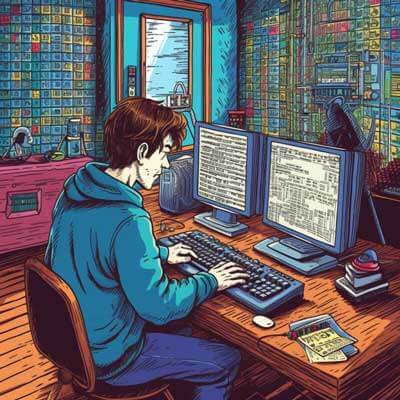
Table of Contents
The field of software development has evolved significantly over the years, and with it, the need for efficient and streamlined processes. DevOps automation tools have emerged as a solution to help software development teams automate various aspects of their workflows, from continuous integration to deployment and monitoring. In this article, we will explore the different categories of DevOps automation tools and their significance in the software development lifecycle.
Continuous Integration in DevOps Automation Tools
Continuous Integration (CI) is a practice that aims to integrate code changes from multiple developers into a central repository regularly. It helps identify integration issues early on and ensures that the codebase remains stable. DevOps automation tools play a crucial role in enabling continuous integration by automating the build, test, and deployment processes.
One popular CI tool is Jenkins, which allows developers to define pipelines that automate the entire CI process. Here's an example of a Jenkins pipeline script that builds a Java project, runs unit tests, and deploys the application:
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean package' } } stage('Test') { steps { sh 'mvn test' } } stage('Deploy') { steps { sh 'mvn deploy' } } } }
In this example, the pipeline consists of three stages: build, test, and deploy. Each stage runs a set of commands using the Maven build tool to compile the code, run tests, and deploy the application, respectively.
Another popular CI tool is CircleCI, which offers a cloud-based platform for automating the CI process. Here's an example of a CircleCI configuration file that builds a Node.js project, runs tests, and publishes test coverage reports:
version: 2 jobs: build: docker: - image: node:12 steps: - checkout - run: npm ci - run: npm test - run: npx nyc report --reporter=text-lcov > coverage.lcov - store_artifacts: path: coverage.lcov
In this example, the configuration file defines a single job named 'build'. The job uses a Node.js Docker image as the execution environment and runs a sequence of commands to install dependencies, run tests, and generate test coverage reports. The coverage report is then stored as an artifact for further analysis.
Related Article: The Path to Speed: How to Release Software to Production All Day, Every Day (Intro)
Configuration Management in DevOps Automation
Configuration management is a vital aspect of DevOps automation, as it ensures that infrastructure and application configurations are consistent and reproducible. Configuration management tools help automate the process of provisioning and managing infrastructure resources, such as servers and networking components.
One widely used configuration management tool is Ansible, which allows you to define infrastructure configurations using a declarative language. Here's an example of an Ansible playbook that installs and configures Nginx on a Ubuntu server:
- name: Install and configure Nginx hosts: web_servers become: true tasks: - name: Install Nginx apt: name: nginx state: present - name: Configure Nginx template: src: nginx.conf.j2 dest: /etc/nginx/nginx.conf notify: restart nginx handlers: - name: restart nginx service: name: nginx state: restarted
In this example, the playbook defines a set of tasks to be executed on the 'web_servers' group of hosts. The tasks install Nginx using the apt package manager and then configure Nginx by copying a template file. Finally, the playbook triggers a handler to restart the Nginx service whenever a configuration change occurs.
Another popular configuration management tool is Puppet, which follows a similar declarative approach to define infrastructure configurations. Here's an example of a Puppet manifest that installs and configures Apache on a CentOS server:
class { 'apache': default_vhost => false, } apache::vhost { 'example.com': docroot => '/var/www/html', port => '80', }
In this example, the manifest declares a class named 'apache' and sets the 'default_vhost' parameter to false, indicating that the default Apache virtual host should not be configured. It then defines a virtual host named 'example.com' with a specific document root and port number.
Infrastructure as Code in DevOps Automation
Infrastructure as Code (IaC) is a practice that involves managing infrastructure resources using code. It enables teams to treat infrastructure configurations as version-controlled artifacts, making them more predictable, reproducible, and scalable.
Terraform is a popular infrastructure as code tool that allows you to define infrastructure resources using a declarative configuration language. Here's an example of a Terraform configuration file that provisions an AWS EC2 instance:
provider "aws" { region = "us-east-1" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" tags = { Name = "example-instance" } }
In this example, the configuration file defines an AWS provider and a resource of type 'aws_instance'. The resource specifies the AMI (Amazon Machine Image) to use and the instance type, along with a set of tags for identification purposes.
Another popular infrastructure as code tool is CloudFormation, which allows you to define infrastructure resources using a JSON or YAML template. Here's an example of a CloudFormation template that provisions an Amazon S3 bucket:
Resources: MyBucket: Type: AWS::S3::Bucket Properties: AccessControl: Private BucketName: my-bucket
In this example, the template defines a resource of type 'AWS::S3::Bucket' with the specified properties. The 'AccessControl' property sets the bucket's access control to private, and the 'BucketName' property sets the name of the bucket to 'my-bucket'.
These examples demonstrate how infrastructure as code tools help automate the provisioning and management of infrastructure resources, making it easier to create and maintain complex environments.
Importance of Version Control in DevOps Automation
Version control is a critical component of DevOps automation, as it enables teams to track changes to source code, infrastructure configurations, and other artifacts. It provides a history of changes, facilitates collaboration, and ensures that changes can be rolled back if needed.
Git is the most widely used version control system in the software development industry. It allows developers to track changes to their codebases and collaborate with other team members effectively. Here's an example of how to create a new Git repository and commit changes:
$ git init Initialized empty Git repository in /path/to/repository $ git add . $ git commit -m "Initial commit" [master (root-commit)]: created 3c3ba3d: "Initial commit" 3 files changed, 16 insertions(+) create mode 100644 file1.txt create mode 100644 file2.txt create mode 100644 file3.txt
In this example, the 'git init' command initializes a new Git repository in the specified directory. The 'git add .' command stages all changes in the repository, and the 'git commit' command creates a new commit with a descriptive message.
Another popular version control system is Subversion (SVN), which follows a centralized model for version control. Here's an example of how to create a new SVN repository and commit changes:
$ svnadmin create /path/to/repository $ svn checkout file:///path/to/repository/trunk my-project Checked out revision 0. $ cd my-project $ touch file1.txt $ svn add file1.txt A file1.txt $ svn commit -m "Initial commit" Adding file1.txt Transmitting file data . Committed revision 1.
In this example, the 'svnadmin create' command creates a new SVN repository in the specified directory. The 'svn checkout' command checks out the repository to a local working copy. The 'svn add' command stages the new file for commit, and the 'svn commit' command creates a new commit with a descriptive message.
These examples highlight the importance of version control in DevOps automation, providing teams with a reliable and efficient way to manage code and configuration changes.
Related Article: Ace Your DevOps Interview: Top 25 Questions and Answers
Containerization in DevOps Automation
Containerization has gained immense popularity in recent years as a means to package applications and their dependencies into lightweight, portable units. DevOps automation tools leverage containerization to streamline the deployment and management of applications across different environments.
Docker is the most widely adopted containerization platform, allowing developers to define and run containers using Docker images. Here's an example of a Dockerfile that builds a Node.js application:
FROM node:12 WORKDIR /app COPY package.json . RUN npm install COPY . . CMD ["npm", "start"]
In this example, the Dockerfile specifies a base image, sets the working directory, and copies the package.json file. It then installs dependencies, copies the application code, and defines the command to start the application.
Another popular containerization tool is Kubernetes, which provides a platform for orchestrating and managing containerized applications. Here's an example of a Kubernetes deployment manifest that deploys a containerized application:
apiVersion: apps/v1 kind: Deployment metadata: name: my-app spec: replicas: 3 template: metadata: labels: app: my-app spec: containers: - name: my-app-container image: my-app-image:latest ports: - containerPort: 8080
In this example, the deployment manifest defines a deployment with three replicas of a containerized application. It specifies the container image to use, along with the port to expose.
These examples demonstrate how containerization simplifies the deployment and management of applications, making them more scalable and portable.
Test Automation in DevOps
Test automation is a crucial aspect of DevOps automation, as it helps ensure the quality and stability of software products. Automation tools enable developers to write and execute tests automatically, reducing the time and effort required for manual testing.
One popular test automation framework is Selenium, which allows developers to write tests that simulate user interactions with web applications. Here's an example of a Selenium test script written in Python:
from selenium import webdriver # Instantiate the WebDriver driver = webdriver.Chrome() # Navigate to the application URL driver.get('https://www.example.com') # Perform actions on the web application element = driver.find_element_by_id('username') element.send_keys('testuser') # Close the WebDriver driver.quit()
In this example, the script uses the Selenium WebDriver to instantiate a Chrome browser instance and navigate to a specific URL. It then interacts with the web application by finding an element with the ID 'username' and entering a test username.
Another popular test automation tool is JUnit, a unit testing framework for Java applications. Here's an example of a JUnit test case:
import org.junit.Assert; import org.junit.Test; public class MyTest { @Test public void testAddition() { int result = Calculator.add(2, 3); Assert.assertEquals(5, result); } }
In this example, the test case uses the JUnit framework to define a test method named 'testAddition'. It calls a method from the 'Calculator' class and asserts that the result is equal to the expected value.
These examples illustrate how test automation tools enable developers to write and execute tests more efficiently, ensuring the quality and reliability of their software products.
Deployment Automation in DevOps
Deployment automation is a critical aspect of DevOps automation, as it helps streamline the process of deploying software applications to various environments. Automation tools enable developers to define and execute deployment pipelines, reducing the risk of human error and ensuring consistent deployments.
One popular deployment automation tool is AWS CodeDeploy, which allows developers to automate the deployment of applications to Amazon EC2 instances, on-premises servers, or Lambda functions. Here's an example of an AWS CodeDeploy deployment configuration:
version: 1 application: my-app deployment_groups: - name: production auto_scaling_groups: - name: my-auto-scaling-group deployment_config_name: CodeDeployDefault.OneAtATime deployment_target: production_tag: true
In this example, the deployment configuration specifies the application name, deployment group, and the auto-scaling group to target. It also specifies the deployment configuration to use and that the deployment should target instances tagged as 'production'.
Another popular deployment automation tool is Jenkins, which can be used to define and execute complex deployment pipelines. Here's an example of a Jenkins pipeline script that deploys a Java application to a Tomcat server:
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean package' } } stage('Deploy') { steps { sh 'cp target/my-app.war /path/to/tomcat/webapps' } } stage('Restart Tomcat') { steps { sh 'service tomcat restart' } } } }
In this example, the pipeline consists of three stages: build, deploy, and restart Tomcat. The build stage uses Maven to package the application, the deploy stage copies the resulting WAR file to the Tomcat webapps directory, and the restart Tomcat stage restarts the Tomcat service.
These examples demonstrate how deployment automation tools enable developers to define and execute deployment processes, reducing the time and effort required for manual deployments.
Role of Release Management in DevOps Automation
Release management is a critical aspect of DevOps automation, as it helps coordinate and manage the release of software applications to different environments. Automation tools enable teams to define and enforce release processes, ensuring that releases are consistent and predictable.
One popular release management tool is Octopus Deploy, which allows developers to automate the deployment of applications to various environments, including on-premises servers, cloud platforms, and containers. Here's an example of a release process defined in Octopus Deploy:
name: My Application Release triggers: - schedule: cron: '0 0 1 * *' channels: - name: Production lifecycle: Production tenant_tags: - production - name: Staging lifecycle: Staging tenant_tags: - staging steps: - name: Deploy to Production deployment_process: steps: - name: Deploy Web Application script: source: ./deploy-web-application.sh
In this example, the release process is triggered by a scheduled cron expression, indicating that the release should occur on the first day of each month. The process includes two channels, one for production and one for staging, each with its own lifecycle and tenant