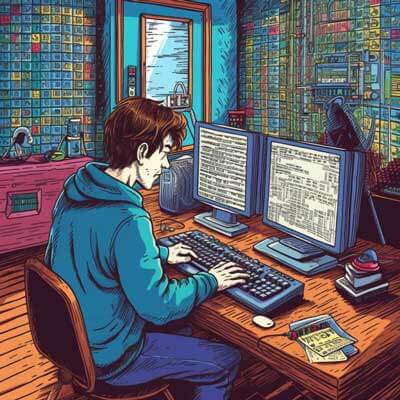
- Understanding Monolithic Architecture
- Benefits of Microservices
- 1. Scalability and Flexibility
- 2. Improved Fault Isolation
- 3. Independent Deployment
- 4. Team Autonomy and Productivity
- 5. Enhanced Scalability with Cloud-Native Technologies
- 6. Simplified Testing and Debugging
- Use Cases for Microservices
- 1. Scalability
- 2. Independent Deployment
- 3. Technology Diversity
- 4. Maintainability
- Real World Examples of Migrating to Microservices
- Breaking Down the Monolith
- Designing Microservices
- 1. Identifying Microservices
- 2. Defining Service Contracts
- 3. Ensuring Data Consistency
- 4. Implementing Communication
- 5. Scaling and Deployment
- Communication Between Microservices
- Scaling Microservices
- Horizontal Scaling
- Vertical Scaling
- Database Scaling
- Monitoring and Autoscaling
- Monitoring and Logging in a Microservices Architecture
- Why Monitoring and Logging Matter in Microservices
- Implementing Monitoring in a Microservices Architecture
- Implementing Logging in a Microservices Architecture
- Handling Data in Microservices
- Security Considerations for Microservices
- Testing Microservices
- Unit Testing
- Integration Testing
- End-to-End Testing
- Challenges in Testing Microservices
- Deploying Microservices
- Containerization
- Orchestration
- Continuous Integration and Deployment (CI/CD)
- Monitoring and Logging
- Managing Microservices in Production
- Service Discovery
- Monitoring and Logging
- Scaling and Load Balancing
- Fault Tolerance and Resilience
- Continuous Integration and Deployment
- Advanced Techniques for Microservices
- Service Discovery
- Circuit Breaker Pattern
- Event-Driven Architecture
- Containerization and Orchestration
- Building Resilient Microservices
- Circuit Breaker Pattern
- Retry Mechanisms
- Timeouts and Timeouts
- Monitoring and Alerting
- Using Containers and Orchestration
- Event-Driven Architecture with Microservices
- Building a Gateway for Microservices
- Why do we need a gateway?
- Key features of a gateway
- Implementing a gateway
- Caching Strategies in a Microservices Environment
- Client-side Caching
- Server-side Caching
- Distributed Caching
- Cache Invalidation
- Integrating Microservices with Legacy Systems
- Understanding Legacy Systems
- Identifying Integration Points
- Implementing Integration Patterns
- Testing and Monitoring
- Gradual Decomposition
Understanding Monolithic Architecture
Monolithic architecture is a traditional software development approach where an application is built as a single, self-contained unit. In this design pattern, all the components of the application, such as the user interface, business logic, and data access layer, are tightly coupled and deployed as one unit.
The monolithic architecture has been widely used for decades and has its advantages. It is relatively easy to develop and test, as all the components are developed together and run in the same environment. Additionally, monolithic applications can be deployed on a single server, making it simpler to manage and scale.
However, as applications grow in size and complexity, monolithic architecture can become challenging to maintain and evolve. Some common issues with monolithic architecture include:
1. Codebase Size: As all the components of the application are bundled together, the codebase tends to grow larger over time. This can make it difficult for developers to understand and modify the codebase efficiently.
2. Scalability: Scaling a monolithic application can be challenging. If one component of the application requires more resources, the entire application needs to be scaled, even if other components don’t require additional resources. This can lead to inefficient resource utilization.
3. Deployment Complexity: Deploying changes to a monolithic application can be complex and risky. A small change in one component might require redeploying the entire application, causing downtime and potential disruptions.
4. Technology Stack Limitations: Monolithic applications often rely on a specific technology stack, making it difficult to adopt new technologies or frameworks. Upgrading one component of the application might require upgrading the entire application, which can be time-consuming and costly.
To illustrate the concept of monolithic architecture, let’s consider a simple web application that allows users to create and manage tasks. In a monolithic design, the application might have the following structure:
my-app/ ├── index.html ├── styles.css ├── app.js └── database.js
In this example, all the components of the application, including the user interface (index.html and styles.css), business logic (app.js), and data access layer (database.js), are bundled together in a single codebase.
As the application grows and evolves, adding new features or making changes to existing ones becomes more challenging. Developers need to be cautious about introducing changes that might impact the entire application.
In the next chapter, we will explore the benefits of migrating from monolithic architecture to microservices and how it can address the limitations of the monolithic approach.
Related Article: Attributes of Components in a Microservice Architecture
Benefits of Microservices
Microservices architecture has gained popularity in recent years due to its numerous benefits. In this chapter, we will explore the advantages of adopting microservices for your software architecture.
1. Scalability and Flexibility
One of the key benefits of microservices is its ability to scale and adapt to changing demands. With a monolithic architecture, scaling the entire application can be challenging and often leads to over-provisioning of resources. In contrast, microservices allow you to scale specific services independently, based on their individual needs. This granular scalability helps optimize resource utilization and ensures that your application can handle increased traffic or workload efficiently.
Additionally, microservices provide flexibility in technology choice. Each service can be developed using different programming languages, frameworks, and databases, based on the specific requirements. This flexibility allows teams to choose the best tool for the job, enabling them to leverage the strengths of different technologies within the same application.
2. Improved Fault Isolation
In a monolithic architecture, a single bug or failure in one component can bring down the entire application. Microservices, on the other hand, isolate failures to individual services. This fault isolation ensures that failures are contained within a service, minimizing the impact on other parts of the application. By separating services, you can improve the overall resilience and availability of your system.
Related Article: Mastering Microservices: A Comprehensive Guide to Building Scalable and Agile Applications
3. Independent Deployment
Microservices enable independent deployment of individual services. This decoupling allows teams to deploy updates, bug fixes, and new features to specific services without affecting the entire application. With a monolithic architecture, any change requires redeploying the entire application, causing downtime and potential disruptions. Independent deployment not only reduces the risk of errors but also enables faster release cycles and continuous delivery.
4. Team Autonomy and Productivity
Microservices promote team autonomy and productivity. Each service can be developed, tested, and deployed independently by a dedicated team. This autonomy allows teams to work at their own pace, using their preferred technologies and practices. As a result, development cycles can be faster, and teams can focus on their specific domain expertise. Additionally, microservices simplify collaboration as teams can work in parallel, reducing dependencies and enabling faster innovation.
5. Enhanced Scalability with Cloud-Native Technologies
Microservices architecture aligns well with cloud-native technologies and practices. Cloud platforms provide built-in support for scaling, monitoring, and managing microservices-based applications. By leveraging containerization and orchestration tools like Docker and Kubernetes, you can easily scale your services based on demand, deploy them across multiple environments, and ensure high availability.
For example, using Kubernetes, you can define a deployment manifest like the following to deploy a microservice:
apiVersion: apps/v1 kind: Deployment metadata: name: my-service spec: replicas: 3 selector: matchLabels: app: my-service template: metadata: labels: app: my-service spec: containers: - name: my-service image: my-service:latest ports: - containerPort: 8080
6. Simplified Testing and Debugging
Microservices architecture simplifies testing and debugging. With smaller, independent services, it becomes easier to write focused unit tests and perform end-to-end testing. Each service can be tested in isolation, mocking dependencies as needed, enabling faster feedback and reducing the risk of regressions.
Debugging is also simplified as failures are contained within a service. When an issue arises, you can focus on the specific service, rather than navigating through a monolithic codebase. This targeted debugging approach reduces the time and effort required to identify and fix issues.
In conclusion, microservices architecture offers several benefits, including scalability, flexibility, fault isolation, independent deployment, team autonomy, enhanced scalability with cloud-native technologies, and simplified testing and debugging. These benefits make microservices an attractive choice for modern software architectures.
Use Cases for Microservices
Microservices have gained popularity in recent years due to their ability to simplify complex software architectures and improve scalability and resilience. In this chapter, we will explore some common use cases where microservices can be beneficial.
1. Scalability
One of the primary reasons for adopting microservices is to achieve scalability. By breaking down a monolithic application into smaller, loosely coupled services, we can scale individual components independently. This allows us to allocate resources efficiently and handle high traffic loads more effectively.
For example, let’s consider an e-commerce platform. By using microservices, we can scale the inventory management service separately from the order processing service. During peak shopping seasons, when the number of orders increases significantly, we can allocate more resources to the order processing service to handle the load without affecting other services.
Here’s an example of how the order processing service might look as a microservice:
// order-processing-service.java @RestController public class OrderController { @PostMapping("/orders") public ResponseEntity<String> createOrder(@RequestBody Order order) { // Process the order and return a response } }
2. Independent Deployment
Microservices enable independent deployment of individual components, allowing teams to work autonomously without interfering with each other’s release cycles. This decoupling of services reduces the risk of deployment failures and allows for faster iterations.
Consider a social media platform where multiple teams are responsible for different features like user authentication, newsfeed, messaging, and notifications. With microservices, each team can deploy their respective services independently, as long as the necessary contracts and APIs are defined. This promotes faster development and enables teams to respond to user feedback more efficiently.
Here’s an example of a user authentication microservice:
# user-authentication-service.py @app.route("/login", methods=["POST"]) def login(): # Authenticate the user and generate a JWT token pass
3. Technology Diversity
Microservices allow for technology diversity within an application, enabling teams to choose the most suitable technology stack for their specific service. This flexibility allows organizations to leverage the strengths of different programming languages, frameworks, and tools.
For instance, a media streaming platform might have a recommendation service that requires complex machine learning algorithms. By implementing this service as a microservice, the team can choose a language like Python, which has extensive libraries for machine learning. Meanwhile, other services can be written in languages like Java or Go, depending on the team’s expertise and requirements.
Here’s an example of a recommendation microservice using Python:
# recommendation-service.py class RecommendationService: def get_recommendations(self, user_id): # Retrieve personalized recommendations based on user preferences and behavior pass
4. Maintainability
Breaking down a monolithic application into smaller services enhances maintainability. Each microservice can be developed, tested, and maintained independently, making it easier to identify and fix issues. Additionally, smaller codebases are generally easier to understand and refactor.
Consider a banking application that handles various functionalities such as account management, transaction processing, and customer support. By decomposing these functionalities into separate microservices, developers can focus on specific areas without worrying about the entire application. This isolation simplifies testing, debugging, and updating individual services without disrupting the overall system.
Here’s an example of a transaction processing microservice:
// transaction-processing-service.ts export class TransactionService { public processTransaction(transaction: Transaction): void { // Process the transaction and update account balances } }
Microservices offer numerous benefits in terms of scalability, independent deployment, technology diversity, and maintainability. However, it’s important to carefully evaluate the requirements and complexities of your application before deciding to migrate from a monolithic architecture. In the next chapter, we will discuss the challenges and considerations involved in migrating from a monolith to microservices architecture.
Real World Examples of Migrating to Microservices
In this chapter, we will explore real-world examples of organizations that have successfully migrated from a monolithic architecture to a microservices architecture. These examples will provide valuable insights into the challenges faced and the strategies employed during the migration process.
1. Netflix: Netflix is a prime example of a company that successfully migrated its monolithic architecture to a microservices-based architecture. To handle the scale and complexity of their streaming platform, Netflix implemented a microservices architecture that allowed them to decouple their systems and scale individual components independently. This migration enabled them to achieve continuous delivery, fault tolerance, and scalability.
2. Amazon: Amazon, one of the largest e-commerce platforms in the world, also adopted a microservices architecture to scale and improve their system’s performance. By breaking down their monolithic application into smaller, independent services, Amazon was able to increase development speed, achieve better fault isolation, and improve system resilience.
3. Uber: Uber, the ride-hailing giant, faced challenges with their monolithic architecture as their platform grew rapidly. To overcome these challenges, they migrated to a microservices architecture. By breaking down their monolithic application into smaller services, Uber was able to develop and deploy new features faster, improve scalability, and enhance the overall reliability of their platform.
4. Spotify: Spotify, a popular music streaming service, also adopted a microservices architecture to improve their system’s performance and scalability. By breaking down their monolithic application into smaller, specialized services, Spotify was able to enhance their development speed, achieve better fault tolerance, and enable independent deployment of services.
These real-world examples highlight the advantages of migrating from a monolithic architecture to a microservices architecture. However, it’s important to note that the migration process can be complex and challenging. Organizations need to carefully plan and execute their migration strategy to ensure a smooth transition.
To give you a taste of how a migration from monolith to microservices could look like, here’s a code snippet that demonstrates a basic microservice written in Node.js:
// orders-service.js const express = require('express'); const app = express(); const port = 3000; app.get('/orders', (req, res) => { // Fetch orders from the database const orders = [ { id: 1, item: 'Product A' }, { id: 2, item: 'Product B' }, { id: 3, item: 'Product C' } ]; res.json(orders); }); app.listen(port, () => { console.log(`Orders service listening at http://localhost:${port}`); });
This code snippet demonstrates a simple orders microservice that exposes an API endpoint to fetch orders from a database. By breaking down a monolithic application into smaller, independent services like this, organizations can achieve greater flexibility, scalability, and maintainability.
In the next chapter, we will explore the challenges and considerations involved in migrating from monolith to microservices architecture.
Breaking Down the Monolith
The first step in migrating from a monolithic architecture to a microservices architecture is to break down the monolith into smaller, more manageable components. This allows for easier development, deployment, and scalability of individual services.
One approach to breaking down a monolith is to identify cohesive areas of functionality within the application and extract them into separate services. For example, if you have an e-commerce application, you might have a monolithic application that handles both product inventory management and order processing. By breaking these functionalities into separate services, you can decouple the code and scale each service independently.
To start the process of breaking down the monolith, you need to analyze the existing codebase and identify potential areas for extraction. Look for modules or components that can be isolated and encapsulated as separate services. These areas should have clear boundaries and minimal dependencies on other parts of the monolith.
Once you have identified the areas for extraction, you can begin the process of refactoring the code. This involves extracting the relevant code and dependencies into separate modules or packages. Depending on the complexity of the monolith, this process can be time-consuming and requires careful planning to ensure that all dependencies are properly managed.
Here’s an example of how you can extract a module from a monolithic codebase:
// Monolithic code public class ProductService { private InventoryService inventoryService; private OrderService orderService; public ProductService() { this.inventoryService = new InventoryService(); this.orderService = new OrderService(); } public void processOrder(Order order) { if (inventoryService.checkInventory(order)) { orderService.placeOrder(order); // ... } else { // ... } } } // Extracted module public class OrderService { public void placeOrder(Order order) { // ... } } public class InventoryService { public boolean checkInventory(Order order) { // ... } }
In this example, we extract the OrderService
and InventoryService
from the ProductService
class. This allows us to manage the order processing and inventory management as separate services.
Once you have extracted the relevant modules or components, you can package them as separate services. These services can be deployed and scaled independently, allowing for greater flexibility and agility in your architecture.
Breaking down a monolith is not a one-time process. It requires ongoing maintenance and refactoring as the application evolves. However, by breaking down the monolith into smaller, more manageable components, you can simplify your software architecture and pave the way for a successful migration to a microservices architecture.
Designing Microservices
When migrating from a monolithic architecture to microservices, designing the new microservices is a critical step. This chapter will guide you through the process of designing microservices that are efficient, scalable, and easy to maintain.
1. Identifying Microservices
The first step in designing microservices is to identify the boundaries of each microservice. This involves analyzing your monolithic application and identifying distinct areas of functionality that can be decoupled into separate services. Consider the Single Responsibility Principle (SRP) and aim for high cohesion within each microservice.
For example, in an e-commerce application, you might have microservices for user management, product catalog, order management, and payment processing. Each microservice should have a clearly defined responsibility and provide a well-defined API.
2. Defining Service Contracts
Once you have identified the microservices, the next step is to define the service contracts. Service contracts define the communication between microservices, including the data format and the APIs.
A common approach is to use RESTful APIs with JSON as the data format. This allows for loose coupling between microservices and enables them to evolve independently. You can also consider using GraphQL or gRPC for more complex scenarios.
Here’s an example of a RESTful API contract for a user management microservice:
GET /users/{id} { "id": "123", "name": "John Doe", "email": "john.doe@example.com" }
3. Ensuring Data Consistency
In a monolithic architecture, data consistency is usually maintained within a single database transaction. However, in a microservices architecture, each microservice has its own database, making it more challenging to ensure data consistency across services.
There are several strategies to address this challenge, including:
– Using a distributed transaction management system, such as Sagas or the Two-Phase Commit protocol.
– Implementing eventual consistency by propagating changes asynchronously and handling conflicts.
– Applying the event sourcing pattern, where changes are recorded as a sequence of events and can be replayed to rebuild state.
Choose the strategy that best fits your application’s requirements and complexity.
4. Implementing Communication
Microservices need to communicate with each other to fulfill their responsibilities. There are different communication patterns you can use, such as synchronous HTTP calls, asynchronous messaging, or event-driven architectures.
For synchronous communication, you can use RESTful APIs or gRPC. Here’s an example of a HTTP call from a product catalog microservice to a user management microservice:
GET /users/123
For asynchronous communication, you can use message brokers like RabbitMQ or Apache Kafka. Here’s an example of sending a message to a payment processing microservice:
Message message = new Message("PaymentCreated", paymentData); messageBroker.send(message);
5. Scaling and Deployment
Microservices allow for independent scaling and deployment, which can improve performance and availability. Consider the scaling requirements of each microservice and choose appropriate deployment strategies, such as containerization with Docker and orchestration with Kubernetes.
Ensure that your microservices are stateless and can be easily replicated to handle increased load. Use load balancers to distribute traffic across multiple instances of a microservice.
In the next chapter, we will dive into the implementation details of migrating a monolithic application to microservices. Stay tuned!
Communication Between Microservices
In a microservices architecture, communication between services is a critical aspect to ensure the overall functionality of the system. Microservices need to interact with each other to exchange data, trigger actions, and maintain consistency across the distributed system. There are various communication patterns and strategies that can be employed to achieve this.
Synchronous Communication
One of the most common ways for microservices to communicate with each other is through synchronous communication. This involves one service sending a request to another service and waiting for a response before proceeding. This type of communication is typically done over HTTP using RESTful APIs.
Here is an example of how a microservice can make a synchronous HTTP request to another microservice using the Python programming language:
import requests response = requests.get('http://example.com/api/users/123') data = response.json() # Process the data received from the microservice
Asynchronous Communication
Another approach to communication between microservices is through asynchronous communication. In this pattern, a service sends a message to a message broker or a message queue, and other services can consume these messages asynchronously. This decouples the sender and receiver, allowing services to operate independently.
One popular messaging system used for asynchronous communication is Apache Kafka. Here’s an example of publishing a message to a Kafka topic using the Java programming language:
import org.apache.kafka.clients.producer.KafkaProducer; import org.apache.kafka.clients.producer.ProducerRecord; Properties properties = new Properties(); properties.put("bootstrap.servers", "localhost:9092"); properties.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); properties.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); KafkaProducer<String, String> producer = new KafkaProducer<>(properties); ProducerRecord<String, String> record = new ProducerRecord<>("my-topic", "key", "value"); producer.send(record); producer.close();
Event-Driven Communication
Event-driven communication is another popular pattern for microservices. It involves services publishing events, and other services subscribing to these events and reacting accordingly. This pattern enables loose coupling between services and allows for scalability and extensibility.
One technology commonly used for event-driven communication is Apache Kafka. Here’s an example of subscribing to events from a Kafka topic using the Java programming language:
import org.apache.kafka.clients.consumer.KafkaConsumer; import org.apache.kafka.clients.consumer.ConsumerRecord; import org.apache.kafka.clients.consumer.ConsumerRecords; Properties properties = new Properties(); properties.put("bootstrap.servers", "localhost:9092"); properties.put("group.id", "my-group"); properties.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); properties.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); KafkaConsumer<String, String> consumer = new KafkaConsumer<>(properties); consumer.subscribe(Collections.singletonList("my-topic")); while (true) { ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100)); for (ConsumerRecord<String, String> record : records) { // Process the event received from the Kafka topic } } consumer.close();
API Gateways
In a microservices architecture, an API gateway can be used to provide a single entry point for clients to interact with the system. The API gateway handles requests from clients and routes them to the appropriate microservices. It can also perform authentication, rate limiting, caching, and other cross-cutting concerns.
One popular API gateway solution is Kong. It acts as an intermediary between clients and microservices, providing a unified interface. Here’s an example of how an API gateway can route a request to a microservice:
GET /api/users/123 HTTP/1.1 Host: example.com HTTP/1.1 200 OK Content-Type: application/json { "id": 123, "name": "John Doe", "email": "john.doe@example.com" }
Scaling Microservices
Scaling microservices is crucial to handle increased traffic and ensure high availability of your application. As your user base grows, your system needs to be able to handle the load efficiently. In this chapter, we will explore different approaches to scaling microservices and discuss best practices.
Horizontal Scaling
One common approach to scaling microservices is horizontal scaling, where you add more instances of a service to distribute the load. This can be achieved by running multiple copies of the same microservice on separate servers or containers. By load balancing the requests across these instances, you can handle a higher number of concurrent users.
Here’s an example of how you can horizontally scale a microservice using Kubernetes:
apiVersion: apps/v1 kind: Deployment metadata: name: my-service spec: replicas: 3 selector: matchLabels: app: my-service template: metadata: labels: app: my-service spec: containers: - name: my-service image: my-service:latest ports: - containerPort: 8080
In the above example, we define a Kubernetes Deployment with three replicas of our microservice. Kubernetes will automatically distribute the load across these replicas, ensuring high availability.
Vertical Scaling
Vertical scaling involves increasing the resources allocated to a single instance of a microservice. This can be achieved by upgrading the hardware or increasing the allocated memory and CPU of the host machine.
While vertical scaling provides a simpler approach, it has limitations in terms of scalability. There is a limit to how much a single instance can handle, and eventually, you may need to resort to horizontal scaling.
Database Scaling
As microservices often rely on databases, scaling your database is also important. There are several approaches to database scaling, depending on the type of database you are using.
For relational databases, you can consider techniques like database sharding or replication. Sharding involves partitioning your data across multiple database instances, while replication involves maintaining multiple copies of the same database for high availability.
NoSQL databases like MongoDB often support horizontal scaling out of the box. By adding more nodes to your database cluster, you can handle increased read and write traffic.
Monitoring and Autoscaling
To ensure your microservices are always available and performing well, it is important to monitor their health and automatically scale them based on demand.
Monitoring tools like Prometheus or Datadog can help you collect metrics and monitor the performance of your microservices. By setting up alerts, you can proactively detect any issues and take necessary action.
Autoscaling frameworks like Kubernetes Horizontal Pod Autoscaler (HPA) can automatically scale your microservices based on predefined metrics such as CPU or memory utilization. This allows your system to dynamically adapt to changing traffic patterns.
Monitoring and Logging in a Microservices Architecture
Monitoring and logging are critical components of any software architecture, and a microservices architecture is no exception. In fact, with the increased complexity and distributed nature of microservices, effective monitoring and logging become even more important.
Why Monitoring and Logging Matter in Microservices
In a monolithic application, it is relatively straightforward to monitor and log events since everything is contained within a single codebase and runtime environment. However, in a microservices architecture, there are multiple services running independently, communicating with each other through various protocols and APIs. This distributed nature makes it challenging to get a holistic view of the system’s health and troubleshoot issues.
Monitoring allows you to track the performance and health of your microservices by collecting and analyzing relevant metrics. It helps you identify bottlenecks, detect anomalies, and ensure that your system is running smoothly. Logging, on the other hand, provides a detailed record of events and activities within your microservices, serving as a valuable tool for debugging, auditing, and compliance.
Implementing Monitoring in a Microservices Architecture
To effectively monitor your microservices architecture, you need to consider the following aspects:
1. Collecting Metrics: Each microservice should expose relevant metrics, such as response time, error rate, throughput, and resource utilization. These metrics can be collected and aggregated using a monitoring system like Prometheus or DataDog. The microservices can expose these metrics through an HTTP endpoint or by integrating with monitoring libraries specific to your programming language or framework.
2. Dashboard and Visualization: Once you have collected the metrics, you can use a monitoring tool to create dashboards and visualize the data. Tools like Grafana or Kibana allow you to create interactive and customizable dashboards, enabling you to monitor the health and performance of your microservices at a glance.
3. Alerting: Setting up alerts is crucial to notify you when something goes wrong or when certain thresholds are breached. Monitoring systems usually provide alerting mechanisms that can trigger notifications via email, Slack, or other channels. By configuring appropriate alerts, you can ensure that you are promptly notified of any critical issues in your microservices.
Implementing Logging in a Microservices Architecture
Logging is essential for understanding the behavior of your microservices and diagnosing issues. Here are some best practices for implementing logging in a microservices architecture:
1. Structured Logging: Use structured logging frameworks like Log4j, Logback, or Serilog to log events and activities in a structured format. Structured logs are easier to search, filter, and analyze, providing valuable insights into your microservices’ behavior.
2. Centralized Log Aggregation: Instead of relying on individual log files from each microservice, it is recommended to use a centralized log aggregation system like ELK Stack (Elasticsearch, Logstash, and Kibana) or Graylog. These tools allow you to collect, store, and analyze logs from all your microservices in a single location, simplifying troubleshooting and debugging.
3. Distributed Tracing: Distributed tracing systems like Zipkin or Jaeger can help you trace requests as they flow across multiple microservices. By instrumenting your microservices with trace IDs, you can gain visibility into the end-to-end flow of requests and identify performance bottlenecks or failures.
Handling Data in Microservices
Microservices architecture brings numerous benefits to software development, including increased scalability, flexibility, and maintainability. However, one of the challenges in this architecture is how to handle data effectively across multiple services. In this chapter, we will explore various strategies and patterns for handling data in microservices.
1. Database per Service
One popular approach is to have a separate database for each microservice. This ensures that each service has complete control over its data and can evolve its schema independently. It also allows teams to choose the most appropriate database technology for their specific needs. However, this approach can introduce data duplication and increase the complexity of managing multiple databases.
2. Shared Database
Another approach is to use a shared database, where all microservices access a single database instance. This can simplify data management since there is no need to replicate data across multiple databases. However, it can also introduce tight coupling between services and create dependencies on the database schema. Changes to the schema may require coordination and synchronization across multiple services.
3. Event Sourcing and CQRS
Event sourcing and Command Query Responsibility Segregation (CQRS) are patterns that can be used to handle data in microservices. Event sourcing involves storing all changes to an application’s state as a sequence of events. These events can be used to reconstruct the current state of the system. CQRS separates the read and write operations, allowing different models and data stores for each. This pattern enables scalability and flexibility but adds complexity to the system.
4. API Composition
API composition is a technique where multiple microservices are combined to provide a unified API. This approach allows clients to retrieve data from multiple services through a single request. It can be implemented using a gateway service that orchestrates requests to the underlying microservices. However, this approach can introduce performance issues and increase the coupling between services.
5. Data Replication and Synchronization
In some cases, it may be necessary to replicate and synchronize data across multiple microservices. This can be achieved using messaging systems or event-driven architectures. For example, when a change occurs in one microservice, it can publish an event that triggers updates in other services. This approach ensures data consistency but requires additional infrastructure and introduces complexity.
Example: Database per Service
# microservice-a.yaml --- apiVersion: v1 kind: Service metadata: name: microservice-a spec: selector: app: microservice-a ports: - port: 8080 targetPort: 8080 --- apiVersion: apps/v1 kind: Deployment metadata: name: microservice-a spec: replicas: 1 selector: matchLabels: app: microservice-a template: metadata: labels: app: microservice-a spec: containers: - name: microservice-a image: myregistry/microservice-a:v1 ports: - containerPort: 8080 env: - name: DB_HOST value: microservice-a-db - name: DB_PORT value: "5432" - name: DB_NAME value: microservice-a-db --- apiVersion: v1 kind: Service metadata: name: microservice-a-db spec: selector: app: microservice-a-db ports: - port: 5432 targetPort: 5432 --- apiVersion: apps/v1 kind: Deployment metadata: name: microservice-a-db spec: replicas: 1 selector: matchLabels: app: microservice-a-db template: metadata: labels: app: microservice-a-db spec: containers: - name: microservice-a-db image: postgres:latest ports: - containerPort: 5432 env: - name: POSTGRES_USER value: admin - name: POSTGRES_PASSWORD value: admin - name: POSTGRES_DB value: microservice-a-db
In this example, we have a microservice called “microservice-a” that has its own dedicated database. The microservice and the database are deployed as separate entities using Kubernetes. This approach allows the microservice to have full control over its data and schema.
In conclusion, handling data in microservices requires careful consideration of various factors such as data ownership, consistency, and scalability. Different strategies and patterns can be employed based on the specific requirements of the system. It is crucial to weigh the trade-offs and select the most suitable approach for your microservices architecture.
Security Considerations for Microservices
As organizations migrate their monolithic applications to microservices, it is important to consider the security implications of this architectural shift. While microservices offer many benefits such as scalability and flexibility, they also introduce new security challenges that need to be addressed. In this chapter, we will explore some of the key security considerations for microservices.
1. Authentication and Authorization
With a monolithic architecture, authentication and authorization are typically handled at the application level. However, in a microservices architecture, each microservice may have its own authentication and authorization mechanisms. This can lead to a fragmented security model and make it harder to enforce consistent security policies across the system.
To address this, it is recommended to implement a centralized authentication and authorization service or use a third-party identity provider. This allows for a unified approach to managing authentication and authorization, reducing complexity and improving security.
2. Communication Security
In a microservices architecture, services communicate with each other over the network. It is crucial to ensure that this communication is secure and protected from eavesdropping, tampering, and other security threats.
The use of Transport Layer Security (TLS) or Secure Sockets Layer (SSL) is recommended to encrypt the communication between services. This ensures that sensitive data is transmitted securely and cannot be intercepted or modified by attackers. Additionally, mutual authentication can be implemented to verify the identity of both the client and the server.
Here is an example configuration for enabling TLS in a microservice written in Java using Spring Boot:
server: port: 8443 ssl: key-store: classpath:keystore.p12 key-store-password: password key-store-type: PKCS12 key-alias: mykey
3. Input Validation and Sanitization
Microservices often expose APIs that can be accessed by external clients. It is crucial to validate and sanitize all incoming data to prevent common security vulnerabilities such as SQL injection, cross-site scripting (XSS), and remote code execution.
Using a well-established framework such as OWASP Java Encoder or OWASP ESAPI can help in validating and sanitizing user input effectively. Additionally, implementing input validation and sanitization at the API gateway level can provide an added layer of protection.
4. Logging and Monitoring
Proper logging and monitoring are essential for detecting and mitigating security incidents in a microservices environment. Each microservice should generate detailed logs containing relevant security events and anomalies.
Centralized log management and monitoring tools can help in aggregating and analyzing logs from multiple microservices, enabling security teams to detect and respond to security threats in a timely manner. Implementing intrusion detection systems (IDS) and security information and event management (SIEM) solutions can further enhance the security posture of the system.
5. Secure Deployment and Configuration Management
Securing the deployment and configuration management process is crucial to prevent unauthorized access and tampering of the microservices infrastructure. Docker containers, Kubernetes, and other container orchestration tools should be configured securely with proper access controls and hardened configurations.
Implementing secure CI/CD pipelines with automated security testing can help ensure that only trusted and verified code is deployed to production environments. Regular vulnerability scanning and patch management should also be performed to address any security vulnerabilities in the deployed microservices.
In conclusion, migrating from a monolithic architecture to microservices requires careful consideration of security implications. By addressing authentication and authorization, communication security, input validation and sanitization, logging and monitoring, and secure deployment and configuration management, organizations can mitigate security risks and ensure the overall security of their microservices architecture.
Testing Microservices
Testing microservices is a crucial aspect of ensuring their reliability and functionality. As microservices are typically deployed independently and communicate with each other, it is important to thoroughly test their individual components as well as their interactions.
In this chapter, we will explore various testing strategies and techniques for microservices, including unit testing, integration testing, and end-to-end testing. We will also discuss the challenges that arise when testing microservices and how to address them.
Unit Testing
Unit testing is the process of testing individual components of a microservice in isolation. It involves writing test cases for each function or method to verify that it behaves as expected. Unit tests are typically written by developers and executed frequently during the development process.
Let’s take a look at an example unit test for a simple microservice written in Java:
// File: UserServiceTest.java import org.junit.Test; import static org.junit.Assert.assertEquals; public class UserServiceTest { @Test public void testGetUser() { UserService userService = new UserService(); User user = userService.getUser(123); assertEquals(123, user.getId()); assertEquals("John Doe", user.getName()); } }
In this example, we create an instance of the UserService class and call the getUser() method with a specific user ID. We then use assertions to verify that the returned user object has the expected ID and name.
Unit tests help identify and fix issues early in the development process, ensuring that each component of a microservice functions correctly in isolation.
Integration Testing
Integration testing focuses on testing the interactions between different microservices. It ensures that the services work together as expected and their communication protocols are properly implemented. Integration tests are typically written by developers and executed during the build and deployment process.
To illustrate integration testing, let’s consider a scenario where we have a microservice that interacts with a database. We can use a tool like Docker to spin up a temporary database for testing purposes. Here’s an example using Python and the pytest framework:
# File: test_user_service.py import pytest from user_service import UserService @pytest.fixture def user_service(): return UserService() @pytest.fixture(autouse=True) def setup_db(): # Set up a temporary database for testing db = create_temporary_database() yield db # Cleanup after the tests destroy_temporary_database(db) def test_get_user(user_service): user = user_service.get_user(123) assert user.id == 123 assert user.name == "John Doe"
In this example, we use the pytest framework to define the test cases. We create a fixture called user_service
that returns an instance of the UserService class. We also create a fixture called setup_db
that sets up a temporary database for testing.
The test_get_user
function then calls the get_user
method of the user_service
fixture and verifies that the returned user object has the expected ID and name.
Integration tests help verify that microservices can communicate and work together effectively.
End-to-End Testing
End-to-end testing involves testing the entire system, including all microservices and their interactions, as a whole. It helps validate the system’s functionality from the user’s perspective. End-to-end tests are typically written by quality assurance engineers and executed in a production-like environment.
To perform end-to-end testing, you can use tools like Selenium or Cypress to simulate user interactions with the system’s user interface. These tools can automate tasks such as filling out forms, clicking buttons, and verifying the correctness of displayed information.
Here’s an example of an end-to-end test using Cypress:
// File: user_spec.js describe('User Management', () => { it('should display user details', () => { cy.visit('/users/123') cy.contains('John Doe') cy.contains('john.doe@example.com') }) })
In this example, we use Cypress to visit the user details page for a specific user ID. We then use assertions to verify that the user’s name and email address are displayed correctly.
End-to-end tests help ensure that the entire system, including all microservices, work together seamlessly to provide the expected functionality to users.
Challenges in Testing Microservices
Testing microservices presents some unique challenges compared to monolithic architectures:
1. Dependency management: Microservices often have external dependencies such as databases, message queues, or third-party APIs. It can be challenging to set up and manage these dependencies for testing purposes.
2. Data consistency: As microservices operate independently, maintaining data consistency across multiple services during testing can be complex. Strategies like test data generation and data mocking can help address this challenge.
3. Service availability: Microservices may have different deployment schedules and dependencies on other services. Ensuring all required services are available and properly configured for testing can be difficult.
4. Test environment setup: Microservices may have different runtime environments, making it important to set up appropriate test environments that closely resemble production environments.
To address these challenges, it is crucial to establish effective testing strategies, use tools and frameworks that support microservices testing, and adopt practices like continuous integration and deployment.
In the next chapter, we will explore containerization and orchestration technologies that can simplify the deployment and management of microservices.
Deploying Microservices
Once you have successfully designed and developed your microservices, the next step is to deploy them. Deploying microservices involves setting up the necessary infrastructure, configuring the deployment environment, and ensuring that your microservices are running smoothly.
Here are some key considerations and best practices for deploying microservices:
Containerization
Containerization is a popular approach for deploying microservices. Containers provide a lightweight and consistent environment that encapsulates your microservices along with their dependencies. Docker is a widely-used containerization platform that simplifies the deployment process.
To containerize your microservices, you need to write a Dockerfile that defines the image for each microservice. Here’s an example of a Dockerfile for a Node.js microservice:
# Use the official Node.js runtime as the base image FROM node:14 # Set the working directory WORKDIR /app # Copy package.json and package-lock.json to the working directory COPY package*.json ./ # Install dependencies RUN npm install # Copy the rest of the application code to the working directory COPY . . # Expose the port that the microservice listens on EXPOSE 3000 # Set the command to start the microservice CMD ["node", "app.js"]
Once you have created the Dockerfile for each microservice, you can build the Docker images and run the containers using Docker commands. Docker Compose is a tool that simplifies the management of multiple Docker containers.
Orchestration
Microservices often need to communicate with each other, and managing their interactions can become complex as the number of microservices grows. Orchestration tools like Kubernetes help you manage and scale your microservices by automating tasks such as deployment, scaling, and load balancing.
Kubernetes uses a declarative approach, where you define the desired state of your microservices in Kubernetes manifests or YAML files. Here’s an example of a Kubernetes Deployment manifest for a microservice:
apiVersion: apps/v1 kind: Deployment metadata: name: example-microservice spec: replicas: 3 selector: matchLabels: app: example-microservice template: metadata: labels: app: example-microservice spec: containers: - name: example-microservice image: example-microservice:latest ports: - containerPort: 3000
You can use the kubectl
command-line tool to apply these manifests and deploy your microservices to a Kubernetes cluster.
Continuous Integration and Deployment (CI/CD)
To streamline the deployment process and ensure the quality of your microservices, it’s recommended to implement a CI/CD pipeline. CI/CD pipelines automate the building, testing, and deployment of your microservices.
Popular CI/CD tools like Jenkins, GitLab CI/CD, and CircleCI integrate well with containerization and orchestration platforms. You can configure these tools to automatically build Docker images, run tests, and deploy your microservices to a staging or production environment.
Here’s an example of a simple Jenkins pipeline for deploying a microservice:
pipeline { agent any stages { stage('Build') { steps { sh 'docker build -t example-microservice .' } } stage('Test') { steps { sh 'docker run example-microservice npm test' } } stage('Deploy') { steps { sh 'kubectl apply -f kubernetes/deployment.yaml' } } } }
By implementing a CI/CD pipeline, you can ensure that your microservices are continuously integrated, tested, and deployed in an automated and reliable manner.
Monitoring and Logging
Monitoring and logging are crucial for the operational success of your microservices. Tools like Prometheus, Grafana, and ELK (Elasticsearch, Logstash, and Kibana) can help you monitor the health, performance, and logs of your microservices.
Instrument your microservices with metrics and logs that provide insights into their behavior. Use monitoring tools to collect and visualize these metrics, and set up alerts to notify you of any anomalies or issues.
Managing Microservices in Production
Managing microservices in a production environment requires careful planning and implementation. Here, we will discuss some best practices and tools that can help simplify the management of microservices.
Service Discovery
In a microservices architecture, services need to be able to discover and communicate with each other. Service discovery plays a crucial role in this process. There are various tools available for service discovery, such as Consul, Etcd, and ZooKeeper.
Here’s an example of using Consul for service discovery in a Node.js application:
const consul = require('consul'); // Initialize Consul client const client = consul({ host: 'localhost', port: 8500, promisify: true, }); // Register a service client.agent.service.register({ name: 'my-service', address: 'localhost', port: 3000, check: { http: 'http://localhost:3000/health', interval: '10s', }, }, (err) => { if (err) throw err; console.log('Service registered'); }); // Discover a service client.agent.service.list((err, services) => { if (err) throw err; console.log('Discovered services:', services); });
Monitoring and Logging
Monitoring and logging are essential for troubleshooting and maintaining the health of microservices. Tools like Prometheus, Grafana, and ELK (Elasticsearch, Logstash, Kibana) stack can help with monitoring and logging in a microservices architecture.
Prometheus is a popular monitoring solution that collects metrics from microservices and provides a powerful query language for analyzing them. Grafana is a visualization tool that can be used with Prometheus to create dashboards for monitoring various metrics.
The ELK stack is commonly used for logging in microservices. Elasticsearch stores the logs, Logstash processes and filters them, and Kibana provides a user-friendly interface for searching and visualizing logs.
Scaling and Load Balancing
Microservices should be designed to scale horizontally to handle increased traffic and load. Load balancing is crucial to distribute incoming requests across multiple instances of a service.
One approach is to use a load balancer like NGINX or HAProxy, which can distribute traffic to multiple instances based on a predefined algorithm (e.g., round-robin or least connections). Another option is to use a container orchestration platform like Kubernetes or Docker Swarm, which can automatically scale services based on defined rules.
Here’s an example of using NGINX as a load balancer:
http { upstream backend { server backend1.example.com; server backend2.example.com; server backend3.example.com; } server { listen 80; location / { proxy_pass http://backend; } } }
Fault Tolerance and Resilience
Microservices should be designed to handle failures gracefully. Implementing circuit breakers, retries, and timeouts can help improve fault tolerance and resilience.
Netflix’s Hystrix library is a popular choice for implementing circuit breakers in microservices. It provides a way to control the flow of traffic between services and handle failures intelligently.
Here’s an example of using Hystrix in a Java microservice:
@HystrixCommand(fallbackMethod = "fallbackMethod") public String performRequest() { // Perform the request // ... // If the request fails, throw an exception throw new RuntimeException("Request failed"); } public String fallbackMethod() { return "Fallback response"; }
Continuous Integration and Deployment
To manage microservices efficiently, it is crucial to have a robust continuous integration and deployment process. Tools like Jenkins, Travis CI, and GitLab CI/CD can automate the build, test, and deployment pipelines.
Using a containerization platform like Docker can simplify the deployment process by packaging each microservice into a container image. Container orchestration platforms like Kubernetes can then manage the deployment and scaling of these containers.
Advanced Techniques for Microservices
Microservices architecture offers several advanced techniques that can further enhance the flexibility, scalability, and maintainability of your software. In this chapter, we will explore some of these techniques and how they can be applied in real-world scenarios.
Service Discovery
In a microservices architecture, where services are constantly being added or removed, it is crucial to have a mechanism for service discovery. Service discovery allows services to locate and communicate with each other without hardcoding their network addresses.
One popular approach to service discovery is to use a service registry, such as Consul or etcd. These registries act as a central repository where services can register themselves and retrieve the network addresses of other services.
Here’s an example of how service discovery can be implemented using Consul and Spring Boot:
@SpringBootApplication @EnableDiscoveryClient public class UserServiceApplication { // ... }
Circuit Breaker Pattern
The Circuit Breaker pattern is a technique used to prevent cascading failures in a distributed system. It provides a fallback mechanism when a service is unavailable or experiencing high latency.
One popular implementation of the Circuit Breaker pattern is Hystrix. Hystrix allows you to wrap service calls with a circuit breaker that can be configured to open when certain thresholds are exceeded. When the circuit breaker is open, fallback logic is executed instead of making the actual service call.
Here’s an example of how to use Hystrix with a RESTful service call in Java:
@HystrixCommand(fallbackMethod = "fallbackMethod") public String getUser(String userId) { // Make the actual service call // ... } public String fallbackMethod(String userId) { // Fallback logic // ... }
Event-Driven Architecture
Event-driven architecture is a powerful technique that enables loose coupling and scalability in a microservices environment. Instead of synchronous request/response communication, services communicate by producing and consuming events.
A popular framework for implementing event-driven architecture is Spring Cloud Stream. It provides abstractions for event publishing and consuming, as well as support for various messaging systems such as Apache Kafka or RabbitMQ.
Here’s an example of how to use Spring Cloud Stream to publish an event:
@Autowired private Source source; public void publishEvent(String message) { source.output().send(MessageBuilder.withPayload(message).build()); }
Containerization and Orchestration
Containerization and orchestration technologies, such as Docker and Kubernetes, play a crucial role in deploying and managing microservices at scale. Containers provide a lightweight and portable environment for running microservices, while orchestration tools automate the deployment, scaling, and monitoring of containers.
Here’s an example of a Dockerfile for containerizing a Java microservice:
FROM openjdk:11-jre-slim WORKDIR /app COPY target/my-service.jar /app CMD ["java", "-jar", "my-service.jar"]
With Kubernetes, you can define a deployment manifest to specify how your microservice should be deployed and managed:
apiVersion: apps/v1 kind: Deployment metadata: name: my-service spec: replicas: 3 selector: matchLabels: app: my-service template: metadata: labels: app: my-service spec: containers: - name: my-service image: my-service:latest ports: - containerPort: 8080
These advanced techniques provide the necessary tools and patterns to build and maintain a robust microservices architecture. By leveraging service discovery, circuit breakers, event-driven architecture, and containerization with orchestration, you can simplify your software architecture and unlock the full potential of microservices.
Building Resilient Microservices
Resilience is a crucial aspect when it comes to building microservices. As microservices are distributed systems, they are more prone to failures, network issues, and service disruptions. In this chapter, we will explore some techniques to make your microservices more resilient and reliable.
Circuit Breaker Pattern
The Circuit Breaker pattern is a design pattern that can help prevent cascading failures in a distributed system. It provides a fail-fast mechanism by monitoring the availability of a service and breaking the circuit if it detects that the service is not responding. This helps in isolating the faulty service and prevents it from affecting the overall system.
Implementing a Circuit Breaker pattern in your microservices can be done using libraries such as Hystrix or resilience4j. These libraries provide an easy way to handle failures and provide fallback mechanisms when a service is unavailable. Here’s an example of how to use Hystrix in a Java microservice:
// Add HystrixCommand annotation to the method @HystrixCommand(fallbackMethod = "fallbackMethod") public String getServiceData() { // Call the external service return externalService.getData(); } // Define the fallback method public String fallbackMethod() { return "Fallback data"; }
In this example, if the external service fails to respond, the fallbackMethod will be called, and the microservice will return the fallback data instead.
Retry Mechanisms
Another important aspect of building resilient microservices is implementing retry mechanisms. Retrying failed requests can help overcome temporary network issues or transient failures. Libraries like resilience4j provide easy-to-use decorators to add retry logic to your microservices.
Here’s an example of using the Retry decorator in a Spring Boot application:
// Add the Retry annotation to the method @Retry(name = "externalServiceRetry") public String getServiceData() { // Call the external service return externalService.getData(); }
In this example, the method will be retried if an exception occurs. You can configure the maximum number of retries, the delay between retries, and other parameters to customize the retry behavior.
Timeouts and Timeouts
Setting appropriate timeouts and retries for your microservices is essential to ensure that they are resilient to slow or unresponsive services. A timeout ensures that a request doesn’t wait indefinitely for a response and gives up after a certain period.
In Java, you can set timeouts using libraries like Hystrix or resilience4j. Here’s an example of setting a timeout in Hystrix:
@HystrixCommand(fallbackMethod = "fallbackMethod", commandProperties = { @HystrixProperty(name = "execution.isolation.thread.timeoutInMilliseconds", value = "1000") }) public String getServiceData() { // Call the external service return externalService.getData(); }
In this example, the timeout for the external service call is set to 1 second. If the service takes longer to respond, the fallback method will be called.
Monitoring and Alerting
Monitoring and alerting play a crucial role in maintaining the resilience of your microservices. By monitoring the health and performance of your services, you can proactively identify failures or bottlenecks and take appropriate actions.
Tools like Prometheus and Grafana can be used to collect and visualize metrics from your microservices. These metrics can include information about the number of requests, response times, error rates, and more. By setting up alerts based on these metrics, you can be notified when a service is experiencing issues and take immediate action to resolve them.
Using Containers and Orchestration
Containerization has become increasingly popular in the world of software development, as it provides a lightweight and portable way to package applications and their dependencies. Containers offer isolation, allowing multiple applications to run on the same host without interfering with each other. This makes them an ideal choice for migrating a monolithic application to microservices.
One of the most popular containerization platforms is Docker. Docker allows you to create containers with all the necessary dependencies and configurations, making it easy to build, ship, and run applications. It provides a consistent environment for your application to run, regardless of the underlying infrastructure.
To containerize your monolithic application, you would need to create a Dockerfile that describes the image of the container. Here’s an example Dockerfile for a Java application:
FROM openjdk:11 COPY . /app WORKDIR /app RUN javac Main.java CMD ["java", "Main"]
In this example, we start from a base image that has Java 11 installed, copy the application code into the container, compile it, and then specify the command to run the application.
Once you have containerized your monolithic application, the next step is to orchestrate the deployment and management of these containers. Container orchestration platforms, such as Kubernetes, help manage the lifecycle of containers, including scaling, load balancing, and health monitoring.
Kubernetes provides a declarative approach to managing containers, allowing you to define the desired state of your application using YAML manifests. Here’s an example deployment manifest for a microservice in Kubernetes:
apiVersion: apps/v1 kind: Deployment metadata: name: my-service spec: replicas: 3 selector: matchLabels: app: my-service template: metadata: labels: app: my-service spec: containers: - name: my-service image: my-service:latest ports: - containerPort: 8080
In this example, we define a deployment with three replicas of our microservice. The deployment ensures that three instances of the container are always running, and Kubernetes takes care of load balancing and scaling based on the defined rules.
With container orchestration, you can easily scale your microservices horizontally by increasing the number of replicas. Kubernetes also provides advanced features like service discovery, which allows different microservices to communicate with each other using DNS.
Using containers and orchestration simplifies the management and deployment of microservices. Containerization ensures that your application runs consistently across different environments, while orchestration platforms like Kubernetes automate many operational tasks, making it easier to scale and manage your microservices.
In the next chapter, we will explore the benefits of using an API gateway in a microservices architecture.
Event-Driven Architecture with Microservices
In the world of microservices, event-driven architecture has gained popularity due to its ability to decouple different components and make them highly scalable and resilient. Event-driven architecture allows services to communicate with each other through events, which are messages that carry information about a specific action or state change.
In an event-driven architecture, services produce and consume events asynchronously. This means that a service can continue to function even if the services it interacts with are unavailable or experiencing high load. Also, services can react to events in real-time, enabling near-instantaneous responses to changes in the system.
To implement event-driven architecture with microservices, you need a reliable message broker that can handle the distribution and delivery of events. One popular choice is Apache Kafka, a distributed streaming platform that provides high-throughput, fault-tolerant messaging.
Let’s take a look at an example of how microservices can interact with each other using events. Suppose we have two microservices: Order Service and Payment Service. When a new order is created, the Order Service publishes an event indicating the creation of the order. The Payment Service subscribes to this event and processes the payment for the order.
Here’s how the Order Service publishes the event using Kafka:
import org.apache.kafka.clients.producer.KafkaProducer; import org.apache.kafka.clients.producer.ProducerRecord; public class OrderService { private KafkaProducer<String, String> producer; public OrderService() { // Initialize the Kafka producer Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer"); props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer"); producer = new KafkaProducer<>(props); } public void createOrder(Order order) { // Create the order // Publish the order created event String topic = "order-events"; String key = order.getId(); String value = "OrderCreated"; ProducerRecord<String, String> record = new ProducerRecord<>(topic, key, value); producer.send(record); } }
And here’s how the Payment Service consumes the event:
import org.apache.kafka.clients.consumer.KafkaConsumer; import org.apache.kafka.clients.consumer.ConsumerRecords; public class PaymentService { private KafkaConsumer<String, String> consumer; public PaymentService() { // Initialize the Kafka consumer Properties props = new Properties(); props.put("bootstrap.servers", "localhost:9092"); props.put("key.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); props.put("value.deserializer", "org.apache.kafka.common.serialization.StringDeserializer"); props.put("group.id", "payment-service"); consumer = new KafkaConsumer<>(props); consumer.subscribe(Collections.singletonList("order-events")); } public void startProcessing() { while (true) { ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100)); for (ConsumerRecord<String, String> record : records) { // Process the order created event String orderId = record.key(); System.out.println("Processing payment for order: " + orderId); } } } }
By using an event-driven architecture, the Order Service and Payment Service are decoupled from each other. The Order Service doesn’t need to know anything about the Payment Service, and vice versa. They only communicate through events, which allows each service to evolve independently.
Event-driven architecture with microservices brings several benefits. It enables loose coupling between services, making it easier to develop, test, and deploy each service independently. It also provides fault tolerance and scalability by allowing services to operate asynchronously and handle events at their own pace.
In conclusion, event-driven architecture is a powerful approach when migrating from a monolithic architecture to microservices. It simplifies the software architecture by decoupling services through events and provides scalability and fault tolerance. Apache Kafka is a popular choice for implementing event-driven architecture in the microservices ecosystem. By embracing event-driven architecture, you can build resilient and scalable microservices that can adapt to changing business requirements.
Building a Gateway for Microservices
When transitioning from a monolithic architecture to a microservices architecture, one of the key components you’ll need to consider is a gateway. The gateway serves as an entry point for all incoming requests and acts as a mediator between the client and the various microservices.
Why do we need a gateway?
In a microservices architecture, each service is responsible for a specific business capability. This means that clients need to interact with multiple services to perform a single operation. Without a gateway, clients would have to manage communication and coordination with each individual service, leading to increased complexity and potential performance issues.
A gateway consolidates the communication between clients and microservices, providing a unified interface for clients to interact with. It allows you to abstract away the details of the underlying microservices, providing a simpler and more cohesive API for clients to consume.
Key features of a gateway
A gateway typically provides several important features that simplify the interaction between clients and microservices:
– **Routing**: The gateway acts as a router, forwarding requests from clients to the appropriate microservice based on the request’s path, headers, or other information.
– **Load Balancing**: To ensure high availability and scalability, a gateway can distribute incoming requests across multiple instances of the same microservice. This helps in handling increased traffic and prevents any single service instance from becoming a bottleneck.
– **Authentication and Authorization**: The gateway can handle authentication and authorization on behalf of the microservices. It can validate client credentials, enforce access control policies, and generate tokens for subsequent requests.
– **Caching**: By caching responses, a gateway can reduce the load on microservices and improve overall system performance. It can store and serve responses for frequently accessed resources without forwarding the request to the underlying services.
– **Logging and Monitoring**: A gateway can capture and log relevant information about incoming requests and responses. It can also provide metrics and monitoring capabilities to gain insights into the overall system performance.
Implementing a gateway
There are several tools and frameworks available for building a gateway. One popular option is **Netflix Zuul**, which is an open-source gateway built on top of the Spring Cloud ecosystem. Zuul provides a rich set of features, including dynamic routing, load balancing, and request filtering.
Here’s an example of a basic Zuul configuration in a zuul.yml
file:
zuul: routes: users: path: /users/** serviceId: user-service products: path: /products/** serviceId: product-service
In this example, any request starting with /users
will be routed to the user-service
, while requests starting with /products
will be routed to the product-service
. Zuul takes care of the routing and load balancing behind the scenes.
Caching Strategies in a Microservices Environment
Caching is an essential aspect of designing a microservices architecture. It can significantly improve the performance and scalability of your application by reducing the load on your services and minimizing external API calls. In this chapter, we will explore different caching strategies that can be employed in a microservices environment.
Client-side Caching
Client-side caching involves caching data directly on the client side, such as in a web browser or a mobile app. This approach can be particularly useful when dealing with static or semi-static data that doesn’t change frequently. By caching this data on the client, you can reduce the number of requests made to the server, resulting in faster response times and improved user experience.
Here’s an example of how client-side caching can be implemented using JavaScript in a web application:
// Check if the data is already cached in the browser const cachedData = localStorage.getItem('cachedData'); if (cachedData) { // Use the cached data processData(JSON.parse(cachedData)); } else { // Fetch the data from the server fetchData() .then(data => { // Cache the data in the browser localStorage.setItem('cachedData', JSON.stringify(data)); processData(data); }) .catch(error => { console.error('Error fetching data:', error); }); }
Server-side Caching
Server-side caching involves caching data at the server level, typically using a caching server like Redis or Memcached. This approach is beneficial when dealing with data that is common across multiple requests or changes infrequently. By caching the data on the server, subsequent requests can be served directly from the cache, reducing the time and resources required to fetch the data from the underlying data source.
Here’s an example of server-side caching using Redis in a Node.js application:
const redis = require('redis'); const client = redis.createClient(); // Check if the data is already cached in Redis client.get('cachedData', (error, cachedData) => { if (cachedData) { // Use the cached data processData(JSON.parse(cachedData)); } else { // Fetch the data from the database fetchData() .then(data => { // Cache the data in Redis client.set('cachedData', JSON.stringify(data)); processData(data); }) .catch(error => { console.error('Error fetching data:', error); }); } });
Distributed Caching
In a microservices architecture, with each service having its own data store, it becomes essential to implement distributed caching. Distributed caching involves caching data across multiple services or instances to ensure consistency and improve performance. This can be achieved using caching solutions like Hazelcast or Apache Ignite.
Here’s an example of distributed caching using Hazelcast in a Java microservice:
import com.hazelcast.core.Hazelcast; import com.hazelcast.core.HazelcastInstance; import com.hazelcast.core.IMap; public class CacheService { private HazelcastInstance hazelcastInstance; private IMap<String, Object> cache; public CacheService() { hazelcastInstance = Hazelcast.newHazelcastInstance(); cache = hazelcastInstance.getMap("myCache"); } public Object get(String key) { return cache.get(key); } public void put(String key, Object value) { cache.put(key, value); } }
In this example, the CacheService
class initializes a Hazelcast instance and provides methods to get and put values in the distributed cache.
Cache Invalidation
One important consideration when using caching is cache invalidation. As data changes over time, it is crucial to keep the cache updated to avoid serving stale or incorrect data. There are various cache invalidation strategies, such as time-based invalidation, event-based invalidation, or manual invalidation.
For example, you can implement time-based invalidation by setting an expiration time for cached data. When the cache expires, subsequent requests will trigger a refresh of the data from the data source.
public void put(String key, Object value, int expirationSeconds) { cache.put(key, value, expirationSeconds, TimeUnit.SECONDS); }
Event-based invalidation involves listening for events or notifications from the data source and invalidating the corresponding cache entries when changes occur. This ensures that the cache remains up to date with the latest data.
Integrating Microservices with Legacy Systems
As organizations modernize their software architecture by migrating from monolithic applications to microservices, one of the biggest challenges they face is integrating their new microservices with existing legacy systems. These legacy systems often have complex dependencies and may not have been designed with interoperability in mind. However, with the right approach, it is possible to successfully integrate microservices with legacy systems and unlock the benefits of a more modular and scalable architecture.
Understanding Legacy Systems
Legacy systems are typically monolithic applications that have been developed and maintained over many years. They often rely on outdated technology stacks and have accumulated a significant amount of technical debt. These systems may lack proper documentation and have tightly coupled components, making them difficult to modify or replace.
When integrating microservices with legacy systems, it is important to have a thorough understanding of how the legacy system works, its data models, and the APIs it exposes. This knowledge will help in identifying the areas where microservices can be integrated without disrupting the existing functionality.
Identifying Integration Points
The first step in integrating microservices with legacy systems is to identify the integration points. These are the areas where the microservices will interact with the legacy system. Integration points can include data synchronization, event-driven communication, or exposing certain functionalities as APIs.
For example, let’s consider a legacy order management system that needs to be integrated with a new microservice responsible for inventory management. The integration point in this case could be a data synchronization mechanism that keeps the inventory data in sync between the microservice and the legacy system.
Implementing Integration Patterns
Once the integration points have been identified, it is important to choose the right integration patterns to ensure smooth communication between the microservices and the legacy systems. Some commonly used integration patterns include:
1. Request/Reply: The microservice makes a request to the legacy system and waits for a response. This pattern is suitable for synchronous communication when immediate responses are required.
// Example of Request/Reply pattern in Java using RESTful API Response response = httpClient.request("GET", "http://legacy-system.com/api/orders/123"); if (response.getStatus() == 200) { Order order = response.getBody(Order.class); // Process the order }
2. Publish/Subscribe: The microservice publishes events to a message broker, and the legacy system subscribes to these events. This pattern is useful for asynchronous communication and decoupling the microservice from the legacy system.
# Example of Publish/Subscribe pattern in Python using RabbitMQ channel.basic_publish(exchange='orders', routing_key='new_order', body=json.dumps(order))
3. Adapter: An adapter acts as a bridge between the microservice and the legacy system, translating requests and responses between the two. This pattern is helpful when the data models or APIs of the microservice and the legacy system are incompatible.
// Example of Adapter pattern in JavaScript using Express.js and SOAP app.get('/api/orders/:id', (req, res) => { legacySystemClient.getOrder(req.params.id, (err, order) => { if (err) { res.status(500).json({ error: 'Failed to fetch order' }); } else { res.json(transformOrder(order)); } }); });
Testing and Monitoring
After implementing the integration, thorough testing and monitoring are crucial to ensure the reliability and performance of the integrated system. Integration tests should cover various scenarios, including both expected and edge cases. Monitoring tools can provide insights into the performance of the integrated system, detect issues, and help with troubleshooting.
Gradual Decomposition
Integrating microservices with legacy systems is often a gradual process. It is advisable to start with low-risk integration points and gradually decompose the monolith into smaller, more manageable microservices. This approach minimizes the impact on the existing system and allows for incremental improvements over time.