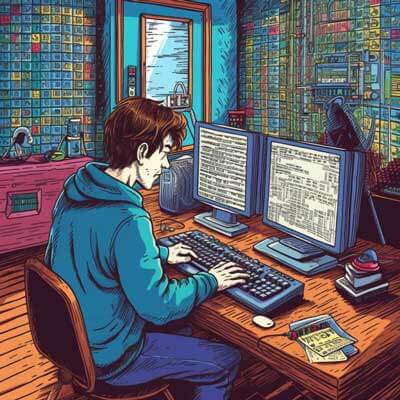
- What is asynchronous programming?
- Example 1: Asynchronous File I/O using Callbacks
- Example 2: Asynchronous HTTP Request using Promises
- How does non-blocking code work?
- Example 1: Non-blocking Code using Callbacks
- Example 2: Non-blocking Code using Promises
- What is event-driven programming?
- Example 1: Event-driven Programming in PHP
- Example 2: Event-driven Programming in JavaScript
- What is the difference between concurrency and parallelism?
- Example 1: Concurrency in PHP
- Example 2: Parallelism in PHP
- What is a callback function?
- Example 1: Callback Function using Anonymous Function
- Example 2: Callback Function using Named Function
- How do promises work in PHP?
- Example 1: Promises in PHP using Guzzle
- Example 2: Promises in PHP using ReactPHP
- What is the async/await syntax in PHP?
- Example 1: Async/Await Syntax in PHP
- Example 2: Async/Await Syntax in PHP using ReactPHP
- How does the event loop work?
- Example: Event Loop in ReactPHP
- What are some common examples of asynchronous tasks?
- Example 1: Asynchronous Network Request in PHP
- Example 2: Asynchronous File I/O in PHP
- How can parallel execution be achieved in PHP?
- Example 1: Parallel Execution using Multithreading in PHP
- Example 2: Parallel Execution using Asynchronous Programming in PHP
- External Sources
What is asynchronous programming?
Asynchronous programming is a programming paradigm that allows tasks to be executed independently of the main program flow. In synchronous programming, tasks are executed one after the other, blocking the execution of subsequent tasks until the current task is completed. This can lead to inefficiencies and delays, especially when tasks involve waiting for external resources, such as network requests or file I/O.
In contrast, asynchronous programming allows tasks to be executed concurrently, without blocking the execution of other tasks. This means that multiple tasks can be started and run in parallel, improving the overall performance and responsiveness of the program. Asynchronous programming is particularly useful in situations where tasks involve waiting for external resources, as it allows other tasks to continue executing while waiting.
In PHP, asynchronous programming can be achieved using various techniques, such as callbacks, promises, and the async/await syntax. These techniques provide mechanisms for handling asynchronous tasks and ensure that the program remains responsive and efficient.
Related Article: How to Work with Arrays in PHP (with Advanced Examples)
Example 1: Asynchronous File I/O using Callbacks
<?php $file = fopen('example.txt', 'r', function ($fileHandle) { // This callback function will be executed asynchronously when the file is opened if ($fileHandle) { // Read the contents of the file asynchronously fread($fileHandle, filesize('example.txt'), function ($data) { echo $data; }); } }); // Continue executing other tasks while waiting for the file to be opened echo "Executing other tasks...\n";
Example 2: Asynchronous HTTP Request using Promises
getAsync('https://api.example.com/data') ->then(function ($response) { // Handle the response asynchronously echo $response->getBody(); }); // Continue executing other tasks while waiting for the HTTP request to complete echo "Executing other tasks...\n";
How does non-blocking code work?
Non-blocking code is a fundamental concept in asynchronous programming. It allows tasks to be executed without blocking the execution of other tasks. In non-blocking code, tasks are designed in such a way that they can be executed asynchronously, without waiting for the completion of other tasks.
Non-blocking code typically involves the use of callbacks or promises. Callbacks are functions that are passed as arguments to other functions and are executed asynchronously when a specific event or task is completed. Promises, on the other hand, are objects that represent the eventual completion or failure of an asynchronous operation and allow for chaining of multiple asynchronous tasks.
When non-blocking code is executed, tasks are initiated and executed asynchronously, and the program flow continues without waiting for the completion of these tasks. This allows other tasks to be executed in parallel, improving the overall performance and responsiveness of the program.
Related Article: How To Make A Redirect In PHP
Example 1: Non-blocking Code using Callbacks
<?php function fetchDataAsync($url, $callback) { // Simulate an asynchronous HTTP request // This function will not block the execution of other tasks $data = file_get_contents($url); $callback($data); } fetchDataAsync('https://api.example.com/data', function ($data) { // Handle the response asynchronously echo $data; }); // Continue executing other tasks without waiting for the HTTP request to complete echo "Executing other tasks...\n";
Example 2: Non-blocking Code using Promises
getAsync('https://api.example.com/data') ->then(function ($response) { // Handle the response asynchronously echo $response->getBody(); }); // Continue executing other tasks without waiting for the HTTP request to complete echo "Executing other tasks...\n";
What is event-driven programming?
Event-driven programming is a programming paradigm that is based on the concept of events and event handlers. In event-driven programming, the flow of the program is determined by events that occur during its execution. These events can be user actions, system events, or messages from other programs.
The core idea behind event-driven programming is that the program responds to events by executing specific event handlers. Event handlers are functions or methods that are executed when a specific event occurs. They are typically registered or attached to the corresponding event, allowing the program to react and respond to events in a flexible and dynamic manner.
Event-driven programming is commonly used in graphical user interfaces (GUIs), web applications, and network programming, where events such as user clicks, mouse movements, or network messages need to be handled asynchronously.
Related Article: How To Add Elements To An Empty Array In PHP
Example 1: Event-driven Programming in PHP
clickHandler = $handler; } public function click() { // Simulate a button click event if ($this->clickHandler) { $this->clickHandler(); } } } $button = new Button(); $button->onClick(function () { // Handle the button click event asynchronously echo "Button clicked!\n"; }); // Simulate a button click $button->click(); // Continue executing other tasks while waiting for events to occur echo "Executing other tasks...\n";
Example 2: Event-driven Programming in JavaScript
// JavaScript example for comparison class Button { constructor() { this.clickHandler = null; } onClick(handler) { this.clickHandler = handler; } click() { // Simulate a button click event if (this.clickHandler) { this.clickHandler(); } } } const button = new Button(); button.onClick(() => { // Handle the button click event asynchronously console.log("Button clicked!"); }); // Simulate a button click button.click(); // Continue executing other tasks while waiting for events to occur console.log("Executing other tasks...");
What is the difference between concurrency and parallelism?
Concurrency and parallelism are related concepts in the context of executing multiple tasks simultaneously, but they have distinct meanings.
Concurrency refers to the ability of a program to execute multiple tasks at the same time, without necessarily running them in parallel. In concurrent programming, tasks are executed independently, but their execution may overlap or interleave. This can be achieved by interleaving the execution of tasks using techniques such as multitasking, multithreading, or event-driven programming.
Parallelism, on the other hand, refers to the execution of multiple tasks simultaneously, using multiple processors or processor cores. In parallel programming, tasks are executed in parallel, and their execution does not overlap or interleave. This can lead to significant performance improvements, especially when tasks can be executed independently and do not require synchronization or communication.
Related Article: How To Check If A PHP String Contains A Specific Word
Example 1: Concurrency in PHP
<?php function task1() { echo "Task 1 start\n"; sleep(1); echo "Task 1 end\n"; } function task2() { echo "Task 2 start\n"; sleep(2); echo "Task 2 end\n"; } // Execute tasks concurrently task1(); task2(); // Continue executing other tasks while waiting for the concurrent tasks to complete echo "Executing other tasks...\n";
Example 2: Parallelism in PHP
getAsync('https://api.example.com/data1'), $client->getAsync('https://api.example.com/data2'), ]; // Execute tasks in parallel $results = GuzzleHttp\Promise\all($promises)->wait(); // Handle the results asynchronously foreach ($results as $response) { echo $response->getBody(); } // Continue executing other tasks while waiting for the parallel tasks to complete echo "Executing other tasks...\n";
What is a callback function?
A callback function is a function that is passed as an argument to another function and is called asynchronously when a specific event or condition occurs. Callback functions are a fundamental concept in asynchronous programming and are commonly used to handle asynchronous tasks or events.
Callback functions allow for flexible and dynamic execution of code, as they can be defined and passed to functions at runtime. They are typically used to handle the results of asynchronous operations, such as file I/O, network requests, or user interactions.
In PHP, callback functions can be defined using various syntaxes, such as anonymous functions, named functions, or object methods. They are often used with functions that support asynchronous or event-driven programming patterns.
Related Article: How To Convert A Php Object To An Associative Array
Example 1: Callback Function using Anonymous Function
<?php function fetchDataAsync($url, $callback) { // Simulate an asynchronous HTTP request // This function will not block the execution of other tasks $data = file_get_contents($url); $callback($data); } fetchDataAsync('https://api.example.com/data', function ($data) { // Handle the response asynchronously echo $data; }); // Continue executing other tasks without waiting for the HTTP request to complete echo "Executing other tasks...\n";
Example 2: Callback Function using Named Function
<?php function fetchDataAsync($url, $callback) { // Simulate an asynchronous HTTP request // This function will not block the execution of other tasks $data = file_get_contents($url); $callback($data); } function handleResponse($data) { // Handle the response asynchronously echo $data; } fetchDataAsync('https://api.example.com/data', 'handleResponse'); // Continue executing other tasks without waiting for the HTTP request to complete echo "Executing other tasks...\n";
How do promises work in PHP?
Promises are objects that represent the eventual completion or failure of an asynchronous operation. They are a useful tool for managing and chaining asynchronous tasks in PHP.
A promise can be in one of three states:
– Pending: The initial state of a promise, before it is fulfilled or rejected.
– Fulfilled: The state of a promise when it is successfully resolved with a value.
– Rejected: The state of a promise when it encounters an error or fails to fulfill.
Promises provide methods for attaching callbacks to handle the fulfillment or rejection of the promise. These callbacks are executed asynchronously when the promise is fulfilled or rejected, allowing for the chaining of multiple asynchronous tasks.
In PHP, promises are commonly used with libraries and frameworks that support asynchronous programming, such as Guzzle, ReactPHP, or Amp. They provide a convenient and structured way of handling asynchronous tasks and managing their results.
Related Article: How To Get The Full URL In PHP
Example 1: Promises in PHP using Guzzle
getAsync($url)->then( function ($response) use ($promise) { // Handle the response asynchronously $promise->resolve($response->getBody()); }, function ($exception) use ($promise) { // Handle the exception asynchronously $promise->reject($exception); } ); return $promise; } fetchDataAsync('https://api.example.com/data')->then( function ($data) { // Handle the response asynchronously echo $data; }, function ($exception) { // Handle the exception asynchronously echo $exception->getMessage(); } ); // Continue executing other tasks while waiting for the promise to be fulfilled or rejected echo "Executing other tasks...\n";
Example 2: Promises in PHP using ReactPHP
addTimer(2, function () use ($deferred) { // Simulate an asynchronous task $deferred->resolve("Task completed"); }); $deferred->promise()->then(function ($result) { // Handle the result asynchronously echo $result; }); // Start the event loop $loop->run(); // Continue executing other tasks while waiting for the promise to be fulfilled echo "Executing other tasks...\n";
What is the async/await syntax in PHP?
The async/await syntax is a language feature that allows for more expressive and readable asynchronous code. It is a popular syntax in languages like JavaScript and C#, and it has been introduced in recent versions of PHP.
The async/await syntax allows developers to write asynchronous code that looks and behaves like synchronous code. It eliminates the need for callbacks or promises and simplifies the handling of asynchronous tasks.
With the async/await syntax, functions can be declared as asynchronous using the async
keyword. Within an asynchronous function, the await
keyword can be used to pause the execution of the function until a promise is fulfilled or rejected. This allows for sequential and linear execution of asynchronous code, making it easier to understand and maintain.
In PHP, the async/await syntax is implemented using generators and the yield
keyword. Asynchronous functions return generators that can be iterated over using yield
, and promises can be awaited using the yield
keyword.
Related Article: How To Fix the "Array to string conversion" Error in PHP
Example 1: Async/Await Syntax in PHP
getBody()->buffer(); return $data; } Loop::run(function () { $data = yield fetchDataAsync('https://api.example.com/data'); echo $data; }); // Continue executing other tasks while waiting for the asynchronous function to complete echo "Executing other tasks...\n";
Example 2: Async/Await Syntax in PHP using ReactPHP
addTimer(2, function () use ($deferred) { // Simulate an asynchronous task $deferred->resolve("Task completed"); }); yield $deferred->promise(); } $loop = Factory::create(); $loop->addCoroutine(function () { $result = yield fetchDataAsync('https://api.example.com/data'); echo $result; }); // Start the event loop $loop->run(); // Continue executing other tasks while waiting for the asynchronous function to complete echo "Executing other tasks...\n";
How does the event loop work?
The event loop is a crucial component of asynchronous programming and event-driven architectures. It is responsible for managing and dispatching events, as well as scheduling the execution of asynchronous tasks.
At a high level, the event loop works by continuously monitoring and processing events in a loop. It waits for events to occur and dispatches them to the appropriate event handlers. When an event handler is executed, it can trigger additional events or schedule the execution of other tasks.
The event loop operates in a single thread, which allows for efficient handling of events and tasks without the need for context switching or expensive thread synchronization. It ensures that tasks are executed in a non-blocking manner, allowing for high performance and scalability.
In PHP, the event loop is commonly used in frameworks and libraries that support asynchronous programming, such as ReactPHP or Amp. These frameworks provide abstractions and APIs for working with the event loop, making it easier to write event-driven code.
Related Article: How To Convert A String To A Number In PHP
Example: Event Loop in ReactPHP
addTimer(1, function () { echo "Timer event\n"; }); $loop->addPeriodicTimer(2, function () { echo "Periodic timer event\n"; }); $loop->addSignal(SIGINT, function () use ($loop) { echo "Signal event\n"; $loop->stop(); }); $loop->run(); // Continue executing other tasks while the event loop is running echo "Executing other tasks...\n";
What are some common examples of asynchronous tasks?
Asynchronous tasks can be found in various areas of software development, and they are particularly useful in situations where tasks involve waiting for external resources or long-running operations. Here are some common examples of asynchronous tasks:
1. Network requests: Performing HTTP requests to external APIs or services asynchronously, allowing other tasks to continue while waiting for the response.
2. File I/O: Reading or writing files asynchronously, improving the efficiency of file operations and allowing other tasks to execute in parallel.
3. Database queries: Executing database queries asynchronously, reducing latency and allowing multiple queries to be executed concurrently.
4. User interactions: Handling user interactions such as button clicks or mouse movements asynchronously, ensuring a responsive user interface.
5. Event processing: Reacting to system events, such as timer events, signal events, or keyboard events, asynchronously to avoid blocking the program’s execution.
6. Image processing: Performing image manipulation or processing tasks asynchronously, improving the performance of image-related operations.
7. Concurrent tasks: Running multiple tasks concurrently, such as parallel data processing, distributed computing, or parallel algorithm execution.
These examples highlight the versatility and benefits of asynchronous programming in various domains, including web development, system programming, and data processing.
Example 1: Asynchronous Network Request in PHP
getAsync('https://api.example.com/data') ->then(function ($response) { // Handle the response asynchronously echo $response->getBody(); }); // Continue executing other tasks while waiting for the HTTP request to complete echo "Executing other tasks...\n";
Related Article: How to Use Loops in PHP
Example 2: Asynchronous File I/O in PHP
<?php $file = fopen('example.txt', 'r', function ($fileHandle) { // This callback function will be executed asynchronously when the file is opened if ($fileHandle) { // Read the contents of the file asynchronously fread($fileHandle, filesize('example.txt'), function ($data) { echo $data; }); } }); // Continue executing other tasks while waiting for the file to be opened echo "Executing other tasks...\n";
How can parallel execution be achieved in PHP?
Parallel execution, which involves running multiple tasks simultaneously, can be achieved in PHP using various techniques and libraries. Here are some approaches to achieve parallel execution in PHP:
1. Multithreading: PHP supports multithreading with extensions like pthreads. Multithreading allows for true parallel execution of tasks by creating multiple threads of execution. However, multithreading in PHP can be challenging and requires careful synchronization and coordination between threads.
2. Process forking: PHP supports process forking, which allows for running multiple processes simultaneously. Each process can execute a separate task, enabling parallel execution. However, inter-process communication and synchronization can be complex and require additional mechanisms like shared memory or message passing.
3. Parallel extensions and libraries: There are extensions and libraries available for PHP that provide abstractions for parallel execution, such as the Parallel extension or the Amp library. These extensions and libraries simplify the process of running tasks in parallel and provide mechanisms for coordination and synchronization.
4. Asynchronous programming: Asynchronous programming allows for executing multiple tasks concurrently, without blocking the execution of other tasks. Asynchronous techniques, such as callbacks, promises, or the async/await syntax, can be used to achieve parallel execution in PHP.
It’s important to consider the specific requirements and constraints of your application when choosing an approach for parallel execution in PHP. Factors such as the nature of the tasks, resource utilization, and the complexity of coordination and synchronization should be taken into account.
Example 1: Parallel Execution using Multithreading in PHP
start(); $thread2->start(); $thread1->join(); $thread2->join(); // Continue executing other tasks while waiting for the threads to complete echo "Executing other tasks...\n";
Related Article: How to Work with PHP Arrays: a Complete Guide
Example 2: Parallel Execution using Asynchronous Programming in PHP
getAsync('https://api.example.com/data1') ->then(function ($response) { // Handle the response asynchronously echo $response->getBody(); }); $promise2 = $client->getAsync('https://api.example.com/data2') ->then(function ($response) { // Handle the response asynchronously echo $response->getBody(); }); // Continue executing other tasks while waiting for the HTTP requests to complete echo "Executing other tasks...\n";
External Sources
– PHP Manual: Asynchronous Programming
– ReactPHP
– Amp PHP
– Guzzle
– Promises/A+ specification
– Concurrency vs Parallelism – Golang Blog