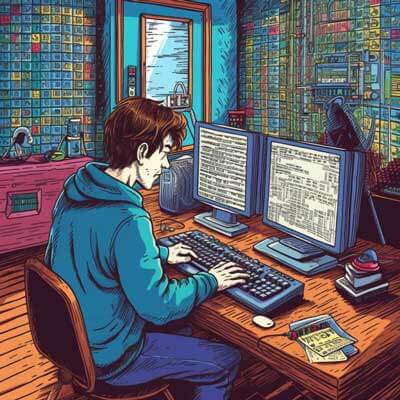
- What is Serverless Architecture?
- The Benefits of Serverless Architecture
- 1. Cost Efficiency
- 2. Scalability and Elasticity
- 3. Reduced Operational Complexity
- 4. Faster Time-to-Market
- Getting Started with Serverless Architecture
- Understanding Serverless Architecture
- Choosing a Serverless Platform
- Writing Serverless Functions
- Deploying Serverless Functions
- Testing and Monitoring Serverless Functions
- Use Cases for Serverless Architecture
- Real World Examples of Serverless Architecture
- Advanced Techniques in Serverless Architecture
- 1. Event-driven Architecture
- 2. Asynchronous Processing
- 3. Cold Start Mitigation
- 4. Distributed Tracing
- 5. Security Considerations
- Scaling and Performance Optimization in Serverless Architecture
- Automatic Scaling
- Concurrency and Throttling
- Caching
- Optimizing Cold Start Time
- Security Considerations in Serverless Architecture
- 1. Authentication and Authorization
- 2. Data Protection
- 3. Function Isolation and Privilege Separation
- 4. Logging and Monitoring
- 5. Third-Party Dependencies
- Monitoring and Debugging in Serverless Architecture
- Logging
- Distributed Tracing
- Metrics and Alerts
- Debugging
- Serverless Frameworks and Tools
- AWS Serverless Application Model (SAM)
- Serverless Framework
- Azure Functions
- Google Cloud Functions
- Integration with Other Services and APIs
- Calling External APIs
- Triggering Events in Other Services
- Working with Data Storage Services
- Data Storage and Management in Serverless Architecture
- 1. Serverless Databases
- 2. Object Storage
- 3. Managed File Systems
- Event-driven Architecture with Serverless
- Understanding Event-driven Architecture
- Implementing Event-driven Architecture with Serverless
- Benefits of Event-driven Architecture
- Building APIs with Serverless Functions
- What are Serverless Functions?
- Benefits of Using Serverless Functions for APIs
- Creating a Serverless API
- Orchestration and Workflow in Serverless Architecture
- Testing and Deployment Strategies for Serverless Applications
- Unit Testing
- Integration Testing
- Deployment Strategies
- Continuous Integration and Deployment
- Best Practices for Serverless Architecture
- Common Challenges and Solutions in Serverless Architecture
- 1. Cold Start Latency
- 2. Limited Execution Time
- 3. Vendor Lock-in
What is Serverless Architecture?
Serverless architecture, also known as Function as a Service (FaaS), is a cloud computing model where the cloud provider manages the infrastructure and automatically provisions, scales, and manages the servers required to run applications. In this model, developers focus on writing and deploying individual functions or pieces of code, rather than managing servers or infrastructure.
In a traditional server-based architecture, developers need to provision and manage servers to handle the application’s workload. This involves tasks like capacity planning, server maintenance, and scaling. However, with serverless architecture, developers can focus solely on writing code and let the cloud provider handle the rest.
Serverless architecture offers several advantages over traditional server-based architectures. Firstly, it allows for automatic scaling based on the actual usage of the application. If an application receives a sudden surge in traffic, the cloud provider can automatically scale up the required resources to handle the load. This eliminates the need for manual scaling and ensures that the application remains responsive even under heavy traffic.
Secondly, serverless architecture provides a pay-per-use pricing model. With traditional servers, developers often pay for resources that are underutilized during periods of low traffic. In contrast, with serverless architecture, developers only pay for the actual execution time of their functions. This can result in significant cost savings, especially for applications with varying workloads.
Another benefit of serverless architecture is improved developer productivity. Since developers don’t need to worry about managing servers, they can focus more on writing code and delivering features. Serverless architecture also promotes modular and reusable code, as functions are designed to be independent and loosely coupled.
Let’s take a look at a simple example to understand how serverless architecture works. Consider a web application that needs to process images uploaded by users. In a traditional server-based architecture, developers would need to provision and manage servers to handle the image processing workload. However, with serverless architecture, developers can write a function specifically for image processing and deploy it as a serverless function.
Here’s an example of an image processing function written in Node.js:
// imageProcessing.js exports.handler = async (event) => { // Process the image // ... return { statusCode: 200, body: 'Image processed successfully', }; };
In this example, the function takes an event parameter, which contains information about the image to be processed. The function can then perform the necessary image processing tasks and return a response.
To deploy this function as a serverless function, you can use a cloud provider like AWS Lambda. The cloud provider takes care of provisioning the necessary resources to run the function and automatically scales them based on the incoming requests.
Serverless architecture has gained popularity in recent years due to its scalability, cost-effectiveness, and developer productivity benefits. It is commonly used for various use cases, such as web and mobile backends, data processing pipelines, and event-driven applications.
Overall, serverless architecture offers a new paradigm for building and deploying applications, where developers can focus on writing code without worrying about managing servers or infrastructure.
Related Article: How to use AWS Lambda for Serverless Computing
The Benefits of Serverless Architecture
Serverless architecture has gained significant popularity in recent years due to its numerous benefits. In this chapter, we will explore some of the key advantages of adopting a serverless approach for building and deploying applications.
1. Cost Efficiency
One of the most significant benefits of serverless architecture is its cost efficiency. With serverless computing, you only pay for the actual usage of your application, rather than provisioning and maintaining a fixed number of servers. This reduces the overhead costs associated with infrastructure management, such as hardware provisioning, software licensing, and system administration.
Consider the following example of a serverless function written in Node.js:
const handler = async (event) => { // Process the event and return a response }; module.exports = { handler };
In this example, you define a single function that gets executed in response to an event. The cloud provider automatically scales the execution environment based on the incoming workload, ensuring optimal resource utilization and cost savings.
2. Scalability and Elasticity
Serverless architecture offers unparalleled scalability and elasticity. As the cloud provider handles the scaling of your application, you don’t have to worry about provisioning additional resources or configuring load balancers. This allows your application to automatically scale to handle any amount of incoming traffic, ensuring a seamless user experience.
Let’s take a look at an example of how serverless architecture enables automatic scaling. Suppose you have an API endpoint that performs image processing tasks. As the number of requests increases, the cloud provider automatically scales the execution environment to handle the workload efficiently.
import boto3 def process_image(event, context): # Process the image and return the result return { 'statusCode': 200, 'body': 'Image processed successfully' }
In this example, a serverless function written in Python receives an event containing the image to be processed. The cloud provider automatically scales the infrastructure to handle concurrent requests, ensuring optimal performance.
3. Reduced Operational Complexity
Serverless architecture simplifies operational complexity by abstracting away the underlying infrastructure management. With serverless computing, you can focus on writing code and delivering business value without worrying about server provisioning, patching, or monitoring.
Consider the following example of a serverless application using AWS Lambda:
Resources: MyFunction: Type: AWS::Lambda::Function Properties: CodeUri: my-function/ Handler: index.handler Runtime: nodejs14.x
In this example, you define the deployment configuration for a serverless function using AWS CloudFormation. The cloud provider takes care of deploying and managing the function, allowing you to focus on developing the application logic.
4. Faster Time-to-Market
Serverless architecture enables faster time-to-market by accelerating the development and deployment cycles. With serverless computing, you can quickly prototype and iterate on your application without worrying about infrastructure setup or deployment pipelines.
Consider the following example of a serverless application using Azure Functions:
public static class MyFunction { [FunctionName("MyFunction")] public static async Task Run( [HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req, ILogger log) { // Process the request and return a response return new OkObjectResult("Request processed successfully"); } }
In this example, a serverless function written in C# using Azure Functions receives an HTTP request and processes it. The cloud provider takes care of deploying and managing the function, allowing you to focus on the application logic.
Serverless architecture empowers developers to build and deploy applications rapidly, enabling organizations to bring new features and products to market faster than ever before.
In the next chapter, we will explore the different use cases where serverless architecture shines and discuss its limitations.
Getting Started with Serverless Architecture
Serverless architecture is a paradigm that allows developers to build and run applications without the need to manage servers or infrastructure. It provides a highly scalable and cost-effective solution for running applications, as you only pay for the actual usage of the resources.
In this chapter, we will explore the basics of serverless architecture and how to get started with building serverless applications.
Understanding Serverless Architecture
Serverless architecture is based on the concept of Function as a Service (FaaS). Instead of deploying an entire application on a server, you break it down into smaller functions that can be executed independently. These functions are triggered by events and run in a managed environment provided by a cloud provider.
The main benefits of serverless architecture are:
– Reduced operational overhead: With serverless, you don’t need to worry about managing servers, scaling, or maintaining infrastructure. The cloud provider takes care of all these aspects, allowing you to focus on writing code.
– Automatic scaling: Serverless platforms automatically scale your functions based on the incoming traffic. You don’t need to provision additional resources or worry about handling peaks in traffic.
– Pay-per-use pricing: With serverless, you only pay for the actual usage of your functions. You don’t have to pay for idle resources, which makes it a cost-effective solution.
Choosing a Serverless Platform
There are several serverless platforms available, each with its own set of features and integrations. Some popular serverless platforms include:
– AWS Lambda: Amazon Web Services (AWS) Lambda is one of the most widely used serverless platforms. It supports multiple programming languages and integrates well with other AWS services.
– Azure Functions: Microsoft Azure Functions is a serverless compute service that allows you to run your code without provisioning or managing servers. It supports multiple programming languages and integrates well with other Azure services.
– Google Cloud Functions: Google Cloud Functions is a serverless execution environment that allows you to run your code in response to events. It supports multiple programming languages and integrates well with other Google Cloud services.
Writing Serverless Functions
Serverless functions are typically small pieces of code that perform a specific task. They can be written in various programming languages, depending on the serverless platform you choose.
Let’s take a look at an example of a serverless function written in Node.js using AWS Lambda:
exports.handler = async (event, context) => { const { name } = JSON.parse(event.body); return { statusCode: 200, body: JSON.stringify({ message: `Hello, ${name}!` }) }; };
In this example, the function receives an HTTP request with a JSON payload containing a “name” property. It then responds with a JSON payload containing a greeting message.
Deploying Serverless Functions
Once you have written your serverless functions, you need to deploy them to a serverless platform. Each platform provides its own deployment mechanism, usually through a command-line interface (CLI) or a graphical user interface (GUI).
For example, to deploy the above AWS Lambda function, you can use the AWS CLI:
$ aws lambda create-function --function-name helloWorld --runtime nodejs14.x --handler index.handler --zip-file fileb://function.zip
This command creates a new AWS Lambda function named “helloWorld” using Node.js 14.x runtime. The function code is packaged in a ZIP file called “function.zip”.
Testing and Monitoring Serverless Functions
Testing and monitoring serverless functions is crucial to ensure their reliability and performance. Most serverless platforms provide tools and services for testing and monitoring functions.
For example, AWS Lambda provides the AWS X-Ray service for distributed tracing and performance monitoring. You can use it to trace requests as they flow through your serverless functions and identify any performance bottlenecks.
Use Cases for Serverless Architecture
Serverless architecture has gained significant popularity in recent years due to its numerous benefits, such as reduced operational costs, improved scalability, and increased development speed. This chapter explores some of the common use cases where serverless architecture shines and provides real-world examples.
1. Web Applications
Serverless architecture is well-suited for building web applications that require frequent updates and can experience unpredictable traffic patterns. By leveraging serverless services like AWS Lambda or Azure Functions, developers can focus on writing application logic without worrying about server management. This allows for faster deployment and flexibility to handle varying user loads. Consider the following example of a serverless web application using AWS Lambda and API Gateway:
// File: index.js (AWS Lambda Function) exports.handler = async (event) => { const response = { statusCode: 200, body: 'Hello, serverless world!', }; return response; };
2. Event Processing and Streaming
Serverless architecture is an excellent choice for event-driven applications that process and react to real-time data streams. Services like AWS EventBridge, AWS Kinesis, or Azure Event Grid enable developers to handle events from various sources, such as IoT devices, application logs, or user actions. This allows for scalable event processing without the need to provision and manage dedicated servers. Here’s an example of processing events using AWS Lambda:
// File: index.js (AWS Lambda Function) exports.handler = async (event) => { for (const record of event.Records) { console.log(`Processing event: ${JSON.stringify(record)}`); // Perform event processing logic here } };
3. Microservices Architecture
Serverless architecture is an ideal fit for implementing microservices, where each service performs a specific function independently. By leveraging serverless functions, developers can build and deploy individual microservices that can be easily integrated with other services using APIs or message queues. This modular approach allows for better scalability and maintainability. Consider this example of a serverless microservice using AWS Lambda:
// File: index.js (AWS Lambda Function) exports.handler = async (event) => { // Process input event const result = await someLogic(event); // Return response return { statusCode: 200, body: JSON.stringify(result), }; };
4. Batch Processing and Cron Jobs
Serverless architecture is well-suited for executing periodic tasks, such as batch processing or scheduled jobs. Services like AWS Step Functions or Azure Logic Apps enable developers to define workflows and schedule tasks without the need for managing servers. This allows for efficient resource utilization and cost savings. Here’s an example of a serverless cron job using AWS CloudWatch Events and AWS Lambda:
// File: index.js (AWS Lambda Function) exports.handler = async (event) => { // Perform batch processing or scheduled task console.log('Executing cron job...'); // ... };
These are just a few examples of how serverless architecture can be applied to various use cases. Whether it’s building web applications, processing real-time events, implementing microservices, or executing scheduled tasks, serverless architecture offers a flexible and scalable solution. The next chapter will explore the challenges and considerations when adopting serverless architecture.
Real World Examples of Serverless Architecture
Serverless architecture has gained popularity in recent years due to its flexibility, scalability, and cost-effectiveness. Many companies and organizations have adopted serverless architecture to build and deploy their applications. In this section, we will explore some real-world examples of serverless architecture and see how it has been used to solve various challenges.
1. AWS Lambda: AWS Lambda is one of the most popular serverless computing platforms, provided by Amazon Web Services (AWS). It allows you to run your code without provisioning or managing servers. Lambda functions are event-driven and can be triggered by various AWS services like S3, DynamoDB, API Gateway, etc. Many companies, including Netflix, Airbnb, and NASA, have leveraged AWS Lambda to build scalable and cost-efficient applications.
Here’s an example of a simple AWS Lambda function written in Python:
import json def lambda_handler(event, context): body = { "message": "Hello, world!" } response = { "statusCode": 200, "body": json.dumps(body) } return response
2. Google Cloud Functions: Google Cloud Functions is Google’s serverless computing platform, similar to AWS Lambda. It allows you to write and deploy functions that automatically scale in response to events. Cloud Functions can be triggered by events from various Google Cloud services, such as Cloud Storage, Pub/Sub, and Firestore. Companies like Coca-Cola, Spotify, and Snapchat have utilized Google Cloud Functions for their applications.
Here’s an example of a simple Google Cloud Function written in Node.js:
exports.helloWorld = (req, res) => { const message = 'Hello, world!'; res.status(200).send(message); };
3. Microsoft Azure Functions: Microsoft Azure Functions is a serverless compute service provided by Microsoft Azure. It allows you to run your code in a serverless environment without worrying about infrastructure management. Azure Functions support multiple programming languages and can be triggered by various Azure services like Blob Storage, Event Hubs, and Cosmos DB. Companies like BMW, Adobe, and 3M have utilized Azure Functions for their applications.
Here’s an example of a simple Azure Function written in C#:
using System.Net; using Microsoft.AspNetCore.Mvc; using Microsoft.Extensions.Logging; public static async Task Run(HttpRequest req, ILogger log) { log.LogInformation("C# HTTP trigger function processed a request."); string responseMessage = "Hello, world!"; return new OkObjectResult(responseMessage); }
These are just a few examples of how serverless architecture is being used in the real world. Many other cloud providers and platforms offer serverless computing capabilities, allowing developers to focus on writing code without the need to manage servers.
Serverless architecture has revolutionized the way applications are built and deployed, providing flexibility, scalability, and cost savings. By leveraging serverless technologies, companies can develop and scale their applications faster and more efficiently, ultimately delivering better products and services to their customers.
Advanced Techniques in Serverless Architecture
Serverless architecture provides a flexible and scalable approach to building applications. In this chapter, we will explore advanced techniques that can enhance the performance, reliability, and security of serverless applications.
1. Event-driven Architecture
Event-driven architecture is a fundamental concept in serverless architecture. Instead of relying on traditional request-response patterns, serverless applications respond to events triggered by external services or user actions. This enables applications to be highly responsive and scalable.
Here’s an example of an event-driven function in Python:
import json def event_handler(event, context): # Process the event data event_data = json.loads(event['body']) # Perform necessary actions based on the event # ... return { 'statusCode': 200, 'body': json.dumps({'message': 'Event processed successfully'}) }
2. Asynchronous Processing
Asynchronous processing is a technique used to decouple the execution of tasks from the request-response cycle. It allows the serverless application to handle multiple tasks in parallel, improving overall performance and responsiveness.
Here’s an example of asynchronous processing using AWS Lambda and SNS:
import boto3 def process_task(event, context): # Perform time-consuming task # ... # Notify completion using SNS sns = boto3.client('sns') sns.publish( TopicArn='arn:aws:sns:us-west-2:123456789012:task-completion', Message='Task completed successfully' )
3. Cold Start Mitigation
Cold start refers to the delay experienced when a function is invoked for the first time or after a period of inactivity. To mitigate cold starts and improve performance, there are several techniques available:
– Provisioned Concurrency: By pre-warming function instances, you can reduce cold starts. This feature is available in some serverless platforms like AWS Lambda.
– Keep Warm Functions: Implementing a separate function that periodically invokes the main function keeps the function instance warm, reducing cold start times.
4. Distributed Tracing
Distributed tracing allows you to monitor and analyze the behavior of a serverless application across multiple functions and services. It helps identify performance bottlenecks, latency issues, and errors. Tools like AWS X-Ray and OpenTelemetry provide distributed tracing capabilities for serverless architectures.
5. Security Considerations
When working with serverless architecture, it is crucial to consider security measures to protect your application and data. Some best practices include:
– Least Privilege Principle: Assign the minimum necessary permissions to your serverless functions.
– Secure Configuration: Ensure that sensitive configuration values, such as API keys or database credentials, are securely stored and not exposed in your code.
– Input Validation: Validate and sanitize user input to prevent common security vulnerabilities like injection attacks.
In this chapter, we explored advanced techniques in serverless architecture, including event-driven architecture, asynchronous processing, cold start mitigation, distributed tracing, and security considerations. By leveraging these techniques, you can build robust and scalable serverless applications.
Scaling and Performance Optimization in Serverless Architecture
Scaling and performance optimization are crucial aspects of serverless architecture. With serverless computing, the cloud provider manages the infrastructure, allowing developers to focus on writing code without worrying about server management. However, as the user base grows and the workload increases, it becomes essential to scale the serverless application to maintain performance and ensure a smooth user experience.
Automatic Scaling
One of the significant benefits of serverless architecture is automatic scaling. Cloud providers like AWS Lambda, Azure Functions, and Google Cloud Functions offer built-in auto-scaling capabilities. These services automatically scale the number of function instances based on the incoming request rate. When the number of requests exceeds the capacity of the current instances, the cloud provider provisions additional instances to handle the load. This automatic scaling ensures that your application can handle sudden spikes in traffic without any manual intervention.
Concurrency and Throttling
Concurrency refers to the number of requests that can be processed simultaneously by a serverless function. Each cloud provider has its own concurrency limits, which can be configured to optimize the performance of your serverless application. It’s important to understand these limits and adjust them according to your application’s requirements.
Throttling is a mechanism to control the rate at which requests are processed. It helps prevent abuse and ensures fair resource allocation. When the number of incoming requests exceeds the concurrency limit, the cloud provider may start throttling requests, which means some requests will be rejected and receive an error response. To avoid throttling, you can request a limit increase or implement backoff and retry strategies in your code.
Here’s an example of how you can handle throttling in AWS Lambda using the AWS SDK for JavaScript:
const AWS = require('aws-sdk'); const lambda = new AWS.Lambda(); const invokeLambdaFunction = async (payload) => { try { const response = await lambda.invoke({ FunctionName: 'myLambdaFunction', Payload: JSON.stringify(payload) }).promise(); return JSON.parse(response.Payload); } catch (error) { if (error.code === 'TooManyRequestsException') { // Implement backoff and retry logic here } throw error; } };
Caching
Caching is another technique to improve the performance of serverless applications. By caching frequently accessed data or computation results, you can reduce the number of calls made to external services or expensive computations. This not only improves the response time but also reduces the cost of your serverless functions.
Many cloud providers offer caching services like Amazon ElastiCache, Azure Cache for Redis, or Google Cloud Memorystore, which can be integrated with your serverless application. Additionally, you can also leverage in-memory caching within your functions using libraries like Redis or Memcached.
Optimizing Cold Start Time
Cold start is the delay that occurs when a serverless function is invoked for the first time or after a certain period of inactivity. During a cold start, the cloud provider needs to allocate resources and set up the execution environment for the function, which can result in increased latency.
To optimize cold start time, you can:
1. Use a smaller deployment package to reduce the time required for deployment and initialization.
2. Utilize provisioned concurrency, a feature provided by some cloud providers, to pre-warm function instances and reduce cold starts.
3. Implement application-level caching to reuse resources across multiple invocations.
It’s worth noting that while cold starts can impact the performance of your serverless application, they are typically less significant for functions with frequent invocations.
In conclusion, scaling and performance optimization are critical considerations in serverless architecture. Automatic scaling, managing concurrency and throttling, caching, and optimizing cold start time are key strategies to ensure your serverless application can handle increased workloads while providing an optimal user experience.
Security Considerations in Serverless Architecture
Security is a crucial aspect to consider when designing and implementing serverless architectures. While serverless platforms provide various security benefits, there are still some important considerations to keep in mind. In this chapter, we will explore some key security considerations in serverless architecture.
1. Authentication and Authorization
Authentication and authorization are fundamental aspects of securing any application, including those built using serverless architecture. It is important to ensure that only authorized users or systems can access your serverless functions and resources.
One common approach is to use authentication mechanisms such as JSON Web Tokens (JWT) or OAuth 2.0 to authenticate clients before allowing them to invoke serverless functions. These mechanisms verify the identity of the client and provide an access token that can be used for subsequent requests.
Authorization can be achieved by implementing appropriate access controls and permissions for each serverless function. For example, you can use AWS Identity and Access Management (IAM) policies to define fine-grained permissions for different functions and resources.
2. Data Protection
Data protection is another critical aspect of security in serverless architecture. When handling sensitive data, it is important to ensure its confidentiality, integrity, and availability.
One way to protect data is by using encryption. You can encrypt data both at rest and in transit. For example, you can encrypt data stored in a database using server-side encryption or implement transport layer security (TLS) to encrypt data transmitted over the network.
Additionally, it is important to carefully handle and manage any secrets or sensitive information used by your serverless functions. Avoid hardcoding secrets in your code and instead use secure storage solutions such as AWS Secrets Manager or environment variables.
3. Function Isolation and Privilege Separation
Serverless platforms typically provide function-level isolation, where each function runs in its own environment. This provides a level of security by preventing functions from accessing each other’s resources or data.
However, it is still crucial to ensure that your serverless functions have the appropriate level of privilege separation. Avoid granting excessive permissions to functions and follow the principle of least privilege. Only provide the necessary permissions for each function to perform its intended tasks.
4. Logging and Monitoring
Logging and monitoring are essential for detecting and responding to security incidents in serverless architectures. By monitoring your serverless functions and their associated resources, you can quickly identify any suspicious activities or potential security breaches.
Make sure to enable logging for your serverless functions and configure log retention policies. You can use services like Amazon CloudWatch to aggregate and analyze logs from multiple functions and gain insights into their behavior.
In addition to logging, consider implementing real-time monitoring and alerting mechanisms. This can be achieved using services like AWS CloudTrail, which provides a comprehensive audit trail of API calls made within your serverless environment.
5. Third-Party Dependencies
Serverless architectures often rely on third-party dependencies such as libraries, frameworks, or external APIs. While these dependencies can greatly enhance the functionality of your serverless functions, they also introduce potential security risks.
It is important to regularly update and patch any third-party dependencies to address known security vulnerabilities. Keep a close eye on security announcements and updates from the providers of these dependencies and ensure that you promptly apply any necessary fixes.
Furthermore, carefully review and validate the security practices of third-party dependencies before incorporating them into your serverless architecture. Consider performing security assessments and audits to ensure their reliability and trustworthiness.
By considering these security considerations in serverless architecture, you can build robust and secure applications that protect your data and resources. Remember that security should be an ongoing process, and it is important to regularly review and update your security measures as new threats and vulnerabilities emerge.
Monitoring and Debugging in Serverless Architecture
Monitoring and debugging are crucial aspects of serverless architecture, as they allow developers to identify and resolve issues in their applications. In a serverless environment, where code is executed in response to events, monitoring and debugging can be more challenging compared to traditional architectures. This chapter explores various techniques and tools that can help with monitoring and debugging in serverless architecture.
Logging
Logging is one of the primary ways to monitor and debug serverless applications. It involves capturing and storing relevant information about the execution of the code. Serverless platforms typically provide built-in logging capabilities that allow developers to log important events and messages during the execution of their functions.
Here’s an example of logging in a Node.js function deployed on AWS Lambda:
exports.handler = async (event, context) => { console.log('Received event:', event); console.log('Function name:', context.functionName); // Your code logic here return 'Function executed successfully'; };
In this example, we use the console.log
function to log the received event and the function name. These logs can be viewed in the AWS CloudWatch Logs console, which provides a centralized location for monitoring and troubleshooting serverless applications.
Distributed Tracing
Distributed tracing is a technique that helps in understanding the flow of requests across multiple services or functions in a serverless application. It provides visibility into how requests propagate through different components, making it easier to identify bottlenecks or performance issues.
There are several tools available for distributed tracing in serverless architectures, such as AWS X-Ray, OpenTelemetry, and Jaeger. These tools allow you to instrument your code and capture information about requests, including latency, errors, and dependencies.
Here’s an example of using AWS X-Ray for distributed tracing in a Python function deployed on AWS Lambda:
import aws_xray_sdk.core aws_xray_sdk.core.patch_all() def lambda_handler(event, context): # Your code logic here return { 'statusCode': 200, 'body': 'Function executed successfully' }
In this example, we import the aws_xray_sdk.core
module and call the patch_all()
function to enable X-Ray tracing. This will automatically instrument your code to capture trace information, which can then be visualized in the AWS X-Ray console.
Metrics and Alerts
Monitoring serverless applications involves collecting and analyzing metrics to gain insights into their performance and behavior. Metrics provide valuable information about resource usage, function invocations, and other relevant data points.
Serverless platforms often provide built-in metrics and monitoring capabilities. For example, AWS Lambda automatically collects metrics such as invocation count, duration, and error rates. These metrics can be visualized in the AWS CloudWatch Metrics console or used to set up alarms and alerts.
Here’s an example of setting up an alarm based on a metric in AWS CloudWatch:
Resources: MyAlarm: Type: AWS::CloudWatch::Alarm Properties: AlarmDescription: "High error rate" Namespace: AWS/Lambda MetricName: Errors Dimensions: - Name: FunctionName Value: MyLambdaFunction Statistic: SampleCount Period: 60 EvaluationPeriods: 1 Threshold: 1 ComparisonOperator: GreaterThanOrEqualToThreshold
In this example, we define a CloudWatch Alarm that triggers when the error count for a specific Lambda function (MyLambdaFunction) exceeds a threshold of 1. This allows you to receive notifications when errors occur, helping you proactively identify and address issues in your serverless applications.
Debugging
Debugging serverless applications can be challenging due to the distributed nature of the architecture. However, there are techniques and tools available to help with debugging in a serverless environment.
Many serverless platforms provide debugging capabilities that allow developers to set breakpoints, inspect variables, and step through code during execution. For example, AWS Lambda supports remote debugging using IDEs like Visual Studio Code and PyCharm.
Here’s an example of using the AWS Toolkit for Visual Studio Code to debug a Node.js function deployed on AWS Lambda:
1. Install the AWS Toolkit extension in Visual Studio Code.
2. Open the function code in Visual Studio Code.
3. Set breakpoints in the code.
4. Use the “Start Debugging” option to deploy and execute the function in debug mode.
5. The debugger will stop at the breakpoints, allowing you to inspect variables and step through the code.
Debugging tools like these can greatly simplify the process of identifying and fixing issues in serverless applications.
Monitoring and debugging are essential for maintaining the health and performance of serverless applications. By leveraging logging, distributed tracing, metrics, and debugging tools, developers can gain insights into their applications, troubleshoot issues, and ensure the efficient operation of their serverless architecture.
Serverless Frameworks and Tools
Serverless architecture has gained significant popularity in recent years due to its scalability, cost efficiency, and reduced operational overhead. To facilitate the development and deployment of serverless applications, several frameworks and tools have emerged. These frameworks and tools simplify the process of building, deploying, and managing serverless applications by providing a higher-level abstraction and automating common tasks.
AWS Serverless Application Model (SAM)
The AWS Serverless Application Model (SAM) is an open-source framework that extends AWS CloudFormation to simplify the deployment of serverless applications. It provides a simplified syntax for defining serverless resources such as functions, APIs, and event sources. SAM templates are written in YAML or JSON and can be easily deployed using the AWS Command Line Interface (CLI) or the AWS Management Console.
Here’s an example of a SAM template defining a basic serverless application with a Lambda function and an API Gateway:
AWSTemplateFormatVersion: "2010-09-09" Transform: AWS::Serverless-2016-10-31 Resources: MyFunction: Type: AWS::Serverless::Function Properties: Handler: index.handler Runtime: nodejs12.x CodeUri: ./ Events: MyApi: Type: Api Properties: Path: /my-api Method: get
Serverless Framework
The Serverless Framework is a popular open-source framework that supports multiple cloud providers, including AWS, Azure, and Google Cloud Platform. It simplifies the development and deployment of serverless applications by providing a unified and vendor-agnostic way to define functions, events, and resources. The framework supports various programming languages and provides a command-line interface for managing serverless projects.
Here’s an example of a Serverless Framework configuration file defining a serverless function with an HTTP event:
service: my-service provider: name: aws runtime: nodejs12.x functions: hello: handler: handler.hello events: - http: path: hello method: get
Azure Functions
Azure Functions is a serverless computing platform provided by Microsoft Azure. It allows developers to build and deploy event-driven, scalable, and cost-effective applications without worrying about infrastructure management. Azure Functions supports multiple programming languages and provides integration with various Azure services and event sources.
Here’s an example of an Azure Functions JavaScript function with an HTTP trigger:
module.exports = async function (context, req) { context.log('JavaScript HTTP trigger function processed a request.'); const responseMessage = "Hello, Azure Functions!"; context.res = { body: responseMessage }; }
Google Cloud Functions
Google Cloud Functions is a serverless computing platform provided by Google Cloud Platform. It allows developers to build and deploy event-driven applications using various programming languages. Google Cloud Functions integrates well with other Google Cloud services and provides automatic scaling, pay-per-execution pricing, and easy deployment.
Here’s an example of a Google Cloud Functions Python function with an HTTP trigger:
def hello_http(request): return 'Hello, Google Cloud Functions!'
These are just a few examples of the serverless frameworks and tools available in the market. Each framework and tool has its own unique features, syntax, and ecosystem. When choosing a serverless framework or tool, consider factors such as supported cloud providers, programming language support, community support, and integration capabilities with other services.
Integration with Other Services and APIs
Serverless architecture allows for seamless integration with various services and APIs, enabling developers to build powerful and scalable applications. This chapter explores how serverless functions can interact with external services and APIs to enhance functionality and create dynamic applications.
Calling External APIs
One of the key advantages of serverless architecture is the ability to easily integrate with external APIs. Whether you need to fetch data from a third-party service or send data to another application, serverless functions can handle these tasks efficiently.
Here’s an example of a serverless function written in JavaScript using AWS Lambda that fetches data from the OpenWeatherMap API:
const fetch = require('node-fetch'); exports.handler = async function(event, context) { const apiKey = 'YOUR_API_KEY'; const city = event.queryStringParameters.city; const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${apiKey}`; const response = await fetch(url); const data = await response.json(); return { statusCode: 200, body: JSON.stringify(data) }; };
In this example, the function receives a city parameter through the event object and uses it to construct the API request URL. It then fetches the weather data for that city and returns it as the response.
Triggering Events in Other Services
Serverless functions can also be used to trigger events in other services, such as sending notifications, updating databases, or initiating workflows. This enables seamless integration between different components of your application.
For instance, let’s say you want to send a notification to a user whenever a new record is added to a database. You can use a serverless function to achieve this. Here’s an example using AWS Lambda and Amazon Simple Notification Service (SNS):
const AWS = require('aws-sdk'); const sns = new AWS.SNS(); exports.handler = async function(event, context) { const message = event.Records[0].Sns.Message; // Send a notification using SNS await sns.publish({ Message: message, TopicArn: 'YOUR_TOPIC_ARN' }).promise(); return { statusCode: 200, body: 'Notification sent successfully' }; };
In this example, the function receives the message from an SNS topic subscription and uses the AWS SDK to publish a notification to another SNS topic.
Working with Data Storage Services
Serverless architecture seamlessly integrates with various data storage services, such as databases, object storage, and caching systems. This allows you to build applications that can store and retrieve data efficiently.
For example, if you are using AWS Lambda, you can easily interact with Amazon DynamoDB, a fully managed NoSQL database service. Here’s an example of a Lambda function that retrieves data from DynamoDB:
const AWS = require('aws-sdk'); const dynamodb = new AWS.DynamoDB.DocumentClient(); exports.handler = async function(event, context) { const params = { TableName: 'YOUR_TABLE_NAME', Key: { id: 'YOUR_RECORD_ID' } }; const data = await dynamodb.get(params).promise(); return { statusCode: 200, body: JSON.stringify(data.Item) }; };
In this example, the function retrieves a record from DynamoDB based on its ID. The retrieved data is then returned as the response.
Data Storage and Management in Serverless Architecture
Serverless architecture allows developers to focus on writing code without the need to manage infrastructure. However, data storage and management are still crucial aspects of any serverless application. In this chapter, we will explore various options for storing and managing data in a serverless architecture.
1. Serverless Databases
Serverless databases are designed specifically for serverless applications, providing scalability and flexibility. They handle scaling and provisioning of resources automatically, allowing developers to focus on building the application logic. Some popular serverless databases include:
– Amazon DynamoDB: A fully managed NoSQL database service provided by AWS. It offers high scalability, low latency, and automatic scaling based on demand.
– Google Cloud Firestore: A flexible, scalable NoSQL database provided by Google Cloud. It offers real-time synchronization and seamless integration with other Google Cloud services.
– Azure Cosmos DB: A globally distributed, multi-model database service provided by Microsoft Azure. It supports multiple database models, including document, key-value, graph, and column-family.
Here’s an example of using DynamoDB in a serverless application:
import boto3 dynamodb = boto3.resource('dynamodb') table = dynamodb.Table('my_table') def lambda_handler(event, context): # Perform operations on the DynamoDB table response = table.get_item(Key={'id': '123'}) item = response['Item'] # ... return { 'statusCode': 200, 'body': 'Success' }
2. Object Storage
Object storage is ideal for storing and retrieving large amounts of unstructured data, such as images, videos, and documents. It provides high durability and availability, making it suitable for serverless applications. Popular object storage services include:
– Amazon S3: A highly scalable and durable object storage service provided by AWS. It offers fine-grained access controls, versioning, and lifecycle management.
– Google Cloud Storage: A scalable and secure object storage service provided by Google Cloud. It offers multi-regional, regional, and nearline storage classes to optimize costs.
– Azure Blob Storage: A massively scalable and secure object storage service provided by Microsoft Azure. It supports hot, cool, and archive storage tiers for cost optimization.
Here’s an example of using Amazon S3 in a serverless application:
const AWS = require('aws-sdk'); const s3 = new AWS.S3(); exports.handler = async (event) => { // Fetch an object from S3 const params = { Bucket: 'my-bucket', Key: 'my-object-key' }; const data = await s3.getObject(params).promise(); // ... return { statusCode: 200, body: 'Success' }; };
3. Managed File Systems
Managed file systems provide shared file storage that can be accessed by multiple serverless functions concurrently. They are suitable for scenarios where multiple functions need access to the same set of files. Some popular managed file system services include:
– Amazon EFS: A fully managed, highly available, and durable file system provided by AWS. It supports concurrent access from multiple instances and containers.
– Google Cloud Filestore: A scalable and high-performance file storage service provided by Google Cloud. It offers POSIX-compliant file system semantics and provides high throughput.
– Azure Files: A fully managed file share service provided by Microsoft Azure. It supports SMB and NFS protocols and can be accessed from both cloud and on-premises deployments.
Here’s an example of using Amazon EFS in a serverless application:
import os def lambda_handler(event, context): # Read from a file in Amazon EFS file_path = '/mnt/efs/my-file.txt' with open(file_path, 'r') as file: content = file.read() # ... return { 'statusCode': 200, 'body': 'Success' }
In this chapter, we explored various options for storing and managing data in a serverless architecture. Serverless databases, object storage, and managed file systems provide scalable and flexible solutions for different data storage requirements. Choose the appropriate storage service based on your application’s needs and leverage the benefits of serverless architecture.
Event-driven Architecture with Serverless
Event-driven architecture is a key aspect of serverless computing. It allows applications to respond to events and triggers, enabling a highly scalable and responsive system. In this chapter, we will explore the concept of event-driven architecture and how it can be implemented with serverless technologies.
Understanding Event-driven Architecture
Event-driven architecture (EDA) is a software design pattern that emphasizes the production, detection, and consumption of events. An event can be any occurrence or notification of interest within a system. Examples of events include user actions, data updates, messages, or even system-level events like a new file being created.
In an event-driven architecture, components of the system communicate through events. When an event occurs, it triggers a response from one or more components that have subscribed to that event. This decoupled communication pattern allows for loose coupling, scalability, and flexibility.
Implementing Event-driven Architecture with Serverless
Serverless computing platforms, such as AWS Lambda or Azure Functions, are particularly well-suited for implementing event-driven architectures. These platforms provide the infrastructure and tools necessary to handle events and execute code in response.
Let’s take a look at a simple example of implementing event-driven architecture with AWS Lambda. Suppose we have an application that needs to process images whenever they are uploaded to an S3 bucket. We can use Lambda to process the images as soon as they are uploaded by subscribing to the S3 bucket’s “ObjectCreated” event.
import boto3 def process_image(event, context): s3 = boto3.client('s3') for record in event['Records']: bucket_name = record['s3']['bucket']['name'] key = record['s3']['object']['key'] # Process the image # ...
In the above code snippet, we define a Lambda function called process_image
that is triggered whenever an object is created in the specified S3 bucket. The function retrieves the bucket name and key from the event payload and then performs the required image processing operations.
This simple example demonstrates the power of event-driven architecture with serverless. By leveraging serverless platforms like AWS Lambda, we can easily respond to events and scale our application based on demand.
Benefits of Event-driven Architecture
Event-driven architecture offers several benefits for building scalable and resilient systems:
1. Scalability: By decoupling components and leveraging serverless platforms, event-driven architectures can scale dynamically based on the volume of events.
2. Flexibility: Components can be added or removed without affecting the overall system. New functionalities can be easily implemented by subscribing to relevant events.
3. Fault tolerance: Event-driven systems are inherently resilient as components can handle failures independently. If one component fails, others can continue processing events.
4. Real-time responsiveness: Events are processed as they occur, allowing for real-time or near-real-time responsiveness to user actions or system events.
Building APIs with Serverless Functions
Serverless architecture provides a great way to build scalable and cost-effective APIs. By leveraging serverless functions, developers can focus on writing code rather than managing infrastructure. In this chapter, we will explore how to build APIs using serverless functions.
What are Serverless Functions?
Serverless functions, also known as function-as-a-service (FaaS), are small units of code that are executed on demand. They are event-driven, meaning they are triggered by specific events such as HTTP requests, database changes, or timers. Serverless functions are stateless, meaning they don’t retain any information between invocations.
Benefits of Using Serverless Functions for APIs
Using serverless functions to build APIs offers several benefits:
1. Scalability: Serverless functions can easily scale to handle high traffic loads without any manual intervention. The cloud provider takes care of resource allocation and scaling based on the demand.
2. Cost-Efficiency: With serverless functions, you only pay for the actual execution time and resources used. There are no fixed costs for idle resources, making it a cost-effective option.
3. Reduced Operational Overhead: Serverless functions abstract away the infrastructure management, allowing developers to focus solely on writing business logic. Deployment, scaling, and monitoring are handled by the cloud provider.
4. Easy Integration: Serverless functions can easily integrate with various services and APIs. This makes it convenient to incorporate third-party services or connect to databases, message queues, or other backend systems.
Creating a Serverless API
To create a serverless API, we need to define the endpoints and the corresponding serverless functions that will handle the requests. Let’s take a look at an example using AWS Lambda and API Gateway.
First, we need to define the serverless function that will handle the HTTP requests. Here’s an example using Node.js:
// api.js exports.handler = async (event) => { // Extract the HTTP method and request body from the event const { httpMethod, body } = event; // Process the request based on the HTTP method if (httpMethod === 'GET') { // Handle GET request return { statusCode: 200, body: JSON.stringify({ message: 'Hello, World!' }), }; } else if (httpMethod === 'POST') { // Handle POST request with the request body return { statusCode: 200, body: JSON.stringify({ message: `Received: ${body}` }), }; } else { // Return an error for unsupported HTTP methods return { statusCode: 400, body: JSON.stringify({ message: 'Unsupported HTTP method' }), }; } };
Next, we need to configure the API Gateway to route the requests to our serverless function. This can be done through the AWS Management Console or using an infrastructure-as-code tool like AWS CloudFormation or Terraform.
Once the API Gateway is configured, we can start making requests to our API endpoints. For example, to make a GET request to our API, we can use the following URL:
GET https://api.example.com/hello
This will trigger the serverless function, which will respond with a JSON object containing the message “Hello, World!”.
Similarly, we can make a POST request to our API with a request body:
POST https://api.example.com/hello Content-Type: application/json { "name": "John Doe" }
This will trigger the serverless function, which will respond with a JSON object containing the message “Received: { “name”: “John Doe” }”.
By combining serverless functions with API Gateway, we can easily create powerful and scalable APIs without worrying about managing servers or infrastructure.
In the next chapter, we will explore how to secure serverless APIs and handle authentication and authorization.
Orchestration and Workflow in Serverless Architecture
In serverless architecture, orchestration refers to the process of managing and coordinating the execution of individual functions or services within an application. It plays a crucial role in ensuring that the different components of a serverless application work together seamlessly to deliver the desired functionality.
One popular approach to orchestration in serverless architecture is through the use of workflow management tools. These tools provide a way to define and manage the flow of data and execution between various functions or services. They allow developers to create complex workflows by defining the sequence of steps and the dependencies between them.
One such tool is AWS Step Functions, a fully managed service that lets you coordinate the components of your application as a series of steps in a visual workflow. With Step Functions, you can define the sequence of steps, handle error conditions, and even implement retries and timeouts. It provides a way to build resilient and scalable serverless applications.
Here’s an example of a simple Step Functions workflow definition in JSON format:
{ "Comment": "A simple workflow example", "StartAt": "Step1", "States": { "Step1": { "Type": "Task", "Resource": "arn:aws:lambda:us-east-1:123456789012:function:Function1", "End": true } } }
In this example, the workflow starts at “Step1” and executes a Lambda function called “Function1”. Once the function completes, the workflow ends. This is a basic example, but Step Functions allows you to define more complex workflows with branching, parallel execution, and error handling.
Another popular tool for workflow management in serverless architecture is Apache Airflow. Airflow is an open-source platform that allows you to programmatically author, schedule, and monitor workflows. It provides a rich set of operators and connections to integrate with various services and systems, making it highly flexible and extensible.
Here’s an example of a simple Airflow DAG (Directed Acyclic Graph) definition in Python:
from airflow import DAG from airflow.operators.python import PythonOperator from datetime import datetime def task1(): print("Executing Task 1") dag = DAG( "my_dag", description="A simple DAG example", start_date=datetime(2022, 1, 1), schedule_interval="@daily", ) task_1 = PythonOperator( task_id="task1", python_callable=task1, dag=dag, ) task_1
In this example, we define a DAG called “my_dag” that starts on January 1, 2022, and runs daily. It consists of a single task called “task1” that executes a Python function. Airflow provides a web UI where you can monitor the status and execution of your workflows.
These are just two examples of workflow management tools that can be used in serverless architecture. Depending on your specific requirements and preferences, there are many other options available, such as Azure Logic Apps, Google Cloud Workflows, or IBM Cloud Functions.
In conclusion, orchestration and workflow management are essential aspects of serverless architecture. They allow developers to design and coordinate the execution of complex workflows, ensuring that the different components of a serverless application work together seamlessly. By leveraging the power of workflow management tools, developers can build scalable, resilient, and efficient serverless applications.
Testing and Deployment Strategies for Serverless Applications
Testing and deploying serverless applications can be different from traditional application development due to the unique characteristics of serverless architecture. In this chapter, we will explore some strategies for testing and deploying serverless applications effectively.
Unit Testing
Unit testing is an important practice in software development, and it is equally important for serverless applications. Unit tests help ensure that each function within a serverless application behaves as expected.
When writing unit tests for serverless applications, it is crucial to consider the specific event triggers and input/output formats. For example, if you have a serverless function triggered by an HTTP request, you should create unit tests that simulate different types of requests with varying input data. This can help uncover any potential issues with your function’s logic or dependencies.
Here’s an example of a unit test for a serverless function written in JavaScript using the Jest testing framework:
// math.js exports.add = (a, b) => a + b; // math.test.js const math = require('./math'); test('adds 1 + 2 to equal 3', () => { expect(math.add(1, 2)).toBe(3); });
Integration Testing
Integration testing involves testing the interactions between different components or services within a serverless application. These tests help ensure that the application’s components work together correctly and that integrations with external services function as expected.
When performing integration testing for serverless applications, it is essential to consider any third-party services or APIs that your functions interact with. Mocking or stubbing these services can be helpful to control the test environment and simulate different scenarios.
For example, if your serverless function reads data from a database, you can use a testing library like AWS SDK Mock to simulate the database calls and provide predefined responses. This allows you to test your function’s behavior without relying on a real database.
Deployment Strategies
Deploying serverless applications involves packaging and deploying individual functions or a collection of functions, along with their associated configurations and dependencies.
One common deployment strategy for serverless applications is to use a cloud provider’s infrastructure as code (IaC) tool, such as AWS CloudFormation or Azure Resource Manager. These tools allow you to define your application’s resources, including functions, event triggers, and permissions, as code. This approach helps ensure consistent and repeatable deployments.
Another deployment strategy is to use a serverless framework like Serverless Framework or AWS SAM (Serverless Application Model). These frameworks provide higher-level abstractions and simplify the deployment process by automating the packaging and deployment of serverless applications. They also offer additional features like environment variable management and built-in support for different cloud providers.
Here’s an example of a Serverless Framework configuration file (serverless.yml) that defines a serverless function:
service: my-serverless-app provider: name: aws runtime: nodejs14.x functions: hello: handler: handler.hello events: - http: path: hello method: get
In this example, the configuration defines a single function named “hello” that is triggered by an HTTP GET request to the “/hello” path.
Continuous Integration and Deployment
To ensure the stability and quality of your serverless applications, it is recommended to incorporate continuous integration (CI) and continuous deployment (CD) practices into your development workflow.
CI involves automatically building and testing your serverless application whenever changes are committed to the source code repository. Popular CI tools like Jenkins, Travis CI, or CircleCI can be used to set up and configure these automated build and test processes.
CD goes a step further by automatically deploying your serverless application to a testing or production environment after successful CI. This allows you to rapidly deploy new features or bug fixes to your application while maintaining a high level of confidence in its stability.
Best Practices for Serverless Architecture
Serverless architecture offers many benefits such as scalability, cost-efficiency, and reduced operational complexity. However, to make the most out of serverless, it’s important to follow some best practices. In this chapter, we will explore some key best practices for serverless architecture.
1. Design small, single-purpose functions
When designing your serverless application, it’s crucial to break down your logic into small, single-purpose functions. This approach allows for better scalability and reusability. Each function should have a clear purpose and do one thing well. For example, instead of creating a monolithic function that handles both user authentication and data processing, separate these concerns into different functions. This enables easier maintenance, testing, and troubleshooting.
2. Optimize function execution time
Function execution time is a critical factor in serverless architecture. To ensure optimal performance, it’s important to minimize the execution time of your functions. Avoid unnecessary dependencies and heavy computations. Additionally, leverage caching and memoization techniques to reduce the need for repetitive computations. Remember that faster execution time not only improves user experience but also reduces costs by minimizing the time your functions spend running.
3. Use event-driven architecture
Serverless architecture is well-suited for event-driven applications. Instead of relying on traditional request-response patterns, design your application to respond to events. Events can be triggered by various sources such as HTTP requests, database changes, or messaging systems. This approach allows your application to scale automatically based on the incoming workload. AWS Lambda, for example, supports a wide range of event sources, including Amazon S3, DynamoDB, and SNS.
4. Implement proper error handling
Error handling is important in any application, and serverless architectures are no exception. Implement robust error handling mechanisms to handle exceptions and failures gracefully. Use appropriate error codes and messages to provide meaningful feedback to clients. Additionally, consider implementing retry logic and implementing appropriate circuit breakers to handle transient failures.
5. Leverage managed services
Serverless architectures often rely on various managed services provided by cloud providers. These services abstract away the underlying infrastructure, allowing you to focus on your application logic. Leverage these managed services, such as AWS Lambda, Azure Functions, or Google Cloud Functions, to offload operational tasks like server management, scaling, and monitoring. By relying on managed services, you can reduce operational overhead and improve the overall resilience of your application.
6. Implement security measures
Security should be a top priority in serverless architecture. Implement appropriate security measures to protect your application and data. Use encryption for sensitive data at rest and in transit. Implement access controls and least privilege principles to ensure that only authorized entities can access your functions and resources. Regularly monitor and audit your serverless functions for any vulnerabilities or suspicious activities.
Implementing these best practices will help you build reliable, scalable, and cost-effective serverless architectures. By designing small, single-purpose functions, optimizing execution time, using event-driven architecture, implementing proper error handling, leveraging managed services, and implementing security measures, you can unlock the full potential of serverless architecture in your applications.
Common Challenges and Solutions in Serverless Architecture
Serverless architecture offers many benefits, such as reduced operational costs, improved scalability, and faster time-to-market. However, like any technology, it also comes with its own set of challenges. In this chapter, we will explore some of the common challenges faced when building serverless applications and discuss potential solutions to overcome them.
1. Cold Start Latency
One of the primary challenges in serverless architecture is the cold start latency, which refers to the delay experienced when a function is invoked for the first time or after a period of inactivity. This delay occurs because the cloud provider needs to allocate resources to run the function, resulting in increased response times.
To mitigate this problem, one solution is to implement resource pooling. By keeping a pool of pre-initialized instances of functions, you can reduce the impact of cold starts. Another approach is to use provisioned concurrency, a feature offered by some cloud providers, which allows you to keep a certain number of instances warm at all times.
Here’s an example of how to implement resource pooling in a Node.js application using AWS Lambda:
const pool = []; exports.handler = async (event) => { let instance; if (pool.length > 0) { instance = pool.pop(); } else { instance = initializeFunction(); // Create a new instance } const result = await instance(event); pool.push(instance); // Reuse the instance for future invocations return result; };
2. Limited Execution Time
Serverless functions typically have a maximum execution time limit imposed by the cloud provider. This limit is usually in the range of a few minutes. If your function exceeds this time limit, it will be terminated, leading to potential data loss or incomplete operations.
To address this challenge, you can break down long-running tasks into smaller, more manageable chunks. By using event-driven architectures and task queues, you can distribute the workload across multiple function invocations and ensure that each task completes within the time limits.
Here’s an example of how to use AWS Step Functions to orchestrate a long-running task:
{ "StartAt": "ProcessTask", "States": { "ProcessTask": { "Type": "Task", "Resource": "arn:aws:lambda:us-east-1:123456789012:function:processTask", "End": true } } }
3. Vendor Lock-in
Another challenge in serverless architecture is the risk of vendor lock-in. Each cloud provider has its own set of proprietary services and APIs, making it difficult to switch between providers without significant effort.
To mitigate this risk, you can adopt a cloud-agnostic approach by using open-source frameworks and abstractions. For example, frameworks like Serverless Framework and AWS SAM (Serverless Application Model) provide a unified way to define and deploy serverless applications across multiple cloud providers.
Here’s an example of a serverless.yml file defining a serverless application using the Serverless Framework:
service: my-serverless-app provider: name: aws runtime: nodejs14.x region: us-east-1 functions: hello: handler: handler.hello events: - http: path: hello method: get
By using such frameworks, you can write code once and deploy it to multiple cloud providers, reducing the risk of vendor lock-in.
In this chapter, we discussed some of the common challenges faced when building serverless applications and explored potential solutions to overcome them. By understanding and addressing these challenges, you can harness the full potential of serverless architecture and build robust and scalable applications.