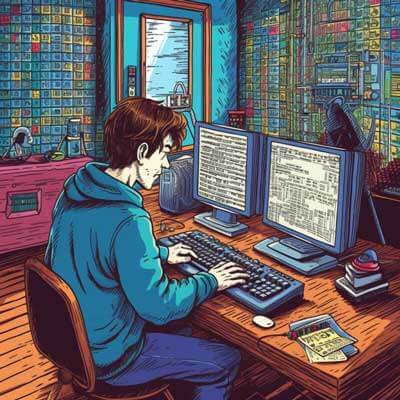
Table of Contents
Parsing a string to a date in JavaScript can be done using the built-in Date object and its methods. Here are two possible approaches to accomplish this task:
Approach 1: Using the Date constructor
One way to parse a string to a date in JavaScript is by using the Date constructor. The Date constructor accepts various formats for the date string, including ISO 8601, RFC 2822, and a few others. Here's an example:
const dateString = '2022-03-15'; const date = new Date(dateString); console.log(date); // Output: Wed Mar 15 2022 00:00:00 GMT+0000 (Coordinated Universal Time)
In this example, we create a new Date object by passing the date string '2022-03-15' to the Date constructor. The resulting date object represents the parsed date.
It's important to note that the date string should be in a format recognized by the Date constructor. If the string format is not recognized, the Date object will default to an "Invalid Date" representation.
Related Article: How to Scroll to the Top of a Page Using Javascript
Approach 2: Using the Date.parse() method
Another way to parse a string to a date in JavaScript is by using the Date.parse() method. The Date.parse() method parses a date string and returns the number of milliseconds since January 1, 1970, 00:00:00 UTC. Here's an example:
const dateString = '2022-03-15'; const milliseconds = Date.parse(dateString); const date = new Date(milliseconds); console.log(date); // Output: Wed Mar 15 2022 00:00:00 GMT+0000 (Coordinated Universal Time)
In this example, we first use the Date.parse() method to parse the date string '2022-03-15' and obtain the number of milliseconds representing that date. We then create a new Date object by passing the milliseconds value to the Date constructor.
Using the Date.parse() method allows for more flexibility in terms of the input date string format. It can handle a wider range of formats, including some non-standard formats. However, it's still important to ensure that the input string follows a valid date format to obtain accurate results.
Best Practices
Related Article: How to Access Cookies Using Javascript
When parsing a string to a date in JavaScript, it's important to follow some best practices to ensure accurate results:
1. Specify the date format: When parsing a date string, it's recommended to use a well-defined date format, such as ISO 8601 (e.g., 'YYYY-MM-DD') or RFC 2822 (e.g., 'Dow, DD Mon YYYY HH:mm:ss'). Specifying the format helps avoid ambiguity and ensures consistent parsing across different platforms and environments.
2. Validate the input: Before parsing a date string, consider validating the input to ensure it follows the expected format. This can help catch potential errors or inconsistencies in the input before attempting to parse it.
3. Use UTC for consistency: When working with dates, consider using UTC (Coordinated Universal Time) to ensure consistency across different time zones. JavaScript's Date object provides methods such as getUTCFullYear(), getUTCMonth(), and getUTCDate() for retrieving the UTC components of a date.
4. Consider using third-party libraries: JavaScript has several third-party libraries, such as Moment.js and Luxon, that provide advanced date parsing and manipulation capabilities. These libraries can handle complex date parsing scenarios and offer additional features not available in the built-in Date object.