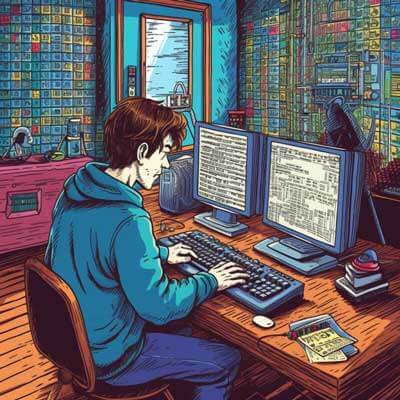
Table of Contents
Getting Started with Promises
Promises are a powerful tool for managing asynchronous JavaScript. They provide a clean and intuitive syntax for handling asynchronous operations, making code more readable and maintainable. In this chapter, we will explore the basics of working with Promises.
To get started with Promises, we first need to understand their three states: pending, fulfilled, and rejected. When a Promise is created, it is in the pending state. It can then transition to either the fulfilled state, meaning the operation was successful, or the rejected state, meaning the operation encountered an error.
Creating a Promise is straightforward. We use the Promise
constructor and provide a callback function with two parameters: resolve
and reject
. These parameters are functions that we can call to either fulfill or reject the Promise.
Let's take a look at a simple example:
const promise = new Promise((resolve, reject) => { // Simulating an asynchronous operation setTimeout(() => { const randomNumber = Math.random(); if (randomNumber >= 0.5) { resolve(randomNumber); } else { reject(new Error('Random number is less than 0.5')); } }, 1000); });
In the above example, we create a Promise that simulates an asynchronous operation using setTimeout
. If the randomly generated number is greater than or equal to 0.5, we call the resolve
function with the number as the parameter. Otherwise, we call the reject
function with an Error
object.
Once a Promise is created, we can use its then
method to handle the fulfilled state and its catch
method to handle the rejected state. The then
method takes a callback function as its parameter, which will be executed when the Promise is fulfilled. The catch
method also takes a callback function as its parameter, which will be executed when the Promise is rejected.
Continuing with our example:
promise .then((result) => { console.log('Promise fulfilled:', result); }) .catch((error) => { console.error('Promise rejected:', error); });
In the above code, we call the then
method on our promise
and pass a callback function that logs the fulfilled result to the console. If the Promise is rejected, the catch
method is called with a callback function that logs the error to the console.
Promises can also be chained together using the then
method. Each then
callback can return a new Promise, allowing for sequential execution of asynchronous operations.
const fetchUser = () => { return new Promise((resolve, reject) => { setTimeout(() => { const user = { name: 'John Doe', age: 30 }; resolve(user); }, 1000); }); }; const fetchPosts = (user) => { return new Promise((resolve, reject) => { setTimeout(() => { const posts = ['Post 1', 'Post 2', 'Post 3']; resolve({ user, posts }); }, 1000); }); }; fetchUser() .then((user) => fetchPosts(user)) .then((data) => { console.log('User:', data.user); console.log('Posts:', data.posts); }) .catch((error) => { console.error('Error:', error); });
In this example, we define two functions, fetchUser
and fetchPosts
, each returning a Promise that resolves with some data. We can then chain these Promises together, passing the result of the first Promise to the second Promise. Finally, we log the user and posts data to the console.
Understanding Promises and their syntax is fundamental for working with asynchronous JavaScript.
Related Article: How to Compare Arrays in Javascript
Understanding Callback Functions
In JavaScript, a callback function is a function that is passed as an argument to another function and is executed after a specific event or action occurs. Callback functions are commonly used in asynchronous programming to handle the results of an asynchronous operation.
Callbacks are an essential part of understanding how to write asynchronous code in JavaScript. They allow us to execute code after an asynchronous operation has completed, rather than blocking further execution until the operation is finished.
Here's a simple example of a callback function:
function fetchData(callback) { // Simulate an asynchronous operation setTimeout(function () { const data = "Some data"; callback(data); }, 1000); } function processData(data) { console.log("Processing data: " + data); } fetchData(processData);
In the code snippet above, we have two functions: fetchData
and processData
. The fetchData
function simulates an asynchronous operation using setTimeout
and then calls the callback
function with the fetched data after a delay of 1 second. The processData
function logs the processed data to the console.
By passing the processData
function as an argument to the fetchData
function, we ensure that processData
is executed only after the data has been fetched. This allows us to avoid blocking the execution of other code while waiting for the data to be retrieved.
Callbacks can also be used to handle errors in asynchronous operations. Here's an example that demonstrates error handling with callbacks:
function fetchData(callback, errorCallback) { // Simulate an asynchronous operation setTimeout(function () { const error = true; if (error) { errorCallback("An error occurred"); } else { const data = "Some data"; callback(data); } }, 1000); } function processData(data) { console.log("Processing data: " + data); } function handleError(error) { console.log("Error: " + error); } fetchData(processData, handleError);
In this example, the fetchData
function now takes two callback functions as arguments: callback
and errorCallback
. If an error occurs during the asynchronous operation, the errorCallback
function is called with the error message. Otherwise, the callback
function is called with the retrieved data.
By providing separate error handling callbacks, we can handle errors in a more controlled manner and take appropriate action based on the error.
Callbacks are a powerful and widely used concept in JavaScript for handling asynchronous operations. They allow us to write code that executes in response to events or actions, without blocking the execution of other code. However, as more and more asynchronous operations are nested, callback hell can occur, making code difficult to read and maintain.
Creating and Resolving Promises
ES6 Promises are objects that represent the eventual completion or failure of an asynchronous operation. They allow you to write cleaner and more readable asynchronous code by avoiding callback hell.
To create a new Promise, you can use the Promise
constructor. It takes a single argument, a function that is called the executor function. This function takes two parameters, resolve
and reject
, which are both functions themselves.
The resolve
function is used to fulfill the promise with a value. If you call resolve
with a value, the promise is considered fulfilled, and any callbacks attached to the promise using the then
method will be called with that value.
The reject
function is used to reject the promise with a reason. If you call reject
with a reason, the promise is considered rejected, and any callbacks attached to the promise using the catch
method will be called with that reason.
Here's an example of creating and resolving a promise:
const myPromise = new Promise((resolve, reject) => { // Simulating an asynchronous operation setTimeout(() => { const randomNumber = Math.random(); if (randomNumber { console.log('Promise fulfilled with value:', value); }).catch((reason) => { console.error('Promise rejected with reason:', reason); });
In this example, we create a new promise that simulates an asynchronous operation using setTimeout
. Inside the executor function, we generate a random number and use it to either resolve or reject the promise. If the random number is less than 0.5, we call resolve
with the number. Otherwise, we call reject
with an error object.
After creating the promise, we attach callbacks to it using the then
and catch
methods. The then
method is called when the promise is fulfilled, and the catch
method is called when the promise is rejected. In each callback, we log the value or reason respectively.
By using promises, we can handle both successful and failed asynchronous operations in a more structured and readable way. Promises provide a way to chain asynchronous operations together and handle errors in a central place using the catch
method.
Handling Promise Rejections
In JavaScript, promises are a powerful tool for handling asynchronous operations. However, just like any other code, there might be cases where things go wrong and a promise ends up being rejected. In this chapter, we will explore how to handle promise rejections effectively.
When a promise is rejected, it means that there was an error or an exception occurred during the execution of the asynchronous operation. To handle these rejections, we can use the .catch()
method provided by promises. This method allows us to specify a callback function that will be called when the promise is rejected.
Here's an example that demonstrates how to handle promise rejections using the .catch()
method:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Handle the data }) .catch(error => { // Handle the error });
In the code snippet above, we are making a network request using the fetch()
function. We then chain .then()
methods to handle the response and the data. Finally, we use the .catch()
method to handle any errors that might occur during the execution.
It's important to note that the .catch()
method will catch any rejections that occur in the promise chain before it. If an error occurs in any of the preceding .then()
methods, the error will be caught by the .catch()
method.
Additionally, the .catch()
method returns a new promise, which allows us to chain multiple .catch()
methods if needed. This can be useful when we want to handle different types of errors separately.
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Handle the data }) .catch(error => { // Handle network errors }) .catch(error => { // Handle JSON parsing errors });
In the code snippet above, we have added another .catch()
method to handle JSON parsing errors separately from network errors.
In some cases, we might want to handle a specific type of error differently. To achieve this, we can throw a specific error object in the promise chain and catch it with the .catch()
method. Here's an example:
fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => { // Handle the data }) .catch(error => { // Handle network errors });
In the code snippet above, we throw a custom Error
object if the network response is not ok. This allows us to catch and handle the specific error in the .catch()
method.
By effectively handling promise rejections, we can gracefully handle errors and exceptions in our asynchronous code. This helps to ensure that our applications are robust and provide a better user experience.
Related Article: Resolving Declaration File Issue for Vue-Table-Component
Error Handling
Error handling is an essential part of working with promises. When chaining promises, it is important to handle errors at each step of the chain. By using the catch()
method, we can catch and handle any errors that occur in the chain.
fetchUserData() .then(user => fetchUserPosts(user.id)) .then(posts => { // Handle the fetched posts console.log(posts); }) .catch(error => { // Handle any errors console.error(error); });
In the example above, if an error occurs in either the fetchUserData()
or fetchUserPosts()
functions, the error will be caught by the catch()
method and the appropriate error handling code will be executed.
Working with Multiple Promises
When working with asynchronous JavaScript, it is common to encounter scenarios where you need to work with multiple promises simultaneously. This is where the true power of ES6 promises shines through. With promises, you can easily manage multiple asynchronous operations and handle the results once all promises have resolved.
One common use case for working with multiple promises is when you need to fetch data from multiple APIs and combine the results. Let's say you have two APIs, one for fetching user details and another for fetching user posts. You want to fetch the user details and posts simultaneously, and once both promises have resolved, you want to display the data on your webpage.
Here's how you can achieve this using ES6 promises:
const userDetailsPromise = fetch('https://api.example.com/user-details'); const userPostsPromise = fetch('https://api.example.com/user-posts'); Promise.all([userDetailsPromise, userPostsPromise]) .then(([userDetailsResponse, userPostsResponse]) => { // Handle the responses here const userDetails = userDetailsResponse.json(); const userPosts = userPostsResponse.json(); // Display the data on the webpage console.log('User Details:', userDetails); console.log('User Posts:', userPosts); }) .catch(error => { // Handle any errors that occurred during the fetch requests console.error('Error:', error); });
In the code snippet above, we create two promises using the fetch
function to make asynchronous API calls. We pass these promises to the Promise.all
method, which takes an array of promises and returns a new promise that resolves when all the promises in the array have resolved.
Once the promises have resolved, the then
method is called, and we can access the responses from the API calls. In this example, we call the json
method on the response objects to extract the JSON data.
Finally, we display the user details and posts on the webpage. If any errors occur during the fetch requests, the catch
method is called, allowing us to handle and display the error message.
Working with multiple promises can also be useful when you need to perform multiple asynchronous operations in a specific order. You can chain promises together using the then
method to ensure that each operation is executed in the desired sequence.
Here's an example that demonstrates chaining promises:
const fetchData = () => { return fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Process the data return data.map(item => item.name); }) .then(names => { // Display the processed data console.log('Names:', names); }) .catch(error => { // Handle any errors that occurred during the fetch request or data processing console.error('Error:', error); }); }; fetchData();
In this example, we define a function fetchData
that fetches data from an API and processes it. We chain multiple then
methods to ensure that the operations are executed in the specified order. If any errors occur during the fetch request or data processing, the catch
method is called to handle the error.
Working with multiple promises allows you to handle complex asynchronous scenarios in a more organized and efficient manner. Whether you need to fetch data from multiple APIs simultaneously or execute multiple operations in a specific sequence, ES6 promises provide a clean and powerful solution.
Using Promise.race
The Promise.race
method is a powerful tool in JavaScript that allows you to handle multiple promises concurrently and get the result of the first promise that resolves or rejects. This can be especially useful when you need to perform a task that depends on the result of multiple asynchronous operations, but you only care about the fastest one.
The syntax for Promise.race
is straightforward. You pass an array of promises as an argument to the method, and it returns a new promise that settles with the value or reason of the first promise in the array to settle. Let's take a look at an example:
const promise1 = new Promise((resolve, reject) => { setTimeout(() => { resolve('Promise 1 resolved'); }, 2000); }); const promise2 = new Promise((resolve, reject) => { setTimeout(() => { reject('Promise 2 rejected'); }, 1000); }); Promise.race([promise1, promise2]) .then(result => { console.log(result); }) .catch(error => { console.error(error); });
In this example, we create two promises: promise1
and promise2
. promise1
resolves after 2 seconds, while promise2
rejects after 1 second. We pass both promises to Promise.race
and handle the result using then
and catch
methods.
Since promise2
rejects before promise1
resolves, the catch
block will be executed, and the error message "Promise 2 rejected" will be logged to the console. If the order of promises was reversed, the then
block would be executed instead with the resolved value of "Promise 1 resolved".
Promise.race
can be particularly useful in scenarios where you want to enforce a timeout for an asynchronous operation. For example, you can create a new promise that resolves or rejects after a specific duration, and combine it with another promise that performs an asynchronous task. Whichever promise settles first will determine the final outcome.
const timeoutPromise = new Promise((resolve, reject) => { setTimeout(() => { reject('Timeout'); }, 5000); }); const fetchDataPromise = fetch('https://api.example.com/data'); Promise.race([timeoutPromise, fetchDataPromise]) .then(response => { console.log('Data received:', response); }) .catch(error => { console.error('Error:', error); });
In this example, we create a timeoutPromise
that rejects after 5 seconds, simulating a timeout. We also have a fetchDataPromise
that performs an HTTP request to retrieve data from an API. Whichever promise settles first will determine if we receive the data or encounter a timeout.
Promise.race
is a versatile method that allows you to handle multiple promises concurrently and respond to the first one that resolves or rejects. It can be a valuable tool for managing asynchronous operations in JavaScript, providing more control and flexibility in your code.
Async/Await: A Syntactic Sugar for Promises
Working with Promises can still be a bit verbose and may require nesting multiple .then()
methods to handle different scenarios. To address this issue, ES8 (ES2017) introduced a new feature called Async/Await, which is essentially a syntactic sugar for working with Promises.
Async/Await allows you to write asynchronous code that looks and behaves more like synchronous code, making it easier to read and understand. It is built on top of Promises and provides a simpler way to write asynchronous code without the need for explicit Promises and .then()
chains.
To understand how Async/Await works, let's first take a look at an example using Promises. Suppose we have a function that fetches data from an API:
function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => { resolve('Data received from API'); }, 2000); }); } fetchData() .then(data => { console.log(data); }) .catch(error => { console.error(error); });
In this example, the fetchData()
function returns a Promise that resolves after a 2-second delay. We then use the .then()
method to handle the resolved value and the .catch()
method to handle any errors.
Now, let's rewrite the code using Async/Await:
async function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => { resolve('Data received from API'); }, 2000); }); } async function fetchDataWrapper() { try { const data = await fetchData(); console.log(data); } catch (error) { console.error(error); } } fetchDataWrapper();
In this example, we added the async
keyword before the fetchData()
function declaration, indicating that it is an asynchronous function. We also added the async
keyword before the fetchDataWrapper()
function declaration.
Inside the fetchDataWrapper()
function, we use the await
keyword to pause the execution until the fetchData()
Promise is resolved or rejected. The await
keyword can only be used inside an async
function.
Using Async/Await, the code becomes more linear and easier to follow. It eliminates the need for chaining .then()
methods and allows us to handle errors using a simple try-catch
block.
It's important to note that Async/Await works by wrapping Promises, so any function that returns a Promise can be used with Async/Await.
Async/Await also allows us to handle multiple asynchronous operations in parallel. We can use await
with multiple Promises, and the execution will pause until all the Promises have resolved or any of them is rejected.
async function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => { resolve('Data received from API'); }, 2000); }); } async function fetchUser() { return new Promise((resolve, reject) => { setTimeout(() => { resolve('User fetched from API'); }, 1000); }); } async function fetchDataAndUser() { try { const [data, user] = await Promise.all([fetchData(), fetchUser()]); console.log(data); console.log(user); } catch (error) { console.error(error); } } fetchDataAndUser();
In this example, we have two functions, fetchData()
and fetchUser()
, each returning a Promise. Inside the fetchDataAndUser()
function, we use Promise.all()
to wait for both Promises to resolve. The await
keyword is used to pause the execution until both Promises have resolved.
Async/Await is widely supported in modern browsers and can be used in Node.js as well. However, if you need to support older browsers or environments that don't have native support for Async/Await, you can use transpilers like Babel to convert the code to ES5 syntax.
Overall, Async/Await is a powerful feature that simplifies asynchronous programming in JavaScript. It provides a more intuitive and readable way to write asynchronous code, making it easier to handle Promises and perform multiple asynchronous operations.
Related Article: How to Check If a Value is an Object in JavaScript
Error Handling in Async/Await
Asynchronous programming in JavaScript can sometimes lead to unexpected errors. When using async/await, it's important to handle these errors effectively to ensure your code behaves as expected. In this chapter, we will explore how to handle errors in async/await functions.
When an error occurs in an async function, it is thrown just like any other JavaScript error. To handle these errors, we can use try/catch blocks. By wrapping the await keyword inside a try block, we can catch any errors that occur during the asynchronous operation.
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('An error occurred:', error); } }
In the example above, we fetch data from an API using the fetch function. If an error occurs during the API call or while parsing the response, the catch block will be executed and the error will be logged to the console.
It's worth noting that when an error is caught in an async function, the function will return a rejected promise. This allows us to handle the error using the same mechanisms we would use with regular promises.
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return data; } catch (error) { throw new Error('Failed to fetch data'); } } fetchData() .then(data => console.log('Data:', data)) .catch(error => console.error('An error occurred:', error));
In the example above, if the fetchData function encounters an error, it throws a new Error object. This will cause the returned promise to be rejected. We can then use the .catch() method to handle the error.
Async functions also support the use of the finally block, which will always be executed, regardless of whether an error occurred or not. This can be useful for performing cleanup tasks, such as closing database connections or releasing resources.
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); return data; } catch (error) { throw new Error('Failed to fetch data'); } finally { console.log('Cleanup tasks here'); } }
In the example above, the finally block will be executed regardless of whether an error occurred during the API call or while parsing the response. This allows us to perform any necessary cleanup tasks.
In summary, error handling in async/await functions is similar to error handling in regular JavaScript code. By using try/catch blocks, we can effectively handle errors that occur during asynchronous operations. Additionally, the use of the finally block allows us to perform cleanup tasks.
Working with Promises in Real World Examples
Promises are a powerful tool in JavaScript for handling asynchronous operations. In this chapter, we will explore some real-world examples of how to work with promises effectively.
Example 1: Fetching Data from an API
One common use case for promises is fetching data from an API. Let's say we want to retrieve a list of users from a remote server using the fetch
function. Here's how we can accomplish this using promises:
fetch('https://api.example.com/users') .then(response => response.json()) .then(data => { // Process the retrieved data console.log(data); }) .catch(error => { // Handle any errors that occurred during the request console.error(error); });
In this example, the fetch
function returns a promise that resolves to the server's response. We can then use the then
method to parse the response as JSON and process the data. If any errors occur during the request, the catch
method will handle them.
Example 2: Chaining Promises
Promises can also be chained together to perform a series of asynchronous operations. Let's consider a scenario where we need to fetch user data and then fetch additional details for each user. Here's how we can achieve this using promise chaining:
fetch('https://api.example.com/users') .then(response => response.json()) .then(users => { // Fetch additional details for each user const promises = users.map(user => { return fetch(`https://api.example.com/users/${user.id}`); }); return Promise.all(promises); }) .then(responses => { // Process the additional details const details = responses.map(response => response.json()); console.log(details); }) .catch(error => { // Handle any errors that occurred during the requests console.error(error); });
In this example, we first fetch a list of users and then create an array of promises to fetch additional details for each user. We use the Promise.all
method to wait for all the promises to resolve before processing the data. Finally, we can access the additional details and perform any necessary operations.
Related Article: How to Add a Key Value Pair to a Javascript Object
Example 3: Error Handling with Promises
Promises provide a convenient way to handle errors in asynchronous code. Let's say we have a function that performs an asynchronous operation and may encounter an error. Here's how we can handle errors using promises:
function performAsyncOperation() { return new Promise((resolve, reject) => { // Simulate an asynchronous operation setTimeout(() => { const error = new Error('Something went wrong'); reject(error); }, 2000); }); } performAsyncOperation() .then(() => { // Operation succeeded console.log('Operation completed successfully'); }) .catch(error => { // Handle the error console.error(error.message); });
In this example, the performAsyncOperation
function returns a promise that either resolves or rejects based on the outcome of the asynchronous operation. We can then use the then
method to handle the successful case and the catch
method to handle any errors that occur.
These real-world examples demonstrate the flexibility and power of promises in handling asynchronous JavaScript. By understanding how to work with promises effectively, you can write more robust and maintainable code.
Promisifying Callback-based Functions
Promises are a powerful tool in JavaScript for handling asynchronous operations. However, many existing libraries and APIs still use the traditional callback pattern. To take advantage of promises, we can convert these callback-based functions into functions that return promises. This process is known as "promisifying" callback-based functions.
Promisifying a callback-based function involves wrapping it in a new function that returns a promise. This new function will execute the original function and resolve or reject the promise based on the callback's result.
Let's consider an example where we have a callback-based function called getUserData
that fetches user data from an API and passes the result to a callback function:
function getUserData(userId, callback) { // Simulating an asynchronous API call setTimeout(() => { const userData = { id: userId, name: "John Doe" }; callback(null, userData); }, 1000); }
To promisify this function, we can create a new function called getUserDataAsync
that returns a promise instead of accepting a callback:
function getUserDataAsync(userId) { return new Promise((resolve, reject) => { getUserData(userId, (error, data) => { if (error) { reject(error); } else { resolve(data); } }); }); }
In the above code, we create a new promise and pass a callback function to getUserData
. Inside the callback, we check if an error occurred. If an error is present, we reject the promise with the error. Otherwise, we resolve the promise with the data retrieved from the API.
Now we can use the getUserDataAsync
function with promises instead of callbacks:
getUserDataAsync(123) .then((data) => { console.log("User data:", data); }) .catch((error) => { console.error("Error:", error); });
By promisifying the getUserData
function, we can now use promises to handle the asynchronous result. The .then
method is used to handle the resolved promise, while the .catch
method is used to handle any errors that occurred during the asynchronous operation.
Promisifying callback-based functions allows us to take advantage of promises' syntax and features, such as chaining and error handling. It simplifies the code and makes it more readable by avoiding the "callback hell" problem.
In some cases, you may find libraries or utility functions that provide built-in methods to promisify callback-based functions. These can save you the effort of manually promisifying each function.
References:
Caching with Promises
One powerful use case for Promises in JavaScript is caching data. Caching allows us to store the results of expensive operations so that we can retrieve them quickly in the future without having to repeat the operation. This can be particularly useful when working with asynchronous operations, where the result may not be immediately available.
With Promises, we can easily implement a caching mechanism by creating a cache object that stores the resolved Promise for each unique request. Here's an example:
const cache = {}; function fetchData(url) { if (cache[url]) { return cache[url]; // Return the cached Promise if it exists } const promise = new Promise((resolve, reject) => { // Perform the expensive operation, such as making an API request // Once the operation is complete, resolve the Promise with the result // or reject it with an error if something went wrong // ... // Simulating an API request with a timeout setTimeout(() => { const data = { /* ... */ }; cache[url] = Promise.resolve(data); // Cache the resolved Promise resolve(data); }, 1000); }); cache[url] = promise; // Cache the Promise return promise; }
In this example, we define a cache
object to store the resolved Promises. When the fetchData
function is called with a URL, it first checks if the Promise for that URL already exists in the cache. If it does, it returns the cached Promise. Otherwise, it creates a new Promise and performs the expensive operation, such as making an API request.
Once the operation is complete, the Promise is resolved with the result, and the resolved Promise is cached for future use. Subsequent calls to fetchData
with the same URL will return the cached Promise without repeating the expensive operation.
Here's an example usage of the fetchData
function:
fetchData('https://api.example.com/data') .then((data) => { // Handle the resolved data // ... }) .catch((error) => { // Handle any errors that occurred during the operation // ... });
In this example, we call the fetchData
function with a URL and handle the resolved data in the .then
callback. If an error occurs during the operation, it is caught in the .catch
callback.
Caching with Promises can help improve the performance of your application by reducing the need to repeat expensive operations. It allows you to store and retrieve data quickly, making your application more responsive and efficient.
Remember to be mindful of caching stale data. If the data being fetched can change frequently, you may need to implement a strategy to invalidate or update the cache when necessary.
Advanced Promise Techniques
ES6 Promises provide powerful tools for handling asynchronous JavaScript code. In this chapter, we will explore some advanced techniques that can be used with Promises to handle complex scenarios.
Related Article: How to Reverse an Array in Javascript Without Libraries
Chaining Promises
One of the key benefits of using Promises is the ability to chain multiple asynchronous operations together. This allows us to avoid callback hell and write more readable code.
To chain Promises, we use the then
method, which allows us to specify a callback function that will be executed when the Promise is resolved. This callback function can also return a Promise, which will be used as the input for the next then
method in the chain.
Here's an example that demonstrates how to chain Promises:
const fetchData = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve('Data fetched successfully!'); }, 2000); }); }; fetchData() .then((data) => { console.log(data); return new Promise((resolve, reject) => { setTimeout(() => { resolve('Data processed successfully!'); }, 2000); }); }) .then((data) => { console.log(data); }) .catch((error) => { console.error(error); });
In the above example, we define a fetchData
function that returns a Promise that resolves after a 2-second delay. We then chain two then
methods to handle the fetched data and the processed data. If any error occurs during the Promise chain, the catch
method will be called.
Promise.all
The Promise.all
method allows us to execute multiple Promises in parallel and wait for all of them to resolve before continuing. It takes an array of Promises as input and returns a new Promise that resolves with an array of the resolved values.
Here's an example that demonstrates how to use Promise.all
:
const fetchUserData = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve('User data fetched successfully!'); }, 2000); }); }; const fetchProfileData = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve('Profile data fetched successfully!'); }, 3000); }); }; Promise.all([fetchUserData(), fetchProfileData()]) .then((data) => { console.log(data); }) .catch((error) => { console.error(error); });
In the above example, we define two functions fetchUserData
and fetchProfileData
, which return Promises that resolve after a certain delay. We use Promise.all
to execute both Promises in parallel and wait for both of them to resolve. The resolved data from both Promises is then logged to the console.
Promise.race
The Promise.race
method allows us to execute multiple Promises in parallel and wait for the first one to resolve or reject. It takes an array of Promises as input and returns a new Promise that resolves or rejects with the value or error of the first resolved or rejected Promise.
Here's an example that demonstrates how to use Promise.race
:
const fetchUserData = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve('User data fetched successfully!'); }, 2000); }); }; const fetchProfileData = () => { return new Promise((resolve, reject) => { setTimeout(() => { reject('Profile data fetch failed!'); }, 3000); }); }; Promise.race([fetchUserData(), fetchProfileData()]) .then((data) => { console.log(data); }) .catch((error) => { console.error(error); });
In the above example, we define two functions fetchUserData
and fetchProfileData
, which return Promises that resolve or reject after a certain delay. We use Promise.race
to execute both Promises in parallel and wait for the first one to resolve or reject. The resolved or rejected value of the first Promise is then logged to the console.
Async/Await
ES8 introduced the async/await
syntax, which provides a more concise and synchronous-like way of working with Promises. It allows us to write asynchronous code that looks and behaves like synchronous code, making it easier to understand and maintain.
To use async/await
, we mark a function as async
, which allows us to use the await
keyword inside the function to wait for Promises to resolve. The await
keyword can only be used inside async
functions.
Here's an example that demonstrates how to use async/await
:
const fetchData = () => { return new Promise((resolve, reject) => { setTimeout(() => { resolve('Data fetched successfully!'); }, 2000); }); }; const processData = async () => { try { const data = await fetchData(); console.log(data); const processedData = await new Promise((resolve, reject) => { setTimeout(() => { resolve('Data processed successfully!'); }, 2000); }); console.log(processedData); } catch (error) { console.error(error); } }; processData();
In the above example, we define a fetchData
function that returns a Promise that resolves after a 2-second delay. We then define an async
function processData
that uses await
to wait for the Promises to resolve. If any error occurs during the execution of the Promises, the catch
block will be executed.
These advanced Promise techniques can greatly simplify the handling of asynchronous JavaScript code and make it more readable and maintainable. Experiment with these techniques to enhance your understanding and productivity when working with Promises.
Related Article: How To Check For An Empty String In Javascript
Creating a Custom Promise
ES6 Promises provide a convenient way to work with asynchronous JavaScript code. However, there may be situations where you need to create your own custom Promise. In this chapter, we will explore how to create a custom Promise in JavaScript.
To create a custom Promise, you need to understand the basic structure and behavior of a Promise. A Promise is an object that represents the eventual completion or failure of an asynchronous operation, and it has three states: pending, fulfilled, or rejected.
Let's start by creating a simple custom Promise that resolves after a certain delay. We can use the setTimeout function to simulate an asynchronous operation. Here's an example:
function delay(ms) { return new Promise(function (resolve, reject) { setTimeout(resolve, ms); }); } delay(2000) .then(function () { console.log("Promise resolved after 2 seconds"); }) .catch(function (error) { console.error("Promise rejected:", error); });
In the above code, the delay
function takes a parameter ms
which represents the delay in milliseconds. It returns a new Promise that resolves after the specified delay using the setTimeout
function. We can then use the then
method to handle the resolved state and the catch
method to handle any errors.
You can also create a custom Promise that rejects instead of resolving. Here's an example:
function throwError() { return new Promise(function (resolve, reject) { setTimeout(reject, 2000, new Error("Promise rejected")); }); } throwError() .then(function () { console.log("Promise resolved"); }) .catch(function (error) { console.error("Promise rejected:", error.message); });
In the above code, the throwError
function returns a new Promise that rejects after a delay of 2 seconds. The setTimeout
function is used with the reject
parameter to simulate a rejected Promise. We can handle the rejected state using the catch
method.
Creating a custom Promise allows you to encapsulate your own asynchronous operations and handle their completion or failure using the familiar Promise syntax. It gives you more control over the behavior of your asynchronous code and makes it easier to reason about.
Understanding Promise Libraries
When working with ES6 Promises, you have the option to use built-in Promise functionality that is available in modern JavaScript environments. However, there are also several promise libraries available that provide additional features and utilities to simplify asynchronous programming.
These promise libraries offer various advantages, such as better error handling, advanced composition and chaining of promises, and the ability to work with multiple promises concurrently. Some of the popular promise libraries include:
1. Q: Q is a powerful promise library that provides a rich set of features for handling asynchronous operations. It supports promise chaining, error handling, and cancellation of promises. Q also provides a mechanism for transforming values and promises using the then
method.
Here's an example of how you can use Q to create and chain promises:
const Q = require('q'); const add = (a, b) => { const deferred = Q.defer(); setTimeout(() => { if (typeof a === 'number' && typeof b === 'number') { deferred.resolve(a + b); } else { deferred.reject(new Error('Invalid input')); } }, 1000); return deferred.promise; }; add(2, 3) .then(result => console.log(result)) // Output: 5 .catch(error => console.error(error)); // Output: Error: Invalid input
2. Bluebird: Bluebird is another popular promise library known for its performance and extensive feature set. It provides advanced error handling, promise cancellation, and concurrency control. Bluebird also supports promise chaining and transformation using the then
method.
Here's an example of how you can use Bluebird to create and chain promises:
const Promise = require('bluebird'); const multiply = (a, b) => { return new Promise((resolve, reject) => { setTimeout(() => { if (typeof a === 'number' && typeof b === 'number') { resolve(a * b); } else { reject(new Error('Invalid input')); } }, 1000); }); }; multiply(2, 3) .then(result => console.log(result)) // Output: 6 .catch(error => console.error(error)); // Output: Error: Invalid input
3. Axios: Although Axios is primarily an HTTP client library, it also provides a built-in promise implementation. Axios allows you to make asynchronous HTTP requests and handle responses using promises. It supports common promise methods like then
, catch
, and finally
.
Here's an example of how you can use Axios to make an HTTP request and handle the response using promises:
const axios = require('axios'); axios.get('https://api.example.com/data') .then(response => console.log(response.data)) .catch(error => console.error(error));
These are just a few examples of promise libraries available in the JavaScript ecosystem. Each library has its own set of features and advantages, so it's important to choose the one that best suits your needs.
Using Promises with Fetch API
The Fetch API is a modern, built-in JavaScript API that provides a way to make HTTP requests. It returns a Promise that resolves to the Response object representing the response to the request. This makes it a perfect candidate to be used with Promises for handling asynchronous operations in JavaScript.
To use Promises with the Fetch API, you can simply chain a Promise's then()
method to handle the response. Here's an example:
fetch('https://api.example.com/data') .then(response => { if (!response.ok) { throw new Error('Network response was not ok'); } return response.json(); }) .then(data => { console.log(data); }) .catch(error => { console.error('Error:', error); });
In the above example, we make a request to the URL 'https://api.example.com/data' using the Fetch API. We chain the then()
method to handle the response. Inside the first then()
callback, we check if the response is not okay (i.e., the response status is not in the range of 200-299). If it's not okay, we throw an error. Otherwise, we call the json()
method on the response object to parse the response body as JSON. Finally, we log the parsed data to the console.
If there is an error at any point in the Promise chain, the catch()
method will be called to handle the error. In the example above, we log the error message to the console.
Using Promises with the Fetch API allows us to handle asynchronous HTTP requests in a more elegant and readable way. We can easily chain multiple promises together to perform sequential or parallel requests, handle errors, and process the response data.
It's worth noting that the Fetch API has built-in support for handling various types of data, including JSON, FormData, and Blobs. You can specify the request headers, request method, request body, and other options using the Fetch API's parameters.
To learn more about the Fetch API, you can refer to the official documentation: https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API.
Implementing Promises in Node.js
ES6 promises provide a clean and elegant way to handle asynchronous operations in JavaScript. In this chapter, we will explore how to implement promises in Node.js, a popular server-side JavaScript runtime environment.
To start using promises in Node.js, we need to understand how to create and consume them. Let's begin by creating a simple promise.
const myPromise = new Promise((resolve, reject) => { // Perform an asynchronous operation setTimeout(() => { const randomNumber = Math.random(); if (randomNumber > 0.5) { resolve(randomNumber); } else { reject(new Error('Number less than 0.5')); } }, 1000); }); myPromise .then((result) => { console.log('Resolved:', result); }) .catch((error) => { console.error('Rejected:', error); });
In the above code snippet, we create a promise using the Promise
constructor. Inside the constructor, we perform an asynchronous operation using setTimeout
to simulate a delay. If the randomly generated number is greater than 0.5, the promise is resolved with the number. Otherwise, it is rejected with an error.
We can consume the promise using the then
and catch
methods. The then
method is called when the promise is resolved, and it receives the resolved value as an argument. The catch
method is called when the promise is rejected, and it receives the error as an argument.
Promises also provide a way to chain multiple asynchronous operations together. This can be achieved by returning a promise inside the then
method. Let's see an example:
function getUserData(userId) { return new Promise((resolve, reject) => { // Fetch user data from an API setTimeout(() => { const userData = { id: userId, name: 'John Doe', age: 25 }; resolve(userData); }, 1000); }); } function getUserPosts(userData) { return new Promise((resolve, reject) => { // Fetch user posts from an API setTimeout(() => { const userPosts = ['Post 1', 'Post 2', 'Post 3']; resolve({ ...userData, posts: userPosts }); }, 1000); }); } getUserData(123) .then(getUserPosts) .then((userDataWithPosts) => { console.log('User data with posts:', userDataWithPosts); }) .catch((error) => { console.error('Error:', error); });
In the above code snippet, we define two functions that return promises: getUserData
and getUserPosts
. The getUserData
function fetches user data from an API, and the getUserPosts
function fetches user posts from an API.
By chaining the promises returned by these functions using the then
method, we can retrieve the user data along with their posts in a sequential manner. The resolved value from the previous promise is passed as an argument to the next then
method.
Node.js also provides some built-in modules that use promises. One such module is fs.promises
for file system operations. Here's an example:
const fs = require('fs').promises; fs.readFile('file.txt', 'utf-8') .then((data) => { console.log('File content:', data); }) .catch((error) => { console.error('Error:', error); });
In this example, we use the readFile
method from the fs.promises
module to read the contents of a file asynchronously. The method returns a promise, which we can consume using the then
and catch
methods.
Implementing promises in Node.js allows us to write more readable and maintainable asynchronous code. By utilizing promises, we can handle errors more effectively and chain multiple asynchronous operations together for a more streamlined execution flow.
Related Article: How to Encode a String to Base64 in Javascript
Best Practices for Working with Promises
Promises are a powerful tool for handling asynchronous JavaScript code. However, to effectively use promises and avoid common pitfalls, it's important to follow some best practices. In this chapter, we'll discuss some of these best practices.
1. Always Handle Errors
When working with promises, it's crucial to handle errors properly. If a promise is rejected and the error is not caught, it will be silently ignored, leading to unexpected behavior in your application. To handle errors, use the .catch()
method or the try...catch
statement.
Here's an example of handling errors using the .catch()
method:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Process the data }) .catch(error => { // Handle the error console.error(error); });
2. Avoid the Pyramid of Doom
One of the main advantages of promises is their ability to chain multiple asynchronous operations in a readable way. However, if you're not careful, you can end up with a pyramid of nested .then()
calls, also known as the "Pyramid of Doom". This can make your code hard to read and maintain.
To avoid the Pyramid of Doom, use the .then()
method to chain promises instead. This allows you to separate the different steps of your asynchronous code and makes it easier to follow the flow.
Here's an example of chaining promises:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Process the data return fetch('https://api.example.com/otherdata'); }) .then(response => response.json()) .then(otherData => { // Process the other data }) .catch(error => { // Handle errors console.error(error); });
3. Use Promise.all() for Parallel Execution
In some cases, you may need to perform multiple asynchronous operations in parallel and wait for all of them to complete before continuing. The Promise.all()
method allows you to do this easily.
Here's an example of using Promise.all()
to fetch data from multiple endpoints simultaneously:
const promises = [ fetch('https://api.example.com/data1'), fetch('https://api.example.com/data2'), fetch('https://api.example.com/data3') ]; Promise.all(promises) .then(responses => Promise.all(responses.map(response => response.json()))) .then(data => { // Process the data }) .catch(error => { // Handle errors console.error(error); });
Related Article: How To Remove Duplicates From A Javascript Array
4. Be Mindful of Promise Chaining
When chaining promises, it's important to be aware of how values are passed between .then()
callbacks. By default, the value returned by a .then()
callback becomes the resolved value of the next promise in the chain. However, if you don't return anything from a .then()
callback, the next promise will be resolved with an undefined value.
To avoid unexpected behavior, always make sure to return a value from your .then()
callbacks, even if it's just a pass-through of the input.
Here's an example that demonstrates this:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Process the data return processData(data); }) .then(result => { // Use the result }) .catch(error => { // Handle errors console.error(error); });
These are just a few best practices to keep in mind when working with promises. By following these guidelines, you can write cleaner and more maintainable asynchronous JavaScript code.