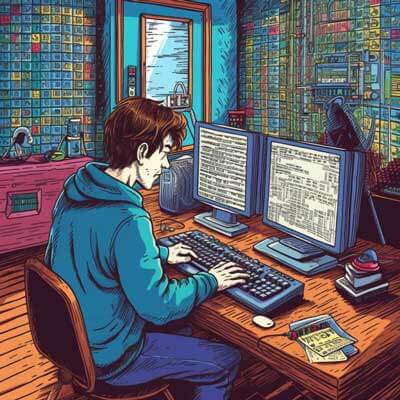
- Troubleshooting Common Pip Install Errors
- Understanding Dependency Resolution in Python Packages
- The Importance of Virtual Environments for Package Installation
- Exploring Alternative Python Package Managers
- Managing Different Versions of Python Packages
- Resolving Conflicts Between Python Packages
- Troubleshooting Pip Install Not Working
- Common Issues and Solutions for Installing FastAPI
- Additional Resources
Troubleshooting Common Pip Install Errors
When working with Python packages, using pip to install and manage dependencies is a common practice. However, there are times when the pip install command fails, leading to frustration and confusion. In this section, we will explore some common pip install errors and how to troubleshoot them.
One common error is the “Could not find a version that satisfies the requirement” message. This error occurs when pip cannot find a suitable version of the package you are trying to install. To troubleshoot this error, you can try specifying a different version of the package or checking if the package is available in the Python Package Index (PyPI).
$ pip install fastapi==0.65.2
Another common error is the “No matching distribution found” message. This error occurs when pip cannot find a distribution of the package that matches your system’s architecture and Python version. To troubleshoot this error, you can try upgrading pip to the latest version or checking if the package is compatible with your system.
$ pip install --upgrade pip
Related Article: Executing API Calls with FastAPI
Understanding Dependency Resolution in Python Packages
Dependency resolution is a critical aspect of managing Python packages. When you install a package using pip, it automatically resolves and installs the dependencies required by that package. However, there are cases where the dependency resolution process can fail, leading to installation errors.
To understand dependency resolution, let’s consider an example where you want to install FastAPI, a popular web framework. FastAPI has its own set of dependencies, such as pydantic and starlette. When you run the pip install fastapi command, pip will first check if these dependencies are already installed. If not, pip will attempt to install them before installing FastAPI.
However, if there are conflicting dependencies or if a compatible version of a dependency cannot be found, the dependency resolution process will fail. In such cases, you may encounter errors like “Could not find a version that satisfies the requirement” or “No matching distribution found”.
The Importance of Virtual Environments for Package Installation
Virtual environments are a crucial tool for managing Python packages and their dependencies. They provide an isolated environment where you can install and test packages without affecting the system-wide Python installation.
Creating a virtual environment is easy using the virtualenv package. First, install virtualenv using pip:
$ pip install virtualenv
Once installed, you can create a new virtual environment by running the following command:
$ virtualenv myenv
This will create a new directory named “myenv” that contains the isolated Python environment. To activate the virtual environment, use the following command:
$ source myenv/bin/activate
With the virtual environment activated, you can now install packages without affecting the system-wide Python installation. This helps prevent conflicts between different versions of packages and ensures that your project’s dependencies are isolated.
Exploring Alternative Python Package Managers
While pip is the most widely used package manager for Python, there are alternative package managers available that offer additional features and capabilities. These alternative package managers can be helpful in troubleshooting pip install failures and managing packages more effectively.
One such alternative is conda, which is part of the Anaconda distribution. Conda not only manages Python packages but also supports packages from other programming languages and non-Python dependencies. To use conda, you need to install Anaconda or Miniconda, which includes conda as the default package manager.
$ conda install fastapi
Another alternative is poetry, a modern dependency management tool for Python. Poetry simplifies the process of managing dependencies and project environments by providing a declarative approach. Poetry also allows for easily specifying dependencies and their versions in a pyproject.toml file.
$ poetry add fastapi
Related Article: How to Add an Additional Function to a FastAPI Loop
Managing Different Versions of Python Packages
Managing different versions of Python packages is crucial for ensuring the stability and compatibility of your project. However, it can also introduce challenges when installing packages using pip.
To install a specific version of a package, you can use the == operator followed by the version number. For example, to install version 0.65.2 of FastAPI, you can run the following command:
$ pip install fastapi==0.65.2
Alternatively, you can use the >= operator followed by the minimum required version. This allows pip to install the latest compatible version of the package. For example, to install any version of FastAPI that is greater than or equal to 0.65.2, you can run the following command:
$ pip install fastapi>=0.65.2
Resolving Conflicts Between Python Packages
Conflicts between Python packages can occur when different packages have conflicting dependencies or when different versions of the same package are required by different packages. Resolving these conflicts is essential to ensure the smooth installation and functioning of your project.
One way to resolve conflicts is by using a dependency resolver like pip-tools. Pip-tools provides a higher level of control over dependency resolution by allowing you to define a requirements.txt file that specifies the exact versions of packages and their dependencies. You can then use pip-sync to install the exact versions specified in the requirements.txt file.
To use pip-tools, first, install it using pip:
$ pip install pip-tools
Next, create a requirements.in file where you can specify the packages and their versions:
fastapi==0.65.2 pydantic==1.8.2 starlette==0.14.2
Then, use pip-compile to generate the requirements.txt file:
$ pip-compile requirements.in
Finally, use pip-sync to install the exact versions specified in the requirements.txt file:
$ pip-sync requirements.txt
Troubleshooting Pip Install Not Working
If the pip install command is not working at all, there might be an issue with your Python installation or environment configuration. Here are some steps you can take to troubleshoot and resolve the issue:
1. Check if pip is installed: Run the following command to verify if pip is installed correctly:
$ pip --version
If the command returns an error or does not display the version number, it means that pip is not installed. In such cases, you need to install pip using the appropriate method for your operating system.
2. Verify the Python installation: Ensure that Python is installed correctly on your system and that the Python executable is added to the system’s PATH environment variable. You can check the Python version by running the following command:
$ python --version
If the command returns an error or does not display the version number, it means that Python is not installed or configured correctly. Refer to the Python documentation for instructions on how to install and configure Python.
3. Check the system’s PATH environment variable: Ensure that the directory containing the pip executable is added to the system’s PATH environment variable. This allows the system to find the pip command when it is executed. You can check the PATH variable by running the following command:
$ echo $PATH
If the directory containing the pip executable is not listed in the output, you need to add it to the PATH variable. Refer to your operating system’s documentation for instructions on how to modify the PATH variable.
4. Update pip: If pip is installed but not working, try updating it to the latest version using the following command:
$ pip install --upgrade pip
This will ensure that you have the latest version of pip, which may include bug fixes and improvements.
If none of the above steps resolve the issue, it is recommended to seek help from the Python community or consult the official Python documentation for further assistance.
Related Article: How to Integrate FastAPI with PostgreSQL
Common Issues and Solutions for Installing FastAPI
FastAPI is a useful and efficient web framework for building APIs with Python. However, like any other package, it can sometimes pose challenges during installation. Here are some common issues that you may encounter when installing FastAPI and their possible solutions:
1. “Could not find a version that satisfies the requirement fastapi”: This error occurs when pip cannot find a compatible version of FastAPI. To resolve this issue, make sure you have specified the correct version of FastAPI in the pip install command, or try upgrading pip to the latest version.
2. “No matching distribution found for fastapi”: This error occurs when pip cannot find a distribution of FastAPI that matches your system’s architecture and Python version. To resolve this issue, make sure you are using the correct version of Python and check if FastAPI is compatible with your system.
3. “Command ‘uvicorn’ not found”: This error occurs when the Uvicorn server, which is used by FastAPI, is not installed or not accessible. To resolve this issue, make sure you have installed Uvicorn using pip before running your FastAPI application.
4. “ImportError: No module named ‘fastapi'”: This error occurs when the FastAPI module is not installed or not accessible. To resolve this issue, make sure you have installed FastAPI using pip and that you are importing it correctly in your Python code.
5. “ModuleNotFoundError: No module named ‘pydantic'”: This error occurs when the Pydantic module, which is a dependency of FastAPI, is not installed or not accessible. To resolve this issue, make sure you have installed Pydantic using pip before installing FastAPI.
Additional Resources
– Python Package Index (PyPI)
– FastAPI GitHub Repository
– FastAPI – Failed Installation Solution