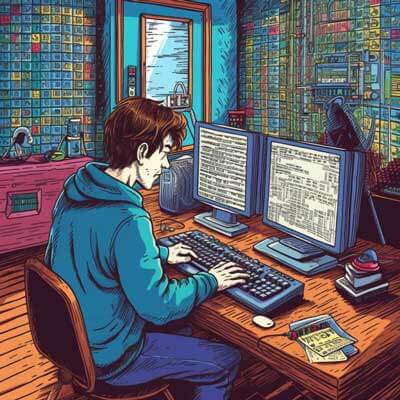
- Django Authentication
- Enterprise Authentication
- Implementing Single Sign-On with SAML in Django
- Implementing OAuth2 Authentication in Django for Enterprise Use
- Enterprise Authorization
- Implementing Role-Based Access Control in Django Apps
- Enterprise Integration
- Integrating Django with Okta for Enterprise Identity Management
- Enterprise Search
- Integrating Elasticsearch for Enterprise Search in Django
- Enterprise Logging
- Best Practices for Auditing and Logging in Django Apps
- Compliance in Django Apps
- Ensuring Compliance in Django Applications
- Enterprise Single Sign-On
- Implementing Single Sign-On with SAML, OAuth2, and OIDC
- Implementing Single Sign-On with SAML in Django
- Implementing OAuth2 Authentication in Django for Enterprise Use
- Enterprise Identity Providers
- Available Options for Enterprise Identity Providers Integration with Django
- Implementing Permission Management in Django for Enterprise Applications
- Benefits of Using OIDC for Single Sign-On in Django
- Additional Resources
Django Authentication
Django provides a robust authentication system out of the box, allowing developers to easily authenticate users, handle sessions, and manage user permissions. The authentication system includes features such as user registration, login, logout, and password reset.
To enable authentication in a Django app, you need to configure the authentication backends and define the user model. By default, Django uses the built-in User model, but you can also create a custom user model if you have additional user fields or require specific functionality.
Here’s an example of setting up authentication in a Django app:
# settings.py AUTHENTICATION_BACKENDS = [ 'django.contrib.auth.backends.ModelBackend', # Add any additional authentication backends here ] AUTH_USER_MODEL = 'myapp.CustomUser'
The AUTHENTICATION_BACKENDS
setting specifies the authentication backends to use. In the example above, we’re using the default ModelBackend
, which authenticates against the database. You can add additional backends for different authentication methods, such as LDAP or external identity providers.
The AUTH_USER_MODEL
setting allows you to specify a custom user model. In this example, we’re using a CustomUser
model defined in the myapp
app.
Related Article: 16 Amazing Python Libraries You Can Use Now
Enterprise Authentication
Enterprise authentication refers to the process of authenticating users against an enterprise identity provider (IdP) rather than using the built-in authentication system. This allows organizations to centralize user management and leverage existing IdP infrastructure.
There are several protocols commonly used for enterprise authentication, including SAML, OAuth2, and OpenID Connect (OIDC). Let’s explore each of these protocols and how they can be implemented in Django.
Implementing Single Sign-On with SAML in Django
SAML (Security Assertion Markup Language) is a widely adopted protocol for exchanging authentication and authorization data between an identity provider and a service provider. It enables single sign-on (SSO) functionality, allowing users to authenticate once and access multiple applications without having to re-enter their credentials.
To implement SAML-based SSO in Django, you can use the djangosaml2
library, which provides a Django integration for SAML. Here’s an example of how to configure SAML authentication in Django:
# settings.py INSTALLED_APPS = [ 'djangosaml2', # Other installed apps ] AUTHENTICATION_BACKENDS = [ 'django.contrib.auth.backends.ModelBackend', 'djangosaml2.backends.Saml2Backend', # Other authentication backends ] SAML2_AUTH = { 'DEFAULT_NEXT_URL': '/', 'PLUGINS': { 'saml2_local': { 'ACTIVE': True, 'ATTRIBUTES_MAP': { 'email': 'Email', 'first_name': 'FirstName', 'last_name': 'LastName', }, } }, }
In this example, we’ve added the djangosaml2
app to the INSTALLED_APPS
setting and included the Saml2Backend
in the AUTHENTICATION_BACKENDS
setting. We’ve also configured the SAML2_AUTH
setting with the necessary parameters, such as the default URL to redirect to after successful authentication and the attribute mapping for user data.
Implementing OAuth2 Authentication in Django for Enterprise Use
OAuth2 is an industry-standard protocol for authorization, allowing users to grant access to their data to third-party applications without sharing their credentials. It is often used for authentication in web and mobile applications, including enterprise applications.
To implement OAuth2 authentication in Django, you can use the django-oauth-toolkit
library, which provides an implementation of the OAuth2 server specification. Here’s an example of how to configure OAuth2 authentication in Django:
# settings.py INSTALLED_APPS = [ 'oauth2_provider', # Other installed apps ] AUTHENTICATION_BACKENDS = [ 'django.contrib.auth.backends.ModelBackend', 'oauth2_provider.backends.OAuth2Backend', # Other authentication backends ] OAUTH2_PROVIDER = { 'SCOPES': { 'read': 'Read access', 'write': 'Write access', } }
In this example, we’ve added the oauth2_provider
app to the INSTALLED_APPS
setting and included the OAuth2Backend
in the AUTHENTICATION_BACKENDS
setting. We’ve also configured the OAUTH2_PROVIDER
setting with the desired OAuth2 scopes for read and write access.
Related Article: Database Query Optimization in Django: Boosting Performance for Your Web Apps
Enterprise Authorization
In addition to authentication, enterprise applications often require fine-grained authorization to control access to different resources and functionalities based on user roles and permissions. Django provides a flexible authorization system that allows you to define and manage permissions at a granular level.
Implementing Role-Based Access Control in Django Apps
Role-Based Access Control (RBAC) is a widely used authorization model that assigns permissions to roles, and then assigns roles to users. This approach simplifies permission management by grouping users with similar access requirements under roles.
In Django, you can implement RBAC using the built-in django.contrib.auth
module. Here’s an example of how to define roles and assign permissions to them:
from django.contrib.auth.models import Group, Permission from django.contrib.contenttypes.models import ContentType # Create the roles admin_group, _ = Group.objects.get_or_create(name='Admin') manager_group, _ = Group.objects.get_or_create(name='Manager') user_group, _ = Group.objects.get_or_create(name='User') # Define the permissions content_type = ContentType.objects.get_for_model(MyModel) admin_permissions = Permission.objects.filter(content_type=content_type) manager_permissions = Permission.objects.filter(content_type=content_type, codename__in=['change_mymodel', 'delete_mymodel']) # Assign permissions to roles admin_group.permissions.set(admin_permissions) manager_group.permissions.set(manager_permissions)
In this example, we’re creating three groups: Admin
, Manager
, and User
. We’re also defining permissions based on a model (MyModel
) and assigning them to the respective groups.
To check if a user has a specific permission, you can use the user.has_perm()
method:
if user.has_perm('myapp.change_mymodel'): # User has permission to change MyModel
Enterprise Integration
Enterprise applications often need to integrate with external systems and services, such as enterprise identity providers, enterprise search engines, and logging and auditing tools. Django provides a flexible architecture and various libraries to facilitate these integrations.
Related Article: Django 4 Best Practices: Leveraging Asynchronous Handlers for Class-Based Views
Integrating Django with Okta for Enterprise Identity Management
Okta is a popular cloud-based identity and access management (IAM) platform that provides enterprise-grade authentication and user management capabilities. By integrating Django with Okta, you can leverage Okta’s user management features and enable SSO for your Django app.
To integrate Django with Okta, you can use the django-okta-auth
library, which provides an Okta authentication backend for Django. Here’s an example of how to configure the Okta authentication backend:
# settings.py INSTALLED_APPS = [ 'okta_oauth2_backend', # Other installed apps ] AUTHENTICATION_BACKENDS = [ 'django.contrib.auth.backends.ModelBackend', 'okta_oauth2_backend.OktaBackend', # Other authentication backends ] OKTA_OAUTH2_CLIENT_ID = 'your-okta-client-id' OKTA_OAUTH2_CLIENT_SECRET = 'your-okta-client-secret' OKTA_OAUTH2_ORG_URL = 'https://your-okta-org-url'
In this example, we’ve added the okta_oauth2_backend
app to the INSTALLED_APPS
setting and included the OktaBackend
in the AUTHENTICATION_BACKENDS
setting. We’ve also configured the necessary Okta OAuth2 parameters, such as the client ID, client secret, and Okta organization URL.
Enterprise Search
Enterprise applications often require advanced search capabilities to efficiently retrieve and analyze data. Django provides integration with popular search engines like Elasticsearch, allowing you to implement useful search functionality in your Django app.
Integrating Elasticsearch for Enterprise Search in Django
Elasticsearch is a distributed, RESTful search and analytics engine that provides fast and scalable full-text search capabilities. By integrating Elasticsearch with Django, you can leverage its advanced search features, such as fuzzy matching, faceted search, and relevance scoring.
To integrate Elasticsearch with Django, you can use the django-elasticsearch-dsl
library, which provides a high-level API for interacting with Elasticsearch. Here’s an example of how to configure Elasticsearch integration in Django:
# settings.py INSTALLED_APPS = [ 'django_elasticsearch_dsl', # Other installed apps ] ELASTICSEARCH_DSL = { 'default': { 'hosts': 'localhost:9200', }, } ELASTICSEARCH_INDEX_NAMES = { 'myapp.documents.MyDocument': 'myapp', }
In this example, we’ve added the django_elasticsearch_dsl
app to the INSTALLED_APPS
setting and configured the Elasticsearch connection details. We’ve also defined the index names for our Elasticsearch documents.
To define an Elasticsearch document in Django, you can use the Document
class provided by django_elasticsearch_dsl
. Here’s an example of how to define a document:
from django_elasticsearch_dsl import Document, fields from myapp.models import MyModel class MyDocument(Document): class Index: name = 'myapp' settings = { 'number_of_shards': 1, 'number_of_replicas': 0, } field1 = fields.TextField(attr='field1') field2 = fields.TextField(attr='field2') class Django: model = MyModel
In this example, we’re defining a MyDocument
document that maps to the MyModel
model. We’re also defining two fields, field1
and field2
, which are indexed in Elasticsearch.
Related Article: String Comparison in Python: Best Practices and Techniques
Enterprise Logging
Logging is a critical aspect of enterprise applications as it allows developers and system administrators to monitor and troubleshoot issues. Django provides a flexible logging framework that allows you to configure loggers, handlers, and formatters to suit your application’s needs.
Best Practices for Auditing and Logging in Django Apps
When it comes to logging in Django apps, there are several best practices to follow:
1. Use a dedicated logger for each Django app or module to separate log messages and make it easier to filter and analyze logs.
import logging logger = logging.getLogger('myapp')
2. Configure different log levels for different environments (e.g., development, staging, production) to control the verbosity of logs.
# settings.py LOGGING = { 'version': 1, 'disable_existing_loggers': False, 'handlers': { 'console': { 'class': 'logging.StreamHandler', }, }, 'root': { 'handlers': ['console'], 'level': 'INFO', }, 'loggers': { 'myapp': { 'handlers': ['console'], 'level': 'DEBUG', }, }, }
In this example, we’re configuring the root logger to log at the INFO
level and the myapp
logger to log at the DEBUG
level.
3. Use structured logging with JSON or other formats that allow easy parsing and analysis of log messages.
import logging import json logger = logging.getLogger('myapp') def log_event(event_type, message): logger.info(json.dumps({'event_type': event_type, 'message': message}))
In this example, we’re logging events as JSON objects, which can be easily parsed and analyzed by log management tools.
4. Include contextual information in log messages, such as request IDs, user IDs, and timestamps, to aid in troubleshooting and analysis.
import logging import uuid logger = logging.getLogger('myapp') def log_event(event_type, message): logger.info(f'[{uuid.uuid4()}] [{event_type}] {message}')
In this example, we’re including a unique request ID in each log message.
Compliance in Django Apps
Compliance is a crucial aspect of enterprise applications, especially in industries with strict regulations like healthcare, finance, and government. Django provides various features and libraries that can help you ensure compliance in your Django apps.
Related Article: How to Replace Strings in Python using re.sub
Ensuring Compliance in Django Applications
To ensure compliance in Django applications, you can follow these best practices:
1. Secure data storage: Use encryption and secure storage mechanisms for sensitive data, such as passwords and personally identifiable information (PII).
2. Access control: Implement fine-grained access control mechanisms, such as RBAC, to control access to sensitive data and functionalities.
3. Audit logging: Implement comprehensive audit logging to track user actions, system events, and data access. Store logs securely and ensure they are tamper-proof.
4. User consent and data privacy: Implement mechanisms to obtain user consent for data collection and processing. Ensure compliance with data privacy regulations, such as GDPR.
5. Security testing: Conduct regular security testing, including vulnerability scanning, penetration testing, and code review, to identify and address potential security issues.
6. Compliance documentation: Maintain clear and up-to-date documentation of compliance requirements, controls, and procedures. Regularly review and update the documentation as regulations evolve.
Enterprise Single Sign-On
Single Sign-On (SSO) is a mechanism that allows users to authenticate once and access multiple applications or services without having to re-enter their credentials. SSO simplifies the user experience and improves security by reducing the need for multiple passwords.
Implementing Single Sign-On with SAML, OAuth2, and OIDC
Django provides support for implementing SSO with various protocols, including SAML, OAuth2, and OpenID Connect (OIDC). Let’s explore how you can implement SSO with these protocols in Django.
Related Article: How to Work with CSV Files in Python: An Advanced Guide
Implementing Single Sign-On with SAML in Django
SAML (Security Assertion Markup Language) is a widely adopted protocol for implementing SSO. To implement SSO with SAML in Django, you can use the djangosaml2
library, which provides a Django integration for SAML.
Refer to the “Implementing Single Sign-On with SAML in Django” section in the “Enterprise Authentication” subsection for a detailed example and code snippets.
Implementing OAuth2 Authentication in Django for Enterprise Use
OAuth2 is an industry-standard protocol for authorization, but it can also be used for implementing SSO. To implement SSO with OAuth2 in Django, you can use the django-oauth-toolkit
library, which provides an OAuth2 server implementation for Django.
Refer to the “Implementing OAuth2 Authentication in Django for Enterprise Use” section in the “Enterprise Authentication” subsection for a detailed example and code snippets.
Enterprise Identity Providers
Enterprise identity providers (IdPs) are systems that manage user authentication and authorization for enterprise applications. Integrating Django with enterprise IdPs allows you to leverage existing user management infrastructure and enable SSO for your Django app.
Related Article: How to Work with Lists and Arrays in Python
Available Options for Enterprise Identity Providers Integration with Django
Django provides integration options for various enterprise identity providers, including:
– Okta: Use the django-okta-auth
library, as described in the “Integrating Django with Okta for Enterprise Identity Management” section in the “Enterprise Integration” subsection.
– Azure AD: Use the django-auth-adfs
library, which provides an integration with Azure Active Directory Federation Services (ADFS).
Refer to the respective library documentation for detailed instructions on integrating Django with these identity providers.
Implementing Permission Management in Django for Enterprise Applications
Permission management is crucial in enterprise applications to control access to different resources and functionalities based on user roles and permissions. In Django, you can implement permission management using the built-in django.contrib.auth
module.
Refer to the “Implementing Role-Based Access Control in Django Apps” section in the “Enterprise Authorization” subsection for a detailed example and code snippets.
Benefits of Using OIDC for Single Sign-On in Django
OpenID Connect (OIDC) is an extension of OAuth2 that provides identity verification capabilities, making it ideal for implementing SSO. OIDC offers several benefits for SSO in Django:
1. Standardized protocol: OIDC is an industry-standard protocol that is widely supported by identity providers and service providers, ensuring interoperability and ease of integration.
2. User attributes and claims: OIDC allows the exchange of user attributes and claims, providing additional information about the user during authentication.
3. Modern authentication features: OIDC supports modern authentication features such as multi-factor authentication (MFA) and identity federation, enhancing the security and flexibility of SSO implementations.
4. OAuth2 compatibility: OIDC builds on top of OAuth2, leveraging its authorization capabilities while adding identity verification features.
Related Article: How to Use Switch Statements in Python
Additional Resources
– Django Authentication Tutorial
– Implementing Django Authentication for Enterprise Applications
– Securing Django Apps in an Enterprise Environment