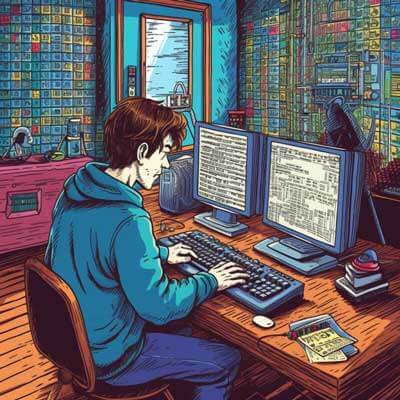
Table of Contents
In today's digital world, integrating payment gateways into web applications has become a necessity for many businesses. Spring Boot, with its simplicity and ease of use, provides a great framework for integrating payment gateways seamlessly. In this article, we will explore how to integrate two popular payment gateways, Stripe and PayPal, into a Spring Boot application.
Integrating Stripe with Spring Boot
Stripe is a widely used payment gateway that allows businesses to accept online payments. Integrating Stripe with Spring Boot is straightforward and can be done in a few simple steps.
To integrate Stripe with Spring Boot, you will need to add the Stripe Java library as a dependency in your project. Add the following dependency to your pom.xml
file:
com.stripe stripe-java 20.59.0
Once you have added the dependency, you can start using the Stripe API in your Spring Boot application. Here is an example of how to create a payment intent using Stripe:
import com.stripe.Stripe; import com.stripe.exception.StripeException; import com.stripe.model.PaymentIntent; import com.stripe.param.PaymentIntentCreateParams; @RestController @RequestMapping("/api/payments") public class PaymentController { @PostMapping("/create-payment-intent") public ResponseEntity createPaymentIntent() { Stripe.apiKey = "YOUR_STRIPE_SECRET_KEY"; try { PaymentIntentCreateParams params = new PaymentIntentCreateParams.Builder() .setCurrency("usd") .setAmount(1000) .build(); PaymentIntent paymentIntent = PaymentIntent.create(params); return ResponseEntity.ok(paymentIntent.getClientSecret()); } catch (StripeException e) { return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build(); } } }
In this example, we create a PaymentIntent
object using the Stripe Java library and return the client secret to the client-side. The client can then use this client secret to complete the payment process.
Related Article: How to Implement a Delay in Java Using java wait seconds
Integrating PayPal with Spring Boot
PayPal is another popular payment gateway that provides a secure and reliable way to accept online payments. Integrating PayPal with Spring Boot is also a straightforward process.
To integrate PayPal with Spring Boot, you will need to add the PayPal Java SDK as a dependency in your project. Add the following dependency to your pom.xml
file:
com.paypal.sdk rest-api-sdk 1.14.0
Once you have added the dependency, you can start using the PayPal API in your Spring Boot application. Here is an example of how to create a payment using PayPal:
import com.paypal.api.payments.*; import com.paypal.base.rest.APIContext; import com.paypal.base.rest.PayPalRESTException; @RestController @RequestMapping("/api/payments") public class PaymentController { @PostMapping("/create-payment") public ResponseEntity createPayment() { Payment payment = new Payment(); payment.setIntent("sale"); Payer payer = new Payer(); payer.setPaymentMethod("paypal"); payment.setPayer(payer); RedirectUrls redirectUrls = new RedirectUrls(); redirectUrls.setReturnUrl("http://localhost:8080/api/payments/execute-payment"); redirectUrls.setCancelUrl("http://localhost:8080/api/payments/cancel-payment"); payment.setRedirectUrls(redirectUrls); Transaction transaction = new Transaction(); Amount amount = new Amount(); amount.setCurrency("USD"); amount.setTotal("10.00"); transaction.setAmount(amount); payment.setTransactions(Collections.singletonList(transaction)); try { APIContext apiContext = new APIContext("YOUR_PAYPAL_CLIENT_ID", "YOUR_PAYPAL_CLIENT_SECRET", "sandbox"); Payment createdPayment = payment.create(apiContext); return ResponseEntity.ok(createdPayment.getLinks().get(1).getHref()); } catch (PayPalRESTException e) { return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build(); } } @GetMapping("/execute-payment") public ResponseEntity executePayment(@RequestParam("paymentId") String paymentId, @RequestParam("PayerID") String payerId) { try { APIContext apiContext = new APIContext("YOUR_PAYPAL_CLIENT_ID", "YOUR_PAYPAL_CLIENT_SECRET", "sandbox"); Payment payment = Payment.get(apiContext, paymentId); PaymentExecution paymentExecution = new PaymentExecution(); paymentExecution.setPayerId(payerId); Payment executedPayment = payment.execute(apiContext, paymentExecution); return ResponseEntity.ok(executedPayment.getState()); } catch (PayPalRESTException e) { return ResponseEntity.status(HttpStatus.INTERNAL_SERVER_ERROR).build(); } } @GetMapping("/cancel-payment") public ResponseEntity cancelPayment() { return ResponseEntity.ok("Payment canceled"); } }
In this example, we create a Payment
object using the PayPal Java SDK and redirect the client to the PayPal payment page. Once the payment is completed, PayPal will redirect the client back to the return URL specified in the RedirectUrls
object. The client can then use the paymentId
and PayerID
to execute the payment.
Integrating payment gateways like Stripe and PayPal into your Spring Boot application can greatly enhance the functionality and user experience of your application. With the examples provided above, you can easily integrate these payment gateways into your Spring Boot projects and start accepting online payments.
Setting up Voice Functionalities with Twilio and Spring Boot
Twilio is a cloud communications platform that provides APIs for building voice, video, and messaging applications. Integrating Twilio with Spring Boot allows developers to easily add voice functionalities to their applications. In this section, we will explore how to set up voice functionalities with Twilio and Spring Boot.
To get started with Twilio, you will need to create an account and obtain your Twilio credentials, including your Account SID and Auth Token. Once you have your credentials, you can start integrating Twilio with your Spring Boot application.
Enabling Voice Calling with Twilio
To enable voice calling with Twilio, you will need to add the Twilio Java SDK as a dependency in your project. Add the following dependency to your pom.xml
file:
com.twilio.sdk twilio-java-sdk 8.1.0
Once you have added the dependency, you can start using the Twilio API in your Spring Boot application. Here is an example of how to make a voice call using Twilio:
import com.twilio.Twilio; import com.twilio.rest.api.v2010.account.Call; import com.twilio.type.PhoneNumber; @RestController @RequestMapping("/api/voice") public class VoiceController { @PostMapping("/make-call") public ResponseEntity makeCall() { Twilio.init("YOUR_TWILIO_ACCOUNT_SID", "YOUR_TWILIO_AUTH_TOKEN"); Call.creator( new PhoneNumber("RECIPIENT_PHONE_NUMBER"), new PhoneNumber("YOUR_TWILIO_PHONE_NUMBER"), new URI("http://example.com/twilio/voice") ).create(); return ResponseEntity.ok("Call initiated"); } @PostMapping("/twilio/voice") public TwiML handleVoiceRequest() { VoiceResponse response = new VoiceResponse.Builder() .say(new Say.Builder("Hello, this is a Twilio voice call").build()) .build(); return new VoiceResponseTwimlConverter().toTwiML(response); } }
In this example, we use the Twilio Java SDK to initiate a voice call. We provide the recipient's phone number, our Twilio phone number, and a URL that Twilio will use to handle the call. The URL should point to a controller method that generates TwiML, which is Twilio's XML-based markup language for defining voice call flows. In the handleVoiceRequest
method, we create a VoiceResponse
object and use the TwiML builder to define the voice call flow.
Related Article: Java Printf Method Tutorial
Implementing SMS Functionalities in Spring Boot using Twilio
In addition to voice functionalities, Twilio also provides APIs for sending and receiving SMS messages. Integrating SMS functionalities with Spring Boot can be done using the Twilio Java SDK. Here is an example of how to implement SMS functionalities in Spring Boot using Twilio:
import com.twilio.Twilio; import com.twilio.rest.api.v2010.account.Message; import com.twilio.type.PhoneNumber; @RestController @RequestMapping("/api/sms") public class SMSController { @PostMapping("/send-message") public ResponseEntity sendMessage() { Twilio.init("YOUR_TWILIO_ACCOUNT_SID", "YOUR_TWILIO_AUTH_TOKEN"); Message.creator( new PhoneNumber("RECIPIENT_PHONE_NUMBER"), new PhoneNumber("YOUR_TWILIO_PHONE_NUMBER"), "Hello, this is a Twilio SMS message" ).create(); return ResponseEntity.ok("Message sent"); } }
In this example, we use the Twilio Java SDK to send an SMS message. We provide the recipient's phone number, our Twilio phone number, and the message content. Twilio takes care of delivering the message to the recipient.
Adding voice and SMS functionalities to your Spring Boot application using Twilio can greatly enhance the communication capabilities of your application. With the examples provided above, you can easily integrate Twilio into your Spring Boot projects and start building voice and messaging applications.
Best Practices for Building Chatbots in Spring Boot
Chatbots have become increasingly popular in recent years, offering businesses an effective way to automate customer interactions. Spring Boot provides a robust framework for building chatbots that can be integrated into various messaging platforms. In this section, we will explore some best practices for building chatbots in Spring Boot.
Designing Conversational Flows
One of the key aspects of building a successful chatbot is designing effective conversational flows. Conversational flows define the structure and logic of how the chatbot interacts with users. When designing conversational flows, consider the following best practices:
- Keep it simple: Start with simple conversational flows and gradually add complexity as needed. Avoid overwhelming users with too many options or steps.
- Use natural language: Design the chatbot to understand and respond to natural language inputs. Use NLP (Natural Language Processing) techniques to extract intent and entities from user messages.
- Handle errors gracefully: Plan for error scenarios and design the chatbot to handle them gracefully. Provide clear error messages and offer suggestions or alternatives to help users recover from errors.
- Provide fallback options: In case the chatbot doesn't understand a user's input or encounters an error, provide fallback options such as a human agent or a menu of frequently asked questions.
- Test and iterate: Test the conversational flows with real users and gather feedback to identify areas for improvement. Iteratively refine the chatbot based on user feedback.
Using Spring Integration for Chatbot Integration
Spring Integration is a useful framework that simplifies the integration of systems and applications. It provides a wide range of components and patterns for building chatbot integrations. When building chatbots in Spring Boot, consider the following best practices for using Spring Integration:
- Use message-driven architecture: Design the chatbot as a message-driven system, where messages flow through channels and are processed by components. This allows for loose coupling and scalability.
- Leverage Spring Integration components: Take advantage of the various Spring Integration components such as routers, transformers, and filters to handle different aspects of chatbot interactions.
- Implement message handlers: Use message handlers to process incoming messages and generate appropriate responses. Message handlers can be implemented as Spring beans and configured in the integration flow.
- Use external connectors: Integrate the chatbot with external systems and platforms using Spring Integration connectors. For example, use the Slack connector to build a Slack chatbot or the Facebook Messenger connector to build a Facebook Messenger chatbot.
- Implement error handling: Handle errors and exceptions in the integration flow. Use error channels and error handlers to gracefully handle errors and provide meaningful error messages to users.
Related Article: How to Convert List to Array in Java
Developing Voice Applications using Spring Boot
Voice applications have gained popularity with the rise of voice assistants like Amazon Alexa and Google Assistant. Spring Boot provides a great framework for developing voice applications that can be deployed on various voice platforms. In this section, we will explore how to develop voice applications using Spring Boot.
Using Spring MVC for Voice Application Development
Spring MVC (Model-View-Controller) is a widely used framework for building web applications in Spring Boot. It provides a structured approach to handling HTTP requests and generating responses. When developing voice applications using Spring Boot, consider the following best practices for using Spring MVC:
- Define voice controllers: Create controllers specifically for handling voice requests. These controllers should implement methods that handle voice intents or commands.
- Use voice-specific annotations: Use voice-specific annotations provided by the voice platform SDK to map voice intents or commands to controller methods. For example, use the @AlexaIntent
annotation provided by the Alexa Skills Kit SDK to map Alexa intents to controller methods.
- Handle voice request and response formats: Voice platforms have their own request and response formats. Handle these formats appropriately in the controller methods. Use the voice platform SDKs to parse incoming requests and generate appropriate responses.
- Implement session management: Voice applications often require session management to maintain context between user interactions. Implement session management using the voice platform SDKs and store session data in a persistent storage if needed.
- Leverage Spring MVC features: Take advantage of the various features provided by Spring MVC such as request mapping, request validation, and exception handling to build robust voice applications.
Using External Voice Platforms
In addition to developing voice applications using Spring MVC, you can also leverage external voice platforms to build and deploy voice applications. Voice platforms like Amazon Alexa and Google Assistant provide SDKs and tools for developing voice applications. When using external voice platforms, consider the following best practices:
- Follow platform-specific guidelines: Each voice platform has its own guidelines and best practices. Familiarize yourself with the platform-specific guidelines and follow them when developing voice applications.
- Use platform-specific SDKs: Leverage the SDKs provided by the voice platforms to handle voice requests and generate responses. These SDKs often provide utilities for parsing voice requests, handling intents, and generating speech responses.
- Test on the platform: Test your voice applications thoroughly on the voice platform itself. Use the testing tools provided by the platform to ensure that your application works correctly in different scenarios.
- Publish your application: If you are developing voice applications for a specific voice platform, consider publishing your application in the platform's marketplace. This allows users to discover and use your voice application.
Developing voice applications using Spring Boot provides a flexible and scalable approach. Whether you choose to use Spring MVC or external voice platforms, following best practices will help you build robust and user-friendly voice applications.
Libraries and Frameworks for Integrating Payment Gateways with Spring Boot
Integrating payment gateways with Spring Boot can be made easier by leveraging existing libraries and frameworks. In this section, we will explore some popular libraries and frameworks that can be used for integrating payment gateways with Spring Boot.
Related Article: How to Manage Collections Without a SortedList in Java
Spring Cloud Stripe
Spring Cloud Stripe is a library that provides seamless integration between Spring Cloud and the Stripe payment gateway. It provides a set of Spring Boot starters and auto-configuration classes that simplify the integration process. With Spring Cloud Stripe, you can easily configure and interact with the Stripe API using Spring Boot annotations and properties.
To integrate Spring Cloud Stripe with your Spring Boot application, you will need to add the following dependency to your pom.xml
file:
org.springframework.cloud spring-cloud-starter-stripe 2.2.2.RELEASE
Once you have added the dependency, you can start using Spring Cloud Stripe in your application. For example, you can use the @EnableStripe
annotation to enable the Stripe auto-configuration and access the Stripe API using the StripeTemplate
bean. Here is an example:
import org.springframework.cloud.stripe.EnableStripe; import org.springframework.cloud.stripe.StripeTemplate; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @RestController @EnableStripe public class PaymentController { private final StripeTemplate stripeTemplate; public PaymentController(StripeTemplate stripeTemplate) { this.stripeTemplate = stripeTemplate; } @GetMapping("/api/payments") public String makePayment() { // Use the StripeTemplate to interact with the Stripe API // ... return "Payment successful"; } }
With Spring Cloud Stripe, you can easily integrate the Stripe payment gateway into your Spring Boot application and start processing payments.
Spring PayPal
Spring PayPal is a library that provides integration with the PayPal payment gateway. It offers a set of Spring Boot starters and auto-configuration classes that simplify the integration process. With Spring PayPal, you can easily configure and interact with the PayPal API using Spring Boot annotations and properties.
To integrate Spring PayPal with your Spring Boot application, you will need to add the following dependency to your pom.xml
file:
org.springframework.boot spring-boot-starter-paypal 2.5.3
Once you have added the dependency, you can start using Spring PayPal in your application. For example, you can use the @EnablePayPal
annotation to enable the PayPal auto-configuration and access the PayPal API using the PayPalRestTemplate
bean. Here is an example:
import org.springframework.boot.autoconfigure.EnableAutoConfiguration; import org.springframework.boot.autoconfigure.condition.ConditionalOnClass; import org.springframework.boot.autoconfigure.condition.ConditionalOnProperty; import org.springframework.boot.context.properties.EnableConfigurationProperties; import org.springframework.context.annotation.Configuration; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RestController; @Configuration @EnableConfigurationProperties(PayPalProperties.class) @ConditionalOnClass(PayPalRestTemplate.class) @ConditionalOnProperty(prefix = "spring.paypal", name = "clientId") public class PayPalAutoConfiguration { // Auto-configuration logic // ... } @RestController public class PaymentController { private final PayPalRestTemplate payPalRestTemplate; public PaymentController(PayPalRestTemplate payPalRestTemplate) { this.payPalRestTemplate = payPalRestTemplate; } @GetMapping("/api/payments") public String makePayment() { // Use the PayPalRestTemplate to interact with the PayPal API // ... return "Payment successful"; } }
With Spring PayPal, you can easily integrate the PayPal payment gateway into your Spring Boot application and start processing payments.
Advantages of Using Stripe as a Payment Gateway in Spring Boot
Stripe is a popular payment gateway that provides a robust set of features for accepting online payments. When integrating payment gateways into Spring Boot applications, using Stripe as the payment gateway offers several advantages.
Simplicity and Ease of Use
One of the key advantages of using Stripe in Spring Boot is its simplicity and ease of use. Stripe provides a well-documented API and a set of client libraries, including the Stripe Java library, that make it easy to integrate Stripe into Spring Boot applications. The API is designed to be developer-friendly and provides clear and concise methods for handling payment processing.
Related Article: PHP vs Java: A Practical Comparison
Wide Range of Payment Options
Stripe supports a wide range of payment options, including credit cards, digital wallets, and alternative payment methods. This allows businesses to provide their customers with flexible payment options and cater to a broader audience. With Stripe, you can easily handle one-time payments, recurring subscriptions, and complex payment flows.
Advanced Fraud Detection and Security
Stripe offers advanced fraud detection and security features to protect businesses and their customers from fraudulent activities. It provides built-in fraud detection tools, such as Radar, that use machine learning algorithms to identify and prevent fraudulent transactions. Stripe also handles PCI compliance, reducing the burden on businesses to maintain the security of customer payment data.
Developer-Friendly Tools and Documentation
Stripe provides a range of developer-friendly tools and documentation that make it easy to integrate and test payment functionalities. It offers a comprehensive dashboard for managing payments, subscriptions, and customer data. The documentation provides detailed guides, tutorials, and code examples for integrating Stripe into various platforms and frameworks, including Spring Boot.
Transparent Pricing and Competitive Fees
Stripe offers transparent pricing and competitive fees for businesses of all sizes. Its pricing model is straightforward, with no setup fees, monthly fees, or hidden charges. Stripe charges a small percentage fee per transaction, making it cost-effective for businesses, especially startups and small businesses.
Using Stripe as a payment gateway in Spring Boot applications provides simplicity, flexibility, and advanced features for handling online payments. With its developer-friendly approach and comprehensive documentation, integrating Stripe into Spring Boot applications is a straightforward process.
Related Article: How to Use and Manipulate Arrays in Java
Using PayPal as a Payment Gateway in Spring Boot
PayPal is a widely recognized and trusted payment gateway that allows businesses to accept online payments securely. When integrating payment gateways into Spring Boot applications, using PayPal as the payment gateway offers several advantages.
Global Reach and Wide Customer Base
One of the key advantages of using PayPal in Spring Boot is its global reach and wide customer base. PayPal is accepted in over 200 countries and supports multiple currencies, making it a suitable payment solution for businesses with international customers. By integrating PayPal into your Spring Boot application, you can tap into PayPal's extensive customer base and reach a broader audience.
Seamless Integration and Developer Tools
PayPal provides a range of developer tools and APIs that make it easy to integrate PayPal into Spring Boot applications. The PayPal Java SDK, for example, provides a set of Java classes and methods for interacting with the PayPal API. PayPal's developer documentation offers detailed guides, tutorials, and code examples to help developers integrate PayPal into their applications.
Multiple Payment Options
PayPal offers multiple payment options, including credit cards, debit cards, and PayPal accounts. This allows businesses to provide their customers with a variety of payment methods and cater to different preferences. With PayPal, you can easily handle one-time payments, recurring subscriptions, and express checkout flows.
Related Article: How to Fix the java.lang.reflect.InvocationTargetException
Trust and Security
PayPal is widely recognized for its trust and security. It employs advanced fraud detection and prevention mechanisms to protect businesses and their customers from fraudulent activities. PayPal handles the security of customer payment data and complies with industry standards, such as PCI DSS (Payment Card Industry Data Security Standard).
Buyer and Seller Protection
PayPal offers buyer and seller protection programs that provide added security for transactions. Buyer protection covers eligible purchases made through PayPal, offering refunds or reimbursement for certain issues. Seller protection helps protect sellers from unauthorized transactions and chargebacks.
Using PayPal as a payment gateway in Spring Boot applications provides global reach, seamless integration, multiple payment options, trust, and security. With its wide customer base and extensive developer tools, integrating PayPal into Spring Boot applications is a straightforward process.
Implementing Voice Functionalities in Spring Boot
Implementing voice functionalities in Spring Boot allows developers to build voice applications that can be integrated with various voice platforms. In this section, we will explore how to implement voice functionalities in Spring Boot using the Twilio API.
Setting up Twilio Voice
To implement voice functionalities in Spring Boot, you will need to set up Twilio Voice. Twilio Voice allows you to make and receive phone calls, as well as control call flows using TwiML, which is Twilio's XML-based markup language for defining voice call flows. Here is an example of how to set up Twilio Voice in Spring Boot:
1. Create a Twilio account: Sign up for a Twilio account at https://www.twilio.com/ and obtain your Twilio credentials, including your Account SID and Auth Token.
2. Add the Twilio Java SDK as a dependency in your project. Add the following dependency to your pom.xml
file:
com.twilio.sdk twilio-java-sdk 8.1.0
3. Configure your Twilio credentials in your Spring Boot application. You can use the application.properties
file or the application.yml
file to configure your Twilio credentials. Here is an example of how to configure your Twilio credentials in application.properties
:
twilio.accountSid=YOUR_TWILIO_ACCOUNT_SID twilio.authToken=YOUR_TWILIO_AUTH_TOKEN twilio.phoneNumber=YOUR_TWILIO_PHONE_NUMBER
4. Implement a controller to handle incoming voice requests. Here is an example of a controller that handles incoming voice requests and generates TwiML to control call flows:
import com.twilio.twiml.VoiceResponse; import com.twilio.twiml.voice.Say; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RestController; @RestController public class VoiceController { @PostMapping("/api/voice") public VoiceResponse handleIncomingCall() { Say say = new Say.Builder("Hello, this is a voice response from your Spring Boot application").build(); return new VoiceResponse.Builder().say(say).build(); } }
In this example, we use the Twilio Java SDK to handle incoming voice requests and generate a simple voice response using TwiML.
5. Configure your voice platform to forward incoming calls to your Spring Boot application. This step will vary depending on the voice platform you are using. Consult the documentation of your voice platform to learn how to configure call forwarding to your Spring Boot application.
Related Article: Critical Algorithms and Data Structures in Java
Handling Incoming SMS Messages in Spring Boot with Twilio
Handling incoming SMS messages in Spring Boot allows developers to build SMS-based applications and respond to user requests via SMS. In this section, we will explore how to handle incoming SMS messages in Spring Boot using the Twilio API.
Setting up Twilio SMS
To handle incoming SMS messages in Spring Boot, you will need to set up Twilio SMS. Twilio SMS allows you to send and receive SMS messages programmatically. Here is an example of how to set up Twilio SMS in Spring Boot:
1. Create a Twilio account: Sign up for a Twilio account at https://www.twilio.com/ and obtain your Twilio credentials, including your Account SID and Auth Token.
2. Add the Twilio Java SDK as a dependency in your project. Add the following dependency to your pom.xml
file:
com.twilio.sdk twilio-java-sdk 8.1.0
3. Configure your Twilio credentials in your Spring Boot application. You can use the application.properties
file or the application.yml
file to configure your Twilio credentials. Here is an example of how to configure your Twilio credentials in application.properties
:
twilio.accountSid=YOUR_TWILIO_ACCOUNT_SID twilio.authToken=YOUR_TWILIO_AUTH_TOKEN twilio.phoneNumber=YOUR_TWILIO_PHONE_NUMBER
4. Implement a controller to handle incoming SMS messages. Here is an example of a controller that handles incoming SMS messages and responds with a simple SMS message:
import com.twilio.twiml.MessagingResponse; import com.twilio.twiml.messaging.Body; import com.twilio.twiml.messaging.Message; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RestController; @RestController @RequestMapping("/api/sms") public class SMSController { @PostMapping("/incoming") public MessagingResponse handleIncomingSMS() { Body body = new Body.Builder("This is a response from your Spring Boot application").build(); Message message = new Message.Builder().body(body).build(); return new MessagingResponse.Builder().message(message).build(); } }
In this example, we use the Twilio Java SDK to handle incoming SMS messages and generate a simple SMS response using TwiML.
5. Configure your SMS platform to forward incoming SMS messages to your Spring Boot application. This step will vary depending on the SMS platform you are using. Consult the documentation of your SMS platform to learn how to configure SMS forwarding to your Spring Boot application.