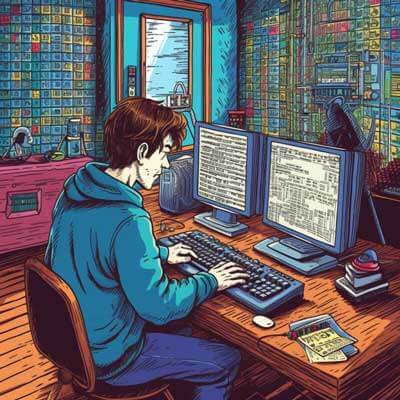
Table of Contents
Introduction to Keywords and Identifiers
Keywords and identifiers are fundamental concepts in Python programming. In this chapter, we will explore the differences between keywords and identifiers and their significance in Python code.
Keywords in Python are reserved words that have predefined meanings and cannot be used as identifiers. They are an essential part of the language syntax and serve specific purposes. On the other hand, identifiers are user-defined names used to identify variables, functions, classes, modules, and other objects in Python code.
Let's delve deeper into keywords and identifiers with some examples.
Related Article: How to Join Sequences in Python
Detailed Explanation on Keywords
Keywords in Python are words that have predefined meanings and are reserved for specific purposes. These words cannot be used as identifiers, such as variable names or function names. Python has a set of keywords that are part of the language syntax and cannot be changed or redefined.
Here are some examples of keywords in Python:
import keyword print(keyword.kwlist)
This code snippet will display a list of all the keywords in Python. Some common keywords include if
, else
, for
, while
, def
, class
, import
, and return
.
Keywords play a crucial role in defining the structure of Python code. They determine the flow of control, define loops and conditional statements, and specify how functions and classes are defined. It is important to familiarize yourself with the keywords and their usage in order to write correct and efficient Python code.
Types of Keywords: Reserved and Non-reserved
Keywords in Python can be classified into two types: reserved and non-reserved keywords.
Reserved keywords are part of the Python language syntax and have predefined meanings. They cannot be used as identifiers and serve specific purposes in the code. Examples of reserved keywords include if
, else
, for
, while
, def
, and class
.
Non-reserved keywords, also known as built-in functions or methods, are words that have special meanings in Python but are not reserved. These keywords can be used as identifiers, but it is generally not recommended to do so to avoid confusion. Examples of non-reserved keywords include print
, len
, str
, list
, dict
, and range
.
Let's take a look at some examples to understand the difference between reserved and non-reserved keywords:
# Reserved keyword as an identifier def if_function(): print("This is a function named 'if_function'.") # Non-reserved keyword as an identifier print = "Hello, World!" print(print)
In the first example, the function if_function
has a reserved keyword if
as part of its identifier. This is allowed in Python, but it can lead to confusion and is generally discouraged.
In the second example, the non-reserved keyword print
is used as an identifier for a variable. This is legal in Python, but it is not recommended because it overrides the built-in print
function and can cause unexpected behavior.
Understanding the distinction between reserved and non-reserved keywords is important for writing clean and maintainable Python code.
Keywords: Real World Examples
Keywords are an integral part of Python programming and are used extensively in real-world applications. Let's explore some practical examples of how keywords are used in Python code.
Example 1: Conditional Statements
age = 25 if age >= 18: print("You are an adult.") else: print("You are a minor.")
In this example, the if
and else
keywords are used to define a conditional statement. Depending on the value of the variable age
, either the message "You are an adult" or "You are a minor" will be printed. The keywords if
and else
determine the flow of control in the code.
Example 2: Looping Statements
numbers = [1, 2, 3, 4, 5] for num in numbers: print(num)
In this example, the for
keyword is used to define a loop that iterates over the elements of the numbers
list. The variable num
takes on the value of each element in the list, and the print
statement is executed for each iteration. The for
keyword controls the repetition of the code block.
These are just a few examples of how keywords are used in real-world Python code. Understanding their usage and applying them correctly is essential for writing effective and efficient Python programs.
Related Article: Python's Dict Tutorial: Is a Dictionary a Data Structure?
Best Practices with Keywords
When working with keywords in Python, it is important to follow certain best practices to ensure code readability, maintainability, and avoid potential issues. Here are some best practices to keep in mind:
1. Avoid using keywords as identifiers: As mentioned earlier, keywords have predefined meanings in Python and should not be used as variable names, function names, or any other identifiers. This can lead to confusion and errors.
2. Use meaningful variable and function names: Choose descriptive names for your variables and functions that clearly convey their purpose. This makes your code more readable and understandable.
3. Use keywords as intended: Keywords have specific purposes in Python, such as defining control flow, loops, functions, and classes. Use them according to their intended purpose to ensure code clarity and maintainability.
4. Be consistent with indentation and formatting: Proper indentation and consistent formatting help improve the readability of your code. Follow the PEP 8 style guide for Python code to maintain a consistent coding style across your projects.
Performance Considerations Using Keywords
Keywords in Python do not have a direct impact on the performance of your code. They are part of the language syntax and are interpreted by the Python interpreter. However, there are certain performance considerations to keep in mind when using keywords:
1. Avoid unnecessary branching: Excessive use of conditional statements (if
, else
, elif
) can impact the performance of your code, especially if the conditions are complex or involve expensive operations. Consider optimizing your code by reducing unnecessary branching.
2. Optimize loops: Loops, such as for
and while
, can be performance bottlenecks if not used efficiently. Avoid unnecessary iterations and use optimized looping techniques like list comprehensions or generator expressions when appropriate.
3. Use built-in functions: Python provides a rich set of built-in functions that are optimized for performance. Utilize these functions instead of reinventing the wheel or writing custom solutions that may be less efficient.
4. Profile and optimize your code: If you observe performance issues in your code, use profiling tools to identify performance bottlenecks and optimize the critical sections of your code. This may involve algorithmic improvements, data structure optimizations, or caching techniques.
Advanced Techniques for Keywords Usage
While keywords in Python have predefined meanings and usage, there are advanced techniques and patterns that can enhance your code's functionality and expressiveness. Here are a few advanced techniques for using keywords:
1. Keyword arguments: Python allows passing arguments to functions using keyword arguments, where arguments are specified by name instead of their position. This provides flexibility and improves code readability. For example:
def greet(name, age): print(f"Hello, {name}! You are {age} years old.") greet(name="Alice", age=25)
In this example, the function greet
takes two keyword arguments name
and age
. By specifying the arguments by name when calling the function, the code becomes more self-explanatory.
2. Context managers: Context managers, implemented using the with
statement, allow you to manage resources that need to be acquired and released safely. Python provides a built-in keyword with
for using context managers. For example:
with open("file.txt", "r") as file: contents = file.read() print(contents)
In this example, the with
keyword is used to create a context manager for opening a file. The file is automatically closed when the code block under the with
statement is exited, ensuring proper resource cleanup.
3. Decorators: Decorators are a powerful feature in Python that allow you to modify the behavior of functions or classes without changing their source code. Decorators are implemented using the @
symbol followed by the decorator function name. For example:
def debug(func): def wrapper(*args, **kwargs): print(f"Calling function {func.__name__}") return func(*args, **kwargs) return wrapper @debug def add(a, b): return a + b result = add(3, 5) print(result)
In this example, the debug
function is a decorator that adds debug logging to the add
function. The @debug
syntax is used to apply the decorator to the add
function.
These advanced techniques demonstrate how keywords can be leveraged to enhance the functionality and expressiveness of your Python code.
Common Errors in Keywords Usage and Their Solutions
When working with keywords in Python, it is common to encounter certain errors or pitfalls. Let's explore some common errors and their solutions:
1. SyntaxError: invalid syntax: This error occurs when you use keywords incorrectly or in an unexpected context. For example:
for = 10
In this example, the keyword for
is used as an identifier, causing a SyntaxError. To fix this, choose a different variable name that is not a keyword.
2. NameError: name 'x' is not defined: This error occurs when you use an identifier that has not been defined or is out of scope. For example:
if x > 0: print("Positive")
If the variable x
is not defined or assigned a value before this code, a NameError will occur. To fix this, make sure to define and assign a value to the variable before using it.
3. AttributeError: 'str' object has no attribute 'append': This error occurs when you try to use a keyword as a method or attribute on an object that does not support it. For example:
message = "Hello, World!" message.append("!")
In this example, the keyword append
is used as a method on a string object, resulting in an AttributeError. To fix this, use the appropriate method or operation for the object type, such as concatenation for strings.
These are just a few examples of common errors related to keyword usage. Understanding these errors and their solutions will help you write bug-free Python code.
Related Article: How To Reset Index In A Pandas Dataframe
Code Snippet: Demonstrating Keywords in Conditional Logic
Here's a code snippet that demonstrates the usage of keywords in conditional logic:
num = 10 if num > 0: print("Positive") elif num < 0: print("Negative") else: print("Zero")
In this code snippet, the if
, elif
, and else
keywords are used to define a conditional statement. Depending on the value of the variable num
, the corresponding message will be printed. The keywords control the flow of execution based on the condition.
Code Snippet: Keywords in Looping Statements
Here's a code snippet that demonstrates the usage of keywords in looping statements:
fruits = ["apple", "banana", "orange"] for fruit in fruits: print(fruit)
In this code snippet, the for
keyword is used to define a loop that iterates over the elements of the fruits
list. The variable fruit
takes on the value of each element in the list, and the print
statement is executed for each iteration. The for
keyword controls the repetition of the code block.
Code Snippet: Keywords in Error Handling
Here's a code snippet that demonstrates the usage of keywords in error handling:
try: x = 1 / 0 except ZeroDivisionError: print("Cannot divide by zero")
In this code snippet, the try
and except
keywords are used to handle exceptions. The code inside the try
block is executed, and if an exception of type ZeroDivisionError
occurs, the code inside the corresponding except
block is executed. The keywords control the flow of execution based on the occurrence of an exception.
Code Snippet: Keywords in Function Definitions
Here's a code snippet that demonstrates the usage of keywords in function definitions:
def greet(name): print(f"Hello, {name}!") greet(name="Alice")
In this code snippet, the def
keyword is used to define a function named greet
. The keyword argument name
is specified, allowing the caller to pass the argument by name when calling the function. The keyword controls the behavior of the function definition.
Related Article: Seamless Integration of Flask with Frontend Frameworks
Code Snippet: Keywords in Class Definitions
Here's a code snippet that demonstrates the usage of keywords in class definitions:
class Rectangle: def __init__(self, width, height): self.width = width self.height = height def area(self): return self.width * self.height rect = Rectangle(width=5, height=3) print(rect.area())
In this code snippet, the class
keyword is used to define a class named Rectangle
. The __init__
method is defined using the def
keyword, which is a special method called when creating an instance of the class. The self
keyword represents the instance of the class. The width
and height
arguments are passed as keyword arguments when creating an instance of the class. The keywords control the behavior of the class definition and instance creation.
In-depth Look at Identifiers
Identifiers are user-defined names used to identify variables, functions, classes, modules, and other objects in Python code. In this chapter, we will explore the rules for creating identifiers, their use cases, and best practices.
Rules for Creating Identifiers
In Python, identifiers are subject to certain rules and conventions. Here are the rules for creating valid identifiers:
1. Valid characters: Identifiers can contain letters (both lowercase and uppercase), digits, and underscores. They must start with a letter or an underscore, but cannot start with a digit. For example, my_variable
, myFunction
, and _private_variable
are valid identifiers.
2. Case-sensitivity: Python is case-sensitive, so my_variable
and my_Variable
are different identifiers.
3. Reserved words: Identifiers cannot be reserved keywords. They should not have the same name as Python keywords, such as if
, else
, for
, while
, def
, and class
. Use meaningful and descriptive names for your identifiers to avoid conflicts.
4. Length: Identifiers can be of any length, but it is recommended to keep them concise and meaningful. Long and convoluted identifiers can make your code harder to read and understand.
5. PEP 8 conventions: Follow the naming conventions defined in the PEP 8 style guide for Python code. Use lowercase letters and underscores for variable and function names (my_variable
, my_function
), and use CamelCase for class names (MyClass
).
Adhering to these rules ensures that your identifiers are consistent, readable, and free from conflicts with reserved keywords.
Use Cases for Identifiers
Identifiers are used throughout Python code to give names to variables, functions, classes, modules, and other objects. Here are some common use cases for identifiers:
1. Variable names: Identifiers are used to name variables, which store data values. Meaningful variable names help you understand the purpose and content of the data being manipulated. For example:
age = 25 name = "Alice"
2. Function names: Identifiers are used to name functions, which encapsulate a set of instructions. Descriptive function names make your code more readable and convey the purpose of the function. For example:
def calculate_area(length, width): return length * width
3. Class names: Identifiers are used to name classes, which define blueprints for creating objects. Class names should be in CamelCase to distinguish them from functions and variables. For example:
class Rectangle: def __init__(self, length, width): self.length = length self.width = width
4. Module names: Identifiers are used to name modules, which are files containing Python code. Module names should be concise and descriptive, following the same rules as variable names. For example:
import my_module
Identifiers are essential for giving names to various elements in your code and enable you to refer to them throughout your program.
Related Article: How to Use Double Precision Floating Values in Python
Identifiers: Real World Examples
Identifiers are used extensively in real-world Python code. Let's explore some practical examples of how identifiers are used:
Example 1: Variable Names
name = "Alice" age = 25 print(f"Hello, {name}! You are {age} years old.")
In this example, the identifiers name
and age
are used to store values and refer to them later in the code. The names are meaningful and help convey the purpose of the variables.
Example 2: Function Names
def calculate_area(length, width): return length * width result = calculate_area(5, 3) print(result)
In this example, the identifier calculate_area
is used to define a function that calculates the area of a rectangle. The name of the function clearly indicates its purpose.
Example 3: Class Names
class Rectangle: def __init__(self, length, width): self.length = length self.width = width def area(self): return self.length * self.width rect = Rectangle(5, 3) print(rect.area())
In this example, the identifier Rectangle
is used to define a class that represents a rectangle. The class provides methods like area
to perform operations on the rectangle objects.
These are just a few examples of how identifiers are used in real-world Python code. Descriptive and meaningful names enhance code readability and maintainability.
Best Practices with Identifiers
When working with identifiers in Python, it is important to follow certain best practices to ensure code readability, maintainability, and avoid potential issues. Here are some best practices to keep in mind:
1. Use descriptive names: Choose names that clearly convey the purpose and meaning of the identifier. Avoid cryptic abbreviations or single-letter variable names, as they can make your code harder to understand.
2. Follow naming conventions: Follow the naming conventions defined in the PEP 8 style guide for Python code. Use lowercase letters and underscores for variable and function names (my_variable
, my_function
), and use CamelCase for class names (MyClass
).
3. Be consistent: Maintain consistency in naming across your codebase. Use similar naming conventions for similar entities to make your code more cohesive and easier to navigate.
4. Avoid single-use variables: Minimize the use of single-use variables that are used only once and have no meaningful name. Instead, directly use the value or expression in the appropriate context. This reduces clutter and improves code readability.
Performance Considerations Using Identifiers
Identifiers in Python do not have a direct impact on the performance of your code. They are used to refer to objects and do not affect the execution speed or memory usage.
However, there are certain performance considerations to keep in mind when working with identifiers:
1. Avoid excessive variable creation: Creating too many variables unnecessarily can lead to increased memory usage and slower execution. Use variables judiciously and avoid creating excessive temporary variables.
2. Minimize attribute lookups: Attribute lookups, such as accessing object attributes or calling object methods, come with some overhead. Minimize attribute lookups in performance-critical sections of your code by storing frequently used attributes in local variables.
3. Optimize data structures: The choice of data structures can impact the performance of your code. Choose the appropriate data structures based on the specific requirements of your program. For example, use dictionaries for fast key-value lookups and lists for sequential access.
4. Use efficient algorithms and operations: The choice of algorithms and operations can significantly affect the performance of your code. Choose algorithms with optimal time and space complexity and use optimized operations provided by Python's built-in functions and libraries.
Advanced Techniques for Identifiers Usage
While identifiers in Python are primarily used for naming variables, functions, classes, and other objects, there are advanced techniques and patterns that can enhance your code's functionality and organization. Here are a few advanced techniques for using identifiers:
1. Name mangling: Python provides a name mangling mechanism to make attributes private within a class. By prefixing an attribute name with double underscores (__
), it becomes a "private" attribute that is not directly accessible outside the class. For example:
class MyClass: def __init__(self): self.__private_attribute = 10 def __private_method(self): print("Private method") obj = MyClass() print(obj._MyClass__private_attribute) # Name mangling to access private attribute obj._MyClass__private_method() # Name mangling to call private method
In this example, the attributes __private_attribute
and __private_method
are "private" within the MyClass
class and can be accessed or called using name mangling.
2. Dunder methods: Dunder (double underscore) methods are special methods in Python that have double underscores at the beginning and end of their names. These methods define the behavior of objects in specific contexts. For example, the __len__
dunder method defines the behavior of the len()
function when used with an object. By implementing dunder methods, you can customize the behavior of your objects in various situations.
3. Context managers: Context managers, implemented using the with
statement, allow you to manage resources that need to be acquired and released safely. Python provides a built-in keyword with
for using context managers. For example, the open()
function returns a context manager that can be used to safely open and close files.
These advanced techniques demonstrate how identifiers can be used to implement advanced functionality and improve the organization of your Python code.
Related Article: Python File Operations: How to Read, Write, Delete, Copy
Common Errors in Identifiers Usage and Their Solutions
When working with identifiers in Python, it is common to encounter certain errors or pitfalls. Let's explore some common errors and their solutions:
1. NameError: name 'x' is not defined: This error occurs when you use an identifier that has not been defined or is out of scope. For example:
print(x)
If the variable x
is not defined or assigned a value before this code, a NameError will occur. To fix this, make sure to define and assign a value to the variable before using it.
2. SyntaxError: invalid syntax: This error occurs when you use invalid characters or violate the naming rules for identifiers. For example:
my-variable = 10
In this example, the identifier my-variable
contains a hyphen, which is not allowed. To fix this, use an underscore instead of a hyphen or choose a different name that adheres to the naming rules.
3. AttributeError: 'str' object has no attribute 'append': This error occurs when you try to use an identifier as a method or attribute on an object that does not support it. For example:
message = "Hello, World!" message.append("!")
In this example, the identifier append
is used as a method on a string object, resulting in an AttributeError. To fix this, use the appropriate method or operation for the object type, such as concatenation for strings.
These are just a few examples of common errors related to identifier usage. Understanding these errors and their solutions will help you write bug-free Python code.
Code Snippet: Identifier Naming in Complex Applications
Here's a code snippet that demonstrates identifier naming in complex applications:
class Customer: def __init__(self, name, age): self.name = name self.age = age def make_purchase(self, product): print(f"{self.name} purchased {product}.") class Product: def __init__(self, name, price): self.name = name self.price = price def apply_discount(self, discount): self.price -= discount customer = Customer("Alice", 25) product = Product("Phone", 1000) product.apply_discount(100) customer.make_purchase(product.name)
In this code snippet, identifiers are used to name classes (Customer
, Product
), objects (customer
, product
), attributes (name
, age
, price
), and methods (make_purchase
, apply_discount
). The names are meaningful and convey the purpose of the entities they represent.
Code Snippet: Identifier Naming for Readability
Here's a code snippet that demonstrates identifier naming for readability:
def calculate_area(length, width): return length * width def print_result(result): print(f"The calculated area is: {result}") l = 5 w = 3 area = calculate_area(l, w) print_result(area)
In this code snippet, identifiers are used to name functions (calculate_area
, print_result
), variables (length
, width
, result
, l
, w
, area
), and function parameters. The names are chosen to be descriptive and improve the readability of the code.
Code Snippet: Identifier Naming for Maintainability
Here's a code snippet that demonstrates identifier naming for maintainability:
class Employee: def __init__(self, name, age, department): self.name = name self.age = age self.department = department def update_age(self, new_age): self.age = new_age def update_department(self, new_department): self.department = new_department employee = Employee("Alice", 25, "Engineering") employee.update_age(26) employee.update_department("Product Management")
In this code snippet, identifiers are used to name a class (Employee
), object (employee
), attributes (name
, age
, department
), and methods (update_age
, update_department
). The names are chosen to be descriptive and reflect the purpose of the entities they represent. This improves the maintainability of the code by making it easier to understand and modify.
Related Article: How to Use Hash Map In Python
Code Snippet: Identifier Naming for Scalability
Here's a code snippet that demonstrates identifier naming for scalability:
class ShoppingCart: def __init__(self): self.items = [] def add_item(self, item): self.items.append(item) def remove_item(self, item): self.items.remove(item) def calculate_total(self): total = 0 for item in self.items: total += item.price return total cart = ShoppingCart() cart.add_item(Product("Phone", 1000)) cart.add_item(Product("Headphones", 100)) cart.add_item(Product("Charger", 20)) total = cart.calculate_total() print(f"The total cost is: ${total}")
In this code snippet, identifiers are used to name a class (ShoppingCart
), object (cart
), attribute (items
), methods (add_item
, remove_item
, calculate_total
), and a variable (total
). The names are chosen to be descriptive and reflect the purpose of the entities they represent. This allows the code to scale by easily adding or modifying functionality related to the shopping cart.
These code snippets demonstrate how identifiers are used in various contexts and highlight the importance of choosing meaningful and descriptive names for improved code readability, maintainability, and scalability.