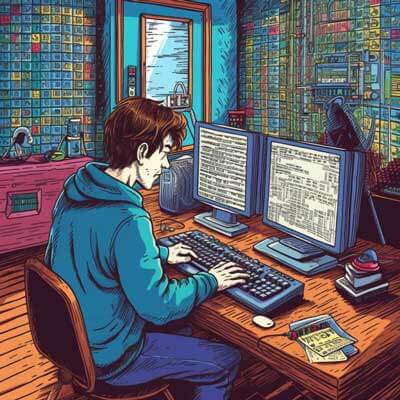
When working with Laravel, you may encounter the “Error 419: Page Expired” issue when making a POST request. This error usually occurs when the CSRF token validation fails. Laravel uses CSRF (Cross-Site Request Forgery) tokens to protect against cross-site scripting attacks. This error can be frustrating, but fortunately, there are several ways to fix it.
1. Verify the CSRF Token
The first step to fix the “Error 419: Page Expired” issue is to ensure that your forms include the CSRF token field. This token is typically included in the form as a hidden input field. You can generate the CSRF token using the @csrf
Blade directive. Here’s an example:
@csrf <!-- Your form fields here -->
Make sure that your forms include this CSRF token field. Without it, Laravel will not be able to validate the request and will throw the “Error 419: Page Expired” error.
Related Article: How to Delete An Element From An Array In PHP
2. Check the Session Configuration
If the CSRF token is properly included in your forms and you are still experiencing the “Error 419: Page Expired” issue, you may need to check your session configuration. Laravel uses session cookies to store the CSRF token. If the session configuration is incorrect, the token may not be stored or retrieved correctly, leading to the error.
Open the config/session.php
file and verify the following settings:
'same_site' => 'lax', 'http_only' => true,
Ensure that the same_site
option is set to 'lax'
and the http_only
option is set to true
. These settings are recommended to ensure proper CSRF token handling and prevent the “Error 419: Page Expired” issue.
3. Clear Browser Cache and Cookies
Sometimes, the “Error 419: Page Expired” issue can be caused by outdated or corrupted browser cache and cookies. Clearing your browser cache and cookies can help resolve this problem. The steps to clear cache and cookies vary depending on the browser you are using. Here are some general instructions:
– For Google Chrome: Go to the browser settings, navigate to “Privacy and security,” and click on “Clear browsing data.” Make sure to select the “Cached images and files” and “Cookies and other site data” options, and then click “Clear data.”
– For Mozilla Firefox: Go to the browser settings, navigate to “Privacy & Security,” and scroll down to the “Cookies and Site Data” section. Click on “Clear Data” and make sure the “Cookies and Site Data” and “Cached Web Content” options are selected. Finally, click “Clear.”
– For Microsoft Edge: Go to the browser settings, navigate to “Privacy, search, and services,” and click on “Choose what to clear.” Select the “Cookies and other site data” and “Cached images and files” options, and then click “Clear.”
After clearing the cache and cookies, try accessing your application again and see if the “Error 419: Page Expired” issue persists.
4. Check Server Time and Timezone
Incorrect server time and timezone settings can also cause the “Error 419: Page Expired” issue in Laravel. Ensure that your server’s time and timezone are correctly configured. You can check and update the timezone in the config/app.php
file by setting the timezone
option to your desired timezone. For example:
'timezone' => 'UTC',
Make sure the server time and timezone are synchronized to prevent any time-related issues that could affect the CSRF token validation.
Related Article: Processing MySQL Queries in PHP: A Detailed Guide
5. Verify Session Configuration in Load Balancer
If you are running your Laravel application behind a load balancer or proxy server, ensure that the load balancer is correctly configured to handle session persistence. The load balancer should be configured to forward the necessary headers for session management, such as X-CSRF-TOKEN
and X-XSRF-TOKEN
. Consult your load balancer or proxy server documentation for specific instructions on configuring session persistence.
6. Disable CSRF Protection (Not Recommended)
If none of the above solutions work for your specific situation, you can consider disabling CSRF protection as a last resort. However, this is not recommended as it compromises the security of your application. To disable CSRF protection, comment out the VerifyCsrfToken
middleware in the $middleware
array of the app/Http/Kernel.php
file:
protected $middleware = [ // \App\Http\Middleware\VerifyCsrfToken::class, // Other middleware... ];