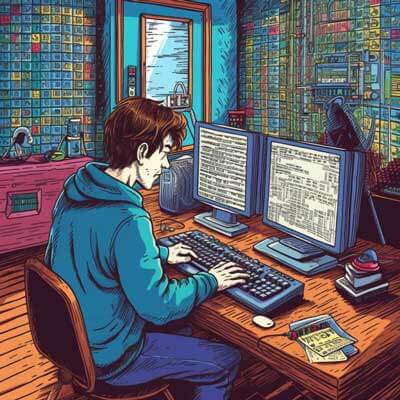
- Getting Started with Terraform and AWS
- Step 1: Install Terraform
- Step 2: Set Up AWS Credentials
- Step 3: Initialize a Terraform Project
- Step 4: Apply Your Terraform Configuration
- Step 5: Destroy Your Infrastructure
- Understanding Infrastructure as Code
- Provisioning AWS Resources with Terraform
- Initializing a Terraform Project
- Defining AWS Provider Configuration
- Provisioning an EC2 Instance
- Provisioning an S3 Bucket
- Provisioning a DynamoDB Table
- Applying Terraform Changes
- Destroying Provisioned Resources
- Managing AWS Infrastructure with Terraform
- 1. Organizing Your Terraform Code
- 2. Using Terraform Modules
- 3. Terraform State Management
- 4. Managing Sensitive Data
- Designing Highly Available AWS Architecture
- Understanding Availability Zones
- Load Balancing and Auto Scaling
- Database Replication
- Monitoring and Alerting
- Automating Infrastructure Deployment with Terraform
- Scaling and Load Balancing in AWS with Terraform
- Auto Scaling Groups
- Load Balancers
- Scaling Policies
- Securing Your AWS Infrastructure with Terraform
- 1. Implementing IAM Roles and Policies
- 2. Implementing Network Security
- 3. Managing Secrets and Sensitive Data
- 4. Continuous Security Monitoring
- Monitoring and Logging in AWS with Terraform
- CloudWatch Metrics and Alarms
- CloudTrail Logging
- Centralized Logging with CloudWatch Logs
- Cost Optimization Techniques with Terraform
- Managing AWS Secrets with Terraform
- Using Environment Variables
- Using HashiCorp Vault
- Combining Environment Variables and HashiCorp Vault
- Deploying Serverless Applications with Terraform
- Creating AWS Lambda Functions
- Triggering Lambda Functions with AWS API Gateway
- Deploying Serverless Applications
- Integrating Terraform with CI/CD Pipelines
- Why integrate Terraform with CI/CD pipelines?
- Setting up your CI/CD pipeline
- Best practices for integrating Terraform with CI/CD pipelines
- Troubleshooting and Debugging Terraform Deployments
Getting Started with Terraform and AWS
Terraform is an open-source infrastructure as code (IaC) tool that allows you to define and provision your infrastructure in a declarative manner. With Terraform, you can easily manage and scale your infrastructure deployments on AWS. In this chapter, we will guide you through the process of getting started with Terraform and AWS.
Related Article: Terraform Advanced Tips on Google Cloud
Step 1: Install Terraform
To get started, you need to install Terraform on your local machine. Terraform supports all major operating systems, including Windows, macOS, and Linux.
You can download the appropriate Terraform package for your operating system from the official Terraform website: https://www.terraform.io/downloads.html. Once downloaded, follow the installation instructions specific to your operating system.
Step 2: Set Up AWS Credentials
To interact with your AWS account using Terraform, you need to configure your AWS credentials.
Start by creating an IAM user in your AWS account with the necessary permissions for Terraform. The recommended approach is to create a separate IAM user with limited permissions that only allow Terraform to manage resources within a specific scope.
Once you have created the IAM user, obtain the access key and secret access key for the user. You can do this by navigating to the IAM console in your AWS account, selecting the user, and generating the access keys.
To configure your AWS credentials on your local machine, you can either set environment variables or use the AWS CLI configuration file. For example, using the AWS CLI configuration file, you would create or modify the file located at ~/.aws/credentials
and add the following:
[default] aws_access_key_id = YOUR_ACCESS_KEY aws_secret_access_key = YOUR_SECRET_KEY
Step 3: Initialize a Terraform Project
Once you have installed Terraform and configured your AWS credentials, you can initialize a new Terraform project.
Create a new directory for your project and navigate to that directory in your terminal. Then, create a new file called main.tf
to define your infrastructure.
In the main.tf
file, you can start by configuring the AWS provider and defining your resources. Here’s an example that creates an EC2 instance:
provider "aws" { region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" tags = { Name = "example-instance" } }
In the above example, we specified the AWS region and created an EC2 instance using a specific Amazon Machine Image (AMI) and instance type. We also added a tag to the instance for better organization.
Related Article: Quick and Easy Terraform Code Snippets
Step 4: Apply Your Terraform Configuration
Once you have defined your infrastructure in the main.tf
file, you can apply your Terraform configuration to create the resources on AWS.
In your terminal, navigate to the directory containing your main.tf
file and run the following command:
terraform init
This command initializes the Terraform project and downloads the necessary provider plugins.
Next, run the following command to preview the changes that Terraform will make:
terraform plan
This command provides a summary of the resources that will be created, modified, or destroyed.
Finally, apply your Terraform configuration by running the following command:
terraform apply
Terraform will prompt you to confirm the changes before applying them. If you’re satisfied with the changes, type “yes” to proceed.
Terraform will then provision the resources on AWS according to your configuration.
Step 5: Destroy Your Infrastructure
If you no longer need your infrastructure, you can use Terraform to destroy it and remove all associated resources.
To destroy your infrastructure, navigate to the directory containing your main.tf
file and run the following command:
terraform destroy
Terraform will prompt you to confirm the destruction of all resources. If you’re sure, type “yes” to proceed.
Terraform will then destroy the resources on AWS, ensuring that all associated resources are removed.
Congratulations! You have successfully started using Terraform to manage your AWS infrastructure. In the next chapters, we will explore advanced tips and techniques to streamline your infrastructure deployment using Terraform and AWS.
Understanding Infrastructure as Code
Infrastructure as Code (IaC) is a methodology that allows you to define and manage your infrastructure using machine-readable files. With IaC, you can treat your infrastructure in the same way you treat your application code, applying version control, testing, and automation practices to infrastructure deployment.
One of the most popular tools for implementing IaC is Terraform. Terraform is an open-source provisioning tool that enables you to define and create your infrastructure using a declarative configuration language. By describing your infrastructure as code, you can easily manage and automate the provisioning of resources on cloud platforms like AWS.
Terraform uses a simple syntax called HashiCorp Configuration Language (HCL) to define the desired state of your infrastructure. This state includes resources such as virtual machines, storage buckets, databases, and networking components. By writing Terraform configuration files, you can specify the desired state and let Terraform handle the provisioning and management of the resources.
Let’s take a look at a simple Terraform configuration file that provisions an Amazon EC2 instance:
provider "aws" { region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this example, we define the AWS provider we want to use, specifying the region where our resources will be provisioned. Then, we create an EC2 instance resource, specifying the Amazon Machine Image (AMI) and the instance type.
By running terraform apply
, Terraform reads the configuration file, compares the desired state with the current state, and makes the necessary changes to reach the desired state. It provisions the EC2 instance, and if any changes are made to the configuration, Terraform will update the infrastructure accordingly.
Terraform also allows you to write more complex configurations using variables, modules, and data sources. Variables enable you to define reusable values that can be passed to your configuration, allowing for more dynamic and flexible infrastructure. Modules provide a way to organize and reuse Terraform configurations, making it easier to manage larger infrastructures. Data sources allow you to import information from external sources, such as AWS, and use it in your configuration.
Additionally, Terraform supports the use of remote state, which allows you to store the state file in a remote location, such as Amazon S3. This enables collaboration and allows multiple team members to work on the same infrastructure.
By adopting Infrastructure as Code with Terraform, you can achieve several benefits. It helps you avoid manual configuration and reduces the risk of human error. It provides a version-controlled and auditable history of changes, making it easier to track and roll back infrastructure modifications. It also enables you to automate the provisioning of resources, allowing for faster and more reliable deployments.
In the next sections, we will explore advanced tips and techniques to streamline your infrastructure deployment using Terraform and AWS.
Related Article: Terraform Tutorial & Advanced Tips
Provisioning AWS Resources with Terraform
Terraform is a powerful infrastructure-as-code tool that allows you to provision and manage your infrastructure resources in a declarative manner. In this chapter, we will explore how to provision AWS resources using Terraform, enabling you to streamline your infrastructure deployment process.
Before we begin, ensure that you have the Terraform CLI installed and configured with your AWS credentials. You can find detailed instructions on how to set up Terraform and AWS credentials in the official Terraform documentation.
Initializing a Terraform Project
To get started, create a new directory for your Terraform project. Open a terminal or command prompt and navigate to the directory. Run the following command to initialize your Terraform project:
terraform init
This command downloads the necessary provider plugins and sets up your project.
Defining AWS Provider Configuration
To interact with AWS resources, you need to define a provider configuration block in your Terraform code. This block specifies the necessary credentials and AWS region to use. Here’s an example:
provider "aws" { access_key = "YOUR_ACCESS_KEY" secret_key = "YOUR_SECRET_KEY" region = "us-west-2" }
Replace YOUR_ACCESS_KEY
and YOUR_SECRET_KEY
with your actual AWS access key and secret key. Additionally, set the appropriate AWS region for your deployment.
Provisioning an EC2 Instance
Let’s say you want to provision an EC2 instance using Terraform. You can define an EC2 resource block in your Terraform code to specify the instance details. Here’s an example:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" key_name = "my-key-pair" subnet_id = "subnet-0123456789abcdef0" }
In this example, we are creating an EC2 instance with the specified AMI, instance type, key pair, and subnet. Replace the values with the appropriate ones for your environment.
Provisioning an S3 Bucket
To provision an S3 bucket using Terraform, define an S3 bucket resource block in your code. Here’s an example:
resource "aws_s3_bucket" "example" { bucket = "my-example-bucket" acl = "private" }
In this example, we are creating an S3 bucket with the specified name and access control list (ACL). Customize the bucket name to suit your needs.
Provisioning a DynamoDB Table
If you need to provision a DynamoDB table, you can define a DynamoDB table resource block in your Terraform code. Here’s an example:
resource "aws_dynamodb_table" "example" { name = "my-example-table" billing_mode = "PAY_PER_REQUEST" hash_key = "id" attribute { name = "id" type = "N" } }
In this example, we are creating a DynamoDB table with the specified name, billing mode, and hash key. Customize the values to match your requirements.
Applying Terraform Changes
Once you have defined your AWS resources in Terraform, you can apply the changes by running the following command:
terraform apply
Terraform will analyze the configuration and prompt you to confirm the changes. Type “yes” to proceed with the provisioning process.
Destroying Provisioned Resources
If you no longer need the provisioned AWS resources, you can destroy them using Terraform. Run the following command:
terraform destroy
Terraform will identify the resources defined in your configuration and prompt you to confirm the destruction. Type “yes” to proceed with the resource deletion.
By leveraging Terraform’s infrastructure-as-code capabilities, you can easily provision and manage your AWS resources, enabling you to streamline your infrastructure deployment process.
Managing AWS Infrastructure with Terraform
Terraform is a powerful tool that allows you to manage your AWS infrastructure as code. With Terraform, you can define and provision your infrastructure using a declarative configuration language. This chapter will guide you through some advanced tips for managing your AWS infrastructure effectively with Terraform.
1. Organizing Your Terraform Code
As your infrastructure grows, it becomes essential to organize your Terraform code in a structured manner. By following best practices, you can make your code more maintainable and easier to understand.
One common approach is to use a modularized directory structure. You can separate different resources into their own modules and create a main.tf file that calls these modules. This modular approach helps in reusability and keeps your codebase clean.
Another essential aspect of organizing your Terraform code is using variables and outputs. By defining variables, you can make your code more flexible and easier to configure. Outputs, on the other hand, allow you to expose important information about your infrastructure, such as IP addresses or resource IDs.
Here’s an example of how you can define variables and outputs in your Terraform code:
# variables.tf variable "instance_type" { description = "The EC2 instance type" default = "t2.micro" } # main.tf resource "aws_instance" "example" { instance_type = var.instance_type # ... } output "instance_ip" { value = aws_instance.example.private_ip }
2. Using Terraform Modules
Terraform modules are reusable units of Terraform configuration that can be used across projects. Modules can encapsulate complex resources and configurations, making it easier to manage infrastructure across different environments.
By using modules, you can abstract away the complexity of provisioning resources and provide a simple interface for other teams or projects to consume your infrastructure.
Here’s an example of how you can use a module in your Terraform code:
# main.tf module "vpc" { source = "terraform-aws-modules/vpc/aws" version = "2.0.0" name = "my-vpc" cidr = "10.0.0.0/16" } resource "aws_instance" "example" { instance_type = "t2.micro" vpc_id = module.vpc.vpc_id # ... }
3. Terraform State Management
Terraform uses a state file to keep track of the resources it manages. The state file is crucial for Terraform to understand the current state of your infrastructure and make accurate changes.
To manage your Terraform state effectively, you can store it remotely using a backend. AWS provides a backend called Terraform State in Amazon S3, which allows you to store and manage your state files securely.
By using a remote backend, you can collaborate with your team more effectively and ensure consistency across multiple Terraform runs.
Here’s an example of how you can configure a remote backend in your Terraform code:
# backend.tf terraform { backend "s3" { bucket = "my-terraform-state" key = "terraform.tfstate" region = "us-west-2" } }
4. Managing Sensitive Data
When working with Terraform, it’s common to encounter sensitive data such as API keys, passwords, or private keys. It’s crucial to handle this sensitive data securely and avoid committing it to your version control system.
Terraform provides a feature called “input variables” that allows you to pass sensitive data securely. You can use the sensitive
argument to ensure that the value of the variable is not shown in the command output or the state file.
Here’s an example of how you can define a sensitive variable in your Terraform code:
# variables.tf variable "aws_access_key" { description = "AWS access key" type = string sensitive = true } # main.tf provider "aws" { access_key = var.aws_access_key # ... }
By using input variables with the sensitive
argument, you can ensure that sensitive data is handled securely within your Terraform code.
These advanced tips will help you streamline your AWS infrastructure deployment with Terraform. By organizing your code, using modules, managing state effectively, and handling sensitive data securely, you can achieve more efficient and maintainable infrastructure management.
Designing Highly Available AWS Architecture
Designing a highly available architecture is crucial for ensuring the reliability and fault tolerance of your AWS infrastructure. By distributing your workload across multiple availability zones (AZs) and implementing redundancy, you can minimize the impact of failures and provide a seamless experience for your users.
Understanding Availability Zones
Availability Zones are physically separate data centers within a region that are interconnected through high-speed networks. Each AZ is designed to be independent and isolated, with its own power, cooling, and networking infrastructure. By deploying resources across multiple AZs, you can protect your applications and data from failures that may occur in a single AZ.
In Terraform, you can specify the AZs by using the availability_zones
argument in the resource definition. For example, to create an EC2 instance in two different AZs, you can use the following code snippet in your Terraform configuration file (e.g., main.tf
):
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" availability_zone = "us-west-2a" } resource "aws_instance" "example2" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" availability_zone = "us-west-2b" }
Load Balancing and Auto Scaling
To achieve high availability and scalability, it’s important to distribute the incoming traffic evenly across multiple instances and automatically adjust the capacity based on demand. AWS provides Elastic Load Balancing (ELB) and Auto Scaling features to fulfill these requirements.
ELB automatically distributes incoming traffic across multiple instances in different AZs, providing fault tolerance and improving the overall availability of your application. By using Terraform, you can define an ELB and attach it to your instances. Here’s an example of how you can create an ELB in your Terraform configuration file:
resource "aws_elb" "example" { name = "my-elb" availability_zones = ["us-west-2a", "us-west-2b"] listener { instance_port = 80 instance_protocol = "http" lb_port = 80 lb_protocol = "http" } }
Auto Scaling allows you to automatically adjust the number of instances based on predefined conditions, such as CPU utilization or network traffic. By using Terraform, you can define an Auto Scaling group and specify the desired capacity, minimum and maximum number of instances, and scaling policies. Here’s an example of how you can create an Auto Scaling group in your Terraform configuration file:
resource "aws_autoscaling_group" "example" { name = "my-asg" min_size = 2 max_size = 5 desired_capacity = 2 health_check_type = "EC2" availability_zones = ["us-west-2a", "us-west-2b"] launch_template { id = aws_launch_template.example.id version = "$Latest" } }
Database Replication
To ensure high availability for your databases, you can implement replication across multiple AZs. AWS provides the Amazon RDS Multi-AZ feature, which automatically replicates your database instance to a standby instance in a different AZ. This standby instance can be promoted to the primary instance in case of a failure.
In Terraform, you can enable Multi-AZ replication by setting the multi_az
attribute to true
in your RDS resource definition. Here’s an example of how you can create an RDS instance with Multi-AZ replication in your Terraform configuration file:
resource "aws_db_instance" "example" { engine = "mysql" instance_class = "db.t2.micro" allocated_storage = 10 multi_az = true availability_zone = "us-west-2a" # Other configuration attributes... }
Monitoring and Alerting
Monitoring your highly available architecture is essential for detecting and responding to any issues or failures. AWS provides CloudWatch, a monitoring service that collects and tracks metrics, logs, and events from your AWS resources. You can configure CloudWatch alarms to send notifications or trigger automated actions when certain conditions are met, such as CPU utilization exceeding a threshold.
Terraform allows you to define CloudWatch alarms and associate them with specific resources. Here’s an example of how you can create a CloudWatch alarm in your Terraform configuration file:
resource "aws_cloudwatch_metric_alarm" "example" { alarm_name = "high-cpu-utilization" comparison_operator = "GreaterThanOrEqualToThreshold" evaluation_periods = 2 metric_name = "CPUUtilization" namespace = "AWS/EC2" period = 300 statistic = "Average" threshold = 80 alarm_description = "This metric checks CPU utilization" alarm_actions = [aws_sns_topic.example.arn] insufficient_data_actions = [] dimensions { InstanceId = aws_instance.example.id } }
By implementing monitoring and alerting, you can proactively identify and address any issues in your highly available AWS architecture.
Remember to carefully consider your application’s requirements and implement the appropriate design patterns and best practices to achieve high availability in AWS.
Automating Infrastructure Deployment with Terraform
Automating infrastructure deployment is a crucial aspect of managing your AWS resources efficiently. Terraform, an open-source infrastructure as code tool, provides a powerful way to automate the provisioning and management of your infrastructure.
With Terraform, you can define your infrastructure resources in a declarative configuration file, which is written in HashiCorp Configuration Language (HCL). This configuration file, typically named main.tf
, describes the desired state of your infrastructure.
Let’s take a look at an example of a basic Terraform configuration for deploying an EC2 instance in AWS:
provider "aws" { access_key = "" secret_key = "" region = "us-west-2" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" }
In this example, we define an AWS provider and an EC2 instance resource. The provider block specifies the AWS credentials and region to use. The resource block defines an EC2 instance with the specified AMI and instance type.
To deploy this infrastructure, you can run the following Terraform commands:
terraform init terraform plan terraform apply
The terraform init
command initializes the working directory and downloads the necessary provider plugins. The terraform plan
command generates an execution plan, showing the changes that will be applied to your infrastructure. Finally, the terraform apply
command applies the changes and deploys your infrastructure.
Terraform provides several features that make it easy to automate your infrastructure deployment:
- State Management: Terraform maintains a state file that keeps track of the resources it manages. This state file is used to plan and apply changes to your infrastructure.
- Resource Dependencies: You can define dependencies between resources, ensuring that they are created in the correct order.
- Provisioning: Terraform allows you to run scripts or provisioners after creating resources. This enables you to perform additional configuration or setup tasks.
- Modules: You can organize your Terraform code into reusable modules, making it easier to manage and share infrastructure configurations.
By automating your infrastructure deployment with Terraform, you can ensure consistency and repeatability in your infrastructure provisioning process. It also enables you to version control your infrastructure code and collaborate effectively with other team members.
In addition to deploying individual resources, Terraform supports the creation of entire environments or infrastructures. By defining multiple resources in your configuration file, you can easily provision complex infrastructure setups, including networking, security groups, load balancers, and more.
Terraform integrates seamlessly with other AWS services, allowing you to leverage the full power of the AWS ecosystem. Whether you need to deploy a simple EC2 instance or a complex multi-tier application, Terraform provides the flexibility and automation capabilities to streamline your infrastructure deployment on AWS.
Next, we will explore advanced tips and best practices for using Terraform with AWS, including managing sensitive data, handling dependencies, and implementing infrastructure as code principles.
Scaling and Load Balancing in AWS with Terraform
Scaling and load balancing are critical aspects of any infrastructure deployment in AWS. Terraform provides powerful tools and features to help streamline the process and ensure that your application can handle high traffic and workload demands. In this chapter, we will explore advanced tips and techniques for scaling and load balancing in AWS using Terraform.
Auto Scaling Groups
Auto Scaling Groups (ASGs) are a fundamental component of scaling applications in AWS. ASGs automatically adjust the number of instances in response to changing conditions, such as increased traffic or CPU utilization. With Terraform, you can define ASGs and their associated resources, such as launch configurations and load balancers, in a declarative manner.
Let’s take a look at an example of defining an Auto Scaling Group in Terraform:
resource "aws_launch_configuration" "example" { name_prefix = "example" image_id = "ami-12345678" instance_type = "t2.micro" security_groups = [aws_security_group.example.id] } resource "aws_autoscaling_group" "example" { name = "example" launch_configuration = aws_launch_configuration.example.name min_size = 2 max_size = 10 desired_capacity = 2 vpc_zone_identifier = [aws_subnet.example.id] health_check_type = "ELB" health_check_grace_period = 300 tag { key = "Name" value = "example" propagate_at_launch = true } }
In this example, we define an Auto Scaling Group named “example” that uses a launch configuration called “example”. The ASG has a minimum size of 2 instances, a maximum size of 10 instances, and a desired capacity of 2 instances. It is associated with a subnet, and health checks are performed using an Elastic Load Balancer (ELB).
Load Balancers
Load balancers distribute incoming traffic across multiple instances to improve availability and handle high traffic loads. Terraform allows you to define and configure load balancers in AWS, including classic load balancers (ELBv1) and application load balancers (ALB).
Here’s an example of defining an application load balancer in Terraform:
resource "aws_lb" "example" { name = "example" load_balancer_type = "application" subnets = [aws_subnet.example.id] security_groups = [aws_security_group.example.id] enable_deletion_protection = true } resource "aws_lb_target_group" "example" { name = "example" port = 80 protocol = "HTTP" vpc_id = aws_vpc.example.id target_type = "instance" } resource "aws_lb_listener" "example" { load_balancer_arn = aws_lb.example.arn port = 80 protocol = "HTTP" default_action { type = "forward" target_group_arn = aws_lb_target_group.example.arn } }
In this example, we define an application load balancer named “example” that listens on port 80 and forwards traffic to a target group. The load balancer is associated with a subnet and a security group. We also enable deletion protection to prevent accidental deletion of the load balancer.
Scaling Policies
To automatically adjust the capacity of your infrastructure based on demand, you can define scaling policies in Terraform. Scaling policies define the conditions under which instances are added or removed from an Auto Scaling Group.
Here’s an example of defining a scaling policy in Terraform:
resource "aws_autoscaling_policy" "example" { name = "example" autoscaling_group_name = aws_autoscaling_group.example.name adjustment_type = "ChangeInCapacity" scaling_adjustment = 2 }
In this example, we define a scaling policy named “example” that is associated with an Auto Scaling Group. The policy increases the capacity of the ASG by 2 instances when triggered.
Securing Your AWS Infrastructure with Terraform
Securing your AWS infrastructure is of utmost importance to protect your resources and sensitive data. With Terraform, you can implement various security measures to ensure the integrity and confidentiality of your infrastructure. In this chapter, we will explore some advanced tips to secure your AWS infrastructure using Terraform.
1. Implementing IAM Roles and Policies
One of the fundamental aspects of securing your AWS infrastructure is implementing fine-grained access control using IAM (Identity and Access Management) roles and policies. With Terraform, you can define and manage IAM roles and policies as code, ensuring consistent and auditable access management.
To create an IAM role in Terraform, you can use the aws_iam_role
resource type. Here’s an example of creating an IAM role with a policy that allows read-only access to S3:
resource "aws_iam_role" "s3_read_only_role" { name = "s3-read-only-role" assume_role_policy = <<EOF { "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Principal": { "Service": "ec2.amazonaws.com" }, "Action": "sts:AssumeRole" } ] } EOF } resource "aws_iam_policy" "s3_read_only_policy" { name = "s3-read-only-policy" description = "Allows read-only access to S3" policy = <<EOF { "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Action": [ "s3:Get*", "s3:List*" ], "Resource": "*" } ] } EOF } resource "aws_iam_role_policy_attachment" "s3_read_only_attachment" { role = aws_iam_role.s3_read_only_role.name policy_arn = aws_iam_policy.s3_read_only_policy.arn }
This example creates an IAM role named "s3-read-only-role" and attaches a policy named "s3-read-only-policy" to it. The policy allows read-only access to S3.
2. Implementing Network Security
To secure your AWS infrastructure at the network level, you can use Terraform to define and manage security groups, network ACLs (Access Control Lists), and VPC (Virtual Private Cloud) configurations.
For example, to create a security group that allows inbound SSH access from a specific IP range, you can use the aws_security_group
resource type. Here’s an example:
resource "aws_security_group" "ssh_access_sg" { name = "ssh-access-sg" description = "Allow inbound SSH access" ingress { from_port = 22 to_port = 22 protocol = "tcp" cidr_blocks = ["192.168.0.0/24"] } }
This example creates a security group named “ssh-access-sg” that allows inbound SSH access from the IP range “192.168.0.0/24”.
3. Managing Secrets and Sensitive Data
Handling secrets and sensitive data securely is crucial for protecting your AWS infrastructure. Terraform provides several mechanisms to manage secrets, such as using environment variables, input variables, or external tools like HashiCorp Vault.
To manage secrets using environment variables, you can reference them in your Terraform configuration using the var
function. For example:
resource "aws_db_instance" "database" { # ... username = var.db_username password = var.db_password # ... }
In this example, the values for the db_username
and db_password
variables can be provided via environment variables.
Alternatively, you can use input variables to prompt for sensitive information during Terraform execution. For example:
variable "db_password" { type = string description = "The password for the database" sensitive = true } resource "aws_db_instance" "database" { # ... password = var.db_password # ... }
In this case, Terraform will hide the input prompt and mask the password while typing.
4. Continuous Security Monitoring
Ensuring continuous security monitoring of your AWS infrastructure is crucial to detect and respond to any potential security threats. Terraform can integrate with various monitoring and logging tools to provide real-time security insights.
For example, you can use Terraform to configure CloudWatch Logs to collect and analyze logs from your infrastructure. Here’s an example of creating a CloudWatch Log Group:
resource "aws_cloudwatch_log_group" "logs" { name = "/aws/terraform-logs" retention_in_days = 7 }
This example creates a CloudWatch Log Group named “/aws/terraform-logs” with a retention period of 7 days.
By integrating Terraform with monitoring tools like CloudWatch, you can gain visibility into your infrastructure’s security posture and respond to security events effectively.
Implementing these advanced security tips using Terraform will help you secure your AWS infrastructure and protect your resources and data from unauthorized access.
Monitoring and Logging in AWS with Terraform
When deploying infrastructure on AWS using Terraform, it is essential to ensure that your systems are properly monitored and logs are collected. Monitoring allows you to track the health and performance of your resources, while logging enables you to capture important information for troubleshooting and auditing purposes. In this chapter, we will explore various techniques and best practices for monitoring and logging in AWS using Terraform.
CloudWatch Metrics and Alarms
Amazon CloudWatch provides a comprehensive set of monitoring tools to monitor AWS resources and applications in real-time. With Terraform, you can easily configure CloudWatch metrics and alarms to monitor the performance and health of your infrastructure.
To create a CloudWatch metric using Terraform, you can use the aws_cloudwatch_metric_alarm
resource. For example, the following code snippet creates a CloudWatch metric alarm that triggers when the CPU utilization of an EC2 instance exceeds a certain threshold:
resource "aws_cloudwatch_metric_alarm" "example" { alarm_name = "example" comparison_operator = "GreaterThanOrEqualToThreshold" evaluation_periods = "2" metric_name = "CPUUtilization" namespace = "AWS/EC2" period = "60" statistic = "Average" threshold = "90" alarm_description = "This metric checks the CPU utilization of the EC2 instance." alarm_actions = [aws_sns_topic.example.arn] dimensions = { InstanceId = aws_instance.example.id } }
This example creates an alarm that triggers when the average CPU utilization of an EC2 instance exceeds 90% for two consecutive 60-second periods. When the alarm is triggered, it sends a notification to an SNS topic specified by aws_sns_topic.example.arn
.
CloudTrail Logging
AWS CloudTrail provides a detailed history of the API calls made within your AWS account, including who made the call, the source IP address, and when it occurred. By enabling CloudTrail logging, you can gain valuable insights into the activities happening in your infrastructure.
To enable CloudTrail logging using Terraform, you can use the aws_cloudtrail
resource. Here’s an example that creates a CloudTrail trail with an S3 bucket for log storage:
resource "aws_s3_bucket" "example" { bucket = "example-cloudtrail-logs" } resource "aws_cloudtrail" "example" { name = "example" s3_bucket_name = aws_s3_bucket.example.id is_multi_region_trail = true enable_log_file_validation = true include_global_service_events = true tags = { Name = "Example CloudTrail" } }
In this example, a CloudTrail trail named “example” is created, and the logs are stored in an S3 bucket named “example-cloudtrail-logs”. The is_multi_region_trail
attribute is set to true to enable multi-region logging, and enable_log_file_validation
is set to true to ensure the integrity of log files. Additionally, include_global_service_events
is set to true to capture events from all AWS regions.
Centralized Logging with CloudWatch Logs
CloudWatch Logs allows you to collect, monitor, and analyze logs from various AWS resources, such as EC2 instances and Lambda functions. By centralizing your logs in CloudWatch Logs, you can easily search and analyze log data from a single location.
To configure centralized logging with CloudWatch Logs using Terraform, you can use the aws_cloudwatch_log_group
and aws_cloudwatch_log_subscription_filter
resources. Here’s an example that creates a log group and a subscription filter for an EC2 instance:
resource "aws_instance" "example" { // Instance configuration } resource "aws_cloudwatch_log_group" "example" { name = "/aws/ec2/instance/example" retention_in_days = 30 } resource "aws_cloudwatch_log_subscription_filter" "example" { name = "example" log_group_name = aws_cloudwatch_log_group.example.name filter_pattern = "" destination_arn = aws_kinesis_firehose_delivery_stream.example.arn }
In this example, a CloudWatch log group named “/aws/ec2/instance/example” is created, and logs from the EC2 instance are stored in this log group. The retention_in_days
attribute specifies how long the logs should be retained. Additionally, a log subscription filter is created to stream the logs to a Kinesis Data Firehose delivery stream specified by aws_kinesis_firehose_delivery_stream.example.arn
.
Monitoring and logging are critical aspects of managing your infrastructure in AWS. By leveraging Terraform’s capabilities, you can easily configure monitoring metrics, alarms, and logging resources to ensure the health, performance, and security of your AWS resources.
Cost Optimization Techniques with Terraform
As organizations scale their infrastructure in the cloud, managing costs becomes an important consideration. Terraform, with its ability to provision and manage infrastructure as code, offers several techniques to optimize costs in AWS deployments. In this chapter, we will explore some advanced cost optimization techniques with Terraform.
1. Right-sizing Resources:
One of the most effective ways to optimize costs is by right-sizing resources. This involves choosing the appropriate size for your EC2 instances, RDS instances, or any other resource based on their actual usage. By accurately matching resource size with workload requirements, you can avoid over-provisioning and reduce unnecessary costs.
Terraform provides the flexibility to define resource sizes using variables. You can create a variable for the desired instance size and reference it in your Terraform configuration. By easily adjusting this variable, you can experiment with different resource sizes and identify the optimal configuration.
Here’s an example of defining an EC2 instance size in Terraform using variables:
variable "instance_type" { description = "EC2 Instance Type" default = "t2.micro" } resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = var.instance_type // other configuration }
2. Spot Instances:
AWS Spot Instances offer significant cost savings compared to On-Demand or Reserved Instances. These instances are available at a much lower price, with the caveat that they can be interrupted by AWS when demand exceeds supply. However, certain workloads can tolerate interruptions and make good use of Spot Instances.
Terraform allows you to provision Spot Instances by specifying the spot_price
argument in the aws_instance
resource block. By defining a maximum price you are willing to pay for the Spot Instance, you can control costs while still benefiting from the lower prices.
Here’s an example of provisioning a Spot Instance in Terraform:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" spot_price = "0.01" // other configuration }
3. Scheduled Scaling:
Many workloads have predictable patterns of usage, such as increased demand during business hours or specific days of the week. By scaling resources up or down based on these patterns, you can optimize costs without sacrificing performance.
Terraform integrates with AWS Auto Scaling to automate the scaling process. You can define scheduled scaling actions using the aws_autoscaling_schedule
resource block in Terraform. By specifying the desired capacity and the scheduled start and end times, you can control the number of instances running at different times.
Here’s an example of scheduling scaling actions in Terraform:
resource "aws_autoscaling_schedule" "example" { scheduled_action_name = "scale_up" min_size = 1 max_size = 10 desired_capacity = 5 recurrence = "0 9 * * MON-FRI" // other configuration }
4. Resource Tagging:
Properly tagging resources is essential for effective cost management. Tags allow you to categorize resources, track costs, and apply cost-allocation policies. With Terraform, you can easily add tags to your resources using the tags
argument in resource blocks.
Here’s an example of adding tags to an EC2 instance in Terraform:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" tags = { Name = "ExampleInstance" Environment = "Production" // other tags } // other configuration }
5. Cost Explorer Integration:
AWS Cost Explorer provides detailed insights into your AWS costs and usage. By integrating with Cost Explorer, you can analyze and visualize cost data to identify optimization opportunities. Terraform supports the integration with Cost Explorer through the aws_cost_category_rule
resource block.
Here’s an example of integrating with Cost Explorer in Terraform:
resource "aws_cost_category_rule" "example" { rule { rule_name = "ExampleRule" value = "ExampleValue" inherited_value = "ExampleInheritedValue" cost_category_name = "ExampleCategory" } // other configuration }
Implementing these cost optimization techniques with Terraform can help you maximize the value of your AWS infrastructure while minimizing unnecessary expenses. By right-sizing resources, leveraging Spot Instances, scheduling scaling actions, properly tagging resources, and integrating with Cost Explorer, you can achieve significant cost savings in your AWS deployments.
Managing AWS Secrets with Terraform
Managing secrets is a critical aspect of infrastructure deployment, especially when working with cloud platforms like AWS. Terraform provides a convenient and secure way to manage secrets by leveraging its built-in support for environment variables and HashiCorp Vault.
Using Environment Variables
One common approach to managing secrets with Terraform is through environment variables. Environment variables allow you to pass sensitive data to your Terraform scripts without hardcoding them directly in your codebase. This helps keep your secrets secure and prevents accidental exposure.
To use environment variables in your Terraform code, you can reference them using the var.
syntax. For example, if you have an environment variable named AWS_SECRET_ACCESS_KEY
, you can use it in your Terraform configuration like this:
provider "aws" { access_key = var.AWS_ACCESS_KEY_ID secret_key = var.AWS_SECRET_ACCESS_KEY }
By using environment variables, you can store your secrets securely outside of your codebase and easily share them across different environments or teams.
Using HashiCorp Vault
HashiCorp Vault is a popular open-source tool for managing secrets. Terraform provides seamless integration with Vault, allowing you to retrieve secrets dynamically during infrastructure provisioning.
To use Vault with Terraform, you need to authenticate with Vault and retrieve the secrets using its API. Once you have the secrets, you can use them in your Terraform configuration.
Here’s an example of how to retrieve secrets from Vault and use them in your Terraform code:
data "vault_generic_secret" "my_secrets" { path = "secret/aws" } provider "aws" { access_key = data.vault_generic_secret.my_secrets.data["access_key"] secret_key = data.vault_generic_secret.my_secrets.data["secret_key"] }
In this example, we are using the vault_generic_secret
data source to retrieve the secrets stored under the “secret/aws” path in Vault. We then use these secrets to configure the AWS provider.
By using HashiCorp Vault, you can centralize the management of your secrets, enforce access controls, and rotate your secrets regularly for improved security.
Combining Environment Variables and HashiCorp Vault
In some cases, you may want to combine environment variables and HashiCorp Vault to manage your secrets. This allows you to leverage the flexibility of environment variables while still benefiting from the centralized management and access controls provided by Vault.
For example, you can use environment variables as fallback values if the corresponding secrets are not found in Vault. This way, you can provide a seamless experience for developers working in local environments while still enforcing the use of Vault in production.
Here’s an example of how to combine environment variables and Vault in your Terraform code:
data "vault_generic_secret" "my_secrets" { path = "secret/aws" } provider "aws" { access_key = var.AWS_ACCESS_KEY_ID != "" ? var.AWS_ACCESS_KEY_ID : data.vault_generic_secret.my_secrets.data["access_key"] secret_key = var.AWS_SECRET_ACCESS_KEY != "" ? var.AWS_SECRET_ACCESS_KEY : data.vault_generic_secret.my_secrets.data["secret_key"] }
In this example, we first check if the environment variables for the AWS access key and secret key are set. If they are, we use them directly. Otherwise, we retrieve the secrets from Vault using the vault_generic_secret
data source.
By combining environment variables and HashiCorp Vault, you can strike a balance between convenience and security when managing secrets in your Terraform infrastructure.
Deploying Serverless Applications with Terraform
Serverless architectures have gained significant popularity in recent years due to their scalability, cost-effectiveness, and ease of management. Terraform, with its infrastructure-as-code approach, can streamline the deployment of serverless applications on AWS. In this chapter, we will explore advanced tips for deploying serverless applications using Terraform.
Creating AWS Lambda Functions
AWS Lambda is a serverless computing service that allows you to run code without provisioning or managing servers. With Terraform, you can define and deploy Lambda functions as part of your infrastructure.
To create a Lambda function using Terraform, define a resource block of type aws_lambda_function
. Here’s an example of how to create a simple Lambda function that prints “Hello, World!” when invoked:
resource "aws_lambda_function" "hello_world" { function_name = "hello-world" runtime = "python3.8" handler = "index.handler" filename = "hello_world.zip" role = aws_iam_role.lambda_execution_role.arn source_code_hash = filebase64sha256("hello_world.zip") }
In the above example, we specify the function name, runtime (Python 3.8 in this case), handler (the entry point to the function), the filename of the deployment package, and the IAM role for the function’s execution.
Triggering Lambda Functions with AWS API Gateway
AWS API Gateway can be used to create RESTful APIs that trigger Lambda functions. With Terraform, you can define the API Gateway and its associated resources.
To create an API Gateway and configure it to trigger a Lambda function, define a resource block of type aws_api_gateway_rest_api
. Here’s an example:
resource "aws_api_gateway_rest_api" "example" { name = "example-api" description = "Example API" } resource "aws_api_gateway_resource" "example" { rest_api_id = aws_api_gateway_rest_api.example.id parent_id = aws_api_gateway_rest_api.example.root_resource_id path_part = "example" } resource "aws_api_gateway_method" "example" { rest_api_id = aws_api_gateway_rest_api.example.id resource_id = aws_api_gateway_resource.example.id http_method = "POST" authorization = "NONE" } resource "aws_api_gateway_integration" "example" { rest_api_id = aws_api_gateway_rest_api.example.id resource_id = aws_api_gateway_resource.example.id http_method = aws_api_gateway_method.example.http_method type = "AWS_PROXY" uri = aws_lambda_function.hello_world.invoke_arn integration_http_method = "POST" } resource "aws_lambda_permission" "example" { statement_id = "AllowAPIGatewayInvoke" action = "lambda:InvokeFunction" function_name = aws_lambda_function.hello_world.function_name principal = "apigateway.amazonaws.com" source_arn = "${aws_api_gateway_rest_api.example.execution_arn}/*/*/*" }
In this example, we define the API Gateway, a resource within the API, a method (HTTP POST) for the resource, and an integration with the Lambda function. Lastly, we grant permission for the API Gateway to invoke the Lambda function.
Deploying Serverless Applications
To deploy serverless applications with Terraform, you can organize your code into modules. Modules allow you to encapsulate and reuse infrastructure configurations.
Here’s an example directory structure for a serverless application:
serverless-app/ ├── main.tf ├── variables.tf ├── outputs.tf ├── lambda/ │ ├── main.py │ └── requirements.txt └── api/ └── main.tf
In the main.tf
file of the serverless-app
directory, you can define the Lambda function, API Gateway, and any other AWS resources required for your application. The lambda
directory contains the code for the Lambda function, and the api
directory contains the Terraform configuration for the API Gateway.
By organizing your code this way, you can easily manage and deploy your serverless applications using Terraform.
Integrating Terraform with CI/CD Pipelines
Continuous Integration and Continuous Deployment (CI/CD) pipelines have become an essential part of modern software development practices. These pipelines automate the process of building, testing, and deploying code changes, ensuring that software updates are delivered quickly and reliably. Integrating Terraform with CI/CD pipelines allows you to extend this automation to your infrastructure deployments, making it easier to manage and scale your cloud infrastructure on AWS.
Why integrate Terraform with CI/CD pipelines?
Integrating Terraform with CI/CD pipelines brings several benefits to your infrastructure deployment process. By including Terraform code in your pipeline, you can ensure that any changes to your infrastructure are version-controlled, tested, and deployed in a controlled and repeatable manner. This eliminates the need for manual intervention or ad-hoc deployments, reducing the risk of errors and improving consistency.
Setting up your CI/CD pipeline
To integrate Terraform with your CI/CD pipeline, you need to configure your pipeline to execute the necessary Terraform commands. This typically involves setting up a build environment with the required dependencies and executing Terraform commands within that environment.
Here’s an example of a basic CI/CD pipeline configuration using popular tools like Jenkins and AWS CodePipeline:
# .jenkinsfile pipeline { agent any stages { stage('Build') { steps { // Checkout source code from version control git 'https://github.com/your-repo.git' // Install and configure Terraform sh 'curl -O https://releases.hashicorp.com/terraform/0.15.5/terraform_0.15.5_linux_amd64.zip' sh 'unzip terraform_0.15.5_linux_amd64.zip' // Execute Terraform commands sh './terraform init' sh './terraform plan' sh './terraform apply -auto-approve' } } } }
In this example, the pipeline checks out the source code from a Git repository, installs and configures Terraform, and then executes Terraform commands to initialize, plan, and apply changes to the infrastructure.
Best practices for integrating Terraform with CI/CD pipelines
When integrating Terraform with CI/CD pipelines, it’s important to follow some best practices to ensure the reliability and security of your infrastructure deployments:
1. **Use infrastructure as code**: Store your Terraform code in version control alongside your application code. This allows you to track changes, collaborate with team members, and roll back changes if necessary.
2. **Separate environments**: Create separate environments (e.g., development, staging, production) to isolate your infrastructure deployments. This helps prevent accidental changes to production environments and allows for easier testing and validation.
3. **Automate testing**: Incorporate automated testing of your infrastructure deployments into your CI/CD pipeline. This can include running validation tests against your infrastructure or using tools like Terraform’s built-in plan command to detect any potential issues before applying changes.
4. **Secure sensitive information**: Avoid storing sensitive information (e.g., AWS access keys, database passwords) directly in your Terraform code. Instead, use environment variables or a secrets management service to securely store and retrieve this information during the pipeline execution.
5. **Implement rollback mechanisms**: In case of deployment failures or issues, have rollback mechanisms in place to revert to a known good state. This can include using Terraform’s state management features or leveraging infrastructure snapshots.
Troubleshooting and Debugging Terraform Deployments
Deploying infrastructure with Terraform can be a seamless and efficient process, but occasionally you may encounter issues or errors. In this chapter, we will explore some common troubleshooting and debugging techniques to help you overcome these challenges and ensure successful deployments.
1. Understand the Error Messages
When Terraform encounters an error, it provides detailed error messages that can help you understand the issue. It is crucial to carefully read and comprehend these messages to pinpoint the root cause of the problem. The error messages often include information such as the specific resource or configuration that caused the error, along with suggestions for resolving it. By analyzing the error messages, you can quickly identify and rectify the issue.
2. Use Terraform Commands for Debugging
Terraform provides several helpful commands for debugging your deployments. These commands allow you to inspect the current state of your infrastructure, validate your configuration files, and perform other useful tasks. Here are a few commands that can assist you in troubleshooting:
– terraform validate
: This command checks the syntax and validity of your Terraform configuration files. It helps identify any syntax errors or typos in your code.
– terraform plan
: Running this command provides a detailed preview of the changes that Terraform will make to your infrastructure. It allows you to review the planned modifications and catch any potential issues before applying the changes.
– terraform state list
: Use this command to list all the resources managed by Terraform. It helps you understand the current state of your infrastructure and identify any discrepancies or inconsistencies.
– terraform show
: Running this command displays the current state of your infrastructure in a human-readable format. It can be useful for reviewing the current state and comparing it to the desired state defined in your Terraform configuration.
3. Enable Debug Logging
If you are facing complex or persistent issues, enabling debug logging can provide valuable insights into the inner workings of Terraform. By enabling debug logs, you will have access to detailed information about the actions Terraform is performing, the requests it is making to the AWS APIs, and the responses it receives. This can help you understand the sequence of operations and troubleshoot any issues that may arise.
To enable debug logging, set the TF_LOG
environment variable to debug
before running any Terraform commands:
$ export TF_LOG=debug
This will generate verbose logs that can assist you in diagnosing and resolving problems. Remember to disable debug logging when you no longer need it to reduce clutter in your logs.
4. Leverage Terraform Community and Documentation
The Terraform community is vibrant and active, with numerous resources available for troubleshooting and debugging. If you encounter an issue, chances are someone else has faced a similar problem before. Explore the Terraform documentation, user forums, and online communities to find answers to your questions or seek guidance from experienced users.
Additionally, the official Terraform documentation provides detailed explanations of each Terraform resource, data source, and provider. Familiarize yourself with the available documentation to gain a deep understanding of the Terraform ecosystem and its capabilities.
In this chapter, we explored some essential techniques for troubleshooting and debugging Terraform deployments. By understanding error messages, using Terraform commands effectively, enabling debug logging, and leveraging the Terraform community and documentation, you will be well-equipped to overcome any challenges that arise during your infrastructure deployment journey.