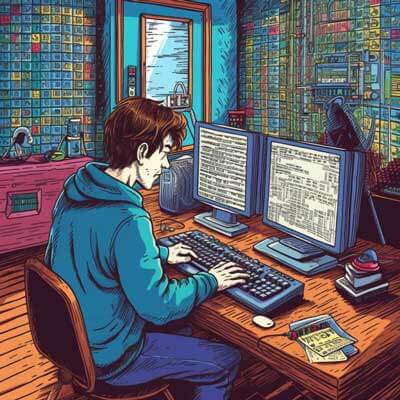
- Third-party tools for FastAPI Integration
- Open source tools for FastAPI Integration
- Styling applications with Bootstrap templates
- Elasticsearch integration in FastAPI
- Advanced search functionalities with FastAPI and Elasticsearch
- FastAPI integration with databases
- SQLAlchemy integration with FastAPI
- MySQL integration with FastAPI using SQLAlchemy
- PostgreSQL integration with FastAPI using SQLAlchemy
- Motor integration with FastAPI for MongoDB
- MongoDB integration with FastAPI using Motor
- Popular third-party tools for FastAPI integration
- Integrating Elasticsearch for advanced search functionalities in FastAPI
- Benefits of using SQLAlchemy for MySQL and PostgreSQL integration with FastAPI
- Setting up FastAPI with databases using SQLAlchemy
- Using Motor for MongoDB integration with FastAPI
- Best practices for integrating third-party and open source tools with FastAPI
- Recommended resources and tutorials for integrating third-party tools with FastAPI
- Example of integrating Elasticsearch for advanced search functionalities in FastAPI
- Optimizing FastAPI performance with third-party and open source tools
- Additional Resources
Third-party tools for FastAPI Integration
FastAPI is a useful and modern web framework for building APIs with Python. While FastAPI provides many built-in features and functionalities, there are times when you may want to integrate third-party tools to enhance the capabilities of your FastAPI applications. In this section, we will explore some popular third-party tools that can be integrated with FastAPI.
One such tool is Bootstrap, a popular open-source CSS framework that allows you to easily style your FastAPI applications. Bootstrap provides a range of pre-built components and styles that can be used to create modern and responsive user interfaces. By integrating Bootstrap with FastAPI, you can quickly and efficiently style your applications without having to write complex CSS code from scratch.
To integrate Bootstrap with FastAPI, you can start by adding the necessary CSS and JavaScript files to your HTML templates. Here is an example of how you can include Bootstrap in your FastAPI application:
<title>FastAPI with Bootstrap</title> <div class="container"> <h1>Welcome to my FastAPI application!</h1> <p>This is a sample paragraph.</p> <button class="btn btn-primary">Click me!</button> </div>
In this example, we have included the necessary CSS and JavaScript files from a CDN (Content Delivery Network). You can also download the Bootstrap files and host them locally if you prefer.
Related Article: How To Exit Python Virtualenv
Open source tools for FastAPI Integration
In addition to third-party tools, there are also several open-source tools that can be integrated with FastAPI to enhance its capabilities. One such tool is Elasticsearch, a useful search and analytics engine. By integrating Elasticsearch with FastAPI, you can add advanced search functionalities to your applications.
To integrate Elasticsearch with FastAPI, you can use the official Elasticsearch Python client library called “elasticsearch-py”. This library provides a high-level API for interacting with Elasticsearch from Python.
Here is an example of how you can integrate Elasticsearch with FastAPI:
from fastapi import FastAPI from elasticsearch import Elasticsearch app = FastAPI() es = Elasticsearch() @app.get("/search") async def search(query: str): results = es.search(index="my_index", body={"query": {"match": {"content": query}}}) return results["hits"]["hits"]
In this example, we have defined a /search
endpoint that accepts a query
parameter. When a GET request is made to this endpoint, the search
function is executed. The function uses the Elasticsearch client to perform a search query on an index called “my_index”. The search results are then returned as the response.
Styling applications with Bootstrap templates
Bootstrap is a popular CSS framework that provides a set of pre-built components and styles for creating modern and responsive web interfaces. By using Bootstrap templates, you can easily style your FastAPI applications without having to write complex CSS code from scratch.
To get started with styling your FastAPI applications using Bootstrap templates, you can use a tool called “FastAPI-Static-Files”. This tool allows you to serve static files, such as CSS and JavaScript, directly from your FastAPI application.
Here is an example of how you can use Bootstrap templates with FastAPI:
First, install the necessary dependencies:
pip install fastapi-static-files
Next, create a directory called “static” in your FastAPI project and copy the Bootstrap CSS and JavaScript files into it. You can download these files from the Bootstrap website.
mkdir static cp bootstrap.min.css static/ cp bootstrap.min.js static/
Then, add the following code to your FastAPI application:
from fastapi import FastAPI from fastapi.staticfiles import StaticFiles app = FastAPI() app.mount("/static", StaticFiles(directory="static"), name="static") @app.get("/") async def index(): return {"message": "Hello, FastAPI with Bootstrap!"}
In this example, we have mounted the “static” directory as a static file directory in our FastAPI application. This allows us to serve the Bootstrap CSS and JavaScript files directly from the application.
Now, when you access the root URL of your FastAPI application, you will see a simple message displayed with the Bootstrap styles applied.
Elasticsearch integration in FastAPI
Elasticsearch is a useful search and analytics engine that can be integrated with FastAPI to add advanced search functionalities to your applications. Elasticsearch provides a distributed, RESTful API that allows you to perform search queries on large volumes of data with high performance and scalability.
To integrate Elasticsearch with FastAPI, you can use the official Elasticsearch Python client library called “elasticsearch-py”. This library provides a high-level API for interacting with Elasticsearch from Python.
Here is an example of how you can integrate Elasticsearch with FastAPI:
First, install the necessary dependencies:
pip install elasticsearch fastapi uvicorn
Next, create a new file called “main.py” and add the following code:
from fastapi import FastAPI from elasticsearch import Elasticsearch app = FastAPI() es = Elasticsearch() @app.get("/search") async def search(query: str): results = es.search(index="my_index", body={"query": {"match": {"content": query}}}) return results["hits"]["hits"]
In this example, we have defined a /search
endpoint that accepts a query
parameter. When a GET request is made to this endpoint, the search
function is executed. The function uses the Elasticsearch client to perform a search query on an index called “my_index”. The search results are then returned as the response.
Related Article: How to Integrate Python with MySQL for Database Queries
Advanced search functionalities with FastAPI and Elasticsearch
FastAPI provides a useful and flexible framework for building APIs, and when combined with Elasticsearch, you can create applications with advanced search functionalities. In this section, we will explore some advanced search functionalities that can be implemented using FastAPI and Elasticsearch.
One such functionality is fuzzy search, which allows users to find results even if their search query contains spelling mistakes or typos. Elasticsearch provides a fuzzy query that can be used to perform fuzzy searches on text fields.
Here is an example of how you can implement fuzzy search using FastAPI and Elasticsearch:
from fastapi import FastAPI from elasticsearch import Elasticsearch app = FastAPI() es = Elasticsearch() @app.get("/search") async def search(query: str): results = es.search( index="my_index", body={ "query": { "fuzzy": { "content": { "value": query, "fuzziness": "AUTO" } } } } ) return results["hits"]["hits"]
In this example, we have modified the /search
endpoint to perform a fuzzy search on the “content” field of the Elasticsearch index. The fuzzy
query is used with the value
parameter set to the search query and the fuzziness
parameter set to “AUTO”, which allows Elasticsearch to automatically determine the fuzziness based on the length of the search query.
Another advanced search functionality is faceted search, which allows users to filter search results based on specific criteria. Elasticsearch provides a feature called aggregations that can be used to implement faceted search.
Here is an example of how you can implement faceted search using FastAPI and Elasticsearch:
from fastapi import FastAPI from elasticsearch import Elasticsearch app = FastAPI() es = Elasticsearch() @app.get("/search") async def search(query: str, filter: str): body = { "query": { "match": { "content": query } }, "aggs": { "filtered_results": { "filter": { "term": { "category": filter } } } } } results = es.search(index="my_index", body=body) return results["hits"]["hits"]
In this example, we have added a new filter
parameter to the /search
endpoint to specify the category to filter the search results. The search query is performed using the match
query, and the aggregation is used to filter the results based on the specified category.
These are just a few examples of the advanced search functionalities that can be implemented using FastAPI and Elasticsearch. By combining the power and flexibility of FastAPI with the scalability and performance of Elasticsearch, you can create applications with highly effective search capabilities.
FastAPI integration with databases
FastAPI provides built-in support for integrating with databases, allowing you to easily connect your applications to a variety of database systems. In this section, we will explore different database integration options available in FastAPI and how to set them up.
One popular choice for database integration in FastAPI is SQLAlchemy, a useful and flexible Object-Relational Mapping (ORM) library for Python. SQLAlchemy provides a high-level API for interacting with databases, allowing you to write database queries using Python code instead of raw SQL.
To use SQLAlchemy with FastAPI, you need to install the necessary dependencies:
<a href="https://www.squash.io/troubleshooting-pip-install-failures-with-fastapi/">pip install fastapi</a> sqlalchemy
Once you have installed the dependencies, you can start integrating SQLAlchemy with FastAPI. Here is an example of how you can set up FastAPI with SQLAlchemy for MySQL and PostgreSQL:
First, import the necessary modules and create a SQLAlchemy Engine
object:
from fastapi import FastAPI from sqlalchemy import create_engine app = FastAPI() engine = create_engine("postgresql://user:password@localhost/dbname")
In this example, we have created a SQLAlchemy Engine
object for connecting to a PostgreSQL database. You can replace the connection URL with the appropriate URL for your MySQL or PostgreSQL database.
Next, you can define a SQLAlchemy Session
class and create a session instance for each request:
from fastapi import Depends from sqlalchemy.orm import Session # Define the Session class class SessionLocal: def __init__(self, engine): self.Session = sessionmaker(bind=engine) def __call__(self): session = self.Session() try: yield session finally: session.close() # Create a session instance for each request def get_db(): db = SessionLocal(engine) try: yield db finally: db.close() @app.get("/") async def index(db: Session = Depends(get_db)): # Perform database operations using the session ...
In this example, we have defined a SessionLocal
class that creates a SQLAlchemy Session
object for each request. The get_db
function is used as a dependency to inject the session instance into the route functions.
SQLAlchemy integration with FastAPI
SQLAlchemy is a useful Object-Relational Mapping (ORM) library for Python that provides a high-level API for interacting with databases. FastAPI provides built-in support for integrating with SQLAlchemy, allowing you to easily connect your FastAPI applications to a variety of database systems.
To integrate SQLAlchemy with FastAPI, you need to install the necessary dependencies:
pip install fastapi sqlalchemy
Once you have installed the dependencies, you can start integrating SQLAlchemy with FastAPI. Here is an example of how you can set up FastAPI with SQLAlchemy:
First, import the necessary modules and create a SQLAlchemy Engine
object:
from fastapi import FastAPI from sqlalchemy import create_engine app = FastAPI() engine = create_engine("sqlite:///./mydatabase.db")
In this example, we have created a SQLAlchemy Engine
object for connecting to a SQLite database. You can replace the connection URL with the appropriate URL for your database system.
Next, you can define a SQLAlchemy Session
class and create a session instance for each request:
from fastapi import Depends from sqlalchemy.orm import Session from sqlalchemy.orm import sessionmaker # Define the Session class class SessionLocal: def __init__(self, engine): self.Session = sessionmaker(bind=engine) def __call__(self): session = self.Session() try: yield session finally: session.close() # Create a session instance for each request def get_db(): db = SessionLocal(engine) try: yield db finally: db.close() @app.get("/") async def index(db: Session = Depends(get_db)): # Perform database operations using the session ...
In this example, we have defined a SessionLocal
class that creates a SQLAlchemy Session
object for each request. The get_db
function is used as a dependency to inject the session instance into the route functions.
Related Article: 16 Amazing Python Libraries You Can Use Now
MySQL integration with FastAPI using SQLAlchemy
FastAPI provides built-in support for integrating with MySQL databases using the SQLAlchemy ORM library. SQLAlchemy allows you to interact with MySQL databases using Python code, making it easier to manage and query your data.
To integrate MySQL with FastAPI using SQLAlchemy, you need to install the necessary dependencies:
pip install fastapi sqlalchemy mysql-connector-python
Once you have installed the dependencies, you can start integrating MySQL with FastAPI. Here is an example of how you can set up FastAPI with SQLAlchemy for MySQL:
First, import the necessary modules and create a SQLAlchemy Engine
object:
from fastapi import FastAPI from sqlalchemy import create_engine app = FastAPI() engine = create_engine("mysql+mysqlconnector://user:password@localhost/dbname")
In this example, we have created a SQLAlchemy Engine
object for connecting to a MySQL database. Replace “user”, “password”, “localhost”, and “dbname” with the appropriate values for your MySQL database.
Next, you can define a SQLAlchemy Session
class and create a session instance for each request:
from fastapi import Depends from sqlalchemy.orm import Session from sqlalchemy.orm import sessionmaker # Define the Session class class SessionLocal: def __init__(self, engine): self.Session = sessionmaker(bind=engine) def __call__(self): session = self.Session() try: yield session finally: session.close() # Create a session instance for each request def get_db(): db = SessionLocal(engine) try: yield db finally: db.close() @app.get("/") async def index(db: Session = Depends(get_db)): # Perform database operations using the session ...
In this example, we have defined a SessionLocal
class that creates a SQLAlchemy Session
object for each request. The get_db
function is used as a dependency to inject the session instance into the route functions.
PostgreSQL integration with FastAPI using SQLAlchemy
FastAPI provides built-in support for integrating with PostgreSQL databases using the SQLAlchemy ORM library. SQLAlchemy allows you to interact with PostgreSQL databases using Python code, making it easier to manage and query your data.
To integrate PostgreSQL with FastAPI using SQLAlchemy, you need to install the necessary dependencies:
pip install fastapi sqlalchemy psycopg2
Once you have installed the dependencies, you can start integrating PostgreSQL with FastAPI. Here is an example of how you can set up FastAPI with SQLAlchemy for PostgreSQL:
First, import the necessary modules and create a SQLAlchemy Engine
object:
from fastapi import FastAPI from sqlalchemy import create_engine app = FastAPI() engine = create_engine("postgresql://user:password@localhost/dbname")
In this example, we have created a SQLAlchemy Engine
object for connecting to a PostgreSQL database. Replace “user”, “password”, “localhost”, and “dbname” with the appropriate values for your PostgreSQL database.
Next, you can define a SQLAlchemy Session
class and create a session instance for each request:
from fastapi import Depends from sqlalchemy.orm import Session from sqlalchemy.orm import sessionmaker # Define the Session class class SessionLocal: def __init__(self, engine): self.Session = sessionmaker(bind=engine) def __call__(self): session = self.Session() try: yield session finally: session.close() # Create a session instance for each request def get_db(): db = SessionLocal(engine) try: yield db finally: db.close() @app.get("/") async def index(db: Session = Depends(get_db)): # Perform database operations using the session ...
In this example, we have defined a SessionLocal
class that creates a SQLAlchemy Session
object for each request. The get_db
function is used as a dependency to inject the session instance into the route functions.
Motor integration with FastAPI for MongoDB
FastAPI provides built-in support for integrating with MongoDB using the Motor library. Motor is an asynchronous Python driver for MongoDB that allows you to interact with MongoDB databases using Python code.
To integrate Motor with FastAPI, you need to install the necessary dependencies:
pip install fastapi motor
Once you have installed the dependencies, you can start integrating Motor with FastAPI. Here is an example of how you can set up FastAPI with Motor for MongoDB:
First, import the necessary modules and create a Motor MotorClient
object:
from fastapi import FastAPI from motor.motor_asyncio import AsyncIOMotorClient app = FastAPI() client = AsyncIOMotorClient() @app.on_event("startup") async def startup_event(): app.mongodb = client["mydatabase"] @app.on_event("shutdown") async def shutdown_event(): client.close()
In this example, we have created a Motor MotorClient
object for connecting to a MongoDB database. The AsyncIOMotorClient
class is used to create an asynchronous MongoDB client. We have also defined startup and shutdown event handlers to connect and disconnect from the MongoDB database.
Next, you can define a route that interacts with the MongoDB database:
from fastapi import Depends from motor.motor_asyncio import AsyncIOMotorClient @app.get("/") async def index(client: AsyncIOMotorClient = Depends(get_mongodb)): collection = client["mycollection"] documents = await collection.find().to_list(length=None) return documents
In this example, we have defined a route that fetches all documents from a MongoDB collection. The get_mongodb
function is used as a dependency to inject the MongoDB client into the route function.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
MongoDB integration with FastAPI using Motor
FastAPI provides built-in support for integrating with MongoDB using the Motor library. Motor is an asynchronous Python driver for MongoDB that allows you to interact with MongoDB databases using Python code.
To integrate MongoDB with FastAPI using Motor, you need to install the necessary dependencies:
pip install fastapi motor
Once you have installed the dependencies, you can start integrating MongoDB with FastAPI. Here is an example of how you can set up FastAPI with Motor for MongoDB:
First, import the necessary modules and create a Motor MotorClient
object:
from fastapi import FastAPI from motor.motor_asyncio import AsyncIOMotorClient app = FastAPI() client = AsyncIOMotorClient() @app.on_event("startup") async def startup_event(): app.mongodb = client["mydatabase"] @app.on_event("shutdown") async def shutdown_event(): client.close()
In this example, we have created a Motor MotorClient
object for connecting to a MongoDB database. The AsyncIOMotorClient
class is used to create an asynchronous MongoDB client. We have also defined startup and shutdown event handlers to connect and disconnect from the MongoDB database.
Next, you can define a route that interacts with the MongoDB database:
from fastapi import Depends from motor.motor_asyncio import AsyncIOMotorClient @app.get("/") async def index(client: AsyncIOMotorClient = Depends(get_mongodb)): collection = client["mycollection"] documents = await collection.find().to_list(length=None) return documents
In this example, we have defined a route that fetches all documents from a MongoDB collection. The get_mongodb
function is used as a dependency to inject the MongoDB client into the route function.
Popular third-party tools for FastAPI integration
FastAPI is a useful and modern web framework for building APIs with Python. While FastAPI provides many built-in features and functionalities, there are times when you may want to integrate third-party tools to enhance the capabilities of your FastAPI applications. In this section, we will explore some popular third-party tools that can be integrated with FastAPI.
1. Celery: Celery is a distributed task queue framework that allows you to offload time-consuming tasks to worker processes. By integrating Celery with FastAPI, you can handle long-running tasks asynchronously, improving the performance and scalability of your applications.
2. Redis: Redis is an in-memory data structure store that can be used as a database, cache, and message broker. By integrating Redis with FastAPI, you can improve the performance of your applications by caching frequently accessed data or by using Redis as a message broker for inter-process communication.
3. JWT (JSON Web Tokens): JWT is a standard for securely transmitting information between parties as a JSON object. By integrating JWT with FastAPI, you can implement authentication and authorization mechanisms in your applications, allowing you to secure your API endpoints and manage user sessions.
4. Pydantic: Pydantic is a data validation and settings management library that provides runtime validation and parsing of data structures. By integrating Pydantic with FastAPI, you can easily define and validate request and response models, improving the reliability and maintainability of your applications.
These are just a few examples of the popular third-party tools that can be integrated with FastAPI. By leveraging the capabilities of these tools, you can enhance the functionality and performance of your FastAPI applications.
Integrating Elasticsearch for advanced search functionalities in FastAPI
FastAPI is a useful web framework for building APIs with Python, and Elasticsearch is a useful search and analytics engine. By integrating Elasticsearch with FastAPI, you can add advanced search functionalities to your applications.
To integrate Elasticsearch with FastAPI, you can use the official Elasticsearch Python client library called “elasticsearch-py”. This library provides a high-level API for interacting with Elasticsearch from Python.
Here is an example of how you can integrate Elasticsearch with FastAPI:
First, install the necessary dependencies:
pip install fastapi elasticsearch
Next, create a new file called “main.py” and add the following code:
from fastapi import FastAPI from elasticsearch import Elasticsearch app = FastAPI() es = Elasticsearch() @app.get("/search") async def search(query: str): results = es.search(index="my_index", body={"query": {"match": {"content": query}}}) return results["hits"]["hits"]
In this example, we have defined a /search
endpoint that accepts a query
parameter. When a GET request is made to this endpoint, the search
function is executed. The function uses the Elasticsearch client to perform a search query on an index called “my_index”. The search results are then returned as the response.
Related Article: Converting Integer Scalar Arrays To Scalar Index In Python
Benefits of using SQLAlchemy for MySQL and PostgreSQL integration with FastAPI
SQLAlchemy is a useful Object-Relational Mapping (ORM) library for Python that provides a high-level API for interacting with databases. When integrating FastAPI with MySQL and PostgreSQL, using SQLAlchemy offers several benefits:
1. Simplified Database Operations: SQLAlchemy allows you to write database queries using Python code instead of raw SQL. This makes it easier to perform common database operations such as inserting, updating, and querying data. The use of Python code also makes your database operations more maintainable and less prone to SQL injection attacks.
2. Database Abstraction: SQLAlchemy provides a database abstraction layer that allows you to work with different database systems using a unified API. This means that you can switch between different database systems (such as MySQL and PostgreSQL) without having to change your application code. This provides flexibility and reduces the effort required to support multiple database systems.
3. ORM Features: SQLAlchemy provides advanced ORM features such as object tracking, lazy loading, and relationship management. These features allow you to work with your database data as Python objects, making it easier to write complex data models and relationships. SQLAlchemy also includes support for transactions, caching, and connection pooling, which can improve the performance and scalability of your applications.
4. Integration with FastAPI: SQLAlchemy integrates seamlessly with FastAPI, allowing you to easily connect your FastAPI applications to MySQL and PostgreSQL databases. FastAPI provides built-in support for dependency injection, which can be used to inject a SQLAlchemy session instance into your route functions. This allows you to perform database operations using the session, making your code more concise and readable.
Setting up FastAPI with databases using SQLAlchemy
FastAPI provides built-in support for integrating with databases using SQLAlchemy, a useful Object-Relational Mapping (ORM) library for Python. SQLAlchemy allows you to interact with databases using Python code, making it easier to manage and query your data. In this section, we will explore how to set up FastAPI with databases using SQLAlchemy.
To get started, you need to install the necessary dependencies:
pip install fastapi sqlalchemy
Once you have installed the dependencies, you can start setting up FastAPI with databases using SQLAlchemy. Here is an example of how you can do it:
First, import the necessary modules and create a SQLAlchemy Engine
object:
from fastapi import FastAPI from sqlalchemy import create_engine app = FastAPI() engine = create_engine("sqlite:///./mydatabase.db")
In this example, we have created a SQLAlchemy Engine
object for connecting to a SQLite database. You can replace the connection URL with the appropriate URL for your database system.
Next, you can define a SQLAlchemy Session
class and create a session instance for each request:
from fastapi import Depends from sqlalchemy.orm import Session from sqlalchemy.orm import sessionmaker # Define the Session class class SessionLocal: def __init__(self, engine): self.Session = sessionmaker(bind=engine) def __call__(self): session = self.Session() try: yield session finally: session.close() # Create a session instance for each request def get_db(): db = SessionLocal(engine) try: yield db finally: db.close() @app.get("/") async def index(db: Session = Depends(get_db)): # Perform database operations using the session ...
In this example, we have defined a SessionLocal
class that creates a SQLAlchemy Session
object for each request. The get_db
function is used as a dependency to inject the session instance into the route functions.
Using Motor for MongoDB integration with FastAPI
FastAPI provides built-in support for integrating with MongoDB using the Motor library. Motor is an asynchronous Python driver for MongoDB that allows you to interact with MongoDB databases using Python code.
To use Motor for MongoDB integration with FastAPI, you need to install the necessary dependencies:
pip install fastapi motor
Once you have installed the dependencies, you can start integrating Motor with FastAPI. Here is an example of how you can do it:
First, import the necessary modules and create a Motor MotorClient
object:
from fastapi import FastAPI from motor.motor_asyncio import AsyncIOMotorClient app = FastAPI() client = AsyncIOMotorClient() @app.on_event("startup") async def startup_event(): app.mongodb = client["mydatabase"] @app.on_event("shutdown") async def shutdown_event(): client.close()
In this example, we have created a Motor MotorClient
object for connecting to a MongoDB database. The AsyncIOMotorClient
class is used to create an asynchronous MongoDB client. We have also defined startup and shutdown event handlers to connect and disconnect from the MongoDB database.
Next, you can define a route that interacts with the MongoDB database:
from fastapi import Depends from motor.motor_asyncio import AsyncIOMotorClient @app.get("/") async def index(client: AsyncIOMotorClient = Depends(get_mongodb)): collection = client["mycollection"] documents = await collection.find().to_list(length=None) return documents
In this example, we have defined a route that fetches all documents from a MongoDB collection. The get_mongodb
function is used as a dependency to inject the MongoDB client into the route function.
Related Article: How To Convert A Tensor To Numpy Array In Tensorflow
Best practices for integrating third-party and open source tools with FastAPI
Integrating third-party and open-source tools with FastAPI can greatly enhance the capabilities and functionalities of your applications. However, it is important to follow best practices to ensure a smooth integration and maintainable codebase. In this section, we will explore some best practices for integrating third-party and open-source tools with FastAPI.
1. Read the Documentation: Before integrating any third-party or open-source tool with FastAPI, make sure to thoroughly read the documentation. Understanding the tool’s features, requirements, and APIs will help you make informed decisions and avoid common pitfalls.
2. Use Dependency Injection: FastAPI provides built-in support for dependency injection, allowing you to easily inject dependencies into your route functions. Use dependency injection to inject instances of third-party tools or libraries into your application. This promotes modularity and makes your code more testable and maintainable.
3. Version Compatibility: Ensure that the version of the third-party tool or library you are integrating with FastAPI is compatible with FastAPI and other dependencies. Incompatible versions can lead to unexpected behavior or errors.
4. Error Handling: Properly handle errors and exceptions that may occur during integration. Use try-except blocks or error handlers to gracefully handle errors and provide meaningful error messages to the users.
5. Configuration Management: Use a configuration management system to manage the configuration settings of the third-party tools or libraries. This allows you to easily modify the settings without modifying the code.
6. Use Abstractions: Whenever possible, use abstractions or wrappers to encapsulate the integration logic. This allows you to easily switch between different tools or libraries without affecting the rest of the codebase.
7. Test the Integration: Write comprehensive unit tests and integration tests to ensure that the integration works as expected. This helps catch any issues or bugs early on and ensures the stability and reliability of your application.
8. Monitor and Debug: Monitor the integration for any errors or performance issues. Use logging and monitoring tools to track the usage and performance of the integrated tools or libraries.
9. Stay Updated: Keep track of updates and new releases of the integrated tools or libraries. Updates may contain bug fixes, improvements, or new features that can enhance the functionality or security of your application.
Recommended resources and tutorials for integrating third-party tools with FastAPI
Integrating third-party tools with FastAPI can greatly enhance the capabilities and functionalities of your applications. If you are looking to learn more about integrating third-party tools with FastAPI, here are some recommended resources and tutorials:
1. FastAPI documentation: The official FastAPI documentation provides detailed information about the features and capabilities of FastAPI. It also contains examples and tutorials on how to integrate third-party tools and libraries with FastAPI.
2. FastAPI GitHub repository: The GitHub repository of FastAPI contains a wealth of information and resources. You can find examples, code snippets, and discussions related to integrating third-party tools with FastAPI.
3. PyPI: The Python Package Index (PyPI) is a repository of Python packages. You can search for specific tools or libraries that you want to integrate with FastAPI and find their documentation, installation instructions, and examples.
4. Stack Overflow: Stack Overflow is a popular question and answer website for software developers. You can search for specific integration challenges or issues you are facing and find answers and solutions provided by the community.
5. Official documentation and forums of the third-party tools: Most third-party tools have their own official documentation and forums. These resources often provide detailed information, examples, and best practices for integrating the tools with different frameworks, including FastAPI.
6. Online tutorials and blog posts: Many software developers and organizations publish tutorials and blog posts on integrating third-party tools with FastAPI. These resources often provide step-by-step instructions, code examples, and practical tips for integrating specific tools.
Example of integrating Elasticsearch for advanced search functionalities in FastAPI
In this example, we will demonstrate how to integrate Elasticsearch with FastAPI to add advanced search functionalities to your applications.
First, install the necessary dependencies:
pip install fastapi elasticsearch
Next, create a new file called “main.py” and add the following code:
from fastapi import FastAPI from elasticsearch import Elasticsearch app = FastAPI() es = Elasticsearch() @app.get("/search") async def search(query: str): results = es.search(index="my_index", body={"query": {"match": {"content": query}}}) return results["hits"]["hits"]
In this example, we have defined a /search
endpoint that accepts a query
parameter. When a GET request is made to this endpoint, the search
function is executed. The function uses the Elasticsearch client to perform a search query on an index called “my_index”. The search results are then returned as the response.
To test the integration, you can run the FastAPI application using the following command:
uvicorn main:app --reload
Once the application is running, you can make a GET request to the /search
endpoint with a query parameter. For example, you can use the following command:
curl "http://localhost:8000/search?query=example"
This will return the search results from Elasticsearch based on the query parameter.
Related Article: How to Normalize a Numpy Array to a Unit Vector in Python
Optimizing FastAPI performance with third-party and open source tools
FastAPI is a high-performance web framework for building APIs with Python. However, there are times when you may need to optimize the performance of your FastAPI applications even further. By leveraging third-party and open-source tools, you can enhance the performance of your FastAPI applications. In this section, we will explore some tools and techniques for optimizing FastAPI performance.
1. Load Balancers: Use load balancers to distribute incoming requests across multiple instances of your FastAPI application. Load balancers ensure that the workload is evenly distributed, improving response times and handling more concurrent requests.
2. Caching: Implement caching mechanisms to store frequently accessed data in memory. Tools like Redis or Memcached can be used as cache stores to improve the performance of read-heavy applications. By caching data, you can reduce the load on your database and improve response times.
3. Asynchronous Tasks: Offload time-consuming tasks to background processes using tools like Celery or RQ. By executing tasks asynchronously, your FastAPI application can respond to requests quickly, improving overall performance.
4. API Gateways: Use API gateways like Kong or Tyk to manage API traffic and provide additional capabilities such as rate limiting, authentication, and request/response transformation. API gateways can help optimize performance by reducing the load on your FastAPI application.
5. Request Profiling: Profile your FastAPI application to identify performance bottlenecks and areas for optimization. Tools like Py-Spy or cProfile can help you understand which parts of your code are consuming the most resources.
6. Performance Monitoring: Use monitoring tools like Prometheus or New Relic to monitor the performance of your FastAPI application in real-time. Monitoring metrics such as response times, CPU usage, and memory usage can help you identify performance issues and optimize accordingly.
7. Database Optimization: Optimize your database queries and schema design to improve performance. Use tools like SQLAlchemy’s query optimization features, database indexes, and query caching to reduce query response times.
8. Content Delivery Networks (CDNs): Use CDNs like Cloudflare or Akamai to cache and deliver static assets, reducing the load on your FastAPI application and improving response times for users located far away from your server.
Additional Resources
– Setting up FastAPI with MySQL and SQLAlchemy
– Using FastAPI with PostgreSQL