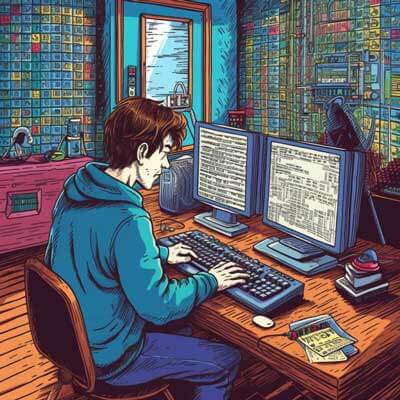
To get the full URL in PHP, you can use a combination of server variables and PHP functions. In this answer, we will explore two possible approaches to achieve this.
Approach 1: Using Server Variables
One way to get the full URL in PHP is by using server variables such as $_SERVER['HTTP_HOST']
, $_SERVER['REQUEST_URI']
, and $_SERVER['HTTPS']
. These variables provide information about the server, the request, and the protocol being used.
Here’s an example code snippet that demonstrates how to use these server variables to get the full URL:
$protocol = isset($_SERVER['HTTPS']) && $_SERVER['HTTPS'] === 'on' ? 'https' : 'http'; $host = $_SERVER['HTTP_HOST']; $uri = $_SERVER['REQUEST_URI']; $fullUrl = $protocol . '://' . $host . $uri; echo $fullUrl;
Explanation:
– The $_SERVER['HTTPS']
variable is used to check if the request is using a secure HTTPS protocol. If it is set to 'on'
, then the protocol is HTTPS; otherwise, it is HTTP.
– The $_SERVER['HTTP_HOST']
variable contains the hostname of the server.
– The $_SERVER['REQUEST_URI']
variable contains the path and query string of the current request.
– The $fullUrl
variable concatenates the protocol, host, and URI to form the full URL.
Using this approach, you can reliably retrieve the full URL of the current request in PHP.
Related Article: How To Fix the "Array to string conversion" Error in PHP
Approach 2: Using PHP’s built-in http_build_url
function
Another approach is to use PHP’s built-in http_build_url
function. This function constructs a URL by merging an associative array representation of a URL with a base URL.
Here’s an example code snippet that demonstrates how to use http_build_url
to get the full URL:
$urlComponents = parse_url($_SERVER['REQUEST_URI']); $fullUrl = http_build_url($_SERVER['HTTP_HOST'], $urlComponents); echo $fullUrl;
Explanation:
– The parse_url
function is used to parse the URL into its components (e.g., scheme, host, path, query string).
– The http_build_url
function takes the hostname ($_SERVER['HTTP_HOST']
) and the parsed URL components as arguments and constructs the full URL.
This approach can be useful if you need to manipulate or modify specific components of the URL before constructing the full URL.
Reasons for Asking
The question “How to get the full URL in PHP” may arise for various reasons, including:
– Building dynamic links or redirects based on the current URL.
– Generating canonical URLs for SEO purposes.
– Logging or tracking the full URL for analytics or debugging purposes.
By understanding how to retrieve the full URL in PHP, developers can address these requirements more effectively.
Alternative Ideas and Best Practices
1. Using a Framework: If you are working with a PHP framework such as Laravel or Symfony, these frameworks often provide utility functions or helpers to retrieve the full URL. Consult the documentation of your chosen framework for the recommended approach.
2. Encapsulating the Logic: To avoid repeating the code to get the full URL in multiple places, consider encapsulating the logic in a reusable function or class method. This can make your code more maintainable and easier to modify in the future.
3. Handling Edge Cases: Keep in mind that the approaches mentioned above may not cover all edge cases. For example, if the URL contains special characters or non-standard components, additional handling may be required. Consider testing your code with different URL scenarios to ensure its robustness.
Related Article: How To Convert A Php Object To An Associative Array