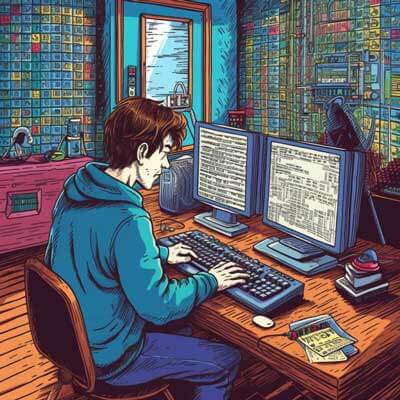
- Introduction to Microservice Architecture
- Key Features of Microservices
- 1. Service Independence
- 2. Polyglot Persistence
- Implementation Example: Service Discovery
- Implementation Example: Scalability
- Implementation Example: Fault Tolerance
- Implementation Example: Resilience
- Individual Components of Microservices
- 1. API Gateway
- 2. Event Bus
- Use Cases
- 1. E-commerce Applications
- 2. Internet of Things (IoT)
- Best Practices
- 1. Single Responsibility Principle
- 2. Domain-Driven Design
- Real World Examples
- 1. Netflix
- 2. Uber
- Performance Considerations
- 1. Network Latency
- 2. Distributed Data Management
- Advanced Techniques
- 1. Serverless Computing
- 2. Reactive Programming
- Code Snippet Ideas
- 1. Service-to-Service Communication with REST
- 2. Asynchronous Messaging with RabbitMQ
- Error Handling
- 1. Circuit Breaker Pattern
- 2. Centralized Logging and Monitoring
Introduction to Microservice Architecture
Microservice architecture is an architectural style that structures an application as a collection of small, loosely coupled services. Each service focuses on a specific business capability and can be developed, deployed, and scaled independently. This approach allows for greater flexibility, scalability, and resilience compared to traditional monolithic architectures.
Related Article: How to Migrate a Monolith App to Microservices
Key Features of Microservices
Microservices offer several key features that make them attractive for building complex, scalable applications. Some of these features include:
1. Service Independence
In a microservice architecture, each service is independent and can be developed and deployed separately. This allows teams to work on different services concurrently, enabling faster development cycles and reducing dependencies.
// Example of a microservice in Java using Spring Boot @RestController @RequestMapping("/users") public class UserController { @Autowired private UserService userService; @GetMapping("/{id}") public User getUser(@PathVariable("id") Long id) { return userService.getUserById(id); } // Other CRUD operations... }
2. Polyglot Persistence
Microservices allow for the use of different databases and storage technologies depending on the specific needs of each service. This enables developers to choose the most suitable persistence mechanism for each service, such as relational databases, NoSQL databases, or in-memory caches.
# Example of a microservice's configuration file in YAML spring: datasource: url: jdbc:mysql://localhost:3306/users username: root password: password jpa: database-platform: org.hibernate.dialect.MySQL5Dialect hibernate: ddl-auto: update
Related Article: Mastering Microservices: A Comprehensive Guide to Building Scalable and Agile Applications
Implementation Example: Service Discovery
Service discovery is a critical aspect of microservice architecture, as it enables services to locate and communicate with each other dynamically. One popular implementation of service discovery is using a service registry, such as Netflix Eureka.
// Example of a service registration in Java using Netflix Eureka and Spring Cloud @SpringBootApplication @EnableEurekaServer public class ServiceRegistryApplication { public static void main(String[] args) { SpringApplication.run(ServiceRegistryApplication.class, args); } }
Implementation Example: Scalability
Scalability is a crucial requirement for microservices to handle varying workloads effectively. One common approach is to use containerization technologies like Docker and orchestration platforms like Kubernetes to dynamically scale services based on demand.
# Example of a Kubernetes deployment configuration file in YAML apiVersion: apps/v1 kind: Deployment metadata: name: user-service spec: replicas: 3 selector: matchLabels: app: user-service template: metadata: labels: app: user-service spec: containers: - name: user-service image: my-registry/user-service:latest ports: - containerPort: 8080
Implementation Example: Fault Tolerance
Fault tolerance is a critical aspect of microservice architecture to ensure the system remains operational even in the presence of failures. One approach is to use circuit breakers, such as Netflix Hystrix, to isolate failing services and prevent cascading failures.
// Example of circuit breaker implementation in Java using Netflix Hystrix @Service public class UserService { @HystrixCommand(fallbackMethod = "getDefaultUser") public User getUserById(Long id) { // Call user service API // Return user details } public User getDefaultUser(Long id) { // Return default user details } }
Implementation Example: Resilience
Resilience is the ability of microservices to recover from failures and continue performing their intended functions. One technique is to implement retry mechanisms with exponential backoff to handle transient failures.
// Example of retry mechanism implementation in Java using Spring Retry @Retryable(maxAttempts = 3, backoff = @Backoff(delay = 1000)) public void processOrder(Order order) { // Process order logic }
Individual Components of Microservices
Microservices typically consist of various individual components, each responsible for a specific aspect of the application. Some common components include:
1. API Gateway
The API gateway acts as a single entry point for client applications to interact with the microservices. It handles request routing, authentication, and aggregation of data from multiple services.
// Example of an API gateway implementation in Java using Spring Cloud Gateway @Configuration public class GatewayConfiguration { @Bean public RouteLocator routeLocator(RouteLocatorBuilder builder) { return builder.routes() .route("user-service", r -> r.path("/users/**") .uri("http://user-service")) .route("order-service", r -> r.path("/orders/**") .uri("http://order-service")) .build(); } }
2. Event Bus
An event bus allows microservices to communicate asynchronously by publishing and subscribing to events. This enables loose coupling between services and supports event-driven architectures.
// Example of an event bus implementation in Java using Apache Kafka public class KafkaEventBus { private KafkaProducer producer; public void publishEvent(String topic, String message) { producer.send(new ProducerRecord(topic, message)); } public void subscribeToEvent(String topic) { KafkaConsumer consumer = new KafkaConsumer(properties); consumer.subscribe(Collections.singletonList(topic)); while (true) { ConsumerRecords records = consumer.poll(Duration.ofMillis(100)); for (ConsumerRecord record : records) { // Process event } } } }
Use Cases
Microservice architecture is well-suited for various use cases, including:
1. E-commerce Applications
E-commerce applications often have complex and evolving business requirements. Microservices allow for flexibility, scalability, and continuous delivery, enabling rapid adaptation to changing market conditions.
// Example of an e-commerce microservice for product management in Java @RestController @RequestMapping("/products") public class ProductController { @Autowired private ProductService productService; @GetMapping("/{id}") public Product getProduct(@PathVariable("id") Long id) { return productService.getProductById(id); } // Other CRUD operations... }
2. Internet of Things (IoT)
IoT applications involve a large number of devices generating massive amounts of data. Microservices facilitate the processing and analysis of IoT data by allowing for horizontal scaling and the use of specialized services for data ingestion, storage, and analytics.
# Example of an IoT microservice for data ingestion in Python using Flask from flask import Flask, request app = Flask(__name__) @app.route("/", methods=["POST"]) def ingest_data(): # Process and store IoT data return "Data ingested successfully" if __name__ == "__main__": app.run(host="0.0.0.0", port=8080)
Best Practices
When designing and implementing microservices, it is important to follow certain best practices to ensure the success of the architecture. Some best practices include:
1. Single Responsibility Principle
Each microservice should have a single responsibility that aligns with a specific business capability. This promotes maintainability and reduces complexity within each service.
2. Domain-Driven Design
Applying domain-driven design principles helps to identify bounded contexts and define clear service boundaries. This improves modularity and enables independent development and deployment of microservices.
Real World Examples
Several organizations have successfully adopted microservice architecture in their applications. Some notable examples include:
1. Netflix
Netflix has embraced microservices to build and scale its streaming platform. The use of microservices allows them to continuously innovate and rapidly release new features while maintaining high availability and performance.
2. Uber
Uber relies on microservices to power its ride-sharing platform. Microservices enable Uber to handle millions of concurrent requests, scale geographically, and support various business capabilities such as payments, logistics, and driver management.
Performance Considerations
While microservices offer numerous benefits, they also introduce performance considerations that need to be addressed. Some key considerations include:
1. Network Latency
Microservices communicate over the network, which introduces potential latency. Minimizing network round trips and optimizing service-to-service communication can help mitigate this issue.
2. Distributed Data Management
Managing data across multiple microservices can be challenging. Techniques such as caching, replication, and eventual consistency can be employed to ensure data integrity and performance.
Advanced Techniques
As microservices mature, advanced techniques have emerged to address specific challenges and enhance the architecture. Some advanced techniques include:
1. Serverless Computing
Serverless computing allows developers to focus on writing code without worrying about infrastructure management. It complements microservices by providing event-driven scalability and cost efficiency.
2. Reactive Programming
Reactive programming enables building highly responsive, resilient, and elastic microservices. It leverages asynchronous and non-blocking communication patterns to handle large volumes of concurrent requests.
Code Snippet Ideas
Here are a few code snippet ideas that can be useful when working with microservices:
1. Service-to-Service Communication with REST
// Example of RESTful communication between microservices in Java using Spring Cloud @FeignClient(name = "user-service") public interface UserServiceClient { @GetMapping("/users/{id}") User getUserById(@PathVariable("id") Long id); }
2. Asynchronous Messaging with RabbitMQ
# Example of asynchronous messaging between microservices in Python using RabbitMQ and Celery from celery import Celery app = Celery("tasks", broker="amqp://guest:guest@localhost:5672//") @app.task def process_order(order): # Process order asynchronously if __name__ == "__main__": app.start()
Error Handling
Error handling is an important aspect of microservices to ensure the system remains stable and resilient. Some error handling techniques include:
1. Circuit Breaker Pattern
The circuit breaker pattern allows services to gracefully handle failures and fallback to alternative behavior when a service is unavailable or experiencing issues.
2. Centralized Logging and Monitoring
Centralized logging and monitoring solutions help track errors and performance issues across microservices. Tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Prometheus can be used to gain insights into the system’s health.