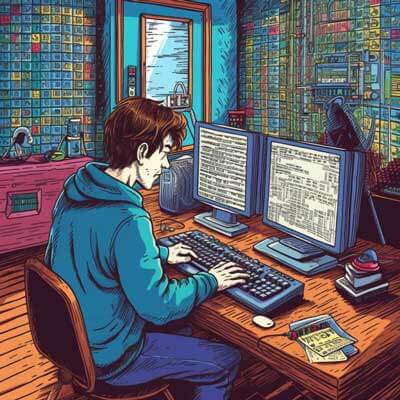
To add elements to an empty array in PHP, you can use several methods. In this answer, we will explore two possible approaches.
Method 1: Using the array_push function
One way to add elements to an empty array is by using the array_push function. This function allows you to add one or more elements to the end of an array. Here is an example:
element1 [1] => element2 [2] => element3 ) ?>
In the above example, we create an empty array $myArray
using the array()
syntax. Then, we use the array_push
function to add three elements to the array. Finally, we use the print_r
function to display the contents of the array.
Related Article: How to Work with Arrays in PHP (with Advanced Examples)
Method 2: Using the square bracket notation
Another way to add elements to an empty array is by using the square bracket notation. This notation allows you to directly assign values to specific array keys. Here is an example:
element1 [1] => element2 [2] => element3 ) ?>
In the above example, we create an empty array $myArray
using the []
syntax. Then, we assign values to specific keys of the array using the square bracket notation. Finally, we use the print_r
function to display the contents of the array.
Why is this question asked?
The question “How to add elements to an empty array in PHP?” is commonly asked by PHP developers who are new to the language or want to refresh their knowledge. It is an essential concept in PHP as arrays are a fundamental data structure used to store and manipulate collections of data. Understanding how to add elements to an array is crucial for building dynamic applications that require data manipulation and storage.
Potential reasons for asking this question
There are several reasons why someone might ask this question:
1. Working with user input: When developing web applications, it is common to receive user input and store it in an array for further processing. Knowing how to add elements to an empty array allows developers to handle user data effectively.
2. Data manipulation: Arrays are a powerful tool in PHP for storing and manipulating data. Being able to add elements to an empty array is essential for performing various operations, such as sorting, filtering, and aggregating data.
3. Dynamic data collection: In many cases, the size and content of an array are not known in advance. Adding elements to an empty array dynamically allows developers to collect and store data as needed during the execution of a program.
Related Article: How To Make A Redirect In PHP
Suggestions and alternative ideas
While the methods described above are effective for adding elements to an empty array in PHP, there are alternative approaches worth considering:
1. Using the array_merge function: If you have an existing array and want to merge it with another array, the array_merge
function can be used. This function takes two or more arrays as arguments and returns a new array containing all the elements from the input arrays. Here is an example:
existing_element1 [1] => existing_element2 [2] => new_element1 [3] => new_element2 ) ?>
2. Using the shorthand square bracket notation: PHP 5.4 introduced a shorthand syntax for creating arrays using square brackets. Instead of using the array()
syntax, you can directly assign values to an array using the shorthand notation. Here is an example:
element1 [1] => element2 [2] => element3 ) ?>
This shorthand syntax can be especially useful when adding elements to an array in a loop or when the keys of the array are not important.
Best practices
When adding elements to an empty array in PHP, it is good practice to follow these guidelines:
1. Initialize the array: Before adding elements to an array, it is a good practice to initialize it as an empty array using either the array()
syntax or the shorthand []
syntax. This ensures that the array is empty and ready to accept new elements.
2. Choose appropriate keys: When using the square bracket notation to add elements to an array, consider using meaningful keys that reflect the purpose and context of the data being stored. This can make the code more readable and maintainable.
3. Avoid overwriting existing keys: When adding elements to an array using the square bracket notation, be cautious not to overwrite existing keys unintentionally. If you need to preserve existing keys, consider using the array_merge
function instead.
4. Sanitize user input: When adding user input to an array, it is essential to sanitize and validate the data to prevent security vulnerabilities such as SQL injection or cross-site scripting (XSS) attacks. Use appropriate sanitization and validation techniques depending on the specific use case.