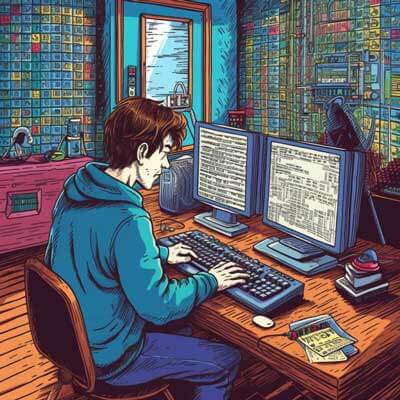
- Method 1: Using the {{EJS3}} class
- Method 2: Retrieving the version from the {{EJS7}} interface
- Recommended method to check the version of MySQL-Connector-Java in Java
- Available libraries or tools to check the version of MySQL-Connector-Java in Java
- Programmatically checking the version of MySQL-Connector-Java in Java
- Best practice to check the version of MySQL-Connector-Java in Java
- Ensuring you’re using the latest version of MySQL-Connector-Java in your Java application
- Additional Resources
There are several ways to check the version of MySQL Connector/J in a Java application. Here are two common methods:
Method 1: Using the Driver
class
The Driver
class in MySQL Connector/J provides a static method getMajorVersion()
and getMinorVersion()
to retrieve the major and minor version numbers, respectively.
import com.mysql.cj.jdbc.Driver; public class ConnectorVersionChecker { public static void main(String[] args) { int majorVersion = Driver.getMajorVersion(); int minorVersion = Driver.getMinorVersion(); System.out.println("MySQL Connector/J version: " + majorVersion + "." + minorVersion); } }
Related Article: Spring Boot Integration with Payment, Communication Tools
Method 2: Retrieving the version from the java.sql.Driver
interface
MySQL Connector/J implements the java.sql.Driver
interface, which provides a method getMajorVersion()
and getMinorVersion()
to retrieve the major and minor version numbers, respectively.
import java.sql.Driver; import java.sql.DriverManager; import java.sql.SQLException; public class ConnectorVersionChecker { public static void main(String[] args) { try { Driver driver = DriverManager.getDriver("jdbc:mysql://localhost:3306/mydatabase"); int majorVersion = driver.getMajorVersion(); int minorVersion = driver.getMinorVersion(); System.out.println("MySQL Connector/J version: " + majorVersion + "." + minorVersion); } catch (SQLException e) { e.printStackTrace(); } } }
Both methods will output the version of MySQL Connector/J being used in the Java application.
Recommended method to check the version of MySQL-Connector-Java in Java
The recommended method to check the version of MySQL Connector/J in Java is by using the Driver
class or the java.sql.Driver
interface, as shown in the previous examples. These methods are provided by MySQL Connector/J itself and are reliable ways to retrieve the version information.
It is important to note that the specific class or interface used may depend on the version of MySQL Connector/J being used or the specific requirements of the application. Developers should consult the documentation or the official MySQL Connector/J website for the appropriate method to use for their specific version.
Available libraries or tools to check the version of MySQL-Connector-Java in Java
Apart from using the built-in methods provided by MySQL Connector/J, there are no specific libraries or tools dedicated to checking the version of MySQL Connector/J in Java.
However, developers can use general-purpose build management tools like Apache Maven or Gradle to manage the dependencies of their Java projects, including MySQL Connector/J. These tools provide features to list and manage the versions of the libraries used in the project. By inspecting the project’s configuration file (e.g., pom.xml
for Maven or build.gradle
for Gradle), developers can easily identify the version of MySQL Connector/J being used.
Related Article: Java Spring Security Customizations & RESTful API Protection
Programmatically checking the version of MySQL-Connector-Java in Java
To programmatically check the version of MySQL Connector/J in Java, you can use the following code snippet:
import com.mysql.cj.jdbc.Driver; public class ConnectorVersionChecker { public static void main(String[] args) { int majorVersion = Driver.getMajorVersion(); int minorVersion = Driver.getMinorVersion(); System.out.println("MySQL Connector/J version: " + majorVersion + "." + minorVersion); } }
This code snippet uses the getMajorVersion()
and getMinorVersion()
methods from the Driver
class in MySQL Connector/J to retrieve the major and minor version numbers, respectively.
Best practice to check the version of MySQL-Connector-Java in Java
The best practice to check the version of MySQL Connector/J in Java is to use the built-in methods provided by MySQL Connector/J itself, such as Driver.getMajorVersion()
and Driver.getMinorVersion()
, or the equivalent methods in the java.sql.Driver
interface.
It is also recommended to document the version of MySQL Connector/J being used in the project’s configuration files or documentation. This helps in maintaining a clear record of the dependencies and facilitates troubleshooting or future upgrades.
Ensuring you’re using the latest version of MySQL-Connector-Java in your Java application
To ensure that you are using the latest version of MySQL Connector/J in your Java application, you can follow these steps:
1. Check the official MySQL Connector/J website or the MySQL community for the latest release of MySQL Connector/J.
2. Update the version number in your project’s configuration file (e.g., pom.xml
for Maven or build.gradle
for Gradle) to the latest version.
3. Run a build command (e.g., mvn clean install
for Maven or gradle build
for Gradle) to fetch the latest version of MySQL Connector/J and update the dependencies in your project.
4. After updating the version, it is important to thoroughly test the application to ensure compatibility and functionality with the latest version of MySQL Connector/J. This includes verifying that all database operations and queries work as expected.
5. Keep an eye on the MySQL Connector/J release notes and updates for any bug fixes, security patches, or new features that may affect your application. Regularly update your project to the latest version to take advantage of these improvements.
Related Article: Tutorial on Integrating Redis with Spring Boot
Additional Resources
– Connect to a MySQL database using mysql-connector-java in Java