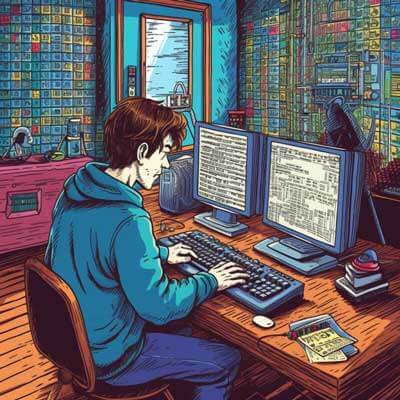
Table of Contents
Variables in JavaScript
In JavaScript, variables are used to store data values. They are the building blocks of any program and allow us to store and manipulate data. To declare a variable in JavaScript, we use the var
, let
, or const
keyword followed by the variable name.
Here are some examples:
// Declaring a variable using var var name = "John"; var age = 25; // Declaring a variable using let let city = "New York"; let isEmployed = true; // Declaring a constant variable using const const pi = 3.14159; const url = "https://example.com";
In the above examples, we declared variables name
and age
using the var
keyword, city
and isEmployed
using the let
keyword, and pi
and url
using the const
keyword.
It is important to note that variables declared with var
are function-scoped, while variables declared with let
and const
are block-scoped.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
Functions in JavaScript
Functions in JavaScript are reusable blocks of code that perform a specific task. They allow us to break our code into smaller, more manageable pieces, making it easier to read, write, and maintain.
To define a function in JavaScript, we use the function
keyword followed by the function name and a pair of parentheses. We can also specify parameters inside the parentheses if the function needs input values. The code to be executed by the function is enclosed in curly braces.
Here are some examples:
// Function without parameters function sayHello() { console.log("Hello!"); } // Function with parameters function greet(name) { console.log("Hello, " + name + "!"); } // Function with a return value function add(a, b) { return a + b; } // Calling the functions sayHello(); // Output: Hello! greet("John"); // Output: Hello, John! console.log(add(2, 3)); // Output: 5
In the above examples, sayHello
is a function that does not take any parameters and logs "Hello!" to the console. greet
is a function that takes a name
parameter and logs a personalized greeting. add
is a function that takes two parameters a
and b
, performs addition, and returns the result.
Objects in JavaScript
In JavaScript, objects are complex data types that allow us to store multiple values as properties and methods. They are a fundamental part of the language and provide a way to represent real-world entities.
To create an object in JavaScript, we can use either the object literal syntax or the new
keyword with the Object
constructor.
Here are some examples:
// Object literal syntax var person = { name: "John", age: 25, isEmployed: true, greet: function() { console.log("Hello, my name is " + this.name); } }; // Creating an object using the Object constructor var car = new Object(); car.make = "Toyota"; car.model = "Camry"; car.year = 2020; // Accessing object properties console.log(person.name); // Output: John console.log(car.make); // Output: Toyota // Calling object methods person.greet(); // Output: Hello, my name is John
In the above examples, we created an object person
using the object literal syntax and an object car
using the Object
constructor. We can access object properties using dot notation (object.property
) and call object methods using the same syntax.
Arrays in JavaScript
Arrays in JavaScript are used to store multiple values in a single variable. They are ordered, indexed collections of data that can be of any type.
To create an array in JavaScript, we use square brackets []
and separate each value with a comma.
Here are some examples:
// Creating an array var numbers = [1, 2, 3, 4, 5]; var fruits = ["apple", "banana", "orange"]; // Accessing array elements console.log(numbers[0]); // Output: 1 console.log(fruits[1]); // Output: banana // Modifying array elements numbers[2] = 10; fruits[0] = "pear"; // Adding elements to an array numbers.push(6); fruits.unshift("grape"); // Removing elements from an array numbers.pop(); fruits.shift(); // Iterating over an array for (var i = 0; i < numbers.length; i++) { console.log(numbers[i]); } fruits.forEach(function(fruit) { console.log(fruit); });
In the above examples, we created an array numbers
containing numbers 1 to 5 and an array fruits
containing different fruits. We can access array elements using square bracket notation (array[index]
), modify elements by assigning new values, add elements using push()
and unshift()
, and remove elements using pop()
and shift()
. We can also iterate over arrays using a for
loop or the forEach()
method.
Related Article: How to Scroll to the Top of a Page Using Javascript
Conditionals in JavaScript
Conditionals in JavaScript are used to make decisions based on different conditions. They allow us to execute different blocks of code depending on whether a condition is true or false.
In JavaScript, we have several conditional statements, including if
, if...else
, if...else if...else
, and the ternary operator (? :
).
Here are some examples:
// Using if statement var age = 25; if (age >= 18) { console.log("You are an adult"); } // Using if...else statement var temperature = 25; if (temperature >= 30) { console.log("It's hot outside"); } else { console.log("It's not too hot"); } // Using if...else if...else statement var time = 14; if (time < 12) { console.log("Good morning"); } else if (time < 18) { console.log("Good afternoon"); } else { console.log("Good evening"); } // Using the ternary operator var isMember = true; var discount = isMember ? 10 : 0; console.log("Discount: " + discount + "%");
In the above examples, we used different conditional statements to make decisions based on different conditions. The if
statement checks if the age
is greater than or equal to 18 and logs a message if it is true. The if...else
statement checks if the temperature
is greater than or equal to 30 and logs different messages based on the condition. The if...else if...else
statement checks the time
and logs different greetings based on the time of the day. Finally, the ternary operator assigns a value to the discount
variable based on the isMember
condition.
Loops in JavaScript
Loops in JavaScript are used to repeatedly execute a block of code until a specific condition is met. They are essential for iterating over arrays, performing repetitive tasks, and controlling program flow.
In JavaScript, we have several types of loops, including for
, while
, do...while
, and the for...in
loop.
Here are some examples:
// Using for loop for (var i = 0; i < 5; i++) { console.log(i); } // Using while loop var count = 0; while (count < 5) { console.log(count); count++; } // Using do...while loop var x = 0; do { console.log(x); x++; } while (x < 5); // Using for...in loop var person = { name: "John", age: 25, city: "New York" }; for (var key in person) { console.log(key + ": " + person[key]); }
In the above examples, we used different types of loops to perform repetitive tasks. The for
loop runs a block of code a specified number of times. The while
loop runs a block of code as long as a condition is true. The do...while
loop is similar to the while
loop, but it always executes the block of code at least once, even if the condition is false. The for...in
loop iterates over the properties of an object.
Events in JavaScript
Events in JavaScript are actions or occurrences that happen in the browser. They can be triggered by the user, such as clicking a button or submitting a form, or they can be triggered by the browser itself, such as when the page finishes loading.
To handle events in JavaScript, we can use event listeners. An event listener is a function that gets called when a specific event occurs. We can attach event listeners to HTML elements to respond to user interactions.
Here are some examples:
// Adding an event listener to a button var button = document.getElementById("myButton"); button.addEventListener("click", function() { console.log("Button clicked"); }); // Adding an event listener to a form var form = document.getElementById("myForm"); form.addEventListener("submit", function(event) { event.preventDefault(); console.log("Form submitted"); });
In the above examples, we added event listeners to a button and a form. When the button is clicked, the function inside the event listener gets called and logs a message to the console. When the form is submitted, the function inside the event listener gets called, and the default form submission behavior is prevented using event.preventDefault()
.
DOM Manipulation in JavaScript
DOM (Document Object Model) manipulation in JavaScript refers to the process of modifying the HTML elements and their attributes on a web page. It allows us to dynamically change the content, style, and structure of a web page based on user interactions or other events.
To manipulate the DOM in JavaScript, we can use methods and properties provided by the document
object.
Here are some examples:
// Changing the text content of an element var heading = document.getElementById("myHeading"); heading.textContent = "New Heading"; // Changing the style of an element var paragraph = document.getElementById("myParagraph"); paragraph.style.color = "red"; // Creating a new element var newElement = document.createElement("p"); newElement.textContent = "New paragraph"; document.body.appendChild(newElement); // Removing an element var oldElement = document.getElementById("oldElement"); oldElement.parentNode.removeChild(oldElement);
In the above examples, we manipulated the DOM by changing the text content of an element, changing the style of an element, creating a new element, and removing an element. We accessed elements using methods like getElementById()
and modified their properties using dot notation.
Related Article: How to Choose: Javascript Href Vs Onclick for Callbacks
Closures in JavaScript
Closures in JavaScript are a useful and often misunderstood concept. They allow functions to access variables from their outer lexical environment even after the outer function has returned. Closures are created when an inner function references variables from its outer function.
Here is an example of a closure:
function outer() { var message = "Hello"; function inner() { console.log(message); } return inner; } var closure = outer(); closure(); // Output: Hello
In the above example, the outer
function defines a variable message
and an inner function inner
that logs the message
to the console. The outer
function returns the inner
function. When we invoke outer
and assign it to the variable closure
, we create a closure. The closure still has access to the message
variable even though the outer
function has finished executing.
Closures are often used to create private variables and encapsulate functionality.
Callbacks in JavaScript
Callbacks in JavaScript are functions that are passed as arguments to other functions and are invoked at a later time. They allow us to control the flow of asynchronous operations, such as making API calls or handling events.
Here is an example of a callback:
function fetchData(callback) { // Simulating an asynchronous operation setTimeout(function() { var data = "Some data"; callback(data); }, 2000); } function processData(data) { console.log("Processing data: " + data); } fetchData(processData); // Output after 2 seconds: Processing data: Some data
In the above example, the fetchData
function simulates an asynchronous operation using setTimeout
. It takes a callback
function as an argument and invokes it after 2 seconds. The processData
function is passed as the callback
argument and logs the processed data to the console.
Callbacks are commonly used in JavaScript to handle asynchronous operations, handle events, and implement control flow patterns like callbacks, promises, and async/await.
How Variables Work in JavaScript
In JavaScript, variables are used to store data values. They are dynamically typed, meaning that the type of a variable is determined at runtime based on the value assigned to it.
When a variable is declared and assigned a value, JavaScript allocates memory to store that value. The variable name serves as a reference to the memory location where the value is stored.
Here is an example:
var x = 5;
In the above example, the variable x
is declared and assigned the value 5
. JavaScript allocates memory to store the value 5
and associates it with the variable x
.
Variables in JavaScript are mutable, which means that their values can be changed during the execution of a program. We can assign a new value to a variable at any time by using the assignment operator (=
).
var x = 5; x = 10; // Changing the value of x
In the above example, the value of the variable x
is initially 5
. We can change the value of x
to 10
by assigning a new value to it.
Variables in JavaScript can also be hoisted, which means that they can be used before they are declared. However, only the variable declaration is hoisted, not the initialization.
console.log(x); // Output: undefined var x = 5;
In the above example, the variable x
is hoisted, but its value is undefined
before the actual assignment.
Understanding how variables work in JavaScript is crucial for writing effective and bug-free code.
Different Types of Functions in JavaScript
In JavaScript, there are several types of functions that serve different purposes and have different ways of being declared and invoked. Understanding the different types of functions is important for writing clean and maintainable code.
Here are some examples of different types of functions in JavaScript:
1. Function Declarations:
function greet(name) { console.log("Hello, " + name + "!"); }
2. Function Expressions:
var greet = function(name) { console.log("Hello, " + name + "!"); };
3. Arrow Functions (ES6):
var greet = (name) => { console.log("Hello, " + name + "!"); };
4. Immediately Invoked Function Expressions (IIFE):
(function() { console.log("Hello, IIFE!"); })();
5. Anonymous Functions:
var greet = function(name) { console.log("Hello, " + name + "!"); };
6. Higher-Order Functions:
function withLogging(fn) { return function(...args) { console.log("Calling function with arguments:", args); var result = fn(...args); console.log("Function returned:", result); return result; }; }
In the above examples, we demonstrated different types of functions. Function declarations and function expressions are the most common ways to define named functions. Arrow functions provide a more concise syntax and lexical scoping of this
. Immediately Invoked Function Expressions (IIFE) are functions that are executed immediately after they are created. Anonymous functions are functions without a name. Higher-order functions are functions that take other functions as arguments or return functions.
Each type of function has its own use case and understanding their differences is important for writing clear and maintainable code.
Related Article: How to Integrate UseHistory from the React Router DOM
Creating and Manipulating Objects in JavaScript
In JavaScript, objects are a fundamental data type that allows us to store multiple values as properties and methods. They are used to represent real-world entities and provide a way to organize and manipulate data.
There are several ways to create and manipulate objects in JavaScript:
1. Object Literal Syntax:
var person = { name: "John", age: 25, isEmployed: true, greet: function() { console.log("Hello, my name is " + this.name); } };
2. Object Constructors:
function Person(name, age, isEmployed) { this.name = name; this.age = age; this.isEmployed = isEmployed; this.greet = function() { console.log("Hello, my name is " + this.name); }; } var person = new Person("John", 25, true);
3. Object.create():
var person = Object.create(null); person.name = "John"; person.age = 25; person.isEmployed = true; person.greet = function() { console.log("Hello, my name is " + this.name); };
4. ES6 Classes:
class Person { constructor(name, age, isEmployed) { this.name = name; this.age = age; this.isEmployed = isEmployed; } greet() { console.log("Hello, my name is " + this.name); } } var person = new Person("John", 25, true);
In the above examples, we demonstrated different ways to create and manipulate objects in JavaScript. Object literal syntax is the simplest way to create an object. Object constructors allow us to define a blueprint for creating multiple objects with the same properties and methods. Object.create()
allows us to create objects with a specified prototype. ES6 classes provide syntactic sugar for creating objects using a class-based syntax.
Once an object is created, we can access and modify its properties using dot notation or bracket notation. We can also add or remove properties and call object methods.
Working with objects is essential for organizing and manipulating data in JavaScript.
Working with Arrays in JavaScript
Arrays in JavaScript are used to store multiple values in a single variable. They are ordered, indexed collections of data that can be of any type.
Working with arrays in JavaScript involves performing various operations, such as accessing elements, modifying elements, adding elements, removing elements, and iterating over elements.
Here are some examples of working with arrays in JavaScript:
1. Accessing Elements:
var numbers = [1, 2, 3, 4, 5]; console.log(numbers[0]); // Output: 1 console.log(numbers[2]); // Output: 3
2. Modifying Elements:
var numbers = [1, 2, 3, 4, 5]; numbers[2] = 10; console.log(numbers); // Output: [1, 2, 10, 4, 5]
3. Adding Elements:
var numbers = [1, 2, 3, 4, 5]; numbers.push(6); console.log(numbers); // Output: [1, 2, 3, 4, 5, 6]
4. Removing Elements:
var numbers = [1, 2, 3, 4, 5]; numbers.pop(); console.log(numbers); // Output: [1, 2, 3, 4]
5. Iterating over Elements:
var numbers = [1, 2, 3, 4, 5]; numbers.forEach(function(number) { console.log(number); });
In the above examples, we demonstrated different operations that can be performed on arrays. We accessed elements using square bracket notation, modified elements by assigning new values, added elements using the push()
method, removed elements using the pop()
method, and iterated over elements using the forEach()
method.
Arrays are widely used in JavaScript for storing and manipulating collections of data.
Understanding JavaScript Conditionals
Conditionals in JavaScript are used to make decisions based on different conditions. They allow us to execute different blocks of code depending on whether a condition is true or false.
In JavaScript, we have several conditional statements, including if
, if...else
, if...else if...else
, and the ternary operator (? :
).
Here are some examples of JavaScript conditionals:
1. if
Statement:
var age = 25; if (age >= 18) { console.log("You are an adult"); }
2. if...else
Statement:
var temperature = 25; if (temperature >= 30) { console.log("It's hot outside"); } else { console.log("It's not too hot"); }
3. if...else if...else
Statement:
var time = 14; if (time < 12) { console.log("Good morning"); } else if (time < 18) { console.log("Good afternoon"); } else { console.log("Good evening"); }
4. Ternary Operator:
var isMember = true; var discount = isMember ? 10 : 0; console.log("Discount: " + discount + "%");
In the above examples, we used different conditional statements to make decisions based on different conditions. The if
statement checks if the age
is greater than or equal to 18 and logs a message if it is true. The if...else
statement checks if the temperature
is greater than or equal to 30 and logs different messages based on the condition. The if...else if...else
statement checks the time
and logs different greetings based on the time of the day. Finally, the ternary operator assigns a value to the discount
variable based on the isMember
condition.
Conditionals are essential for controlling the flow of execution in JavaScript programs.
Different Types of Loops in JavaScript
Loops in JavaScript are used to repeatedly execute a block of code until a specific condition is met. They are essential for iterating over arrays, performing repetitive tasks, and controlling program flow.
In JavaScript, we have several types of loops, including for
, while
, do...while
, and the for...in
loop.
Here are some examples of different types of loops in JavaScript:
1. for
Loop:
for (var i = 0; i < 5; i++) { console.log(i); }
2. while
Loop:
var count = 0; while (count < 5) { console.log(count); count++; }
3. do...while
Loop:
var x = 0; do { console.log(x); x++; } while (x < 5);
4. for...in
Loop:
var person = { name: "John", age: 25, city: "New York" }; for (var key in person) { console.log(key + ": " + person[key]); }
In the above examples, we used different types of loops to perform repetitive tasks. The for
loop runs a block of code a specified number of times. The while
loop runs a block of code as long as a condition is true. The do...while
loop is similar to the while
loop, but it always executes the block of code at least once, even if the condition is false. The for...in
loop iterates over the properties of an object.
Loops are essential for iterating over collections, processing data, and controlling program flow in JavaScript.
Related Article: High Performance JavaScript with Generators and Iterators
Handling Events in JavaScript
Events in JavaScript are actions or occurrences that happen in the browser. They can be triggered by the user, such as clicking a button or submitting a form, or they can be triggered by the browser itself, such as when the page finishes loading.
To handle events in JavaScript, we can use event listeners. An event listener is a function that gets called when a specific event occurs. We can attach event listeners to HTML elements to respond to user interactions.
Here are some examples of handling events in JavaScript:
1. Adding an Event Listener to a Button:
var button = document.getElementById("myButton"); button.addEventListener("click", function() { console.log("Button clicked"); });
2. Adding an Event Listener to a Form:
var form = document.getElementById("myForm"); form.addEventListener("submit", function(event) { event.preventDefault(); console.log("Form submitted"); });
In the above examples, we added event listeners to a button and a form. When the button is clicked, the function inside the event listener gets called and logs a message to the console. When the form is submitted, the function inside the event listener gets called, and the default form submission behavior is prevented using event.preventDefault()
.
Event handling is crucial for building interactive web applications in JavaScript.
DOM Manipulation in JavaScript
DOM (Document Object Model) manipulation in JavaScript refers to the process of modifying the HTML elements and their attributes on a web page. It allows us to dynamically change the content, style, and structure of a web page based on user interactions or other events.
To manipulate the DOM in JavaScript, we can use methods and properties provided by the document
object.
Here are some examples of DOM manipulation in JavaScript:
1. Changing the Text Content of an Element:
var heading = document.getElementById("myHeading"); heading.textContent = "New Heading";
2. Changing the Style of an Element:
var paragraph = document.getElementById("myParagraph"); paragraph.style.color = "red";
3. Creating a New Element:
var newElement = document.createElement("p"); newElement.textContent = "New paragraph"; document.body.appendChild(newElement);
4. Removing an Element:
var oldElement = document.getElementById("oldElement"); oldElement.parentNode.removeChild(oldElement);
In the above examples, we manipulated the DOM by changing the text content of an element, changing the style of an element, creating a new element, and removing an element. We accessed elements using methods like getElementById()
and modified their properties using dot notation.
DOM manipulation is a useful feature of JavaScript that allows us to create dynamic and interactive web pages.
Understanding Closures in JavaScript
Closures in JavaScript are a useful and often misunderstood concept. They allow functions to access variables from their outer lexical environment even after the outer function has returned. Closures are created when an inner function references variables from its outer function.
Here is an example of a closure in JavaScript:
function outer() { var message = "Hello"; function inner() { console.log(message); } return inner; } var closure = outer(); closure(); // Output: Hello
In the above example, the outer
function defines a variable message
and an inner function inner
that logs the message
to the console. The outer
function returns the inner
function. When we invoke outer
and assign it to the variable closure
, we create a closure. The closure still has access to the message
variable even though the outer
function has finished executing.
Closures are often used to create private variables and encapsulate functionality. They allow us to maintain state and access variables that are no longer in scope.
Understanding closures is essential for writing advanced JavaScript code and leveraging their power in various scenarios.
Working with Callbacks in JavaScript
Callbacks in JavaScript are functions that are passed as arguments to other functions and are invoked at a later time. They allow us to control the flow of asynchronous operations, such as making API calls or handling events.
Here is an example of a callback in JavaScript:
function fetchData(callback) { // Simulating an asynchronous operation setTimeout(function() { var data = "Some data"; callback(data); }, 2000); } function processData(data) { console.log("Processing data: " + data); } fetchData(processData); // Output after 2 seconds: Processing data: Some data
In the above example, the fetchData
function simulates an asynchronous operation using setTimeout
. It takes a callback
function as an argument and invokes it after 2 seconds. The processData
function is passed as the callback
argument and logs the processed data to the console.
Callbacks are commonly used in JavaScript to handle asynchronous operations, handle events, and implement control flow patterns like callbacks, promises, and async/await.
Related Article: How to Incorporate a JavaScript Class into a Component
Additional Resources
- JavaScript Variables