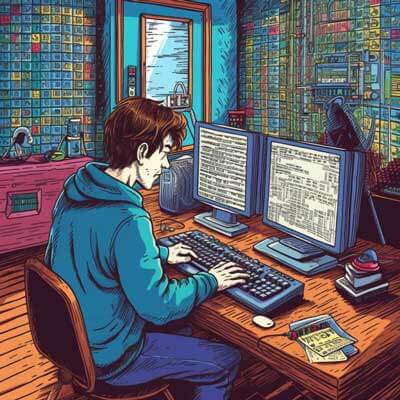
- Getting Started with Reactive Forms in Angular
- Creating Reactive Forms
- Form Controls and Form Groups
- Validation in Reactive Forms
- Handling Form Submissions
- Error Handling in Reactive Forms
- Using Reactive Forms in Complex Web Apps
- Best Practices for Reactive Forms
- 1. Use Form Builders
- 2. Separate Validation Logic
- 3. Use Reactive Form Directives
- Real World Examples of Reactive Forms
- 1. User Registration Form
- 2. Product Order Form
- 3. Contact Us Form
- 4. Multi-Step Form
- Performance Considerations for Reactive Forms
- 1. Change Detection
- 2. Lazy Loading
- 3. Unsubscribing from Observables
- Advanced Techniques for Reactive Forms
- 1. Dynamic Form Controls
- 2. Custom Form Control Components
- Code Snippet 1: Creating a Basic Reactive Form
- Code Snippet 2: Dynamically Adding Form Controls
- Code Snippet 3: Custom Validators in Reactive Forms
- Code Snippet 4: Conditional Validation in Reactive Forms
- Code Snippet 5: Reactive Forms with Nested Form Groups
Angular Reactive Forms provide a useful and flexible way to handle form input validation and data binding in Angular applications. Unlike template-driven forms, reactive forms allow for a more programmatic approach, where form controls and validations are defined in the component class. This gives developers more control over the form’s behavior and allows for easier testing and maintainability.
Reactive forms are built around the concept of observables, which are streams of data that can be observed and reacted to. This makes it easier to handle asynchronous operations and form input changes.
In this chapter, we will explore the basics of Angular Reactive Forms and how to get started using them in your applications.
Getting Started with Reactive Forms in Angular
To get started with reactive forms in Angular, you will need to import the necessary modules and create a form group in your component.
First, import the required modules from the @angular/forms
package in your component file:
import { FormGroup, FormControl, Validators } from '@angular/forms';
Next, create a form group in your component class:
export class MyComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]), password: new FormControl('', Validators.minLength(6)), }); } }
In the above example, we define three form controls: name
, email
, and password
. The second argument to the FormControl
constructor allows us to specify one or more validators for each control. In this case, we use the Validators.required
and Validators.email
validators for the email
control, and the Validators.minLength(6)
validator for the password
control.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Creating Reactive Forms
Creating reactive forms involves defining form controls and form groups to represent the input fields and their validations.
To create a basic reactive form, you need to:
1. Import the necessary form-related modules from @angular/forms
.
2. Create an instance of the FormGroup
class.
3. Define form controls using instances of the FormControl
class.
4. Associate form controls with the form group.
Here’s an example of creating a basic reactive form in Angular:
import { Component } from '@angular/core'; import { FormGroup, FormControl } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl(''), email: new FormControl(''), password: new FormControl('') }); } }
In the above example, we import the necessary modules from @angular/forms
and define a class MyFormComponent
. Inside the constructor, we create an instance of FormGroup
and define three form controls: name
, email
, and password
. Each form control is an instance of FormControl
, which accepts an initial value as an argument.
Form Controls and Form Groups
In Angular Reactive Forms, form controls and form groups are the building blocks for creating forms.
A form control represents an individual input field and its validation rules. It can be a text input, checkbox, radio button, or any other HTML form element. Form controls are instances of the FormControl
class.
A form group is a collection of form controls and/or other form groups. It represents a section of the form and provides a way to organize related form controls. Form groups are instances of the FormGroup
class.
Here’s an example of using form controls and form groups in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ personalInfo: new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]) }), password: new FormControl('', Validators.minLength(6)) }); } }
In the above example, we create a form group called personalInfo
that contains two form controls: name
and email
. We also have a standalone form control called password
. By organizing the form controls into form groups, we create a hierarchical structure that reflects the structure of our form.
Validation in Reactive Forms
Validation is an important aspect of form handling. Angular Reactive Forms provide a rich set of built-in validators that can be used to enforce validation rules on form controls.
To apply validation to a form control, you can pass one or more validators as the second argument to the FormControl
constructor. Validators can be synchronous or asynchronous.
Here’s an example of using built-in validators in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]), password: new FormControl('', Validators.minLength(6)) }); } }
In this example, the name
control is required, the email
control is required and must be a valid email address, and the password
control must have a minimum length of 6 characters.
You can also create custom validators by defining a function that takes a FormControl
object as an argument and returns an object with validation errors, if any. Custom validators can be useful when you need to enforce complex validation rules that are not covered by the built-in validators.
Related Article: How To Use Loop Inside React JSX
Handling Form Submissions
Handling form submissions in Angular Reactive Forms involves listening to the submit
event on the form and reacting to it in the component class.
To handle form submissions, you can attach a method to the submit
event of the form element and implement the desired logic inside that method.
Here’s an example of handling form submissions in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]), password: new FormControl('', Validators.minLength(6)) }); } onSubmit() { if (this.myForm.valid) { // Perform form submission logic here } } }
In this example, we define a method called onSubmit
in the component class, which is called when the form is submitted. Inside this method, we can perform any desired logic, such as sending the form data to a server or displaying a success message.
The valid
property of the form group can be used to check if the form is valid before performing the submission logic. If the form is invalid, you can display error messages or take other appropriate actions.
Error Handling in Reactive Forms
Error handling is an important aspect of form validation in Angular Reactive Forms. When a form control fails validation, you can display error messages to the user to provide feedback on what went wrong.
Angular Reactive Forms provide several mechanisms for error handling, including accessing individual control errors, displaying error messages, and applying CSS classes to highlight invalid fields.
Here’s an example of error handling in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]), password: new FormControl('', Validators.minLength(6)) }); } get nameControl() { return this.myForm.get('name'); } get emailControl() { return this.myForm.get('email'); } }
In this example, we define two getter methods, nameControl
and emailControl
, which return the corresponding form controls. These methods can be used in the template to access control errors and display error messages.
<div> <label for="name">Name</label> <div> <div>Name is required.</div> </div> </div> <div> <label for="email">Email</label> <div> <div>Email is required.</div> <div>Email is invalid.</div> </div> </div> <!-- ... -->
In the template, we bind the form controls to the input fields using the formControlName
directive. We also use the *ngIf
directive to conditionally display error messages based on control validity and whether the control has been touched by the user.
Using Reactive Forms in Complex Web Apps
Reactive forms are well-suited for complex web applications where forms can have dynamic behavior and complex validation rules.
In complex web apps, you may encounter scenarios where form controls need to be dynamically added or removed, form groups need to be nested, or custom form control components need to be created.
Dynamic Addition of Form Controls
In some cases, you may need to dynamically add form controls to a reactive form. This can be done using the addControl
method of the form group.
Here’s an example of dynamically adding form controls in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]) }); } addAddressControl() { this.myForm.addControl('address', new FormControl('', Validators.required)); } }
In this example, we define a method called addAddressControl
that adds a new form control called address
to the form group. The new control is created using the FormControl
constructor, and we specify the Validators.required
validator for the control.
Custom Validators in Reactive Forms
In addition to the built-in validators provided by Angular Reactive Forms, you can create custom validators to enforce specific validation rules.
To create a custom validator, you need to define a function that takes a FormControl
object as an argument and returns an object with validation errors, if any. The function can then be used as a validator for a form control.
Here’s an example of creating a custom validator in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators, AbstractControl } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]), password: new FormControl('', [Validators.required, this.customPasswordValidator]) }); } customPasswordValidator(control: AbstractControl) { if (control.value && control.value.length < 8) { return { invalidPassword: true }; } return null; } }
In this example, we define a custom validator called customPasswordValidator
that checks if the password has a minimum length of 8 characters. If the validation fails, the validator returns an object with the invalidPassword
property set to true
. Otherwise, it returns null
.
The custom validator is then used as one of the validators for the password
control.
Conditional Validation in Reactive Forms
In some cases, you may need to apply validation to a form control based on the value of another control. This is known as conditional validation.
To implement conditional validation in Angular Reactive Forms, you can use the setValidators
method of a form control to dynamically set or remove validators based on certain conditions.
Here’s an example of conditional validation in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ email: new FormControl('', Validators.required), password: new FormControl('') }); this.myForm.get('email').valueChanges.subscribe(email => { const passwordControl = this.myForm.get('password'); if (email === 'admin@example.com') { passwordControl.setValidators(Validators.required); } else { passwordControl.clearValidators(); } passwordControl.updateValueAndValidity(); }); } }
In this example, we subscribe to the valueChanges
observable of the email
control. Whenever the value of the email
control changes, we check if it matches a certain condition (e.g., if the email is ‘admin@example.com’). Based on the condition, we dynamically add or remove the Validators.required
validator from the password
control using the setValidators
and clearValidators
methods. Finally, we call updateValueAndValidity
to update the validation status of the control.
Reactive Forms with Nested Form Groups
In complex web apps, you may need to organize form controls into nested form groups to represent more complex data structures.
To create nested form groups in Angular Reactive Forms, you can define form groups within form groups.
Here’s an example of using nested form groups in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ personalInfo: new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]) }), address: new FormGroup({ street: new FormControl(''), city: new FormControl(''), state: new FormControl(''), zip: new FormControl('') }) }); } }
In this example, we define a nested form group called personalInfo
, which contains the name
and email
form controls. We also have a standalone form group called address
, which contains the street
, city
, state
, and zip
form controls.
This concludes the chapter on using reactive forms in complex web apps. By leveraging the power of reactive forms, you can create highly dynamic and flexible forms that meet the requirements of your application.
Related Article: How To Upgrade Node.js To The Latest Version
Best Practices for Reactive Forms
When working with Angular Reactive Forms, it’s important to follow certain best practices to ensure clean and maintainable code.
1. Use Form Builders
The FormBuilder
class in Angular provides a more concise and readable way to create form groups and controls compared to creating them manually.
Using the FormBuilder
, you can define form controls and groups using a fluent, chainable syntax.
Here’s an example of using the FormBuilder
to create a form group with form controls:
import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor(private formBuilder: FormBuilder) { this.myForm = this.formBuilder.group({ name: ['', Validators.required], email: ['', [Validators.required, Validators.email]], password: ['', Validators.minLength(6)], }); } }
In this example, we inject the FormBuilder
into the component’s constructor and use it to create a form group with form controls. The group
method of the FormBuilder
takes an object where each key represents the control name and the value represents the control’s initial value and validators.
Using the FormBuilder
can make your code more readable and less error-prone, especially for complex forms.
2. Separate Validation Logic
To keep your code organized and maintainable, it’s a good practice to separate the validation logic from the component class.
Instead of defining all the validators inline, you can create reusable validator functions and import them into your component.
Here’s an example of separating validation logic in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl } from '@angular/forms'; import { customEmailValidator } from './validators'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ email: new FormControl('', [Validators.required, customEmailValidator]), }); } }
In this example, we define a custom validator function called customEmailValidator
in a separate file. This function checks if the email is valid using a regular expression, for example.
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
3. Use Reactive Form Directives
Angular provides a set of directives that can be used with reactive forms to simplify common form-related tasks. These directives enhance the functionality and readability of your template code.
Instead of manually binding form controls to input fields using the [formControl]
directive, you can use the formControlName
directive, which is specifically designed for reactive forms.
Here’s an example of using the formControlName
directive in Angular Reactive Forms:
<label>Name</label>
In this example, we bind the name
form control to the input field using the formControlName
directive. This directive automatically connects the form control to the input field and updates the control’s value as the user types.
Real World Examples of Reactive Forms
Reactive forms are widely used in various types of web applications to handle complex form input and validation requirements. Here are a few real-world examples of how reactive forms can be used in practice:
1. User Registration Form
A user registration form typically includes fields like name, email, password, and other optional information. Reactive forms can handle the input validation and submission logic for such forms, ensuring that all required fields are filled out and that email addresses are valid.
Related Article: How To Fix Javascript: $ Is Not Defined
2. Product Order Form
An e-commerce application may have a product order form where users can select products, specify quantities, and provide shipping and payment information. Reactive forms can be used to handle the validation and submission of this complex form, ensuring that all required fields are filled out correctly and that the order is processed accurately.
3. Contact Us Form
A contact form on a website allows users to send messages to the website owner or support team. Reactive forms can be used to validate and submit the form, ensuring that the user provides a valid email address and a message before sending.
4. Multi-Step Form
A multi-step form is a form that is split into multiple sections or steps. Each step collects a subset of information from the user. Reactive forms can be used to handle the validation and navigation logic for such forms, ensuring that the user completes each step correctly before moving on to the next.
These examples demonstrate the versatility and power of reactive forms in handling various form-related scenarios. By leveraging the features and flexibility of reactive forms, you can build robust and user-friendly web applications.
Related Article: Advanced Node.js: Event Loop, Async, Buffer, Stream & More
Performance Considerations for Reactive Forms
While Angular Reactive Forms provide a powerful and flexible way to handle form input and validation, it’s important to consider performance implications, especially for large and complex forms.
Here are some performance considerations to keep in mind when working with reactive forms in Angular:
1. Change Detection
Angular’s change detection mechanism is responsible for detecting changes in the component’s data and updating the view accordingly. When working with reactive forms, the change detection process can become more expensive as the number of form controls and form groups increases.
To optimize performance, you can use the ChangeDetectionStrategy.OnPush
strategy for your component. This strategy tells Angular to only run change detection when the component’s inputs change or when an event is triggered explicitly. This can significantly improve performance for forms with many controls and reduce unnecessary change detection cycles.
2. Lazy Loading
If your form contains sections or fields that are not visible initially or are conditionally shown based on user actions, you can consider lazy loading those sections or fields. This means that the associated form controls are not created or added to the form group until they are needed.
Related Article: Advanced DB Queries with Nodejs, Sequelize & Knex.js
3. Unsubscribing from Observables
When working with reactive forms, it’s common to subscribe to observables like valueChanges
to listen for changes in form controls. However, it’s important to remember to unsubscribe from these observables when they are no longer needed, to prevent memory leaks.
You can use the takeUntil
operator along with a subject to manage subscriptions and unsubscribe automatically when the component is destroyed.
Here’s an example of unsubscribing from observables in Angular Reactive Forms:
import { Component, OnDestroy } from '@angular/core'; import { FormGroup, FormControl } from '@angular/forms'; import { Subject } from 'rxjs'; import { takeUntil } from 'rxjs/operators'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent implements OnDestroy { myForm: FormGroup; private destroy$ = new Subject(); constructor() { this.myForm = new FormGroup({ name: new FormControl('') }); this.myForm.valueChanges.pipe( takeUntil(this.destroy$) ).subscribe(value => { // Handle form value changes }); } ngOnDestroy() { this.destroy$.next(); this.destroy$.complete(); } }
In this example, we create a private destroy$
subject and use the takeUntil
operator to unsubscribe from the valueChanges
observable when the component is destroyed. In the ngOnDestroy
lifecycle hook, we emit a completion signal to the destroy$
subject to complete the subscription.
Advanced Techniques for Reactive Forms
Angular Reactive Forms provide several advanced techniques that can be used to handle complex form-related scenarios. These techniques can enhance the functionality and user experience of your forms.
1. Dynamic Form Controls
Dynamic form controls allow you to add or remove form controls dynamically based on user actions or other conditions. This can be useful when you have a form with variable numbers of fields or when you want to allow users to add or remove input fields on the fly.
To handle dynamic form controls in Angular Reactive Forms, you can use the FormArray
class, which represents an array of form controls.
Here’s an example of using dynamic form controls in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, FormArray, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ items: new FormArray([]) }); } get itemsControl() { return this.myForm.get('items') as FormArray; } addItem() { const item = new FormControl('', Validators.required); this.itemsControl.push(item); } removeItem(index: number) { this.itemsControl.removeAt(index); } }
In this example, we define a form array called items
inside the form group. The itemsControl
getter method returns the form array as a FormArray
object, which allows us to use methods like push
and removeAt
to add or remove form controls dynamically.
The addItem
method adds a new form control to the items
form array, and the removeItem
method removes a form control at a specific index.
Related Article: Implementing i18n and l10n in Your Node.js Apps
2. Custom Form Control Components
In addition to using built-in form controls like text inputs and checkboxes, you can create custom form control components in Angular Reactive Forms. Custom form controls allow you to encapsulate complex logic and behavior into reusable components.
To create a custom form control component, you need to implement the ControlValueAccessor
interface and provide the necessary methods for reading and writing the value of the control.
Here’s an example of creating a custom form control component in Angular Reactive Forms:
import { Component, forwardRef } from '@angular/core'; import { ControlValueAccessor, NG_VALUE_ACCESSOR } from '@angular/forms'; @Component({ selector: 'app-custom-input', template: ` `, providers: [ { provide: NG_VALUE_ACCESSOR, useExisting: forwardRef(() => CustomInputComponent), multi: true } ] }) export class CustomInputComponent implements ControlValueAccessor { value: string; onChange: any = () => {}; writeValue(value: any) { this.value = value; } registerOnChange(fn: any) { this.onChange = fn; } registerOnTouched() {} setDisabledState() {} }
In this example, we create a custom input component that wraps an HTML input element. The custom input component implements the ControlValueAccessor
interface, which requires the implementation of several methods, such as writeValue
, registerOnChange
, and registerOnTouched
.
The writeValue
method is called by Angular when the value of the control needs to be updated. The registerOnChange
method is called to register a callback function that is called whenever the value of the control changes. The registerOnTouched
and setDisabledState
methods are also part of the ControlValueAccessor
interface but are not used in this example.
These advanced techniques demonstrate the flexibility and extensibility of Angular Reactive Forms. By mastering these techniques, you can handle a wide range of form-related scenarios and build more powerful and user-friendly applications.
Code Snippet 1: Creating a Basic Reactive Form
Here’s an example of creating a basic reactive form in Angular:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ name: new FormControl(''), email: new FormControl(''), password: new FormControl('') }); } }
In this example, we import the necessary modules from @angular/forms
and define a class MyFormComponent
. Inside the constructor, we create an instance of FormGroup
and define three form controls: name
, email
, and password
. Each form control is an instance of FormControl
, which accepts an initial value as an argument.
The name
, email
, and password
form controls are then associated with the form group using their respective names.
This basic reactive form can be used as a starting point for building more complex forms in Angular.
Code Snippet 2: Dynamically Adding Form Controls
Here’s an example of dynamically adding form controls in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ items: new FormArray([]) }); } get itemsControl() { return this.myForm.get('items') as FormArray; } addItem() { const item = new FormControl('', Validators.required); this.itemsControl.push(item); } removeItem(index: number) { this.itemsControl.removeAt(index); } }
In this example, we define a form array called items
inside the form group. The itemsControl
getter method returns the form array as a FormArray
object, which allows us to use methods like push
and removeAt
to add or remove form controls dynamically.
The addItem
method adds a new form control to the items
form array, and the removeItem
method removes a form control at a specific index.
This dynamic form control functionality can be useful when you have a form with variable numbers of fields or when you want to allow users to add or remove input fields on the fly.
Related Article: Big Data Processing with Node.js and Apache Kafka
Code Snippet 3: Custom Validators in Reactive Forms
Here’s an example of creating a custom validator in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators, AbstractControl } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ email: new FormControl('', [Validators.required, Validators.email]), password: new FormControl('', [Validators.required, this.customPasswordValidator]) }); } customPasswordValidator(control: AbstractControl) { if (control.value && control.value.length < 8) { return { invalidPassword: true }; } return null; } }
In this example, we define a custom validator called customPasswordValidator
that checks if the password has a minimum length of 8 characters. If the validation fails, the validator returns an object with the invalidPassword
property set to true
. Otherwise, it returns null
.
The custom validator is then used as one of the validators for the password
control. When the form is submitted, the custom validator will be applied to the password
control, and if the password is not at least 8 characters long, the form will be considered invalid.
Using custom validators allows you to enforce complex validation rules that are not covered by the built-in validators provided by Angular Reactive Forms.
Code Snippet 4: Conditional Validation in Reactive Forms
Here’s an example of implementing conditional validation in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ email: new FormControl('', Validators.required), password: new FormControl('') }); this.myForm.get('email').valueChanges.subscribe(email => { const passwordControl = this.myForm.get('password'); if (email === 'admin@example.com') { passwordControl.setValidators(Validators.required); } else { passwordControl.clearValidators(); } passwordControl.updateValueAndValidity(); }); } }
In this example, we subscribe to the valueChanges
observable of the email
control. Whenever the value of the email
control changes, we check if it matches a certain condition (e.g., if the email is ‘admin@example.com’). Based on the condition, we dynamically add or remove the Validators.required
validator from the password
control using the setValidators
and clearValidators
methods. Finally, we call updateValueAndValidity
to update the validation status of the control.
Code Snippet 5: Reactive Forms with Nested Form Groups
Here’s an example of using nested form groups in Angular Reactive Forms:
import { Component } from '@angular/core'; import { FormGroup, FormControl, Validators } from '@angular/forms'; @Component({ selector: 'app-my-form', templateUrl: './my-form.component.html', styleUrls: ['./my-form.component.css'] }) export class MyFormComponent { myForm: FormGroup; constructor() { this.myForm = new FormGroup({ personalInfo: new FormGroup({ name: new FormControl('', Validators.required), email: new FormControl('', [Validators.required, Validators.email]) }), address: new FormGroup({ street: new FormControl(''), city: new FormControl(''), state: new FormControl(''), zip: new FormControl('') }) }); } }
In this example, we define a nested form group called personalInfo
, which contains the name
and email
form controls. We also have a standalone form group called address
, which contains the street
, city
, state
, and zip
form controls.
This nested form group example can be expanded upon to build forms with even more complex structures and validation requirements.