and closing connections, resulting in improved performance.
4. Use Read-Only Transactions: If you only need to read data from the database and don't intend to modify it, consider using read-only transactions. Read-only transactions can improve performance by reducing the amount of overhead associated with transaction management.
5. Batch Database Operations: If you need to perform multiple database operations within a single transaction, consider batching them together. Batch processing reduces the number of round-trips to the database and can significantly improve performance.
6. Avoid Session Flushes: Hibernate automatically flushes the session before executing any query to ensure that the database and session state are synchronized. However, frequent session flushes can impact performance. If possible, defer the session flush until the end of the transaction or request.
7. Use Stateless Sessions: Hibernate provides stateless sessions that do not track changes to entities. Stateless sessions are more lightweight and can provide better performance for read-only operations or bulk data processing.
8. Monitor Session Cache Size: Hibernate maintains a first-level cache (session cache) to improve performance. However, the session cache consumes memory, and large cache sizes can impact performance. Monitor the session cache size and consider evicting entities manually if necessary.
Performance Consideration: Reducing Database Hits with Batch Processing
Reducing the number of database hits is crucial for improving the performance of Hibernate-based applications. Batch processing allows you to combine multiple database operations into a single batch, reducing the number of round-trips to the database. Here's how you can reduce database hits using batch processing in Hibernate:
1. Enable Batch Processing: To enable batch processing, you need to configure Hibernate to use batch updates. Set the hibernate.jdbc.batch_size
property to the desired batch size in the Hibernate configuration.
<hibernate-configuration> <session-factory> <!-- ... other configurations ... --> <property name="hibernate.jdbc.batch_size">50</property> </session-factory></hibernate-configuration>
2. Group Database Operations: Instead of executing database operations one by one, batch them together and execute them as a single batch. Use the save()
, update()
, and delete()
methods of the session to add entities to the batch.
for (int i = 0; i < 1000; i++) { Product product = new Product(); product.setName("Product " + i); session.save(product); if (i % 50 == 0) { session.flush(); // Flush the batch session.clear(); // Clear the batch }}
In this example, we save 1000 Product
entities to the database. After every 50 entities, we flush and clear the session to execute the batch. This reduces the number of round-trips to the database from 1000 to 20.
3. Use Stateless Sessions for Bulk Operations: Hibernate provides stateless sessions that do not track changes to entities. Stateless sessions are more lightweight and can provide better performance for bulk data processing. Use stateless sessions when performing bulk inserts, updates, or deletes.
Session session = sessionFactory.openStatelessSession();Transaction transaction = session.beginTransaction();for (int i = 0; i < 1000; i++) { Product product = new Product(); product.setName("Product " + i); session.insert(product); if (i % 50 == 0) { session.flush(); // Flush the batch session.clear(); // Clear the batch }}transaction.commit();session.close();
In this example, we use a stateless session to insert 1000 Product
entities to the database. We flush and clear the batch after every 50 entities to execute the batch.
By implementing batch processing in Hibernate, you can significantly reduce the number of database hits and improve the performance of your applications.
Related Article: How to Find the Max Value of an Integer in Java
Advanced Technique: Implementing Inheritance in Hibernate
Inheritance is a fundamental concept in object-oriented programming. Hibernate provides various strategies to map inheritance hierarchies to database tables. Here are the different inheritance mapping strategies in Hibernate:
1. Single Table Inheritance: In single table inheritance, all the classes in the inheritance hierarchy are mapped to a single database table. Discriminator columns are used to differentiate between different types of entities.
2. Table Per Class Inheritance: In table per class inheritance, each class in the inheritance hierarchy is mapped to a separate database table. Common properties are duplicated across tables, leading to a denormalized schema.
3. Joined Table Inheritance: In joined table inheritance, each class in the inheritance hierarchy is mapped to a separate database table. Common properties are stored in a shared table, and subclass tables contain only their specific properties.
To implement inheritance in Hibernate, follow these steps:
1. Define the base class with the @Entity
and @Inheritance
annotations:
@Entity@Inheritance(strategy = InheritanceType.XXX)public abstract class BaseEntity { // Common properties and methods}
Replace InheritanceType.XXX
with the desired inheritance mapping strategy (SINGLE_TABLE
, TABLE_PER_CLASS
, or JOINED
).
2. Define the subclasses with the @Entity
annotation:
@Entitypublic class SubClass extends BaseEntity { // Subclass-specific properties and methods}
Repeat this step for each subclass in the inheritance hierarchy.
3. Configure the inheritance mapping in the Hibernate configuration file:
<hibernate-configuration> <session-factory> <!-- ... other configurations ... --> <mapping class="com.example.BaseEntity"/> <mapping class="com.example.SubClass"/> <!-- Add mappings for other subclasses --> </session-factory></hibernate-configuration>
Replace com.example.BaseEntity
and com.example.SubClass
with the appropriate package and class names.
By implementing inheritance in Hibernate, you can model complex class hierarchies and map them to the database efficiently.
Advanced Technique: Using Hibernate Criteria Queries
Hibernate Criteria API provides a programmatic and type-safe way to build database queries using a fluent API. Criteria queries are particularly useful for dynamic queries where the query parameters are not known at compile-time. Here's how you can use Hibernate Criteria API to build queries:
1. Create a criteria builder and a criteria query:
CriteriaBuilder builder = session.getCriteriaBuilder();CriteriaQuery<Product> query = builder.createQuery(Product.class);Root<Product> root = query.from(Product.class);
2. Specify query conditions using predicates:
Predicate pricePredicate = builder.gt(root.get("price"), BigDecimal.valueOf(10.0));query.where(pricePredicate);
3. Add query projections (optional):
query.select(root.get("name"));
4. Execute the query and retrieve the results:
List<Product> products = session.createQuery(query).getResultList();
In this example, we create a criteria builder and a criteria query for the Product
entity. We specify a condition where the price is greater than a certain value. We select only the product names and execute the query to retrieve the results.
Hibernate Criteria API provides a rich set of methods for building complex queries, including support for joins, aggregations, ordering, and paging. By using the Criteria API, you can create dynamic and type-safe queries that are less error-prone and easier to maintain.
Code Snippet: Creating a Session Factory
Creating a session factory is an essential step in setting up a Hibernate environment. The session factory is a heavyweight object that provides sessions, which are used to interact with the database. Here's an example of how to create a session factory in Hibernate:
Configuration configuration = new Configuration();configuration.configure("hibernate.cfg.xml");SessionFactory sessionFactory = configuration.buildSessionFactory();
In this example, we create a Configuration
object and load the Hibernate configuration from the hibernate.cfg.xml
file. The configuration file contains details such as the database connection properties and mapping information. We then build a session factory from the configuration, which can be shared across multiple sessions.
The session factory is a thread-safe object that caches metadata and provides sessions on demand. It is responsible for managing database connections and transaction coordination.
By creating a session factory, you can obtain sessions and perform various operations on entities using Hibernate.
Code Snippet: Implementing a Transaction
Transactions are crucial for maintaining data consistency and integrity in a database. In Hibernate, transactions are used to group a set of operations that should be treated as a single atomic unit of work. Here's an example of how to implement a transaction in Hibernate:
Session session = sessionFactory.openSession();Transaction transaction = session.beginTransaction();try { // Perform database operations session.save(entity1); session.update(entity2); transaction.commit();} catch (Exception e) { transaction.rollback();} finally { session.close();}
In this example, we open a session from the session factory and begin a transaction using the beginTransaction()
method. We perform various database operations within the transaction, such as saving and updating entities. If an exception occurs, we roll back the transaction to discard the changes made so far. Finally, we close the session.
By implementing transactions in Hibernate, you can ensure data consistency and integrity by grouping related operations into a single unit of work.
Related Article: Identifying the Version of Your MySQL-Connector-Java
Code Snippet: Writing a HQL Query
Hibernate Query Language (HQL) provides a powerful and flexible way to query entities and their associations. HQL queries are similar to SQL queries but operate on entities and their properties. Here's an example of how to write an HQL query in Hibernate:
String hql = "SELECT p FROM Product p WHERE p.price > :price";List<Product> products = session.createQuery(hql, Product.class) .setParameter("price", BigDecimal.valueOf(10.0)) .getResultList();
In this example, we write an HQL query that selects products where the price is greater than a specified value. We use the createQuery()
method of the session to create a query object, specify the HQL query string, and set a named parameter. Finally, we execute the query using getResultList()
to retrieve the results as a list of Product
entities.
HQL queries support various features such as joins, projections, aggregations, and ordering. By leveraging HQL, you can express complex queries in a concise and object-oriented manner.
Code Snippet: Configuring a Second-Level Cache
Hibernate provides second-level caching to improve performance by caching entities and query results across sessions. Configuring a second-level cache involves setting up the cache provider and enabling caching for entities. Here's an example of how to configure a second-level cache in Hibernate:
<hibernate-configuration> <session-factory> <!-- ... other configurations ... --> <!-- Enable second-level cache --> <property name="hibernate.cache.use_second_level_cache">true</property> <!-- Configure cache provider --> <property name="hibernate.cache.provider_class">org.hibernate.cache.EhCacheProvider</property> <!-- Enable caching for entities --> <mapping class="com.example.Product"/> <!-- Add mappings for other entities --> </session-factory></hibernate-configuration>
In this example, we enable second-level caching by setting the hibernate.cache.use_second_level_cache
property to true
. We configure the cache provider to use Ehcache by setting the hibernate.cache.provider_class
property. We then enable caching for the Product
entity by adding its mapping to the Hibernate configuration.
By configuring a second-level cache, Hibernate can cache entities and query results, reducing the need for frequent database accesses and improving performance.
Code Snippet: Building a Criteria Query
Hibernate Criteria API provides a programmatic and type-safe way to build database queries. Criteria queries are especially useful for dynamic queries where the query parameters are not known at compile-time. Here's an example of how to build a criteria query in Hibernate:
CriteriaBuilder builder = session.getCriteriaBuilder();CriteriaQuery<Product> query = builder.createQuery(Product.class);Root<Product> root = query.from(Product.class);// Add query conditions using predicatesPredicate pricePredicate = builder.gt(root.get("price"), BigDecimal.valueOf(10.0));query.where(pricePredicate);// Add query projectionsquery.select(root.get("name"));// Execute the query and retrieve the resultsList<Product> products = session.createQuery(query).getResultList();
In this example, we create a criteria builder and a criteria query for the Product
entity. We specify a condition where the price is greater than a certain value. We select only the product names and execute the query to retrieve the results.
Hibernate Criteria API provides a rich set of methods for building complex queries, including support for joins, aggregations, ordering, and paging. By using the Criteria API, you can create dynamic and type-safe queries that are less error-prone and easier to maintain.
Error Handling in Hibernate
Error handling is an important aspect of any application, including those using Hibernate. When working with Hibernate, you may encounter various exceptions and errors. Here are some common exceptions and their meanings:
1. HibernateException: The base exception class for Hibernate-related exceptions. It indicates a generic error or exception occurred during Hibernate operations.
2. MappingException: This exception is thrown when there is an issue with the mapping configuration, such as an invalid mapping file or incorrect annotations.
3. QueryException: This exception is thrown when there is an issue with the Hibernate query, such as a syntax error or an invalid query parameter.
4. ConstraintViolationException: This exception is thrown when a database constraint is violated, such as a unique key or foreign key constraint.
5. StaleObjectStateException: This exception is thrown when concurrent modification of an entity occurs, typically in a multi-user environment. It indicates that the entity being modified has been changed by another user since it was last loaded into the current session.
When handling exceptions in Hibernate, it is important to consider the context and take appropriate actions. Some common error handling strategies include logging the exception, rolling back the transaction, notifying the user, and retrying the operation.
To handle exceptions in Hibernate, you can use try-catch blocks or exception handling mechanisms provided by your application framework. Additionally, you can leverage Hibernate's exception hierarchy to handle specific exceptions or handle them generically using the base HibernateException
class.
Effective error handling in Hibernate can improve application stability and provide meaningful feedback to users in case of errors or exceptions.