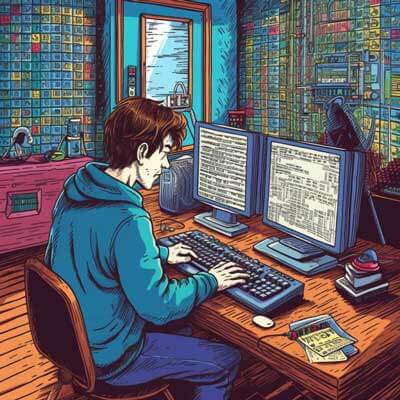
- The Importance of Processing MySQL Queries in PHP
- 1. Data Storage and Retrieval
- 2. Dynamic Content Generation
- Understanding the Syntax for Processing MySQL Queries in PHP
- 1. Using the mysqli Extension
- 2. Using the PDO Extension
- Retrieving the Result Set of a MySQL Query in PHP
- 1. Retrieving the Result Set using the mysqli Extension
- 2. Retrieving the Result Set using the PDO Extension
- Accessing the Returned Data from a MySQL Query in PHP
- 1. Accessing the Data using the mysqli Extension
- 2. Accessing the Data using the PDO Extension
- Displaying the Output of a MySQL Query in PHP
- 1. Displaying the Output using HTML Rendering
- 2. Displaying the Output with Formatting and Manipulation
- Handling MySQL Query Returns in PHP
- 1. Handling Errors
- 2. Handling Empty Result Sets
- Explaining the Process of Returning a MySQL Query Result in PHP
- Fetching a MySQL Query Result in PHP
- 1. Fetching a Single Row
- 2. Fetching Multiple Rows
- Recommended Ways to Obtain a MySQL Query Result in PHP
- 1. Using Prepared Statements
- 2. Using Parameterized Queries
- Step-by-Step Guide to Getting a MySQL Query Result in PHP
- Additional Resources
The Importance of Processing MySQL Queries in PHP
Processing MySQL queries in PHP is a fundamental aspect of web development, as it allows for seamless interaction between the PHP programming language and MySQL databases. MySQL is one of the most popular and widely used relational database management systems, and PHP is a versatile server-side scripting language. Combining these two technologies enables developers to create dynamic web applications that can store, retrieve, and manipulate data efficiently.
There are several reasons why processing MySQL queries in PHP is essential:
Related Article: How to Perform a Full Outer Join in MySQL
1. Data Storage and Retrieval
MySQL provides a reliable and efficient way to store and organize data. By processing MySQL queries in PHP, developers can easily insert, update, delete, and retrieve data from the database. This is particularly important for web applications that require persistent storage of user information, such as registration details, user profiles, and transaction records.
Here’s an example of how to connect to a MySQL database in PHP and execute a simple query to fetch data:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a query to fetch data $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Process the query result if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { echo "Name: " . $row["name"] . ", Age: " . $row["age"] . "<br>"; } } else { echo "No results found."; } // Close the database connection $conn->close(); ?>
This example demonstrates how to establish a connection to a MySQL database using PHP’s mysqli extension, execute a SELECT query to retrieve data from the “users” table, and display the results.
2. Dynamic Content Generation
Processing MySQL queries in PHP enables the dynamic generation of content for web applications. PHP can be embedded within HTML code, allowing developers to fetch data from a MySQL database and populate web pages with dynamic content. This is particularly useful for displaying product listings, blog posts, user comments, and other dynamic elements that require real-time data retrieval.
Here’s an example of how to retrieve data from a MySQL database and generate dynamic content using PHP:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a query to fetch data $sql = "SELECT * FROM products"; $result = $conn->query($sql); // Generate dynamic content if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { echo "<div class='product'>"; echo "<img src='" . $row["image_url"] . "' alt='" . $row["name"] . "'>"; echo "<h3>" . $row["name"] . "</h3>"; echo "<p>" . $row["description"] . "</p>"; echo "<p>Price: $" . $row["price"] . "</p>"; echo "</div>"; } } else { echo "No products available."; } // Close the database connection $conn->close(); ?>
In this example, we retrieve data from the “products” table and generate dynamic content for each product using PHP. The generated HTML code is embedded within PHP echo statements, allowing the data to be dynamically inserted into the web page.
Understanding the Syntax for Processing MySQL Queries in PHP
To process MySQL queries in PHP, you need to understand the syntax for executing SQL statements and retrieving the result set. PHP provides several extensions to interact with MySQL databases, including the mysqli and PDO extensions.
Related Article: How to Fix MySQL Error Code 1175 in Safe Update Mode
1. Using the mysqli Extension
The mysqli extension is a useful and efficient option for processing MySQL queries in PHP. It offers both procedural and object-oriented interfaces for working with databases. Here’s an example of the syntax for executing a SELECT query using the mysqli extension:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a SELECT query $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Process the result set if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { // Process each row of data } } else { // Handle case when no results are found } // Close the database connection $conn->close(); ?>
In this example, we establish a connection to a MySQL database using the mysqli extension’s object-oriented interface. We then execute a SELECT query using the query() method, which returns a result set. The result set can be iterated using a while loop and the fetch_assoc() method to retrieve each row of data.
2. Using the PDO Extension
The PDO (PHP Data Objects) extension is another popular option for processing MySQL queries in PHP. It provides a consistent interface for working with different databases, including MySQL. Here’s an example of the syntax for executing a SELECT query using the PDO extension:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Execute a SELECT query $sql = "SELECT * FROM users"; $stmt = $conn->query($sql); // Process the result set while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { // Process each row of data } } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); } // Close the database connection $conn = null; ?>
In this example, we establish a connection to a MySQL database using the PDO extension and the PDO class. We then execute a SELECT query using the query() method, which returns a PDOStatement object representing the result set. The result set can be iterated using a while loop and the fetch() method with the PDO::FETCH_ASSOC fetch style to retrieve each row of data.
Retrieving the Result Set of a MySQL Query in PHP
When executing a MySQL query in PHP, the result set can be retrieved using the appropriate methods provided by the mysqli or PDO extension. The result set contains the rows of data returned by the query, and it can be processed to extract the desired information.
Related Article: How to Update Records in MySQL with a Select Query
1. Retrieving the Result Set using the mysqli Extension
To retrieve the result set of a MySQL query using the mysqli extension, you can use the query() method to execute the query and obtain a mysqli_result object. The mysqli_result object provides various methods to access the rows of data.
Here’s an example of how to retrieve the result set of a MySQL query using the mysqli extension:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a SELECT query $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Retrieve the result set if ($result->num_rows > 0) { // Process the rows of data while ($row = $result->fetch_assoc()) { // Process each row of data } } // Close the database connection $conn->close(); ?>
In this example, we execute a SELECT query using the query() method, which returns a mysqli_result object representing the result set. We then use the num_rows property to check if there are any rows in the result set, and if so, we iterate over the rows using the fetch_assoc() method to retrieve each row of data.
2. Retrieving the Result Set using the PDO Extension
To retrieve the result set of a MySQL query using the PDO extension, you can use the query() method to execute the query and obtain a PDOStatement object. The PDOStatement object provides various methods to access the rows of data.
Here’s an example of how to retrieve the result set of a MySQL query using the PDO extension:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Execute a SELECT query $sql = "SELECT * FROM users"; $stmt = $conn->query($sql); // Retrieve the result set while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { // Process each row of data } } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); } // Close the database connection $conn = null; ?>
In this example, we execute a SELECT query using the query() method, which returns a PDOStatement object representing the result set. We then use a while loop and the fetch() method with the PDO::FETCH_ASSOC fetch style to retrieve each row of data from the result set.
Accessing the Returned Data from a MySQL Query in PHP
When processing MySQL queries in PHP, accessing the returned data from the result set allows you to retrieve and manipulate the specific values or fields of interest. PHP provides methods and functions to access the data in a result set, making it easy to work with the retrieved information.
Related Article: How to Use MySQL Query String Contains
1. Accessing the Data using the mysqli Extension
To access the returned data from a MySQL query using the mysqli extension, you can use the various methods available in the mysqli_result object. These methods allow you to retrieve specific values or fields from the rows of data.
Here’s an example of how to access the returned data from a MySQL query using the mysqli extension:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a SELECT query $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Access the returned data if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $name = $row["name"]; $age = $row["age"]; // Process the retrieved data } } // Close the database connection $conn->close(); ?>
In this example, we fetch each row of data from the result set using the fetch_assoc() method, which returns an associative array representing the row. We then access the specific values or fields of interest, such as “name” and “age”, by using the corresponding keys in the associative array.
2. Accessing the Data using the PDO Extension
To access the returned data from a MySQL query using the PDO extension, you can use the various methods available in the PDOStatement object. These methods allow you to retrieve specific values or fields from the rows of data.
Here’s an example of how to access the returned data from a MySQL query using the PDO extension:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Execute a SELECT query $sql = "SELECT * FROM users"; $stmt = $conn->query($sql); // Access the returned data while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { $name = $row["name"]; $age = $row["age"]; // Process the retrieved data } } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); } // Close the database connection $conn = null; ?>
In this example, we fetch each row of data from the result set using the fetch() method with the PDO::FETCH_ASSOC fetch style, which returns an associative array representing the row. We then access the specific values or fields of interest, such as “name” and “age”, by using the corresponding keys in the associative array.
Displaying the Output of a MySQL Query in PHP
Displaying the output of a MySQL query in PHP allows you to present the retrieved data to the user in a meaningful and organized manner. PHP provides various techniques for displaying the output, including HTML rendering, formatting, and data manipulation.
Related Article: How to Resolve Secure File Priv in MySQL
1. Displaying the Output using HTML Rendering
One common approach to displaying the output of a MySQL query in PHP is to utilize HTML rendering. By combining PHP code with HTML markup, you can create dynamic web pages that present the retrieved data in a visually appealing format.
Here’s an example of how to display the output of a MySQL query using HTML rendering in PHP:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a SELECT query $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Display the output using HTML rendering if ($result->num_rows > 0) { echo "<table>"; echo "<tr><th>Name</th><th>Age</th></tr>"; while ($row = $result->fetch_assoc()) { echo "<tr>"; echo "<td>" . $row["name"] . "</td>"; echo "<td>" . $row["age"] . "</td>"; echo "</tr>"; } echo "</table>"; } else { echo "No results found."; } // Close the database connection $conn->close(); ?>
In this example, we use HTML tags to structure the output, create a table, and define table headers. Within the while loop, each row of data is encapsulated within HTML table row tags, and the specific values are inserted using PHP echo statements.
2. Displaying the Output with Formatting and Manipulation
In addition to HTML rendering, you can display the output of a MySQL query in PHP with formatting and manipulation. This allows you to modify the retrieved data or apply specific rules to present it in a customized manner.
Here’s an example of how to display the output of a MySQL query with formatting and manipulation in PHP:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a SELECT query $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Display the output with formatting and manipulation if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { $name = strtoupper($row["name"]); $age = $row["age"] >= 18 ? "Adult" : "Minor"; echo "Name: $name, Age: $age<br>"; } } else { echo "No results found."; } // Close the database connection $conn->close(); ?>
In this example, we apply formatting and manipulation to the retrieved data within the while loop. The strtoupper() function is used to convert the name to uppercase, and a conditional operator is used to determine if the age qualifies as an adult or minor. The modified values are then concatenated with additional text using PHP echo statements.
Handling MySQL Query Returns in PHP
When processing MySQL queries in PHP, it is important to handle the different scenarios that can arise from the query returns. This includes handling errors, empty result sets, and other exceptional situations to ensure the smooth execution of the application.
Related Article: Securing MySQL Access through Bash Scripts in Linux
1. Handling Errors
Handling errors is crucial when processing MySQL queries in PHP. It allows you to identify and resolve any issues that may occur during the execution of the queries. PHP provides mechanisms to handle errors, such as exception handling and error reporting.
Here’s an example of how to handle errors when processing MySQL queries in PHP using exception handling:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Execute a SELECT query $sql = "SELECT * FROM users"; $stmt = $conn->query($sql); // Handle errors if ($stmt === false) { throw new Exception("Error executing query."); } // Process the result set while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { // Process each row of data } } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); } catch (Exception $e) { echo "Error: " . $e->getMessage(); } // Close the database connection $conn = null; ?>
In this example, we use try-catch blocks to handle exceptions that may occur during the execution of the MySQL query. If an exception is thrown, the catch block catches the exception and displays an appropriate error message. This allows for better error handling and enables developers to take appropriate action when errors occur.
2. Handling Empty Result Sets
Handling empty result sets is another important aspect of processing MySQL queries in PHP. It allows you to handle scenarios where no data is returned by a query, ensuring that your application behaves correctly and provides appropriate feedback to the user.
Here’s an example of how to handle empty result sets when processing MySQL queries in PHP:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a SELECT query $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Handle empty result sets if ($result->num_rows > 0) { while ($row = $result->fetch_assoc()) { // Process each row of data } } else { echo "No results found."; } // Close the database connection $conn->close(); ?>
In this example, we check if the result set has any rows using the num_rows property. If the result set is not empty, we iterate over the rows and process each row of data. If the result set is empty, we display a message indicating that no results were found.
Explaining the Process of Returning a MySQL Query Result in PHP
The process of returning a MySQL query result in PHP involves several steps, including establishing a database connection, executing the query, retrieving the result set, and processing the returned data. Understanding this process is essential for effectively working with MySQL queries in PHP.
Here’s an overview of the process of returning a MySQL query result in PHP:
1. Establish a Database Connection: Before executing a MySQL query, a connection to the database needs to be established. This involves providing the necessary connection details, such as the hostname, username, password, and database name. PHP provides extensions like mysqli and PDO to establish and manage database connections.
2. Execute the Query: Once the database connection is established, the query can be executed using the appropriate method or function provided by the selected database extension. The query can be a SELECT, INSERT, UPDATE, DELETE, or any other valid SQL statement.
3. Retrieve the Result Set: After executing the query, the result set is returned, containing the rows of data that match the query criteria. The result set can be accessed using the methods or functions provided by the database extension, such as fetch(), fetch_assoc(), or fetchAll().
4. Process the Returned Data: The returned data can be processed by iterating over the result set and accessing the specific values or fields of interest. This allows for further manipulation or presentation of the data, such as displaying it in a web page or performing calculations.
5. Close the Database Connection: Once the data has been processed, it is important to close the database connection to free up system resources and ensure the security of the database. PHP provides methods or functions to close the database connection, such as close() for mysqli or null for PDO.
Related Article: Creating a Bash Script for a MySQL Database Backup
Fetching a MySQL Query Result in PHP
Fetching a MySQL query result in PHP involves retrieving the rows of data from the result set returned by the executed query. PHP provides methods and functions to fetch the data in various formats, allowing for easy manipulation and processing.
1. Fetching a Single Row
To fetch a single row from a MySQL query result in PHP, you can use methods or functions provided by the selected database extension, such as mysqli or PDO. These methods or functions allow you to retrieve the data from the result set and assign it to variables or process it directly.
Here’s an example of how to fetch a single row from a MySQL query result in PHP using the mysqli extension:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Execute a SELECT query $sql = "SELECT * FROM users"; $result = $conn->query($sql); // Fetch a single row if ($result->num_rows > 0) { $row = $result->fetch_assoc(); // Process the fetched row } // Close the database connection $conn->close(); ?>
In this example, we use the fetch_assoc() method to fetch a single row from the result set. The fetched row is assigned to the $row variable, allowing for further processing or manipulation.
2. Fetching Multiple Rows
To fetch multiple rows from a MySQL query result in PHP, you can use loops or iteration constructs to retrieve each row of data. PHP provides methods or functions to fetch the data in various formats, such as associative arrays or objects, allowing for flexible processing.
Here’s an example of how to fetch multiple rows from a MySQL query result in PHP using the PDO extension:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Execute a SELECT query $sql = "SELECT * FROM users"; $stmt = $conn->query($sql); // Fetch multiple rows while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { // Process each fetched row } } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); } // Close the database connection $conn = null; ?>
In this example, we use a while loop to fetch multiple rows from the result set. The fetch() method with the PDO::FETCH_ASSOC fetch style is used to retrieve each row as an associative array. The fetched row can then be processed or manipulated within the loop.
Related Article: Converting MySQL Query Results from True to Yes
Recommended Ways to Obtain a MySQL Query Result in PHP
When obtaining a MySQL query result in PHP, there are several recommended approaches that ensure efficient and secure execution of the queries. These approaches involve using prepared statements, parameterized queries, and secure database connection methods.
1. Using Prepared Statements
Prepared statements are a recommended way to obtain a MySQL query result in PHP. They provide a secure and efficient method of executing SQL statements by separating the query logic from the user-supplied data. Prepared statements use placeholders for dynamic data, which are later bound to specific values, preventing SQL injection attacks.
Here’s an example of how to obtain a MySQL query result using prepared statements in PHP:
setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); // Prepare and execute a SELECT query with parameters $stmt = $conn->prepare("SELECT * FROM users WHERE age > :age"); $stmt->bindParam(":age", $age); $age = 18; $stmt->execute(); // Fetch the result set while ($row = $stmt->fetch(PDO::FETCH_ASSOC)) { // Process each fetched row } } catch (PDOException $e) { echo "Connection failed: " . $e->getMessage(); } // Close the database connection $conn = null; ?>
In this example, we prepare a SELECT query with a parameter placeholder “:age” and bind it to the $age variable using the bindParam() method. This allows us to execute the query multiple times with different parameter values. The result set can then be fetched and processed within the while loop.
2. Using Parameterized Queries
Parameterized queries are another recommended way to obtain a MySQL query result in PHP. They are similar to prepared statements and provide a secure method of executing SQL statements. Parameterized queries use placeholders for dynamic data, which are later replaced with the actual values, preventing SQL injection attacks.
Here’s an example of how to obtain a MySQL query result using parameterized queries in PHP:
connect_error) { die("Connection failed: " . $conn->connect_error); } // Prepare and execute a SELECT query with parameters $sql = "SELECT * FROM users WHERE age > ?"; $stmt = $conn->prepare($sql); $stmt->bind_param("i", $age); $age = 18; $stmt->execute(); // Fetch the result set $result = $stmt->get_result(); while ($row = $result->fetch_assoc()) { // Process each fetched row } // Close the database connection $conn->close(); ?>
In this example, we prepare a SELECT query with a parameter placeholder “?” and bind it to the $age variable using the bind_param() method. This allows us to execute the query multiple times with different parameter values. The result set can then be fetched and processed within the while loop.
Related Article: How To Import a SQL File With a MySQL Command Line
Step-by-Step Guide to Getting a MySQL Query Result in PHP
Getting a MySQL query result in PHP involves several steps, from establishing a database connection to processing the retrieved data. Here’s a step-by-step guide to help you navigate the process effectively:
Step 1: Establish a Database Connection
– Use the appropriate database extension, such as mysqli or PDO, to establish a connection to the MySQL database. Provide the necessary connection details, including the hostname, username, password, and database name. Handle any connection errors that may occur.
Step 2: Execute the MySQL Query
– Use the appropriate method or function provided by the selected database extension to execute the MySQL query. This can be a SELECT, INSERT, UPDATE, DELETE, or any other valid SQL statement. Handle any errors that may occur during the query execution.
Step 3: Retrieve the Result Set
– Use the appropriate method or function provided by the database extension to retrieve the result set returned by the query. The result set contains the rows of data that match the query criteria.
Step 4: Process the Result Set
– Use loops or iteration constructs to process the rows of data in the result set. Access the specific values or fields of interest and perform any necessary manipulation or calculations. Handle empty result sets or other exceptional scenarios accordingly.
Step 5: Close the Database Connection
– Once the data has been processed, close the database connection to free up system resources and ensure the security of the database. Use the appropriate method or function provided by the database extension to close the connection.
Additional Resources
– How to retrieve data from a MySQL query in PHP?
– How to loop through a MySQL query result in PHP?