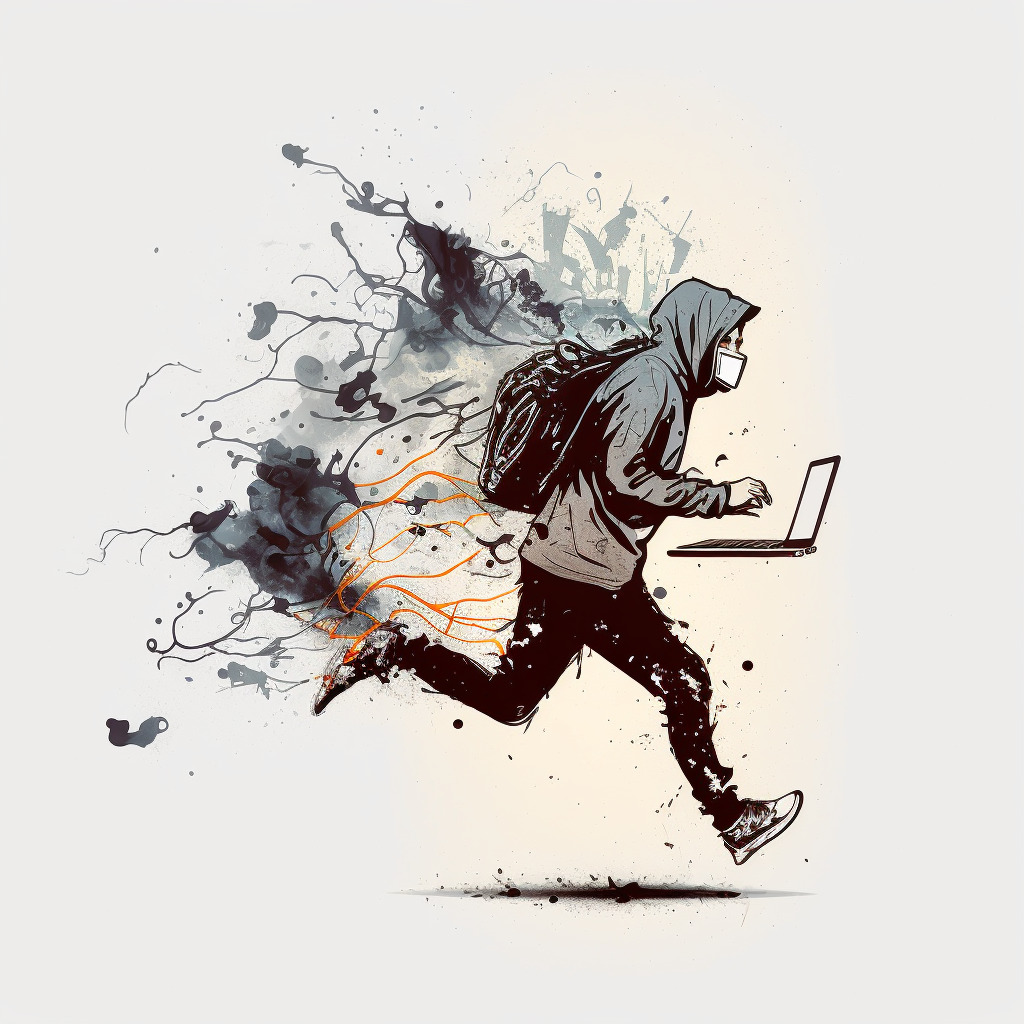
- Intro
- I. Understanding Smoke Testing
- A. Definition and purpose
- B. Importance in the software development lifecycle
- C. Differences between smoke and sanity testing
- II. Identifying Critical Functionalities
- A. Analyzing the application
- B. Prioritizing key features
- C. Involving stakeholders
- III. Designing Effective Smoke Test Cases
- A. Creating comprehensive test scenarios
- B. Focusing on positive test cases
- C. Ensuring test cases are easy to understand and maintain
- IV. Automating Smoke Tests
- A. Benefits of automation
- B. Choosing the right automation tools
- C. Integrating automation into your development process
- V. Integrating Smoke Testing into the Development Pipeline
- A. Identifying the appropriate stage for smoke tests
- B. Coordinating with the development team
- C. Implementing continuous integration and deployment
- VI. Tracking and Reporting Smoke Test Results
- A. Establishing a clear reporting process
- B. Monitoring test results over time
- C. Communicating results to stakeholders
- VII. Continuous Improvement of Smoke Tests
- A. Regularly reviewing and updating test cases
- B. Adapting to changes in the application
- C. Incorporating feedback from the development team and stakeholders
- VIII. Case Study: Successful Smoke Testing in Practice
- A. The challenges faced
- B. Implementing smoke testing best practices
- C. Results and lessons learned
- Conclusion
Intro
In today’s fast-paced software development world, it’s essential to catch critical issues before they make their way into production. Smoke testing plays a vital role in this process, as it provides a quick, high-level assessment of the system’s stability after a new build or release.
By verifying that the most crucial functionalities are working as expected, smoke tests help ensure that the software is ready for further, more in-depth testing. In this blog post, we will discuss the best practices for smoke testing, guiding you through the process of designing and executing effective tests to identify critical issues early in the development lifecycle.
Related Article: The issue with Monorepos
I. Understanding Smoke Testing
A. Definition and purpose
Smoke testing, sometimes referred to as build verification testing or confidence testing, is a preliminary testing process performed on a new build or release of a software application. The primary purpose of smoke testing is to ensure that the application’s critical functionalities are working as expected, and the build is stable enough to proceed with further testing. Smoke tests are typically a small set of high-level test cases that provide a quick assessment of the system’s overall health.
B. Importance in the software development lifecycle
Smoke testing plays a crucial role in the software development lifecycle (SDLC) as it helps identify critical issues early on, saving time and resources in the long run. When a new build is released, it’s essential to verify that the core features are functioning correctly before investing time and effort in more comprehensive testing. By catching critical issues early, smoke testing reduces the risk of discovering major problems later in the development process, when fixing them can be more costly and time-consuming. Furthermore, smoke testing helps ensure that the software is ready for subsequent stages of testing, such as integration, system, and acceptance testing.
Related Article: Ace Your DevOps Interview: Top 25 Questions and Answers
C. Differences between smoke and sanity testing
Although the terms smoke testing and sanity testing are often used interchangeably, there are key differences between the two. While both types of testing aim to determine if the application is stable enough for further testing, their focus and execution differ.
Smoke testing is a broader form of testing, typically conducted when a new build is released to verify that the critical functionalities are working correctly. It is performed early in the SDLC and helps identify major issues before other testing phases begin. Smoke tests are usually pre-defined and can be automated.
On the other hand, sanity testing is a narrower form of testing that focuses on specific components or features that have been modified or added in a recent build. It is conducted later in the SDLC, often after regression testing, to confirm that the changes made have not adversely affected the system’s functionality. Sanity tests are generally more ad-hoc and may not be as easily automated as smoke tests.
In summary, while both smoke and sanity testing serve essential purposes in the SDLC, their focus, execution, and timing differ. Understanding these differences can help you better plan and execute your testing processes, ultimately improving the quality of your software.
II. Identifying Critical Functionalities
A. Analyzing the application
The first step in creating an effective smoke testing process is to identify the critical functionalities of your application. This involves thoroughly analyzing your application and understanding its primary purpose, core features, and user workflows. Gaining a deep understanding of your application’s architecture, dependencies, and user interactions will help you pinpoint the areas where issues could have the most significant impact on the overall system stability and user experience.
Related Article: Why monitoring your application is important (2023 guide)
B. Prioritizing key features
Once you have a clear understanding of your application, it’s time to prioritize the key features that should be included in your smoke tests. These features are typically the ones that are most critical to the application’s functionality, have a high level of complexity, or are frequently used by the end-users. The goal is to focus on the areas of the application that are most likely to cause problems if they fail.
To prioritize the key features, you can start by creating a list of all the essential functionalities and then rank them based on their importance, complexity, and usage. This prioritization will help you focus your smoke testing efforts on the areas that matter the most, ensuring that you catch critical issues early in the development process.
C. Involving stakeholders
Involving stakeholders in the process of identifying critical functionalities is crucial for ensuring that your smoke tests align with the application’s requirements and user expectations. Stakeholders, such as product managers, business analysts, or end-users, can provide valuable insights into the features that are most important to them and the ones that could cause the most significant impact if they fail.
Collaborating with stakeholders during the smoke test planning phase can help you create a more comprehensive and effective smoke testing process. By incorporating their feedback and understanding their priorities, you can ensure that your smoke tests cover the areas that matter the most to your users and stakeholders, ultimately leading to a more stable and reliable application.
III. Designing Effective Smoke Test Cases
A. Creating comprehensive test scenarios
To create effective smoke test cases, you need to develop comprehensive test scenarios that cover the critical functionalities identified in the previous step. These scenarios should represent the most common user workflows and interactions with the application, ensuring that the key features are tested from a user’s perspective.
When creating test scenarios, consider the different ways users may interact with the application and the expected outcomes. For example, if you have an e-commerce application, some critical functionalities might include user registration, login, product search, adding items to the cart, and completing a purchase. The test scenarios should cover these workflows in detail, ensuring that the application behaves as expected.
Here’s an example of a simple test scenario for user registration:
Test Scenario: User Registration 1. Navigate to the registration page. 2. Fill in the required fields with valid data. 3. Click the "Register" button. 4. Verify that a confirmation message appears. 5. Verify that the user is redirected to the dashboard.
B. Focusing on positive test cases
Smoke testing primarily focuses on positive test cases, which are tests that verify that the application behaves correctly under expected conditions. The goal is to confirm that the critical functionalities work as intended, rather than attempting to find all possible edge cases and errors.
For instance, in the user registration example mentioned earlier, a positive test case would involve providing valid input data and ensuring that the registration process is successful.
def test_user_registration_success(): # Setup: Navigate to the registration page and enter valid data. navigate_to_registration_page() enter_valid_registration_data() # Action: Click the "Register" button. click_register_button() # Assert: Verify that a confirmation message appears and the user is redirected to the dashboard. assert is_confirmation_message_displayed() assert is_redirected_to_dashboard()
C. Ensuring test cases are easy to understand and maintain
It’s essential to ensure that your smoke test cases are easy to understand and maintain. This involves writing clear and concise test case descriptions, using descriptive function and variable names, and following the best practices for writing clean and maintainable code.
One way to make your test cases more maintainable is by using the Arrange-Act-Assert (AAA) pattern. This pattern involves organizing your test code into three distinct sections: setting up the test data and preconditions (Arrange), executing the action being tested (Act), and verifying that the expected outcome has occurred (Assert).
Here’s an example of a smoke test case using the AAA pattern:
def test_adding_item_to_cart(): # Arrange: Navigate to the product page and ensure the cart is empty. navigate_to_product_page() clear_shopping_cart() # Act: Add a product to the cart. add_product_to_cart() # Assert: Verify that the product is successfully added to the cart. assert is_product_in_cart()
By following these best practices, you can design effective smoke test cases that provide a quick and accurate assessment of your application’s stability, helping you catch critical issues early in the development process.
IV. Automating Smoke Tests
A. Benefits of automation
Automating smoke tests can provide several benefits to your software development process:
1. Speed: Automated tests can be executed much faster than manual tests, which allows you to quickly assess the stability of a new build and proceed with further testing or development.
2. Consistency: Automated tests follow the same steps each time they are executed, ensuring that the results are consistent and reliable.
3. Reusability: Once created, automated test scripts can be easily reused for future builds, reducing the time and effort needed for manual smoke testing.
4. Continuous Integration: Automated smoke tests can be integrated into your continuous integration pipeline, ensuring that critical issues are caught early and automatically during the development process.
B. Choosing the right automation tools
When choosing a smoke testing automation tool, consider the following factors:
1. Compatibility: The tool should be compatible with your application’s technology stack and your development environment.
2. Ease of use: The tool should be easy to learn and use, with a user-friendly interface and clear documentation.
3. Flexibility: The tool should be flexible enough to handle various test scenarios and adapt to changes in the application’s requirements.
4. Reporting: The tool should provide detailed and easy-to-understand test reports, making it simple to identify and fix issues.
Some popular automation tools for smoke testing include Selenium, TestNG, JUnit, and Pytest. Each of these tools has its advantages and limitations, so it’s essential to evaluate them based on your specific needs and requirements.
C. Integrating automation into your development process
To effectively integrate smoke test automation into your development process, follow these steps:
1. Create a smoke test suite: Develop a suite of automated smoke tests based on the test scenarios and cases designed in the previous steps. Ensure that the tests cover the critical functionalities of your application.
Using the Pytest framework, you can create a smoke test suite like this:
# test_smoke.py def test_user_registration(): # Your test code for user registration def test_login(): # Your test code for user login def test_search_product(): # Your test code for searching a product def test_add_to_cart(): # Your test code for adding an item to the cart def test_checkout(): # Your test code for completing a purchase
2. Set up a test environment: Configure a test environment that closely mirrors your production environment. This will help ensure that your automated tests accurately reflect real-world conditions.
3. Implement version control: Use a version control system, such as Git, to manage your smoke test scripts and keep track of changes.
To add your test suite to a Git repository, execute the following commands:
$ git init $ git add test_smoke.py $ git commit -m "Add smoke test suite" $ git remote add origin https://github.com/yourusername/yourrepository.git $ git push -u origin master
4. Schedule test execution: Configure your smoke tests to run automatically whenever a new build is released or at regular intervals, depending on your development process.
In this example, we will use Jenkins to schedule and run the smoke tests:
- Install the necessary plugins for your project (e.g., Python, Pytest, Git).
- Create a new Jenkins job and configure the Git repository containing your smoke test suite.
- Add a build step to execute the Pytest command:
pytest test_smoke.py
- Schedule the build to run whenever a new build is released or at regular intervals using the “Build Triggers” section.
5. Monitor and analyze test results: Regularly review the test results to identify and fix any issues that arise. Ensure that the relevant stakeholders are informed of the test results and any critical issues that need attention.
After executing your smoke tests in Jenkins, you can view the test results on the build page. The Pytest plugin provides a detailed report with pass/fail status and any error messages or stack traces.
By automating your smoke tests and integrating them into your development process, you can quickly and reliably catch critical issues early on, reducing the risk of major problems making their way into production.
V. Integrating Smoke Testing into the Development Pipeline
A. Identifying the appropriate stage for smoke tests
Smoke tests should be executed early in the development pipeline, typically right after a new build is created and before any further in-depth testing. The primary goal of smoke testing is to quickly identify critical issues that could render the application unusable or unstable. By executing smoke tests early in the pipeline, you can catch these issues before they reach later stages, saving time and effort.
B. Coordinating with the development team
To ensure that smoke testing is effectively integrated into the development pipeline, it’s crucial to coordinate with the development team. This involves:
1. Communicating the purpose and importance of smoke tests to developers, so they understand their role in maintaining the stability of the application.
2. Collaborating with developers to identify the critical functionalities that should be included in the smoke tests, as well as any changes to these functionalities as the application evolves.
3. Encouraging developers to execute smoke tests locally before committing their code to the version control system. This can help catch issues early and reduce the number of broken builds.
For example, developers can run smoke tests locally using the Pytest framework:
$ pytest test_smoke.py
C. Implementing continuous integration and deployment
Integrating smoke tests into your continuous integration (CI) and continuous deployment (CD) pipeline can help ensure that critical issues are caught early and automatically during the development process. Here’s how you can implement this integration using a CI/CD tool like Jenkins:
1. Create a new Jenkins job dedicated to smoke testing and configure it to trigger automatically whenever new code is pushed to the version control system.
2. Add a build step in the Jenkins job to check out the latest version of your code from the version control system (e.g., Git) and execute the smoke tests using the appropriate test runner (e.g., Pytest).
$ git pull origin master $ pytest test_smoke.py
3. Configure the Jenkins job to notify the development team of the test results, either by email or through a messaging platform like Slack.
4. Integrate smoke tests with your deployment process. If the smoke tests pass, proceed with the deployment of the new build to the staging or production environment. If the tests fail, halt the deployment process and notify the development team to fix the issues.
By integrating smoke testing into your development pipeline, you can quickly identify and address critical issues, ensuring a more stable and reliable application throughout the development process.
VI. Tracking and Reporting Smoke Test Results
A. Establishing a clear reporting process
To effectively track and report smoke test results, you should establish a clear and consistent reporting process. This involves:
1. Defining the format and content of the test reports, including details such as the test cases executed, pass/fail status, error messages, and any relevant screenshots or logs.
2. Choosing a centralized location for storing test reports, such as a shared drive or a dedicated test management tool.
3. Setting up automated notifications to inform relevant stakeholders of the test results, either through email or a messaging platform like Slack.
For example, you can use the Pytest framework to generate an XML report of your smoke test results:
$ pytest test_smoke.py --junitxml=smoke_test_report.xml
B. Monitoring test results over time
It’s essential to monitor smoke test results over time to identify trends and patterns that could indicate potential issues in the application. By regularly reviewing test results, you can proactively address any emerging problems before they become critical.
Some key metrics to track include:
1. Test pass/fail rates: Monitor the percentage of tests passing and failing in each smoke test run. An increase in the failure rate could indicate issues with the application or the test suite.
2. Test execution time: Track the time it takes to execute the smoke tests. A significant increase in execution time could indicate performance issues or inefficient test cases.
3. Test coverage: Keep track of the number of critical functionalities covered by the smoke tests. As the application evolves, it’s essential to ensure that new critical features are included in the smoke tests.
C. Communicating results to stakeholders
Effective communication of smoke test results is crucial for ensuring that relevant stakeholders are aware of the application’s stability and any critical issues that need to be addressed. To communicate test results:
1. Share test reports with stakeholders, either through email or a shared drive. Ensure that the reports are clear, concise, and easy to understand.
2. Present test results in team meetings, discussing any issues that were encountered and the steps taken to resolve them.
3. Establish a process for escalating critical issues to the appropriate team members, ensuring that they are addressed promptly and efficiently.
For example, you can use a messaging platform like Slack to automatically notify stakeholders of the smoke test results:
`
# Assuming you have a slack bot set up, you can use a script like this:
# Assuming you have a slack bot set up, you can use a script like this: import os from slack_sdk import WebClient from slack_sdk.errors import SlackApiError client = WebClient(token=os.environ['SLACK_API_TOKEN']) try: response = client.chat_postMessage( channel='#smoke-test-results', text='Smoke Test Results: 10 Passed, 1 Failed' ) except SlackApiError as e: print(f"Error posting message: {e}")
By establishing a clear reporting process, monitoring test results over time, and effectively communicating results to stakeholders, you can ensure that your smoke tests provide valuable insights into the stability and reliability of your application.
VII. Continuous Improvement of Smoke Tests
A. Regularly reviewing and updating test cases
To ensure that your smoke tests remain effective and relevant, it’s crucial to regularly review and update the test cases. This involves:
1. Analyzing test results and identifying any recurring issues or patterns that could indicate problems with the test cases themselves.
2. Reviewing the test cases for clarity and simplicity, ensuring that they are easy to understand, maintain, and update.
3. Updating test cases as needed to address any changes in the application, such as new features or modified functionalities.
For example, if a new critical feature has been added to your application, you should create a new smoke test case for it:
# test_smoke.py # Existing test cases... def test_new_critical_feature(): # Your test code for the new critical feature
B. Adapting to changes in the application
As your application evolves, it’s essential to adapt your smoke tests accordingly. This involves:
1. Identifying new critical functionalities that should be included in the smoke tests, either through discussions with the development team or by analyzing changes to the application’s requirements.
2. Modifying existing test cases as needed to accommodate changes in the application, such as updates to user interfaces, APIs, or data structures.
3. Removing or de-prioritizing test cases that no longer cover critical functionalities, ensuring that the smoke tests remain focused on the most important aspects of the application.
C. Incorporating feedback from the development team and stakeholders
To continuously improve your smoke tests, it’s important to incorporate feedback from the development team and other stakeholders. This involves:
1. Regularly discussing the smoke test results with the development team and stakeholders, seeking their input on any issues encountered and potential improvements to the test cases.
2. Encouraging developers to participate in the creation and maintenance of smoke test cases, fostering a sense of ownership and responsibility for the quality of the application.
3. Implementing improvements based on feedback, such as modifying test cases, updating the reporting process, or refining the smoke testing schedule.
For example, if the development team suggests that the current smoke tests don’t adequately cover a specific functionality, you can work with them to create a new test case that addresses the concern:
# test_smoke.py # Existing test cases... def test_updated_functionality(): # Your test code for the updated functionality, based on feedback from the development team
By regularly reviewing and updating your smoke tests, adapting to changes in the application, and incorporating feedback from the development team and stakeholders, you can ensure that your smoke tests remain effective and continue to provide valuable insights into the stability and reliability of your application.
VIII. Case Study: Successful Smoke Testing in Practice
A. The challenges faced
A medium-sized e-commerce company faced several challenges in their software development process. They experienced frequent critical issues in production, leading to downtime and lost revenue. The development team struggled to identify and fix issues before they reached production, as they lacked a consistent and effective testing process. This situation led the company to explore smoke testing as a potential solution to improve their application stability.
B. Implementing smoke testing best practices
To address these challenges, the company decided to implement smoke testing best practices, which included the following steps:
1. Identifying critical functionalities: The development team worked with product managers and other stakeholders to identify the key features of their e-commerce platform that were critical to the user experience, such as user registration, login, product search, adding items to the cart, and checkout.
2. Designing effective smoke test cases: The team created comprehensive test scenarios and focused on positive test cases to ensure that the critical functionalities were working as expected. They also made sure that the test cases were easy to understand and maintain.
# test_smoke.py def test_user_registration(): # Test code for user registration def test_login(): # Test code for user login def test_search_product(): # Test code for searching a product def test_add_to_cart(): # Test code for adding an item to the cart def test_checkout(): # Test code for completing a purchase
3. Automating smoke tests: The company chose to automate their smoke tests using the Pytest framework and integrated the tests into their continuous integration (CI) pipeline. This allowed them to run the smoke tests automatically whenever new code was pushed to the version control system.
4. Integrating smoke testing into the development pipeline: The company identified the appropriate stage for smoke tests in their pipeline and coordinated with the development team to ensure the tests were executed consistently. They also implemented continuous integration and deployment using tools like Jenkins to automate the entire process.
C. Results and lessons learned
After implementing smoke testing best practices, the company experienced several positive outcomes:
1. Improved application stability: By catching critical issues early in the development process, the company significantly reduced the number of issues reaching production, leading to a more stable and reliable user experience.
2. Faster issue resolution: The development team was able to quickly identify and fix issues before they reached later stages of the development pipeline, saving time and effort.
3. Greater collaboration: The process of identifying critical functionalities and designing test cases encouraged collaboration between the development team, product managers, and other stakeholders, fostering a shared sense of responsibility for the quality of the application.
4. Continuous improvement: The company’s commitment to regularly reviewing and updating their smoke tests, adapting to changes in the application, and incorporating feedback from the development team and stakeholders ensured that their smoke tests remained effective and provided valuable insights into the application’s stability.
Through the successful implementation of smoke testing best practices, the company was able to address their challenges and significantly improve their software development process. This case study demonstrates the value of smoke testing in identifying and resolving critical issues early in the development process, ultimately leading to a more stable and reliable application.
Conclusion
Implementing smoke testing best practices is key to ensuring the stability and reliability of your software. By focusing on critical functionalities, designing test cases that cover key scenarios, automating the process, and integrating smoke tests into your development pipeline, you can catch issues early and prevent them from escalating into more significant problems.
By doing so, you’ll save time, reduce costs, and ultimately deliver a higher-quality product to your users. So, embrace these best practices and watch your software development process become more efficient and robust, giving you the confidence you need for every release.