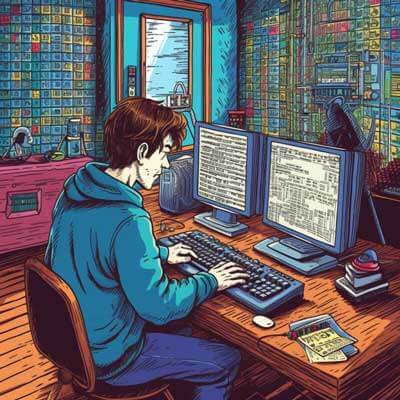
Table of Contents
To check for string equality in JavaScript, you can use the strict equality operator (===
). The strict equality operator compares both the value and the type of the operands, ensuring that both are exactly the same. Here are two possible approaches to check for string equality in JavaScript:
Approach 1: Using the Strict Equality Operator
One way to check for string equality in JavaScript is by using the strict equality operator (===
). This operator compares two values and returns true
if they are equal and of the same type, and false
otherwise. Here's an example:
const string1 = "hello"; const string2 = "world"; if (string1 === string2) { console.log("The strings are equal"); } else { console.log("The strings are not equal"); }
In this example, the strict equality operator is used to compare string1
and string2
. Since they have different values ("hello"
and "world"
), the condition evaluates to false
and the message "The strings are not equal" is printed to the console.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Approach 2: Using the String localeCompare()
Method
Another approach to check for string equality in JavaScript is by using the localeCompare()
method. This method compares two strings and returns a number indicating their relative order. If the strings are equal, it returns 0
. Here's an example:
const string1 = "hello"; const string2 = "world"; if (string1.localeCompare(string2) === 0) { console.log("The strings are equal"); } else { console.log("The strings are not equal"); }
In this example, the localeCompare()
method is used to compare string1
and string2
. Since they have different values, the method returns a number that is not equal to 0
, and the condition evaluates to false
. Therefore, the message "The strings are not equal" is printed to the console.
Best Practices
Related Article: How to Use Classes in JavaScript
When checking for string equality in JavaScript, it is generally recommended to use the strict equality operator (===
). This ensures that both the value and the type of the operands are compared, reducing the chances of unexpected results. However, there are some cases where the localeCompare()
method might be more suitable, such as when comparing strings with different casing or when using specific locale-based comparisons.