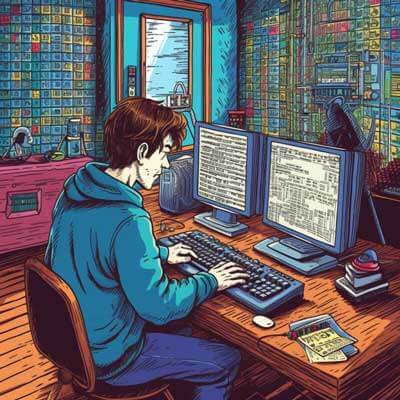
Table of Contents
Introduction to Scanner Class
The Scanner class in Java is a useful tool that allows us to read input from various sources, such as the console, files, or even strings. It provides convenient methods for parsing and extracting different types of data, making it a valuable asset for any Java developer. In this chapter, we will explore the basics of the Scanner class and how to create Scanner objects.
Related Article: Java Do-While Loop Tutorial
Basics of Scanner Class
The Scanner class is part of the java.util package and provides a wide range of methods for reading input. It can handle different types of data, such as integers, floating-point numbers, strings, and more. To use the Scanner class, we need to import it first:
import java.util.Scanner;
Creation of Scanner Objects
To start reading input, we need to create a Scanner object. We can create a Scanner object by passing different sources of input to its constructor. Here are a few examples:
- Reading from the console:
Scanner scanner = new Scanner(System.in);
- Reading from a file:
File file = new File("input.txt"); Scanner scanner = new Scanner(file);
- Reading from a string:
String input = "Hello, World!"; Scanner scanner = new Scanner(input);
Reading Input with Scanner Class
Once we have created a Scanner object, we can start reading input using its various methods. Here are some commonly used methods for reading different types of input:
Related Article: How to Convert a String to Date in Java
Reading Strings
To read a string from the input source, we can use the next()
method. It reads a single word (a sequence of characters separated by whitespace) and returns it as a string.
Scanner scanner = new Scanner(System.in); System.out.print("Enter your name: "); String name = scanner.next(); System.out.println("Hello, " + name + "!");
Reading Integers
To read an integer from the input source, we can use the nextInt()
method. It reads the next token as an integer and returns it.
Scanner scanner = new Scanner(System.in); System.out.print("Enter your age: "); int age = scanner.nextInt(); System.out.println("You are " + age + " years old.");
Reading Doubles
To read a double from the input source, we can use the nextDouble()
method. It reads the next token as a double and returns it.
Scanner scanner = new Scanner(System.in); System.out.print("Enter the temperature: "); double temperature = scanner.nextDouble(); System.out.println("The temperature is " + temperature + " degrees Celsius.");
Use Case: Reading Different Types of Input
Let's consider a use case where we need to read different types of input from the user, such as their name, age, and favorite color. We can use the Scanner class to achieve this:
Scanner scanner = new Scanner(System.in); System.out.print("Enter your name: "); String name = scanner.next(); System.out.print("Enter your age: "); int age = scanner.nextInt(); System.out.print("Enter your favorite color: "); String color = scanner.next(); System.out.println("Hello, " + name + "! You are " + age + " years old and your favorite color is " + color + ".");
In this example, we use the next()
method to read strings and the nextInt()
method to read integers. We can repeat this pattern to read different types of input as needed.
Related Article: How to Sort a List of ArrayList in Java
Use Case: Using Delimiters in Scanner Class
The Scanner class also allows us to use delimiters to separate tokens in the input. By default, the delimiter is whitespace, but we can change it using the useDelimiter()
method. Let's see an example:
Scanner scanner = new Scanner("apple,banana,orange"); scanner.useDelimiter(","); while (scanner.hasNext()) { String fruit = scanner.next(); System.out.println(fruit); }
In this example, we create a Scanner object that reads from a string containing a list of fruits separated by commas. We set the delimiter to a comma using the useDelimiter()
method. Then, we use a loop to iterate through each token and print it. The output will be:
apple banana orange
Best Practice: Closing Scanner Objects
It is good practice to close Scanner objects when we are done using them. Closing a Scanner object releases any system resources associated with it. We can use the close()
method to close a Scanner object. Here's an example:
Scanner scanner = new Scanner(System.in); System.out.println("Enter your name: "); String name = scanner.next(); scanner.close();
By closing the Scanner object, we ensure that any underlying resources, such as file handles, are properly released.
Best Practice: Avoiding Resource Leaks
In addition to closing Scanner objects, we should also handle exceptions properly to avoid resource leaks. When using the Scanner class, it is important to handle potential exceptions that may occur during input reading. This ensures that resources are released even in the event of an error. Here's an example:
Scanner scanner = null; try { scanner = new Scanner(new File("input.txt")); // Read input here } catch (FileNotFoundException e) { System.out.println("File not found: " + e.getMessage()); } finally { if (scanner != null) { scanner.close(); } }
In this example, we create a Scanner object to read from a file. We wrap the creation of the Scanner object in a try-catch block to handle the possibility of a FileNotFoundException
. In the finally
block, we close the Scanner object to release any associated resources.
Real-World Example: Building a Basic Calculator
Let's explore a real-world example of using the Scanner class to build a basic calculator program. The program will prompt the user to enter two numbers and an operator (+, -, *, or /), and then perform the corresponding operation. Here's the code:
Scanner scanner = new Scanner(System.in); System.out.print("Enter the first number: "); double num1 = scanner.nextDouble(); System.out.print("Enter the second number: "); double num2 = scanner.nextDouble(); System.out.print("Enter the operator (+, -, *, /): "); char operator = scanner.next().charAt(0); double result; switch (operator) { case '+': result = num1 + num2; break; case '-': result = num1 - num2; break; case '*': result = num1 * num2; break; case '/': result = num1 / num2; break; default: System.out.println("Invalid operator"); return; } System.out.println("Result: " + result);
In this example, we prompt the user to enter two numbers and an operator. We use the Scanner class to read the numbers and the operator. Then, we perform the corresponding operation based on the operator and display the result.
Related Article: Java Classloader: How to Load Classes in Java
Real-World Example: Developing a Text-Based Game
Another real-world example of using the Scanner class is in the development of a text-based game. In this game, the user will be prompted with different choices and their input will determine the outcome of the game. Here's an example:
Scanner scanner = new Scanner(System.in); System.out.println("Welcome to the Text Adventure Game!"); System.out.println("Choose your path:"); System.out.println("1. Go left"); System.out.println("2. Go right"); System.out.println("3. Go straight"); int choice = scanner.nextInt(); switch (choice) { case 1: System.out.println("You encountered a monster!"); // Handle the encounter break; case 2: System.out.println("You found a treasure!"); // Handle the treasure break; case 3: System.out.println("You reached the end of the path!"); // Handle the end of the path break; default: System.out.println("Invalid choice"); break; }
In this example, we present the user with different choices using the Scanner class. The user's input determines the outcome of the game. We use a switch statement to handle each choice and display the corresponding message.
Performance Consideration: Memory Management
When working with large input sources, such as reading from files, it is important to consider memory management. The Scanner class buffers input internally, and if we are not careful, it can consume a significant amount of memory. To mitigate this, we can set a smaller buffer size when creating the Scanner object. Here's an example:
File file = new File("largefile.txt"); Scanner scanner = new Scanner(file); scanner.useDelimiter("\\Z"); // Read the entire file as a single token scanner = scanner.useDelimiter("\\Z"); // Read the entire file as a single token scanner = new Scanner(file); scanner.useDelimiter("\\A"); // Read the entire file as a single token scanner = new Scanner(file); scanner.useDelimiter("\\Z"); // Read the entire file as a single token
In this example, we create a Scanner object to read from a large file. We set the delimiter to "\\Z" to read the entire file as a single token. By doing this, we reduce the memory usage of the Scanner object.
Performance Consideration: Efficiency of Scanning Methods
The efficiency of scanning methods in the Scanner class can vary depending on the input source. Some methods, such as nextLine()
, can be slower when reading from the console compared to reading from a file. If performance is a concern, it is worth considering alternative methods or strategies for reading input. For example, reading input in larger chunks rather than line by line can improve performance. Additionally, using specialized libraries or techniques for parsing complex input may be more efficient in certain scenarios.
Advanced Technique: Regular Expressions with Scanner Class
The Scanner class supports regular expressions for more advanced input parsing. Regular expressions allow us to define patterns that match specific input formats. We can use the findInLine()
method with regular expressions to find and extract specific patterns from the input. Here's an example:
Scanner scanner = new Scanner("apple1 orange2 banana3"); Pattern pattern = Pattern.compile("\\w+(\\d)"); while (scanner.findInLine(pattern) != null) { MatchResult result = scanner.match(); System.out.println(result.group(1)); }
In this example, we create a Scanner object that reads from a string containing a series of words followed by numbers. We define a regular expression pattern that matches a word followed by a digit. We use the findInLine()
method to search for this pattern in the input. When a match is found, we extract the digit using the group()
method of the MatchResult object.
Related Article: Overriding vs Overloading in Java: Tutorial
Advanced Technique: Scanning Large Files
When working with large files, it is important to optimize the scanning process to improve performance. One way to achieve this is by using the hasNextLine()
method to check if there are more lines to read before calling the nextLine()
method. This can help reduce unnecessary I/O operations. Here's an example:
Scanner scanner = new Scanner(file); while (scanner.hasNextLine()) { String line = scanner.nextLine(); // Process the line }
In this example, we create a Scanner object to read from a file. We use the hasNextLine()
method to check if there are more lines to read, and then call the nextLine()
method to read the line. This approach helps optimize the scanning process and improves performance when dealing with large files.
Code Snippet: Reading a Line of Input
To read a line of input from the console, we can use the nextLine()
method of the Scanner class. Here's an example:
Scanner scanner = new Scanner(System.in); System.out.print("Enter a sentence: "); String sentence = scanner.nextLine(); System.out.println("You entered: " + sentence);
In this example, we use the nextLine()
method to read a line of input from the console. The input is stored in a string variable and then displayed.
Code Snippet: Reading an Integer Input
To read an integer input from the console, we can use the nextInt()
method of the Scanner class. Here's an example:
Scanner scanner = new Scanner(System.in); System.out.print("Enter an integer: "); int number = scanner.nextInt(); System.out.println("You entered: " + number);
In this example, we use the nextInt()
method to read an integer input from the console. The input is stored in an integer variable and then displayed.
Code Snippet: Reading a Double Input
To read a double input from the console, we can use the nextDouble()
method of the Scanner class. Here's an example:
Scanner scanner = new Scanner(System.in); System.out.print("Enter a decimal number: "); double decimal = scanner.nextDouble(); System.out.println("You entered: " + decimal);
In this example, we use the nextDouble()
method to read a double input from the console. The input is stored in a double variable and then displayed.
Related Article: How to Pass Arguments by Value in Java
Code Snippet: Using Delimiters
To use delimiters with the Scanner class, we can use the useDelimiter()
method. Here's an example that reads a series of words separated by commas:
Scanner scanner = new Scanner("apple,banana,orange"); scanner.useDelimiter(","); while (scanner.hasNext()) { String fruit = scanner.next(); System.out.println(fruit); }
In this example, we create a Scanner object that reads from a string containing a list of fruits separated by commas. We set the delimiter to a comma using the useDelimiter()
method. Then, we use a loop to iterate through each token and print it.
Code Snippet: Using Regular Expressions
To use regular expressions with the Scanner class, we can use the findInLine()
method. Here's an example that finds and extracts all occurrences of a pattern in a string:
Scanner scanner = new Scanner("apple1 orange2 banana3"); Pattern pattern = Pattern.compile("\\w+(\\d)"); while (scanner.findInLine(pattern) != null) { MatchResult result = scanner.match(); System.out.println(result.group(1)); }
In this example, we create a Scanner object that reads from a string containing a series of words followed by numbers. We define a regular expression pattern that matches a word followed by a digit. We use the findInLine()
method to search for this pattern in the input. When a match is found, we extract the digit using the group()
method of the MatchResult object.
Error Handling: InputMismatchException
When working with the Scanner class, it is important to handle potential exceptions that may occur during input reading. One common exception is the InputMismatchException, which is thrown when the input does not match the expected format. We can use a try-catch block to handle this exception. Here's an example:
Scanner scanner = new Scanner(System.in); try { System.out.print("Enter an integer: "); int number = scanner.nextInt(); System.out.println("You entered: " + number); } catch (InputMismatchException e) { System.out.println("Invalid input: " + e.getMessage()); }
In this example, we use the nextInt()
method to read an integer input from the console. If the input is not an integer, an InputMismatchException is thrown. We catch the exception in a try-catch block and display an error message.
Error Handling: NoSuchElementException
Another common exception when working with the Scanner class is the NoSuchElementException, which is thrown when there are no more tokens to read. We can use the hasNext()
method to check if there are more tokens before calling methods like next()
. Here's an example:
Scanner scanner = new Scanner(System.in); System.out.print("Enter a word: "); if (scanner.hasNext()) { String word = scanner.next(); System.out.println("You entered: " + word); } else { System.out.println("No input found"); }
In this example, we use the hasNext()
method to check if there are more tokens to read. If there are, we call the next()
method to read the next token. If there are no more tokens, a NoSuchElementException is thrown. We handle this exception by displaying an appropriate message.
Related Article: Critical Algorithms and Data Structures in Java
Error Handling: IllegalStateException
The IllegalStateException can occur when calling certain methods of the Scanner class in an invalid state. For example, if we try to read input after closing the Scanner object, an IllegalStateException is thrown. It is important to ensure that we are using the Scanner object in a valid state to avoid this exception. Here's an example:
Scanner scanner = new Scanner(System.in); scanner.close(); System.out.print("Enter a number: "); // This will throw an IllegalStateException
In this example, we close the Scanner object using the close()
method. After closing the Scanner object, any attempt to read input will result in an IllegalStateException. It is important to be mindful of the state of the Scanner object when working with it.