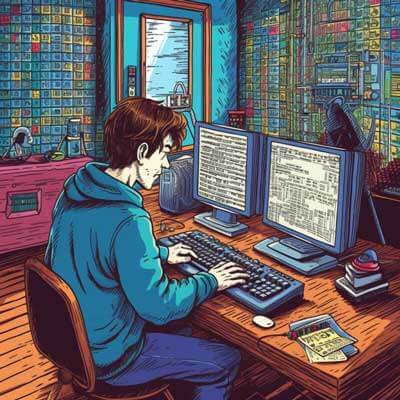
Table of Contents
What is responsive design and why is it important for images?
Responsive design is an approach to web design that aims to create websites that adapt and respond to different screen sizes and devices. This is important for images because it allows them to be displayed in the most optimal way across a wide range of devices, ensuring a consistent and visually appealing user experience.
Related Article: How To Check For An Empty String In Javascript
How do CSS media queries work?
CSS media queries are a useful feature that allows you to apply different styles based on the characteristics of the device or screen that the webpage is being viewed on. Media queries use the @media
rule in CSS to specify the conditions under which certain styles should be applied. Here's an example:
@media screen and (max-width: 600px) { /* Styles for screens with a maximum width of 600px */ }
In this example, the styles within the media query will only be applied when the screen width is 600 pixels or less.
What is the picture element in HTML5?
The <picture>
element is a new HTML5 element that allows you to specify multiple sources for an image, along with different sizes and resolutions, to ensure that the most appropriate image is displayed based on the user's device and screen size. Here's an example:
<picture> <source srcset="large-240-200.jpg" media="(orientation: portrait)" /> <img src="large-298-332.jpg" alt="" /> </picture>
In this example, the browser will choose the source image based on the media query that matches the user's device, ensuring that the image is displayed at the appropriate size.
How does the srcset attribute help in making images responsive?
The srcset
attribute is an attribute that can be added to the <img>
element to specify multiple sources for the image, along with different sizes and resolutions. The browser will then choose the most appropriate source based on the user's device and screen size. Here's an example:
<img src="default.jpg" alt="A responsive image">
In this example, the browser will choose the source image that best matches the width of the image element, ensuring that the image is displayed at the appropriate size.
Related Article: How To Check If an Array Contains a Value In JavaScript
What are some best practices for responsive image design?
- Use the <picture>
element or the srcset
attribute to provide multiple sources for the image.
- Specify different image sizes and resolutions to ensure that the most appropriate image is displayed based on the user's device and screen size.
- Use CSS media queries to apply specific styles to the image based on the characteristics of the device or screen.
- Optimize your images for the web by compressing them and using modern image formats such as WebP or AVIF.
How can CSS flexbox be used to create responsive layouts?
CSS flexbox is a useful layout model that allows you to create flexible and responsive layouts. It provides a simple way to align and distribute space among items in a container. Here's an example of how flexbox can be used to create a responsive layout:
<div class="container"> <div class="item">Item 1</div> <div class="item">Item 2</div> <div class="item">Item 3</div> </div>
.container { display: flex; flex-wrap: wrap; } .item { flex: 1 0 200px; }
In this example, the .container
element is set to display: flex
to create a flex container. The .item
elements are set to flex: 1 0 200px
to specify that they should grow and shrink as necessary, with a minimum width of 200 pixels.
What is CSS grid and how can it be used for responsive design?
CSS grid is a layout model that allows you to create complex grid-based layouts with ease. It provides a two-dimensional grid system that allows you to define both rows and columns. Here's an example of how CSS grid can be used for responsive design:
<div class="container"> <div class="item">Item 1</div> <div class="item">Item 2</div> <div class="item">Item 3</div> </div>
.container { display: grid; grid-template-columns: repeat(auto-fit, minmax(200px, 1fr)); grid-gap: 10px; } .item { background-color: #ddd; padding: 10px; }
In this example, the .container
element is set to display: grid
to create a grid container. The grid-template-columns
property is set to repeat(auto-fit, minmax(200px, 1fr))
to create a flexible grid with a minimum column width of 200 pixels. The grid-gap
property is set to 10px
to add some spacing between the grid items.
What are breakpoints in responsive design?
Breakpoints are specific points in a responsive design where the layout and styling of a webpage change to accommodate different screen sizes or devices. They are typically defined using CSS media queries and are used to ensure that a webpage looks and functions well across a range of devices. Here's an example:
@media screen and (max-width: 600px) { /* Styles for screens with a maximum width of 600px */ } @media screen and (min-width: 601px) and (max-width: 1200px) { /* Styles for screens with a minimum width of 601px and a maximum width of 1200px */ } @media screen and (min-width: 1201px) { /* Styles for screens with a minimum width of 1201px */ }
In this example, three breakpoints are defined using CSS media queries: one for screens with a maximum width of 600px, one for screens with a minimum width of 601px and a maximum width of 1200px, and one for screens with a minimum width of 1201px.
Related Article: How To Add A Class To A Given Element In Javascript
How does the viewport meta tag affect image responsiveness?
The viewport meta tag is used to control the layout and behavior of a webpage on mobile devices. It allows you to specify the initial scale, width, and height of the viewport. By setting the viewport width to the device width, you can ensure that the webpage and its images are displayed at the appropriate size. Here's an example:
<meta name="viewport" content="width=device-width, initial-scale=1" />
In this example, the viewport width is set to the device width using width=device-width
, ensuring that the webpage and its images are displayed at the appropriate size.
What are fluid images and how can they be implemented?
Fluid images are images that scale and resize proportionally to fit their container, ensuring that they always take up the appropriate amount of space. They can be implemented using CSS by setting the max-width
property of the image to 100%
and the height
property to auto
. Here's an example:
<img src="image.jpg" alt="A fluid image" style="max-width: 100%;height: auto">
In this example, the max-width
property is set to 100%
, allowing the image to scale down to fit its container while maintaining its aspect ratio. The height
property is set to auto
, ensuring that the image's height adjusts proportionally to its width.
Additional Resources
- Next.js Image Component Examples