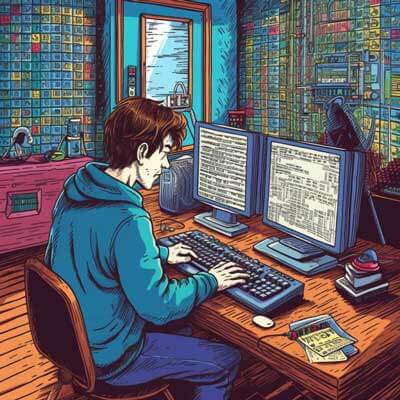
- Getting Started with Terraform
- Installation
- Initializing a Terraform Project
- Writing Your First Terraform Configuration
- Running Terraform Commands
- Understanding Infrastructure as Code
- Benefits of Infrastructure as Code
- Terraform as an Infrastructure as Code Tool
- Creating Your First Terraform Configuration
- Managing Infrastructure with Terraform
- 1. Infrastructure as Code
- 2. State Management
- 3. Terraform Workspaces
- 4. Terraform Modules
- 5. Terraform Cloud
- Understanding and Using Terraform Providers
- Understanding Terraform Providers
- Using Multiple Providers
- Working with Provider Versions
- Finding and Using Provider Documentation
- Advanced Resource Configuration
- 1. Using Terraform Modules
- 2. Dynamic Resource Creation
- 3. Terraform Provisioners
- 4. Terraform Backends
- Working with Variables and Outputs
- Defining Variables
- Using Variables
- Using Outputs
- Organizing Terraform Code with Modules
- What is a Terraform Module?
- Creating a Terraform Module
- Using a Terraform Module
- Module Versioning
- Module Registry
- Managing State with Terraform
- 1. Local State Backend
- 2. Remote State Backend
- 3. State Locking
- 4. Remote State Data
- 5. State Migration
- 6. State Backup and Recovery
- Terraform Best Practices
- 1. Use Version Control
- 2. Modularize Your Code
- 3. Use Variables and Outputs
- 4. Use Terraform Workspaces
- 5. Use Terraform Cloud or Terraform Enterprise
- 6. Leverage Terraform Modules from the Community
- 7. Regularly Update Terraform and Providers
- Terraform Workspaces and Environments
- Creating and Switching between Workspaces
- Managing Environment-specific Configurations
- Sharing Resources across Workspaces
- Using Data Sources in Terraform
- What are Data Sources?
- Using Data Sources
- Using Data Source Attributes
- Using External Data Sources
- Terraform Remote Backends
- What is a Remote Backend?
- Configuring a Remote Backend
- Initializing and Using a Remote Backend
- Benefits of Remote Backends
- Terraform and Cloud Provisioning
- Terraform Providers
- Provisioning Resources
- Terraform and Cloud Provisioning Best Practices
- Terraform and Container Orchestration
- Provisioning Kubernetes with Terraform
- Managing Kubernetes Resources with Terraform
- Other Container Orchestration Platforms
- Terraform and Serverless Computing
- What is Serverless Computing?
- Using Terraform with Serverless
- Benefits of Using Terraform with Serverless
- Infrastructure Automation with Terraform
- 1. Modularize your Terraform code
- 2. Use Terraform workspaces
- 3. Leverage Terraform remote state
- 4. Use Terraform workspaces and remote state together
- 5. Validate and format your Terraform code
- 6. Use Terraform’s plan command
- Terraform and Continuous Integration/Deployment
- Automating Terraform with CI/CD
- Managing Environment-specific Configurations
- Testing Infrastructure Code
- Versioning Infrastructure Code
- Terraform and Configuration Management Tools
- Chef
- Puppet
- Ansible
- Terraform and Security Best Practices
- 1. Use Secure Credentials Management
- 2. Apply Least Privilege Principle
- 3. Use Secure State Storage
- 4. Enable Detailed Logging
- 5. Regularly Update Terraform and Providers
Getting Started with Terraform
Terraform is a powerful infrastructure as code (IaC) tool that allows you to define and manage your infrastructure using a declarative language. In this chapter, we will cover the basics of getting started with Terraform and setting up your first project.
Related Article: Terraform Advanced Tips on Google Cloud
Installation
To begin, you need to install Terraform on your local machine. Terraform is distributed as a binary package that you can download from the official website. Once downloaded, extract the contents of the package and add the Terraform executable to your system’s PATH.
To verify the installation, open a terminal and run the following command:
terraform version
If everything is set up correctly, you should see the version number of Terraform printed on the console.
Initializing a Terraform Project
After installing Terraform, you can start creating your first Terraform project. A Terraform project is organized as a directory containing one or more Terraform configuration files.
To initialize a new Terraform project, create a directory for your project and navigate to it in the terminal. Run the following command to initialize the project:
terraform init
This command initializes the project by downloading the necessary provider plugins and setting up the backend configuration. The backend configuration determines where Terraform stores its state, which is essential for tracking changes to your infrastructure.
Writing Your First Terraform Configuration
Once your project is initialized, you can start writing Terraform configuration files. The main configuration file for a Terraform project is typically named main.tf
. This file contains the infrastructure resources you want to manage.
Let’s start with a simple example of creating an AWS EC2 instance. Create a new file named main.tf
and add the following code:
provider "aws" { region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this example, we define the AWS provider and specify the desired region. We then create an EC2 instance resource with the specified AMI (Amazon Machine Image) and instance type.
Related Article: Quick and Easy Terraform Code Snippets
Running Terraform Commands
With the Terraform configuration in place, you can now execute various Terraform commands to manage your infrastructure.
To see a summary of the changes that Terraform will apply, run the following command:
terraform plan
This command creates an execution plan and displays a list of resources to be created, modified, or destroyed.
To apply the changes and create the infrastructure resources, run the following command:
terraform apply
Terraform will prompt for confirmation before proceeding. Enter “yes” to proceed with the changes.
To destroy the created resources and clean up your infrastructure, use the following command:
terraform destroy
Be cautious when running this command, as it will permanently remove the resources defined in your Terraform configuration.
Understanding Infrastructure as Code
Infrastructure as Code (IaC) is a practice that allows developers to manage and provision infrastructure resources using code rather than manual processes. This approach brings the benefits of version control, collaboration, and automation to infrastructure management.
Terraform, as an IaC tool, enables you to define, provision, and manage infrastructure resources across various cloud providers. It uses a declarative language to describe the desired state of your infrastructure, and then automatically creates or modifies resources to match that state.
By understanding the concepts and principles behind IaC, you can effectively leverage Terraform to manage your infrastructure efficiently.
Benefits of Infrastructure as Code
There are several key benefits to adopting Infrastructure as Code:
1. **Version Control**: With IaC, infrastructure configurations are treated as code and can be stored in version control systems like Git. This enables tracking changes, rolling back to previous versions, and collaborating with other team members.
2. **Reproducibility**: By codifying your infrastructure, you can easily recreate and provision identical environments across different stages of development, testing, and production. This eliminates the need for manual setup and reduces the chances of configuration drift.
3. **Automation**: Infrastructure provisioning can be automated through tools like Terraform, allowing you to define and manage your infrastructure resources using code. This reduces manual effort, speeds up deployments, and improves consistency.
4. **Scalability**: IaC enables you to scale your infrastructure resources up or down by simply modifying the code. With Terraform, you can define and manage complex infrastructure setups, including virtual machines, networks, databases, and more.
Related Article: Terraform Advanced Tips for AWS
Terraform as an Infrastructure as Code Tool
Terraform is a popular choice for implementing Infrastructure as Code due to its simplicity, flexibility, and support for multiple cloud providers. It uses a declarative language called HashiCorp Configuration Language (HCL) to define the desired state of your infrastructure.
Here is an example of a simple Terraform configuration file, main.tf
, that provisions an AWS EC2 instance:
provider "aws" { access_key = "your-access-key" secret_access_key = "your-secret-access-key" region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this example, the provider
block specifies the AWS credentials and region. The resource
block defines an EC2 instance using the specified AMI and instance type.
To provision the infrastructure defined in the configuration file, you can run the following Terraform commands:
terraform init terraform plan terraform apply
Terraform will automatically create or modify the necessary AWS resources to match the desired state defined in the configuration file.
Creating Your First Terraform Configuration
In this chapter, we will walk through the process of creating your first Terraform configuration. This will serve as a foundation for understanding how to use Terraform effectively.
To begin, make sure you have Terraform installed on your machine. If you haven’t done so already, you can download it from the official website: https://www.terraform.io/downloads.html
Once you have Terraform installed, create a new directory for your Terraform project. In this directory, create a file named main.tf
to define your Terraform configuration.
Open main.tf
in a text editor and start by specifying the provider you want to use. Providers are responsible for managing the lifecycle of resources. For this tutorial, we will use the AWS provider as an example:
provider "aws" { region = "us-west-2" }
In this example, we are using the AWS provider and specifying the region as us-west-2
. Feel free to change the region to match your desired location.
Next, we can define the resources we want to create. Let’s start by creating an EC2 instance:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this code snippet, we are creating an EC2 instance using the specified AMI and instance type. Again, you can modify these values to suit your needs.
Once you have defined your resources, save the main.tf
file. Now, open a terminal or command prompt and navigate to the directory where your Terraform configuration is located.
Run terraform init
to initialize your Terraform project. This command will download the necessary provider plugins and set up the backend configuration.
After initialization, you can run terraform plan
to see an execution plan of what Terraform will do. This command will show you any changes that will be made to your infrastructure.
Finally, run terraform apply
to apply the changes and create your resources. Terraform will prompt you to confirm before making any changes.
Congratulations! You have successfully created your first Terraform configuration. You can now manage your infrastructure as code using Terraform.
In the next chapter, we will explore additional features and best practices for advanced usage of Terraform.
Managing Infrastructure with Terraform
In this chapter, we will explore how to effectively manage infrastructure using Terraform. Terraform is a powerful tool that allows you to define and provision infrastructure as code, making it easier to manage and scale your infrastructure.
1. Infrastructure as Code
One of the key concepts in Terraform is Infrastructure as Code (IaC). With IaC, you define your infrastructure in a declarative language and store it in version control. This approach allows you to easily manage and track changes to your infrastructure over time.
Terraform uses its own language called HashiCorp Configuration Language (HCL) to define infrastructure. Here’s an example of a simple Terraform configuration file:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this example, we are defining an AWS EC2 instance resource. We specify the AMI ID and the instance type. Terraform will use this configuration to create and manage the EC2 instance.
2. State Management
Terraform uses a state file to keep track of the resources it manages. The state file contains information about the infrastructure that Terraform is managing, such as resource IDs and metadata. It is critical to manage the state file properly to ensure consistency and avoid conflicts.
By default, Terraform stores the state file locally. However, this can cause issues in a team environment where multiple users are working on the same infrastructure. To address this, Terraform supports remote state backends, such as Amazon S3 or HashiCorp Consul. Using a remote backend allows for better collaboration and ensures the state file is stored securely.
Here’s an example of configuring a remote state backend using S3:
terraform { backend "s3" { bucket = "my-terraform-state" key = "terraform.tfstate" region = "us-east-1" } }
With this configuration, Terraform will store the state file in an S3 bucket named “my-terraform-state” in the US East 1 region. This ensures that the state file is accessible to all team members and can be easily shared across environments.
3. Terraform Workspaces
Terraform workspaces allow you to manage multiple instances of the same infrastructure in an organized manner. Workspaces are useful when you have multiple environments, such as development, staging, and production, and you want to manage them separately.
By default, Terraform creates a workspace called “default”. You can create additional workspaces using the terraform workspace new
command. For example, to create a workspace for the staging environment, you can run:
terraform workspace new staging
Each workspace has its own state file, allowing you to manage the infrastructure for each environment independently. You can switch between workspaces using the terraform workspace select
command.
4. Terraform Modules
Terraform modules are reusable components that encapsulate infrastructure resources and configurations. Modules allow you to abstract and share common infrastructure patterns, making it easier to manage and provision complex infrastructure.
Here’s an example of a simple Terraform module that creates an AWS VPC:
variable "vpc_cidr_block" { description = "CIDR block for the VPC" type = string } resource "aws_vpc" "example" { cidr_block = var.vpc_cidr_block }
With this module, you can create multiple VPCs by providing different values for the vpc_cidr_block
variable. Modules can be published and shared with others, promoting collaboration and code reuse.
5. Terraform Cloud
Terraform Cloud is a managed service by HashiCorp that provides collaboration and automation features for Terraform. It allows you to store and manage your Terraform configurations, state files, and variables in the cloud.
With Terraform Cloud, you can easily collaborate with team members, manage infrastructure across multiple environments, and automate workflows using features like remote execution and policy enforcement. It also provides additional security features, such as fine-grained access controls and audit logs.
To get started with Terraform Cloud, you can sign up for a free account at https://app.terraform.io/signup/account.
In this chapter, we covered the fundamentals of managing infrastructure with Terraform. We discussed Infrastructure as Code, state management, workspaces, modules, and introduced Terraform Cloud. These advanced tips will help you efficiently manage your infrastructure and scale your applications effectively.
Understanding and Using Terraform Providers
In Terraform, providers are plugins that allow you to interact with different infrastructure platforms or services. They enable you to manage resources in various cloud providers, such as AWS, Azure, and Google Cloud, as well as other services like Docker, GitHub, and Kubernetes. In this chapter, we will dive deeper into understanding and using Terraform providers effectively.
Understanding Terraform Providers
Terraform providers are responsible for translating Terraform configurations into API calls for the respective platforms or services. They act as the bridge between Terraform and the infrastructure you are managing. Providers are distributed separately from Terraform itself, allowing you to install and use only the ones you need.
To use a provider, you first need to declare it in your Terraform configuration file using the provider
block. The provider block specifies the name of the provider, as well as any required configuration settings. Here’s an example of declaring the AWS provider in a Terraform configuration file:
provider "aws" { region = "us-west-2" }
In this example, we declare the AWS provider and set the region to “us-west-2”. This tells Terraform to use the AWS provider and target resources in the specified region.
Using Multiple Providers
Terraform allows you to use multiple providers within a single configuration. This is useful when managing resources across different cloud providers or services. You can define multiple provider blocks and specify the provider name and configuration for each. Here’s an example of using both the AWS and Azure providers:
provider "aws" { region = "us-west-2" } provider "azurerm" { features {} }
In this example, we declare the AWS provider and set the region to “us-west-2”. We also declare the Azure provider without any specific configuration. Terraform will use the appropriate provider based on the resources defined in the configuration.
Working with Provider Versions
Providers are regularly updated with bug fixes, new features, and improvements. It is important to manage the versions of the providers you use to ensure compatibility and stability. Terraform supports version constraints for providers, allowing you to specify the acceptable range of provider versions.
To manage provider versions, you can use the required_providers
block in your Terraform configuration file. Here’s an example of declaring a required AWS provider version:
terraform { required_providers { aws = { source = "hashicorp/aws" version = ">= 3.0.0, < 4.0.0" } } }
In this example, we specify that the AWS provider version should be greater than or equal to 3.0.0 but less than 4.0.0. Terraform will automatically download and use the appropriate version of the provider within this version constraint.
Finding and Using Provider Documentation
Each provider has its own documentation that provides detailed information about the available resources, data sources, and configuration options. The documentation also includes examples and best practices for using the provider in Terraform.
To find the documentation for a specific provider, visit the official Terraform Registry website at https://registry.terraform.io/. Search for the provider you want to use, and you will find the documentation along with any additional resources or community modules.
Advanced Resource Configuration
In this chapter, we will explore advanced techniques for configuring resources in Terraform. These tips will help you optimize your infrastructure deployment and make your code more maintainable.
1. Using Terraform Modules
Terraform modules are reusable components that can be used to encapsulate and share common configurations. They allow you to create a higher level of abstraction, making your code more modular and easier to manage. Modules can be used to define complex resources, such as a multi-tier application or a Kubernetes cluster, and can be shared across different projects.
To use a module in your Terraform configuration, you need to define a module block and specify the source where the module is located. For example:
module "vpc" { source = "git::https://github.com/example/vpc-module.git" cidr_block = "10.0.0.0/16" }
This example uses a module named “vpc” from a Git repository. The module expects a parameter called “cidr_block” to be passed.
2. Dynamic Resource Creation
In some cases, you may need to create multiple instances of a resource based on a variable or a list. Terraform allows you to achieve this through dynamic resource creation.
For example, let’s say you want to create multiple AWS EC2 instances based on a list of instance types:
variable "instance_types" { type = list(string) default = ["t2.micro", "t2.small", "t2.medium"] } resource "aws_instance" "example" { count = length(var.instance_types) instance_type = var.instance_types[count.index] # Other resource configuration... }
In this example, the count
parameter is set to the length of the instance_types
list. This will create three EC2 instances with different instance types.
3. Terraform Provisioners
Terraform provisioners allow you to run scripts or commands on the created resources during the deployment process. This can be useful for tasks like installing software, running configuration scripts, or executing additional setup steps.
There are two types of provisioners: local-exec
and remote-exec
. The local-exec
provisioner runs commands on the machine running Terraform, while the remote-exec
provisioner runs commands on the created resource.
Here’s an example of using a local-exec
provisioner to run a script after creating an EC2 instance:
resource "aws_instance" "example" { # Resource configuration... provisioner "local-exec" { command = "./configure.sh ${self.public_ip}" } }
In this example, the local-exec
provisioner runs the configure.sh
script and passes the public IP address of the created EC2 instance as a parameter.
4. Terraform Backends
Terraform backends are used to store the Terraform state, which contains information about the deployed infrastructure. By default, Terraform stores the state file locally, but this can cause issues when working in a team or with multiple workstations.
By configuring a backend, you can store the state file in a remote location, such as an S3 bucket or a Terraform Cloud workspace. This allows for better collaboration and eliminates the need to manage the state file manually.
To configure a backend, you need to add a backend
block in your Terraform configuration. For example, to use an S3 bucket as the backend:
terraform { backend "s3" { bucket = "my-terraform-state" key = "terraform.tfstate" region = "us-west-2" } }
In this example, the Terraform state will be stored in the “my-terraform-state” S3 bucket in the “us-west-2” region.
These advanced techniques will help you take your Terraform usage to the next level. By leveraging modules, dynamic resource creation, provisioners, and backends, you can create more efficient and maintainable infrastructure as code.
Working with Variables and Outputs
In this chapter, we will explore how to work with variables and outputs in Terraform. Variables allow us to define values that can be used throughout our infrastructure code, while outputs provide a way to extract specific information from our infrastructure.
Defining Variables
Variables in Terraform are defined using the variable
block. We can specify the type of the variable, its default value, and even set constraints. Here’s an example of how to define a variable for an AWS access key:
variable "access_key" { type = string description = "AWS access key" default = "XXXXXXXXXXXXXXXXXXXX" }
In this example, we have defined a variable named access_key
of type string
with a default value. We can now use this variable throughout our code by referencing it as var.access_key
.
Using Variables
Once we have defined our variables, we can use them in various parts of our infrastructure code. For example, let’s say we want to create an AWS EC2 instance and use the access key defined in the previous section. We can do this by referencing the variable directly:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" key_name = "mykey" access_key = var.access_key }
In this example, we are setting the access_key
attribute of the aws_instance
resource to the value of the access_key
variable.
Using Outputs
Outputs in Terraform allow us to extract specific information from our infrastructure. This can be useful when we need to reference certain values outside of our Terraform code. To define an output, we use the output
block. Here’s an example of how to define an output for the public IP address of an AWS EC2 instance:
output "public_ip" { value = aws_instance.example.public_ip }
In this example, we have defined an output named public_ip
and set its value to the public_ip
attribute of the aws_instance.example
resource.
We can then use this output in other parts of our code or even retrieve it using the Terraform CLI. For example, to display the value of the public_ip
output, we can run the following command:
terraform output public_ip
This will display the public IP address of the AWS EC2 instance.
Organizing Terraform Code with Modules
Terraform modules are reusable components that allow you to encapsulate and organize your infrastructure code. Modules help to promote code reusability, simplify complex configurations, and enhance the maintainability of your Terraform projects. In this chapter, we will explore how to organize your Terraform code using modules effectively.
What is a Terraform Module?
A Terraform module is a self-contained collection of Terraform configuration files that represent a cohesive set of infrastructure resources. It encapsulates a group of resources and provides a clean interface for consuming them. Modules can be used to represent a single resource or a complex set of resources that work together.
Modules consist of a directory with one or more .tf
files. These files define the resources, variables, and outputs specific to the module. By organizing your infrastructure code into modules, you can easily share and reuse them across different projects and teams.
Creating a Terraform Module
To create a Terraform module, you need to follow a specific directory structure. Let’s say we want to create a module to manage an AWS S3 bucket. The directory structure for the module would look as follows:
s3_bucket/ ├── main.tf ├── variables.tf └── outputs.tf
In the s3_bucket
directory, we have three files: main.tf
, variables.tf
, and outputs.tf
. The main.tf
file defines the resources, such as the S3 bucket and its properties. The variables.tf
file defines the input variables that can be passed to the module, allowing customization. The outputs.tf
file defines the output values that can be accessed by other modules or the root configuration.
Using a Terraform Module
Once you have created a module, you can use it in your Terraform configurations by referencing its source. To use the s3_bucket
module we created earlier, you can add the following code to your Terraform configuration:
module "my_s3_bucket" { source = "github.com/myorg/my_module" version = "1.0.0" bucket_name = "my-bucket" region = "us-west-2" }
In this example, we are using the my_s3_bucket
module, setting the source
to the module’s location and specifying a version. We are also providing values for the bucket_name
and region
variables defined in the module.
Module Versioning
Versioning is crucial when working with modules to ensure the stability and consistency of your infrastructure. You can use any version control system (such as Git) to manage your modules. Terraform supports various versioning systems, including Git tags, branch names, and commit hashes.
When using modules, it is recommended to define a version constraint to ensure a predictable and consistent behavior of your infrastructure code. For example, you can specify a version constraint like >= 1.0.0, < 2.0.0
to allow any version in the 1.x
range but prevent the use of version 2.0.0
or higher.
Module Registry
The Terraform Module Registry is a public repository of reusable modules created by the Terraform community and HashiCorp. It provides a central location where you can discover and share modules for various cloud providers and services.
To use modules from the Terraform Module Registry, you can specify the registry source in your Terraform configuration. For example:
module "my_s3_bucket" { source = "terraform-aws-modules/s3-bucket/aws" version = "2.0.0" bucket_name = "my-bucket" region = "us-west-2" }
In this example, we are using the terraform-aws-modules/s3-bucket/aws
module from the Terraform Module Registry. We specify the version and provide values for the required variables.
Managing State with Terraform
Terraform provides a state management mechanism to keep track of the current state of your infrastructure. The state file is a crucial component that allows Terraform to understand the resources it manages and track any changes made to them. In this chapter, we will explore various techniques for managing the state in Terraform.
1. Local State Backend
By default, Terraform uses a local state backend, which stores the state file on the local disk. While this is convenient for getting started quickly, it has limitations when working with a team or in a distributed environment. When using a local state backend, the state file should be treated as a valuable asset and stored in a version control system to enable collaboration and reproducibility.
2. Remote State Backend
To overcome the limitations of the local state backend, Terraform supports various remote state backends. These backends store the state file remotely and provide features like versioning, locking, and access control. Popular remote state backends include Amazon S3, Azure Blob Storage, and HashiCorp Consul. To use a remote state backend, you need to configure it in your Terraform configuration file.
Here’s an example of configuring an Amazon S3 bucket as a remote state backend:
terraform { backend "s3" { bucket = "my-terraform-state" key = "terraform.tfstate" region = "us-east-1" } }
3. State Locking
State locking is a critical feature when multiple team members are working on the same infrastructure. It prevents concurrent changes to the state file, ensuring consistency and preventing conflicts. Remote state backends like Amazon S3 and Azure Blob Storage provide built-in locking mechanisms. When using a remote state backend, Terraform will automatically acquire and release locks when performing operations.
4. Remote State Data
In addition to managing your infrastructure, Terraform allows you to retrieve and use data from the state file. This feature, known as remote state data, enables you to share information across different Terraform configurations or integrate with external systems. You can access remote state data using the data
block in your Terraform configuration.
Here’s an example of retrieving a variable from a remote state:
data "terraform_remote_state" "other" { backend = "s3" config = { bucket = "other-terraform-state" key = "terraform.tfstate" region = "us-east-1" } } variable "example_variable" { default = data.terraform_remote_state.other.outputs.example_variable }
5. State Migration
Over time, your infrastructure evolves, and you may need to update your Terraform configuration or change the state format. Terraform provides tools to migrate your existing state to match the latest configuration format. The terraform state
command allows you to manipulate the state directly, making it easier to perform state migrations.
For example, if you want to rename a resource in your state file, you can use the following command:
terraform state mv aws_instance.example aws_instance.new_example
6. State Backup and Recovery
To prevent data loss or corruption, it’s essential to regularly back up your state files. In case of accidental deletion or corruption, you can restore the state file from a backup to recover your infrastructure. Consider automating the backup process using scripts or tools to ensure consistency and reliability.
Remember to securely store your state backups to protect sensitive information about your infrastructure.
Terraform Best Practices
Terraform is a powerful infrastructure-as-code tool that allows you to define and manage your infrastructure in a declarative manner. Following best practices can help you write more efficient and maintainable Terraform code. In this chapter, we will discuss some of the key best practices to keep in mind when using Terraform.
1. Use Version Control
Using version control is essential for managing your Terraform codebase. It allows you to track changes, collaborate with others, and easily revert to previous versions if needed. Git is a widely-used version control system that integrates well with Terraform. Make sure to commit your changes regularly and include meaningful commit messages.
2. Modularize Your Code
Modularization is a crucial aspect of writing maintainable Terraform code. By breaking your infrastructure into reusable modules, you can promote code reuse, simplify updates, and improve overall readability. Each module should have a clear purpose and should be self-contained. Avoid hardcoding values inside modules and use variables and outputs to pass values between them.
Here’s an example of a module structure:
├── main.tf ├── vars.tf ├── outputs.tf
3. Use Variables and Outputs
Using variables and outputs allows you to make your Terraform code more flexible and reusable. Variables enable you to parameterize your modules and dynamically configure your infrastructure. Outputs allow you to expose values from your modules for other parts of your infrastructure or external systems to consume. Make sure to define variables and outputs with clear names and descriptions.
Here’s an example of variable and output definitions:
# vars.tf variable "instance_type" { description = "The EC2 instance type" default = "t2.micro" } # outputs.tf output "instance_id" { description = "The ID of the EC2 instance" value = aws_instance.example.id }
4. Use Terraform Workspaces
Terraform workspaces allow you to manage multiple environments or deployments within a single codebase. Each workspace has its own set of state files, enabling you to isolate resources and configurations. It is especially useful when you need to manage infrastructure for different environments like development, staging, and production. Switching between workspaces is as simple as running terraform workspace select
.
5. Use Terraform Cloud or Terraform Enterprise
Terraform Cloud or Terraform Enterprise provides additional features and capabilities that can enhance your Terraform workflow. These tools offer advanced collaboration, remote state management, and version control integration. They also provide a web-based UI for managing infrastructure. Consider using them for larger projects or when working with a team.
6. Leverage Terraform Modules from the Community
The Terraform community maintains a rich ecosystem of modules that can help you accelerate your infrastructure provisioning. These modules are contributed by the community and cover a wide range of use cases, including popular cloud providers, networking, security, and more. Make sure to review the module documentation and use reputable modules with active maintenance.
7. Regularly Update Terraform and Providers
Terraform and its providers regularly release updates that introduce new features, bug fixes, and security enhancements. It is important to keep your Terraform version and provider versions up to date to take advantage of these improvements. Regularly check for updates and review the release notes to understand any potential breaking changes.
In this chapter, we discussed several best practices for using Terraform effectively. By following these practices, you can write more efficient and maintainable infrastructure code. Remember to leverage version control, modularize your code, use variables and outputs, utilize Terraform workspaces, consider using Terraform Cloud or Terraform Enterprise, leverage community modules, and keep your Terraform and provider versions up to date.
Terraform Workspaces and Environments
Terraform workspaces and environments are powerful features that allow you to manage multiple instances of your infrastructure within a single Terraform configuration. They provide a way to organize and isolate your resources, allowing you to deploy and manage different environments (such as development, staging, and production) with ease.
Creating and Switching between Workspaces
To create a new workspace, you can use the terraform workspace new
command followed by the desired workspace name. For example, to create a workspace for your development environment, you can run:
$ terraform workspace new development
Once a workspace is created, you can switch to it using the terraform workspace select
command followed by the workspace name. For example, to switch to the development workspace, you can run:
$ terraform workspace select development
Terraform will automatically create a new environment-specific state file for each workspace, ensuring that changes made in one workspace do not affect resources in another workspace.
Managing Environment-specific Configurations
Workspaces also allow you to manage environment-specific configurations. You can use conditional expressions and variables to adapt your configuration based on the currently selected workspace.
For example, you might have different instance sizes or regions for each environment. You can define variables in your configuration file and set their values based on the workspace. Here’s an example of how you can conditionally set the instance size based on the selected workspace:
variable "instance_size" { description = "The size of the instance" type = string default = "small" validation { condition = var.instance_size != "small" || terraform.workspace != "development" error_message = "Invalid instance size for development workspace" } } resource "aws_instance" "example" { instance_type = var.instance_size # ... }
In this example, the instance_size
variable is conditionally set to "small"
only if the current workspace is “development”. Otherwise, an error will be displayed.
Sharing Resources across Workspaces
Sometimes it’s necessary to share resources between workspaces. For example, you might have a common VPC that needs to be shared across multiple environments. To achieve this, you can use Terraform data sources to reference resources from other workspaces.
For instance, if you have a VPC defined in the “shared” workspace, you can reference it in another workspace like this:
data "terraform_remote_state" "shared" { backend = "local" config = { path = "../shared/terraform.tfstate" } } resource "aws_instance" "example" { vpc_id = data.terraform_remote_state.shared.outputs.vpc_id # ... }
In this example, the terraform_remote_state
data source is used to access the state file of the “shared” workspace. The data.terraform_remote_state.shared.outputs.vpc_id
expression retrieves the VPC ID defined in the “shared” workspace, allowing you to use it in the current workspace.
Using Data Sources in Terraform
Data sources in Terraform allow you to retrieve information from external sources and use them in your infrastructure provisioning. They provide a way to query and import existing resources into your Terraform configuration, which can be extremely useful when you need to reference data from external systems or remote providers.
What are Data Sources?
Data sources in Terraform are blocks of configuration that define how to retrieve information from a specific external source. These sources can be created by Terraform itself or by third-party providers. Some common examples of data sources are AWS S3 buckets, Azure Resource Groups, or GitHub repositories.
When you define a data source, Terraform queries the external system or provider to fetch the required information. This information is then made available as attributes that you can reference in your Terraform configuration.
Using Data Sources
To use a data source in Terraform, you need to define it in your configuration file using the data
block. The data block specifies the type of data source and any required parameters. Here’s an example of how you can define a data source for an AWS S3 bucket:
data "aws_s3_bucket" "example" { bucket = "my-bucket" }
In this example, we are querying an AWS S3 bucket with the name “my-bucket”. The fetched attributes of the bucket can then be referenced using the syntax data...
. For example, to reference the bucket’s ARN, you can use data.aws_s3_bucket.example.arn
.
Using Data Source Attributes
Once you have defined a data source, you can use its attributes in your Terraform configuration. These attributes provide information about the queried resource and can be used in various ways. For example, you can use them to populate variables, configure resources, or create dynamic configurations.
Here’s an example that demonstrates how to use a data source attribute to populate a variable:
data "aws_s3_bucket" "example" { bucket = "my-bucket" } variable "bucket_arn" { default = data.aws_s3_bucket.example.arn }
In this example, the bucket_arn
variable is set to the ARN of the queried AWS S3 bucket. This allows you to reuse the bucket’s ARN in other parts of your configuration.
Using External Data Sources
In addition to built-in data sources, Terraform also allows you to define your own external data sources. These are custom scripts or executables that can be used to fetch data from any external system or provider.
To use an external data source, you need to define it in your configuration file using the external
data source type. You can then call the external data source using the external
function and pass any required parameters.
Here’s an example of how you can define and use an external data source:
data "external" "example" { program = ["python", "${path.module}/my_script.py"] query = { param1 = "value1" param2 = "value2" } } variable "external_data" { default = data.external.example.result }
In this example, we define an external data source that executes a Python script called my_script.py
. The script takes two parameters, param1
and param2
, and returns the result that can be accessed using data.external.example.result
.
Terraform Remote Backends
When working with Terraform, it is common to store the state file remotely in what is called a “remote backend”. This allows for collaboration and enables team members to work on the same infrastructure without conflicts. In this chapter, we will explore the concept of remote backends and learn how to configure and use them effectively.
What is a Remote Backend?
A remote backend is a storage location for Terraform state files that is accessible by multiple users. It allows for concurrent access to the state file, making it easier to collaborate on infrastructure changes. Terraform supports a variety of remote backends, including Amazon S3, Azure Blob Storage, Google Cloud Storage, and more.
Configuring a Remote Backend
To configure a remote backend in Terraform, you need to specify the backend configuration in your Terraform configuration file (typically named backend.tf
). Here is an example of how to configure the S3 backend in AWS:
terraform { backend "s3" { bucket = "my-terraform-state" key = "terraform.tfstate" region = "us-west-2" encrypt = true dynamodb_table = "terraform-state-lock" } }
In this example, we are using the S3 backend, specifying the bucket name, the key (which is the name of the state file), the AWS region, and enabling encryption. We also specify a DynamoDB table for state locking, which prevents concurrent modifications to the state.
Initializing and Using a Remote Backend
Once the backend configuration is in place, you can initialize the backend by running terraform init
. Terraform will prompt you to confirm the initialization of the backend, as it involves copying the local state to the remote backend. After initializing, all future Terraform commands will use the remote backend.
It is important to note that destroying or modifying the backend configuration can result in the loss of the state file, so exercise caution when making changes.
Benefits of Remote Backends
Using a remote backend offers several benefits:
1. Collaboration: Multiple team members can work on the same infrastructure without conflicts.
2. State Locking: Remote backends often provide state locking mechanisms to prevent concurrent modifications.
3. Improved Performance: Remote backends can handle large state files more efficiently, improving Terraform performance.
4. Version Control: By storing the state file remotely, you can easily track changes and rollbacks using version control systems.
Terraform and Cloud Provisioning
Terraform is a powerful tool that allows infrastructure to be provisioned and managed as code. In this chapter, we will explore how Terraform can be used to provision resources in various cloud platforms.
Terraform Providers
Terraform supports a wide range of cloud providers, including Amazon Web Services (AWS), Microsoft Azure, Google Cloud Platform (GCP), and many others. Each cloud provider is represented by a Terraform provider, which allows Terraform to interact with the provider’s API.
To use a specific cloud provider, you need to configure the provider block in your Terraform configuration file. Here’s an example of configuring the AWS provider:
provider "aws" { region = "us-west-2" }
This configuration sets the AWS region to “us-west-2”. You can find the specific configuration options for each provider in the Terraform documentation.
Provisioning Resources
Once you have configured the provider, you can start provisioning resources in your cloud environment. Terraform uses a declarative language to define the desired state of your infrastructure. Here’s an example of provisioning an AWS EC2 instance using Terraform:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
This code defines an AWS EC2 instance resource with the specified AMI (Amazon Machine Image) and instance type. When you run terraform apply
, Terraform will create the EC2 instance in your AWS account.
You can also define dependencies between resources using Terraform. For example, you can specify that an EC2 instance should be created only after a specific VPC (Virtual Private Cloud) has been created. This ensures that resources are provisioned in the correct order.
Terraform and Cloud Provisioning Best Practices
When working with Terraform and cloud provisioning, there are several best practices that can help you improve efficiency and maintainability:
- Use Terraform modules to organize and reuse infrastructure code.
- Separate environment-specific configurations using Terraform workspaces.
- Utilize Terraform remote state management for collaboration and version control.
- Use Terraform data sources to fetch information from existing cloud resources.
- Regularly test and validate your Terraform configurations using
terraform validate
andterraform plan
.
By following these best practices, you can ensure that your Terraform code is modular, reusable, and easy to maintain.
Terraform and Container Orchestration
Container orchestration platforms have become an essential part of modern application development and deployment. They provide the ability to manage and scale containerized applications efficiently. Terraform, being a powerful infrastructure as code tool, can also be used to manage container orchestration platforms such as Kubernetes, Docker Swarm, and Amazon ECS. In this chapter, we will explore how Terraform can be used to provision and manage resources in a container orchestration environment.
Provisioning Kubernetes with Terraform
Kubernetes is one of the most popular container orchestration platforms available today. With Terraform, you can provision a Kubernetes cluster along with the necessary resources such as nodes, pods, services, and ingress controllers. Here’s an example of how you can use Terraform to provision a Kubernetes cluster on AWS:
# main.tf provider "aws" { region = "us-west-2" } module "kubernetes" { source = "terraform-aws-modules/kubernetes/aws" cluster_name = "my-cluster" cluster_version = "1.19.0" vpc_id = "vpc-12345678" subnet_ids = ["subnet-12345678", "subnet-87654321"] }
In the above example, we use the Terraform AWS provider to specify the region where the Kubernetes cluster should be provisioned. We then use a Terraform module specifically designed for provisioning Kubernetes on AWS. The module takes parameters such as the cluster name, version, VPC ID, and subnet IDs.
Managing Kubernetes Resources with Terraform
Once you have provisioned a Kubernetes cluster, you can use Terraform to manage the resources within the cluster. This includes creating and managing deployments, services, ingress controllers, and more. Here’s an example of how you can use Terraform to create a Kubernetes deployment:
# deployment.tf resource "kubernetes_deployment" "my_app" { metadata { name = "my-app" labels = { app = "my-app" } } spec { replicas = 3 selector { match_labels = { app = "my-app" } } template { metadata { labels = { app = "my-app" } } spec { container { image = "my-app:latest" name = "my-app" port { container_port = 8080 } } } } } }
In the above example, we define a Kubernetes deployment resource using the kubernetes_deployment
Terraform resource type. We specify the metadata, replicas, selector, and template for the deployment. Within the template, we define a container with the image name, container name, and port mapping.
Other Container Orchestration Platforms
While Kubernetes is currently the most popular container orchestration platform, Terraform can also be used to provision and manage resources in other platforms such as Docker Swarm and Amazon ECS. The process is similar to provisioning a Kubernetes cluster. You would specify the provider, module, and relevant parameters for the specific platform.
Terraform and Serverless Computing
Serverless computing has gained popularity in recent years due to its ability to abstract away infrastructure management and provide scalable, event-driven computing resources. In this chapter, we will explore how Terraform can be used in conjunction with serverless computing to efficiently deploy and manage serverless applications.
What is Serverless Computing?
Serverless computing, also known as Function as a Service (FaaS), allows developers to write and deploy code without worrying about the underlying infrastructure. With serverless, you only pay for the actual execution time of your functions, rather than for idle resources. This makes it a cost-effective and scalable solution for many use cases.
Using Terraform with Serverless
Terraform, known for its infrastructure-as-code capabilities, can be a powerful tool when combined with serverless computing. Terraform allows you to define and manage your serverless resources in a declarative manner, just like any other infrastructure resource.
To get started with Terraform and serverless, you would typically define your serverless functions, triggers, and other resources using the appropriate provider. For example, if you are using AWS Lambda, you would utilize the AWS provider in Terraform.
Here is a simple example of using Terraform to define an AWS Lambda function:
resource "aws_lambda_function" "example" { function_name = "my-lambda-function" role = aws_iam_role.lambda_exec.arn handler = "index.handler" runtime = "nodejs12.x" filename = "lambda_function.zip" }
In this example, we define an AWS Lambda function with a specific function name, IAM role, handler, runtime, and the filename of the function code. This code can then be version-controlled, shared, and deployed using Terraform.
Benefits of Using Terraform with Serverless
By leveraging Terraform’s capabilities, you can achieve several benefits when working with serverless computing:
1. **Infrastructure as Code**: With Terraform, you can treat your serverless resources as code, allowing for version control, code reviews, and easier collaboration.
2. **Declarative Configuration**: Terraform’s declarative language allows you to define the desired state of your serverless resources, and Terraform takes care of the provisioning and management.
3. **Resource Management**: Terraform provides a consistent, reliable way to create, update, and delete serverless resources, ensuring that your infrastructure is always in the desired state.
4. **Integration with Existing Infrastructure**: Terraform can seamlessly integrate serverless resources with other infrastructure components, such as networking, databases, and storage.
5. **Scalability and Cost Optimization**: With Terraform, you can easily scale your serverless resources up or down based on demand, optimizing costs and ensuring efficient resource allocation.
Infrastructure Automation with Terraform
Infrastructure automation is a key aspect of managing modern cloud-based environments. By automating the provisioning and management of infrastructure resources, organizations can achieve greater efficiency, scalability, and consistency. Terraform, an Infrastructure as Code (IaC) tool developed by HashiCorp, is widely used for automating infrastructure provisioning across multiple cloud providers.
In this chapter, we will explore advanced tips for efficient usage of Terraform to automate your infrastructure.
1. Modularize your Terraform code
As your infrastructure grows in complexity, it becomes essential to organize your Terraform code into reusable modules. Modules in Terraform allow you to encapsulate related resources and configurations, making them easier to manage, share, and reuse across different projects. By modularizing your code, you can avoid duplication, promote consistency, and simplify maintenance.
Here’s an example of a simple Terraform module for provisioning an AWS EC2 instance:
# main.tf variable "ami_id" { type = string default = "ami-0c94855ba95c71c99" } resource "aws_instance" "example" { ami = var.ami_id instance_type = "t2.micro" # ... other configurations }
2. Use Terraform workspaces
Terraform workspaces enable you to manage multiple instances of the same infrastructure in parallel. Workspaces provide isolation and allow you to maintain separate state files for each environment, such as development, staging, and production. This separation ensures that changes made in one workspace do not affect others, reducing the risk of accidental changes to critical environments.
To create a new workspace in Terraform:
$ terraform workspace new dev
To switch between workspaces:
$ terraform workspace select dev
3. Leverage Terraform remote state
Terraform remote state allows you to store your state file in a remote backend, such as Amazon S3 or HashiCorp Terraform Cloud. Storing the state remotely provides better collaboration and consistency when working in a team. It also allows you to share state across multiple projects and environments, facilitating infrastructure changes and deployments.
To configure remote state in Terraform, add the following block to your backend.tf
file:
terraform { backend "s3" { bucket = "my-terraform-state-bucket" key = "my-terraform-state-key" # ... other configurations } }
4. Use Terraform workspaces and remote state together
Combining Terraform workspaces and remote state can be a powerful combination. By using different workspaces with separate remote state, you can manage multiple environments easily. Each workspace can have its own state file, allowing you to track and manage infrastructure changes independently.
For example, assuming you have a workspace for the dev
environment and another for prod
, you can switch to the prod
workspace and deploy changes without affecting the dev
environment.
5. Validate and format your Terraform code
To ensure the quality and consistency of your Terraform code, it’s essential to validate and format it correctly. Terraform provides a built-in command called terraform fmt
that automatically formats your code according to the best practices and style conventions. Running this command periodically helps you maintain a standardized codebase and avoids unnecessary differences in your code repository.
To format your Terraform code:
$ terraform fmt
To validate your Terraform code without applying it:
$ terraform validate
6. Use Terraform’s plan command
Terraform’s plan
command is a powerful tool that allows you to preview the changes that Terraform will apply to your infrastructure. It provides an overview of resource creations, modifications, or deletions before actually applying them. This can help you catch potential issues and ensure that the changes align with your expectations.
To generate and view a plan for your Terraform changes:
$ terraform plan
These advanced tips will help you optimize your usage of Terraform and enhance your infrastructure automation processes. By leveraging modularization, workspaces, remote state, code validation, and the plan command, you can efficiently manage and automate your infrastructure provisioning tasks with Terraform.
Terraform and Continuous Integration/Deployment
Continuous Integration (CI) and Continuous Deployment (CD) are crucial practices in modern software development. They allow teams to automate the process of building, testing, and deploying software, ensuring that changes are quickly and reliably incorporated into production environments. Terraform can be seamlessly integrated into CI/CD pipelines, enabling infrastructure changes to be managed along with application code.
Automating Terraform with CI/CD
Integrating Terraform with CI/CD pipelines allows for the automation of infrastructure changes as part of the software development lifecycle. With CI/CD, each code change triggers a series of actions, including building and testing the code, and deploying it to a staging or production environment. Terraform can be included in these pipelines to automate infrastructure provisioning and management.
To get started with Terraform in your CI/CD pipeline, follow these steps:
1. Create a repository for your infrastructure code, separate from your application code.
2. Set up a CI/CD pipeline using a tool like Jenkins, CircleCI, or GitLab CI/CD.
3. Configure the pipeline to execute Terraform commands, such as terraform init
, terraform plan
, and terraform apply
, as part of the deployment process.
4. Store your Terraform state in a remote backend, such as Amazon S3 or Azure Blob Storage, to ensure consistency and collaboration across pipeline runs.
Managing Environment-specific Configurations
In a typical CI/CD pipeline, you may have multiple environments, such as development, staging, and production. Each environment may require different configurations, such as resource sizes, network settings, or API keys. Terraform provides several mechanisms to manage environment-specific configurations.
One approach is to use Terraform workspaces, which allow you to maintain separate state files for each environment. This enables you to manage environment-specific variables and configurations without duplicating code. For example, you can define a variable that specifies the resource size for each environment:
variable "instance_size" { type = string default = "small" validation { condition = var.instance_size != "" error_message = "Instance size must be provided." } }
Another approach is to use Terraform input variables, which can be set during the deployment process. This allows you to pass environment-specific values to Terraform without modifying the code. For example, you can use environment variables in your CI/CD pipeline to set different values for each environment:
export TF_VAR_instance_size="medium"
Testing Infrastructure Code
Testing infrastructure code is as important as testing application code. Terraform provides a testing framework called Terratest, which allows you to write automated tests for your infrastructure code. Terratest lets you write tests in Go that can spin up real infrastructure resources, provision them using Terraform, and then verify their state.
Here’s an example of a Terratest test that verifies the creation of an AWS EC2 instance:
func TestTerraformEC2Instance(t *testing.T) { terraformOptions := &terraform.Options{ TerraformDir: "../infra", } defer terraform.Destroy(t, terraformOptions) terraform.InitAndApply(t, terraformOptions) instanceID := terraform.Output(t, terraformOptions, "instance_id") instance := aws.GetEC2Instance(t, instanceID, "us-west-2") assert.Equal(t, "t2.micro", instance.InstanceType) assert.Equal(t, "running", instance.State.Name) }
Versioning Infrastructure Code
Just like application code, it is crucial to version control your infrastructure code. This enables you to track changes, collaborate with your team, and roll back to previous versions if necessary. Git is a popular version control system for managing infrastructure code.
By using Git tags, you can mark specific versions of your infrastructure code. This allows you to easily reference and deploy specific versions during your CI/CD pipeline. For example, you can tag a version of your infrastructure code with the Git command:
git tag v1.0.0
Then, in your CI/CD pipeline, you can specify the tagged version to deploy:
terraform init -from-module=git::https://github.com/your-repo/your-infrastructure.git?ref=v1.0.0
Terraform and Configuration Management Tools
In this chapter, we will explore how Terraform can be integrated with popular configuration management tools to enhance the efficiency of infrastructure provisioning and management. Configuration management tools help in automating the configuration and deployment of software across different environments, making them a natural fit with Terraform.
Chef
Chef is a popular configuration management tool that provides a domain-specific language (DSL) for writing infrastructure as code. It allows you to define the desired state of your infrastructure and automatically applies the necessary changes to achieve that state.
Terraform can be seamlessly integrated with Chef to provision and manage infrastructure resources. By using the chef provisioner
in Terraform, you can execute Chef cookbooks and recipes during the provisioning process. This allows you to configure and bootstrap instances with the desired software packages and configurations.
Here’s an example of how to use the Chef provisioner in a Terraform configuration:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "chef" { chef_environment = "dev" run_list = ["recipe[my_cookbook::default]"] } }
In the above example, when Terraform provisions an AWS EC2 instance, it also runs the specified Chef recipe (from the cookbook my_cookbook
) on that instance. This allows you to automate the configuration of the instance using Chef.
Puppet
Another popular configuration management tool is Puppet. Puppet provides a declarative language for defining infrastructure configurations and automating the deployment and management of software.
Similar to Chef, Puppet can be integrated with Terraform using the puppet provisioner
. This provisioner allows you to apply Puppet manifests to provisioned resources, ensuring the desired state of your infrastructure.
Here’s an example of using the Puppet provisioner in Terraform:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "puppet" { manifest_file = "manifests/site.pp" } }
In the above example, Terraform provisions an AWS EC2 instance and applies the specified Puppet manifest (site.pp
) to configure the instance.
Ansible
Ansible is an open-source configuration management tool that focuses on simplicity and ease of use. It uses a simple YAML-based language to define infrastructure configurations and execute tasks on remote hosts.
Terraform can be integrated with Ansible using the local-exec
provisioner. With this provisioner, you can execute Ansible playbooks or ad-hoc commands on provisioned resources.
Here’s an example of using the local-exec provisioner to execute an Ansible playbook:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" provisioner "local-exec" { command = "ansible-playbook -i ${self.private_ip}, playbook.yml" } }
In the above example, Terraform provisions an AWS EC2 instance and executes an Ansible playbook (playbook.yml
) on that instance using the local-exec provisioner.
Terraform and Security Best Practices
In this chapter, we will explore some best practices for ensuring the security of your Terraform deployments. By following these guidelines, you can minimize the risk of unauthorized access, data breaches, and other security vulnerabilities.
1. Use Secure Credentials Management
To protect your sensitive credentials, it is crucial to use a secure credentials management system. Avoid hardcoding credentials directly in your Terraform code or storing them in plain text files. Instead, consider using a secure secrets management tool like HashiCorp Vault or AWS Secrets Manager to store and manage your credentials securely.
Here’s an example of how to use HashiCorp Vault to securely retrieve credentials in a Terraform configuration file:
provider "aws" { access_key = vault_generic_secret.aws_creds.data["access_key"] secret_key = vault_generic_secret.aws_creds.data["secret_key"] } data "vault_generic_secret" "aws_creds" { path = "secret/aws" }
2. Apply Least Privilege Principle
Follow the principle of least privilege when configuring your Terraform IAM roles and policies. Grant only the necessary permissions to perform specific tasks and avoid using overly permissive policies. Regularly review and audit the permissions granted to your Terraform infrastructure to ensure they align with the principle of least privilege.
3. Use Secure State Storage
Protect the state files that Terraform uses to manage your infrastructure. Store them in a secure location with strict access controls. Avoid storing state files in version control systems or insecure storage solutions. Consider using remote state backends like Terraform Cloud, AWS S3, or Azure Blob Storage to securely store and manage your state files.
Here’s an example of configuring Terraform to use an S3 bucket as the remote state backend:
terraform { backend "s3" { bucket = "my-terraform-state-bucket" key = "terraform.tfstate" region = "us-west-2" encrypt = true dynamodb_table = "terraform-state-lock" } }
4. Enable Detailed Logging
Enable detailed logging to provide visibility into your Terraform activities. Logging helps in detecting and investigating security incidents, as well as monitoring the health and performance of your infrastructure. Consider integrating Terraform with centralized log management systems like Elasticsearch, Splunk, or AWS CloudWatch Logs.
To enable detailed logging in Terraform, set the TF_LOG
environment variable to a desired log level:
export TF_LOG=DEBUG
5. Regularly Update Terraform and Providers
Keep your Terraform installation and provider plugins up to date with the latest security patches and bug fixes. Regularly check for updates and follow the release notes of Terraform and its providers to stay informed about security-related updates. Updating to the latest versions ensures that you have the most secure and stable environment for your infrastructure.
These best practices can help you enhance the security of your Terraform deployments. By following these guidelines, you can mitigate security risks and ensure the confidentiality, integrity, and availability of your infrastructure.
Remember, security is an ongoing process, so regularly review and update your security measures to adapt to changing threats and technologies.
Continue reading to learn more about advanced techniques and optimizations in Terraform.