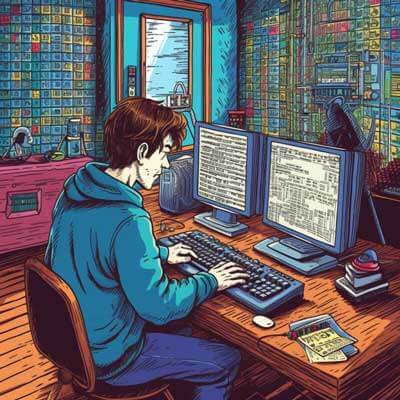
Table of Contents
Answer 1: Using a List and Sorting it Manually
If you need to manage a collection of elements in Java without using a SortedList, you can achieve this by using a regular List and sorting it manually whenever needed. Here's how you can do it:
1. Declare and instantiate a List object to store your elements. For example, let's create a List of integers:
List<Integer> myList = new ArrayList<>();
2. Add elements to the list using the add()
method. For example:
myList.add(5); myList.add(2); myList.add(8);
3. Whenever you need the elements to be sorted, you can use the Collections.sort()
method to sort the List in ascending order. For example:
Collections.sort(myList);
4. If you need to sort the List in descending order, you can create a custom Comparator and pass it as a second argument to the Collections.sort()
method. Here's an example:
Collections.sort(myList, Collections.reverseOrder());
5. You can now retrieve the sorted elements from the List using the regular List methods like get()
or by iterating over the List using a loop. For example:
for (Integer element : myList) { System.out.println(element); }
It's important to note that sorting the List manually whenever needed can have performance implications, especially if the List is large and requires frequent sorting. In such cases, using a SortedList implementation like TreeSet
or PriorityQueue
might be a better choice.
Related Article: How to Work with Java Generics & The Method Class Interface
Answer 2: Using a TreeSet
Related Article: How to Find the Max Value of an Integer in Java
Another way to manage a sorted collection of elements in Java is by using a TreeSet. TreeSet is an implementation of the SortedSet interface, which automatically maintains the elements in sorted order. Here's how you can use a TreeSet:
1. Declare and instantiate a TreeSet object to store your elements. For example, let's create a TreeSet of strings:
Set<String> mySet = new TreeSet<>();
2. Add elements to the TreeSet using the add()
method. For example:
mySet.add("apple"); mySet.add("banana"); mySet.add("cherry");
3. The TreeSet will automatically sort the elements in ascending order based on their natural ordering (e.g., lexicographically for strings). You can retrieve the sorted elements from the TreeSet using the regular Set methods like contains()
or by iterating over the Set using a loop. For example:
for (String element : mySet) { System.out.println(element); }
4. If you need to sort elements in a custom order, you can provide a custom Comparator when instantiating the TreeSet. The Comparator will be used to determine the order of the elements. Here's an example:
Set<Integer> mySet = new TreeSet<>(Comparator.reverseOrder());
In this example, the elements will be sorted in descending order.
Using a TreeSet can be more efficient than sorting a List manually whenever needed because it maintains the sorted order automatically. However, it's important to note that TreeSet has some performance implications, such as slower insertion and removal compared to other Set implementations like HashSet.