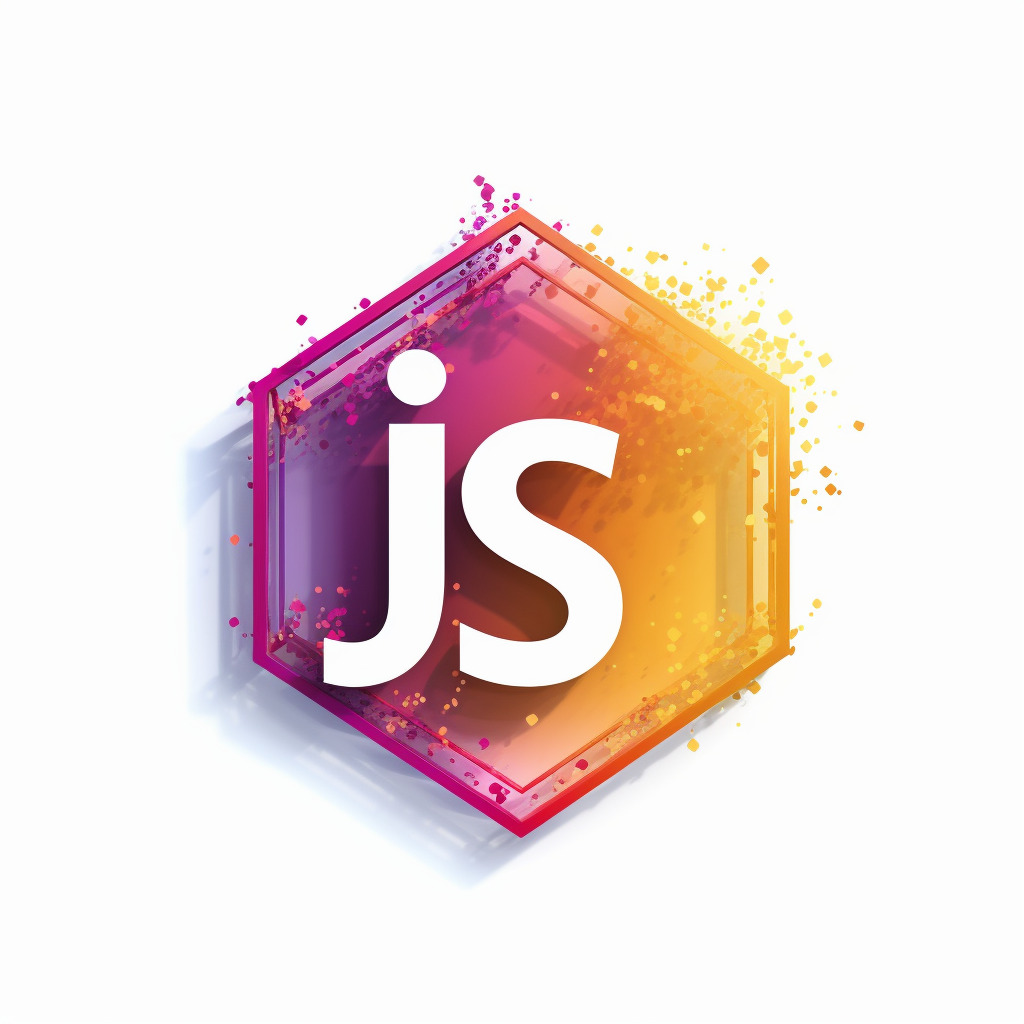
- Understanding Prototypes
- Creating Prototypes
- Manipulating Prototypes
- Benefits of Prototype Inheritance
- Use Cases of Prototype Inheritance
- Mixins
- Object.create()
- Leveraging Prototype Chains for Efficient Code Organization
- Prototypal Inheritance
- Classical Inheritance
- Creating Custom Inheritance Patterns
- Inheriting from a Parent Constructor
- Real World Examples of Prototype Inheritance in Action
- Composition
- Common Pitfalls and Best Practices in Prototype Inheritance
- Avoiding Prototype Pollution
- Modifying the Prototype Object Directly
- Shadowing Properties
- Overusing Inheritance
- Using Object.create() for Inheritance
Understanding Prototypes
One of the key features of JavaScript is its prototype-based inheritance system, which allows objects to inherit properties and methods from other objects. Understanding how prototype inheritance works is crucial for any JavaScript developer.
In JavaScript, every object has an internal property called [[Prototype]]. This property refers to another object, called the prototype object. When we access a property or method on an object, JavaScript first looks for it on the object itself. If it is not found, it then looks for it on the object’s prototype. This process continues recursively until the property or method is found or until the end of the prototype chain is reached.
Let’s illustrate this concept with an example. Consider the following code snippet:
let person = { name: 'John', age: 30, greet() { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); } }; let john = { __proto__: person, occupation: 'Engineer' }; john.greet(); // Output: Hello, my name is John and I am 30 years old.
In this example, we have two objects: person
and john
. The person
object has a name
property, an age
property, and a greet
method. The john
object is created with person
as its prototype using the __proto__
property. As a result, the john
object inherits the name
and age
properties, as well as the greet
method from its prototype.
When we call the greet
method on the john
object, JavaScript first checks if the method exists on the john
object itself. Since it doesn’t, it looks for it on the prototype object (person
). The method is found on the prototype, and it is executed with the john
object as the value of this
.
Related Article: How To Merge Arrays And Remove Duplicates In Javascript
Creating Prototypes
There are multiple ways to create prototypes in JavaScript. One common approach is to use constructor functions and the new
keyword. Constructor functions are regular functions that are used to create objects.
Here’s an example that demonstrates how to create prototypes using constructor functions:
function Animal(name) { this.name = name; } Animal.prototype.sayName = function() { console.log(`My name is ${this.name}.`); }; let dog = new Animal('Rex'); dog.sayName(); // Output: My name is Rex.
In this example, we define a constructor function called Animal
that takes a name
parameter. Inside the constructor function, we assign the name
parameter to the name
property of the newly created object using the this
keyword.
We then add a sayName
method to the prototype of the Animal
constructor function. This method will be shared by all instances of Animal
objects.
Finally, we create a new instance of the Animal
object called dog
and call the sayName
method on it. Since dog
is an instance of Animal
, it has access to the sayName
method through prototype inheritance.
Manipulating Prototypes
JavaScript provides several methods to manipulate prototypes. One such method is the Object.create()
method, which allows us to create a new object with a specified prototype.
Here’s an example that demonstrates how to use the Object.create()
method:
let person = { name: 'John', age: 30 }; let john = Object.create(person); john.occupation = 'Engineer'; console.log(john.name); // Output: John console.log(john.age); // Output: 30
In this example, we create a new object called person
with properties name
and age
. We then create a new object called john
using Object.create(person)
, which sets the prototype of john
to the person
object.
As a result, the john
object inherits the name
and age
properties from the person
object. We can access these properties directly on the john
object.
Benefits of Prototype Inheritance
Prototype inheritance is a powerful feature of JavaScript that allows objects to inherit properties and methods from other objects. This concept provides several benefits and use cases that make it an essential tool for developers. In this chapter, we will explore some of the key advantages of prototype inheritance and discuss how it can be used effectively in JavaScript programming.
Code Reusability
One of the main benefits of prototype inheritance is code reusability. With prototype inheritance, you can define common properties and methods in a prototype object and have other objects inherit those properties and methods. This allows you to avoid duplicating code and promotes a modular approach to programming.
Let’s take a look at an example to illustrate this concept. Suppose we have a prototype object called Animal
, which has a name
property and a speak
method.
function Animal(name) { this.name = name; } Animal.prototype.speak = function() { console.log('Animal speaks'); };
Now, we can create multiple objects that inherit from the Animal
prototype.
function Dog(name) { Animal.call(this, name); } Dog.prototype = Object.create(Animal.prototype); Dog.prototype.constructor = Dog; Dog.prototype.bark = function() { console.log('Dog barks'); }; var dog = new Dog('Buddy'); dog.speak(); // Output: Animal speaks dog.bark(); // Output: Dog barks
In this example, the Dog
object inherits the name
property and speak
method from the Animal
prototype. We can then add additional methods specific to dogs, such as the bark
method. This promotes code reusability, as we don’t need to redefine the common properties and methods for each individual object.
Dynamic Behavior
Prototype inheritance also allows for dynamic behavior in JavaScript objects. Since objects can inherit properties and methods from their prototypes, we can modify or extend the behavior of an object at runtime.
Consider the following example:
function Vehicle() {} Vehicle.prototype.start = function() { console.log('Vehicle started'); }; function Car() {} Car.prototype = Object.create(Vehicle.prototype); Car.prototype.constructor = Car; Car.prototype.start = function() { console.log('Car started'); }; var vehicle = new Vehicle(); var car = new Car(); vehicle.start(); // Output: Vehicle started car.start(); // Output: Car started
In this example, the Car
object inherits the start
method from the Vehicle
prototype. However, we override the behavior of the start
method in the Car
prototype to provide a customized implementation for cars. This dynamic behavior allows us to modify the behavior of objects without altering the original prototype.
Flexible Object Creation
Prototype inheritance enables flexible object creation in JavaScript. It allows us to create objects that share common properties and methods, while also providing the flexibility to modify or extend the behavior of individual objects.
For example, suppose we have a prototype object called Shape
, which has a draw
method. We can create multiple objects that inherit from the Shape
prototype and define their own draw
method.
function Shape() {} Shape.prototype.draw = function() { console.log('Shape is drawn'); }; function Circle() {} Circle.prototype = Object.create(Shape.prototype); Circle.prototype.constructor = Circle; Circle.prototype.draw = function() { console.log('Circle is drawn'); }; function Square() {} Square.prototype = Object.create(Shape.prototype); Square.prototype.constructor = Square; Square.prototype.draw = function() { console.log('Square is drawn'); }; var circle = new Circle(); var square = new Square(); circle.draw(); // Output: Circle is drawn square.draw(); // Output: Square is drawn
In this example, both the Circle
and Square
objects inherit the draw
method from the Shape
prototype. However, each object defines its own implementation of the draw
method, allowing for flexible object creation.
Related Article: JavaScript HashMap: A Complete Guide
Use Cases of Prototype Inheritance
Prototype inheritance can be used in various scenarios to enhance code organization and promote code reusability. Here are a few common use cases:
– Inheritance and the Prototype Chain: Prototype inheritance is the foundation for implementing inheritance and the prototype chain in JavaScript. It allows objects to inherit properties and methods from other objects, creating a hierarchical relationship between objects.
– Object Composition: Prototype inheritance can be used to compose objects by combining multiple prototypes into a single object. This approach promotes code reuse and modular design.
– Extending Built-in Objects: Prototype inheritance allows you to extend built-in JavaScript objects, such as Array
or String
, by adding custom methods to their prototypes. This can be useful for adding functionality to existing objects without modifying their original implementation.
– Object.create(): The Object.create()
method uses prototype inheritance to create a new object with a specified prototype object. This provides a flexible way to create objects with shared behavior.
These are just a few examples of the many use cases of prototype inheritance in JavaScript. By understanding and leveraging this powerful feature, you can enhance your code organization, promote code reusability, and create more flexible and dynamic objects.
Mixins
Mixins are a powerful technique in JavaScript that allow us to combine multiple objects and their prototypes into a single object. This can be useful when we want to reuse functionality across different objects without the need for inheritance.
Let’s say we have two objects, car
and boat
, and we want both of them to have the ability to move. Instead of creating a parent class with a move()
method and having car
and boat
inherit from it, we can use mixins to add the move()
method to both objects.
// Define a mixin object with the move method const movementMixin = { move() { console.log("Moving..."); } }; // Create car object const car = { brand: "Toyota" }; // Create boat object const boat = { type: "Sailboat" }; // Mix the movementMixin into both car and boat Object.assign(car, movementMixin); Object.assign(boat, movementMixin); car.move(); // Output: Moving... boat.move(); // Output: Moving...
In the code snippet above, we define a movementMixin
object that has a move()
method. Then, we use Object.assign()
to mix the movementMixin
into both the car
and boat
objects. Now, both car
and boat
have access to the move()
method.
Object.create()
The Object.create()
method allows us to create a new object with a specified prototype object. This can be useful when we want to create an object that inherits from another object without the need for a constructor function.
// Create a prototype object const animal = { eat() { console.log("Eating..."); } }; // Create a new object with animal as its prototype const dog = Object.create(animal); // Add properties to the dog object dog.breed = "Labrador"; dog.name = "Buddy"; console.log(dog.breed); // Output: Labrador console.log(dog.name); // Output: Buddy dog.eat(); // Output: Eating...
In the code snippet above, we create a animal
object with an eat()
method. Then, we use Object.create()
to create a new object dog
that inherits from animal
. We can then add properties to the dog
object and it also has access to the eat()
method defined in the prototype.
Related Article: How To Remove Duplicates From A Javascript Array
Leveraging Prototype Chains for Efficient Code Organization
In JavaScript, prototype inheritance plays a crucial role in defining the behavior and structure of objects. By understanding prototype chains, we can leverage them to organize our code more efficiently and create reusable code patterns.
Understanding Prototype Chains
Prototype chains are a mechanism in JavaScript that allows objects to inherit properties and methods from other objects. Every object in JavaScript has a prototype, which is another object that it inherits from. This forms a chain of prototypes, hence the name “prototype chain.”
Let’s consider an example to better understand prototype chains:
function Person(name) { this.name = name; } Person.prototype.greet = function() { console.log(`Hello, my name is ${this.name}.`); } const john = new Person('John'); john.greet(); // Output: Hello, my name is John.
In this example, the Person
function acts as a constructor for creating Person
objects. The greet
method is defined on the Person.prototype
object, which means that all Person
objects created using the constructor will have access to this method through the prototype chain.
Leveraging Prototype Chains
By leveraging prototype chains, we can organize our code in a more modular and efficient way. Here are a few ways to do that:
1. Creating reusable methods: By adding methods to the prototype of a constructor function, we ensure that all objects created from that constructor will have access to those methods. This promotes code reusability and avoids duplicating methods across multiple objects.
2. Implementing inheritance: Prototype chains allow us to create inheritance relationships between objects. We can define a constructor function that sets the prototype of its instances to another object, effectively inheriting its properties and methods. This enables us to create more specialized objects while reusing common functionality.
3. Organizing related functionality: By grouping related methods on the prototype of a constructor function, we can keep our code organized and easier to maintain. This allows us to separate concerns and achieve a more modular codebase.
Here’s an example that demonstrates how prototype chains can be used to organize code:
function Animal(name) { this.name = name; } Animal.prototype.eat = function() { console.log(`${this.name} is eating.`); } function Dog(name, breed) { Animal.call(this, name); this.breed = breed; } Dog.prototype = Object.create(Animal.prototype); Dog.prototype.constructor = Dog; Dog.prototype.bark = function() { console.log(`${this.name} is barking.`); } const max = new Dog('Max', 'Labrador'); max.eat(); // Output: Max is eating. max.bark(); // Output: Max is barking.
In this example, we have an Animal
constructor that defines a common behavior for all animals. The Dog
constructor extends the Animal
constructor and adds a specific behavior for dogs. By setting the Dog.prototype
to an instance of Animal.prototype
, we establish a prototype chain that allows instances of Dog
to inherit methods from Animal
.
Prototypal Inheritance
Understanding the differences between prototypal and classical inheritance is crucial for fully grasping the concept of JavaScript prototype inheritance. While both approaches aim to enable object-oriented programming, they differ in their implementation and approach. In this chapter, we will explore the distinctions between prototypal and classical inheritance, their advantages, and their use cases.
Prototypal inheritance is the fundamental inheritance model in JavaScript. It allows objects to inherit properties and methods from other objects, also known as prototypes. In this model, objects are created based on existing objects, which act as blueprints or templates.
In JavaScript, every object has a prototype, except for the root object, Object.prototype
. When a property or method is accessed on an object, JavaScript first checks if the object itself has that property or method. If not found, it looks up the prototype chain until it reaches Object.prototype
. This process continues until the property or method is found or until the end of the prototype chain is reached.
To create an object with prototypal inheritance in JavaScript, we can use the Object.create()
method. This method allows us to specify the prototype object from which the new object will inherit properties and methods.
// Creating a prototype object const carPrototype = { startEngine() { console.log("Engine started"); }, stopEngine() { console.log("Engine stopped"); } }; // Creating a new car object with prototypal inheritance const myCar = Object.create(carPrototype); // Using inherited methods myCar.startEngine(); // Output: Engine started myCar.stopEngine(); // Output: Engine stopped
Prototypal inheritance provides flexibility and simplicity. It allows objects to inherit directly from other objects, promoting code reuse and reducing redundancy. However, it may require more manual handling of object creation and initialization.
Classical Inheritance
Classical inheritance is a concept familiar to developers coming from traditional object-oriented programming languages like Java or C++. It is based on the notion of classes, which are blueprints for creating objects. In this model, objects are instances of classes, and classes define their properties and methods.
JavaScript, being a prototype-based language, does not have built-in support for classical inheritance. However, it can be emulated using constructor functions and the new
keyword.
A constructor function is a regular JavaScript function that is used with the new
keyword to create instances of objects. Inside the constructor function, the this
keyword refers to the new object being created. We can define properties and methods on the this
object, which will be unique to each instance.
// Creating a constructor function function Car() { this.startEngine = function() { console.log("Engine started"); }; this.stopEngine = function() { console.log("Engine stopped"); }; } // Creating a new car object with classical inheritance const myCar = new Car(); // Using instance methods myCar.startEngine(); // Output: Engine started myCar.stopEngine(); // Output: Engine stopped
Classical inheritance provides a familiar and structured approach to object-oriented programming. It allows for the definition of classes and their hierarchies, making it easier to organize and maintain code. However, it can lead to complex class hierarchies and may not be as flexible or dynamic as prototypal inheritance.
Related Article: How To Sum An Array Of Numbers In Javascript
Creating Custom Inheritance Patterns
JavaScript provides us with the ability to create our own custom inheritance patterns by utilizing prototypes. This allows us to define reusable behaviors and characteristics for objects, making our code more modular and easier to maintain.
To create a custom inheritance pattern, we start by defining a constructor function. This function will serve as a blueprint for creating new objects that will inherit its properties and methods. We can then add properties and methods to the constructor’s prototype object, which will be shared by all instances created from this constructor.
Let’s take a look at an example:
function Animal(name) { this.name = name; } Animal.prototype.sayHello = function() { console.log(`Hello, my name is ${this.name}`); }; const animal = new Animal('Leo'); animal.sayHello(); // Output: Hello, my name is Leo
In the above example, we have defined a constructor function Animal
that takes a name
parameter. We have added a method sayHello
to the Animal.prototype
object, which logs a greeting message including the name of the animal. We then create a new instance of Animal
called animal
and invoke the sayHello
method on it, which outputs the greeting message to the console.
Inheriting from a Parent Constructor
To create inheritance between objects, we can utilize the Object.create()
method to set the prototype of a new object to an existing object. This allows the new object to inherit properties and methods from the existing object.
Let’s see an example:
function Cat(name, color) { Animal.call(this, name); this.color = color; } Cat.prototype = Object.create(Animal.prototype); Cat.prototype.constructor = Cat; Cat.prototype.sayMeow = function() { console.log('Meow!'); }; const cat = new Cat('Whiskers', 'gray'); cat.sayHello(); // Output: Hello, my name is Whiskers cat.sayMeow(); // Output: Meow!
In the above example, we have defined a constructor function Cat
that takes a name
and color
parameter. We call the Animal
constructor within the Cat
constructor using Animal.call(this, name)
to inherit the name
property from the Animal
constructor. We then set the prototype of Cat
to Object.create(Animal.prototype)
, which establishes the inheritance relationship between Cat
and Animal
. Finally, we add a new method sayMeow
to the Cat.prototype
object.
Now, any instance created from the Cat
constructor will inherit the properties and methods from both the Cat
and Animal
constructors. We can see this in action when invoking the sayHello
and sayMeow
methods on the cat
object.
Real World Examples of Prototype Inheritance in Action
In the previous chapter, we discussed the concept of prototype inheritance in JavaScript and how it allows objects to inherit properties and methods from other objects. In this chapter, we will explore some real-world examples that demonstrate the power and flexibility of prototype inheritance.
Example 1: Creating a Vehicle Class
Let’s start with a simple example of creating a Vehicle class using prototype inheritance. We’ll define a constructor function for the Vehicle class and add some properties and methods to its prototype.
function Vehicle(make, model, year) { this.make = make; this.model = model; this.year = year; } Vehicle.prototype.start = function() { console.log("Starting the vehicle..."); }; Vehicle.prototype.stop = function() { console.log("Stopping the vehicle..."); };
Now, we can create instances of the Vehicle class and call its methods:
const car = new Vehicle("Toyota", "Camry", 2020); car.start(); // Output: Starting the vehicle... car.stop(); // Output: Stopping the vehicle...
Example 2: Extending the Vehicle Class
Next, let’s extend the Vehicle class to create a Car class. The Car class will inherit the properties and methods from the Vehicle class but also have its own unique properties and methods.
function Car(make, model, year, color) { Vehicle.call(this, make, model, year); this.color = color; } Car.prototype = Object.create(Vehicle.prototype); Car.prototype.constructor = Car; Car.prototype.drive = function() { console.log("Driving the car..."); };
Now, we can create instances of the Car class and call its methods:
const myCar = new Car("Honda", "Civic", 2019, "blue"); myCar.start(); // Output: Starting the vehicle... myCar.stop(); // Output: Stopping the vehicle... myCar.drive(); // Output: Driving the car...
Example 3: Inheriting from Multiple Classes
In JavaScript, it is also possible to inherit from multiple classes using prototype inheritance. Let’s demonstrate this with an example of creating a HybridCar class that inherits from both the Vehicle and Car classes.
function HybridCar(make, model, year, color) { Car.call(this, make, model, year, color); } HybridCar.prototype = Object.create(Car.prototype); HybridCar.prototype.constructor = HybridCar; HybridCar.prototype.charge = function() { console.log("Charging the hybrid car..."); };
Now, we can create instances of the HybridCar class and call its methods:
const myHybridCar = new HybridCar("Toyota", "Prius", 2021, "green"); myHybridCar.start(); // Output: Starting the vehicle... myHybridCar.stop(); // Output: Stopping the vehicle... myHybridCar.drive(); // Output: Driving the car... myHybridCar.charge(); // Output: Charging the hybrid car...
These examples demonstrate how prototype inheritance allows us to create a hierarchy of classes and reuse code effectively. By inheriting properties and methods from other classes, we can build complex and flexible object structures in our JavaScript applications.
Related Article: How To Check If an Array Contains a Value In JavaScript
Composition
Composition is another technique that allows us to combine objects to create more complex objects. Unlike mixins, composition creates a new object that contains references to other objects, rather than copying their properties and methods.
Let’s illustrate composition with an example. Suppose we have two objects: obj1
and obj2
. We want to create a new object, composedObj
, that has access to the properties and methods of both obj1
and obj2
. Here’s an implementation using prototypes:
function compose(...sourceObjs) { return Object.assign({}, ...sourceObjs); } const obj1 = { prop1: 'Object 1 property', method1() { return 'Object 1 method'; } }; const obj2 = { prop2: 'Object 2 property', method2() { return 'Object 2 method'; } }; const composedObj = compose(obj1, obj2); console.log(composedObj.prop1); // Output: Object 1 property console.log(composedObj.method1()); // Output: Object 1 method console.log(composedObj.prop2); // Output: Object 2 property console.log(composedObj.method2()); // Output: Object 2 method
In the above example, we define a compose
function that takes any number of sourceObjs
. It uses Object.assign()
to create a new object and assign the properties and methods of the sourceObjs
to it. The result is a new object, composedObj
, that is composed of both obj1
and obj2
.
Common Pitfalls and Best Practices in Prototype Inheritance
When working with prototype inheritance in JavaScript, there are some common pitfalls that developers may encounter. Understanding these pitfalls and following best practices can help ensure the proper use and implementation of prototype inheritance.
Avoiding Prototype Pollution
Prototype pollution occurs when properties are added to the prototype of an object, and those properties are then accessible to all instances of that object. This can lead to unexpected behavior and bugs.
To avoid prototype pollution, we can use techniques such as Object.freeze() or Object.create(null) to create clean prototypes without any unwanted properties.
// Create a clean prototype object using Object.create(null) const cleanPrototype = Object.create(null); // Add properties to the clean prototype cleanPrototype.name = "John"; cleanPrototype.age = 30; // Freeze the clean prototype to prevent further modifications Object.freeze(cleanPrototype); // Create a new object with the clean prototype const person = Object.create(cleanPrototype); console.log(person.name); // Output: John console.log(person.age); // Output: 30 // Trying to add a new property to the person object will throw an error person.city = "New York"; // TypeError: Cannot add property city, object is not extensible
In the code snippet above, we create a clean prototype object cleanPrototype
using Object.create(null)
. We add properties to the clean prototype and then freeze it using Object.freeze()
to prevent further modifications. Finally, we create a new object person
with the clean prototype. Any attempt to add new properties to the person
object will throw an error.
By following these techniques, we can avoid prototype pollution and ensure that our objects have clean prototypes without any unwanted properties.
These advanced techniques for working with prototype inheritance provide us with more flexibility and control over our object-oriented JavaScript code. Whether it’s using mixins to combine functionality, creating objects with specific prototypes using Object.create()
, manipulating the prototype chain, or avoiding prototype pollution, understanding these concepts helps us write cleaner and more maintainable code.
Related Article: How to Use the JavaScript Filter Array Method
Modifying the Prototype Object Directly
One common mistake is directly modifying the prototype object of a constructor function. This can lead to unexpected behavior and can cause issues when multiple objects inherit from the same prototype. Instead, it is recommended to modify the prototype using the constructor function’s prototype property. Here’s an example:
function Person(name) { this.name = name; } Person.prototype.greet = function() { console.log(`Hello, my name is ${this.name}.`); }; // Incorrect way to modify the prototype Person.prototype.age = 30; // Correct way to modify the prototype Person.prototype = { age: 30, greet: function() { console.log(`Hello, my name is ${this.name} and I am ${this.age} years old.`); } };
By assigning a new object to the prototype property, we avoid modifying the original prototype object and ensure that all instances of the constructor function have access to the updated properties and methods.
Shadowing Properties
Another pitfall is unintentionally shadowing properties in the prototype chain. Shadowing occurs when a property with the same name is defined in a child object, effectively hiding the property from higher levels of the prototype chain. To avoid this, it is important to choose unique property names or use a naming convention that minimizes the chances of shadowing. Here’s an example:
function Animal(name) { this.name = name; } Animal.prototype.speak = function() { console.log(`The ${this.name} makes a sound.`); }; function Dog(name) { Animal.call(this, name); } Dog.prototype = Object.create(Animal.prototype); Dog.prototype.constructor = Dog; // Shadowing the speak method in Dog's prototype Dog.prototype.speak = function() { console.log(`${this.name} barks.`); };
In this example, the speak
method in the Dog
prototype shadows the speak
method in the Animal
prototype. To prevent shadowing, it is recommended to choose a different name for the method in the child object.
Overusing Inheritance
While prototype inheritance can be a powerful tool, it is important to avoid overusing it. In some cases, composition or other design patterns may be more appropriate. Overusing inheritance can lead to tightly coupled code and make it difficult to maintain and modify the application in the future. Consider the specific needs of your application and choose the most appropriate approach.
Related Article: How To Loop Through An Array In Javascript
Using Object.create() for Inheritance
The Object.create()
method can be used to create a new object with a specified prototype. However, it is important to note that this method only creates a shallow copy of the prototype object. Any changes made to the prototype object will affect all instances that inherit from it. If you need to create a separate copy of the prototype, it is recommended to use a constructor function or a class. Here’s an example:
const personPrototype = { greet: function() { console.log(`Hello, my name is ${this.name}.`); } }; const person1 = Object.create(personPrototype); person1.name = 'Alice'; const person2 = Object.create(personPrototype); person2.name = 'Bob'; person1.greet(); // Hello, my name is Alice. person2.greet(); // Hello, my name is Bob. personPrototype.age = 30; person1.greet(); // Hello, my name is Alice. person2.greet(); // Hello, my name is Bob.
In this example, when the age
property is added to the personPrototype
, it affects both person1
and person2
instances. To avoid this, consider using constructor functions or classes for creating separate instances with their own properties.
By understanding these common pitfalls and following best practices, you can effectively utilize prototype inheritance in JavaScript and avoid potential issues in your code.