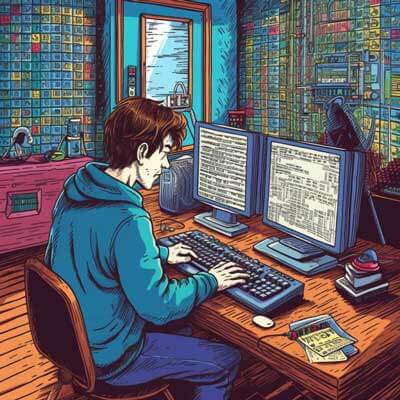
- The Importance of i18n and l10n in Node.js Apps
- Example: Internationalization in Node.js
- Example: Localization in Node.js
- Understanding Unicode and Its Significance in Multilingual Data Storage
- Example: Unicode and Multilingual Data Storage
- Exploring Different Character Encoding Schemes and When to Use Them
- ASCII
- UTF-8
- UTF-16
- ISO-8859
- Handling Text Encoding and Decoding in Node.js
- Encoding Text to Different Formats
- Decoding Text from Different Formats
- The Difference Between Localization and Internationalization
- Best Practices for Implementing i18n and l10n in Node.js Apps
- Separate Text and Translations from Code
- Use String IDs for Translations
- Choose a Robust i18n Library
- Avoid Concatenating Translated Strings
- Test and Validate Translations
- Retrieving Multilingual Data from a Database in Node.js
- Example: Retrieving Multilingual Data from MongoDB
- Effective Text Encoding Strategies for Storing User Input in Multilingual Apps
- Use UTF-8 Encoding for Text Storage
- Validate and Normalize User Input
- Avoid Length Limitations and Use Appropriate Data Types
- Consider Full-Text Indexing
- Converting Text Between Different Character Encodings in Node.js
- Example: Converting Text from UTF-8 to ISO-8859-1
- Advantages of UTF-8 Encoding over Other Schemes
- Compatibility and Backward Compatibility
- Wide Support and Standardization
- Efficient Encoding and Storage
- Complete Unicode Support
- Transliterating Text from One Script to Another in Node.js
- Example: Transliterating Cyrillic Text to Latin Text
- Additional Resources
The Importance of i18n and l10n in Node.js Apps
Internationalization (i18n) and localization (l10n) are crucial aspects of developing Node.js apps that can be used by users from different regions and languages. I18n refers to the process of designing and implementing an app to support multiple languages and cultures, while l10n involves adapting the app to specific languages and regions by translating and customizing content.
Implementing i18n and l10n in Node.js apps is important for several reasons. Firstly, it allows you to reach a global audience and cater to users from different linguistic backgrounds. By providing app content in their native language, you can enhance the user experience and make your app more accessible.
Secondly, i18n and l10n enable you to adhere to cultural norms and preferences of different regions. This includes formatting dates, times, numbers, currencies, and other locale-specific conventions. Adapting your app to local customs not only makes it more user-friendly but also builds trust and credibility among users.
Lastly, implementing i18n and l10n in your Node.js apps future-proofs your application. As your app grows and expands to new markets, having a solid internationalization and localization strategy in place will make it easier to add new languages and regions without significant code changes or rework.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Example: Internationalization in Node.js
To demonstrate how to implement i18n in a Node.js app, we’ll use the popular i18next library. Assume you have an app with a greeting message displayed to the user.
First, install the i18next library using npm:
npm install i18next
Create a file named i18n.js
and add the following code:
const i18next = require('i18next'); const i18nextMiddleware = require('i18next-http-middleware'); // Configure i18next i18next.init({ lng: 'en', fallbackLng: 'en', resources: { en: { translation: { greeting: 'Hello!', }, }, fr: { translation: { greeting: 'Bonjour!', }, }, }, }); // Initialize i18next middleware app.use(i18nextMiddleware.handle(i18next)); // Define a route to display the greeting app.get('/', (req, res) => { res.send(req.t('greeting')); }); // Start the server app.listen(3000, () => { console.log('Server started on port 3000'); });
In this example, we configure i18next with English and French translations for the greeting message. The i18nextMiddleware
middleware is used to handle language detection and translation. Finally, we define a route that sends the translated greeting to the user.
When a user accesses the root URL of your app, the greeting message will be displayed in their preferred language based on their browser settings.
Example: Localization in Node.js
Localization involves adapting your app to specific languages and regions, including translating content and formatting locale-specific data. In Node.js, you can leverage the useful Intl
object to handle localization tasks.
Let’s consider an example where you want to format a date in the user’s preferred locale. Modify the previous code to include date formatting:
app.get('/date', (req, res) => { const date = new Date(); const formattedDate = new Intl.DateTimeFormat(req.language).format(date); res.send(formattedDate); });
In this example, we use the Intl.DateTimeFormat
constructor to create a date formatter based on the user’s preferred language. The req.language
property is set by the i18nextMiddleware
middleware we configured earlier.
When a user accesses the /date
endpoint, the current date will be formatted according to their preferred locale and returned as the response.
Understanding Unicode and Its Significance in Multilingual Data Storage
Unicode is a character encoding standard that aims to represent all the characters used in the world’s writing systems. It provides a unique numeric code, called a code point, for each character.
In the context of multilingual data storage, Unicode is crucial for ensuring that your app can handle and store text in different languages, scripts, and writing systems. Unlike legacy encodings like ASCII or ISO-8859, which only support a limited set of characters, Unicode covers a vast range of characters from various languages and scripts.
Related Article: How To Use Loop Inside React JSX
Example: Unicode and Multilingual Data Storage
Let’s consider a scenario where you need to store user-generated content in a Node.js app that supports multiple languages. To ensure proper storage and retrieval of multilingual text, you should use a database that supports Unicode, such as PostgreSQL or MongoDB.
Assuming you’re using MongoDB, here’s an example of storing and retrieving multilingual text using the official MongoDB Node.js driver:
const { MongoClient } = require('mongodb'); const uri = 'mongodb://localhost:27017'; const client = new MongoClient(uri); async function storeMultilingualText() { try { await client.connect(); const db = client.db('myapp'); const collection = db.collection('messages'); const message = { content: 'こんにちは', // Japanese text language: 'ja', }; await collection.insertOne(message); const storedMessage = await collection.findOne({ language: 'ja' }); console.log(storedMessage.content); } finally { await client.close(); } } storeMultilingualText().catch(console.error);
In this example, we connect to a MongoDB database and store a message object with Japanese text. We then retrieve the stored message based on the language field.
Exploring Different Character Encoding Schemes and When to Use Them
Character encoding is the process of representing characters in a digital format. Different character encoding schemes exist, each with its own advantages and use cases. Let’s explore some commonly used character encoding schemes and when to use them.
ASCII
ASCII (American Standard Code for Information Interchange) is one of the oldest and simplest character encoding schemes. It uses 7 bits to represent characters, allowing for a maximum of 128 characters. ASCII is primarily used for representing characters in the English language and lacks support for non-English characters.
Use ASCII encoding when you’re working with English text or when you need to ensure compatibility with legacy systems that only support ASCII.
Related Article: How To Upgrade Node.js To The Latest Version
UTF-8
UTF-8 (Unicode Transformation Format 8-bit) is the most widely used character encoding scheme. It is backward-compatible with ASCII and supports all Unicode characters, making it suitable for representing text in any language. UTF-8 uses variable-length encoding, meaning that different characters may occupy different numbers of bytes.
Use UTF-8 encoding as the default choice for text encoding in Node.js apps, especially when dealing with multilingual text or when you’re unsure about the input text’s language.
UTF-16
UTF-16 is another Unicode character encoding scheme that uses 16 bits to represent characters. It can represent all Unicode characters, including those outside the Basic Multilingual Plane (BMP). Unlike UTF-8, which uses variable-length encoding, UTF-16 uses fixed-length encoding, with each character occupying either 2 or 4 bytes.
Use UTF-16 encoding when you’re working with languages or scripts that require characters outside the BMP, such as certain historical scripts or less common languages.
ISO-8859
The ISO-8859 series of character encoding schemes, also known as Latin character sets, are widely used in Europe. Each ISO-8859 encoding scheme focuses on a specific group of languages, such as ISO-8859-1 for Western European languages and ISO-8859-5 for Cyrillic languages.
Use ISO-8859 encoding schemes when you’re working with specific languages or regions that are covered by these schemes. However, be aware that ISO-8859 encodings have limitations and may not support all characters required for global multilingual applications.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Handling Text Encoding and Decoding in Node.js
In Node.js, you can handle text encoding and decoding using built-in modules and functions. The Buffer
and String
classes provide methods for encoding and decoding text in various formats.
Encoding Text to Different Formats
To encode text to different formats, you can use the Buffer
class in Node.js. The Buffer
class provides methods to convert a JavaScript string to different encoding formats, such as UTF-8, Base64, or hexadecimal.
Here’s an example of encoding a string to Base64:
const originalText = 'Hello, world!'; const encodedText = Buffer.from(originalText).toString('base64'); console.log(encodedText);
In this example, we convert the originalText
string to a Buffer
object using Buffer.from()
, and then use the toString()
method to encode the Buffer
to Base64 format.
Decoding Text from Different Formats
To decode text from different formats, you can again use the Buffer
class in Node.js. The Buffer
class provides methods to convert encoded data back to JavaScript strings.
Here’s an example of decoding a Base64-encoded string:
const encodedText = 'SGVsbG8sIHdvcmxkIQ=='; const decodedText = Buffer.from(encodedText, 'base64').toString(); console.log(decodedText);
In this example, we use Buffer.from()
with the base64
argument to create a Buffer
object from the encodedText
. We then use toString()
to convert the Buffer
to a JavaScript string.
Related Article: How To Fix Javascript: $ Is Not Defined
The Difference Between Localization and Internationalization
Localization (l10n) and internationalization (i18n) are often used interchangeably, but they have distinct meanings in the context of software development.
Internationalization (i18n) refers to the process of designing and developing an application that can be adapted to different languages, regions, and cultures. It involves separating the user interface (UI) from the application logic and making the UI elements configurable to support different languages and locales. The goal of internationalization is to create a foundation that allows for easy localization in the future.
Localization (l10n), on the other hand, focuses on adapting an application to a specific language, region, or culture. It involves translating the UI elements, content, and other aspects of the application to match the target language and cultural norms. Localization also includes customizing elements like date formats, number formats, and currency symbols to align with the target region.
Best Practices for Implementing i18n and l10n in Node.js Apps
Implementing i18n and l10n in Node.js apps requires careful planning and adherence to best practices. Here are some key best practices to consider:
Separate Text and Translations from Code
To ensure flexibility and maintainability, separate text and translations from your code. Store translations in separate files or a database, allowing for easy updates and additions without modifying the codebase. This approach also facilitates collaboration with translators and localization teams.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
Use String IDs for Translations
Instead of hardcoding translated strings directly in your code, use string IDs as placeholders. Store the actual translations in external files or a database and retrieve them dynamically based on the user’s language preference. This approach decouples the code from the specific translations and simplifies maintenance.
Choose a Robust i18n Library
There are several i18n libraries available for Node.js, such as i18next, gettext, and NodePolyglot. Choose a library that suits your requirements and provides features like pluralization, variable interpolation, and language fallbacks. Consider the library’s community support, documentation, and compatibility with other Node.js libraries and frameworks.
Avoid Concatenating Translated Strings
Avoid concatenating translated strings in your code. Instead, use placeholders or template literals to dynamically insert translated strings into the final output. This approach ensures that translations are accurate and contextually correct, especially when dealing with languages that have different sentence structures or word orders.
Related Article: Advanced DB Queries with Nodejs, Sequelize & Knex.js
Test and Validate Translations
Thoroughly test and validate translations in your Node.js app to ensure correctness and consistency. Use automated tests to verify that translations are correctly applied and that the app behaves as expected in different languages. Additionally, involve native speakers or language experts to review translations for accuracy and cultural appropriateness.
Retrieving Multilingual Data from a Database in Node.js
Retrieving multilingual data from a database in Node.js involves fetching the appropriate language-specific content based on the user’s language preference. This can be achieved by leveraging database queries and integrating them with your i18n solution.
Example: Retrieving Multilingual Data from MongoDB
Assuming you’re using MongoDB as your database, here’s an example of retrieving multilingual data based on the user’s language preference:
const { MongoClient } = require('mongodb'); const uri = 'mongodb://localhost:27017'; const client = new MongoClient(uri); async function getLocalizedData(language) { try { await client.connect(); const db = client.db('myapp'); const collection = db.collection('messages'); const localizedData = await collection.findOne({ language }); return localizedData; } finally { await client.close(); } } // Example usage const userLanguage = 'fr'; // User's preferred language const localizedData = await getLocalizedData(userLanguage); console.log(localizedData.content);
In this example, we connect to a MongoDB database and retrieve a document from the messages
collection based on the user’s preferred language. The language
field in the collection represents the language code, such as ‘en’ for English or ‘fr’ for French.
Related Article: Big Data Processing with Node.js and Apache Kafka
Effective Text Encoding Strategies for Storing User Input in Multilingual Apps
Storing user input in a multilingual app requires careful consideration of text encoding strategies to ensure accurate and efficient data storage. Here are some effective strategies to follow:
Use UTF-8 Encoding for Text Storage
UTF-8 is the recommended encoding scheme for storing multilingual text in Node.js apps. It supports all Unicode characters and is widely supported across different platforms and systems. By using UTF-8 encoding, you can ensure that user input in various languages is stored accurately and can be retrieved without data loss or corruption.
Validate and Normalize User Input
Before storing user input, it’s important to validate and normalize the text to ensure consistency and prevent potential issues. Use input validation techniques to verify that user input conforms to the expected format and character set. Additionally, normalize the text using Unicode normalization forms (such as NFC or NFD) to handle different character representations and avoid duplicated or similar-looking characters.
Related Article: AI Implementations in Node.js with TensorFlow.js and NLP
Avoid Length Limitations and Use Appropriate Data Types
Different languages have varying text lengths and character complexities. When designing your database schema, avoid strict length limitations for text fields to accommodate the potential variations in multilingual input. Additionally, choose appropriate data types for storing text, such as VARCHAR
or TEXT
fields in relational databases, or String
or Text
fields in NoSQL databases.
Consider Full-Text Indexing
If your app requires searching or indexing multilingual text, consider using full-text indexing capabilities provided by your database. Full-text indexing allows efficient searching and retrieval of text data, taking into account language-specific rules like word stemming, stop words, and language-specific behavior.
Converting Text Between Different Character Encodings in Node.js
In some scenarios, you may need to convert text between different character encodings in Node.js. This can be useful when working with legacy systems, integrating with external APIs, or handling data migration. Node.js provides built-in modules and functions to facilitate text encoding conversion.
Related Article: Integrating Node.js and React.js for Full-Stack Applications
Example: Converting Text from UTF-8 to ISO-8859-1
Let’s consider an example where you need to convert text from UTF-8 encoding to ISO-8859-1 encoding:
const { TextEncoder, TextDecoder } = require('util'); function convertTextToISO88591(text) { const encoder = new TextEncoder(); const decoder = new TextDecoder('iso-8859-1'); const utf8Bytes = encoder.encode(text); const iso88591Text = decoder.decode(utf8Bytes); return iso88591Text; } // Example usage const utf8Text = 'Héllo, wörld!'; const iso88591Text = convertTextToISO88591(utf8Text); console.log(iso88591Text);
In this example, we use the TextEncoder
and TextDecoder
classes from the util
module to convert text between different character encodings. The TextEncoder
class is used to encode the input text in UTF-8, while the TextDecoder
class is used to decode the UTF-8 bytes to ISO-8859-1 encoding.
Advantages of UTF-8 Encoding over Other Schemes
UTF-8 encoding offers several advantages over other character encoding schemes, making it the recommended choice for handling multilingual text in Node.js apps.
Compatibility and Backward Compatibility
UTF-8 is backward-compatible with ASCII, which means that any ASCII text is also valid UTF-8 text. This compatibility allows you to seamlessly handle text in different languages, scripts, and regions without changing the encoding scheme. It ensures that legacy ASCII-based systems can handle UTF-8 text without issues.
Related Article: Integrating HTMX with Javascript Frameworks
Wide Support and Standardization
UTF-8 is widely supported across different platforms, systems, and programming languages, including Node.js. It has become the de facto standard for text encoding, ensuring interoperability and consistent behavior across various software and hardware environments.
Efficient Encoding and Storage
UTF-8 uses variable-length encoding, which means that different characters can occupy different numbers of bytes. This allows UTF-8 to efficiently represent both common and less common characters, minimizing storage requirements and reducing the overall size of text data. It also simplifies text processing and manipulation, as individual characters can be easily accessed and modified.
Complete Unicode Support
UTF-8 supports the entire Unicode character set, which includes over 143,000 characters from different scripts, languages, and symbols. By using UTF-8, you can handle text in any language or script without restrictions, ensuring accurate representation and compatibility across different languages.
Given these advantages, it’s clear that UTF-8 encoding is the preferred choice for handling multilingual text in Node.js apps.
Related Article: How To Check Checkbox Status In jQuery
Transliterating Text from One Script to Another in Node.js
Transliteration is the process of converting text from one script to another, typically from a non-Latin script to a Latin script. It is often used to facilitate communication and readability when the target audience is more familiar with the Latin script. Node.js provides several libraries that can be used to transliterate text from one script to another.
Example: Transliterating Cyrillic Text to Latin Text
Let’s consider an example where you want to transliterate Cyrillic text to Latin text using the transliteration
library in Node.js:
const transliteration = require('transliteration'); const cyrillicText = 'Привет, мир!'; const latinText = transliteration.transliterate(cyrillicText); console.log(latinText);
In this example, we use the transliteration
library to transliterate the Cyrillic text “Привет, мир!” to Latin text. The transliterate()
function automatically converts the input text to its Latin script equivalent.
Additional Resources
– Text Encoding and Decoding in Node.js
– Character Encoding