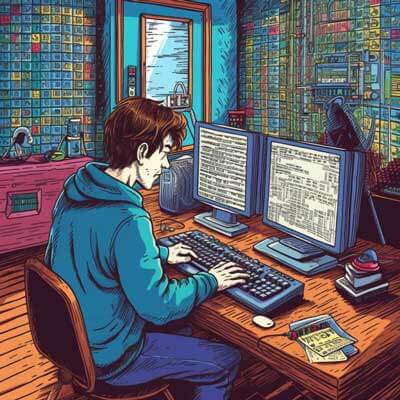
- Getting Started with Terraform
- Installing Terraform
- Initializing a Terraform Project
- Defining Infrastructure Resources
- Provisioning Infrastructure
- Destroying Infrastructure
- Creating and Managing Infrastructure
- Initializing a Terraform Project
- Defining Infrastructure with Terraform Configuration Language (HCL)
- Provisioning Infrastructure
- Managing Infrastructure State
- Destroying Infrastructure
- Using Variables and Data Sources
- Variables
- Data Sources
- Understanding Terraform State
- What is Terraform State?
- State Locking
- Viewing and Managing Terraform State
- Importing Existing Infrastructure into Terraform
- Managing Secrets and Sensitive Data
- Input Variables
- Terraform Cloud and Enterprise
- Third-Party Plugins
- Working with Modules
- Using Provisioners for Customization
- Creating a Provisioner
- Running Provisioners
- Using Connection Parameters
- Using Provisioners with Local Executables
- Using Provisioners with Remote Executables
- Deploying Applications with Terraform
- 1. Deploying a Simple Web Application
- 2. Deploying a Containerized Application on Kubernetes
- 3. Deploying a Serverless Application on AWS Lambda
- Implementing Infrastructure as Code Best Practices
- Handling Remote State and Collaboration
- Scaling and Managing Terraform Projects
- 1. Modularize your code
- 2. Use Terraform workspaces
- 3. Use version control for your Terraform code
- 4. Leverage Terraform Cloud or Terraform Enterprise
- 5. Use Terraform modules from the Terraform Registry
- Using Terraform with Cloud Providers
- 1. Using Terraform with AWS
- 2. Using Terraform with Azure
- 3. Using Terraform with Google Cloud Platform
- Advanced Techniques and Tips
- 1. Variable Interpolation
- 2. Terraform Modules
- 3. Remote State Management
- 4. Conditional Resource Creation
- 5. Terraform Functions
- Troubleshooting and Debugging
- 1. Verify Provider Configuration
- 2. Enable Debugging
- 3. Use Terraform Commands
- 4. Use Terraform Debugging Tools
- 5. Check Provider Documentation and Community Forums
Getting Started with Terraform
Terraform is an open-source infrastructure as code tool that allows you to create, manage, and update your infrastructure resources in a declarative manner. It provides a simple and efficient way to define and provision infrastructure across various cloud providers.
In this chapter, we will guide you through the process of getting started with Terraform. By the end of this chapter, you will have a basic understanding of how to set up Terraform, define your infrastructure as code, and provision resources.
Related Article: Terraform Advanced Tips on Google Cloud
Installing Terraform
To get started with Terraform, you first need to install it on your machine. Follow these steps to install Terraform:
1. Visit the official Terraform website at https://www.terraform.io/.
2. Download the appropriate version of Terraform for your operating system (Windows, macOS, or Linux).
3. Extract the downloaded archive to a directory of your choice.
4. Add the Terraform executable to your system’s PATH variable.
To verify that Terraform is installed correctly, open a terminal and run the following command:
terraform version
If everything is set up correctly, you should see the version of Terraform installed on your machine.
Initializing a Terraform Project
Once Terraform is installed, you can initialize a new Terraform project. The initialization step downloads the required provider plugins and sets up the working directory. Follow these steps to initialize a Terraform project:
1. Create a new directory for your Terraform project.
2. Open a terminal and navigate to the project directory.
3. Run the following command:
terraform init
This command initializes the working directory and downloads the necessary provider plugins. It creates a hidden directory named .terraform
that contains the downloaded plugins and other files required by Terraform.
Defining Infrastructure Resources
After initializing the Terraform project, you can start defining your infrastructure resources. Terraform uses a declarative language called HashiCorp Configuration Language (HCL) to define the desired state of your infrastructure.
Create a new file named main.tf
in your project directory and define your infrastructure resources using HCL. Here’s an example that creates an AWS EC2 instance:
provider "aws" { region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" tags = { Name = "example-instance" } }
In this example, we specified the AWS provider and created an EC2 instance resource. We also defined some attributes such as the AMI ID, instance type, and tags for the instance.
Related Article: Terraform Advanced Tips for AWS
Provisioning Infrastructure
Once you have defined your infrastructure resources, you can provision them using Terraform. Provisioning in Terraform refers to creating and configuring the actual resources defined in your Terraform configuration.
To provision your infrastructure, run the following command in your project directory:
terraform apply
Terraform will analyze your configuration, create an execution plan, and prompt you to confirm the changes before applying them. Review the plan and enter yes
to proceed with the provisioning.
Destroying Infrastructure
If you want to tear down the resources provisioned by Terraform, you can use the destroy command. This command will destroy all the resources defined in your Terraform configuration.
To destroy your infrastructure, run the following command in your project directory:
terraform destroy
Terraform will prompt you to confirm the destruction of your resources. Review the plan and enter yes
to proceed with the destruction.
Now that you have a solid foundation, you can explore more advanced features and concepts of Terraform to manage your infrastructure efficiently.
Creating and Managing Infrastructure
In this chapter, we will cover the basics of creating and managing infrastructure using Terraform. Terraform is an open-source infrastructure as code software tool that allows you to define and provision infrastructure resources in a declarative way.
Related Article: Terraform Tutorial & Advanced Tips
Initializing a Terraform Project
Before you can start using Terraform, you need to initialize a new project. To do this, navigate to the project directory in your terminal and run the following command:
terraform init
This command will download the necessary provider plugins and set up the backend for storing the Terraform state.
Defining Infrastructure with Terraform Configuration Language (HCL)
To define the infrastructure resources you want to create, you use Terraform Configuration Language (HCL). HCL is a domain-specific language (DSL) designed specifically for writing infrastructure code. Let’s take a look at a simple example of defining an AWS EC2 instance:
provider "aws" { region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this example, we specify the AWS provider and the region we want to use. Then, we define an AWS EC2 instance resource with the desired AMI and instance type.
Provisioning Infrastructure
Once you have defined your infrastructure resources, you can provision them by running the following command:
terraform apply
Terraform will analyze the configuration and create or update the necessary resources to match the desired state.
Managing Infrastructure State
Terraform uses a state file to keep track of the resources it manages. The state file contains information about the resources’ current state and helps Terraform determine what changes need to be made. By default, Terraform stores the state locally in a file named “terraform.tfstate”.
However, it is recommended to use a remote backend for storing the state file in a shared location. This allows for collaboration and prevents the state file from being lost or accidentally deleted. Popular remote backends include Amazon S3, Azure Blob Storage, and HashiCorp Terraform Cloud.
Destroying Infrastructure
When you no longer need the infrastructure resources, you can destroy them by running the following command:
terraform destroy
This will remove all the resources defined in your configuration and update the state file accordingly.
Using Variables and Data Sources
When working with Terraform, it is common to have certain values that need to be reused across multiple resources or configurations. To make your code more modular and maintainable, you can use variables and data sources.
Variables
Variables allow you to define dynamic values that can be used throughout your Terraform code. They can be defined in a separate file, typically with a .tfvars
extension, or directly in your main Terraform configuration file.
To define a variable in a .tfvars
file, you can use the following syntax:
variable_name = "value"
For example, if you have a variable called region
with the value “us-west-2”, your .tfvars
file would look like this:
region = "us-west-2"
To use the variable in your Terraform code, you can reference it using the var
keyword. For example:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" region = var.region }
In this example, the region
variable is used as the value for the region
attribute of the aws_instance
resource.
You can pass the variable values to Terraform using the command-line -var
flag or by creating a terraform.tfvars
file. For example:
terraform apply -var="region=us-west-2"
Data Sources
Data sources allow you to fetch information from external sources, such as AWS, to use within your Terraform configurations. They provide a way to reference existing resources or retrieve data that is not managed by Terraform.
To use a data source, you need to define it in your Terraform configuration using the data
block. For example, to fetch information about an AWS availability zone, you can use the following code:
data "aws_availability_zones" "example" { state = "available" }
In this example, we’re using the aws_availability_zones
data source to fetch information about the availability zones in the current region.
You can reference the data source in your Terraform code by using the data
keyword. For example:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" availability_zone = data.aws_availability_zones.example.names[0] }
In this example, the first availability zone returned by the aws_availability_zones
data source is used as the value for the availability_zone
attribute of the aws_instance
resource.
Data sources are typically used to fetch information that is needed for resource configurations, such as retrieving information about existing VPCs or security groups.
Using variables and data sources in your Terraform code can greatly improve its readability and maintainability. Variables allow you to reuse values across different resources, while data sources provide a way to fetch information from external sources.
Understanding Terraform State
Terraform is a powerful infrastructure as code tool that allows you to define and provision infrastructure resources using declarative configuration files. One of the key concepts in Terraform is its state, which is a record of the resources that Terraform manages.
What is Terraform State?
Terraform state is a file that keeps track of the resources Terraform manages and their current state. It is used by Terraform to map real-world resources to your configuration. The state file is usually named terraform.tfstate
and is stored locally by default. However, it can also be stored remotely, such as in a Terraform Cloud workspace or an S3 bucket.
The state file is crucial for Terraform’s operations. It allows Terraform to understand the changes that need to be made to your infrastructure and to make those changes in a predictable and reliable manner. It also helps Terraform to track and manage dependencies between resources.
State Locking
When using Terraform in a collaborative environment, state locking becomes important to prevent concurrent updates that could lead to conflicts. State locking ensures that only one user or process can modify the state at a time. By default, state locking is disabled, but it is highly recommended to enable it when working with Terraform in a team.
Terraform provides various backends for state storage, such as Terraform Cloud, Amazon S3, or HashiCorp Consul. These backends also handle state locking to ensure safe and consistent operations.
Viewing and Managing Terraform State
Terraform provides several commands to interact with the state file. Here are some commonly used commands:
– terraform state list
: This command lists all the resources managed by Terraform.
– terraform state show
: This command displays the current state of a specific resource.
– terraform state mv
: This command renames a resource in the state file.
– terraform state rm
: This command removes a resource from the state file.
It’s important to note that manually modifying the state file can lead to inconsistencies and should be avoided. Always use the Terraform commands to manage and modify the state.
Importing Existing Infrastructure into Terraform
If you have existing infrastructure that was not provisioned using Terraform, you can import it into your Terraform state. This allows Terraform to manage and track the existing resources.
To import a resource, you need to provide its resource type and identifier. For example, to import an AWS EC2 instance, you would use the following command:
$ terraform import aws_instance.my_instance i-1234567890abcdef0
This command tells Terraform to import the EC2 instance with the identifier i-1234567890abcdef0
into the resource named aws_instance.my_instance
in the state file.
Managing Secrets and Sensitive Data
In any modern infrastructure, managing secrets and sensitive data is a critical task. Terraform provides several ways to handle secrets securely, ensuring that sensitive information is not exposed in plain text within your codebase.
Input Variables
One common approach to managing secrets in Terraform is through the use of input variables. Input variables allow you to pass in sensitive information, such as API keys or database passwords, as variables when running the Terraform command.
To define an input variable, create a file with a .tfvars
extension, for example secrets.tfvars
, and specify the sensitive values as key-value pairs:
# secrets.tfvars database_password = "supersecret" api_key = "abcd1234"
To use these input variables in your Terraform code, reference them using the var
syntax:
# main.tf resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" key_name = var.api_key user_data = var.database_password }
When running terraform apply
, pass the variable file with the -var-file
flag:
terraform apply -var-file=secrets.tfvars
This ensures that sensitive data is stored separately from your codebase and can be managed securely.
Terraform Cloud and Enterprise
For larger teams or organizations, Terraform Cloud and Terraform Enterprise provide additional features for managing secrets and sensitive data. These tools allow you to store and manage variables securely within the platform, ensuring that sensitive information is not exposed to unauthorized individuals.
Terraform Cloud and Enterprise integrate with various external systems, such as HashiCorp Vault or AWS Secrets Manager, to securely retrieve and manage secrets during the Terraform execution process.
Third-Party Plugins
If you require more advanced or specific secret management capabilities, you can leverage third-party plugins that integrate with Terraform. These plugins provide additional functionalities, such as encryption, rotation, and dynamic secret retrieval, to enhance the security of your infrastructure.
Plugins like terraform-provider-vault
or terraform-provider-aws-secretsmanager
enable you to interact with external secret management systems directly from your Terraform code.
Working with Modules
When working with Terraform, modules are an essential component that allows you to organize and reuse your code. Modules encapsulate a set of resources and their corresponding configuration, making it easier to manage and share infrastructure code.
To define a module, you create a new directory with a specific structure. Let’s say you want to create a module for provisioning an Amazon EC2 instance. You can start by creating a directory called ec2-instance-module
and the following files inside it:
1. main.tf
: This file contains the main configuration for your module. It defines the resources and their properties. For our EC2 instance module, the main.tf
file might look like this:
resource "aws_instance" "example" { ami = var.ami_id instance_type = var.instance_type subnet_id = var.subnet_id }
2. variables.tf
: This file defines the input variables for your module. These variables allow users of the module to customize its behavior. In our EC2 instance module, we might define variables for the AMI ID, instance type, and subnet ID:
variable "ami_id" { description = "The ID of the AMI to use for the EC2 instance" } variable "instance_type" { description = "The type of the EC2 instance" } variable "subnet_id" { description = "The ID of the subnet to deploy the EC2 instance in" }
3. outputs.tf
: This file defines the outputs of your module. Outputs allow you to expose certain values to be used by other parts of your infrastructure. In our EC2 instance module, we might define an output for the instance’s public IP address:
output "public_ip" { description = "The public IP address of the EC2 instance" value = aws_instance.example.public_ip }
Once you have defined your module, you can use it in your main Terraform configuration by calling it as a module. For example, assuming our EC2 instance module is located in the same directory as our main configuration file, we can use it like this:
module "ec2_instance" { source = "./ec2-instance-module" ami_id = "ami-12345678" instance_type = "t2.micro" subnet_id = "subnet-12345678" }
In the above example, we are using the ec2_instance
module and passing the required variables to it.
Using modules in Terraform allows you to create reusable and modular code, making it easier to build and manage infrastructure. You can also share your modules with others by publishing them to the Terraform Registry or by sharing them directly as Git repositories.
To learn more about modules and how to use them effectively, check out the official Terraform documentation on Working with Modules.
Using Provisioners for Customization
Provisioners in Terraform allow you to run scripts or commands on a resource after it has been created. This is useful for customization tasks such as installing software, configuring settings, or running initialization scripts. In this chapter, we will explore how to use provisioners to customize your infrastructure.
Creating a Provisioner
To create a provisioner, you need to define it within a resource block. Let’s take an example of provisioning an EC2 instance and running a script on it after it is created.
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "local-exec" { command = "echo 'Hello, Terraform!'" } }
In this example, we are using the local-exec
provisioner, which allows us to run a command on the local machine where Terraform is being executed. The command in this case is simply echoing a message.
Running Provisioners
When you apply your Terraform configuration, the provisioners defined within the resource blocks will be executed. You can also specify which provisioners to run using the only
and except
arguments.
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "local-exec" { command = "echo 'Hello, Terraform!'" } provisioner "remote-exec" { inline = [ "sudo apt-get update", "sudo apt-get install -y nginx", ] } }
In this example, we have two provisioners – local-exec
and remote-exec
. The remote-exec
provisioner is used to run commands on the created EC2 instance. In this case, we are updating the package cache and installing the Nginx web server.
Using Connection Parameters
Provisioners that run commands on remote resources, like the remote-exec
provisioner, require connection parameters to establish a connection to the resource. These parameters include the username, password, private key, and host address.
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "remote-exec" { inline = [ "sudo apt-get update", "sudo apt-get install -y nginx", ] connection { host = self.public_ip type = "ssh" user = "ubuntu" private_key = file("~/.ssh/id_rsa") } } }
In this example, we are using the connection
block to provide the necessary parameters for establishing an SSH connection to the EC2 instance. The host
parameter is set to the public IP address of the instance, the user
parameter is set to “ubuntu”, and the private_key
parameter is set to the path of the private key file.
Using Provisioners with Local Executables
In addition to running commands directly, provisioners can also execute local executables on the machine where Terraform is being executed. This can be useful for running custom scripts or installing software.
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "local-exec" { command = "scripts/setup.sh" } }
In this example, the local-exec
provisioner is executing a local script called “setup.sh”. This script could contain any custom logic or commands you want to run on the EC2 instance after it has been created.
Using Provisioners with Remote Executables
Provisioners can also run executables on the remote resource using the remote-exec
provisioner. This is useful for running scripts or commands that are already present on the remote resource.
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "remote-exec" { script_path = "scripts/setup.sh" } }
In this example, the remote-exec
provisioner is executing a script called “setup.sh” that is present on the EC2 instance. This script could be placed on the instance during the creation process, or it could be part of a custom AMI.
Deploying Applications with Terraform
Terraform is a powerful infrastructure as code tool that can be used to deploy and manage applications in a variety of cloud environments. In this chapter, we will explore some quick and easy Terraform code snippets for deploying applications.
1. Deploying a Simple Web Application
To get started, let’s deploy a simple web application using Terraform. We will use an AWS EC2 instance to host our application.
First, create a new file with a .tf extension, such as main.tf
, and add the following code:
provider "aws" { region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" tags = { Name = "example-instance" } }
In this code snippet, we define the AWS provider and specify the region. We then declare an AWS EC2 instance resource, specifying the AMI ID and instance type. Finally, we add tags to the instance for easier identification.
To deploy the web application, navigate to the directory containing the main.tf
file and run the following commands:
terraform init terraform apply
Terraform will initialize the project and apply the configuration, creating the EC2 instance.
2. Deploying a Containerized Application on Kubernetes
Terraform can also be used to deploy containerized applications on Kubernetes clusters. Let’s see how to do that.
Create a new file with a .tf extension, such as kubernetes.tf
, and add the following code:
provider "kubernetes" { config_path = "~/.kube/config" } resource "kubernetes_deployment" "example" { metadata { name = "example-deployment" } spec { replicas = 3 selector { match_labels = { app = "example" } } template { metadata { labels = { app = "example" } } spec { container { image = "nginx:latest" port { container_port = 80 } } } } } }
In this code snippet, we declare the Kubernetes provider and specify the path to the Kubernetes configuration file. We then define a Kubernetes deployment resource, specifying the number of replicas, labels, and container details.
To deploy the containerized application, navigate to the directory containing the kubernetes.tf
file and run the following commands:
terraform init terraform apply
Terraform will initialize the project and apply the configuration, creating the Kubernetes deployment.
3. Deploying a Serverless Application on AWS Lambda
Terraform also supports deploying serverless applications on AWS Lambda. Let’s take a look at an example.
Create a new file with a .tf extension, such as lambda.tf
, and add the following code:
provider "aws" { region = "us-west-2" } resource "aws_lambda_function" "example" { function_name = "example-lambda" runtime = "python3.8" handler = "lambda_handler" filename = "lambda_function.zip" role = aws_iam_role.lambda_role.arn } resource "aws_iam_role" "lambda_role" { name = "example-lambda-role" assume_role_policy = <<EOF { "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Principal": { "Service": "lambda.amazonaws.com" }, "Action": "sts:AssumeRole" } ] } EOF }
In this code snippet, we define the AWS provider and specify the region. We then declare an AWS Lambda function resource, specifying the function name, runtime, handler, filename, and IAM role.
To deploy the serverless application, navigate to the directory containing the lambda.tf
file and run the following commands:
terraform init terraform apply
Terraform will initialize the project and apply the configuration, creating the Lambda function.
These are just a few examples of how Terraform can be used to deploy applications. With Terraform's flexibility and extensive provider ecosystem, the possibilities are endless. Remember to always review and validate your code before applying it to your infrastructure.
Happy deploying with Terraform!
Implementing Infrastructure as Code Best Practices
Implementing infrastructure as code (IaC) best practices is crucial to ensure the reliability, scalability, and maintainability of your infrastructure. Here are some key practices to follow when writing Terraform code:
1. Use Version Control: Store your Terraform code in a version control system like Git to track changes, collaborate with team members, and roll back to previous versions if needed.
2. Separate Environment Configurations: Maintain separate directories for different environments, such as development, staging, and production. This allows you to manage infrastructure configurations specific to each environment easily.
3. Modularize Your Code: Break your infrastructure code into reusable modules to promote code reusability, simplify maintenance, and improve readability. Modules can encapsulate a set of resources and configurations that are used across multiple projects.
Here is an example of a simple Terraform module for creating an AWS EC2 instance:
// main.tf resource "aws_instance" "example_instance" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" } // variables.tf variable "ami" { description = "AMI ID for the EC2 instance" type = string } variable "instance_type" { description = "Instance type for the EC2 instance" type = string }
4. Use Variables: Leverage variables to make your Terraform code more flexible and reusable. Variables allow you to parameterize your infrastructure code and provide values dynamically during deployment.
5. Manage Secrets Securely: Avoid hardcoding sensitive information like access keys, passwords, or API tokens directly in your Terraform code. Instead, use a secrets management solution like AWS Secrets Manager or HashiCorp Vault to securely store and retrieve secrets.
6. Apply Continuous Integration and Delivery (CI/CD) Practices: Integrate your infrastructure code into a CI/CD pipeline to automate testing, validation, and deployment. This ensures that your infrastructure changes are thoroughly tested and deployed consistently.
7. Use Terraform State Management: Terraform state tracks the current state of your infrastructure. Store the state file in a remote backend like Amazon S3 or HashiCorp Terraform Cloud to enable collaboration, versioning, and recovery in case of failures.
8. Implement Infrastructure Testing: Write automated tests to validate the correctness of your infrastructure code. Tools like Terratest or InSpec can be used to write and execute tests against your infrastructure.
9. Document Your Infrastructure: Maintain documentation that describes the purpose, design, and usage of your infrastructure. This helps onboard new team members, troubleshoot issues, and ensure consistent understanding among team members.
By following these best practices, you can enhance the quality, stability, and maintainability of your Terraform code and infrastructure. Remember, infrastructure as code is an iterative process, and continuous improvement is key to managing your infrastructure efficiently.
For more information on Terraform best practices, refer to the official Terraform Style Guide and Recommended Practices documentation.
Handling Remote State and Collaboration
Terraform allows you to store your state remotely, which is essential for collaborating with other team members or managing infrastructure across multiple environments. Storing state remotely ensures that everyone is working with the same version of the infrastructure and prevents conflicts.
There are several options for remote state storage, including Terraform Cloud, Amazon S3, and Azure Blob Storage. In this chapter, we will explore how to configure remote state storage using Terraform Cloud and how to collaborate with other team members.
To configure remote state storage using Terraform Cloud, you need to create a new workspace in Terraform Cloud and link it to your Terraform configuration. Here’s an example of how to configure remote state storage using Terraform Cloud:
terraform { backend "remote" { organization = "" workspaces { name = "" } } }
Replace with your Terraform Cloud organization name and
with the name of the workspace you want to use. Once you have configured remote state storage, you can run
terraform init
to initialize the backend and sync your local state with the remote state.
When collaborating with other team members, Terraform Cloud provides a central location for managing infrastructure changes and sharing state. Multiple team members can work on the same infrastructure simultaneously without conflicts. Terraform Cloud also provides features like access controls, versioning, and notifications.
To collaborate with other team members using Terraform Cloud, you can invite them to the workspace and grant them appropriate access permissions. They can then clone the workspace to their local machine and make changes to the infrastructure code. Here’s an example of how to clone a workspace:
terraform login terraform workspace new terraform init
Replace with the name of the workspace you want to clone. The
terraform login
command authenticates with Terraform Cloud, and the terraform workspace new
command creates a new workspace on your local machine.
After making changes to the infrastructure code, team members can use terraform plan
and terraform apply
commands to preview and apply changes to the infrastructure. Terraform Cloud will automatically update the remote state and notify other team members of the changes.
In this chapter, we explored how to configure remote state storage using Terraform Cloud and how to collaborate with other team members. By leveraging remote state storage and collaboration features, you can ensure consistent infrastructure and streamline your team’s workflow.
Scaling and Managing Terraform Projects
Terraform is a powerful tool for infrastructure provisioning and management, but as your projects grow in size and complexity, it’s important to have strategies in place for scaling and managing your Terraform code. In this chapter, we will explore some best practices and techniques to help you effectively scale and manage your Terraform projects.
1. Modularize your code
As your infrastructure grows, it becomes essential to organize your Terraform code in a modular and reusable manner. Modularization helps in maintaining a separation of concerns, enhances code readability, and promotes code reuse across different projects.
One way to achieve code modularity is by using modules in Terraform. A module is a self-contained collection of Terraform resources that can be used as a building block for your infrastructure. Modules encapsulate a specific set of functionality and can be easily reused across different projects.
Here’s an example of a simple module that provisions an AWS EC2 instance:
// ec2_instance.tf variable "instance_type" { description = "EC2 instance type" type = string } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = var.instance_type }
By modularizing your code, you can define reusable modules for common infrastructure components like EC2 instances, VPCs, or databases. This makes it easier to manage and scale your Terraform projects.
2. Use Terraform workspaces
Terraform workspaces allow you to manage multiple instances of a single Terraform configuration. Workspaces are useful when you need to manage separate environments such as development, staging, and production, each with its own set of infrastructure resources.
Creating a new workspace is as simple as running the following command:
terraform workspace new
Switching between workspaces can be done using the terraform workspace select
command:
terraform workspace select
Each workspace maintains its own state file, allowing you to manage the infrastructure for different environments independently. This helps in isolating changes and reducing the risk of accidentally modifying resources in the wrong environment.
3. Use version control for your Terraform code
Version control is essential for managing any codebase, and Terraform code is no exception. Using a version control system like Git allows you to track changes, collaborate with others, and roll back to previous versions if needed.
Make sure to commit your Terraform code to a version control repository regularly. This ensures that you have a history of changes and makes it easier to review and collaborate with teammates.
Consider using a branching strategy such as GitFlow to manage different stages of development, and tag releases to keep track of versions deployed to different environments.
4. Leverage Terraform Cloud or Terraform Enterprise
Terraform Cloud and Terraform Enterprise are powerful tools that provide additional features and capabilities for managing Terraform projects at scale.
These tools provide a centralized location for storing and managing your Terraform state files, offer collaborative workflows, and enable remote execution of Terraform plans and applies.
By using Terraform Cloud or Terraform Enterprise, you can easily manage access control, enforce policies, and gain visibility into changes made to your infrastructure.
5. Use Terraform modules from the Terraform Registry
The Terraform Registry is a public repository of reusable Terraform modules. It provides a wide range of modules contributed by the community and verified by HashiCorp.
Using modules from the Terraform Registry can save you time and effort in building common infrastructure components. These modules are battle-tested and often follow best practices, ensuring a higher level of reliability in your infrastructure.
To use a module from the Terraform Registry, you can simply reference it in your Terraform code using the module block:
module "vpc" { source = "terraform-aws-modules/vpc/aws" version = "3.0.0" // Module configuration parameters // ... }
Make sure to review the documentation and version compatibility of the module before using it in your project.
Scaling and managing Terraform projects effectively requires careful planning, organization, and the adoption of best practices. By modularizing your code, leveraging Terraform workspaces, using version control, leveraging Terraform Cloud or Terraform Enterprise, and utilizing modules from the Terraform Registry, you can successfully scale and manage your Terraform projects with ease.
Using Terraform with Cloud Providers
Terraform is a powerful infrastructure as code (IaC) tool that allows you to provision and manage your cloud resources using a declarative language. It supports various cloud providers, making it flexible and versatile for different environments. In this chapter, we will explore how to use Terraform with popular cloud providers such as AWS, Azure, and Google Cloud Platform.
1. Using Terraform with AWS
Amazon Web Services (AWS) is one of the most popular cloud providers, and Terraform has excellent support for provisioning AWS resources. With Terraform, you can easily create and manage EC2 instances, S3 buckets, VPCs, and other AWS resources.
To get started, you need to configure your AWS credentials. You can set them as environment variables or use the AWS CLI configuration file. Once you have your credentials set up, you can write your Terraform code to provision AWS resources.
Here is an example of a Terraform configuration file (main.tf
) that provisions an EC2 instance in AWS:
provider "aws" { region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this example, we specify the AWS provider and set the region to “us-west-2”. We then define an EC2 instance resource using the aws_instance
block. We specify the AMI ID and the instance type.
Once you have written your Terraform code, you can initialize the project by running terraform init
. This command downloads the necessary provider plugins and sets up the project.
To provision the resources, run terraform apply
. Terraform will analyze the code and create or update the resources accordingly. You will be prompted to confirm the changes before applying them.
2. Using Terraform with Azure
Microsoft Azure is another popular cloud provider that can be easily integrated with Terraform. Terraform supports provisioning various Azure resources, including virtual machines, storage accounts, and virtual networks.
To use Terraform with Azure, you need to first configure your Azure credentials. You can set them as environment variables or use the Azure CLI configuration file. Once your credentials are set up, you can start writing your Terraform code.
Here is an example of a Terraform configuration file (main.tf
) that provisions an Azure virtual machine:
provider "azurerm" { features {} } resource "azurerm_resource_group" "example" { name = "tf-example-rg" location = "West US 2" } resource "azurerm_virtual_network" "example" { name = "tf-example-vnet" address_space = ["10.0.0.0/16"] location = azurerm_resource_group.example.location resource_group_name = azurerm_resource_group.example.name } resource "azurerm_subnet" "example" { name = "tf-example-subnet" resource_group_name = azurerm_resource_group.example.name virtual_network_name = azurerm_virtual_network.example.name address_prefixes = ["10.0.1.0/24"] } resource "azurerm_network_interface" "example" { name = "tf-example-nic" location = azurerm_resource_group.example.location resource_group_name = azurerm_resource_group.example.name ip_configuration { name = "testconfiguration1" subnet_id = azurerm_subnet.example.id private_ip_address_allocation = "Dynamic" } } resource "azurerm_virtual_machine" "example" { name = "tf-example-vm" location = azurerm_resource_group.example.location resource_group_name = azurerm_resource_group.example.name network_interface_ids = [azurerm_network_interface.example.id] vm_size = "Standard_DS1_v2" storage_image_reference { publisher = "Canonical" offer = "UbuntuServer" sku = "18.04-LTS" version = "latest" } storage_os_disk { name = "myosdisk1" caching = "ReadWrite" create_option = "FromImage" managed_disk_type = "Premium_LRS" } os_profile { computer_name = "hostname" admin_username = "adminuser" admin_password = "Password1234!" } }
In this example, we specify the Azure provider and define various Azure resources such as a resource group, virtual network, subnet, network interface, and virtual machine.
After writing your Terraform code, initialize the project with terraform init
. Then, provision the resources using terraform apply
. Terraform will create or update the resources based on your code.
3. Using Terraform with Google Cloud Platform
Google Cloud Platform (GCP) is another cloud provider that can be easily managed with Terraform. Terraform supports provisioning GCP resources such as compute instances, storage buckets, and networking components.
To use Terraform with GCP, you need to configure your GCP credentials. You can set them as environment variables or use the gcloud CLI configuration. Once your credentials are set up, you can start writing your Terraform code.
Here is an example of a Terraform configuration file (main.tf
) that provisions a GCP compute instance:
provider "google" { region = "us-central1" } resource "google_compute_instance" "example" { name = "tf-example-instance" machine_type = "n1-standard-1" zone = "us-central1-a" boot_disk { initialize_params { image = "ubuntu-os-cloud/ubuntu-1804-lts" } } network_interface { network = "default" } }
In this example, we specify the Google Cloud provider and set the region to “us-central1”. We define a compute instance resource using the google_compute_instance
block. We specify the instance name, machine type, and zone.
Once you have written your Terraform code, initialize the project with terraform init
. Then, provision the resources using terraform apply
. Terraform will create or update the resources based on your code.
Terraform’s support for cloud providers makes it a valuable tool for managing infrastructure across different cloud environments. You can easily provision and manage resources in AWS, Azure, and Google Cloud Platform using Terraform’s declarative syntax and powerful features.
Advanced Techniques and Tips
In this chapter, we will explore some advanced techniques and tips to enhance your Terraform code. These techniques will help you write more efficient and reusable infrastructure-as-code.
1. Variable Interpolation
Terraform supports variable interpolation, which allows you to use the value of one variable inside another. This helps in reducing code duplication and simplifying complex configurations. Here’s an example:
variable "region" { type = string default = "us-west-2" } variable "subnet_count" { type = number default = 3 } resource "aws_subnet" "example" { count = var.subnet_count availability_zone = "${var.region}${count.index}" cidr_block = "10.0.${count.index}.0/24" }
In the above example, we use the value of the region
variable inside the availability_zone
to create subnets in different availability zones.
2. Terraform Modules
Terraform modules are reusable and shareable configurations that can be used across different projects. They encapsulate infrastructure components and provide a way to manage and version them independently. Here’s an example of using a module:
module "vpc" { source = "github.com/example/vpc" vpc_cidr_block = "10.0.0.0/16" }
In the above example, we use a module named vpc
which is hosted on GitHub. The module creates a VPC with the specified CIDR block.
3. Remote State Management
Terraform supports remote state management, which allows you to store your state file in a remote backend. This enables collaboration and ensures consistent state across multiple team members. Here’s an example configuration using AWS S3 as the remote backend:
terraform { backend "s3" { bucket = "my-terraform-state" key = "terraform.tfstate" region = "us-west-2" } }
In the above example, we configure Terraform to store the state file in an S3 bucket named my-terraform-state
.
4. Conditional Resource Creation
Terraform provides the ability to conditionally create resources based on certain conditions. This allows you to dynamically create or destroy resources based on the values of variables or outputs. Here’s an example:
resource "aws_instance" "example" { count = var.create_instance ? 1 : 0 instance_type = "t2.micro" ami = "ami-0c94855ba95c71c99" }
In the above example, the aws_instance
resource will only be created if the create_instance
variable is set to true.
5. Terraform Functions
Terraform provides a rich set of built-in functions that can be used to manipulate and transform values. These functions help in writing more expressive and concise code. Here’s an example:
locals { current_year = formatdate("%Y", timestamp()) } output "current_year" { value = local.current_year }
In the above example, we use the formatdate
function to get the current year and store it in the current_year
local variable.
These advanced techniques and tips will empower you to write more efficient and maintainable Terraform code. Experiment with them to enhance your infrastructure-as-code workflows. Now that you have learned these techniques, you are ready to take your Terraform skills to the next level.
Troubleshooting and Debugging
When working with Terraform, it is not uncommon to encounter errors or unexpected behavior. In this chapter, we will explore some common troubleshooting techniques and debugging tools that can help you identify and resolve issues in your Terraform code.
1. Verify Provider Configuration
If you encounter errors related to a specific provider, the first step is to ensure that the provider is correctly configured. Check the provider configuration block in your Terraform code and verify that you have specified the required provider version and any necessary authentication credentials.
For example, when working with the AWS provider, your configuration might look like this:
provider "aws" { access_key = "AWS_ACCESS_KEY_ID" secret_key = "AWS_SECRET_ACCESS_KEY" region = "us-west-2" }
Make sure the access key and secret key are valid, and the region is set to the desired AWS region.
2. Enable Debugging
Enabling debugging can provide valuable insights into what is happening behind the scenes during Terraform’s execution. To enable debugging, set the TF_LOG
environment variable to DEBUG
before running your Terraform commands.
export TF_LOG=DEBUG
With debugging enabled, Terraform will display detailed logs that can help you track down issues. Be sure to check the logs for any error messages or warnings that might shed light on the problem.
3. Use Terraform Commands
Terraform provides several commands that can be useful for troubleshooting and debugging. Here are a few commonly used commands:
– terraform validate
: Checks the syntax and configuration of your Terraform files without executing the actual deployment. This command can help identify any syntax errors or invalid configurations.
– terraform plan
: Generates an execution plan for your Terraform code without making any changes to your infrastructure. Review the plan output to verify that it matches your expectations.
– terraform show
: Displays the current state of your infrastructure as recorded in the Terraform state file. Use this command to inspect the current state and identify any discrepancies.
4. Use Terraform Debugging Tools
In addition to the built-in commands, there are also third-party tools available that can assist with troubleshooting and debugging Terraform code.
– Terraform Language Server (terraform-ls): Provides language server protocol (LSP) support for Terraform. It offers features like code completion, validation, and hover documentation. Integrating this tool with your IDE can enhance your development experience and help catch potential issues early.
– Terraform fmt: A code formatter for Terraform that automatically formats your code according to the Terraform style guide. Consistent code formatting can make your code more readable and reduce the chances of introducing errors.
5. Check Provider Documentation and Community Forums
If you are still unable to resolve the issue, it can be helpful to consult the official documentation for the relevant provider. Provider documentation often includes troubleshooting guides and common error messages that can point you in the right direction.
Additionally, community forums and discussion boards, such as the Terraform forum, can be a valuable resource. Many experienced Terraform users are active on these platforms and can provide guidance or solutions to specific issues.
Remember that troubleshooting and debugging are iterative processes. It often involves trial and error, so don’t be discouraged if you don’t find an immediate solution. With patience and persistence, you’ll be able to identify and resolve issues in your Terraform code.