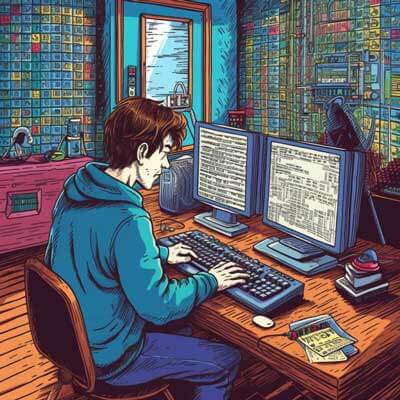
- Introduction
- Comparison of Redis and MongoDB
- Use Cases for Redis
- Use Cases for MongoDB
- Best Practices for Using Redis
- Best Practices for Using MongoDB
- Real World Examples of Redis Implementations
- Real World Examples of MongoDB Implementations
- Performance Considerations for Redis
- Performance Considerations for MongoDB
- Advanced Techniques for Redis
- Advanced Techniques for MongoDB
- Code Snippet Ideas for Redis
- Code Snippet Ideas for MongoDB
- Error Handling in Redis
- Error Handling in MongoDB
Introduction
In the world of NoSQL databases, Redis and MongoDB are two popular choices. While both databases fall under the NoSQL category, they have distinct features and use cases. This article aims to provide a detailed comparison between Redis and MongoDB, highlighting their differences, strengths, and best practices.
Related Article: Tutorial on AWS Elasticache Redis Implementation
Comparison of Redis and MongoDB
Redis and MongoDB differ in their data models, performance characteristics, and use cases. Redis is an in-memory data store that excels in caching and real-time applications, while MongoDB is a document-oriented database that provides flexibility and scalability. Let’s dive deeper into their differences and compare their features through code snippets.
Redis Code Snippet:
SET mykey "Hello Redis" GET mykey
MongoDB Code Snippet:
db.users.insertOne({ name: "John", age: 25 }) db.users.findOne({ name: "John" })
Use Cases for Redis
Redis is widely used in various scenarios that require fast data access and caching. Some common use cases for Redis include:
1. Session Management: Redis can store session data in memory, allowing for quick retrieval and management of user sessions.
Redis Code Snippet:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Set session data r.set('session:user_id', '12345') r.expire('session:user_id', 3600) # Set an expiration time of 1 hour # Retrieve session data user_id = r.get('session:user_id')
2. Real-time Analytics: Redis provides data structures like sorted sets and streams that enable real-time analytics and leaderboard functionality.
Redis Code Snippet:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Add user scores to sorted set r.zadd('leaderboard', {'user1': 100, 'user2': 200, 'user3': 150}) # Retrieve leaderboard leaderboard = r.zrevrange('leaderboard', 0, -1, withscores=True)
Use Cases for MongoDB
MongoDB’s flexible document model makes it suitable for a wide range of use cases. Some common use cases for MongoDB include:
1. Content Management Systems: MongoDB’s schema-less nature allows for dynamic content storage, making it a good choice for building content management systems.
MongoDB Code Snippet:
db.articles.insertOne({ title: "Introduction to MongoDB", content: "MongoDB is a NoSQL database..." }) db.articles.find({ title: "Introduction to MongoDB" })
2. Internet of Things (IoT): MongoDB’s scalability and ability to handle large amounts of data make it a popular choice for IoT applications.
MongoDB Code Snippet:
db.sensors.insertOne({ sensorId: "1234", temperature: 25.5, humidity: 60.2 }) db.sensors.find({ sensorId: "1234" })
Related Article: Leveraging Redis for Caching Frequently Used Queries
Best Practices for Using Redis
To make the most out of Redis, consider the following best practices:
1. Data Partitioning: Distribute data across multiple Redis instances using sharding techniques to improve performance and handle larger datasets.
2. Expire Keys: Set expiration times for keys to ensure the automatic removal of stale data and prevent memory bloat.
Best Practices for Using MongoDB
When working with MongoDB, keep the following best practices in mind:
1. Indexing: Create appropriate indexes to improve query performance and ensure efficient data retrieval.
2. Sharding: Plan for sharding in advance to distribute data across multiple servers and handle increased data volume and traffic.
Real World Examples of Redis Implementations
Redis has been successfully implemented in various real-world scenarios. Here are two examples:
1. Caching Layer: Redis is often used as a caching layer to speed up access to frequently accessed data in web applications.
2. Message Broker: Redis can act as a message broker in distributed systems, facilitating communication between different components.
Related Article: Analyzing Redis Query Rate per Second with Sidekiq
Real World Examples of MongoDB Implementations
MongoDB has found its place in many real-world applications. Let’s explore two examples:
1. E-commerce Platforms: MongoDB’s flexible document model allows for easy product catalog management and efficient handling of large product volumes.
2. Social Media Applications: MongoDB’s scalability and real-time capabilities make it suitable for building social media platforms that handle high traffic and dynamic data.
Performance Considerations for Redis
While Redis offers exceptional performance, it’s essential to consider the following factors for optimal performance:
1. Memory Usage: Redis stores data in memory, so ensure you have enough memory to accommodate your dataset.
2. Persistence Options: Choose the appropriate persistence option based on your use case, considering the trade-off between performance and data durability.
Performance Considerations for MongoDB
To achieve optimal performance with MongoDB, keep the following considerations in mind:
1. Indexing Strategies: Create indexes based on query patterns to speed up data retrieval and improve overall performance.
2. Hardware Selection: Choose appropriate hardware configurations, including high-speed storage and sufficient memory, to support MongoDB’s performance requirements.
Related Article: How to use Redis with Laravel and PHP
Advanced Techniques for Redis
Redis offers advanced features and techniques to solve complex problems. Here are a couple of examples:
1. Pub/Sub Messaging: Redis supports publish/subscribe messaging, enabling real-time communication between different components of a system.
Redis Code Snippet:
import redis # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Subscribe to a channel pubsub = r.pubsub() pubsub.subscribe('channel') # Receive messages for message in pubsub.listen(): print(message)
2. Lua Scripting: Redis allows executing Lua scripts on the server-side, enabling atomic and complex operations.
Redis Code Snippet:
EVAL "return redis.call('GET', KEYS[1])" 1 mykey
Advanced Techniques for MongoDB
MongoDB provides advanced techniques for data manipulation and analysis. Let’s explore a couple of them:
1. Aggregation Pipeline: MongoDB’s aggregation framework allows for complex data transformations and analysis, including grouping, filtering, and computing derived values.
MongoDB Code Snippet:
db.sales.aggregate([ { $match: { date: { $gte: ISODate("2022-01-01"), $lt: ISODate("2022-02-01") } } }, { $group: { _id: "$product", totalSales: { $sum: "$quantity" } } }, { $sort: { totalSales: -1 } }, { $limit: 5 } ])
2. Geospatial Queries: MongoDB supports geospatial queries, allowing for location-based searches and calculations.
MongoDB Code Snippet:
db.places.find({ location: { $near: { $geometry: { type: "Point", coordinates: [longitude, latitude] }, $maxDistance: 1000 } } })
Code Snippet Ideas for Redis
Here are a couple of code snippet ideas to get you started with Redis:
1. Rate Limiting: Use Redis to implement rate limiting functionality, allowing you to control the number of requests a user can make within a specific time period.
2. Caching HTML Fragments: Cache rendered HTML fragments in Redis to improve the performance of dynamic web pages.
Related Article: Tutorial on Redis Docker Compose
Code Snippet Ideas for MongoDB
Explore the following code snippet ideas to leverage MongoDB’s capabilities:
1. Data Aggregation: Use MongoDB’s aggregation pipeline to analyze and transform your data, calculating statistics or creating custom reports.
2. Full-Text Search: Implement full-text search functionality using MongoDB’s text indexes to enable useful search capabilities in your application.
Error Handling in Redis
When working with Redis, proper error handling is crucial. Here’s an example of handling errors in Redis using Python:
Redis Code Snippet:
import redis try: # Connect to Redis r = redis.Redis(host='localhost', port=6379, db=0) # Perform Redis operations r.set('mykey', 'myvalue') value = r.get('mykey') except redis.RedisError as e: print(f"Redis Error: {str(e)}")
Error Handling in MongoDB
MongoDB also requires careful error handling. Here’s an example of handling errors in MongoDB using Node.js:
MongoDB Code Snippet:
const { MongoClient } = require('mongodb'); async function run() { try { // Connect to MongoDB const client = new MongoClient(uri); await client.connect(); // Perform MongoDB operations const db = client.db('mydatabase'); const result = await db.collection('mycollection').findOne({ name: 'John' }); } catch (error) { console.error(`MongoDB Error: ${error}`); } finally { client.close(); } } run();
This article has covered various aspects of Redis and MongoDB, including their features, use cases, best practices, real-world examples, performance considerations, advanced techniques, and code snippet ideas. By understanding the strengths and appropriate use cases of each database, software engineers can make informed decisions when choosing between Redis and MongoDB for their projects.