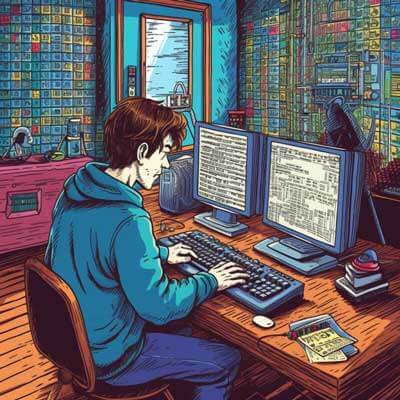
Table of Contents
In Python, there are multiple ways to remove whitespace from a string. This can be useful when processing user input, cleaning up data, or manipulating text. In this answer, we will explore two common methods for removing whitespace in a string using Python.
Method 1: Using the replace() function
Python provides a built-in string method called replace()
that can be used to remove specific characters or substrings from a string. By using this method with a space character as the first argument and an empty string as the second argument, we can effectively remove all whitespace from a given string.
Here is an example of how to use the replace()
function to remove whitespace from a string:
text = " Hello, World! " text = text.replace(" ", "") print(text)
Output:
Hello,World!
Explanation:
- We start with a string text
that contains whitespace at the beginning, end, and between words.
- We use the replace()
function to replace all occurrences of a space character " "
with an empty string ""
.
- The modified string is then assigned back to the text
variable.
- Finally, we print the modified string, which no longer contains any whitespace.
Related Article: How to Delete a Column from a Pandas Dataframe
Method 2: Using regular expressions
Regular expressions provide a powerful and flexible way to match and manipulate text patterns in Python. The re
module in Python's standard library provides functions and methods for working with regular expressions. We can use a regular expression pattern to match whitespace characters and remove them from a string.
Here is an example of how to use regular expressions to remove whitespace from a string:
import re text = " Hello, World! " text = re.sub(r"\s", "", text) print(text)
Output:
Hello,World!
Explanation:
- We import the re
module to work with regular expressions in Python.
- Similar to the previous method, our initial string text
contains whitespace at various positions.
- We use the re.sub()
function to substitute matches of a regular expression pattern with a replacement string. In this case, the pattern "\s"
matches any whitespace character.
- The replacement string ""
is used to remove the matched whitespace characters.
- The modified string is then assigned back to the text
variable.
- Finally, we print the modified string, which no longer contains any whitespace.
Why would someone need to remove whitespace from a string?
There can be various reasons why someone would want to remove whitespace from a string in Python. Some potential reasons include:
1. Data Cleaning: When working with data, it is common to encounter strings that contain unnecessary whitespace. Removing this whitespace ensures data consistency and helps in further processing or analysis.
2. User Input Processing: When handling user input, it is essential to sanitize and normalize the input data. Removing whitespace from user-provided strings helps eliminate any unintentional leading or trailing spaces that may cause issues.
3. String Manipulation: In some cases, string manipulation tasks may require removing whitespace to meet specific formatting requirements or to perform operations that depend on whitespace-free strings.
Suggestions and alternative ideas
While the methods mentioned above are commonly used to remove whitespace from a string in Python, here are a few alternative ideas and suggestions:
1. Strip Leading and Trailing Whitespace: If you only need to remove leading and trailing whitespace from a string, you can use the strip()
method. This method removes all leading and trailing characters specified in the argument, which, in this case, would be whitespace.
text = " Hello, World! " text = text.strip() print(text)
Output:
Hello, World!
Explanation:
- The strip()
method removes leading and trailing whitespace from the string text
but preserves any whitespace between words.
2. Preserve Single Spaces: If you want to remove consecutive whitespace characters but preserve single spaces between words, you can use regular expressions with the pattern "\s+"
.
import re text = " Hello, World! " text = re.sub(r"\s+", " ", text) print(text)
Output:
Hello, World!
Explanation:
- The regular expression pattern "\s+"
matches one or more consecutive whitespace characters.
- The re.sub()
function replaces all matches of the pattern with a single space character, effectively collapsing consecutive whitespace into a single space.
Related Article: How to Import Files From a Different Folder in Python
Best practices
When removing whitespace from a string in Python, consider the following best practices:
1. Use the most appropriate method: Depending on your specific requirements, choose the method that best fits the context of your problem. If you only need to remove leading and trailing whitespace, use the strip()
method. If you want to remove all whitespace, including spaces between words, either the replace()
method or regular expressions can be used.
2. Consider performance implications: If you are working with large amounts of data or need to perform the whitespace removal operation frequently, consider the performance implications of the chosen method. In some cases, using the replace()
method might be more efficient than regular expressions, especially if the pattern is simple.
3. Test and validate: Before using whitespace removal techniques on production data or user input, thoroughly test and validate the behavior of your chosen method. Consider edge cases, such as strings with no whitespace or strings that consist entirely of whitespace, to ensure the desired results.
4. Document your code: When removing whitespace from a string, especially if the operation is part of a larger codebase or project, consider adding comments or documentation to explain the rationale and expected behavior of the code. This helps other developers understand the purpose of the whitespace removal and ensures maintainability.