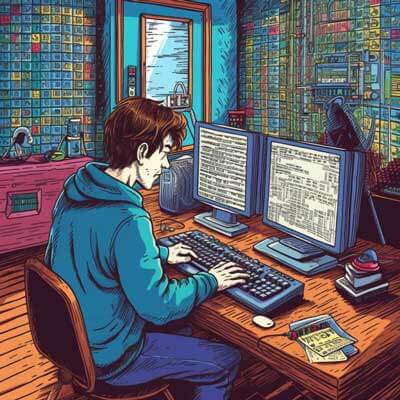
- Getting Started with Terraform on Google Cloud
- main.tf
- Understanding the Basics of Terraform
- Common Use Cases for Terraform on Google Cloud
- Provisioning Cloud Resources
- Managing Network Infrastructure
- Deploying Applications
- Continuous Integration and Continuous Deployment (CI/CD)
- Creating and Managing Resources with Terraform
- Initializing a Terraform Project
- Defining Resources
- Applying Terraform Changes
- Managing Terraform State
- Destroying Resources
- Working with Variables and Data Types
- Declaring Variables
- Assigning Values to Variables
- Using Variables in Configuration
- Data Types
- Using Modules to Organize and Reuse Terraform Code
- What are Modules?
- Benefits of Using Modules
- Creating and Using Modules
- Module Composition
- Implementing Dependencies and Resource Ordering
- Implicit Dependencies
- Explicit Dependencies
- Resource Ordering
- Working with Remote State Configuration
- Managing Secrets and Sensitive Data
- Using Google Cloud Secret Manager
- Encrypting Sensitive Data with Google Cloud KMS
- Using Terraform Workspaces for Isolation
- Using Functions and Expressions in Terraform
- Mathematical Functions
- String Functions
- Conditional Expressions
- Implementing Loops and Conditionals in Terraform
- Loops
- Conditionals
- Managing Terraform State and Backends
- Working with Terraform Providers and Providers Configuration
- Adding a Provider
- Provider Configuration
- Provider Aliases
- Provider Overrides
- Third-Party Providers
- Deploying and Managing Infrastructure as Code in Real-world Scenarios
- Organizing Your Terraform Code
- Managing Secrets and Sensitive Data
- Version Control and Collaboration
- Handling State Management
- Monitoring and Observability
- Advanced Techniques for Terraform on Google Cloud
- Using Terraform Modules
- Using Terraform Workspaces
- Using Terraform Backends
- Using Terraform Providers
- Optimizing Terraform Performance and Efficiency
- Troubleshooting and Debugging Terraform Deployments
- Understanding Error Messages
- Using Terraform Commands to Debug
- Using Logging and Monitoring
- Interacting with the Google Cloud Platform (GCP) API
- Seeking Help from the Community
- Monitoring and Auditing Terraform Deployments
- Monitoring with Stackdriver
- Logging and Auditing with Cloud Audit Logs
- Security Monitoring with Security Command Center
- Continuous Monitoring and Automation with Cloud Functions
- Automating Terraform Deployments with CI/CD Pipelines
- Securing Terraform Deployments in Production Environments
- Limiting Access to Terraform State
- Securely Storing Sensitive Data
- Enable Audit Logging
- Regularly Review and Update Access Controls
- Implement Infrastructure as Code Best Practices
- Scaling and Managing Large-scale Infrastructure with Terraform
- Modularize Your Code
- Use Terraform Workspaces
- Leverage Terraform State
- Implement Version Control
- Utilize Terraform Modules from the Community
Getting Started with Terraform on Google Cloud
Terraform is a powerful tool for managing infrastructure as code, and it integrates seamlessly with Google Cloud. We will guide you through the process of getting started with Terraform on Google Cloud.
Step 1: Install Terraform
Before you can start using Terraform, you need to install it on your local machine. You can download the latest version of Terraform from the official website: https://www.terraform.io/downloads.html. Once downloaded, follow the installation instructions for your operating system.
Step 2: Set Up Google Cloud Credentials
To interact with Google Cloud using Terraform, you need to set up your Google Cloud credentials. Follow these steps to do so:
1. Create a new project in the Google Cloud Console.
2. Enable the necessary APIs for the services you plan to use.
3. Generate a service account key for your project.
4. Download the JSON key file and store it in a safe location on your local machine.
Step 3: Initialize a Terraform Configuration
Once you have Terraform installed and your Google Cloud credentials set up, you are ready to initialize a Terraform configuration. A Terraform configuration is a set of files that describe the infrastructure you want to create.
Create a new directory for your Terraform configuration and navigate into it using your command-line interface. Then, create a new file named main.tf
and open it in a text editor. This file will contain your Terraform code.
Related Article: Quick and Easy Terraform Code Snippets
main.tf
provider "google" { credentials = file("") project = "" region = "" } resource "google_compute_instance" "my_instance" { name = "my-instance" machine_type = "n1-standard-1" zone = "" tags = ["http-server"] boot_disk { initialize_params { image = "debian-cloud/debian-9" } } network_interface { network = "default" access_config { // Ephemeral IP } } }
Make sure to replace ,
,
, and
with the appropriate values.
Step 4: Apply the Terraform Configuration
To apply the Terraform configuration and create the infrastructure, run the following command in your terminal:
terraform init
This command initializes the Terraform working directory.
terraform apply
This command creates or updates the infrastructure according to the Terraform configuration.
Terraform will display a plan of the changes it will make. Review the plan and confirm by typing “yes” when prompted. Terraform will then provision the resources specified in your configuration.
Step 5: Verify the Infrastructure
Once the Terraform apply command completes successfully, you can verify that the infrastructure was created by navigating to the Google Cloud Console. You should see the resources specified in your Terraform configuration.
Congratulations! You have successfully started your Terraform journey on Google Cloud. You can now leverage the power of Terraform to manage your infrastructure as code.
Understanding the Basics of Terraform
Terraform is an open-source infrastructure as code (IaC) tool that allows you to define and manage your infrastructure in a declarative manner. It provides a simple and efficient way to provision and manage resources across various cloud providers, including Google Cloud.
With Terraform, you define your infrastructure using a high-level configuration language called HashiCorp Configuration Language (HCL). This language allows you to express the desired state of your infrastructure, including resources such as virtual machines, networks, storage, and more.
The Terraform configuration files are typically organized into modules, which are reusable components that encapsulate a set of resources and their dependencies. These modules can be shared and reused across different projects, enabling you to build infrastructure as code in a modular and scalable way.
To get started with Terraform on Google Cloud, you need to have the following prerequisites:
1. Install Terraform: You can download and install Terraform from the official website (https://www.terraform.io/downloads.html) based on your operating system.
2. Create a Google Cloud project: You will need a Google Cloud project to deploy your infrastructure. If you don’t have a project yet, you can create one using the Google Cloud Console (https://console.cloud.google.com/).
3. Set up authentication: Terraform needs credentials to access your Google Cloud project. You can create a service account and generate a key file (JSON format) using the Google Cloud Console. Make sure to grant the necessary roles and permissions to the service account.
Once you have the prerequisites in place, you can start writing your Terraform configuration files. A basic Terraform configuration file is named main.tf
and typically contains the definition of the resources you want to provision. Here’s an example of a simple configuration file that provisions a virtual machine on Google Cloud:
provider "google" { credentials = file("path/to/keyfile.json") project = "your-project-id" region = "us-central1" } resource "google_compute_instance" "vm_instance" { name = "my-vm" machine_type = "n1-standard-2" zone = "us-central1-a" boot_disk { initialize_params { image = "debian-cloud/debian-10" } } network_interface { network = "default" access_config { } } }
In this example, we specify the google
provider to authenticate and interact with Google Cloud. We set the credentials file path, project ID, and region. Then, we define a google_compute_instance
resource named vm_instance
that represents a virtual machine. We specify the desired properties such as name, machine type, zone, boot disk, and network configuration.
To apply this configuration and provision the resources, you need to run the following commands in the terminal:
terraform init terraform apply
The terraform init
command initializes the working directory and downloads the necessary provider plugins. The terraform apply
command creates or updates the resources defined in your configuration file based on the desired state.
Terraform keeps track of the state of your infrastructure in a state file (by default named terraform.tfstate
). This file is used to map the resources you defined in your configuration to the actual resources provisioned in your cloud provider. It is important to keep this file secure and versioned.
We covered the basics of Terraform and how to get started with Google Cloud. We learned about the Terraform configuration files, modules, and the process of provisioning resources. We will explore more advanced features and best practices for using Terraform on Google Cloud.
Common Use Cases for Terraform on Google Cloud
Terraform is a powerful infrastructure as code tool that allows you to define and manage your infrastructure in a declarative manner. When it comes to using Terraform on Google Cloud, there are several common use cases that can benefit from its capabilities. We will explore some of these use cases and provide examples to demonstrate how Terraform can be leveraged effectively.
Related Article: Terraform Advanced Tips for AWS
Provisioning Cloud Resources
One of the primary use cases for Terraform on Google Cloud is provisioning cloud resources. With Terraform, you can define the desired state of your infrastructure using code and then apply that code to create and manage your resources in a consistent and reproducible manner.
For example, let’s say you want to provision a Google Compute Engine instance. You can define the necessary configuration in a Terraform file, specifying properties like machine type, image, network, and disk. Here’s an example of how this can be done in a Terraform configuration file (main.tf) using the Google Cloud provider:
provider "google" { project = "your-project-id" region = "us-central1" } resource "google_compute_instance" "example" { name = "example-instance" machine_type = "n1-standard-1" zone = "us-central1-a" boot_disk { initialize_params { image = "debian-cloud/debian-10" } } network_interface { network = "default" access_config {} } }
By running terraform apply
, Terraform will create the Compute Engine instance based on the defined configuration.
Managing Network Infrastructure
Another common use case for Terraform on Google Cloud is managing network infrastructure. With Terraform, you can define and manage networks, subnets, firewall rules, and load balancers, among other resources.
For example, let’s say you want to create a VPC network with a subnet and firewall rules. You can define the configuration in a Terraform file, like the following example:
resource "google_compute_network" "vpc_network" { name = "my-vpc-network" auto_create_subnetworks = false } resource "google_compute_subnetwork" "subnet" { name = "my-subnet" ip_cidr_range = "10.0.0.0/24" network = google_compute_network.vpc_network.self_link region = "us-central1" } resource "google_compute_firewall" "firewall" { name = "allow-http" network = google_compute_network.vpc_network.self_link allow { protocol = "tcp" ports = ["80"] } source_ranges = ["0.0.0.0/0"] }
By running terraform apply
, Terraform will create the VPC network, subnet, and firewall rules as specified in the configuration.
Deploying Applications
Terraform can also be used to deploy applications on Google Cloud. By combining Terraform with other tools like Kubernetes or Cloud Functions, you can automate the deployment process and manage the underlying infrastructure as well.
For example, you can define a Kubernetes cluster using Terraform and then use other tools like Helm or Kustomize to deploy your applications onto the cluster. Here’s an example of how you can define a Kubernetes cluster in Terraform using the Google Cloud provider:
resource "google_container_cluster" "cluster" { name = "my-cluster" location = "us-central1" initial_node_count = 3 master_auth { username = "" password = "" } } output "kubeconfig" { value = google_container_cluster.cluster.master_auth[0].cluster_ca_certificate }
By running terraform apply
, Terraform will create the Kubernetes cluster, and you can then use the generated kubeconfig output to interact with the cluster and deploy your applications.
Related Article: Terraform Tutorial & Advanced Tips
Continuous Integration and Continuous Deployment (CI/CD)
Terraform can play a crucial role in CI/CD pipelines by automating the provisioning and management of infrastructure resources as part of the deployment process. By integrating Terraform with tools like Jenkins, GitLab CI/CD, or CircleCI, you can ensure that your infrastructure is always up-to-date and in sync with your application code.
For example, you can define a pipeline that triggers a Terraform run whenever there are changes to your infrastructure code. This can include creating or updating resources, as well as destroying resources that are no longer needed. By automating these steps, you can ensure that your infrastructure is always in the desired state and reduce the risk of manual errors.
Creating and Managing Resources with Terraform
Terraform is a powerful tool that allows you to create and manage resources on Google Cloud Platform (GCP) using infrastructure as code. We will explore how to create and manage resources with Terraform, providing you with advanced tips to streamline your workflow.
Initializing a Terraform Project
Before you can start creating resources with Terraform, you need to initialize a Terraform project in your working directory. This is done by running the following command:
terraform init
This command will download and install the necessary provider plugins and set up your project to use Terraform. Make sure you have the appropriate credentials and permissions to access the desired GCP project.
Defining Resources
To create resources with Terraform, you need to define them in a Terraform configuration file. This file typically has a .tf
extension and follows a declarative syntax. Here’s an example of how to define a Google Compute Engine instance:
resource "google_compute_instance" "my_instance" { name = "example-instance" machine_type = "n1-standard-1" zone = "us-central1-a" boot_disk { initialize_params { image = "debian-cloud/debian-9" } } network_interface { network = "default" access_config { } } }
In this example, we define a Google Compute Engine instance with a specific name, machine type, and zone. We also specify a boot disk and a network interface. You can customize these settings to fit your needs.
Applying Terraform Changes
Once you have defined your resources, you can apply the changes to create them in your GCP project. Use the following command to apply the changes:
terraform apply
Terraform will compare the desired state defined in your Terraform configuration file with the current state of your GCP project and make the necessary changes to align them. It will prompt you for confirmation before making any changes.
Managing Terraform State
Terraform keeps track of the state of your infrastructure in a state file. This file is essential for Terraform to manage and update your resources correctly. By default, Terraform stores the state file locally, but it is recommended to use a remote backend for better collaboration and reliability.
You can configure a remote backend by adding the following block in your Terraform configuration file:
terraform { backend "gcs" { bucket = "my-terraform-state-bucket" prefix = "terraform/state" } }
In this example, we configure a Google Cloud Storage (GCS) backend to store the state file in a bucket named “my-terraform-state-bucket”. This ensures that the state is stored remotely and can be accessed by the entire team.
Destroying Resources
When you no longer need a resource or want to clean up your GCP project, you can destroy the resources created by Terraform. Use the following command to destroy the resources:
terraform destroy
Terraform will identify the resources created in your GCP project and remove them. It will prompt you for confirmation before destroying any resources. Be cautious when using this command, as it permanently deletes the resources.
Working with Variables and Data Types
One of the key features of Terraform is its ability to use variables and data types to make your infrastructure code more flexible and reusable. We will explore how to work with variables and different data types in Terraform on Google Cloud.
Declaring Variables
To declare a variable in Terraform, you can use the variable
block. Variables can be defined in a separate file, conventionally named variables.tf
, or directly in the main Terraform configuration file. Here’s an example of declaring a variable in a separate file:
# variables.tf variable "project_id" { description = "The ID of the Google Cloud project." type = string }
In the above example, we have declared a variable named project_id
of type string
with an optional description. This variable can now be used throughout the Terraform configuration.
Assigning Values to Variables
To assign a value to a variable, you can use the terraform.tfvars
file or pass the values using the command-line flags. The terraform.tfvars
file is commonly used to store variable values, and it should be git-ignored to avoid committing sensitive information. Here’s an example of assigning a value to the project_id
variable in terraform.tfvars
:
# terraform.tfvars project_id = "my-gcp-project"
Alternatively, you can pass the variable values using command-line flags when running Terraform commands:
terraform apply -var="project_id=my-gcp-project"
Using Variables in Configuration
Once you have declared and assigned values to your variables, you can use them in your Terraform configuration. To reference a variable, use the var
keyword followed by the variable name. Here’s an example of using the project_id
variable in a Google Cloud resource block:
# main.tf resource "google_compute_instance" "my_instance" { name = "my-instance" machine_type = "n1-standard-1" zone = "us-central1-a" project = var.project_id }
In the above example, the project
attribute of the google_compute_instance
resource is set to the value of the project_id
variable.
Data Types
Terraform supports several data types, including strings, numbers, lists, maps, and booleans. You can specify the data type for a variable using the type
argument in the variable
block. Here are a few examples:
variable "region" { type = string } variable "instance_count" { type = number } variable "tags" { type = list(string) } variable "metadata" { type = map(string) } variable "enable_monitoring" { type = bool }
In the above examples, we have declared variables with different data types. These variables can then be used to define and configure resources in your Terraform configuration.
Using Modules to Organize and Reuse Terraform Code
When working with Terraform on Google Cloud, it is crucial to have a well-organized codebase that is easy to maintain and reuse. One way to achieve this is by using modules. We will explore how to use modules effectively in your Terraform projects.
What are Modules?
Modules in Terraform are self-contained packages of Terraform configurations that can be used to create and manage infrastructure resources. They allow you to encapsulate a set of resources with defined inputs and outputs, making it easier to work with and reuse code.
Modules can be created for various purposes, such as provisioning a specific resource or a group of related resources. They can also be shared and reused across different projects or teams.
Benefits of Using Modules
Using modules in your Terraform code offers several benefits:
1. Reusability: Modules can be shared and reused across different projects, enabling you to standardize your infrastructure provisioning while reducing duplication of code.
2. Abstraction: Modules abstract away the complexity of underlying resources, allowing you to focus on the high-level architecture of your infrastructure.
3. Modularity: Modules offer a modular approach to designing your infrastructure, making it easier to manage and update individual components without impacting the entire system.
4. Testing: Modules can be independently tested, ensuring their correctness and reliability before being used in production environments.
Creating and Using Modules
To create a module, you need to define a folder structure and files that contain your Terraform code. The structure typically includes a main.tf file that defines the resources, variables.tf to specify input variables, and outputs.tf to define the outputs of the module.
Let’s take an example of a module for provisioning a Google Cloud Storage bucket. Here’s how the folder structure might look:
gcs-bucket/ ├── main.tf ├── variables.tf └── outputs.tf
In the main.tf file, you would define the necessary resources for creating the storage bucket:
# main.tf resource "google_storage_bucket" "bucket" { name = var.bucket_name location = var.bucket_location # Additional configuration options... }
In the variables.tf file, you can define the input variables required for the module:
# variables.tf variable "bucket_name" { description = "The name of the storage bucket." } variable "bucket_location" { description = "The location of the storage bucket." default = "us-central1" }
And in the outputs.tf file, you can define the outputs that the module provides:
# outputs.tf output "bucket_name" { value = google_storage_bucket.bucket.name } output "bucket_url" { value = google_storage_bucket.bucket.url }
Once you have created the module, you can use it in your Terraform code by referencing its source. For example:
# main.tf module "my_gcs_bucket" { source = "./modules/gcs-bucket" bucket_name = "my-bucket" bucket_location = "us-west1" }
By using the module, you can easily provision a Google Cloud Storage bucket with the specified configuration.
Module Composition
Modules can be composed together to build more complex infrastructure. You can use the outputs of one module as inputs to another module, creating a hierarchical structure.
For example, if you have a module for provisioning a virtual machine and another module for setting up a load balancer, you can combine them by passing the output of the virtual machine module into the load balancer module:
# main.tf module "my_vm" { source = "./modules/vm" # VM module configuration... } module "my_lb" { source = "./modules/lb" vm_ip = module.my_vm.vm_ip # Load balancer module configuration... }
This composition approach allows you to create more complex infrastructure setups while maintaining modularity and reusability.
Implementing Dependencies and Resource Ordering
When working with Terraform on Google Cloud, it is crucial to define dependencies and resource ordering correctly to ensure that resources are provisioned in the right order and meet any dependencies they have on each other. This article will explore various techniques for implementing dependencies and resource ordering in your Terraform code.
Implicit Dependencies
Terraform automatically detects dependencies between resources based on their configuration. If one resource references another in its configuration, Terraform will understand that there is a dependency and provision the resources accordingly.
For example, consider a scenario where you want to create a Compute Engine instance and attach a persistent disk to it. You can achieve this by using the google_compute_instance
and google_compute_disk
resources. The instance resource references the disk resource in its configuration:
resource "google_compute_instance" "my_instance" { name = "my-instance" machine_type = "n1-standard-1" zone = "us-central1-a" boot_disk { initialize_params { image = "debian-cloud/debian-10" } } # Reference to the disk resource disk { source = google_compute_disk.my_disk.self_link } } resource "google_compute_disk" "my_disk" { name = "my-disk" type = "pd-ssd" size = 100 zone = "us-central1-a" }
In this example, Terraform will automatically understand that the instance resource depends on the disk resource because of the disk
block. When you run terraform apply
, Terraform will first create the disk resource and then create the instance resource.
Explicit Dependencies
In some cases, you may need to specify dependencies explicitly, especially when there are no direct references between resources. You can use the depends_on
argument to define explicit dependencies between resources.
For example, let’s say you have a scenario where you want to create a Cloud Storage bucket and a Cloud Functions function. The function needs to be deployed after the bucket is created. You can achieve this by using the google_storage_bucket
and google_cloudfunctions_function
resources. To specify the dependency explicitly, you can use the depends_on
argument:
resource "google_storage_bucket" "my_bucket" { name = "my-bucket" } resource "google_cloudfunctions_function" "my_function" { name = "my-function" runtime = "nodejs14" source_archive_bucket = google_storage_bucket.my_bucket.name source_archive_object = "my-function.zip" depends_on = [google_storage_bucket.my_bucket] }
In this example, the depends_on
argument ensures that the function resource is created only after the bucket resource is created. Terraform will provision the resources accordingly.
Resource Ordering
Resource ordering allows you to control the order in which resources are provisioned, even when there are no explicit dependencies between them. Terraform provides the depends_on
meta-argument to define resource ordering.
For example, let’s say you have a scenario where you want to create a virtual private cloud (VPC) network and a Cloud NAT gateway. The NAT gateway depends on the VPC network being created first. You can use the depends_on
meta-argument to enforce the order:
resource "google_compute_network" "my_network" { name = "my-network" auto_create_subnetworks = false } resource "google_compute_router" "my_router" { name = "my-router" network = google_compute_network.my_network.self_link } resource "google_compute_router_nat" "my_nat" { name = "my-nat" router = google_compute_router.my_router.name nat_ip_allocate_option = "AUTO_ONLY" depends_on = [google_compute_network.my_network] }
In this example, the depends_on
meta-argument ensures that the NAT gateway resource is created only after the VPC network resource is created, even though there is no direct reference between them. Terraform will provision the resources in the desired order.
Implementing dependencies and resource ordering correctly is essential for managing the lifecycle of your resources on Google Cloud using Terraform. Whether it’s through implicit dependencies, explicit dependencies, or resource ordering, Terraform provides flexible options to ensure that your resources are provisioned in the required sequence.
Working with Remote State Configuration
As your infrastructure grows and becomes more complex, managing the state of your Terraform deployments becomes increasingly important. Terraform uses a local state file to track the resources it manages. However, this approach can become problematic in a collaborative or shared environment, where multiple team members may be working on the same infrastructure.
To overcome these challenges, Terraform provides a feature called Remote State Configuration. With this feature, you can store the state file in a remote backend, such as Google Cloud Storage, Amazon S3, or HashiCorp’s Terraform Cloud. Storing the state remotely allows for easier collaboration, better security, and better management of the state file.
To configure a remote state in Terraform, you need to modify your Terraform configuration files to specify the backend and its configuration. Here’s an example of configuring a Google Cloud Storage backend:
terraform { backend "gcs" { bucket = "my-state-bucket" prefix = "terraform/state" } }
In this example, we are using Google Cloud Storage as the backend and specifying a bucket named “my-state-bucket” to store the state file. The prefix “terraform/state” is used to organize the state files within the bucket.
Once you have configured the backend, you can initialize Terraform to migrate the local state to the remote backend:
terraform init
Terraform will prompt you to confirm the migration, and if you agree, it will copy the existing state file to the remote backend. From this point forward, Terraform will use the remote state for all future operations.
Working with remote state offers several benefits. First, it allows multiple team members to collaborate on the same infrastructure without conflicts. Each team member can pull the latest state from the remote backend, make changes, and push the updated state back to the backend. This eliminates the need for manual coordination or merging of state files.
Second, remote state provides better security and access control. You can manage access to the remote backend using IAM policies or other access control mechanisms provided by the backend service. This ensures that only authorized individuals can modify the infrastructure state.
Third, remote state enables better management and visibility of your infrastructure. You can easily query the state file stored in the remote backend to get information about the deployed resources. This can be useful for auditing, troubleshooting, or generating reports about your infrastructure.
Managing Secrets and Sensitive Data
When working with infrastructure-as-code tools like Terraform, it’s important to handle secrets and sensitive data securely. This article will cover some advanced tips for managing secrets and sensitive data when using Terraform on Google Cloud.
Using Google Cloud Secret Manager
Google Cloud Secret Manager is a secure and convenient way to store secrets such as API keys, passwords, and certificates. It provides a central place to manage and control access to secrets, and integrates well with Terraform.
To use Secret Manager with Terraform, you can create a secret, store the value securely, and then reference it in your Terraform code. Here’s an example of how you can retrieve a secret from Secret Manager and use it in a Terraform resource:
# main.tf data "google_secret_manager_secret_version" "my_secret" { secret = "projects/my-project/secrets/my-secret/versions/latest" } resource "google_compute_instance" "my_instance" { # ... metadata = { "my-secret" = data.google_secret_manager_secret_version.my_secret.secret_data } # ... }
In this example, we’re retrieving the latest version of a secret called “my-secret” stored in Secret Manager and using it as metadata for a Compute Engine instance.
Encrypting Sensitive Data with Google Cloud KMS
Google Cloud Key Management Service (KMS) allows you to encrypt and decrypt sensitive data using encryption keys managed by Google. You can use KMS to encrypt data in Terraform variables or in files stored in Google Cloud Storage.
To encrypt sensitive data in Terraform variables with KMS, you can use the google_kms_crypto_key
resource to create a key, and then use the google_kms_crypto_key_version
resource to encrypt the data. Here’s an example:
# main.tf resource "google_kms_crypto_key" "my_key" { name = "my-key" location = "global" } resource "google_kms_crypto_key_version" "my_key_version" { crypto_key_id = google_kms_crypto_key.my_key.id algorithm = "GOOGLE_SYMMETRIC_ENCRYPTION" } resource "google_compute_instance" "my_instance" { # ... metadata = { "my-secret" = google_kms_crypto_key_version.my_key_version.encrypt("my-sensitive-data") } # ... }
In this example, we’re creating a KMS key and a key version, and then encrypting the sensitive data “my-sensitive-data” using the key version. The encrypted data can be used as metadata for a Compute Engine instance.
Using Terraform Workspaces for Isolation
Terraform workspaces provide a way to isolate resources and state files for different environments, such as development, staging, and production. This can be useful for managing secrets and sensitive data because it allows you to have separate secrets for each workspace.
You can create a workspace by running the command terraform workspace new
. Once you’ve created a workspace, you can switch to it using terraform workspace select
. Each workspace has its own set of variables and state file, allowing you to manage secrets and sensitive data separately for each environment.
$ terraform workspace new dev $ terraform workspace select dev
In this example, we’re creating a new workspace called “dev” and then switching to it. You can then define and manage secrets specific to the “dev” environment.
Using Functions and Expressions in Terraform
Terraform provides a powerful set of functions and expressions that allow you to manipulate and transform data within your infrastructure code. These functions can be used to perform calculations, modify strings, generate random values, and much more. We will explore some advanced tips for using functions and expressions in Terraform on Google Cloud.
Mathematical Functions
Terraform provides a range of mathematical functions that can be used to perform calculations within your infrastructure code. These functions include basic arithmetic operations like addition, subtraction, multiplication, and division, as well as more advanced functions like exponentiation, rounding, and taking the absolute value of a number.
Here is an example that demonstrates the use of mathematical functions in Terraform:
variable "num1" { type = number default = 10 } variable "num2" { type = number default = 5 } output "sum" { value = num1 + num2 } output "absolute_value" { value = abs(num1 - num2) }
In the above example, we define two variables, num1
and num2
, with default values of 10 and 5 respectively. We then use the +
operator to calculate the sum of num1
and num2
, and the abs
function to calculate the absolute value of the difference between num1
and num2
. The results are then printed as outputs.
String Functions
Terraform also provides a variety of string functions that can be used to manipulate and transform strings within your infrastructure code. These functions include operations like concatenation, substring extraction, string length calculation, and case conversion.
Here is an example that demonstrates the use of string functions in Terraform:
variable "name" { type = string default = "John Doe" } output "upper_case" { value = upper(var.name) } output "substring" { value = substr(var.name, 0, 4) }
In the above example, we define a variable name
with a default value of “John Doe”. We then use the upper
function to convert the value of name
to uppercase, and the substr
function to extract the first four characters from name
. The results are then printed as outputs.
Conditional Expressions
Terraform allows you to use conditional expressions to control the flow of your infrastructure code based on certain conditions. These expressions can be used to conditionally assign values to variables, resources, or outputs, based on the result of a condition.
Here is an example that demonstrates the use of conditional expressions in Terraform:
variable "environment" { type = string default = "production" } variable "instance_type" { type = string default = "n1-standard-1" } resource "google_compute_instance" "instance" { name = "my-instance" machine_type = var.environment == "production" ? "n1-highmem-4" : var.instance_type zone = "us-central1-a" }
In the above example, we define two variables, environment
and instance_type
, with default values of “production” and “n1-standard-1” respectively. We then use a conditional expression to assign a different value to the machine_type
property of the google_compute_instance
resource based on the value of the environment
variable. If the environment
variable is set to “production”, the machine_type
is set to “n1-highmem-4”, otherwise it is set to the value of the instance_type
variable. This allows us to selectively choose a different machine type for our instances based on the environment.
Implementing Loops and Conditionals in Terraform
When working with Terraform on Google Cloud, you’ll often need to handle complex scenarios that require loops and conditionals. Fortunately, Terraform provides several mechanisms to implement these features effectively.
Loops
Loops allow you to repeat a set of actions or configurations multiple times. This is particularly useful when you need to create multiple resources of the same type or apply a similar configuration to multiple instances. Terraform supports loops through the use of the count
and for_each
meta-arguments.
The count
meta-argument allows you to specify the number of resource instances you want to create. For example, let’s say you want to create three Google Compute Engine instances:
resource "google_compute_instance" "example" { count = 3 # ... instance configuration ... }
In this example, Terraform will create three instances, and each instance will have its own unique index. You can reference these instances using the index, like google_compute_instance.example[0]
, google_compute_instance.example[1]
, and google_compute_instance.example[2]
.
On the other hand, the for_each
meta-argument allows you to iterate over a collection, such as a map or set, to create multiple resource instances. For instance, suppose you have a map that defines the configuration for multiple Google Cloud Storage buckets:
variable "buckets" { type = map(object({ location = string # ... other attributes ... })) } resource "google_storage_bucket" "example" { for_each = var.buckets location = each.value.location # ... bucket configuration ... }
In this case, Terraform will create a bucket for each item in the var.buckets
map. You can reference these instances using their unique keys, like google_storage_bucket.example["bucket1"]
, google_storage_bucket.example["bucket2"]
, and so on.
Conditionals
Conditionals allow you to make decisions based on certain conditions. Terraform provides the if
and for
expressions to implement conditionals effectively.
The if
expression allows you to conditionally include or exclude a resource or a block of configuration based on a condition. For example, let’s say you want to create a Google Cloud Pub/Sub topic only in a specific environment:
resource "google_pubsub_topic" "example" { if = var.environment == "production" # ... topic configuration ... }
In this case, the google_pubsub_topic.example
resource will be created only if the var.environment
variable is set to “production”.
The for
expression allows you to iterate over a collection and filter the elements based on a condition. For instance, suppose you have a list of Google Cloud Storage buckets, and you want to create a firewall rule only for buckets with a specific attribute:
variable "buckets" { type = list(object({ name = string firewall = bool # ... other attributes ... })) } resource "google_compute_firewall" "example" { for_each = { for b in var.buckets : b if b.firewall } # ... firewall rule configuration ... }
In this example, Terraform will create a firewall rule for each bucket in the var.buckets
list that has the firewall
attribute set to true
.
Implementing loops and conditionals in Terraform allows you to handle complex scenarios and automate your infrastructure provisioning effectively. Whether you need to create multiple resources or make decisions based on conditions, Terraform provides the necessary tools to accomplish these tasks efficiently.
Managing Terraform State and Backends
One of the key aspects of using Terraform on Google Cloud is managing the Terraform state. Terraform state is a representation of your infrastructure in a JSON format, and it keeps track of the resources created and managed by Terraform. Managing the state file is crucial because it allows Terraform to understand the current state of your infrastructure and make changes accordingly.
By default, Terraform stores the state locally in a file named terraform.tfstate
. However, when working in a team or across multiple machines, it’s important to use a remote backend to store and share the state file. A remote backend enables collaboration, consistency, and ensures that everyone is working with the same state, avoiding conflicts and ensuring that infrastructure changes are tracked.
Google Cloud Storage is a popular choice for a remote backend on Google Cloud. It provides a highly available and scalable storage solution for your state files. To configure Terraform to use Google Cloud Storage as a backend, you need to create a bucket to store the state file. You can do this manually through the Google Cloud Console or use the following Terraform code:
terraform { backend "gcs" { bucket = "my-terraform-state-bucket" prefix = "terraform/state" } }
In the example above, we configure the Google Cloud Storage backend with a bucket named “my-terraform-state-bucket” and a prefix of “terraform/state”. The prefix is a directory-like structure within the bucket to organize your state files. You can customize these values to match your requirements.
Once you have configured the backend, you can initialize Terraform by running terraform init
. This command downloads the necessary providers and sets up the remote backend. After initialization, Terraform will start using the remote backend to store the state file.
Using a remote backend offers several advantages. It allows you to easily share the state file with your team, enabling collaboration and avoiding conflicts. It also provides a backup of your state file, protecting it from accidental deletion or corruption. Additionally, it enables Terraform’s remote operations, such as remote plan and remote apply, which can be useful for automating infrastructure changes.
Working with Terraform Providers and Providers Configuration
Terraform providers are plugins that enable Terraform to interact with different cloud platforms, APIs, or services. We’ll explore how to work with Terraform providers and configure them for use in your Google Cloud projects.
Adding a Provider
To add a provider to your Terraform project, you need to declare it in your Terraform configuration file. The provider block specifies the provider’s name and version. For example, to use the Google Cloud provider:
terraform { required_providers { google = { source = "hashicorp/google" version = "3.5.0" } } } provider "google" { credentials = file("path/to/credentials.json") project = "your-project-id" region = "us-central1" }
In the example above, we declare the Google Cloud provider with version 3.5.0. We also specify the path to the credentials file, the project ID, and the region to use.
Provider Configuration
Provider configuration allows you to customize the behavior of the provider. For example, you can specify the region, project ID, or credentials for a provider. These configurations are typically specified in the provider block.
In the Google Cloud provider example above, we set the project and region in the provider block. However, there are additional configuration options available for each provider. You can refer to the provider’s documentation for a complete list of configuration options.
Provider Aliases
In some cases, you may need to use multiple instances of the same provider in your Terraform configuration. For example, if you are managing resources in multiple Google Cloud projects, you can use provider aliases to differentiate them.
provider "google" { credentials = file("path/to/credentials1.json") project = "project-id-1" region = "us-central1" } provider "google" { alias = "project2" credentials = file("path/to/credentials2.json") project = "project-id-2" region = "us-east1" }
In the example above, we define two instances of the Google Cloud provider. The second instance is given an alias, “project2”. This allows us to reference specific providers in our resource blocks.
Provider Overrides
Provider overrides are useful when you need to customize provider configurations for specific resources or modules within your Terraform configuration. You can override any configuration option for a specific resource or module.
resource "google_compute_instance" "my_instance" { provider = google.project2 # Resource configuration... }
In the example above, we override the provider for a specific resource, google_compute_instance
, by specifying provider = google.project2
. This allows us to use a different provider configuration for that resource.
Third-Party Providers
In addition to the built-in providers, Terraform supports third-party providers, which are created by the community. These providers enable you to manage resources in other cloud platforms or services.
You can install third-party providers using the terraform init
command, just like you would with built-in providers. The provider block for a third-party provider will specify the source and version.
terraform { required_providers { aws = { source = "hashicorp/aws" version = "3.57.0" } } } provider "aws" { region = "us-west-2" # AWS credentials configuration... }
In the example above, we declare the AWS provider with version 3.57.0. We also specify the region and any required credentials.
Deploying and Managing Infrastructure as Code in Real-world Scenarios
Infrastructure as Code (IaC) has revolutionized the way we manage and deploy infrastructure in the cloud. With Terraform, an open-source IaC tool, you can define and provision your infrastructure using declarative configuration files. We will explore some advanced tips for deploying and managing infrastructure as code in real-world scenarios on Google Cloud.
Organizing Your Terraform Code
As your infrastructure grows, it becomes crucial to organize your Terraform code in a structured and maintainable way. Here are a few recommendations to keep your codebase organized:
– Use a modular approach: Break down your infrastructure into reusable modules. This promotes code reusability and makes it easier to manage and maintain your codebase.
– Separate environments: Create separate directories or workspaces for each environment (e.g., development, staging, production). This allows you to manage and deploy infrastructure specific to each environment.
– Leverage Terraform workspaces: Terraform workspaces enable you to manage multiple states for different environments. This can be useful when you have multiple deployments running concurrently.
Managing Secrets and Sensitive Data
In real-world scenarios, you often need to manage sensitive information such as API keys, passwords, or certificates. Terraform provides several options for securely managing secrets:
– Use Terraform variables: Define sensitive data as variables and store them in separate variable files. Avoid committing these files to your version control system to prevent exposing sensitive information.
– Leverage Terraform Cloud or Vault: Terraform Cloud and HashiCorp Vault offer secure options for managing secrets. They provide encryption at rest and in-transit, access controls, and audit logs.
Version Control and Collaboration
Version control plays a crucial role in managing infrastructure code in real-world scenarios. Here are some best practices for version control and collaboration with Terraform:
– Use a version control system: Git is a popular choice for version control. Keep your Terraform codebase in a Git repository to track changes, collaborate with team members, and roll back to previous versions if needed.
– Leverage Git branching: Use feature branches for new changes and create pull requests for code reviews. This helps ensure that changes are properly reviewed and tested before merging into the main branch.
– Integrate with CI/CD pipelines: Integrate Terraform with your CI/CD pipelines to automate the testing and deployment of your infrastructure code. This helps maintain consistency and reduces manual errors.
Handling State Management
Terraform uses a state file to store information about your infrastructure. Proper state management is essential for maintaining the desired state of your infrastructure. Here are a few tips for handling state management:
– Enable remote state storage: Store your Terraform state file in a remote location, such as Google Cloud Storage or an S3 bucket. Remote state storage provides better collaboration, versioning, and security.
– Enable state locking: Enable state locking to prevent concurrent access to the state file. This ensures that only one person can make changes to the infrastructure at a time, reducing the risk of conflicts.
Monitoring and Observability
Monitoring and observability are crucial for managing infrastructure in real-world scenarios. Here are a few tips to enhance monitoring and observability with Terraform:
– Leverage Google Cloud Monitoring and Logging: Integrate your infrastructure with Google Cloud Monitoring and Logging to collect metrics, logs, and traces. Use these insights to monitor the health and performance of your infrastructure.
– Utilize Terraform providers: Terraform providers, such as the Google Cloud provider, often provide resource-specific monitoring and observability features. Take advantage of these features to gain deeper insights into your infrastructure.
Advanced Techniques for Terraform on Google Cloud
When working with Terraform on Google Cloud, there are several advanced techniques you can leverage to optimize your infrastructure provisioning and management. We will explore some of these techniques and how they can be applied.
Using Terraform Modules
Terraform modules allow you to encapsulate and reuse configurations, making it easier to manage and maintain your infrastructure code. By organizing your infrastructure into reusable modules, you can promote code reusability, reduce duplication, and improve collaboration among team members.
To create a module, you can define a separate Terraform configuration file (usually with a .tf extension) that represents a specific set of resources or functionality. This configuration file can then be called from other Terraform projects using the module block. Here’s an example of using a module to provision a Google Cloud Storage bucket:
module "storage_bucket" { source = "terraform-google-modules/storage-bucket/google" version = "~> 2.0" name = "my-bucket" location = "us-central1" storage_class = "REGIONAL" }
In this example, we’re using the official terraform-google-modules/storage-bucket/google
module to provision a storage bucket. The module takes parameters such as the bucket name, location, and storage class, and abstracts the underlying Terraform resources required to provision the bucket.
Using Terraform Workspaces
Terraform workspaces provide a way to create multiple instances of your infrastructure, each with its own state file. This can be useful when you need to manage different environments (e.g., development, staging, production) or when working on different feature branches.
To create a new workspace, you can use the terraform workspace new
command. For example, to create a workspace for a development environment, you can run:
terraform workspace new dev
Once you’ve created a workspace, you can switch between workspaces using the terraform workspace select
command. For example, to switch to the production workspace, you can run:
terraform workspace select prod
By using workspaces, you can manage the state of each environment separately, allowing you to make changes to one environment without affecting others. This helps minimize the risk of unintended changes and provides a better way to manage and organize your infrastructure code.
Using Terraform Backends
Terraform backends are responsible for storing the state of your infrastructure. By default, Terraform stores the state locally in a file named terraform.tfstate
. However, this can lead to issues when working in a team or when provisioning infrastructure from multiple machines.
To address this, you can configure a remote backend, such as Google Cloud Storage, to store the Terraform state remotely. This allows multiple team members to collaborate on the same infrastructure code and provides a more reliable and scalable way to manage state.
Here’s an example of configuring a Google Cloud Storage backend in your Terraform configuration:
terraform { backend "gcs" { bucket = "my-tfstate-bucket" prefix = "terraform/state" } }
In this example, we’re configuring the Google Cloud Storage backend with a bucket named my-tfstate-bucket
and a prefix of terraform/state
. Terraform will automatically store and retrieve the state from this remote location, ensuring that all team members are working with the latest state.
Using Terraform Providers
Terraform providers are responsible for interacting with various cloud providers and services. Google Cloud Platform has its own official provider, which allows you to provision and manage resources within Google Cloud.
To use the Google Cloud provider, you need to configure it in your Terraform configuration file. Here’s an example:
provider "google" { project = "my-project" region = "us-central1" }
In this example, we’re configuring the Google Cloud provider with the project ID and region. This allows Terraform to authenticate and interact with Google Cloud Platform using the provided credentials.
Using Terraform providers, you can provision and manage a wide range of resources within Google Cloud, such as Compute Engine instances, Cloud Storage buckets, and Cloud Functions.
By leveraging these advanced techniques, you can enhance your Terraform workflow on Google Cloud, making it more efficient, scalable, and collaborative.
Optimizing Terraform Performance and Efficiency
Terraform is a powerful infrastructure as code tool that allows you to define and manage your infrastructure in a declarative manner. However, as your infrastructure grows, you may encounter performance issues and inefficiencies. We will explore some advanced tips to help optimize the performance and efficiency of your Terraform deployments on Google Cloud.
1. Use Terraform Workspaces
Terraform workspaces allow you to manage multiple environments, such as development, staging, and production, within the same configuration. By isolating each environment in its own workspace, you can ensure that changes made in one environment do not affect others. This not only improves the efficiency of your deployments but also provides a safe way to test and iterate on your infrastructure changes.
To create a new workspace, use the following command:
terraform workspace new
To switch to a different workspace, use the following command:
terraform workspace select
2. Leverage Terraform Modules
Terraform modules allow you to encapsulate reusable infrastructure configurations. By using modules, you can avoid duplicating code and manage your infrastructure at a higher level of abstraction. This not only improves the efficiency of your deployments but also makes it easier to maintain and update your infrastructure as it evolves.
Here’s an example of how to use a module in your Terraform configuration:
module "my_module" { source = "path/to/module" variable1 = "value1" variable2 = "value2" }
3. Enable Terraform Parallelism
By default, Terraform performs resource creation, modification, and destruction operations sequentially. However, you can enable parallelism to improve the performance of your deployments. This allows Terraform to execute multiple operations concurrently, reducing the overall deployment time.
To enable parallelism, set the parallelism
option in your Terraform configuration:
parallelism = 10
4. Use Terraform State Management
Terraform state is a crucial component for tracking and managing your infrastructure. However, as your infrastructure grows, the state file can become large and slow down operations. To optimize the performance, consider using remote state management using tools like Google Cloud Storage or Terraform Cloud.
Here’s an example of configuring Terraform to use Google Cloud Storage for remote state management:
terraform { backend "gcs" { bucket = "my-bucket" prefix = "terraform/state" } }
5. Utilize Terraform Plan
Before applying changes to your infrastructure, it’s recommended to use the terraform plan
command. This command shows you a preview of the changes that Terraform will perform. By reviewing the plan, you can identify potential issues or unnecessary changes, improving the efficiency of your deployments.
To generate a plan, use the following command:
terraform plan
6. Optimize Resource Dependencies
Terraform determines the order in which resources are created based on their dependencies. By carefully defining dependencies, you can optimize the deployment process and reduce the time taken to provision resources. Take time to review and optimize the dependency graph of your Terraform configuration.
For example, if you have resources that can be created in parallel, define their dependencies accordingly:
resource "google_compute_instance" "instance1" { # ... } resource "google_compute_instance" "instance2" { # ... } resource "google_compute_instance" "instance3" { # ... } resource "google_compute_firewall" "firewall" { depends_on = [ google_compute_instance.instance1, google_compute_instance.instance2, google_compute_instance.instance3 ] # ... }
These are just a few advanced tips to optimize the performance and efficiency of your Terraform deployments on Google Cloud. By implementing these best practices and continually monitoring and refining your infrastructure, you can ensure smooth and efficient operations.
Troubleshooting and Debugging Terraform Deployments
Deploying infrastructure using Terraform on Google Cloud is a powerful way to automate your infrastructure provisioning. However, as with any complex system, things can sometimes go wrong. We will explore some common issues you may encounter when using Terraform and how to troubleshoot and debug them effectively.
Understanding Error Messages
When Terraform encounters an error during deployment, it provides detailed error messages that can help you identify the issue. It is important to carefully read and understand these messages to pinpoint the problem. The error messages usually include the resource causing the issue, the specific error, and additional information such as line numbers in the Terraform configuration files.
For example, if you encounter an error stating that a resource already exists, you may need to check if the resource was manually created outside of Terraform or if it was provisioned by a different Terraform configuration.
Using Terraform Commands to Debug
Terraform provides several commands that can help you debug and troubleshoot issues with your infrastructure deployment. Some useful commands include:
– terraform validate
: Checks the syntax and configuration of your Terraform files without making any changes. This command can help identify syntax errors or missing required fields.
– terraform plan
: Generates an execution plan showing what actions Terraform will take when applying the configuration. This can help you identify potential issues or conflicts before actually applying the changes.
– terraform state list
: Lists all resources managed by Terraform. This command can help you verify the current state of your infrastructure and detect any inconsistencies.
– terraform refresh
: Updates the Terraform state file with the latest information from the infrastructure. This command can be useful when there are discrepancies between the actual infrastructure and the Terraform state.
Using Logging and Monitoring
Logging and monitoring can be instrumental in troubleshooting Terraform deployments. By enabling detailed logging, you can track the execution flow and identify any errors or unexpected behavior. Google Cloud provides various logging options, such as Stackdriver Logging, where you can view logs for your infrastructure deployments.
Additionally, monitoring the performance and health of your infrastructure can help you identify and resolve issues proactively. Google Cloud offers tools like Stackdriver Monitoring, which allows you to set up custom dashboards and alerts to monitor critical metrics.
Interacting with the Google Cloud Platform (GCP) API
Sometimes, issues with Terraform deployments can be related to the underlying GCP API. In such cases, it can be helpful to interact directly with the GCP API to diagnose and troubleshoot the problem.
GCP provides a user-friendly web interface, the Google Cloud Console, where you can manually perform actions that Terraform would execute. By comparing the results of these manual actions with your Terraform configuration, you can identify differences or errors.
You can also use tools like gcloud
, the Google Cloud command-line interface, to interact with the GCP API. This can be particularly useful for testing and troubleshooting API-related issues.
Seeking Help from the Community
If you encounter a problem that you are unable to solve on your own, it can be beneficial to seek help from the Terraform community. The Terraform community is active and supportive, with various forums and discussion groups where you can ask questions and get assistance.
The official Terraform documentation and the Terraform GitHub repository are excellent resources for finding answers to common issues. You can also participate in the Terraform community Slack channel or join relevant forums, such as the HashiCorp Discuss forum, to engage with experienced Terraform users and share your challenges.
We explored some effective ways to troubleshoot and debug Terraform deployments on Google Cloud. Understanding error messages, using Terraform commands, leveraging logging and monitoring, interacting with the GCP API, and seeking help from the community are all valuable strategies to overcome any issues you may encounter. By mastering these techniques, you can ensure smooth deployments and maintain a reliable infrastructure.
Monitoring and Auditing Terraform Deployments
Monitoring and auditing your Terraform deployments on Google Cloud is crucial for maintaining visibility and control over your infrastructure. By implementing effective monitoring and auditing practices, you can ensure that your deployments are running smoothly and detect any issues or security vulnerabilities early on.
Monitoring with Stackdriver
Google Cloud provides a powerful monitoring and logging solution called Stackdriver. You can use Stackdriver to collect, analyze, and visualize metrics and logs from your Terraform deployments. By monitoring key metrics such as CPU usage, memory usage, and network traffic, you can gain insights into the health and performance of your infrastructure.
To enable Stackdriver monitoring for your Terraform deployments, you can use the google_monitoring_notification_channel
resource to create a notification channel. This channel can be configured to send alerts based on specific metrics or events. You can also use the google_monitoring_alert_policy
resource to define custom alerting policies for your deployments.
resource "google_monitoring_notification_channel" "email_channel" { display_name = "Email Channel" type = "email" labels = { email_address = "your-email@example.com" } } resource "google_monitoring_alert_policy" "high_cpu_alert" { display_name = "High CPU Alert" combiner = "OR" notification_channels = [google_monitoring_notification_channel.email_channel.name] conditions { condition_threshold { filter = "metric.type=\"compute.googleapis.com/instance/cpu/utilization\"" duration = "60s" comparison = "COMPARISON_GT" threshold_value = 0.8 } } }
Logging and Auditing with Cloud Audit Logs
Cloud Audit Logs provide a comprehensive record of activity in your Google Cloud environment, including changes made through Terraform deployments. By enabling Cloud Audit Logs, you can track and audit configuration changes, resource creation and deletion, and access control modifications.
To enable Cloud Audit Logs for your Terraform deployments, you can use the google_project_service
resource to enable the cloudaudit.googleapis.com
service. Once enabled, you can view the logs in the Cloud Console or export them to other logging and analysis tools.
resource "google_project_service" "audit_logs" { service = "cloudaudit.googleapis.com" }
Security Monitoring with Security Command Center
Google Cloud’s Security Command Center provides a centralized dashboard for monitoring and managing the security of your infrastructure. It offers a wide range of security monitoring capabilities, including vulnerability scanning, threat detection, and security health analytics.
To enable Security Command Center for your Terraform deployments, you can use the google_security_center_organization_config
resource to enable the service for your organization. Once enabled, Security Command Center will scan your infrastructure for security vulnerabilities and provide recommendations for remediation.
resource "google_security_center_organization_config" "security_center" { org_id = "YOUR_ORG_ID" enable_security_command_center = true }
Continuous Monitoring and Automation with Cloud Functions
To automate monitoring tasks and perform continuous monitoring of your Terraform deployments, you can leverage Google Cloud Functions. Cloud Functions allows you to run arbitrary code in response to events, such as changes to your infrastructure or specific metrics reaching a threshold.
For example, you can create a Cloud Function that triggers when a new resource is created or modified through Terraform. This function can then perform automated checks or validations to ensure that the resource meets your security and compliance requirements.
def monitor_terraform_changes(event, context): resource_name = event['resource']['name'] resource_type = event['resource']['type'] if resource_type == 'google_compute_instance': # Perform security checks on the instance # ... elif resource_type == 'google_storage_bucket': # Perform access control checks on the bucket # ... # ... # Log monitoring results print(f"Monitoring results for resource {resource_type}/{resource_name}")
By combining the power of Terraform, Stackdriver, Cloud Audit Logs, Security Command Center, and Cloud Functions, you can effectively monitor and audit your Terraform deployments on Google Cloud. This ensures the security, performance, and compliance of your infrastructure, enabling you to quickly identify and address any issues that may arise.
Automating Terraform Deployments with CI/CD Pipelines
Automating the deployment of your infrastructure using Terraform is a great way to ensure consistency and reproducibility. However, manually running Terraform commands every time you need to make a change can be time-consuming and error-prone. This is where continuous integration and continuous deployment (CI/CD) pipelines come in handy.
CI/CD pipelines allow you to automate the process of building, testing, and deploying your infrastructure code. By integrating Terraform with a CI/CD pipeline, you can automatically trigger the deployment whenever changes are made to your code repository, ensuring that your infrastructure is always up to date.
There are several popular CI/CD tools available, such as Jenkins, CircleCI, and GitLab CI/CD. We will focus on using GitLab CI/CD as an example to demonstrate how to automate Terraform deployments.
To get started, you will need a GitLab account and a project with your Terraform code repository. Once you have set up your repository, you can create a .gitlab-ci.yml
file in the root of your repository to define your CI/CD pipeline.
Here is an example .gitlab-ci.yml
file that demonstrates a basic CI/CD pipeline for Terraform:
stages: - plan - apply terraform: stage: plan image: hashicorp/terraform:latest script: - terraform init - terraform plan -out=tfplan artifacts: paths: - tfplan deploy: stage: apply image: hashicorp/terraform:latest script: - terraform apply tfplan
In this example, we define two stages: plan
and apply
. The plan
stage initializes Terraform and generates a plan file (tfplan
). The apply
stage applies the changes using the plan file generated in the previous stage.
By specifying the image
as hashicorp/terraform:latest
, we ensure that the pipeline runs in an environment with Terraform pre-installed.
Once you have committed and pushed the .gitlab-ci.yml
file to your repository, GitLab will automatically detect the file and trigger the pipeline whenever changes are made to your repository.
You can customize your pipeline further by adding additional stages, such as testing or linting, or by including environment-specific variables and secrets.
By automating your Terraform deployments with CI/CD pipelines, you can significantly streamline your workflow and reduce the risk of manual errors. Additionally, you can easily track and roll back changes using version control tools.
Remember to regularly review and update your CI/CD pipeline as your infrastructure code evolves to ensure the smooth deployment of your Terraform configurations.
Securing Terraform Deployments in Production Environments
Terraform is a powerful tool for infrastructure automation, but when working with production environments, it’s crucial to ensure the security of your deployments. We will discuss some advanced tips for securing Terraform deployments in production environments on Google Cloud.
Limiting Access to Terraform State
One of the first steps in securing Terraform deployments is to restrict access to the Terraform state files. These files contain sensitive information about your infrastructure, such as resource IDs, IP addresses, and credentials. It’s essential to ensure that only authorized individuals or systems can access and modify these files.
To limit access to the Terraform state, you can use Google Cloud Identity and Access Management (IAM) roles and policies. By granting the necessary permissions only to specific users or service accounts, you can control who can read, write, or modify the state files.
Here’s an example of how you can define an IAM policy to restrict access to the Terraform state bucket:
resource "google_storage_bucket_iam_binding" "terraform_state_bucket_iam" { bucket = google_storage_bucket.terraform_state.bucket role = "roles/storage.objectAdmin" members = [ "user:terraform-admin@example.com", "serviceAccount:terraform@project-id.iam.gserviceaccount.com", ] }
In this example, we grant the roles/storage.objectAdmin
role to both a user (terraform-admin@example.com
) and a service account (terraform@project-id.iam.gserviceaccount.com
), allowing them to perform administrative actions on the Terraform state bucket.
Securely Storing Sensitive Data
In many cases, Terraform deployments require sensitive data, such as API keys, passwords, or certificates. It’s crucial to handle this data securely to prevent unauthorized access or exposure.
One approach is to use Google Cloud Secret Manager to store and manage sensitive data securely. Secret Manager provides a centralized and encrypted storage for secrets, and you can retrieve them securely during your Terraform deployments.
Here’s an example of how you can retrieve a secret from Secret Manager and use it in your Terraform configuration:
data "google_secret_manager_secret_version" "database_password" { secret = "projects/my-project/secrets/database-password/versions/latest" } resource "google_sql_database_instance" "my_database" { password = data.google_secret_manager_secret_version.database_password.secret_data // ... }
In this example, we retrieve the latest version of the database-password
secret from Secret Manager and use it as the password for a Google Cloud SQL database instance.
Enable Audit Logging
To maintain visibility into your Terraform deployments and track any changes or actions, it’s essential to enable audit logging. Audit logs provide a detailed record of activity within your infrastructure, including who performed the action, what was modified, and when it occurred.
In Google Cloud, you can enable audit logging for various services, including Compute Engine, Cloud Storage, and Cloud IAM. By enabling audit logs, you can monitor and analyze the activities related to your Terraform deployments, helping you identify any potential security issues or unauthorized changes.
To enable audit logging for a specific service, you can use the Google Cloud Console or the Cloud SDK command-line tool.
Regularly Review and Update Access Controls
As your infrastructure evolves, it’s crucial to regularly review and update access controls to ensure that only authorized individuals or systems have the necessary permissions. This includes granting or revoking access to specific resources, adjusting IAM roles and policies, and removing any unnecessary privileges.
Google Cloud provides tools like the IAM Recommender and IAM Analyzer to help you identify and manage access control issues. These tools can analyze your IAM policies and provide recommendations for improving security and reducing the risk of unauthorized access.
Regularly reviewing and updating access controls is an essential part of maintaining the security of your Terraform deployments in production environments.
Implement Infrastructure as Code Best Practices
While not specific to securing Terraform deployments, following infrastructure as code (IaC) best practices can significantly enhance the security of your infrastructure. Some key practices include:
– Using version control to manage and track changes to your Terraform code.
– Regularly reviewing and testing your Terraform code for security vulnerabilities.
– Implementing a code review process to ensure that changes to your infrastructure follow security best practices.
– Using secure defaults and avoiding hardcoding sensitive information in your Terraform code.
– Regularly updating and patching your infrastructure components to address any security vulnerabilities.
By following these best practices, you can minimize the risk of security issues and ensure that your Terraform deployments are secure in production environments.
We discussed some advanced tips for securing Terraform deployments in production environments on Google Cloud. By implementing these security measures, you can ensure the confidentiality, integrity, and availability of your infrastructure.
Scaling and Managing Large-scale Infrastructure with Terraform
As your infrastructure grows and becomes more complex, it becomes crucial to have a reliable and efficient way to scale and manage it. Terraform provides powerful features that can help you scale and manage large-scale infrastructure on Google Cloud.
Modularize Your Code
Modularizing your Terraform code allows you to break down your infrastructure into smaller, reusable components. This approach improves code organization, promotes code reuse, and simplifies maintenance. You can create separate modules for different parts of your infrastructure, like networking, compute instances, or databases.
Here’s an example of a module that creates a Google Compute Engine instance:
module "compute_instance" { source = "path/to/module" project = var.project_id zone = var.zone instance_name = "my-instance" machine_type = "n1-standard-1" }
By encapsulating your infrastructure into modules, you can easily reuse them across different environments or projects. This not only saves time but also ensures consistency and reduces the chances of errors.
Use Terraform Workspaces
Terraform workspaces allow you to manage multiple environments or deployments within a single Terraform configuration. Each workspace acts as an isolated environment, with its own set of resources and variables. This feature is particularly useful for managing infrastructure at scale.
To create a new workspace, use the terraform workspace new
command:
terraform workspace new prod
To switch between workspaces, use the terraform workspace select
command:
terraform workspace select dev
By using workspaces, you can easily manage and apply changes to different environments without having to maintain separate configurations for each one.
Leverage Terraform State
Terraform state is a crucial component in managing large-scale infrastructure. It keeps track of the resources that Terraform manages and their current state. By default, Terraform stores the state locally in a file called terraform.tfstate
, but it’s recommended to use a remote state backend for collaboration and scalability.
Google Cloud Storage can be used as a remote backend for storing Terraform state. To configure it, add the following block to your Terraform configuration:
terraform { backend "gcs" { bucket = "my-terraform-state-bucket" prefix = "terraform/state" } }
Using a remote state backend allows multiple team members to work on the same infrastructure concurrently, reduces the likelihood of conflicts, and provides a reliable and centralized source of truth for your infrastructure state.
Implement Version Control
Using version control for your Terraform code is essential, especially when managing large-scale infrastructure. Version control systems like Git enable you to track changes, collaborate with others, and roll back to previous versions if needed.
It’s recommended to store your Terraform code in a Git repository and use branching and tagging strategies to manage different versions or releases of your infrastructure.
Here’s an example of creating a new branch and committing changes to Git:
git checkout -b feature/new-feature git add . git commit -m "Add new feature"
By implementing version control, you can effectively manage and track changes to your infrastructure codebase, ensuring stability and reproducibility.
Utilize Terraform Modules from the Community
The Terraform community offers a wide range of pre-built modules that can help you accelerate your infrastructure deployment. These modules cover a variety of use cases, from provisioning Kubernetes clusters to setting up logging and monitoring.
You can find a collection of Terraform modules on the Terraform Registry, which is the official module repository. These modules are typically maintained by the community and are a great resource for reusing battle-tested infrastructure code.
To use a module from the Terraform Registry, add the module block to your configuration and specify the source:
module "my_module" { source = "organization/module_name/google" version = "1.0.0" # Module-specific variables }
By leveraging existing modules, you can save time and effort when building and managing large-scale infrastructure.
Scaling and managing large-scale infrastructure with Terraform requires careful planning and implementation. By following these advanced tips, you can improve code organization, enhance scalability, and streamline collaboration, making the management of your infrastructure more efficient and reliable.