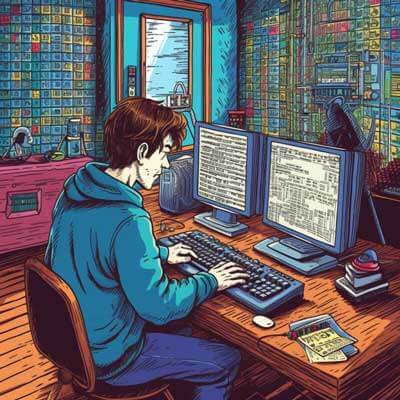
Table of Contents
Benefits of Automation in Software Development
Automation plays a critical role in software development, particularly in the context of DevOps. By automating various tasks and processes, software teams can streamline their workflows, increase efficiency, and reduce errors. Here are some key benefits of automation in software development:
1. Improved productivity: Automation eliminates the need for manual, repetitive tasks, allowing developers to focus on more valuable activities. This leads to increased productivity and faster delivery of software.
2. Consistency and reproducibility: Automation ensures that the same steps are followed every time, reducing the risk of human error and ensuring consistent results. This is particularly important in tasks like building and deploying software.
Example: Continuous Integration (CI) is a common practice in DevOps that involves automatically building and testing software whenever changes are made to the code repository. Tools like Jenkins can be used to automate the CI process. Below is an example of a Jenkinsfile, which defines the CI pipeline using Jenkins:
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean package' } } stage('Test') { steps { sh 'mvn test' } } stage('Deploy') { steps { sh 'mvn deploy' } } } }
3. Reduced time to market: Automation helps in accelerating the software development lifecycle, enabling organizations to release new features and updates more quickly. This gives businesses a competitive edge by allowing them to respond to market demands faster.
4. Improved quality: Automation allows for the implementation of rigorous testing and quality control processes. By automating code quality checks, testing, and monitoring, issues can be identified and resolved early in the development cycle, leading to higher-quality software.
Related Article: The Path to Speed: How to Release Software to Production All Day, Every Day (Intro)
Streamlining Continuous Integration with Automation
Continuous Integration (CI) is a key practice in DevOps that aims to merge code changes frequently and automatically test them to catch integration errors early. Automation plays a vital role in streamlining the CI process. Here are two examples of how automation can be used to streamline CI:
1. Automated Build and Test: Tools like Jenkins, TeamCity, and Travis CI can be used to automate the build and test process. These tools can be configured to monitor the code repository and trigger a build and test whenever changes are detected.
Example: In Jenkins, a build job can be configured to automatically trigger whenever a new commit is pushed to the repository. The job can be set up to run the build commands and execute the test suite. Here is an example of a Jenkins job configuration:
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean package' } } stage('Test') { steps { sh 'mvn test' } } } }
2. Automated Code Review: Code review is an essential part of the CI process to ensure the quality and maintainability of the codebase. Manual code reviews can be time-consuming and error-prone. Automation can help by automatically analyzing code and providing feedback on issues like coding standards violations, potential bugs, and security vulnerabilities.
Example: Tools like SonarQube and CodeClimate can be integrated into the CI pipeline to perform automated code review. These tools analyze the codebase and generate reports highlighting issues and providing recommendations for improvement.
Automating Code Quality Checks
Code quality is crucial for the success of any software project. Automating code quality checks helps ensure that coding standards are followed, potential bugs are detected, and the overall maintainability of the codebase is improved. Here are two examples of code quality checks that can be automated:
1. Linting: Linting is the process of analyzing code for potential errors, bugs, and stylistic issues. By automating the linting process, developers can ensure that the codebase adheres to coding standards and best practices.
Example: In Python, tools like Flake8 and Pylint can be used to automate the linting process. These tools analyze the code and provide feedback on issues like syntax errors, unused variables, and code complexity. Here is an example of using Flake8 in a Python project:
$ flake8 myproject
2. Static Code Analysis: Static code analysis involves analyzing the codebase for potential bugs, security vulnerabilities, and other issues without actually executing the code. By automating static code analysis, developers can identify potential issues early in the development process.
Example: SonarQube is a popular tool for automating static code analysis. It supports multiple programming languages and provides a comprehensive set of rules to detect issues like code smells, security vulnerabilities, and potential bugs. Here is an example of running SonarQube analysis on a Java project:
$ sonar-scanner
Leveraging Automation for Deployment and Release Management
Deployment and release management are critical aspects of the software development lifecycle. Automation can greatly simplify and streamline these processes, leading to faster and more reliable deployments. Here are two examples of how automation can be leveraged for deployment and release management:
1. Automated Deployment: Automating the deployment process reduces the risk of errors and ensures consistency across different environments. Tools like Ansible, Puppet, and Chef can be used to automate the provisioning and configuration of servers and the deployment of software.
Example: Ansible is a popular tool for automating deployment and configuration management. It uses a declarative language to define the desired state of the system, and then performs the necessary steps to achieve that state. Here is an example of an Ansible playbook for deploying a web application:
- name: Deploy web application hosts: webservers tasks: - name: Copy application code copy: src: /path/to/application dest: /var/www/html - name: Restart web server service: name: nginx state: restarted
2. Release Automation: Release automation involves automating the process of releasing software to production. This includes tasks like creating release packages, managing version numbers, and coordinating the deployment of new releases.
Example: Tools like Jenkins and GitLab CI/CD provide built-in support for release automation. They allow for the automation of tasks like creating release branches, generating release packages, and triggering deployment pipelines. Here is an example of a Jenkins pipeline for release automation:
pipeline { agent any stages { stage('Build') { steps { sh 'mvn clean package' } } stage('Test') { steps { sh 'mvn test' } } stage('Release') { steps { sh 'mvn release:prepare release:perform' } } stage('Deploy') { steps { sh 'mvn deploy' } } } }
Related Article: How to use AWS Lambda for Serverless Computing
Enhancing Testing Efficiency through Automation
Testing is a critical aspect of software development, and automation can greatly enhance testing efficiency. By automating various testing tasks, developers can save time, reduce errors, and improve the overall quality of the software. Here are two examples of how automation can enhance testing efficiency:
1. Automated Unit Testing: Unit testing involves testing individual units of code in isolation to ensure their correctness. Automating unit testing allows for faster and more frequent execution of tests, enabling developers to catch issues early in the development process.
Example: Testing frameworks like JUnit for Java and pytest for Python provide support for automated unit testing. These frameworks allow developers to define test cases and assertions, and then run the tests automatically. Here is an example of a JUnit test case in Java:
import org.junit.jupiter.api.Test; import static org.junit.jupiter.api.Assertions.*; public class MyMathTest { @Test public void testAdd() { MyMath math = new MyMath(); int result = math.add(2, 3); assertEquals(5, result); } }
2. Automated Integration Testing: Integration testing involves testing the interaction between different components of a system to ensure their proper integration. Automating integration testing allows for the creation of comprehensive test suites that can be executed automatically, reducing the effort required for manual testing.
Example: Tools like Selenium and Cypress can be used to automate integration testing of web applications. These tools simulate user interactions and validate the behavior of the application. Here is an example of a Selenium test in Java:
import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.By; import org.openqa.selenium.WebElement; public class SeleniumTest { public static void main(String[] args) { // Set path to chromedriver executable System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver"); // Create a new instance of the Chrome driver WebDriver driver = new ChromeDriver(); // Navigate to the application driver.get("https://example.com"); // Find the search input element WebElement searchInput = driver.findElement(By.name("q")); // Enter a search query searchInput.sendKeys("Hello, World!"); // Submit the form searchInput.submit(); // Wait for the search results page to load driver.findElement(By.id("search-results")); // Close the browser driver.quit(); } }
Automating Infrastructure Provisioning and Configuration
Provisioning and configuring the necessary infrastructure for software development and deployment can be a complex and time-consuming process. Automation can greatly simplify this process, allowing developers to provision and configure infrastructure resources on-demand. Here are two examples of how automation can be used for infrastructure provisioning and configuration:
1. Infrastructure as Code: Infrastructure as Code (IaC) is an approach to infrastructure provisioning and management that uses code to define and manage infrastructure resources. By using IaC tools, developers can define the desired state of the infrastructure in code, and the tools will automatically provision and configure the necessary resources.
Example: Tools like Terraform and AWS CloudFormation provide support for infrastructure provisioning using code. These tools allow developers to define the desired infrastructure resources, such as virtual machines, networks, and storage, in a declarative language. Here is an example of a Terraform configuration file for provisioning an AWS EC2 instance:
resource "aws_instance" "example" { ami = "ami-0c94855ba95c71c99" instance_type = "t2.micro" tags = { Name = "Example Instance" } }
2. Configuration Management: Configuration management tools allow for the automation of software configuration tasks, such as installing packages, configuring services, and managing system settings. These tools ensure consistency and repeatability in the configuration of infrastructure resources.
Example: Tools like Ansible, Puppet, and Chef provide support for configuration management. They allow developers to define the desired configuration of infrastructure resources in code, and the tools will automatically apply the configuration to the resources. Here is an example of an Ansible playbook for configuring an Nginx web server:
- name: Configure Nginx hosts: webservers tasks: - name: Install Nginx apt: name: nginx state: present - name: Copy Nginx configuration file copy: src: nginx.conf dest: /etc/nginx/nginx.conf - name: Restart Nginx service: name: nginx state: restarted
Monitoring and Alerting Automation in DevOps
Monitoring and alerting are crucial for ensuring the availability and performance of software systems. Automation can greatly simplify and enhance the monitoring and alerting process in DevOps. Here are two examples of how automation can be used for monitoring and alerting:
1. Automated Monitoring: Monitoring tools can be used to collect and analyze data about the performance and health of software systems. By automating the monitoring process, developers can gain real-time insights into the behavior of their systems and detect issues before they impact the users.
Example: Tools like Prometheus and Nagios provide support for automated monitoring. These tools can be configured to collect metrics from various sources, such as servers, applications, and databases, and generate alerts based on predefined thresholds. Here is an example of a Prometheus configuration file for monitoring a web server:
scrape_configs: - job_name: 'web_server' metrics_path: '/metrics' static_configs: - targets: ['localhost:8080']
2. Automated Alerting: Alerting systems notify the relevant stakeholders when certain predefined conditions are met. By automating the alerting process, developers can ensure that the right people are notified promptly when issues occur, enabling them to take immediate action.
Example: Tools like Grafana and PagerDuty provide support for automated alerting. These tools can be configured to monitor predefined metrics and send alerts when certain thresholds are exceeded. Here is an example of a Grafana alert configuration:
{ "alert": { "name": "High CPU Usage", "message": "CPU usage is above the threshold", "conditions": [ { "evaluator": { "type": "gt", "params": [90] }, "reducer": { "type": "avg", "params": [], }, }, ], "notifications": [ { "type": "email", "settings": { "addresses": ["admin@example.com"] } } ] } }
Automating Log Management and Analysis
Log management and analysis are crucial for troubleshooting and understanding the behavior of software systems. Automation can simplify the log management process and enable efficient log analysis. Here are two examples of how automation can be used for log management and analysis:
1. Centralized Log Collection: By automating the collection of logs from various sources, developers can have a centralized view of the logs, making it easier to analyze and troubleshoot issues. Tools like Elasticsearch, Logstash, and Kibana (ELK stack) can be used for centralized log collection and analysis.
Example: The ELK stack provides a comprehensive solution for log management and analysis. It consists of Elasticsearch, which is used for indexing and searching logs; Logstash, which is used for log collection and parsing; and Kibana, which is used for log visualization and analysis. Here is an example of a Logstash configuration file for collecting logs from a web server:
input { file { path => "/var/log/nginx/access.log" start_position => "beginning" } } output { elasticsearch { hosts => ["localhost:9200"] index => "nginx-access-%{+YYYY.MM
Related Article: Intro to Security as Code
Additional Resources
- Continuous Integration in DevOps Automation