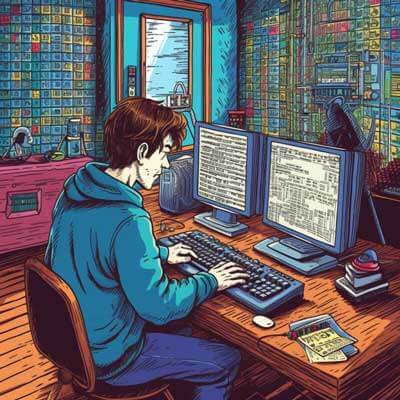
Table of Contents
Introduction to Command Line Arguments
Command line arguments are values passed to a program when it is executed through the command line interface. These arguments provide a way to customize the behavior of the program without modifying its source code. In Python, command line arguments are accessed through the sys.argv
list, where sys
is a built-in module in Python.
To demonstrate how command line arguments work, consider the following example:
import sys def main(): # Print the command line arguments print(sys.argv) if __name__ == "__main__": main()
When running this script from the command line with the command python script.py arg1 arg2
, the program will output ['script.py', 'arg1', 'arg2']
. The first element of sys.argv
is always the name of the script itself.
Related Article: How to Use 'In' in a Python If Statement
The Role of Command Line Arguments
Command line arguments play a crucial role in making Python programs more flexible and configurable. They allow users to provide input to a program without the need to modify its source code. Command line arguments can be used to specify file names, program options, or any other values that affect the behavior of the program.
For example, consider a script that calculates the sum of numbers provided as command line arguments:
import sys def main(): # Remove the script name from the arguments args = sys.argv[1:] # Convert arguments to integers and calculate the sum numbers = [int(arg) for arg in args] total = sum(numbers) # Print the result print(f"The sum of the numbers is: {total}") if __name__ == "__main__": main()
Running this script with the command python sum.py 5 10 15
will output The sum of the numbers is: 30
, as it calculates the sum of the provided numbers.
Using Command Line Arguments
In Python, command line arguments are accessed through the sys.argv
list. This list contains all the arguments passed to the program, including the script name itself. To use command line arguments effectively, you need to extract the relevant values from sys.argv
and process them accordingly.
Here's an example of how to use command line arguments to control the behavior of a program:
import sys def main(): # Check if the "--verbose" flag is present if "--verbose" in sys.argv: print("Verbose mode enabled") # Get the value of the "--file" option file_option_index = sys.argv.index("--file") file_name = sys.argv[file_option_index + 1] print(f"File name: {file_name}") if __name__ == "__main__": main()
Running this script with the command python program.py --verbose --file data.txt
will output:
Verbose mode enabled File name: data.txt
In this example, the script checks if the --verbose
flag is present in sys.argv
and prints a message accordingly. It also retrieves the value of the --file
option by finding its index in sys.argv
and accessing the next element.
Parsing Command Line Arguments
While accessing command line arguments directly from sys.argv
works for simple cases, it can become cumbersome for more complex scenarios. Python provides several libraries and modules to simplify the parsing of command line arguments. One popular option is the argparse
module, which provides a powerful and flexible way to define and parse command line arguments.
To demonstrate the usage of argparse
, consider the following example:
import argparse def main(): parser = argparse.ArgumentParser(description="Process some integers.") parser.add_argument("numbers", metavar="N", type=int, nargs="+", help="an integer to be processed") parser.add_argument("--verbose", action="store_true", help="enable verbose mode") args = parser.parse_args() if args.verbose: print("Verbose mode enabled") total = sum(args.numbers) print(f"The sum of the numbers is: {total}") if __name__ == "__main__": main()
In this example, we define two command line arguments using argparse
: numbers
and --verbose
. The numbers
argument expects one or more integers, while --verbose
is an optional flag. The argparse.ArgumentParser
class is used to create an argument parser, and the add_argument
method is used to define the arguments.
Running this script with the command python program.py 5 10 15 --verbose
will output:
Verbose mode enabled The sum of the numbers is: 30
Here, argparse
takes care of parsing the command line arguments, validating the input, and providing helpful error messages when the input is incorrect.
Related Article: How to Use Class And Instance Variables in Python
Option and Argument Parsing with optparse
In addition to argparse
, Python also provides the optparse
module for parsing command line options and arguments. optparse
offers a similar functionality to argparse
, but with a slightly different syntax.
Here's an example of how to use optparse
to parse command line options and arguments:
import optparse def main(): parser = optparse.OptionParser() parser.add_option("-v", "--verbose", action="store_true", dest="verbose", help="enable verbose mode") parser.add_option("-f", "--file", dest="file_name", help="specify a file name") (options, args) = parser.parse_args() if options.verbose: print("Verbose mode enabled") if options.file_name: print(f"File name: {options.file_name}") # Process any additional arguments for arg in args: print(f"Additional argument: {arg}") if __name__ == "__main__": main()
In this example, we define two options using optparse
: -v, --verbose
and -f, --file
. The --verbose
option is a flag that enables verbose mode, while --file
takes an argument specifying a file name. The optparse.OptionParser
class is used to create an option parser, and the add_option
method is used to define the options.
Running this script with the command python program.py -v -f data.txt additional_argument
will output:
Verbose mode enabled File name: data.txt Additional argument: additional_argument
Here, optparse
takes care of parsing the command line options and arguments, and provides convenient access to the values through the options
and args
variables.
Command Line Arguments Use Cases
Command line arguments are a powerful tool that can be used in a variety of scenarios. Here are three common use cases where command line arguments are particularly helpful:
Use Case: Batch Processing
Batch processing involves applying the same operation to multiple inputs in an automated manner. Command line arguments can be used to specify the inputs and control the behavior of the batch processing script.
For example, consider a script that resizes a collection of images:
import sys from PIL import Image def resize_images(input_folder, output_folder, size): # Logic to resize images def main(): if len(sys.argv) != 4: print("Usage: python resize.py input_folder output_folder size") return input_folder = sys.argv[1] output_folder = sys.argv[2] size = int(sys.argv[3]) resize_images(input_folder, output_folder, size) if __name__ == "__main__": main()
In this example, the script expects three command line arguments: input_folder
, output_folder
, and size
. These arguments define the source folder for the images, the destination folder for the resized images, and the desired size for the output images, respectively.
Running this script with the command python resize.py input_images output_images 300
would resize the images in the input_images
folder, save the resized images in the output_images
folder, and set the size of the output images to 300 pixels.
Use Case: Configuration Options
Command line arguments can also be used to specify configuration options for a program. This allows users to easily customize the behavior of the program without modifying its source code.
For example, consider a script that performs data analysis on a given dataset:
import sys def analyze_data(input_file, output_file, columns): # Logic to analyze data def main(): if len(sys.argv) != 4: print("Usage: python analyze.py input_file output_file columns") return input_file = sys.argv[1] output_file = sys.argv[2] columns = sys.argv[3].split(",") analyze_data(input_file, output_file, columns) if __name__ == "__main__": main()
In this example, the script expects three command line arguments: input_file
, output_file
, and columns
. These arguments define the input file for the data analysis, the output file for the analysis results, and the columns to be analyzed, respectively.
Running this script with the command python analyze.py data.csv results.txt "column1,column2,column3"
would analyze the columns column1
, column2
, and column3
in the data.csv
file and save the results in the results.txt
file.
Related Article: How to Use Python Time Sleep
Use Case: File Input and Output Specification
Command line arguments can also be used to specify file input and output locations for a program. This allows users to easily process files without the need to hardcode the file names in the source code.
For example, consider a script that converts a given file from one format to another:
import sys def convert_file(input_file, output_file): # Logic to convert file def main(): if len(sys.argv) != 3: print("Usage: python convert.py input_file output_file") return input_file = sys.argv[1] output_file = sys.argv[2] convert_file(input_file, output_file) if __name__ == "__main__": main()
In this example, the script expects two command line arguments: input_file
and output_file
. These arguments define the input file to be converted and the output file where the converted data will be stored, respectively.
Running this script with the command python convert.py input.txt output.csv
would convert the input.txt
file to the CSV format and save it as output.csv
.
Best Practices for Command Line Arguments
When working with command line arguments, it is important to follow some best practices to ensure the usability and maintainability of your programs. Here are a few best practices to consider:
The Importance of Error Handling
Error handling is crucial when dealing with command line arguments. It is important to check the validity of the provided arguments and provide helpful error messages when the input is incorrect or incomplete. This helps users understand how to use the program correctly and avoids unexpected behavior or crashes.
For example, consider the following code snippet that checks if the correct number of arguments is provided:
import sys def main(): if len(sys.argv) != 3: print("Usage: python program.py arg1 arg2") return # Rest of the program logic if __name__ == "__main__": main()
In this example, the script expects two command line arguments. If the number of arguments is not equal to 3, a usage message is printed, and the program exits. This provides a clear indication to the user about the correct usage of the program.
Dealing with Parsing Errors
When using libraries or modules like argparse
or optparse
for parsing command line arguments, it is important to handle parsing errors properly. These libraries typically raise specific exceptions when the input is incorrect or invalid. Handling these exceptions allows you to provide meaningful error messages to the user and gracefully exit the program when necessary.
For example, consider the following code snippet that handles parsing errors using argparse
:
import argparse def main(): parser = argparse.ArgumentParser() parser.add_argument("numbers", metavar="N", type=int, nargs="+", help="an integer to be processed") try: args = parser.parse_args() total = sum(args.numbers) print(f"The sum of the numbers is: {total}") except argparse.ArgumentError as e: print(f"Error: {e}") return if __name__ == "__main__": main()
In this example, if the user provides invalid input for the numbers
argument, an argparse.ArgumentError
is raised. The code inside the except
block catches this exception, prints an error message, and exits the program.
Related Article: How to Add New Keys to a Python Dictionary
Real World Example: Implementing a File Converter
Let's consider a real-world example of implementing a file converter using command line arguments. This script converts a given text file from one encoding to another.
import sys def convert_file(input_file, output_file, input_encoding, output_encoding): try: with open(input_file, "r", encoding=input_encoding) as f_in: with open(output_file, "w", encoding=output_encoding) as f_out: for line in f_in: f_out.write(line) except FileNotFoundError: print("Error: Input file not found.") except UnicodeDecodeError: print("Error: Unable to decode input file.") except UnicodeEncodeError: print("Error: Unable to encode output file.") def main(): if len(sys.argv) != 5: print("Usage: python convert.py input_file output_file input_encoding output_encoding") return input_file = sys.argv[1] output_file = sys.argv[2] input_encoding = sys.argv[3] output_encoding = sys.argv[4] convert_file(input_file, output_file, input_encoding, output_encoding) if __name__ == "__main__": main()
In this example, the script expects four command line arguments: input_file
, output_file
, input_encoding
, and output_encoding
. These arguments define the input file to be converted, the output file where the converted data will be stored, the encoding of the input file, and the encoding of the output file, respectively.
Running this script with the command python convert.py input.txt output.txt utf-8 latin-1
would convert the input.txt
file from UTF-8 encoding to Latin-1 encoding and save it as output.txt
.
Real World Example: Automating Data Analysis
Let's explore another real-world example of using command line arguments to automate data analysis. This script calculates the average value of a specific column in a CSV file.
import sys import csv def calculate_average(input_file, column): try: with open(input_file, "r") as f: reader = csv.reader(f) header = next(reader) column_index = header.index(column) total = 0 count = 0 for row in reader: value = float(row[column_index]) total += value count += 1 average = total / count print(f"The average value of column '{column}' is: {average}") except FileNotFoundError: print("Error: Input file not found.") except ValueError: print("Error: Invalid column or data format.") except ZeroDivisionError: print("Error: No data found.") def main(): if len(sys.argv) != 3: print("Usage: python analyze.py input_file column") return input_file = sys.argv[1] column = sys.argv[2] calculate_average(input_file, column) if __name__ == "__main__": main()
In this example, the script expects two command line arguments: input_file
and column
. These arguments define the input file to be analyzed and the column for which the average value will be calculated, respectively.
Running this script with the command python analyze.py data.csv price
would calculate the average value of the price
column in the data.csv
file.
Performance Consideration: Option Parsing Speed
When working with command line arguments, it's important to consider performance, especially when parsing a large number of options. Different libraries or modules for parsing command line arguments may have different performance characteristics.
For example, let's compare the performance of argparse
and optparse
by parsing a large number of options:
import argparse import optparse import time def argparse_example(): parser = argparse.ArgumentParser() for i in range(10000): parser.add_argument(f"--option{i}") args = parser.parse_args() def optparse_example(): parser = optparse.OptionParser() for i in range(10000): parser.add_option(f"--option{i}") (options, args) = parser.parse_args() start_time = time.time() argparse_example() end_time = time.time() print(f"argparse: {end_time - start_time} seconds") start_time = time.time() optparse_example() end_time = time.time() print(f"optparse: {end_time - start_time} seconds")
In this example, we define 10,000 options using both argparse
and optparse
. We then measure the time it takes to parse these options. Running this script shows that argparse
generally performs better than optparse
when parsing a large number of options.
Keep in mind that the actual performance may vary depending on the specific use case and the complexity of the options being parsed.
Performance Consideration: Argument Validation
Another performance consideration when working with command line arguments is argument validation. Validating and processing command line arguments can be an intensive task, especially when dealing with complex validations or large amounts of data.
To ensure good performance, it's important to optimize argument validation by following these best practices:
- Avoid unnecessary validation: Only validate the arguments that require validation. Skipping unnecessary validations can significantly improve performance.
- Use efficient data structures: Choose appropriate data structures for storing and validating command line arguments. Using efficient data structures, such as sets or dictionaries, can lead to better performance.
- Implement early exit conditions: If an argument fails validation, exit early rather than continuing with unnecessary processing. This can save processing time and resources.
Related Article: Converting cURL Commands to Python
Advanced Technique: Dynamic Argument Generation
In some scenarios, the set of command line arguments may not be known in advance and needs to be dynamically generated based on certain conditions or configurations. Dynamic argument generation allows you to create command line interfaces that adapt to different situations.
To demonstrate dynamic argument generation, consider the following example:
import argparse def main(): parser = argparse.ArgumentParser() # Add a required argument parser.add_argument("input_file", help="input file path") # Add an optional argument based on a condition if condition: parser.add_argument("--optional_arg", help="optional argument") args = parser.parse_args() if __name__ == "__main__": main()
In this example, the script conditionally adds an optional argument to the argument parser based on some condition. If the condition is met, the --optional_arg
argument is added; otherwise, it is not included. This allows the command line interface to adapt to different situations dynamically.
By using dynamic argument generation, you can create flexible and adaptable command line interfaces that provide the necessary options based on runtime conditions or configurations.
Advanced Technique: Nested Argument Parsing
In some cases, it may be necessary to define nested command line arguments, where certain arguments are only valid or required depending on other arguments. argparse
provides a way to define nested arguments using subparsers.
Here's an example of how to use nested argument parsing with argparse
:
import argparse def main(): parser = argparse.ArgumentParser() subparsers = parser.add_subparsers(dest="command") # Define the "command1" command command1_parser = subparsers.add_parser("command1") command1_parser.add_argument("arg1", help="argument for command1") # Define the "command2" command command2_parser = subparsers.add_parser("command2") command2_parser.add_argument("arg2", help="argument for command2") args = parser.parse_args() if args.command == "command1": print(f"Executing command1 with argument: {args.arg1}") elif args.command == "command2": print(f"Executing command2 with argument: {args.arg2}") if __name__ == "__main__": main()
In this example, we define two commands, "command1" and "command2", using subparsers. Each command has its own set of arguments. The argparse.ArgumentParser
class is used to create the main argument parser, and the add_subparsers
method is used to define the subparsers.
Running this script with the command python program.py command1 value1
would execute "command1" with the argument "value1".
By using nested argument parsing, you can create command line interfaces with hierarchical structures and define different sets of arguments for different commands or subcommands.
Code Snippet: Basic Command Line Argument Parsing
Here's a basic code snippet that demonstrates how to parse command line arguments using sys.argv
:
import sys def main(): # Remove the script name from the arguments args = sys.argv[1:] # Process the command line arguments for arg in args: print(arg) if __name__ == "__main__": main()
In this example, the script removes the script name from sys.argv
and processes the remaining command line arguments. Each argument is printed on a separate line.
Running this script with the command python program.py arg1 arg2 arg3
would output:
arg1 arg2 arg3
This basic code snippet serves as a starting point for handling command line arguments using sys.argv
.
Code Snippet: Using optparse for Option Parsing
Here's a code snippet that demonstrates how to use optparse
for parsing command line options:
import optparse def main(): parser = optparse.OptionParser() parser.add_option("-v", "--verbose", action="store_true", dest="verbose", help="enable verbose mode") parser.add_option("-f", "--file", dest="file_name", help="specify a file name") (options, args) = parser.parse_args() if options.verbose: print("Verbose mode enabled") if options.file_name: print(f"File name: {options.file_name}") # Process any additional arguments for arg in args: print(f"Additional argument: {arg}") if __name__ == "__main__": main()
In this example, we define two options using optparse
: -v, --verbose
and -f, --file
. The --verbose
option is a flag that enables verbose mode, while --file
takes an argument specifying a file name. The optparse.OptionParser
class is used to create an option parser, and the add_option
method is used to define the options.
Running this script with the command python program.py -v -f data.txt additional_argument
would output:
Verbose mode enabled File name: data.txt Additional argument: additional_argument
This code snippet demonstrates how to use optparse
for parsing command line options and arguments.
Related Article: How To Exit/Deactivate a Python Virtualenv
Code Snippet: Handling Argument Errors
Here's a code snippet that demonstrates how to handle argument errors when using argparse
:
import argparse def main(): parser = argparse.ArgumentParser() parser.add_argument("numbers", metavar="N", type=int, nargs="+", help="an integer to be processed") try: args = parser.parse_args() total = sum(args.numbers) print(f"The sum of the numbers is: {total}") except argparse.ArgumentError as e: print(f"Error: {e}") return if __name__ == "__main__": main()
In this example, if the user provides invalid input for the numbers
argument, an argparse.ArgumentError
is raised. The code inside the except
block catches this exception, prints an error message, and exits the program.
Running this script with the command python program.py 1 2 a
would output:
Error: argument numbers: invalid int value: 'a'
This code snippet demonstrates how to handle argument errors when using argparse
.
Code Snippet: Implementing a Progress Indicator
Here's a code snippet that demonstrates how to implement a progress indicator using command line arguments:
import sys import time def process_data(data, show_progress): total = len(data) for i, item in enumerate(data): # Process item if show_progress: progress = (i + 1) / total * 100 sys.stdout.write(f"\rProgress: {progress:.2f}%") sys.stdout.flush() time.sleep(0.1) print("\nProcessing complete") def main(): data = [1, 2, 3, 4, 5] if "--show-progress" in sys.argv: show_progress = True else: show_progress = False process_data(data, show_progress) if __name__ == "__main__": main()
In this example, the script processes a list of data items. If the --show-progress
flag is present in sys.argv
, a progress indicator is displayed during the processing. The progress indicator is updated after processing each item and shows the percentage of completion.
Running this script with the command python program.py --show-progress
would display a progress indicator during the processing of the data items.
This code snippet demonstrates how to implement a progress indicator using command line arguments and the sys.stdout
stream.
Code Snippet: Creating a Command Line Interface for a Web API
Here's a code snippet that demonstrates how to create a command line interface for a web API:
import sys import requests def get_data(url): response = requests.get(url) if response.status_code == 200: data = response.json() return data else: print(f"Error: Failed to retrieve data from {url}") return None def main(): if len(sys.argv) != 2: print("Usage: python program.py url") return url = sys.argv[1] data = get_data(url) if data: # Process the retrieved data pass if __name__ == "__main__": main()
In this example, the script retrieves data from a web API based on the provided URL. The URL is passed as a command line argument. If the retrieval is successful, the data is processed; otherwise, an error message is displayed.
Running this script with the command python program.py https://api.example.com/data
would retrieve data from the specified URL and process it.
This code snippet demonstrates how to create a command line interface for interacting with a web API.